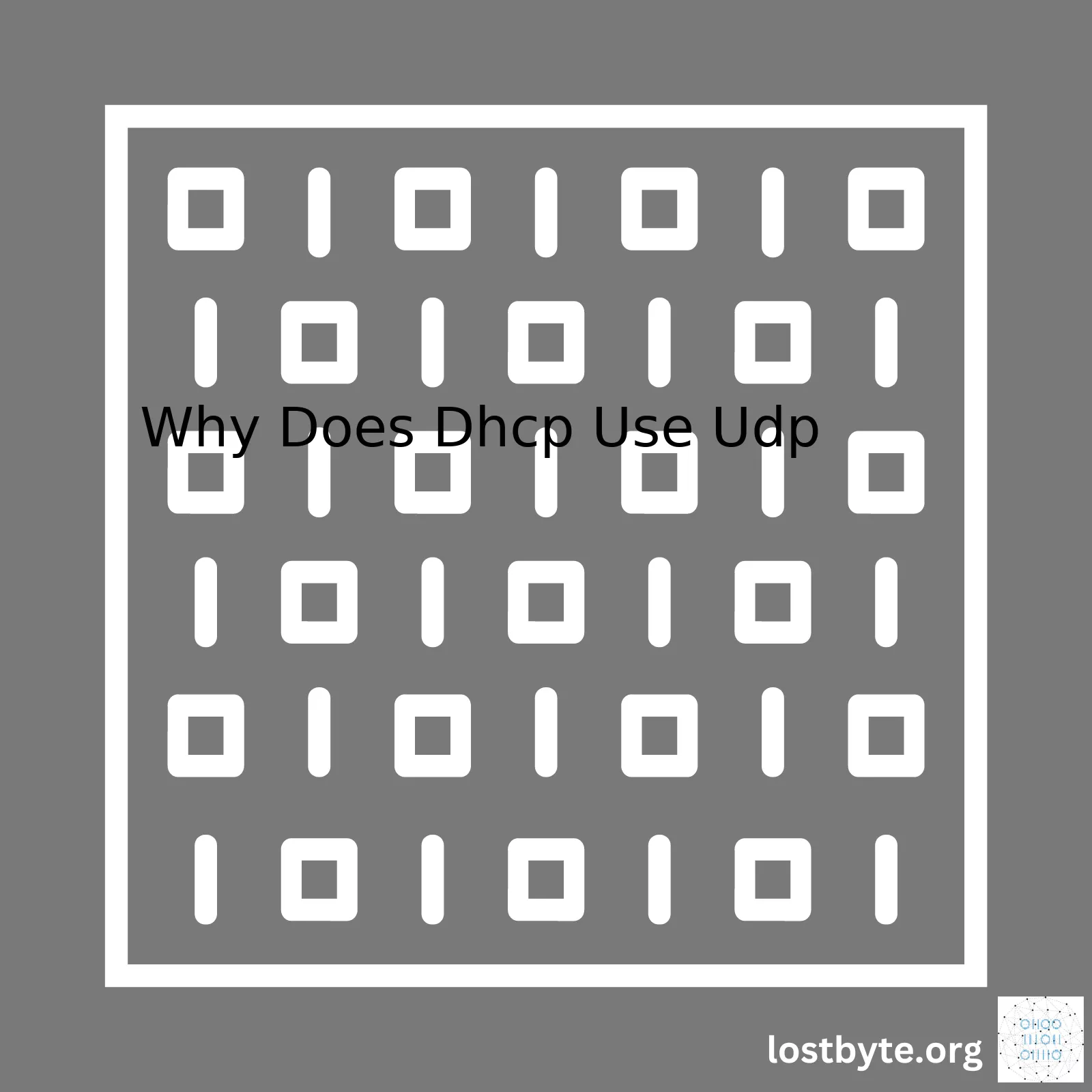
Feature | Description |
---|---|
DHCP uses UDP | The Dynamic Host Configuration Protocol (DHCP) uses the User Datagram Protocol (UDP) as its transport protocol. UDP is a connectionless protocol, meaning that it doesn’t need to establish connections before sending data. This makes it more suitable for DHCP, which needs to broadcast on the network and interact with devices even before they have an IP address. |
UDP enables broadcasting | Using UDP allows DHCP to perform broadcasting, which is essential for it to operate. When a device connects to a network, it sends out a DHCP discovery message as a broadcast. Since the device doesn’t yet have an IP address, it couldn’t make such a request using a connection-oriented protocol like TCP. |
Speed of UDP | UDP is faster than TCP (Transmission Control Protocol) since it has less overhead. This speed matters when a new device connects to the network, as it needs to get its IP configuration quickly. Using a slower protocol could disrupt the user’s experience. |
Efficiency of UDP | With UDP, data are sent in small packets without any form of sequencing or ordering. If a packet gets lost, there is no mechanism in place within the UDP itself to ask for retransmission. This design fits well with DHCP: if a DHCP transaction fails, simply starting over is typically quicker and easier than dealing with complex retransmission scenarios. |
The essence of DHCP using UDP lies in the inherent characteristics of the latter as a transport protocol. UDP’s connectionless nature is particularly significant for DHCP which needs to function smoothly even before devices are formally connected with their allocated IP addresses. UDP comes into action when a new device tries to communicate with the DHCP server through a broadcast message.
