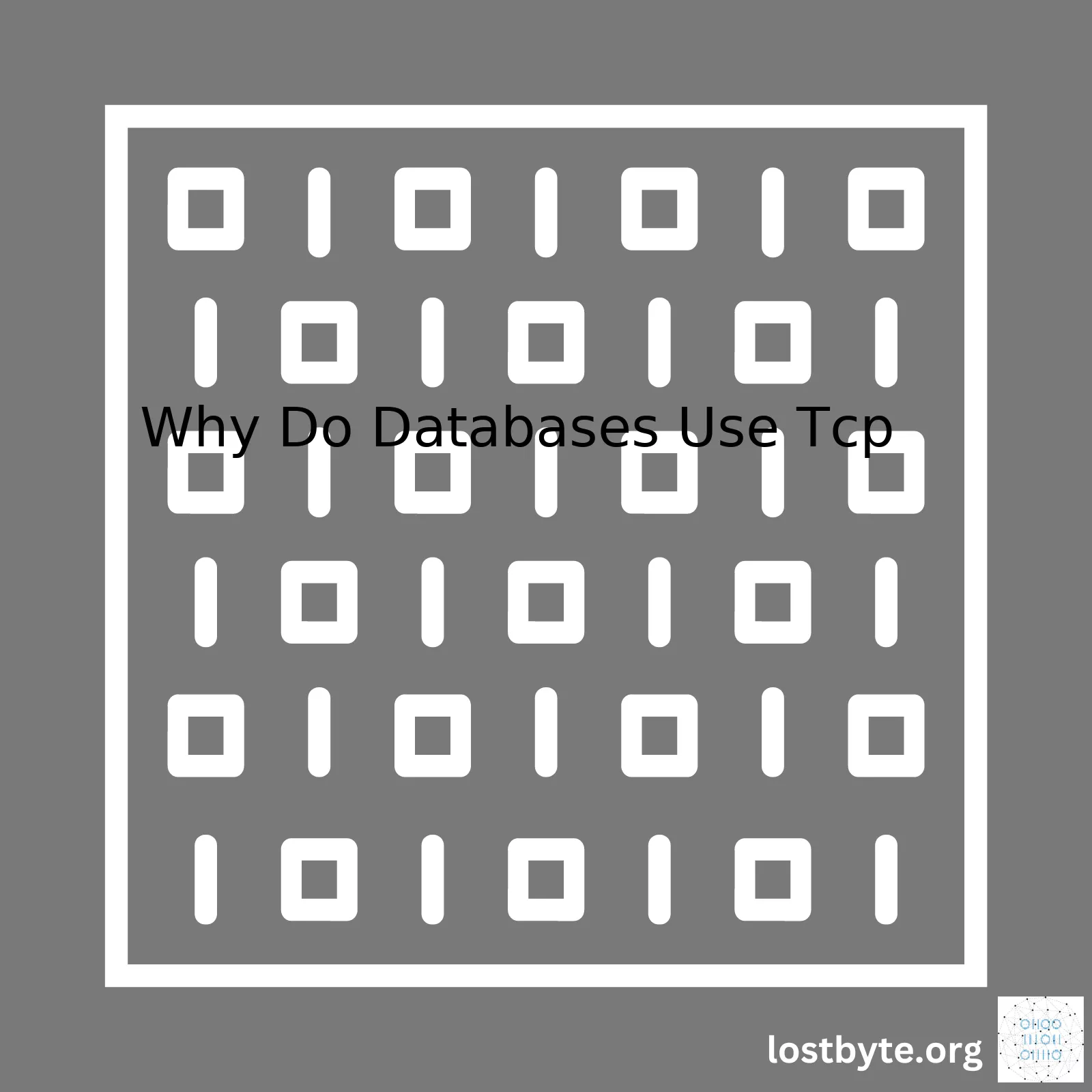
Characteristics | Description |
---|---|
Reliability | TCP ensures that all data packets are received correctly, which is crucial for databases where accuracy of information is paramount. |
Ordered data transmission | TCP ensures data packets are sent and received in the order they were transmitted. Therefore, a database’s structured data integrity is maintained. |
Error Checking | TCP possesses error checking capabilities on packets before transmission and upon receipt. For databases where preventing corrupt records is essential, this feature is most beneficial. |
Flow Control | TCP performs packet buffering by delaying their sending when network congestion is detected. This flow control mechanism prevents traffic overloading hence ensuring a smooth database operations across networks. |
Databases make use of Transmission Control Protocol (TCP) primarily due to its characteristics that guarantee data reliability and integrity during transmission. TCP is a connection-oriented protocol which means it establishes a reliable connection between the sender and receiver before actual data transmission begins. It uses various features like checksum-based error-checking mechanism, sequence numbers for ordered data transmission, acknowledgments, and retransmission of lost packets, all of which add to the overall trustworthiness of the transmitted data. In addition, TCP has components that detect network congestion and execute appropriate mechanisms such as slowing down the sending rate or buffering packets as part of its flow-control capabilities, thus, preventing potential network crashes. These are vital for database operations, especially across networks, where handling large chunks of data securely and accurately can significantly impact the performance and robustness of applications relying on these databases.
Here’s a simple example of establishing a TCP connection in Python using the socket module:
import socket # Create a TCP/IP socket sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM) server_address = ('localhost', 12345) print('Connecting to %s port %s' % server_address) # Connect the socket to the port specified above sock.connect(server_address)
This code snippet illustrates how TCP can be used to maintain reliable communications, ensuring your databases can successfully and safely communicate across the web. While there are other communication protocols available, the key role played by TCP in handling databases can’t be underestimated due to its strong orientation towards reliability and orderly communication.
