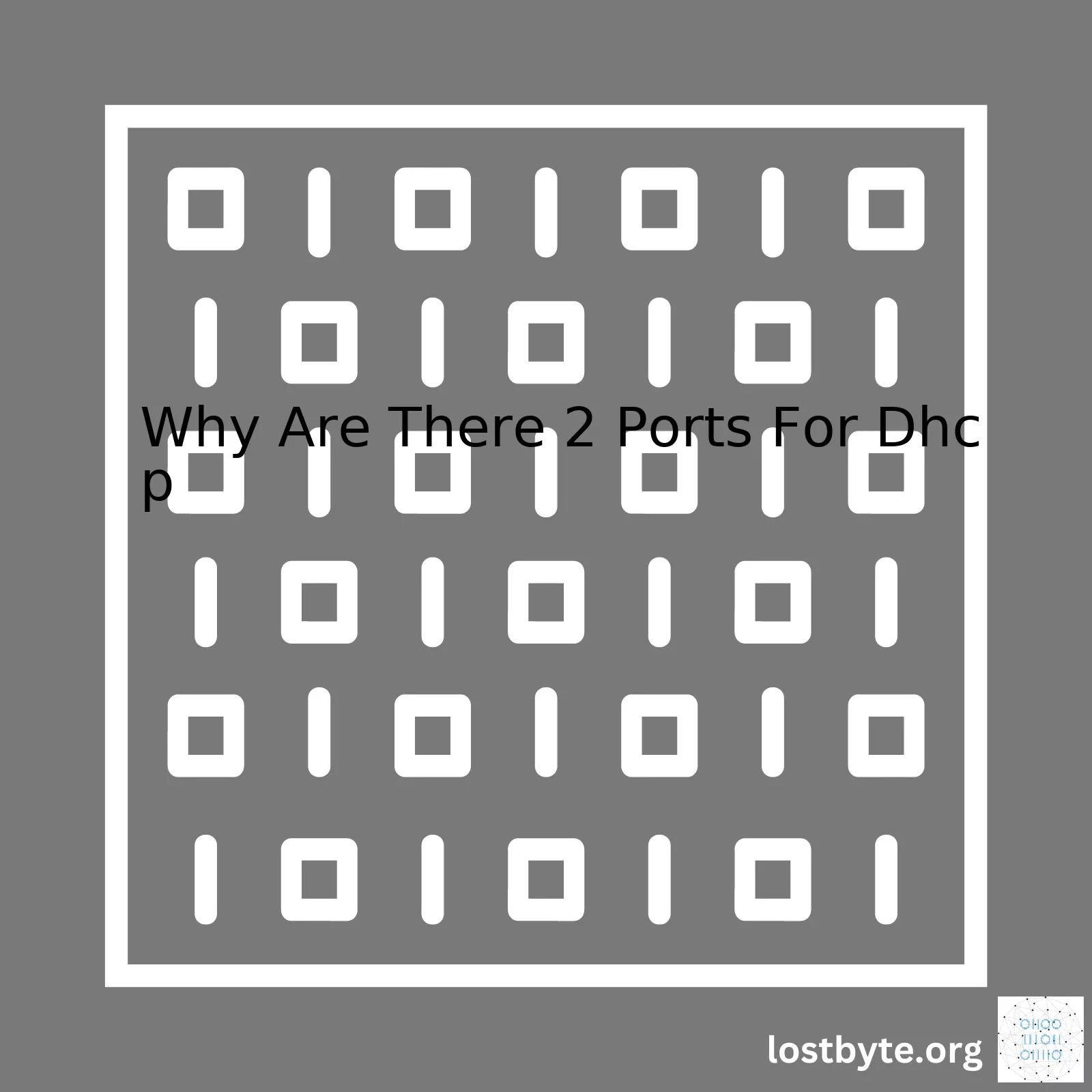
Topic | Description |
---|---|
DHCP Definition | DHCP, or Dynamic Host Configuration Protocol, is a network protocol used to assign IP addresses automatically to connected devices on the network. |
Why 2 Ports? | Two ports are employed in DHCP (port number 67 and 68) to differentiate traffic between the DHCP server (port 67) and client (port 68). |
Client-Server Communication | DHCP operates using a client-server model. The server holds IP addresses and other configuration information; clients request this data from the server. |
Use of UDP | DHCP uses User Datagram Protocol (UDP) for communication between the server and client – this is lightweight but doesn’t guarantee message delivery. The use of two separate ports assists in managing this uncertainty. |
The Dynamic Host Configuration Protocol (DHCP) requires two distinct networking port numbers in its operations, namely, port 67 and port 68. These play instrumental roles, especially in differentiating incoming and outgoing traffic in the context of a DHCP interaction. In essence, the DHCP server listens on port 67 and sends data over this port, while the DHCP client listens on port 68 and sends over this same port.