Without UDP, this initial communication would not be possible, as the device wouldn’t have the necessary IP address that connection-oriented protocols like TCP require. Also, being a lightweight protocol with low latency, UDP ensures swift assignment and renewal of IP addresses aided by its ability to send data in small packets without having to manage sequences or orderings.
Moreover, in case of any transmission failure, UDP’s simplicity plays a part where it’s easier to restart the entire process rather than taking on the burden of tracking missing packets and asking for them to be retransmitted. Therefore, it can be stated that DHCP’s reliance on UDP allows efficient IP address management and seamless user experience on various networks.
Reference: Coding Nuts and Bolts of the DHCP Protocol.Dynamic Host Configuration Protocol (DHCP) is a critical part of any network infrastructure and implements a configuration protocol, serving up IP addresses along with other network parameters to clients. One of the underpinnings of DHCP and its operation is UDP or User Datagram Protocol.
The underlying reason as to why DHCP uses UDP lies in the functionality, and how these two network principles operate in tandem:
– Daunting Efficiency: The use of UDP as the transport protocol for DHCP provides efficiency. Unlike TCP, UDP is a connectionless protocol. It does not require an elaborate handshake process before data transfer can begin, thereby reducing overhead costs in transmission.
Considering that DHCP is intended to be lightweight and efficient, especially in environments where many DHCP discover messages may be transmitted due to frequent client movements, this makes UDP ideally suited over TCP.
// DHCP client using UDP
clientSocket = socket(AF_INET, SOCK_DGRAM, IPPROTO_UDP);
– Validating Reliability: Another aspect to consider is reliability. Although UDP is typically deemed unreliable because it does not confirm the receipt of packets as TCP does, it yields appropriate reliability within the local network context. DHCP operations are predominantly LAN-based and packet loss on local networks is generally negligible.
– Broadcast Capability: DHCP operates using a client-server model where the DHCP server allocates IP addresses to clients. This involves two key communication types – unicast communication (one-to-one) and broadcast communication (one-to-all). Broadcast communication becomes crucial during DHCP’s address lease process. Upon connecting to a network, the DHCP client does not have an assigned IP address hence it resorts to broadcasting requests.
TCP does not support broadcasting, whereas UDP inherently does.
xxxxxxxxxx
// Client sending a broadcast message over UDP
broadcastAddr.sin_family = AF_INET;
broadcastAddr.sin_port = htons(UDP_PORT);
broadcastAddr.sin_addr.s_addr = htonl(INADDR_BROADCAST);
While TCP fits well for applications that require high reliability where data needs to be delivered in exact order and drops are unacceptable, such as web servers, FTP, etc., applications like DHCP benefit from being built on top of UDP because they need good performance and no strict delivery guarantees.
Finally, it is important to understand that UDP and DHCP allow for rapid, efficient networking with minimal overheads. A fact captured in the initial design choices that were made when implementing protocols like DHCP. These decisions have enabled seamless and dynamic IP management within diverse scales of networks.
For further reading, feel free to browse through this article “Learn the benefits and drawbacks of TCP and UDP”.
Remember, all network protocols including DHCP and UDP are designed with a specific purpose in mind and each one has found their niches based on their unique properties and the demands of particular applications.DHCP or Dynamic Host Configuration Protocol is a network protocol used in IP networks. Interestingly, DHCP includes automatic configuration of IP addresses for every device on your network.
The primary functionalities of DHCP are:
-
xxxxxxxxxx
111IP address allocation:
The DHCP server assigns an IP address to each device on the network.
-
xxxxxxxxxx
111Lease renewal and refreshing:
DHCP leases out IP addresses for a limited time period and allows for their renewal or refreshing post-expiry.
-
xxxxxxxxxx
111Delivery of other configuration details:
Apart from IP addresses, DHCP servers also deliver other related configuration details such as subnet masks, default gateways, etc.
Now, answering why exactly DHCP makes use of UDP or User Datagram Protocol is a fun hardware/software journey.
The core rationale derives from the inherent characteristics of UDP. Unlike TCP (Transmission Control Protocol), which ensures data delivery through retransmissions, acknowledgments, error checking, and flow control – UDP offers none of those merits. You might ask – Why would you prefer a less reliable protocol?
Well, when it comes to DHCP, some of its essential operations, such as broadcasting, require connection-less and lightweight communication – a key trait delivered by UDP. When a client machine connects to a network, it has no IP address initially. It broadcasts a request for one, and the DHCP server responds with an IP address assignment.