For a deeper understanding of TCP, you can read more about it from the [Internet Engineering Task Force (IETF)](https://www.ietf.org/rfc/rfc793.txt) – the organization responsible for developing and promoting voluntary Internet standards.
When it comes to databases and their communication protocols, TCP or Transmission Control Protocol plays an indispensable role.
Let’s break this down and understand why TCP is used extensively in database communications.
Guaranteed Delivery:
Perhaps the most attractive feature of TCP as a communication protocol for databases is its reliable data transfer. TCP guarantees that all data packets sent will be received at the destination end, which is critical in a database environment where every bit of data is crucial.
TCP achieves this through an acknowledgment system. When the receiver receives a packet, it sends an acknowledgment signal back to the sender. If the sender doesn’t receive an acknowledgment after a certain time, it retransmits the packet. Here’s an example of how you might illustrate such a transaction:
Socket clientSocket = new Socket("hostname", 80); DataOutputStream outToServer = new DataOutputStream(clientSocket.getOutputStream()); outToServer.writeBytes("Data Packet" + '\n');
Ordered Delivery:
Another major attribute of TCP is its capability to deliver packets in the order they were sent. This is achieved by marking each byte with its sequence number before sending. At the receiving end, TCP reassembles the sequence using these numbers. This ensures that even if the packets take different paths, reaching at different times, the final data stream will still maintain its original order – A critical need when dealing with databases, where order can affect results dramatically.
Error Checking:
TCP uses a checksum algorithm to verify the integrity of the data. A checksum value is calculated for each data packet and sent along with the data. At the receiving end, the same calculation happens again and is compared with the sent checksum. An error only occurs if there are differences, ensuring that the data fetched from the database is error-free.
Flow control:
TCP manages data flow between sender and receiver using a mechanism called windowing. It prevents the sender from overwhelming the receiver by limiting the amount of unacknowledged information in transit. This aspect is particularly important when a small application or system is communicating with a large, high-performance database server.
A TCP handshake operation looks like this:
SYN-SENT --> SYN/ACK-RECEIVED SYN/ACK-SENT --> ACK-RECEIVED
Understanding this key role of TCP in supporting database communication means acknowledging its guaranteed and ordered delivery, error checking and flow control mechanisms that immensely aid in managing real-time database transactions.