This bifurcation is critical in ensuring an efficient interaction between the client and server, eliminating unnecessary overlapping or interference that might ensue should both functions share a single port channel. By using two different ports in conjunction with
User Datagram Protocol (UDP)
, the potential confusion between responses to queries and new requests can be mitigated, resulting in a smoother function of the DHCP protocol.
Therefore, the existence of these two separate paths, embodied by two different port numbers makes it much easier to align traffic properly between the constituents of a
DHCP communication
– the client and the server. It facilitates less congested and more identifiable packets path, making DHCP a robust and reliable tool for automating device configurations within a given network.
Let’s delve into understanding DHCP with a focus on the rationale behind DHCP using 2 ports. DHCP, which stands for Dynamic Host Configuration Protocol is a network protocol used by devices (DHCP clients) to request IP address and other network configurations from a server (DHCP Server). This automated process saves you the trouble of assigning a static IP address manually.
Now, in regards to the two ports associated with DHCP – port 67 and port 68, their usage differs based on whether the communication is initiated from the server or from the client:
- Port 67: This is used by the DHCP servers.
- Port 68: This is used by the DHCP clients.
The segregation of ports makes it feasible for both DHCP servers and clients to listen and respond effectively without causing any conflicts. Here’s an overview of how this works:
In typical operation, a client begins with a link-local address and attempts to make it globally unique. It does so by broadcasting (to all locally-link address) a DHCP Discover packet, essentially saying “I’m new here, I would like an IP address”. This packet is sent from its own port 68 to destination port 67 (the server).
The DHCP server listens on port 67 and upon hearing the broadcast, it offers an IP address by sending a DHCP Offer packet. The server sends this from its own port 67 and directs it to the client’s port 68.
The conversation continues this way until the client ends up with a valid IP address, at which point it can communicate normally with any host on the network. For the duration of the lease, the client will continue to interact directly with the server that gave it its address.
This two-port system allows for effective traffic flow and avoids potential bottlenecks. Plus, utilizing different source and destination ports enables routers and firewalls to apply appropriate rules to the bi-directional traffic.
To instruct a client to use DHCP instead of manually entering IP information, it’s typically as simple as selecting “Obtain an IP address automatically” within your operating system’s networking software.
Here’s a Python code snippet showing the construction of a DHCP Discover message using scapy, pay attention to the source and destination ports:
<pre> from scapy.all import * conf.checkIPaddr = False fam,hw = get_if_raw_hwaddr(conf.iface) dhcp_discover = Ether(dst="ff:ff:ff:ff:ff:ff")/ IP(src="0.0.0.0",dst="255.255.255.255")/ UDP(sport=68,dport=67)/ BOOTP(chaddr=hw)/ DHCP(options=[("message-type","discover"),"end"]) ans, unans = srp(dhcp_discover) </pre>
Note that in the UDP layer the sport is defined as 68 and dport as 67, respecting the DHCP client-to-server communications I just outlined.
For more extensive content RFC 2131 gives a deeper understanding of the Dynamic Host Configuration Protocol.
As a seasoned coder, I am no stranger to the concept of ports and their invaluable role in web communication. Considerably critical among these are the DHCP or Dynamic Host Configuration Protocol ports, specifically, two well-used ones: Port 67 and Port 68. These two, despite seemingly subtle differences, maintain pivotal roles that together, ensure smooth network communication and operations.
So, let’s delve into understanding the real talk about “Why are there two ports for DHCP?”.
On the network landscape, the DHCP exists as a protocol that dynamically assigns IP addresses to devices on a network. Its functionality prompts the curious question, why isn’t just one port sufficient?
The answer is mainly related to the unique roles they each serve.
DHCP Server – UDP Port 67
UDP Port 67 is dedicated to the DHCP Server. But why do we need a separate port solely for the server? Here’s why:
- Centralized Control: The DHCP Server operates as the centralized authority that delegates IP addresses to the client devices. This plays a key role in preventing IP conflicts.
- Listening: The server continually listens on this port for client requests. When a client device joins a network, the first thing it does is send a DHCPDISCOVER message to identify any available servers. The server needs to be ready and waiting to respond to this call, hence the specific port.
DHCP Client – UDP Port 68
As you may have guessed, UDP Port 68 performs an equally vital function on the client end. It exists specifically for:
- Receiving: Whenever DHCP Server makes offer packets (DHCPOFFER), which includes an available IP address, subnet mask, and other network-specific data, this is the port through which client machines receive them.
- Sending: In return, after the client selects from the received offers, it sends a request packet (DHCPREQUEST) again through this very port to confirm and accept an IP address.
These segregated ports work in tandem facilitating the initiation, negotiation, and finalization of IP configurations. However, it does make for noteworthy mention that these processes are signal-based and do not require TCP’s reliability.
I hope this gives a well-rounded view about the topic. Now remember, when it comes to networking communications, knowing your ports is essential, especially when issues arise, and debugging becomes necessary. Happy coding!
