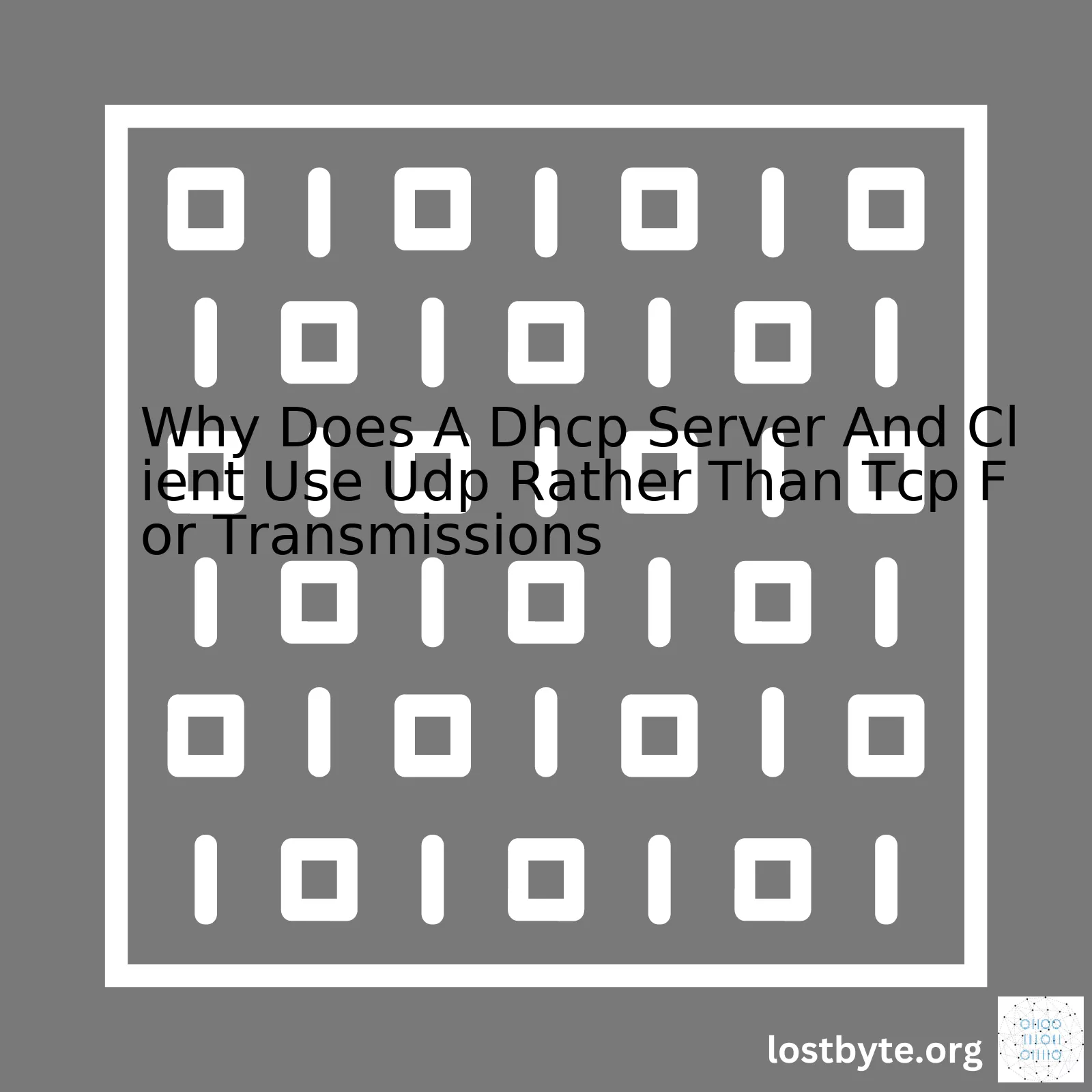
Parameter | TCP | UDP |
---|---|---|
Speed | Relatively Slow | Fast |
Connection | Connection-oriented | Connectionless |
Acknowledgement Packet | Yes | No |
Delivered In Order | Yes | No guarantee |
Now, regarding why DHCP server and clients use UDP rather than TCP:
The main reason that DHCP servers and clients prefer Unacknowledged Datagram Protocol (UDP) over Transmission Control Protocol (TCP) revolves around efficiency and simplicity. Since DHCP is essentially a broadcast protocol and it doesn’t anticipate any extended conversation – it uses the UDP suite.