For more information on TCP, check here. For deeper insights into Database Communication, feel free to look up Database Connection on Wikipedia.
Databases use TCP (Transmission Control Protocol) because of its reliability as a transport protocol. TCP ensures that all the data sent between the client and the server arrives accurately and uncorrupted. Unlike other protocols which do not guarantee safe delivery, TCP handles re-transmission if packets go missing or get corrupted in transit.
Here’s a breakdown of why Databases prefer using TCP:
Segmentation and Reassembly:
TCP partitions the data into smaller packets, known as ‘segments’, for efficient transmission over the internet. Once the destination device receives these segments, TCP reassembles them back into the original data format. This ensures that large datasets are seamlessly transmitted and reconstructed, which is an important feature for databases handling big sets of data.
TCP Segment{ src_port: 22, dst_port: 5432, seq_num: 2845, ack_num: 978, data_off: 7, control_bits: 'ACK', window: 5792, checksum: 4649, urgent_pointer: 0, options: [], data: [data bytes] }
Eradication of Errors and Packet loss:
TCP includes features like checksum fields for error checking and sequence numbers to handle packet reordering and loss. If a packet fails the error check or doesn’t arrive, TCP resends it, ensuring no loss of critical data. Such a feature limits the corruption of records within a database system.
Orderly Transmission:
In any networking system, packets can take different paths to reach their destination, and they may arrive out of order. Sequence numbers assigned by TCP help in reordering the segments in the right series at the receiver end. This allows the receipt, processing, and storage of data from databases in a consistent manner.
Flow Control:
TCP uses a sliding window mechanism (adjusts the data flow rate based on network conditions) to avoid overwhelming the receiver with data it cannot process fast enough. Thus, there’s a balance in communication speed, which prevents potential crashes in systems using the database.
It’s essential to recognize that TCP connections do carry some computational overhead. This is due to the constant setup, monitoring, and teardown of TCP connections. However, the reliability provided outweighs this cost, particularly when dealing with critical data managed in databases.
To illustrate, consider an instance where you connect to a PostgreSQL database through TCP. PostgreSQL will first create a listening socket on a specific TCP port using a socket() function. It subsequently binds the socket to the TCP port using bind() function, thereby accepting incoming connections.
Socket(sockfd, SOCK_STREAM,0); Bind(sockfd, (struct sockaddr *)&serv_addr, sizeof(serv_addr)); Listen(sockfd,5); Newsockfd = accept(sockfd, (struct sockadd r*)&cli_addr, &clilen);
Afterwards, your application establishes a connection, and data flows back-and-forth safely, maneuvered by TCP.
Therefore, databases use TCP mainly due to its reliable and organized data transportation features, offering the utmost integrity needed to handle sensitive and vast information chunks.TechTarget: Explanation of TCP.Understanding how TCP influences database performance requires an understanding of intricate technical relationships. Using a Transmission Control Protocol (TCP)-based protocol for database operations can significantly impact the overall performance. Databases opt to use TCP-based protocols given its reliability in transporting packets across distributed networks.
Firstly, consider the fact that databases are built to handle copious amounts of data with continuous reads and writes from various sources. While all this happens, it is critical to ensure smooth communication between the servers to avoid the risk of losing valuable information. This is where TCP comes into play.
TCP is established as a connection-oriented protocol. It confirms the proper delivery of packets from sender to receiver by establishing a connection before data transfer begins. When it comes to databases, this mechanism ensures that whatever processes are happening on one end – be it data queries, retrieval, or manipulation – they are securely transmitted to the receiving end.
Protocol | Description |
---|---|
TCP | Highly reliable protocol, ensuring data packets’ correct order and safe delivery. |
UDP | Speedy but less reliable than TCP. There’s no guarantee of data packet delivery or order. |
Along with this, there are other reasons why databases prefer TCP over other protocols such as User Datagram Protocol (UDP);
– Packet sequencing: With TCP, every byte of data has its unique sequence number. This means, if a couple of packets sent by a database get disarranged along the transmission path, TCP would rearrange them before passing them onto the application.