This communication happens over UDP Ports. The client machine sends DHCPDISCOVER/DHCPREQUEST messages to the UDP destination port 67, and the server sends DHCPOFFER/DHCPACK messages to the UDP destination port 68.
Let’s look at a sample DHCP packet exchange sequence:
xxxxxxxxxx
Client Server
——— ———
DHCPDISCOVER |
------------> | (Broadcast)
| DHCPOFFER
<---------- |
DHCPREQUEST |
------------> |
| DHCPACK
<------------ |
Also, since DHCP doesn't require maintaining a session state, it is logical to avoid the overhead associated with TCP, making UDP a perfect fit.
So, to sum up, UDP suits DHCP because it's lightweight, it supports broadcasting, and it simplifies travel across routers and firewalls. However, the lack of congestion control means that networks must be designed to prevent saturation with DHCP traffic, which could potentially cause packet loss.
Moreover, here is an interesting Link for further reading about "Why does DHCP use UDP?" that elaborates more on the subject.When evaluating why DHCP (Dynamic Host Configuration Protocol) uses UDP (User Datagram Protocol), it's important to delve deep into the characteristics and functionalities of both protocols.
DHCP is an auto-configuration protocol used on IP networks. It operates on a client-server model where DHCP Client requests for and gets IP from DHCP Server source.
UDP, on the other hand, is one of the core protocols in the Internet protocol suite. It's connectionless, meaning communication doesn't require a connection setup phase, each message (or datagram) includes full destination address information, and the receiving computer sends no acknowledgement of receipt.
Reasons as to why DHCP employs UDP include:
Efficiency:
UDP plays a significant role in making DHCP efficient due to its stateless nature. The server doesn't have to keep track of what state the client workstation might be in. This low overhead protocol doesn’t demand a cyclic redundancy check, flow control, sequencing or acknowledgements, hence utilizes less bandwidth - ideal for applications like DHCP whereby vast numbers of clients request addresses simultaneously.
xxxxxxxxxx
Client --(DHCP discover)--> 255.255.255.255
Server --(DHCP offer)-->255.255.255.255
Client --(DHCP Request)--> 255.255.255.255
Server --(DHCP Acknowledgement)--> Client
Broadcast capability:
Since DHCP server might not always know the specific address of all the clients in a network, it makes use of UDP’s broadcast feature. Using UDP allows a DHCP client that does not yet have an IP address to communicate with a DHCP server.
Error Checking:
Although UDP offers minimum functionalities, it checks for errors. The sender bundles data into packets called datagrams and forwards them to the recipient sans checking if they're received or arranged correctly at the receivers' end. However, it does add a checksum to every datagram to help the receiver identify corrupt packages. For DHCP operations, this simple error-checking mechanism suffices.
Swift Connection:
In a TCP/IP environment, users expect a swift connection when using web browsers or sending emails. Considering this, DHCP's leverage on UDP enables quick transmission times without worrying about numerous connections to manage. Due to no three-way handshake as in TCP, the communication turns out to be faster.
As seen in the above points, UDP provides several features crucial for DHCP functionality. Its simplicity, efficiency, speed, and robustness in handling many simultaneous communications makes it the appropriate choice for DHCP.UDP, or User Datagram Protocol, plays an essential role in ensuring seamless network communication. One of its key roles is to provide a transport mechanism for the Dynamic Host Configuration Protocol (DHCP) which harnesses UDP's potentials for delivering time-efficient and streamlined performance.
The Role of UDP
The UDP performs an important function by enabling application programs to send messages, also known as datagrams, within a networked computer environment. This protocol, due to its connectionless nature, ensures that there are no delays in communication, and data is transmitted quickly without obligatory handshaking dialogues.
xxxxxxxxxx
// Example of code illustrating the principle of UDP
import java.net.*;
public class UDPServer {
public static void main(String[] args) throws Exception {
// Creating Socket
DatagramSocket ds = new DatagramSocket(3000);
byte[] buf = new byte[1024];
// Create Datagram Packet
DatagramPacket dp = new DatagramPacket(buf, 1024);
ds.receive(dp);
String str = new String(dp.getData(), 0, dp.getLength());
// Data display
System.out.println(str);
ds.close();
}
DHCP and UDP
Why does DHCP use UDP then? The choice boils down to its reliability and efficiency. As a protocol that assigns IP addresses dynamically to devices in a network, DHCP relies on the speed and simplicity of UDP. With UDP, DHCP can swiftly allocate IP addresses, hence enhancing overall network performance.
UDP-DHCP Interaction for Network Communication
Here's an overview of the interaction between DHCP and UDP:
- DHCP begins the process using UDP as its transport layer.
- A device sends a DHCP discover message encapsulated in a UDP packet to initiate getting an IP address.
- The server acknowledges this request with a DHCP offer message.
- The client responds back with a DHCP request also packed inside a UDP packet to accept the offered IP address.
- Finally, the server sends a DHCP acknowledgement to finalize the assignment.
These interactions demonstrate precisely why DHCP is reliant on UDP for efficient, uninterrupted network communication.