To give you more context, let’s break down their foundational differences:
– UDP as the ‘Speed’ row suggests, is much faster than TCP. This is partially because UDP is lighter in nature; it does not carry around the extra weight of tracking and ordering packets as TCP does.
– Moreover, UDP establishes instantaneous ‘connectionless’ communication as stated in the ‘Connection’ row. Unlike TCP, UDP doesn’t conduct a three-way handshake to establish a connection before transferring packets between sender and receiver. This results in less overhead and quicker responses, advantageous for DHCP which works on ‘trust-and-response’ mechanism.
– Acknowledgement of sent packets, or lack thereof, is a fundamental difference between TCP and UDP. TCP will confirm receipt of transmitted packets. In contrast, as shown in our ‘Acknowledgement Packet’ row, UDP does away with acknowledgements, further increasing efficiency.
Remember, DHCP transactions are short and meaningful: A client asks for an IP address, and the server responds with one. This four-step process (Discover-Offer-Request-Acknowledge), known as DORA, amplifies the need for speed, simplicity and efficiency, hence the preference for UDP.
If you wish to delve even deeper, I encourage you to explore the online RFC2131 – Dynamic Host Configuration Protocol, the primary source for understanding the design decisions behind DHCP’s infrastructure.
// The code snippet below illustrates a simplistic raw implementation of a DHCP Discover packet using UDP in Python
import socket
from dhcp_messages import DhcpDiscover
sock = socket.socket(socket.AF_INET, socket.SOCK_DGRAM)
dhcp_discover = DhcpDiscover()
sock.sendto(bytes(dhcp_discover), ('', 67))
This python code sends a DHCP Discover packet to a broadcast address using UDP. It succinctly showcases how swift and simple it is to implement DHCP through UDP.
UDP Vs TCP: Relevance to DHCP Server and Client Transmissions
When establishing a communication stream between two devices across a network, Transmission Control Protocol (TCP) and User Datagram Protocol (UDP) are the two primary options available. Despite their similarities, these protocols have critical differences that make them more optimal for varying situations and thus provide a clear answer for why DHCP server and client transmissions favor UDP over TCP.
TCP:
TCP is a connection-oriented protocol. It ensures that files or messages are broken down into small packets and accurately delivered from source to destination. TCP features error checking and recovery as well as sequencing to ensure data completeness, and it will retransmit missing or corrupted pieces. While these features contribute to data integrity, they also come with added overhead, meaning potential increases in latency and processing time.
xxxxxxxxxx
TCP Example (Python):
import socket
s = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
s.connect((HOST, PORT))
s.sendall(b'Hello, world')
data = s.recv(1024)
s.close()
UDP:
Conversely, UDP is a connection-less protocol. Unlike TCP, UDP doesn’t include the same protocol mechanisms for guaranteeing reliability, order, and data integrity. It sends packets without notifying or waiting for an acknowledgement from the recipient, also known as ‘fire-and-forget’. This means it requires less overhead than TCP, resulting in faster transmission times.
xxxxxxxxxx
UDP Example (Python):
import socket
s = socket.socket(socket.AF_INET, socket.SOCK_DGRAM)
s.sendto(MESSAGE, (UDP_IP, UDP_PORT))
It’s important to note this does not make UDP inherently ‘worse’ than TCP. Rather, it makes UDP better suited for specific scenarios where speed is crucial, and packet loss doesn’t critically affect the operation – which is why it is favored for DHCP server-client transmissions.
Focusing on DHCP Server-Client Communications: Why Choose UDP?
DHCP (Dynamic Host Configuration Protocol) plays a vital role in networks by dynamically assigning IP addresses to hosts as they join the network. Under the hood, this happens over four essential stages, often called DORA process: Discovery, Offer, Request, and Acknowledgement.