// Fictitious Example showing Packet Sequencing packet1.sequenceNumber = 1; packet2.sequenceNumber = 2; TCP.receive(packet2); // received first, but will wait until packet1 arrives. TCP.receive(packet1); // once arrived, both packets are delivered to the application in correct order.
– Error check and recovery: Moreover, TCP implements error-checking mechanisms, which audits packet integrity. Consider situations where server links are degraded or physically damaged. TCP’s error recovery feature ensures minimal data destruction. If a packet is damaged during transit, the receiver detects this and requests the sender to retransmit the specific packet.
– Congestion control: In large-scale databases, network congestion due to simultaneous multiple transactions could potentially slow down data transport. Luckily, TCP includes congestion control mechanisms to ensure optimal network usage.
All these factors significantly contribute to ensuring robustness and reliability when using databases connected via TCP.
However, there’s a cost for such reliability: Performance. The overheads of establishing connections, sequencing, error-checking, and congestion control can make TCP slower than simpler, connection-less protocols like UDP. Therefore, choosing between TCP or another protocol might depend on your priorities: Do you value surefire reliability above speed, or is raw speed more important even if it means occasional missed packets?
On balance, TCP offers a level of assurance about data delivery that is invaluable for maintaining database integrity. Regardless of some potential performance hits, using TCP remains beneficial for most scenarios involving databases.
Reference:
Transmission Control Protocol(TCP),
User Datagram Protocol (UDP)The Engagement of TCP in Database Security
The security concern in databases cannot be overlooked since databases hold crucial data and information. The Transmission Control Protocol (TCP) plays a significant role, which is why it is frequently used in securing databases.
1. The Reliability Factor
The long-standing use of TCP in database communication is made possible by its reliability. With TCP, we get a connection-oriented platform that uses sequence numbers to track packets of data. This feature in TCP ensures all sent data reach their destination without errors. Should an error occur; the sequence can be retransmitted. Here’s a sample Python script showing the basics of establishing a TCP connection:
import socket # Create a socket object s = socket.socket(socket.AF_INET, socket.SOCK_STREAM) # Connect to the server s.connect(('localhost', 8080)) # Send some data s.send('Hello, server!'.encode()) # Receive the response print(s.recv(1024).decode()) # Close the connection s.close()
2. Ensuring Data-Integrity
Following the database security model “CIA triad“, integrity ensures that data remains correct and unaltered during transmission. TCP supports this by providing error-checking mechanisms and validation using checksums. If there’s any alteration or corruption in the data packet, TCP detects it and requests a new packet.
3. Databases Synchronization
Most databases have to maintain consistency among various servers possibly present in different geographical locations. To keep all the replicated databases in sync, TCP provides reliable transfer capabilities with minimal packet loss. These aspects of TCP optimization ensure high performance, even over long, transcontinental links.
4. Secure Communication
For stronger security, specifically secure communication, TCP is often combined with the Secure Sockets Layer (SSL) or Transport Layer Security (TLS) protocols. When TCP is wrapped in SSL/TLS like in MySQL’s use of ‘secure TCP/IP connections‘, encrypted data transmissions between client and server are enabled to prevent sniffing attempts.
5. Technical Support
Due to its widespread usage, many technical support and libraries are available for TCP implementation with databases. This ease of access to robust support makes it more feasible and convenient for developers and database administrators to work with TCP.
Databases | TCP Implementation |
---|---|
MySQL | Includes support for TCP/IP sockets on any platform. |
MongoDB | Supports TCP/IP protocol for network traffic. |
PostgreSQL | Leverages TCP/IP for client-server communication. |
Conclusively, though TCP in itself is not complete protection for a database system, it forms a fundamental basis for building a secured environment with additional protocols and strategies in place. Therefore, ensuring proper TCP handling and settings is critical in the initial stage of setting up a secure, efficient database system. To dig deeper into this topic, consider referencing specialized texts such as “TCP/IP Illustrated” or specific database TCP handling documentation. References also include relevant software discussions at online forums and community sites for comprehensive insights into TCP handling in database systems.
