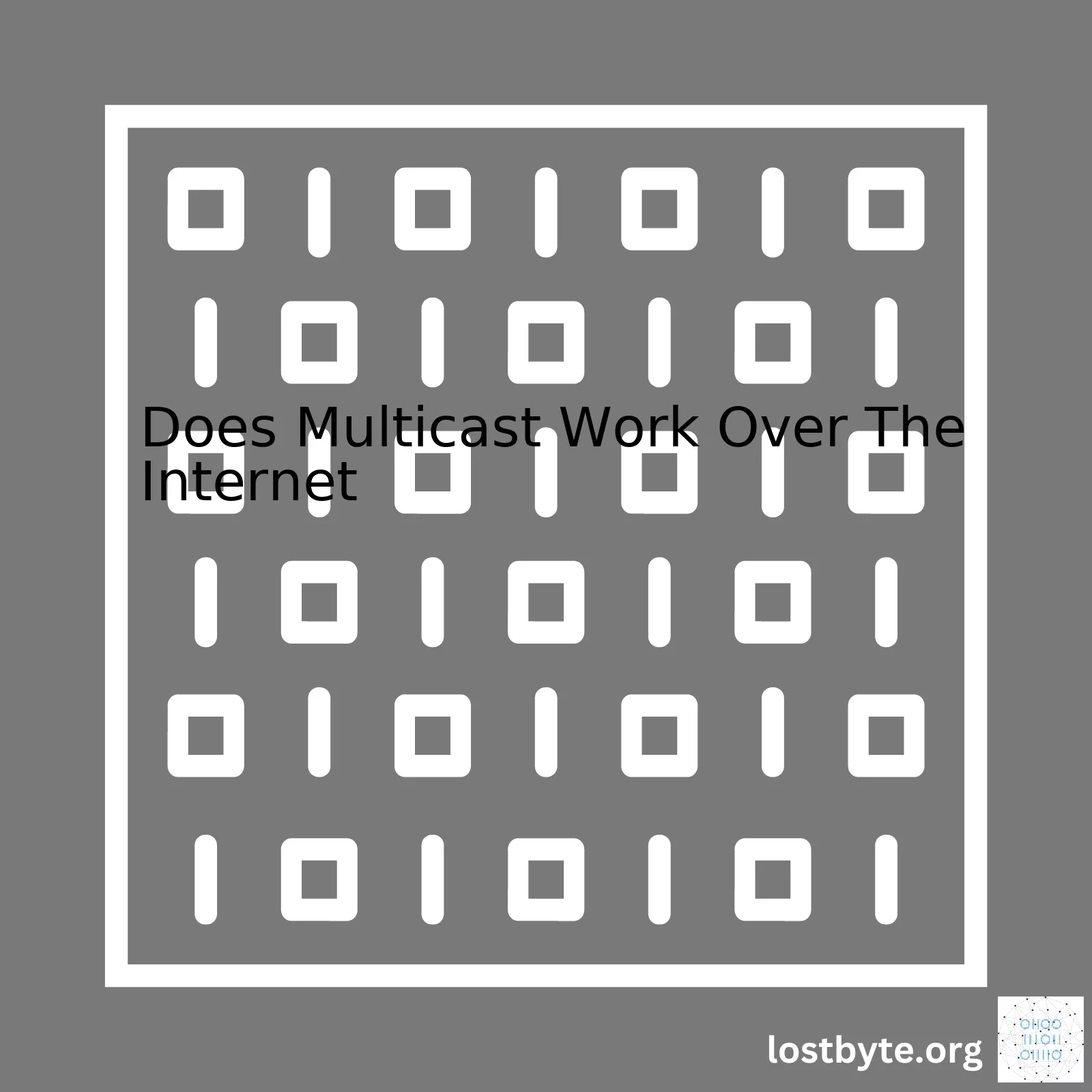
Characteristics | Description |
---|---|
Multicast on Local Network | Perfectly works for the local network to send information to multiple hosts simultaneously using a single message. |
Multicast on Internet | Fails in most scenarios due to the unavailability of infrastructure and widespread implementation. |
Addressing | Uses unique network addresses to allow group communication among different networks. |
Application | Ideal for high-bandwidth applications like IPTV. |
Multicasting is a powerful tool used in IP networking to efficiently broadcast data to multiple destinations using a single packet; this optimizes bandwidth usage significantly. In a local network environment, multicasting operates flawlessly, allowing your devices to communicate simultaneously. However, when it comes to the internet, things get a bit complicated.