Additionally, the robustness of UDP also comes into play when dealing with BOOTP relay agents in broader network scopes. These agents forward DHCP messages between subnets, with UDP bearing the load of these inter-subnet communications incredibly well.
To read more about the subject, you can visit the Internet Engineering Task Force's official RFC page for DHCP. There, you'll find deep dives into the working specifics of DHCP and how UDP rolls in its operations.
In summary, by providing a no-nonsense, high-speed data transfer capability, UDP enables DHCP to carry out high-performance IP assignments across networked devices for a seamless communication experience.The fundamental reason why DHCP (Dynamic Host Configuration Protocol) uses UDP (User Datagram Protocol) revolves around its core attributes of being connectionless and stateless, which matches the needs of DHCP perfectly.
To understand this in depth, we should first uncover what exactly DHCP and UDP are:
DHCP - Dynamic Host Configuration Protocol: This is a network management protocol used on IP networks where your DHCP server dynamically assigns an IP address and other network configuration parameters to each device on your network, so they can communicate with other IP networks Wikipedia.
UDP - User Datagram Protocol: A transport level protocol that does not rely on previously established connections and session for data transmission, reducing the overhead required as opposed to its counterpart TCP (Transmission Control Protocol). In simple language, it forwards packets without concerning whether they reach their destination or not. Whether packets get lost, arrive out of order, or contain errors doesn't affect UDP's operations Wikipedia.
With these understandings, we can now drill deeper into why DHCP utilizes UDP and the benefits this delivers:
Low Overhead
When working with large networks it becomes crucial to manage resource usage effectively. Since UDP lacks rigorous error checking, sequencing and acknowledgment routines kept by TCP, the processing power required by DHCP servers is less intense, permitting improved scalability and performance.
xxxxxxxxxx
// Example: DHCPDiscover message in UDP
// Source Port = 68
// Destination Port = 67
Efficiency in Broadcast Messaging
As DHCP starts communication over an 'IP broadcast', it essentially sends messages to everyone on the subnet. Hence, it requires a mechanism that allows one sending point but multiple receiving points without ensuring guaranteed delivery, which syncs seamlessly with the behavior of UDP.
xxxxxxxxxx
//Example: DHCPOffer message in UDP
//Source Port = 67
//Destination Port = 68
Statelessness
As DHCP assigns IP addresses, often the two parties need to be unaware of each other's existence prior to communicating. This calls for a protocol that is capable of facilitating communication without maintaining session information or having a predetermined route, properties achievable through UDP.
Error Handling isn't Required
Since the DHCP transaction is short-lived only requiring four exchanges (DHCP Discover, DHCP Offer, DHCP Request, DHCP Acknowledgement), the need to ensure every packet reached its destination isn't necessary, thus not having error-checking mechanisms like TCP becomes advantageous.
xxxxxxxxxx
//Basic DHCP handshake in UDP
//Client broadcasts DHCPDISCOVER
//Server(s) responds DHCPOFFER(s)
//Client requests one offer with DHCPREQUEST broadcase
//Server confirms with DHCPACK
In conclusion, while there may be potential downsides to employing UDP due to its lack of error correction and guarantee of packet delivery, these are not significant concerns when focused on DHCP's function. The benefits overwhelmingly outperform the drawbacks, making UDP a natural alliance to DHCP in our hyper-connected and ever-evolving digital landscape.DHCP (Dynamic Host Configuration Protocol) uses UDP (User Datagram Protocol) for its operation. This is as a result of the roles, functionalities, and the nature of the DHCP's operational characteristics that require a connectionless protocol type like UDP.
Connectionless Communication:
DHCP operates in a connectionless manner which is perfectly served by the use of UDP being a connectionless protocol.
xxxxxxxxxx
Source PC ------(DHCP Discover)---> Broadcast
Source PC <-----(DHCP Offer)------- DHCP Server
Source PC ------(DHCP Request)---> Broadcast
Source PC <-----(DHCP Ack)--------- DHCP Server
When a host powers up and tries to connect to a network, it does not know about any other device on the network, including DHCP servers. What the host does is send a 'DHCP Discover' message as a broadcast. All machines on that local network will get this broadcast message, but only DHCP servers will respond to it with a 'DHCP Offer'.
Using TCP would mean establishing a session before communication can take place. However, at this early stage, the host knows neither the IP address of itself nor any DHCP server. So, it's not possible to establish a TCP session. Hence the need for UDP as it enables broadcasting to communicate with DHCP servers when other details are unknown.
Reduced overhead:
UDP is a faster protocol because it has less overhead compared to TCP (Transmission Control Protocol). It does not provide mechanisms such as delivery confirmation, ordered packets, re-transmission of lost packets which are provided by TCP.
When dealing with DHCP operations such as acquiring an IP address or renewing it, there is a need for speed, making UDP the perfect choice. What's more, the information exchanged during the DHCP process is small and quite manageable, so the extensive features of TCP are not required.