Upon examination of these steps, it becomes evident why UDP is the preferred choice:
- Rapid Transmission: As addressed earlier, UDP generally allows for faster data transmission due to its lower overhead and lack of acknowledgement-back-and-forth. For DHCP, reacting quickly to new requests ensures smooth operations and quicker connections.
- Broadcast Ability: A key functionality of DHCP is the capability to broadcast discovery and offer messages to multiple machines at once. TCP is designed for one-on-one communication, making UDP more efficient for this multi-cast scenario.
- Stateless Operation: Another aspect of DHCP is that it is stateless, i.e., DHCP servers don’t need “memory” of previous interactions. A stateless protocol such as UDP aligns better with this functionality compared to the connection-oriented nature of TCP.
To conclude, while both TCP and UDP serve crucial roles in networking, the inherent characteristics of DHCP mirror those of UDP, making UDP the optimal option for DHCP server-client transactions.In the world of Internet protocols, two major heavyweights are TCP (Transmission Control Protocol) and UDP (User Datagram Protocol). Both serve as communication protocol deployment tools for web-based applications to transport data across networks, more specifically internet.
A point to remember immediately is that TCP and UDP have their unique characteristics which justify their particular usage scenarios. While HTTP (Hypertext Transfer Protocol), SMTP (Simple Mail Transfer Protocol), FTP (File Transfer Protocol) primarily rely on TCP’s reliable and ordered data delivery, DNS (Domain Name System) and DHCP (Dynamic Host Configuration Protocol) bridal rides with UDP with their penchant for lightweight data transmission.
Firstly, let’s take a quick delve into the characteristics of these protocols. TCP, being connection-oriented, demands confirmation for every packet sent at each step. This means evident overheads, but it also offers predictability since the number of transmitted packets equals the number of acknowledged ones. On the other side of the spectrum, UDP, a connection-less protocol, performs no such verification. This concept may connote to unreliability due to un-accountability, but it’s beneficial in many time-sensitive situations where speed prevails over complete reliability.
It becomes evident from this understanding why a DHCP server and a DHCP client use UDP rather than TCP for their transmissions.
Primarily, DHCP operates using a “Fire-and-Forget” model – i.e., send out requests or replies and not bother about whether they reached their destination. The design rationale here orbits around simplicity and efficiency. There’s no need for session initiation and termination, saving network resources in chasing after protocol integrity.
Indeed, initial DHCP Discover and Offer messages are broadcasted. Broadcast messages would not mesh well with TCP, primarily given its connection-centric nature. Using TCP would be akin to ‘shooting a fly with a bazooka’ – an overkill that potentially consumes more resources extending the delay.
Speaking of delays, in highly congested networks, TCP’s aggressive retransmissions can actually exacerbate clean bandwidth further reducing efficiency. Using UDP allows for more balanced use of network infrastructure.
Below is a rudimentary example how a DHCP transaction looks like:
xxxxxxxxxx
DHCP Client -> DHCP Server : DHCP DISCOVER
DHCP Server -> DHCP Client : DHCP OFFER
DHCP Client -> DHCP Server : DHCP REQUEST
DHCP Server -> DHCP Client : DHCP ACK
As seen from above, there’s minimal protocol complexity, thus enhancing operational efficiency.
Finalizing, while TCP and UDP both carry significant roles in data transmission across the internet, their selective employment is heavily influenced by operations’ complexities and demands. In the DHCP’s case, the simplistic, efficient, and transient nature of its operations coincides with UDP’s design philosophy making it a perfect fit for handling DHCP transmissions.Understanding the choice of the User Datagram Protocol (UDP) in the process of DHCP communications necessitates a deeper look into its operational principles. UDP is one of the core protocols within the Internet protocol suite, designed to send messages known as datagrams over an IP network. It offers connectionless and limited error-checking mechanisms, making it faster and more scalable than the TCP protocol.