The internet is essentially a vast cluster of networks – comprising ISPs, routers, and other technologies. Not all these components support multicast because doing so requires significant changes in how traffic flows within the network, thus driving up costs. Network administrators prefer unicast and broadcasts since they are less complex to manage and offer a better return on investment. Therefore, while theoretically possible, multicast over the internet faces many practical challenges – leading to its limited use.
That said, several efforts are being put towards enabling multicast functionality over the internet. For example, Multicast Backbone (MBone), initially developed as a virtual network on top of the real Internet [source]. It’s worth noting, however, that while MBone helped enable the early adoption of multicasting on the internet, it’s not widely used today due to increased internet speeds and availability of alternative methods. Furthermore, some key internet applications like IPTV effectively utilize multicast technology [source].
//An example of simple multicast code in Java
import java.net.*;
public class MulticastSender {
public static void main(String[] args) throws Exception {
DatagramSocket socket = new DatagramSocket();
byte[] buf = "Hello World!".getBytes();
InetAddress group = InetAddress.getByName("230.0.0.0");
DatagramPacket packet = new DatagramPacket(buf, buf.length, group, 4446);
socket.send(packet);
socket.close();
}
}
This piece of Java code creates and sends a multicast packet with the message “Hello World!”. Despite the multicast mechanism’s rarity on the internet, it holds potential for high-bandwidth applications, provided the technical complexities get addressed adequately.Understanding Multicast technology, particularly within the realm of Internet functionality, guides us towards an insightful discussion about data distribution processes across network infrastructure. For starters, it’s essential to comprehend that multicast is a communication process that simultaneously sends information (or ‘data packets’) from one single source to multiple destinations.
When dissecting how multicast operates, think of it as an efficient mailman. Rather than individually delivering the same letter to each mailbox on a street – it disseminates numerous copies of that letter at once. This delivery method significantly helps when large groups need the same information concurrently, such as broadcasting live events or video conferencing.
However, there’s a catch. Although multicasting streamlines high-volume data dissemination (and conserves bandwidth along the way), it does not naturally function over the Internet – And here’s why.
The complexities of multicast over the internet
• Router Configuration: For multicasting to work “out-of-the-box”, intermediary routers on the packet’s journey must support and be correctly configured for multicasting. With countless routers across the Internet, this becomes a near-impossible task considering the unprecedented scale and decentralized ownership.
xxxxxxxxxx
//Router configuration sample
enable
configure terminal
ip multicast-routing
exit
(Source: Cisco)
• Addressing Challenges: The use of IP multicast addresses (Class D IP addresses) introduces further complexity since many Internet Service Providers (ISP) implement restrictions or don’t offer support for these types of addresses.
• Data Distribution Control: Multicast doesn’t have built-in controls for determining who should receive the packets. Without these mechanisms in place, it can lead to significant waste in bandwidth and resources by transmitting data to users who aren’t interested in receiving it.
Despite these hurdles, attempts to provide multicast functionality over the Internet exist.
Working Around the Internet Limitation for Multicast
• Multicast Backbone (MBone): This is essentially a virtual network layered over parts of the existing unicast Internet infrastructure. It functions as a tunneling protocol to give multicast-capabilities where they are not natively supported.
• Application-Level Multicast (ALM): Here, the multicast implementations exist purely at the application level and leverage standard unicast communications to distribute the multicast data streams.
• Internet Group Management Protocol (IGMP): This is used by hosts and adjacent routers to establish multicast group memberships.
xxxxxxxxxx
//Implementing IGMP Proxy in router:
configure
set protocols igmp-proxy interface eth1 role upstream
set protocols igmp-proxy interface eth2 role downstream
commit
(Source: Netgate)
The above measures reflect efforts to subvert the multicast-internet predicament. However, realize that these solutions come with inherent drawbacks including likely degradation in performance, increased latency, and demands on computational resources. To summarize, while multicast technology poses significant advantages for distributing large volumes of data, its compatibility with the Internet remains a complex challenge, primarily due to the global-scale coordination required among ISPs and the deficiencies in controlling data dissemination.