Port numbers:
The port numbers used by DHCP are 67 and 68, specifically assigned to DHCP servers and clients, respectively. These port numbers lie in the range of Well Known Ports (0 to 1023), denoting their universal significance in the context of networking. The reference code snippet is:
xxxxxxxxxx
udp.SrcPort = 68;
udp.DstPort = 67;
In summary, the mechanics behind the usage of UDP by DHCP involves factors such as being connectionless, having reduced overhead and using specific port numbers. Considering these reasons, DHCP uses UDP due to its simplicity, low overhead, and ability to broadcast messages, which aligns with the requirements and operations of DHCP.
For further reading, please check out these resources:
RFC 2131 (DHCP),
RFC 768 (UDP).The correlation between the Dynamic Host Configuration Protocol (DHCP) and service speed is intricately linked to the DHCP's use of the User Datagram Protocol (UDP).
First, a bit on what DHCP and UDP are:
xxxxxxxxxx
DHCP
is a network management protocol used on Internet Protocol (IP) networks for automatically assigning IP addresses and other communication parameters to devices connected to the network. Essentially, this eliminates the need for a network administrator to manually assign these addresses.
xxxxxxxxxx
UDP
, on the other hand, is one of the core members of the internet protocol suite. It's connectionless and does not require handshaking dialogues, leading to simpler setup for transmission channels and data flows on the network.
Now, how do these two relate?
When it comes to quick service and swift responses, especially in server-client communications across a network, both DHCP and UDP play significant roles. The most prominent connection between these two becomes evident when you consider why DHCP uses UDP.
The Perks of DHCP Using UDP:
- Faster Speeds: UDP brings a speed advantage due to its minimal protocol requirements. Unlike TCP, there's no need for establishing connections and waiting for acknowledgments before sending data. This aspect greatly increases the speed of transmitting DHCP packets across the network.
- Stateless Operation: Being stateless, UDP allows DHCP servers to quickly move onto the next request without having to keep track of existing connections or states. Thus, any delay related to maintaining connection states does not impact the service speed.
- Broadcast Ability: UDP supports broadcasting, a key feature that DHCP needs. For instance, when a device seeks an IP configuration from a DHCP server, it broadcasts a request to the entire network. Only a protocol that supports broadcasting can enable such operations efficiently.
In summary, the DHCP protocol's service speed benefits greatly from the simplicity, efficiency and broadcasting capabilities when using UDP as opposed to other protocols like the Transmission Control Protocol (TCP). This lowered complexity and increased bandwidth result in faster speeds when DHCP assigns IP addresses or facilitates client-server communication.
Here is a simplified version of DHCP using UDP:
Client | Action | Server |
---|---|---|
DHCP Discover (Broadcast) | ||
&x2191; | &x2193; | |
DHCP Offer (Unicast) | ||
&x2193; | &x2191; | |
DHCP Request (Broadcast) | ||
&x2191; | &x2193; | |
DHCP ACK (Unicast) |
Step by step explanation:
1. The client sends a DHCP discover message, broadcast to all devices on the network.
2. The server gets this discover message.
3. The DHCP server responds with a DHCP offer, which is unicast to the client. This message contains the network configuration settings.
4. On receiving the DHCP offer, the client broadcasts a DHCP request, requesting the offered address from the DHCP server
5. The server receives this request and replies with a DHCP acknowledgement message(unicast), indicating that the network layer has been properly configured
This simplified process reflects the efficiency of DHCP via UDP. It handles requests seamlessly, resulting in quicker end-user experiences when accessing the network services. For more detailed information — check the IETF's official RFC2131 that explains DHCP here. For more about UDP, see its dedicated RFC768 available here.When the subject arises of why DHCP (Dynamic Host Configuration Protocol) uses UDP (User Datagram Protocol) instead of TCP (Transmission Control Protocol), it's mostly about efficiency and less about reliability in the traditional machine-to-machine sense.
To explore this question, let's delve into a few reasons why DHCP utilizes UDP:
Connectionless Nature
Unlike TCP, which is connection-oriented protocol and requires a connection to be established before any data transfer occurs, UDP is a connectionless protocol. This means that packets can be sent without any setup processes or teardowns. This property comes in handy when configuring multiple clients, as the server doesn't need to maintain a long-lasting connection with every client. The DHCP process can go like this:
xxxxxxxxxx
Client -> DISCOVER -> Server
Server -> OFFER -> Client
Client -> REQUEST -> Server
Server -> ACK -> Client
Each arrow represents one UDP communication. This sequence allows hosts to discover DHCP servers' network configuration parameters, such as IP addresses.
Broadcast Ability
UDP supports broadcasting where you send a single packet to all devices on a network. When a new device enters a network and wants to get an IP address, it doesn’t know who the DHCP server is, so it broadcasts a "DHCP Discover" message to everyone on the network. Only UDP has the ability to do this effectively because TCP is unicast and point-to-point.