For the Dynamic Host Configuration Protocol (DHCP), which operates on a server/client model, UDP is a more suitable choice due to several reasons:
Connection establishment timeliness
TCP requires a three-way handshake for establishing connections: SYN, SYN-ACK, and ACK. It means that before any data is transferred between the DHCP server and client, there must be a successful establishment of the TCP connection.
xxxxxxxxxx
Client ------- SYN -------> Server
Client <---- SYN-ACK ------ Server
Client ------- ACK -------> Server
On the contrary, the DHCP process does not need to establish a connection before the start of communication. A DHCP client broadcasts a request to all devices within the same subnet without knowledge of the available servers. Thus, waiting for a fully set up TCP connection can result in unnecessary time loss. The UDP avoids such time-consuming process by serving in a fire-and-forget mode. The presence or absence of a dedicated receiver is not a concern.
Scalability
The ephemeral nature of communications in the DHCP process requires a protocol that can handle this efficiently. While connected to each client, the TCP needs resources to store the state of the connections that may demand excessive memory in heavy traffic situations, thus limiting its scalability. UDP, being a connectionless protocol, doesn’t keep track of individual clients, hence maintaining optimal resource usage even in high traffic conditions.
Error correction redundancy
Both TCP and DHCP have their error correction mechanisms—while TCP uses positive acknowledgments and re-transmissions; DHCP uses a direct retry mechanism from the application layer itself. Given that, employing TCP for transmissions would mean double error checks, resulting in inefficiency.
In many ways, the lightweight and faster UDP complements the design goals of DHCP—its efficiency, scalability, and broad network reach. Notably, DHCP requests are sent as broadcast frames which, practically, only UDP can support because broadcasting involves sending information to multiple receivers simultaneously, unsuitable for the connection-oriented TCP.
Given these factors, there are no surprises as to why DHCP designs opted for UDP despite the benefits the TCP holds in terms of reliability during transmission. For DHCP, UDP presents a solution tailored-fit to its conditions and requirements—proof that sometimes, less could indeed be more.
Code Example:
A simplified representation of how a DHCP client/server interaction looks can be as follows:
xxxxxxxxxx
Client ------- DHCPDISCOVER ------> Server
Client <------- DHCPOFFER --------- Server
Client ------- DHCPREQUEST ------> Server
Client <------- DHCPACK ----------- Server
Each interaction for requesting, offering, acquiring, and acknowledging the IP address is handled via UDP. These simple exchanges embody the minimal overhead and the quick and efficient distribution of IP addresses and other related configuration details characteristic of DHCP implementations.When dealing with networking communication protocols, standing out are the Dynamic Host Configuration Protocol (DHCP) and the User Datagram Protocol (UDP). Both play crucial roles in establishing internet connections, yet they operate based on different principles. The DHCP server and its clients heavily rely on UDP for transmission because it provides benefits fitting to their functionality.
Essentially, DHCP is a network management protocol used for automating the process of configuring devices on IP networks. It enables your device to obtain a valid IP address and other configuration information from a DHCP server.
Here's a simplified version of how DHCP works:
xxxxxxxxxx
1. DHCP Discover: When a device (newly connected to the network) needs IP configuration, it broadcasts a "DHCP Discover" message on the network.
2. DHCP Offer: The DHCP server responds by sending a "DHCP Offer", proposing an available IP address and extra related parameters.
3. DHCP Request: If the client accepts this offer, it sends back a "DHCP Request" as an acknowledgment.
4. DHCP Ack: Finally, the DHCP server sends "DHCP Ack" including lease time and other configuration details.
These messages are aggregated into UDP datagrams before transmission over the network.
While TCP guarantees delivery of packets and maintains session connections, it comes with higher overheads due to this reliable service, which includes acknowledgement packets, sequencing, and checksums. Conversely, the simple structure of UDP, which doesn't need setup or termination of sessions, makes it more lightweight and faster. Therefore, UDP provides an efficient choice for DHCP as it involves simple request-response communication without the need for persistence between the client and server.