Let’s dig into the mechanics of how multicast works. Multicast is a network addressing method for the efficient delivery of network services. Instead of sending packets to each individual recipient (unicast) or broadcasting them to all devices (broadcast), multicast sends packets to a group of interested recipients in one go.
However, the question here seems centered on whether multicast can function over the internet.
To answer this accurately requires grasping two critical concepts:
- Multicast groups
- Routing protocols that support multicast
Multicast Groups:
A ‘multicast group’ is formed by devices wanting to receive certain data (like a live video stream). They indicate their intention by joining a multicast group with a specific IP address. This process involves Internet Group Management Protocol (IGMP), part of the Internet protocol suite.
When information is to be sent, it is delivered to this IP address – this is the multicast traffic. The routers or switches in between decide where to forward the packet based on the group requests they’ve received.
xxxxxxxxxx
Add code:
// An application joins a multicast source
setsockopt(sd, IPPROTO_IP, IP_ADD_MEMBERSHIP, &mreq, sizeof(mreq))
Routing Protocols Supporting Multicast:
For multicast to work across an entire internet would require that all routers along the way support the same multicast routing protocol – which they don’t. Many routers on the internet are not configured for multicast traffic and will simply drop such packets.
However, there are solutions for multicast communication over the internet:
- Anycast: A method combining unicast and multicast. It transmits from one sender to the nearest receiver in a group of potential receivers.
- Tunnelling: Multicast traffic is encapsulated within unicast or other types of packets to traverse portions of the network that don’t support multicast.
- Application Level Multicast: Multicasting is emulated at the application level rather than the network level. Information is spread via a overlay peer-to-peer network; each application receives a single stream and passes on to other recipients. An example of these is Content Delivery Networks (CDN).
It’s worth mentioning that multicast is widely used on private enterprise networks and multimedia content delivery networks. However, due to reasons including router hardware/software limitations, lack of widespread standardisation, security and quality-of-service concerns, multicast is generally not used on the public internet.
Finally, I want to address the most common internet scenario we encounter: streaming media. Even though it feels like you’re ‘broadcasting’ a video to many people online, in reality each viewer receives a separate unicast stream. This is known as HTTP Live Streaming (HLS). Despite its inefficiency compared to true multicasting, HLS has advantages in terms of compatibility and adaptability to different network conditions.
I hope this explanation provides a better understanding of Multicast and its relation to the internet landscape today. For further reading about those topics, I recommend visiting the following websites:
References:
The Differences Between Multicast and Unicast.
What is Multicast?.
IP Multicast Wikipedia.
Internet Protocol (IP) multicasting is a networking technique where one host transmits data to many recipients at once. IP Multicasting gives the ability to deliver data to multiple hosts simultaneously without putting an overhead on the source host to maintain and manage multiple connections. Multicasting uses Class D IP addresses in which a group of destination computers (known as a hosts group) are identified by a single IP address.
However, when it comes to multicasting over the Internet, things get a bit convoluted. In principle, multicast should work over the Internet. But due to several critical reasons, this idealistic scenario is not currently in place.
Here’s why:
1. Lack of Infrastructure Support:
Multicast routing requires specialized routers capable of recognizing and processing multicast packets, which is significantly different from normal IP packets. Many routers, particularly older ones, and some networks do not support multicasting, making the transmission of these types of packets impossible.
2. ISP and Network Policies:
Packet forwarding in multicasting is more complex than unicasting. It involves operations like creating distribution trees and managing packet replication. Many Internet Service providers (ISPs) and networks forbid the propagation of multicast packets due to administrative complexities, hardware limitations, and increased network loads.
xxxxxxxxxx
// Example of Java DatagramPacket for sending and receiving multicast
import java.net.*;
class MulticastSocketDemo{
public static void main(String[] args) throws Exception {
InetAddress group=InetAddress.getByName("224.0.0.1");
// Create a multicast socket.