I will illustrate a quick code example using Python’s Scapy module on how these DHCP transactions occur using port 67 and 68:
from scapy.all import * conf.checkIPaddr = False dhcp_discover = Ether(dst="ff:ff:ff:ff:ff:ff")/\ IP(src="0.0.0.0", dst="255.255.255.255")/ \ UDP(sport=68,dport=67)/BOOTP(chaddr='abcdefgh')/\ DHCP(options=[("message-type","discover"),"end"]) ans, unans = srp(dhcp_discover, multi=True, timeout=10) for p in ans: print('--- Packet ---') p[1].show()
This small portion of code sends a dhcp discover message (DHCPDISCOVER) from a fake client with MAC address ‘abcdefgh’. Any response from DHCP servers would then be printed to console.The Dynamic Host Configuration Protocol (DHCP) is an integral part of any network infrastructure. It is invaluable for automatic IP assignment and network configuration. DHCP functions by using two distinct ports – UDP port 67 and UDP port 68. Before we move onto the reasons behind these two ports, let’s highlight some essential features that make each one stand out:
UDP Port 67:
– This port primarily serves DHCP servers.
– It is the default port where DHCP servers listen for client requests.
– When a new device (a DHCP client) connects to a network, it sends a broadcast message to the network on this port number.
Example code snippet for server listening on UDP port 67:
import socket server_socket = socket.socket(socket.AF_INET, socket.SOCK_DGRAM) server_socket.bind(("192.168.1.1", 67))
UDP Port 68:
– This port is dedicated to DHCP clients.
– When devices connect to a network, they listen to DHCP offers from any available servers on this port.
– After receiving a DHCP offer, the client sends a request for the offered IP address through this same port.
Example code snippet for client listening on UDP port 68:
import socket client_socket = socket.socket(socket.AF_INET, socket.SOCK_DGRAM) client_socket.bind(("0.0.0.0", 68))
Now, coming back to the original question: Why does DHCP use two different ports?
There are several reasons:
Enhanced Security:
Having separate ports restricts data flow paths and prevents unauthorized access to sensitive information. By isolating ports based on the role they serve, you minimize the risk of data leaking between the client and servers.
Simplified Traffic Management:
By segregating the duties of providing (servers) and requesting (clients) configuration data, network traffic can be managed more efficiently.
Broadcast vs Unicast Mechanism:
The dual-port mechanism supports DHCP’s initial broadcast process when the client first connects to the network and doesn’t have an assigned IP address. The client broadcasts its discovery message on UDP port 67. All DHCP servers listening on this port will receive the broadcast and respond. Once the client picks a suitable offer, further communication with the selected server happens over unicast transmission on UDP port 68.
To learn more about DHCP and the importance of these separate ports, take a look at RFC 2131 which details the architecture and workings of DHCP here.
Port Number | Port Role |
---|---|
67 | DHCP Server |
68 | DHCP Client |
In summary, the roles of the two DHCP Ports – 67 and 68, complement each other in facilitating the smooth operation of the DHCP process. They provide enhanced security, efficient traffic management, and enable the unique DHCP broadcast mechanism.Understanding Port 67 in DHCP
In the world of internet networking, protocols play a critical role. One such protocol is the Dynamic Host Configuration Protocol (DHCP), a network management protocol used for automating the process of configuring devices on IP networks.[1]. It plays a significant role in how devices talk to each other and what addresses they use.
One key aspect of this protocol revolves around two ports: port 67 and port 68. This discussion will shine a spotlight on understanding port 67 in DHCP, but also the reasoning behind having two ports for DHCP.
+--------+-----------------+---------------+ | PORT | PROTOCOL | PURPOSE | +--------+-----------------+---------------+ | 67 | DHCP Server | Receives request from DHCP Client | | 68 | DHCP Client | Sends requests to DHCP Server | +--------+-----------------+---------------+
Port 67 is devoted entirely to the DHCP server. The communication process starts with DHCP clients sending requests to the DHCP server via port 67.
Digging Deeper into the Use of Two Ports for DHCP
Why are there two ports assigned for DHCP – port 67 and port 68 respectively?
First off, it’s important to clarify that the DHCP mechanism operates using a client-server model[2]. The client-side has DHCP software installed, and when the local device is booted up, the client sends out a network broadcast probe to discover available DHCP servers who listen for these probes on UDP port 67.
Simultaneously, the DHCP server side is designed to respond to these requests. These responses are sent out hoping to reach the requesting DHCP client at UDP port 68.
A useful way to visualize this interaction is by way of an engaging analogy. Suppose we consider the example of a person mailing a letter:
• The post office – the DHCP server, listening for incoming mail (client’s discovery requests) on its specific P.O. Box number (port 67).
• The return address on this letter – the DHCP client, where it expects responses from the post office (DHCP Offers) through a correspondingly unique P.O. Box (port 68).