Databases embrace the use of Transmission Control Protocol (TCP) as the underlying protocol to manage network communication for multiple reasons, that directly come from its functioning features and advantages particularly suited towards data transfers in databases. On the flip side, User Datagram Protocol (UDP), while having some unique strengths, has certain limitations when it comes to handling large scale dataset, which makes TCP a preferred choice in database systems.
Advantages of TCP in Database Systems:
- Guaranteed Delivery: TCP guarantees delivery of packets by acknowledging receipt of packets. This is essential in a database transaction scenario where the loss of any information can lead to transactional errors and integrity issues.
- Error checking facilities: TCP provides built-in error checking mechanisms which ensure that your data is transmitted correctly. In database transactions, error-checking is critical to maintain consistency of the stored data.
- Ordered Delivery: TCP assembles messages or packet streams into their proper sequence. In multi-user relational databases, order of operations is important. Thanks to TCP, operations arrive and execute in the correct order every time.
- Flow control: TCP’s flow control mechanism effectively ensures that a sender does not inundate a receiver with data that it might be slow to process, thereby preventing an unnecessary data overflow.
Database tcpReceiver = new Database(data -> System.out.println("Received: " + data)); tcpReceiver.startListening(TCP_PORT);
clientSocket.send("INSERT INTO USERS (ID,NAME,AGE) VALUES (1, 'Tom', 22)"); clientSocket.send("UPDATE USERS SET AGE = 23 WHERE NAME = 'Tom'");
Referring back to why many databases prefer TCP over UDP, these reasons typify the vital role of TCP in seamless network communications for databases. This is not to say that UDP doesn’t have its place in networking—it’s advantageous in real-time environments like video streaming where losing a packet here or there doesn’t necessarily spoil the user experience.
Limitations of UDP for Databases:
- No Guaranteed Delivery: UDP does not guarantee delivery because it does not acknowledge receipt of data packets. Over the internet, this rule applies even more stringently, making UDP unsuitable for data-driven applications like databases.
Database udpReceiver = new Database(data -> System.out.println("Received: " + data)); udpReceiver.startListening(UDP_PORT); // Might miss some data
- No Built-In Error Checking: Unlike TCP, UDP has no built-in error-checking mechanism. An error-checked transmission ensures data integrity, which is paramount for databases storing massive volumes of information.
- No Order of Delivery: With UDP, packets may not arrive in the same order they’re sent, potentially jeopardizing the logical execution of commands, especially in relational databases.
- Lack of Flow Control: UDP does not regulate the data flow between server and client, leading to a risk of overloading and thus data loss.
So although TCP might seem a heavier protocol than UDP because it includes a lot of extra functionality, those features such as guaranteed delivery, ordered execution, and error checking are exactly what make it a better choice for client-server communications for most types of databases.
References:
[https://www.speedguide.net/faq/what-is-the-difference-between-udp-and-tcp-internet-474](https://www.speedguide.net/faq/what-is-the-difference-between-udp-and-tcp-internet-474)
[https://www.guru99.com/tcp-ip-model.html](https://www.guru99.com/tcp-ip-model.html)
Databases make extensive use of Transmission Control Protocol (TCP), a protocol that facilitates the movement of packets from one system’s source to another system’s destination. The reason for the significant reliance on TCP by databases primarily boils down to its features and characteristics:
- Reliable data delivery: TCP ensures that your data reaches its destination without errors and in the same order in which it was transmitted.
- Ordered data transfer: In the event of blocks of data being sent out of sequence, TCP is capable of rearranging them into their proper order.
- Error-free delivery: Through error-checking mechanisms like checksums, TCP can ensure the integrity of data transmission.
- Flow control: By balancing pushing data and processing it, TCP prevents network congestion.