Protocol | Description |
---|---|
TCP |
Gurantees packet delivery but may incur high overhead. |
UDP |
No connection setup or teardown, faster, and efficent for request-response communications like DHCP. |
Moreover, using TCP would pose issues for DHCP operations, notably during the "DHCP Discover” message since the client doesn't have a valid IP address at this stage. Since TCP requires IP addresses of both parties for establishing a connection, it wouldn't be useful in this scenario.
Also, using UDP allows DHCP servers to work seamlessly in broadcast and multicast environments that are common in various network infrastructure setups.
Consequently, the characteristics of UDP align well with those of the DHCP server and client processes, making it the more preferred approach over TCP.
For further reading, you might want to check RFC 2131 detailing DHCP protocol and its workings, and RFC 768 explaining the User Datagram Protocol (UDP).Alright, the Dynamic Host Configuration Protocol (DHCP) extensively uses UDP or User Datagram Protocol over TCP which stands for Transmission Control Protocol predominantly due to efficiency reasons. Although TCP's ability to provide reliability is commendable, its usage with DHCP can lead to unnecessary complexities and inefficiencies.
Firstly, let's discuss a little about how DHCP works before diving into why does a DHCP server and client prefer UDP over TCP for transmissions:
DHCP operates on a client-server model, helping computer systems to automatically request and receive an IP address from a network server. This eliminates the need for manual configuration of IP addresses for each device on the network.
To elaborate on this process:
- The client sends DHCPDISCOVER message to locate a DHCP server.
- Upon receiving, the server responds back with a DHCPOFFER message, extending an available IP address lease offer.
- Post getting the offer, the client requests for the IP address using DHCPREQUEST message.
- Finally, the server acknowledges this request through DHCPACK message, confirming that the client can use the allocated IP address.
Now, allow me to articulate the reasons behind the utilization of UDP for these various message types in DHCP transmission mechanics:
- Lesser overhead: TCP includes substantial overhead because it establishes a 3-way handshake and guarantees packet delivery, which could add notable traffic to the network. UDP, being a connectionless protocol, bypasses the whole session creation process.
xxxxxxxxxx
<p>\\ TCP 3-way handshake: SYN, SYN-ACK, ACK \\ UDP: No handshake.</p>
- Efficiency: As TCP ensures reliable data delivery through retransmission of lost packets, it may not be efficient for DHCP. Many a time, the retransmission results in duplicate IP address allocation as the server might fail to recognize its own retransmissions, leading to confusion and potential network conflicts.
- Broadcast Ability: UDP supports broadcasting wherein the same message can be sent to every system in a network segment whereas TCP only supports unicast which is one-to-one data transfer.
xxxxxxxxxx
<p>\\ UDP: DHCPDISCOVER, DHCPOFFER, DHCPREQUEST, DHCPACK \\ TCP: Limited to Unicast.</p>
Cumulatively, although TCP's added features ensure data integrity and security, they are not necessary for DHCP operations. In contrast, choosing UDP for DHCP allows for increased speed, reduced network complexity, and enhanced broadcast capabilities. All these factors collude to make UDP a more favorable choice than TCP for DHCP transmission mechanics.
You can find detailed documentation regarding how the interaction between DHCP and UDP works on [RFC2131](https://tools.ietf.org/html/rfc2131), where all the technical terms discussed here are elaborated upon.When disseminating information about internet protocols, it's vital to understand the fundamental distinctions between them and how they're used. In the case of Dynamic Host Configuration Protocol (DHCP) and User Datagram Protocol (UDP), their alliance is not merely coincidental - it serves key purposes in computational communications.
By design, DHCP servers and clients utilise UDP for information transmission rather than Transmission Control Protocol (TCP). This choice isn't arbitrary; there are several reasons why UDP is preferred:
Ease of Implementation
Implementing UDP is simpler compared to TCP because UDP is a connectionless protocol. There's no requirement for handshaking before communication starts, making the process swifter and less resource-intensive. The lesser complexity thus translates into economic use of resources on both the server and client ends. A typical interaction would be like this:
xxxxxxxxxx
client -- DHCP Discover -->
Server
<------ DHCP Offer --------
client -- DHCP Request --->
Server
<------- DHCP Ack ----------
Also, since DHCP messages are relatively small, using a lighter-weight protocol like UDP is fitting.