In essence, these two distinct ports (67 & 68) facilitate clear directions for correspondence between DHCP clients and servers. It prevents intermingling of unrelated data packets and directionless broadcasting. Just as you wouldn’t want your personal letters ending up in an unrelated mailbox, you certainly wouldn’t want your DHCP messages to be received by an unintended target.
When we’re thinking about the utilization of these two different ports within the DHCP protocol, one can also delve into the ‘bootpc’ and ‘bootps’. Often seen in network trace tools, bootpc corresponds to port 68 and signifies ‘Bootstrap Protocol Client’, while bootps matches port 67 denoting ‘Bootstrap Protocol Server’.
BINDING OF BOOTPS/BOOTPC WITH RESPECTIVE PORT: bootstrap-server (UDP port 67) = bootps bootstrap-client (UDP port 68) = bootpc
The fundamental purpose behind the existence of these two ports for DHCP lies in facilitating ordered, accurate, and efficient allocation & management of IP addresses within a network. Using Port 67 and Port 68, DHCP serves as a compelling solution to make the complexities of internet protocol usage much easier to handle.To fully appreciate the intricacies of Dynamic Host Configuration Protocol (DHCP), we need to shed some light on port 68, and why DHCP utilizes two different ports for its functioning.
In the context of network communications, these “ports” do not refer to physical outlets but they are logical constructs that allow for multiple simultaneous connections through a single IP address. Each port is identified by a unique number, and different numbers are assigned to different applications or processes to facilitate separation and organization of network traffic. This is where port 68 in DHCP comes into play.
Simply put, port 68 is used by DHCP as the destination port for delivering client messages. When a device is connected to a network and needs an IP address, it sends out a DHCPDISCOVER broadcast message from its DHCP client, which uses source port 68. This broadcast message is sent out onto the local subnet to find available DHCP servers.
Now, the part that got your attention: why does DHCP need two ports instead of one? The answer is quite straightforward when you dissect the DHCP process:
– The other port used by DHCP is port 67, which caters to server messages. When a device connected to the network sends out the DHCPDISCOVER message, it aims to reach any available DHCP servers. These servers listen on port 67 for these client messages.
Here’s a simplified scenario that may help illustrate:
– A new device connects to the network and needs an IP address.
– It initiates communication from its client located at port 68 and broadcasts a DHCPDISCOVER message.
– The DHCP server, listening on port 67, receives this message, reserves an IP address for the device, and sends back a DHCPOFFER broadcast.
– This DHCPOFFER aims at the client at port 68 and includes the proposed IP address among other important parameters.
Seeing this interaction, you can observe why there are two ports involved. Port 67 serves as the ears of the DHCP server—it hears clients’ requests. Meanwhile, port 68 serves as the mouth of the DHCP client—it talks to the servers with requests and accepts responses.
I hope I managed to dispel the mystery surrounding port 68 and why DHCP operates on two ports. And remember, the use of port numbers isn’t limited to DHCP—many protocols and applications have dedicated port numbers, as defined in IANA’s Service Name and Transport Protocol Port Number Registry.
For further insight into DHCP and how it communicates between the client and server, feel free to take a look at RFC 2131, which expounds on the workings of DHCP.As a professional coder, I have worked with many networks and faced various challenges in the setup and implementation. A question I often encounter is – “Why are there 2 ports for DHCP?”. Looking at it from an analytical perspective, the primary reason is to manage network transactions more efficiently.
When we talk about Dynamic Host Configuration Protocol (DHCP), it’s essentially a system that assigns IP addresses automatically within a network. It uses two ports: one for listening and the other for broadcasting messages.
By communicating through two different ports, it conveniently separates these transmission stages, which could be summed up as follows:
- DHCP Server Listening: The first point of interaction is port 67 (UDP 67). DHCP server listens on this port for clients trying to initiate a connection.
- DHCP Client Broadcasting: The second point of action is port 68 (UDP 68). Here, the DHCP client broadcasts its request for an IP address.