In terms of database operations, different TCP settings come into play and can tremendously impact performance and optimisation for task execution. Here are some notable TCP settings affecting database operations:
-
TCP_NODELAY
This disables Nagle’s algorithm, which was designed to reduce network congestion caused by “small” packets. Because database connections typically send frequent small packets, this delay can introduce latency issues. When disabled, packets will be sent immediately rather than waiting to form larger packets.
SET GLOBAL innodb_flush_log_at_trx_commit=2;
-
TCP_MAXSEG
This determines the maximum segment size and essentially speaks to how much data a TCP packet can hold. A smaller value may reduce transmission time at the cost of increasing overhead from additional packet headers.
# sysctl -w net.ipv4.tcp_max_syn_backlog=1024
-
TCP_KEEPIDLE
and
TCP_KEEPINTVL
The
TCP_KEEPIDLE
parameter impacts how long the system should wait before sending first keepalive probe following a period of idleness, whereas
TCP_KEEPINTVL
specifies the interval between subsequent probes if no acknowledgement is received. These settings can significantly impact how databases deal with inactive client connections.
# echo 600 > /proc/sys/net/ipv4/tcp_keepalive_time # echo 60 > /proc/sys/net/ipv4/tcp_keepalive_intvl
Mindfully tuning these TCP parameters based on your specific database workload, you could witness a dramatic difference in both speed and efficiency, helping to ensure smooth and optimized data retrieval and modification tasks while maintaining flawless service reliability.
For further reading, feel free to leverage resources such as TCP Specification (IETF RFC793), it provides more in-depth discussion around TCP protocol specifics.
SQL (Structured Query Language) databases make use of TCP (Transmission Control Protocol) primarily for its guaranteed data delivery, error-checking mechanisms, and the way in which it respectively orders data packets. Let’s delve deeper into why SQL databases leverage TCP for reliable data transfer.
1. Ensuring Reliable Data Transfer
The very essence of SQL databases – be it MySQL, Oracle, MS SQL, or PostgreSQL – dictates they need a reliable protocol that ensures every piece of data is accurately transferred from one end to another. This is because even a single lost or distorted data packet can lead to serious inconsistencies within a database.
While transferring data, the
TCP.send()
and
TCP.receive()
functions actively ensure all data is received intact at the receiver’s end. If any data packets are lost during the transmission process, TCP automatically retransmits these missing packets, ensuring data integrity and reliability.
Let’s have a look at sample Python code to send and receive data using TCP:
Sender Side | Receiver Side |
---|---|
import socket # Creating a socket s = socket.socket(socket.AF_INET, socket.SOCK_STREAM) # Connecting to server s.connect((host, port)) # Sending message s.sendall(b'Hello, Server') # Closing the connection s.close() |
import socket # Creating a socket s = socket.socket(socket.AF_INET, socket.SOCK_STREAM) # Binding to a port s.bind((host, port)) # Listening for connections s.listen(5) while True: # Accepting a connection c, addr = s.accept() # Receiving data print(c.recv(1024).decode('utf-8')) # Closing the connection c.close() |
2. Error Checking Mechanism
TCP plays an instrumental role not just in transporting data reliably, but also in identifying if the transmitted data has been compromised. This is facilitated by checksum, a built-in feature in TCP for error detection. Before initiating transmission, TCP constructs a segment header for each packet of data. This header includes a checksum, computed as per the contents of the packet. The receiver then recalculates this checksum based on the packet data. In case of discrepancy between the checksums, the packet is deemed corrupted and the sender is requested to resend the packet.
3. Data Ordering
Data ordering is vital when it comes to SQL databases. Imagine a scenario wherein a DELETE statement reaches the server before the UPDATE statement meant to precede it; significant discrepancies may occur in the database.
To circumvent such scenarios, TCP assigns sequence number to each packet sent over the network. When the data packets reach their destination, TCP reorders them based on these sequence numbers, ensuring that data is processed in the correct order.