Flexibility in Broadcasting
One of the most substantial advantages of using UDP over TCP is its capacity to support broadcasting. With DHCP, when newly configured or rebooted hosts join the network, they need an IP address but may lack specific information such as the DHCP server's IP address.
Here, UDP's power of supporting broadcast comes in handy because the host can send a DHCP request as a broadcast message, ensuring all devices on the network receive it, including the DHCP server. Broadcasting is a feature that TCP does not provide, which becomes a determining factor in choosing UDP for DHCP.
Multicast Support
UDP goes beyond just broadcasting to supporting multicasting as well which is directing a single datagram towards multiple recipients. This functionality aligns well with the dynamic nature of DHCP, optimising communication between the server and the client(s).
Applying our understanding from above, we can represent DHCP transmissions using UDP through the following table:
Transmission | Type | Description |
DHCP Discover | Broadcast | The client sends a broadcast message searching for a DHCP server. |
DHCP Offer | Unicast | The server responds with a unicast offer detailing available IP information. |
DHCP Request | Broadcast/Unicast | The client either broadcasts its acceptance to all nodes or unicasts it directly to the offering DHCP server. |
DHCP Acknowledgement | Unicast | The server finalizes the transaction by acknowledging the client's request. |
The intricacies of using UDP over TCP in DHCP exhibits not only a fundamental comprehension of protocol implementation but also guides designers and tech professionals in developing effective networking architectures. Detailed discussions about this topic can even be found in resources like this RFC2131-DHCP.In the realm of internet protocols, the incidence of Transmission Control Protocol (TCP) and User Datagram Protocol (UDP) taking respective roles for specific web operations is not uncommon. However, when it comes to Dynamic Host Configuration Protocol (DHCP), there is indeed a pertinent question we must address: Why does a DHCP server and client make use of UDP rather than TCP for transmissions?
Firstly, it's important to grasp what exactly DHCP does. In essence, it allows computers on a network to get their configuration parameters like IP addresses dynamically from a server. This occurs without needing manual configuration by a network administrator. A key detail here is that this transaction between the server and client has a lightweight, ephemeral nature.
Now, in case of using UDP over TCP for DHCP transmissions, there are a couple of critical reasons:
- Connectionless protocol: The most noteworthy advantage of UDP is its "connectionless" model. Unlike TCP which requires establishing a connection before data transfer begins, UDP sends packets without pre-establishing connections. This plays out well for DHCP transactions, wherein clients and servers need to communicate swiftly without investing time in setting up a connection. Using UDP significantly reduces overall network latency.
xxxxxxxxxx
UDP Datagram format:
| Source port | Destination port |
| Length | Checksum |
| Data.....
- Broadcast capability: When a new device triggers a DHCP request, it can only send a broadcast packet since it does not know the DHCP server's IP address. Limited by design, TCP does not accommodate broadcast or multicast. This is where UDP shines as it naturally supports broadcasting, meeting an essential requirement in the context of DHCP.
Despite these compelling reasons for employing UDP in DHCP transactions, one might still question if there is any role TCP can play here at all?
Technically, whatever UDP can do, TCP can do as well - including the job of delivering DHCP traffic. Yes, sure, TCP would have to deal with its overheads originating from connection establishment, error recovery, etc. But if that were not a concern, for instance, in smaller networks where transmission reliability is favored over performance; one might want to consider TCP.
However, the value in using TCP for DHCP transactions tends to diminish when you factor in the reality of a busy network, full of devices continuously connecting/disconnecting. Keeping track of all active TCP connections on a DHCP server can lead to more complex, resource-intensive orchestration — arguably an unnecessary strain considering how DHCP was intended to work.
That said, no formal implementation of TCP exists in any part of a DHCP transaction. Though, other components used alongside DHCP may tap into TCP's reliability for specific tasks such as Active Directory replication, DNS updates, etc.