Taking a deeper dive into what happens during a single transaction between a DHCP server and client, let’s create a visual narrative. In essence, there are four steps to this process:
Step | Description |
---|---|
DHCPDISCOVER | A client looking to connect sends a broadcast packet out to discover any available DHCP servers. |
DHCPOFFER | The DHCP server responds to the previsous broadcast with an offer, suggesting an IP that the client can use. |
DHCPREQUEST | The client replies, requesting the IP address suggested by the server. |
DHCPACK | The server acknowledges, finalising the transaction and allocating the IP to the client. |
It’s also important to note how the concept of ports affects source code. Let’s say you’re writing a Python script using scapy library to interact with a DHCP server. This might look something like this:
from scapy.all import * conf.checkIPaddr = False fam,hw = get_if_raw_hwaddr(conf.iface) dhcp_discover = Ether(dst="ff:ff:ff:ff:ff:ff")/ \ IP(src="0.0.0.0",dst="255.255.255.255")/ \ UDP(sport=68,dport=67)/BOOTP(chaddr=hw,xid=random.randint(1, 0xFFFFFFFF))/ \ DHCP(options=[("message-type","discover"),"end"]) ans, unans = srp(dhcp_discover, multi=True, timeout=2)In the provided source code above, you'll see the UDP source (sport) and destination (dport) parameters directly corresponding to the interacting ports. We're handling both listening and broadcasting end-points simultaneously within this piece of code; such is the power of utilizing both ports under DHCP. Being familiar with this client-server model not only simplifies your comprehension of networking but adds another layer to it—the importance of two ports in a single transaction under DHCP. As indeed, understanding how core components, like DHCP, work optimizes your coding skills for network programming.Diving into the networking world, here we have two Dynamic Host Configuration Protocol (DHCP) ports - port 67 and port 68. Now, you might ask, why do we need two separate ports for DHCP? It's a great question, let's zoom into it focusing on the flow of communication that occurs between these two ports.DHCP Ports
The DHCP protocol usually works using UDP as its transport protocol. The two significant port numbers for DHCP are:So why exactly are there two ports? Here we go.
- Port 67: DHCP server listening port
- Port 68: DHCP client listening port
DHCP Server – Port 67
Qualifying as the server-side, port67is where the DHCP server waits to hear any incoming requests from DHCP clients. To put it simply, this specific port is the "hotline" that listens when clients dial in with their requests like "Hey, Server, I need an IP address!". All these requests from the client to the server communicates through UDP port
67.
DHCP Client – Port 68
Now the other side of the story, i.e., our port no
68. This is where the DHCP client waits to get responses from DHCP servers. So, once the DHCP server replies to the clients' request for an IP address saying something like, "Hey, I got an IP address for you!", the response travels back through UDP port
68. The client system stays alert in order to listen if anyone has replied through this port.
This clear distinction of roles for each port setup is beneficial. Think of a busy beehive, having well-defined roles makes things orderly, ensuring no clash in communication or in listening.
Communication Flow Between the Ports
Now, let’s look at the communication flow between these ports in a bit more detail:
1. The client broadcasts a DHCPDISCOVER message on the network via UDP port
67, looking for available DHCP servers.
2. Available DHCP servers pick up this "call", pulling the signal from UDP port
67and then prepare a DHCPOFFER message with an IP address offer.
3. Once the DHCP server sends the DHCPOFFER, it travels out from its end and goes back to the client through UDP port
68.
4. Post receiving the DHCPOFFER(s), the client chooses one, broadcasting a DHCPREQUEST message again through UDP port
67to let the selected DHCP server acknowledge the acceptance of the IP address.
5. Finally, the DHCP server receives the DHCPREQUEST and completes the process by sending a DHCPACK message back to the client via UDP port
68. This confirms the assigned IP address.
Do note that in all communications above, we used the word "broadcast". That's because DHCP uses Broadcast IP addressing for its initial stage. If the client doesn’t know which IP address it should use, it cannot use a specific IP address allied form of communication. Therefore, it necessitates broadcasting to allow every device on the network to accept the packet.
Reference:
RFC 2131 provides the original detailed specification for the DHCP protocol, including the use of these ports.To give you a sliver of how the code aspect might appear, consider the Python codes for creating UDP sockets and binding them to these specific ports:
Server-side (Port 67):
import socket sock = socket.socket(socket.AF_INET, socket.SOCK_DGRAM) sock.bind(('0.0.0.0', 67))Client-side (Port 68):
import socket sock = socket.socket(socket.AF_INET, socket.SOCK_DGRAM) sock.bind(('0.0.0.0', 68))Ultimately, understanding that the two distinct DHCP ports, and the flow of communication amongst them, is crucial to comprehend the DHCP operation holistically. The dual-port system allows both server and client to independently communicate and listen on their terms, facilitating smooth functionality within the network.Certainly, let's jump into the world of network protocols and shed light on the mystery of why there are two ports for DHCP.
To understand this matter more profoundly, we need first to jog our memories about what DHCP (Dynamic Host Configuration Protocol) is. In a nutshell, it is a network protocol that empowers computers to automatically request and obtain an IP address from a server. The primary purpose of the DHCP is to simplify the process of managing and maintaining IP configurations in large networks.source
Now, onto the two-port question. To facilitate seamless communication between DHCP clients and servers, we need two UDP ports:
Port 67and
Port 68. These ports serve a particular purpose in this interactive process.
Port Purpose Port 67 DHCP Server Port — DHCP Servers listen on this port for incoming client requests. Port 68 DHCP Client Port — Clients use this port to receive broadcasted messages from the server.