Better Efficiency
As the primary function of DHCP is to assign IP addresses dynamically to clients, the overhead created by TCP would not necessarily offer significant added value nor reliability benefits. TCP's delivery acknowledgment mechanism would be too time-consuming for a service whose role is based on assigning transient IP addresses to machines from a predefined pool. In contrast, UDP is more lightweight and faster than TCP due to its lack of connection establishment and data verification.
Flexibility
Another pertinent factor is the flexibility of UDP. The sender does not have to wait for receipt confirmation for each packet sent, unlike in TCP. Because of this, messages can be dispatched quicker. The nature of DHCP requests and assignments being short-lived interactions perfectly fits well with UDP.
Regarding implications on system performance, there’s a double-edged sword here. On the one hand, since UDP is light and fast, this results in lesser demand on system resources when processing the UDP/DHCP interaction. On the other hand, being lightweight also means that UDP lacks the delivery assurance inherent in TCP. This might result in scenarios wherein a DHCP message could potentially be lost and an IP address might not be assigned to a client. However, in practice, given network speeds and hardware reliability, this issue seldom occurs. Additionally, even in circumstances where it does happen, DHCP includes retransmission strategies which ensure that a client will repeat its request until an address is successfully obtained thus still maintaining system performance integrity.
The choice of using UDP for DHCP despite its lack of some extensive feature set ostensibly offered by TCP is guided by balancing out various needs including simplicity, speed, resource usage, and suitability to use case, which hints at prudent protocol design. For better understanding you can refer TCP & UDPCertainly, understanding the technicalities behind choosing UDP (User Datagram Protocol) over TCP (Transmission Control Protocol) for DHCP (Dynamic Host Configuration Protocol) requires an in-depth knowledge of both protocols and their uses, specifically regarding the network communication process.
UDP and TCP Differences
To initiate the discussion, let's first clarify that TCP and UDP are transport protocols. They are utilized for sending bits of data — known as packets — over the Internet. Both have unique characteristics that make them suitable for different applications.
xxxxxxxxxx
TCP
is connection-oriented, meaning it ensures the delivery of packets in the same sequence they were sent. This makes it reliable but slower because of the robust error-checking mechanisms.
xxxxxxxxxx
UDP
on the other hand, is a connectionless protocol. It doesn't guarantee the delivery or the order of packets, making it quicker and more efficient for certain functionalities, but also less reliable where data integrity is concerned.
The choice between these two protocols essentially relies on whether you need reliability or speed in your application.
Speed and Efficiency are Crucial for DHCP
DHCP is designed to assign IP addresses to devices connected to a network. Given its function, speed and efficiency are much more critical than ensuring a strictly ordered delivery of data packets. The process needs to be conducted quickly and consumes as minimal network resources as possible; hence
xxxxxxxxxx
UDP
, with its fast and light-weight capabilities, is the preferred choice.
Further, by its very nature, DHCP uses a broadcast approach when assigning an IP address. That means DHCP messages must reach all hosts within the local network segment, something that
xxxxxxxxxx
UDP
caters to perfectly.
DHCP Processes favor UDP Characteristics
Take note that a device getting its IP address via DHCP goes through four steps, encapsulated in a DORA process (Discover, Offer, Request, Acknowledgement).
xxxxxxxxxx
Discover
step, the client sends out a broadcast message searching for a DHCP server. Because it's a broadcast and doesn't know yet who to connect with, it cannot establish a TCP session with a specific server. Instead, it uses
xxxxxxxxxx
UDP
to send a "connection-less" broadcast.
xxxxxxxxxx
Offer
,
xxxxxxxxxx
Request
, and
xxxxxxxxxx
Acknowledgement
steps follow the Discover step. All these three steps can implement TCP theoretically, but remember that consistency isn't necessarily a priority for these operations. These steps involve negotiation about the provided IP address which, if doesn't go as expected, the DORA process simply restarts. So, a missed message wouldn't fundamentally halt the process. Here again,
xxxxxxxxxx
UDP
helps keep things efficient and quick.
To back up these points, you may find a detailed exploration into the differences between TCP and UDP in this lifewire post. As well, the complete walkthrough of DHCP DORA Process is nicely laid out on Tutorials Point.
I hope this provides some thorough insights into why DHCP predominantly uses UDP instead of TCP. Though, it's important to note that depending on varying network rules and requirements, there could be exceptions to this norm.The primary reason DHCP uses UDP (User Datagram Protocol) over TCP (Transmission Control Protocol) revolves around the functionality and attributes of both protocols and how they apply to the DHCP's requirements.
Firstly, let's remark on the differences between TCP and UDP:
-
xxxxxxxxxx
111TCP
: A connection-oriented protocol that ensures the delivery of packets in the order in which they were sent. It is reliable due to acknowledgements after each packet received and re-transmission of lost packets.
-
xxxxxxxxxx
111UDP
: A connectionless protocol that doesn't guarantee the delivery or order of packets – it just sends them. It typically used for applications where speed is a dominant factor as there is no need for establishing and terminating a connection.