So, while TCP's reliability makes it ideal for applications that require all data to arrive without issues, such as emails or webpages, UDP turns out to be more suitable for services like video streaming, VoIP, or indeed DHCP, where speed takes precedence over perfect delivery, and loss of some data does not significantly degrade performance. Hence, in general practice and design, DHCP sticks with UDP for its process.
Sources:
RFC 2131 – Dynamic Host Configuration Protocol
UDP - WikipediaIn a client-server model, the transmission of packets is crucial to understand as it is the fundamental basis upon which data moves from one point to another in a network. A notable example is how the Dynamic Host Configuration Protocol (DHCP) server and clients interact. Notably, this process utilizes User Datagram Protocol (UDP), rather than Transmission Control Protocol (TCP), with several relevant reasons for this preference.
On UDP, DHCP chooses to transmit its data for several key reasons:
Efficiency
The first reason revolves around efficiency. Both UDP and TCP are transport protocols that are used for sending bits of data — known as packets — over the Internet. However, they do it differently.
While TCP ensures the delivery of the sent packets by establishing a connection between sender and receiver before transmitting data, and also validating that each packet reached its intended destination, UDP, on the other hand, sends the data without any of these confirmations. It simply blasts out the data to the recipient(s). This lack of handshaking and validation makes UDP protocol more efficient, speedier, and perfectly suitable for services like DHCP where speed, more than reliability, is paramount.
Broadcasting Capability
Another reason is based on the architecture of DHCP itself. A DHCP client doesn't have an IP address when communicating with a DHCP server to request one. This is effectively done using broadcasts, something that TCP does not offer. UDP supports broadcasting whereas TCP does not, so when a client initiates a request, it does so via UDP broadcast. The UDP protocol enables the DHCP server to send a "mass message" to all devices within a certain network, something that would not be feasible with TCP because of its inherent nature of establishing point-to-point connections.
Lower protocol overhead
Lastly, UDP has less overhead compared to TCP, making it faster and more scalable. With TCP there are additional steps involved in the form of back-and-forths necessary to establish and terminate a connection. Not having to deal with these overheads lowers the latency times associated with communication and once again underscores an important usability factor in situations where a rapid response may be crucial.
A simple representation of the UDP and TCP headers can show us the difference in complexity. Here's a basic HTML table illustrating this:
UDP Header | TCP Header |
---|---|
xxxxxxxxxx 1 5 1 Source port (16 bits) 2 Destination port (16 bits) 3 Length (16 bits) 4 Checksum (16 bits) 5 |
xxxxxxxxxx 1 13 13 1 Source port (16 bits) 2 Destination port (16 bits) 3 Sequence number (32 bits) 4 Acknowledgment number (32 bits) 5 Data offset (4 bits) 6 Reserved (6 bits) 7 Flags (6 bits) 8 Window size (16 bits) 9 Checksum (16 bits) 10 Urgent pointer (16 bits) 11 Options (0-320 bits, divisible by 32) 12 Padding (variable) 13 |
Just from viewing the two simplicity stands out for the UDP header. As you can imagine, the simpler design leads to less processing for both the server and the client, making for speedier communication.
In conclusion, while both TCP and UDP play pivotal roles in modern communication networks, UDP offers distinct advantages in the case of DHCP server-client interactions - mainly due to its efficiency, capability to broadcast messages, and reduced overhead costs.
For a deeper dive into the workings of TCP, UDP, and their role in computer networks, some fantastic resources are available online such as 'Computer Networking: A Top-Down Approach' which provides a great conceptual understanding here .The Dynamic Host Configuration Protocol (DHCP) utilizes User Datagram Protocol (UDP) rather than the Transmission Control Protocol (TCP) for transmissions because:
- Overhead. TCP requires a three-way handshake (SYN, SYN-ACK, ACK), so if DHCP were to use TCP instead of UDP, it would introduce excessive overhead and complexity.
html
xxxxxxxxxx
CLIENT ----SYN----> SERVER
CLIENT <--SYN-ACK-- SERVER
CLIENT ----ACK----> SERVER