Also, they will send requests to the server via this port.Let's briefly take a look at how it all works:
Client Broadcast (DHCPDISCOVER) ---> Server (Port 67) Server Offer (DHCPOFFER) ---> Broadcast to (Port 68) Client Request (DHCPREQUEST) ---> Server (Port 67) Server Acknowledge (DHCPACK) ---> Broadcast to (Port 68)The above code showcases the four steps in what's known as the DORA Process (Discover, Offer, Request, Acknowledge). Throughout this cycle, the client discovers servers, receives offers, requests IP addresses, and finally, acknowledges the received IP.source
Evidently, these two ports are integral to facilitating this exchange and making sure the right data gets to the right place.
Implementing this dual-port system is highly beneficial since it helps prevent casual or accidental interference. When the two parties communicate over different ports, it reduces the chance of messages being corrupted or interfered with by other processes on the same system. Therefore, having one dedicated port each for the server and client simplifies this process, ensuring a smoother flow of information.
In summary, the dual port system in DHCP enhances network efficiency by enabling accurate identification and communication between the sender (client) and receiver (server). Hence, understanding this interaction between the two ports can provide a perspective on how efficient network allocation practices are carried out in real-world scenarios.When examining technical protocols, especially DHCP (Dynamic Host Configuration Protocol), an interesting detail stands out: it utilizes two ports. This naturally begs the question, why does DHCP require two separate ports? To unravel this mystery, we need to dive deep into what DHCP is and how it works.
DHCP is a network protocol employed on IP networks, where a server dynamically assigns an IP address and other network configurations to each device. This automation eradicates the necessity for a network administrator to manually configure these settings.
Now, let's answer the main query - the reason for two ports. The demarcation boils down to the
DHCP Discover/Broadcastand
DHCP Offer/Unicast. Here are their roles:
- DHCP Discover/Broadcast (UDP 67): The client machine that connects to the network sends a 'Discover' message in order to identify any available DHCP servers in the network. The destination port for this broadcast message is UDP port 67.
- DHCP Offer/Unicast (UDP 68): The DHCP server then responds with an 'Offer' message, which is directed towards the client machine. In this case, the destination port is UDP port 68.
Incorporating these two ports facilitates a seamless and harmonious communication between the client and the server.
Let's visualize this using a simple table:
Message Type Description Destination Port DHCP Discover/Broadcast The client sends a message to find DHCP servers in the network. UDP 67 DHCP Offer/Unicast The server sends a message to offer IP addresses and network configurations. UDP 68 To better grasp this concept, consider this Python sample code that emulates a basic DHCP transaction:
import scapy.all as scapy def dhcp_discover(): ethernet = scapy.Ether(dst="ff:ff:ff:ff:ff:ff") ip = scapy.IP(src="0.0.0.0", dst="255.255.255.255") udp = scapy.UDP(sport=68, dport=67) bootp = scapy.BOOTP(chaddr="client_mac") dhcp = scapy.DHCP(options=[("message-type","discover"), "end"]) packet = ethernet/ip/udp/bootp/dhcp return packet def dhcp_offer(): ethernet = scapy.Ether(src="server_mac", dst="client_mac") ip = scapy.IP(src="server_ip", dst="client_ip") udp = scapy.UDP(sport=67, dport=68) bootp = scapy.BOOTP(yiaddr="offered_ip") dhcp = scapy.DHCP(options=[("message-type","offer"), "end"]) packet = ethernet/ip/udp/bootp/dhcp return packet scapy.sendp(dhcp_discover()) scapy.sendp(dhcp_offer())Overall, the use of two ports in DHCP not only defines specific roles for each of them but it also simplifies the communication pathway between the server and the client. The presence of these two different ports for separate tasks ensures that the integrity and the efficiency of the network connection will always be maintained, and thus enabling a productive interconnection between various devices.When we talk about DHCP (Dynamic Host Configuration Protocol), we immediately associate it with two ports: port 67 and port 68. These are the default ports used in IP networking to convey DHCP information, and they serve a significant feat. The primary reason behind using not only one, but two ports for DHCP is to effectively handle server-to-client and client-to-server communication.
Understanding the Role of Each Port
To understand the reasons and implications better, let's descipher the role these ports play:
- Port 67: This UDP port is used by DHCP servers for the listen directive.
- Port 68: DHCP clients use this UDP port for sending data to the servers.
So, why do we have two separate ports instead of one? The necessity appears from how the DHCP protocol manages data transfer. To work efficiently and without interference, DHCP requires both server-side listening and client-side sending. As these actions need individual paths, the utilization of two ports becomes inevitable. It resolves an array of problems that could occur if only one port was employed:
Difficulties that Could Arise When Using Only One Port
1. Synchronization issues: If there were only one port, it would be tremendously challenging to manage requests from multiple DHCP clients simultaneously. It would create synchronization complications causing either bottleneck or conflicts among the packets.
2. Packet transmission interference: Another snag would be that whenever transmission is active, the uncommon path could exacerbate the disturbance, leading to packet loss and impairing network efficiency.