MulticastSocket s=new MulticastSocket(3333);
// Join the Multicast group.
s.joinGroup(group);
String msg="Hello";
DatagramPacket datagramPacket=new DatagramPacket(msg.getBytes(), msg.length(),group,3333);
// Sending the message
s.send(datagramPacket);
// Close the socket
s.close();
}
}
3. No End-to-End Guarantee:
Despite the network infrastructure supporting multicast, there is no guarantee that every intended recipient will receive the data. In the presence of congestion or node failures within the network, packets may be lost.
On a brighter note, technology primarily designed for use in regional or local area networks – such as RTP (Real-time Transport Protocol), IGMP (Internet Group Management Protocol), PIM (Protocol Independent Multicast) – may effectively support multicasting source.
Although widespread VLAN (Virtual Local Area Network) multicasts are currently adopted broadly, deploying end-to-end multicast over the internet still faces significant hurdles. Applications in need of multicast services must rely on solutions like application-level multicast techniques or multicast-to-unicast conversion at edge servers in the Content Delivery Networks.
So, the question “Does multicast work over the Internet?” can be answered as follows: while theoretically possible, practical deployment of multicasting over the Internet presents significant challenges. However, advancements in both hardware and software are gradually enabling better support for internet multicasting.Multicasting and unicasting are communication methods used in networking to transmit data. They seem similar at first glance, but there are key differences that set them apart. Understanding these differences provides vital insights into network performance, efficiency, and scalability.
Unicasting
Unicasting is the most common form of network communication. It occurs when a single sender (or server) sends information to a single receiver (or client). This method is typically used for one-to-one communication over the Internet.
In the code snippet below, we are using Python’s
xxxxxxxxxx
socket
library for a simple implementation of unicasting:
Code Snipet |
---|
xxxxxxxxxx 1 21 21 1 import socket 2 3 # create a socket object 4 serversocket = socket.socket(socket.AF_INET, socket.SOCK_STREAM) 5 6 # bind the socket to a public host, 7 # and a well-known port 8 serversocket.bind((socket.gethostname(), 8080)) 9 10 # become a server socket 11 serversocket.listen(5) 12 13 while True: 14 # accept connections from outside 15 (clientsocket, address) = serversocket.accept() 16 17 # now do something with the clientsocket 18 # in this case, we will pretend this is a threaded server 19 ct = client_thread(clientsocket) 20 ct.run() 21 |
Multicasting
On the contrary, multicasting enables a single sender to send information to multiple receivers at once. It is mainly favored for group communication or live streaming services where data can be sent to numerous clients simultaneously.
Below, we simulate a multicast sender using Python’s
xxxxxxxxxx
socket
library:
Code Snippet |
---|
xxxxxxxxxx 1 15 15 1 import socket 2 import struct 3 4 # Set up the socket as a multicast sender 5 sock = socket.socket(socket.AF_INET, socket.SOCK_DGRAM) 6 7 # Set the time-to-live for messages, so they don't go past the local network segment. 8 ttl = struct.pack('b', 1) 9 sock.setsockopt(socket.IPPROTO_IP, socket.IP_MULTICAST_TTL, ttl) 10 11 # Send the message to multicast group 12 message = b'This is the multicast message' 13 multicast_group = ('224.3.29.71', 10000) 14 sock.sendto(message, multicast_group) 15 |
Does Multicast Work Over The Internet?
Even though it sounds appealing, multicasting isn’t commonly employed over the public internet due to technical difficulties. Among the challenges include:
– Many network devices on the Internet aren’t designed to handle multicast traffic, which leads to failed transmissions and packet loss.
– Another setback is that Internet Service Providers (ISPs) need to offer multicast support across their networks. Even if only one ISP within the chain doesn’t provide support, multicast won’t work since the packets wouldn’t cross that area of the network.
– Lastly but more critically, multicasting raises security concerns as it enables traffic amplification, making it an excellent tool for distributed denial of service (DDoS) attacks if left unregulated.
Consequently, multicast is mostly used within Local Area Networks (LANs) or targeted applications with specialized infrastructure and protocols like IPTV distribution.
For further reference on multicasting and how it works, consider reviewing the Cisco IP Multicast Technology Overview.
Unquestionably, one primary advantage of multicast over traditional unicast or broadcast is its potential to significantly improve bandwidth efficiency. But to fully grasp this, we first need to understand what exactly multicast is and how multicast traffic flows on a network.