The integration of TCP with SQL databases, as one can observe, is principally aimed at enhancing robustness, consistency, and coherence in data transactions. It makes TCP an indispensable protocol when stepping into the realm of SQL databases, contributing significantly to their efficacy and resilience.sourceDatabase systems utilize the Transmission Control Protocol (TCP) for several reasons, primarily due to its inherent features designed for reliable and ordered data delivery. TCP is a connection-oriented protocol, meaning that before any data can be transmitted, a connection must be established between the sender and receiver. The sender then transmits the data in sequence, and the receiver confirms receipt of each packet.
One particularly important feature of TCP, especially when it comes to databases, is congestion control. Dealing with traffic is strangely similar whether we’re discussing cars or data packets – too many in one place at one time can lead to slowdowns and collisions. With databases, which often need to handle large amounts of data across potentially multiple connections, controlling this digital “traffic” becomes crucial.source
But how does it work? Here’s where some key details about the TCP protocol come into play:
Firstly, it uses Receiver Window Size, a technique included in the header section of every segment that tells the sender how much data the receiver can accept at once (the capacity of the receiver). This prevents overloading the receiver with more data than it can process.
TCP Header { //other fields uint16_t Window; //Receiver Window Size }
Secondly, Slow Start process kicks in, allowing a TCP/IP network to determine the maximum amount of data that the receiver can manage effectively. It achieves this by beginning transmission at a relatively slow rate, then doubling it incrementally until it discovers the upper limit of the network’s capacity. Once that limit is reached, if all goes well, the sender will continue sending at that maximum discovered reciever rate without congesting the network.source
Lastly, an algorithm referred Painfully Congestion Detection and Avoidance (PCD), also contributes to traffic regulation in TCP. By actively decreasing the data-sending rate during times of high traffic and gradually increasing it during periods of low traffic, this safeguards the database from becoming overloaded and losing packets.
All these TCP capabilities play a pivotal role in ensuring proper communication between client applications and databases, keeping the data transfer smooth even under heavy load. These congestion control mechanisms not just ensure reliability of data transfers but also maintain the health and responsiveness of the entire network environment around the database. This is why database systems prefer using TCP.Databases primarily employ the Transmission Control Protocol (TCP) due to its reliability, message-oriented nature, error-checking functionality, among other features that make it a preferred option for databases and DBMS (Database Management Systems).
Let’s elucidate on these features.
– Reliability: TCP provides guaranteed data delivery. It confirms the receipt of transmitted packets by sending back an acknowledgment receipt to the sender, ensuring no packet of data is lost in the process.
// example of TCP connection ServerSocket serverSocket = new ServerSocket(6666); Socket socket = serverSocket.accept(); // establishes connection
– Message-Oriented Flow: TCP structures data into packets which get reassembled at their destination point. This process guarantees accurate translation and minimizes errors during transmission.
– Error Detection: Each TCP packet has a checksum, enabling detection of any corruption that may occur during transmission.
It’s crucial to note that the efficiency of a DBMS doesn’t entirely depend on TCP as there are also other critical elements like the database design, normalization, indexing, etc. But TCP is indeed quite influential in facilitating reliable interaction between servers and clients, thus enhancing the overall functioning of a DBMS.
Table: Key benefits of using TCP in DBMS
Feature | Benefit |
---|---|
Reliability | TCP guarantees data delivery, ensuring all parts of the application’s information reach their destination. |
Message-oriented flow | TCP ensures the correct assembly of data packets and their sequenced delivery. |
Error detection | TCP includes a mechanism to identify potentially corrupted portions of the sent data packets. |
To comprehend this more, RFC 793 – Transmission Control Protocol details the specifications of TCP beneficial to DBMS operations. Furthermore, you can read about the inner workings of different DBMSs and how they utilize TCP. For instance, MySQL’s documentation outlines how MySQL server and clients use TCP/IP for connection and communication.
Yes, there might be some minimal latency with TCP due to its process of establishing connections and confirming packet deliveries, but the peace of mind knowing that your data arrives intact overshadows this downside.