3. Limitation on Simultaneous Communication: A single port can also limit simultaneous bi-directional communication between the server and client because each port can handle only one type of service at a time.
How Two Ports resolve These Issues
The architecture of the DHCP protocol necessitates the use of two ports to overcome these roadblocks smoothly.
1. Efficiency: We ensure more efficient data transfers with less chance of conflict or packet loss, significantly improving the speed and reliability of the network.
2. Simultaneous Bi-directional Communication: With one port dedicated to listening (for servers) and another to sending (for clients), it allows for simultaneous bi-directional communication.
These reasons provide a compelling capacity to understand DHCP's dual-port necessity. Now, let's examine a basic example showcasing this process:
Client -- DHCP Discover(port 68) --> Server(port 67) Server -- DHPC Offer(port 67) --> Client(port 68) Client -- DHCP Request(port 68) --> Server(port 67) Server -- DHCP ACK(port 67) --> Client(port 68)This cycle repeats every time a client interacts with the DHCP server for IP allocation, ensuring a clear, conflict-free communication channel thanks to the use of these two ports. This understanding helps us appreciate the complexities and intricacies associated with seemingly trivial facets of computer networking. And as a coder, it adds another feather to my problem-solving arsenal.When dealing with network communications, particularly in a DHCP (Dynamic Host Configuration Protocol) setting, you might encounter Communication errors. This can happen when there is an issue transmitting data across the four-way transaction sides, i.e., both from client to server and server to client side. To troubleshoot these issues, it's important to take a methodical approach that not only helps to identify the problem but also provides key insights into improving the overall communication system.
First off, let me clarify one of the fundamentals: why there are two ports for DHCP.
DHCP operates on two different UDP ports: port 67 and 68. Port 67 is used by the DHCP server, and port 68 is used by the client. When a client communicates to a server, it sends a request from its own port 68 to the server's port 67. The server, in return, responds from its port 67 to the client's port 68. This differentiation of ports allows for clear and organized traffic flow between servers and clients. It tells routers and switches exactly where to send response packets and how to handle incoming packets. It's also what makes it possible for multiple clients to communicate with the server simultaneously without any data overlap.
Using Wireshark to see the packet Transfer: The Client (from its port 68) C -> S (to Server port 67) Server (from its port 67) S -> C ((to Client port 68)Now that we've clarified why there are two ports for DHCP, let's dive deeper into some primary troubleshooting strategies if problems occur during these four-way transactions transmissions:
1. Confirm Network Connectivity: Ensure both parties(Server and Client) have stable network connectivity. One way to do this is by pinging the router or using methods like trace route to confirm the network path.
Ping Command Example: ping 192.168.1.12. Trace and Analyze Packet Transfers: Use a tool like Wireshark or tcpdump to monitor and analyze the DHCP packets being sent and received. Look out for any anomalies or unexpected behaviors. For example, you should see a DHCPOFFER from the server after a DHCPDISCOVER message from the client.
Wireshark command to filter DHCP packets: dhcp3. Check Server logs: A significant step is checking your DHCP server logs. This will provide crucial information about the server's action, including responses(or lack thereof) to client requests.
4. Reconfigure or Restart Services: Sometimes, the root issue may be faulty configurations, in which case you'll need to re-check and correct your DHCP server and client settings. Restarting the service afterward is also a good practice to reboot the changes.
5. Update System Software: If all else fails, updating your software (Operating System, Firmware, network drivers, etc.) can sometimes resolve unexpected issues. An outdated software can often cause compatibility and functionality problems.
Remember, sorting through networking issues often involves a lot of trial and error. It's not always straightforward since the problems can be caused by various factors. The key is to maintain a methodical approach, gradually eliminating potential causes and zeroing down to the actual source of the problem. Whether the trouble is due to incorrect configuration, software bug, or hardware fault, patience, and in-depth understanding of your network architecture are compulsory.
You can also refer to RFC 2131, which exhaustively defines the DHCP protocol and covers prevalent issues alongside their viable solutions .Every interconnected device on a computer network has an IP address. DHCP, or the Dynamic Host Configuration Protocol, is responsible for assigning these IP addresses in a network. This protocol operates over two ports: port 67 and port 68. The reason DHCP uses both these ports revolve around the implementation of its client-server architecture and also aid in enhancing security at both ends of this spectrum.
In a typical DHCP interaction, servers listen on UDP (User Datagram Protocol) port number 67 while clients listen on UDP port number 68. This interplay allows the DHCP server to send responses to the DHCP clients, facilitating communication between them while mitigating risks associated with unauthorized access or malicious activity.
Let's delve deeper and understand how these ports offer security measures:
1. Port 67:
This is where the DHCP server listens for requests from DHCP clients. Assigning a specific port for this purpose ensures a dedicated channel through which the server can operate. This reduces the risk of potential interference or confusion with other server tasks.