Multicast is a method in the Internet Protocol (IP) for sending messages that will be delivered to a group of destination computers simultaneously. In other words, a single data packet is sent by the source and replicated by the network devices (like switches and routers), generating copies only when the network path diverges. This means only minimal traffic load is put on the source, the result being improved bandwidth usage.
Consider this:
– If you wanted to send a large video file to hundreds of recipients using unicast transmission, you’d have to transmit separate copies of that file individually to each recipient.
– On the contrary, if you were to use multicast distribution, you would only send one copy, and that would then be intelligently duplicated down the line as needed.
As a matter of fact, multicast communications share bandwidth communally and only use what is necessary, reducing congestion and providing substantial savings in both bandwidth consumption and network resources.
However, bear with me here – it’s not all sunshine and rainbows! The ‘multicast’ concept does have its limitations, especially when it involves the public Internet. Despite the Internet being based on IP, most of its infrastructure does not support native multicast communication.
You might think, “Why?”. Well, there are a few compelling reasons:
– Multicast routing implies extra complexity on routers as they not only have to find paths from point A to B but also efficiently distribute to multiple receivers.
– Lack of control over traffic since anyone can join a multicast group, potentially leading to security risks.
– Difficulty in implementing widespread multicasting due to its requirement of enhanced capacities of a broad range of equipment.
As a solution, various tunneling protocols like GRE or VPN systems are often used to allow carrying of multicast traffic over these networks that do not natively support multicasting. Moreover, application-scale multicast methodologies such as IP Multicast over Logical Overlay Networks (MOLNs) or protocol-agnostic scalable multicast (PASM) could offer promising alternatives.[1]
These techniques encapsulate multicast data packets within unicast or broadcast packets to transport them across networks that don’t support it nativity. However, they’re generally just patchwork solutions — they fail to exploit the full potential of efficient bandwidth usage inherent in multicast communication.
In short, while multicast has vast potential to enhance bandwidth efficiency, the widespread utility of native multicast over the Internet faces significant hurdles.
Here is a snippet of multicast code:
xxxxxxxxxx
import java.net.*;
class Multicast {
public static void main(String args[]) throws Exception {
InetAddress group = InetAddress.getByName("228.5.6.7");
MulticastSocket s = new MulticastSocket(6789);
s.joinGroup(group);
byte[] buf = new byte[1000];
DatagramPacket recv = new DatagramPacket(buf, buf.length);
s.receive(recv);
System.out.println(new String(recv.getData()));
s.leaveGroup(group);
s.close();
}
}
The above Java code demonstrates a simple implementation of joining a multicast group, receiving data, and then leaving the group. An understanding of socket programming is essential to comprehend multicast implementations.
I hope you found this deep-dive useful! Remember, while multicast does promise bandwidth efficiency, its application over the general internet tends to be limited due to various technical and security challenges. Future innovations may, however, propel multicast into more common-use scenarios.
References
1. [MolnCast: Multicast Over Logical Overlay Networks](https://ieeexplore.ieee.org/document/7403926)
The multicast system in networks is a unique approach that allows data to be delivered simultaneously from a source point to multiple destinations. Designed to enable efficient group communication, multicast technology reduces traffic load on networks by transmitting a single copy of data across the network backbone before splitting at downstream points to individual receivers.
Consider a tree with many branches representing a multicast network — data travels from root to branch tips, reproducing only when necessary. Interestingly, the Internet, as we know it, is designed around a unicast model, where data travels from a single source to a single destination. Yes, this raises questions about implementing multicast over the Internet.
The Dilemma: Does Multicast work over the Internet?
Generally, multicast works efficiently within Local Area Networks (LANs) which have routers configured to facilitate multicasting. Internet Service Providers (ISPs), on the other hand, do not usually support multicasting due to scalability issues and the administrative overhead associated with mass data distribution.
However, it’s important to note that multicast can technically function over the internet. But it demands detailed network management, global coordination, and dedicated resources on a scale that most ISPs wouldn’t commit. For this reason, successful multicast implementation on a larger scale is often limited to either VPN environments or managed networks.
A Framework for Successful Multicast Implementation
To implement a successful multicast over the internet, it’s crucial to address several technical complexities. Some strategies that could help include:
- Inter-Domain Multicast Routing: Special multicast routing protocols like Protocol Independent Multicast sparse mode (PIM-SM) must be configured to guide data packets across various network segments.