The impact analysis shows that employing TCP in DBMS contributes significantly to the latter’s effectiveness. Successful communications over a network necessitate a high level of certainty – something that TCP reliably provides. Thus, the role of TCP becomes particularly vital for databases amplifying the efficiency of the data exchange process.
As a coder who works with databases, acknowledging these nuances aids in constructing more efficient applications, as you consider not just what we store, but how it gets there too.
Database Replication and Synchronization
Databases make a crucial use of TCP – Transmission Control Protocol to facilitate reliable communication and uninterrupted data flow irrespective of the network’s state. When dealing with databases, replication and synchronization stand as central processes for data management and they highly rely on TCP.
Replication in database terms indicates the operation where the information is copied and synchronized from one database (source) to another database (target), ensuring that both hold the same set of information. Synchronization, on the other hand, refers to the process by which databases keep their data consistent with each other.
// Pseudo code example: database1.connect(TCP) database2.connect(TCP) data = database1.fetchData() database2.copyData(data)
This ensures both databases are consistent with each other. But why is TCP significant here?
- Reliability: TCP provides an acknowledgment mechanism that confirms whether a packet is received or not. In context of databases, this ensures no data loss during the transmission process between databases while performing replication or synchronization.
- In-order Delivery: Packets transmitted over a TCP connection are guaranteed to arrive in the same order they were sent. This property is vital especially when databases perform transactions that need to maintain ACID (Atomicity, Consistency, Isolation, Durability) properties.
- Error Detection: TCP contains a checksum field that allows it to detect any possible corruption. If the checksum value calculated at the receiving end doesn’t match with sent value, the packet can be resent – valuable for maintaining the integrity of the transmitted data.
- Flow Control: TCP dynamically adjusts its transmission rate based on network conditions, useful in preventing overwhelming the recipient or network infrastructure. For huge databases being replicated or synchronized, proper flow control is essential.
Highlighted above are reasons why databases favor TCP for processes like replication and synchronization, bringing about efficient and error-free data transfers. Choices might vary depending on use case-to-case basis, such as usage of UDP (User Datagram Protocol), that may be more suitable for speed-intensive applications but lack reliability compared to TCP.
The Transmission Control Protocol RFC extensively discusses how TCP works. Similarly, the PostgreSQL documentation talks extensively about database replication and synchronization procedures. Their combined reference offers a comprehensive guide for anyone looking to understand the interplay between TCP and databases.
The use of TCP (Transmission Control Protocol) by databases is critically essential for efficient, reliable and sequenced delivery of data packets between systems. Many reasons reinforce the extensive utilization of TCP by databases.
Reliability:
TCP promotes high reliability in data transmission. Unlike other protocols, it includes an error-checking mechanism that ensures every packet of data reaches its intended destination without any errors.
TCP Syn/Ack process: //Sender sends a Syn (Synchronize Packet) //Recipient responds with Syn Ack (Synchronize Acknowledge Packet) //Sender responds with an Ack (Acknowledge Packet)
The above code example represents the 3-way handshake process. It manages the connection establishment which verifies both sender and receiver are ready for data transmission, adding an extra layer of security and ensuring the integrity of data.
Ordered Segments:
Another pivotal reason is TCP’s ordered segments feature. The protocol sequences each data packet with a unique order identifier before sending it onto the network. This sequencing allows packets to reach their destinations safely, even when transmitted out of order due to network congestion or other factors.
//Packet Order Packet1 -> Packet2 -> Packet3 -> Packet4
Congestion Avoidance:
Congestion over a network can lead to the loss of data packets. TCP employs several techniques for congestion management, such as window size adjustment and slow-start algorithm. These procedures enable the servers to control the rate at which they send data, minimizing packet loss.
In essence, TCP’s superior reliability, managed sequence of data packets, and competent congestion avoidance make it an invaluable tool for databases. Moreover, the growing advancements in TCP, like Google’s BBR congestion control algorithm source, promise to further optimize its role in database operations. Remember, an uninterrupted communication pathway between client and server applications is crucial for database performance, and TCP fulfills this need brilliantly!