DHCP (Dynamic Host Configuration Protocol), which dynamically assigns IP-addresses to devices in a network, utilizes the UDP protocol instead of TCP. Now why is this? Here are some points to consider:
Bootstrap Problem With TCP
TCP needs an IP address to establish a connection. But in the case of DHCP clients, they contact the server before they have an IP address. This chicken-egg situation creates a compatibility issue with TCP. Can't start a TCP session without knowing who you are; can't discover who you are without starting a TCP session.
This problem is resolved by using UDP that doesn't require any pre-established session or connection. Clients can send broadcast messages via UDP to discover DHCP servers initially.
Broadcasting Capability
TCP isn't designed for broadcasting whereas UDP supports it. As DHCP mainly works based on broadcasting messages (IP request, IP lease etc.), UDP's broadcasting capability makes it a compatible choice.
Less Overhead
Compared to TCP, UDP has less overhead because of its simple structure and lack of flow control or error checking. This becomes advantageous when catering to multiple requests in a large network.
Reliability In Case Of Dropped Packets
While TCP guarantees delivery via acknowledgments and packet re-transmission, it could result in unnecessary delays for temporary connections such as DHCP. With UDP, if a packet is dropped due to network congestion or other issues, the DHCP client will simply send a new request after the current one times out. This provides an inherent fallback mechanism.
In the world of communicating computer systems, different protocols serve various purposes. For DHCP, UDP proves to be a more efficient and suitable alternative to TCP. Even though transmitting data over UDP might seem riskier compared to its reliable counterpart TCP, given the ephemeral nature and requirements of DHCP communications, these "risks" actually become beneficial elements. However, it still depends on the network implementation to adopt the proper combination of using TCP/UDP based on their specific needs and use cases.
For further detailed understanding, you can refer resources like RFC 2131 which elaborates on DHCP's architecture and communication mechanism.
Also, for more information about UDP:
xxxxxxxxxx
// Packet structure
0 7 8 15 16 23 24 31
+--------+--------+--------+--------+
| Source | Destination |
| Port | Port |
+--------+--------+--------+--------+
| | |
| Length | Checksum |
+--------+--------+--------+--------+
|
| data octets ...
+---------------- ...
And here's a brief excerpt demonstrating how a DHCP negotiation unfolds utilizing UDP:
xxxxxxxxxx
// Client broadcast to locate available servers
SERVER: OFFER (broadcast from server offering IP address)
CLIENT: REQUEST (client requesting offered address from server)
SERVER: ACK (server acknowledging, lease made).
Takeaway is, UDP wins over TCP for DHCP due to bootstrap problem, broadcasting ability, less overhead, and handling dropped packets efficiently.The DHCP (Dynamic Host Configuration Protocol) in networking makes use of UDP (User Datagram Protocol) because of its simplicity, scalability and stateless nature. They work together to simplify the networking needs by automating the IP address and other network settings on network clients.
UDP and DHCP: The Perfect Combination
DHCP uses UDP as its transport protocol as it delivers a fast and lightweight solution for systems to send messages without establishing a connection.
xxxxxxxxxx
Client --> DHCPDISCOVER --> Server
Client <-- DHCPOFFER <-- Server
Client --> DHCPREQUEST --> Server
Client <-- DHCPACK/DHCPNACK <-- Server
In each step of this process, a message is sent between the client and the server. And all these communication steps happen using the UDP protocol.
Impact on Networking Needs
Combining UDP with DHCP simplifies the networking needs in several ways:
* IP Address Management: DHCP dynamically assigns IP addresses. It eliminates need for manual allocation, therefore reduces errors.
* Server Client Communication: With UDP, communication is initiated almost immediately as it doesn't require TCP's three-way handshake.
* Lightweight: UDP is less resource-intensive which makes the processes quick and efficient
* No Connection Overhead: UDP being stateless contributes to making maintenance simple.
DCHP’s Use of UDP Ports
It's noteworthy that DHCP specifically operates over UDP ports 67 and 68. The server listens on port 67 for client requests, and the client listens on port 68 for server responses:
xxxxxxxxxx
Server: UDP Port 67
Client: UDP Port 68
By opting to make use of UDP, DHCP provides a more streamlined and effortless way to address network configuration challenges. When dealing with the complexities of network management, the DHCP/UDP combination contributes a great deal towards simplifying matters.
While often overlooked, the relationship between DHCP and UDP plays a pivotal role in networking. Simplifying the administration of network settings, easing communications between servers and clients, and doing it efficiently and at scale, is a task that many network administrators are grateful for. Reference from Cisco.
Finally, understanding these intricacies goes a long way to managing and troubleshooting network issues effectively. Consequently, it might not be wrong to say that a combination of DHCP and UDP indeed forms the backbone of modern day networking needs.In the realm of networking, DHCP (Dynamic Host Configuration Protocol) plays a pivotal role by assigning IP addresses dynamically to devices so they can communicate with the other devices on the network. To comprehend how reliability is maximized with DUP in DHCP, it's important to acknowledge that DHCP employs UDP (User Datagram Protocol) rather than TCP (Transmission Control Protocol). Let me delve into the reasons behind this choice and thus shed some light on maximizing reliability.