- Group Management: The Internet Group Management Protocol (IGMP) should be implemented for group management tasks such as joining and leaving multicast groups.
- Resource Reservation: Resource reservation protocols like RSVP (Resource Reservation Protocol) can help manage data flows and ensure Quality of Service (QoS).
As an example let’s take the scenario of setting up PIM-SM on a router using Cisco CLI:
xxxxxxxxxx
Router(config)# ip multicast-routing distributed
Router(config)# interface GigabitEthernet0/0
Router(config-if)# ip pim sparse-mode
This sets up the router for distributed multicast routing and enables PIM-SM on an interface. With these commands, the router will start establishing multicast trees and forward packets to interested hosts once they join the group using IGMP.
Remember, large-scale deployments also need to optimize for Any Source Multicast (ASM) or Source Specific Multicast (SSM) models depending on the application requirements. To wrap up, while multicast over the internet is technically feasible, it poses non-trivial challenges related to setup, administration, and resource management. Asking if multicast “works” over the internet is equivalent to asking if your car “works” on muddy, uneven terrain- yes, if it’s well-equipped and properly driven.Relevance of Using Multicast Over the Internet
Multicasting over the internet is a vital technology that allows for efficient data streaming and broadcasting. This technology is incredibly beneficial in various applications like multimedia content delivery, live audio/video streaming, update dissemination and so on.
• Data Efficiency: In essence, multicast allows a single packet to be dispatched to multiple recipients simultaneously by creating a ‘one-to-many’ or ‘many-to-many’ distribution scheme. Instead of sending individual copies of the same packet to each recipient, multicast sends a single copy. This facilitates not only efficient bandwidth utilization but also server resources.
xxxxxxxxxx
// A simple multicast example
MulticastSocket socket = new MulticastSocket(4446);
InetAddress group = InetAddress.getByName("230.0.0.0");
socket.joinGroup(group);
• Real-Time Communication: Multicast holds critical importance in supporting real-time applications. Services like live broadcasting, real-time gaming, video conferencing etc. use multicast technology to maintain timely and synchronous communication.
However, the implementation of multicast technology over the public internet has several limitations and technical challenges.
Limitations of Using Multicast Over the Internet
Even though multicast has significant advantages, implementing it on a global scale over the Internet is fraught with several challenges:
• Scalability Issues: The IP multicast model lacks scalability as the number of groups and members increase, particularly within larger networks such as the Internet. Managing memberships and subscription data become increasingly complex and resource-draining as the network grows.
• Network Infrastructure: Not all routers support multicasting. Non-supportive, older generation routers, switches and other networking devices deployed across different regions pose significant impediments to deploying multicasting globally across the Internet.
xxxxxxxxxx
// Check if your router supports multicast
$ ping 224.0.0.1
• Control and Security Problems: Multicast does not inherently provide mechanisms for access control or secure communications. Unsolicited or malicious users may exploit this, risking security breaches or Denial-of-Service attacks.
To summarize, though multicasting presents significant advantages in efficiency and real-time communication support, its deployment over the Internet faces substantial barriers related to scalability, infrastructure, control, and security.
For more nuances and case studies around “Does Multicast Work Over The Internet,” refer to The Multicast Dissemination Problem by Yakov Rekhter and “Multicast Over the Internet” SpringerLink chapter.The Internet Group Management Protocol (IGMP) plays a pivotal role in the implementation of multicast networking over the standard internet protocol suite, predominantly on the IPv4 network. IGMP helps with the management and regulation of IP multicast groups – which form the backbone of multicasting technology.
But before we delve into understanding IGMP, it’s important to clarify what exactly multicasting is, and how it functions over the internet. Unlike unicast messages that are sent directly from a singular source to a single recipient, or broadcast messages that are addressed to all nodes in a network, multicast messages are dispatched from one/multiple sources to a defined group of destinations on a network. This makes them considerably more efficient when dealing with applications like live video streaming, where the same data needs to spread to multiple recipients in real time.
html
Online gaming, IPTV delivery and distance learning are some additional multipoint applications leveraging multicast transmission.