// Example Code showing Server Listening on Port 67 var dgram = require('dgram'); var server = dgram.createSocket('udp4'); server.on('listening', function () { var address = server.address(); console.log('DHCP Server listening on ' + address.address + ":" + address.port); }); server.bind(67);By limiting the actions on this port to only those related to DHCP, we create a more stable environment that's less susceptible to attacks by minimizing points of vulnerability.
2. Port 68:
Similarly, DHCP clients use port 68 to listen for responses from the DHCP server. By clearly defining how and where the communications are received, system administrators can set up appropriate firewalls and other security measures to protect against outside intrusion.
//Example Code showing Client Listening on Port 68 var dgram = require('dgram'); var client = dgram.createSocket('udp4'); client.on('message', function (message, remote) { console.log('A new message has been received' ); }); client.bind(68);By controlling the exit and entry points for data exchange, this port-assignment strategy diminishes the chances of unwanted access and improves overall network security.
In the broader context of security measures, DHCP offers some additional benefits:
- IP Address Management: DHCP helps avoid IP conflicts and heightens security by ensuring each connected device has a unique IP address.
- Network Access Control: DHCP provides options to control the range of IPs assigned to devices thus regulating who gets network access.Risk Management: DHCP logs provide significant data which can be crucial for identifying any erroneous or malicious attempt trying to harm your network.^1^
So, as you can see, the utility of two different ports for DHCP is not just a product of its architecture but significantly contributes towards its security scheme. Therefore, understanding their roles and functions can help optimize your network operation and bolster your security front.
The arrangement of IP addresses through double gates or ports deals with the intricacies and allocation in a communication network. Specifically, the scenario you've mentioned utilizes two "ports" - 67 and 68, essential to the DHCP (Dynamic Host Configuration Protocol). Let's delve deeper into why these two ports are used for DHCP.DHCP is a network protocol functioning on application layer of the TCP/IP model. Its major responsibility lies in managing network parameters, including IP address management. With DHCP, devices on a network can automatically receive an IP address required for network communication.
The two ports, 67 and 68, have differing roles associated with them:
1. Port 67: This port is utilized by the DHCP servers. When your computing device initially boots up, it communicates using what we refer as a DHCPDISCOVER message which is directed towards the broadcast IP address `255.255.255.255` aiming to find available DHCP servers out there. Port 67 is the listener or receiver for these messages.
2. Port 68: On contrary, Port 68 listens for data from the DHCP servers. Oftentimes, a singular network has more than one DHCP server functioning simultaneously to handle large traffic load or ensure redundancy if one fails. After all the information packets are sent to port 67 on all the DNS servers, they respond back with their respective DHCPOFFER packets. These are then received on port 68 by our computing device.
Here's how the conversation generally flows:
Step 1: Client (at port 68) -> Broadcasts DHCPDISCOVER -> Port 67 Step 2: Server (at port 67) -> Returns DHCPOFFER -> Port 68 Step 3: Client (at port 68) -> Sends DHCPREQUEST -> Port 67 Step 4: Server (at port 67) -> Dispatches DHCPACK -> Port 68It's significant to note that by reserving two different ports for the dispatch and receipt of communication, it helps avoid loop backs and conflict of packets. The client and server do not run into any addressing conflicts as they both use different ports for sending and receiving, and thus it enhances the efficiency and reliability of communication between clients and servers.
RFC (Request For Comments) 2131 throws sufficient light on elaborations and advancements of DHCP over BOOTP. It chronicles numerous key features including automatic allocation, dynamic assignment with a lease timer, networking parameter ability such as subnet mask, default gateway etc.
Thus, while allocating IP addresses, to ensure reliable performance and effective communication, two ports—67 and 68—are incorporated within DHCP.When we talk about the existence of two ports for Dynamic Host Configuration Protocol (DHCP), it's important to understand the specific role each port serves. Primarily, there are two elements at play here:
1. The DHCP server
2. The DHCP clientThe DHCP server listens on UDP port 67. This is where the server waits for DHCP requests from clients. On the other end, the DHCP client uses UDP port 68 as it is designed to listen for responses from a DHCP server after sending out its request.
//Port numbers for DHCP #define DHCP_SERVER_PORT 67 #define DHCP_CLIENT_PORT 68To describe it in a more structured manner:
Device Port Number DHCP Server UDP 67 DHCP Client UDP 68 These separate ports are required because DHCP relies on a client-server model. The segregation facilitates the clear flow of requests and responses between the two entities and helps prevent any conflicts or communication issues.
It is also worth noting that the use of different ports has roots in security. Having DHCP servers and clients operate on separate ports enhances the architecture's safety by making it less prone to certain types of attacks. Notably, an attacker cannot send DHCP responses directly to a client unless they can access the protected UDP port 68.
Therefore, when it comes to addressing why two ports for DHCP exist, it's primarily due to the inherently distinctive roles of these ports in ensuring reliable, secure, and efficient communication between DHCP clients and servers.