UDP is a connectionless protocol. This means there isn't much overhead because it foregoes formal connections and error checking. In contrast, TCP is a connection-oriented protocol which needs a formal handshake process to establish a connection before data transfer takes place. From an operational point of view, DHCP requires fleeting interaction between the server and the client. Hence, DHCP using UDP ensures efficiency as there's no need to set up and manage TCP sessions for transient communications.
Consider the following code snippet demonstrating the lightweight property UDP brings to DHCP:
xxxxxxxxxx
// Create a DHCP Discover Packet
DHCPCore dhcpc = new DHCPCore(0, 67, 68);
// Use udp for sending packets
UDPPacket udp = new UDPPacket(dhcpc, srcip, 67);
Reliability in the context of DHCP doesn't come from confirmation that the data has been safely delivered - like TCP would ensure - but rather from the capability to maintain its function even with high host mobility due to different networks or frequent network configuration changes.
That’s where DUP comes into play. DHCP works closely with DNS update protocol or DUP to maximize the system's reliability. By doing so, DHCP updates DNS anytime a new lease provision occurs or when a device's IP address changes. This concurrent updating reduces potential errors and ensures that all nodes within the network have accurate records of IP addresses, resulting in maximum reliability.
Let's put these concepts visually in terms of table:
DHCP | UDP | DUP | |
Efficiency | High (due to dynamic IP allocation) | High (lightweight protocol without session setup) | Moderate (constant DNS record maintenance) |
Reliability | Moderate (Depends on continuous availability) | Low (Does not ensure delivery) | High (Ensures DNS and DHCP are always synchronized) |
Justifying DHCP's usage of UDP alongside DUP becomes clear once one understands the ephemeral nature of the DHCP communication model. Involving TCP, with its emphasis on ensuring delivery, might cause an unnecessary burden both on performance and system resources. Employing UDP, a leaner protocol with less "ceremony," allows DHCP to do what it does best with minimal fuss. Coupled with DUP to handle the inevitable changes in the network structure, it maintains an optimal hold on reliability.
For more information regarding [DHCP and UDP](https://www.cisco.com/en/US/tech/tk648/tk361/technologies_tech_note09186a0080094aee.shtml), Cisco provides quite comprehensive documentation. For my fellow coders out there, you might find the DHCP [library for Java](https://github.com/intrbiz/dhcp4j) pretty interesting. Remember that nothing tops hands-on experience in getting a firm grasp on these protocols!The Dynamic Host Configuration Protocol (DHCP) makes use of User Datagram Protocol (UDP) as its transmission protocol. Given their inherent differences, you might wonder, why DHCP chose UDP over popular Transmission Control Protocol (TCP).
Here are several reasons:
-
Efficiency:
-
Unicast limitations:
-
Broadcast advantages:
When it comes to assigning IP addresses, efficiency is key. The connectionless nature of UDP requires fewer resources and provides higher speed, making it quite apt for DHCP. Unlike TCP, which establishes a robust connection before data transfer leading to time and resource consumption, UDP directly sends datagrams without this setup stage, gaining efficiency.
While TCP is potentially a suitable choice, it fails when the client configuration is in question. When computers first connect to a network, they don't have an IP address assigned, making unicast communications impossible since TCP uses IP-based unicast connections.
In situations like above, where the client doesn't possess an valid IP, UDP’s broadcast capability stands out. UDP enables data to be sent to every device on the network by broadcasting. Such functionality isn't possible with TCP, making UDP the prime requirement for DHCP's functionality.
xxxxxxxxxx
# Sample function in Python demonstrating UDP client-server communication for better understanding.
import socket
def server_program():
server_socket = socket.socket(socket.AF_INET, socket.SOCK_DGRAM)
server_socket.bind(('localhost', 5000))
while True:
message, address = server_socket.recvfrom(1024)
print("Received message from client: " + message.decode())
server_socket.sendto("Hello from server!".encode(), address)
def client_program():
client_socket = socket.socket(socket.AF_INET, socket.SOCK_DGRAM)
client_socket.sendto("Hello from client!".encode(), ('localhost', 5000))
while True:
message, address = client_socket.recvfrom(1024)
print("Received message from server: " + message.decode())
@section
To wrap it up, DHCP employs UDP due to its efficiency, flexibility, and compatibility with broadcast messaging protocols. These advantages make UDP more suitable than TCP for DHCP applications. It isn’t merely a matter of preference, but rather a technical necessity driven by the major objective of DHCP – efficiently managing the distribution of IP addresses within networks.
You can obtain additional information about DHCP and UDP from the official documentation of the Internet Engineering Task Force (RFC 2131). I also recommend network technology articles on NetworkWorld for further learning.