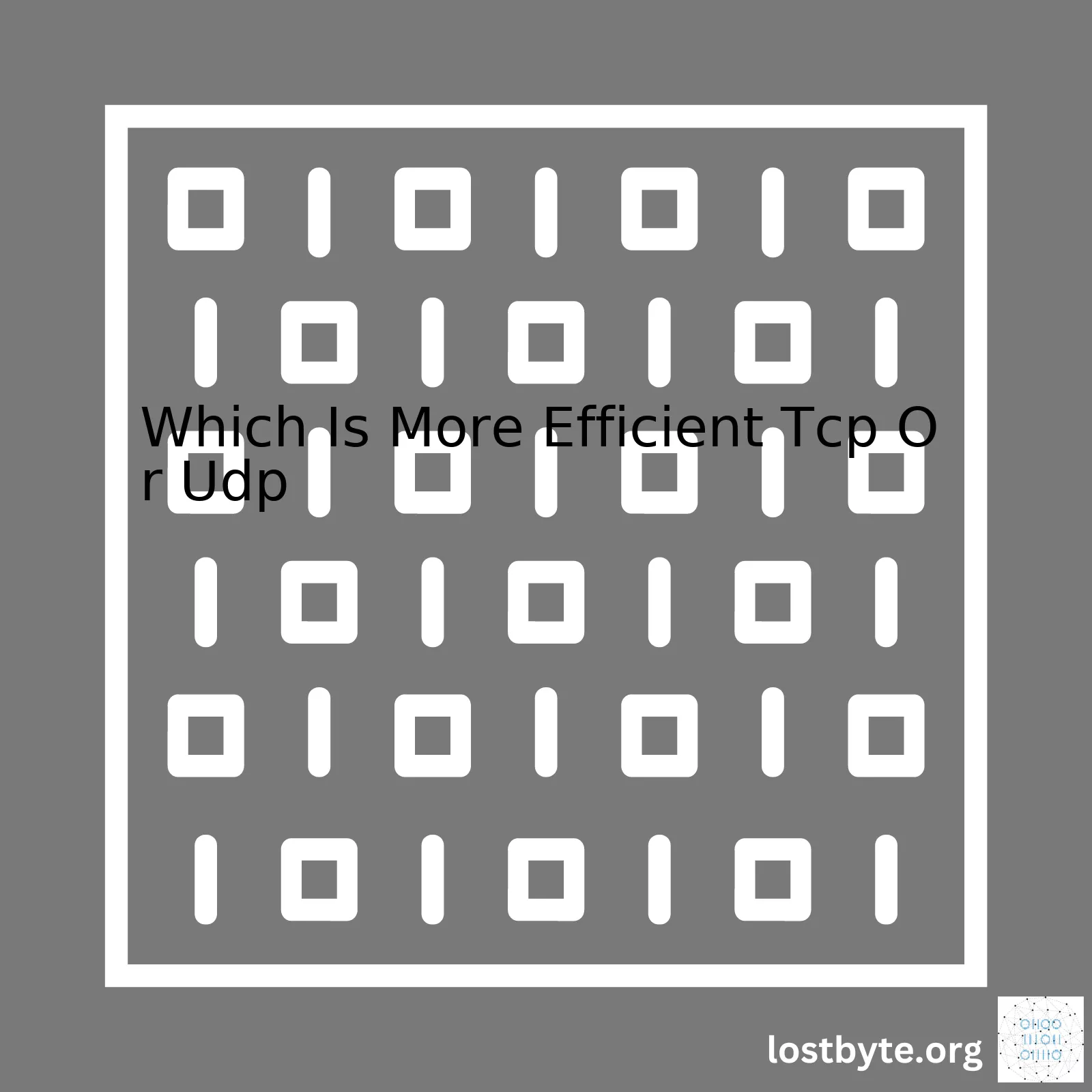
Aspects | TCP | UDP |
---|---|---|
Connection Type | Connection-oriented | Connectionless |
Error Checking | Yes | No |
Speed | Slower | Faster |
Order of Delivery | Maintains order | No particular order |
Reliability | High | Low |
In terms of efficiency, it largely depends on the specific context and what you are trying to accomplish.
Let’s dig deep into each parameter:
Connection Type: TCP is connection-oriented, initiating communication using a three-way handshake, which guarantees that the destination is ready for communication and can acknowledge receipt of packets. This feature could be critical if ensuring packet delivery is paramount, but it could also introduce overhead that reduces perceived ‘efficiency’. On the other hand, UDP is connectionless. It transmits packets without establishing a connection which makes it more efficient for use-cases like broadcasting where the overhead of establishing connections is unnecessary.
Error Checking: TCP does error checking, thus ensuring the correctness of data transmission. This makes TCP a good option when the integrity of data is crucial. However, this process can slow the operation down. UDP doesn’t provide such feature, making its operations faster; however, it cannot guarantee the correctness of data transmitted.
Speed: UDP is faster as it does not need to establish connections, nor does it require an acknowledgement for packet receipt. TCP, due to its features that assure data reliability, often results in being slower – consuming more bandwidth and time – hence might be viewed as less “efficient.”
Order of Delivery: TCP delivers packets in the order they were sent which is useful for data that must arrive in a specific sequence. Yet, it may imply inefficiencies when the order is not important. UDP sends packets in any order, appealing to scenarios where delivery speed matters more than sequence.
Reliability: TCP offers high reliability for your data transmission. It re-transmits lost packets and ensures data aren’t damaged or lost during transmission. This feature could cut both ways: while making TCP reliable, it also brings along additional bandwidth use and latency, potentially reducing efficiency. UDP, conversely, provides less reliability but with lesser latency too.
Thus, neither TCP nor UDP overwhelms the other overall. The choice of protocol entirely depends on the application needs. If speed is prime, then UDP wins; if reliability is prime, then TCP has the upper hand. Suitability should be based on these parameters rather than labeling one as ‘more efficient’ universally. So it is not about Which is more efficient, TCP or UDP? but it mostly leans towards What do you need more? Speed or Reliability?. Choose the best tool for your task based on your requirements!
In the world of internet communication, Transmission Control Protocol (TCP) and User Datagram Protocol (UDP) play a pivotal role. TCP and UDP are part of the internet protocol suite that makes up the basis of data conversation. They may differ according to efficiency in different scenarios because they have very distinct inherent characteristics. So, is TCP more efficient or UDP? Let’s jump right into it!
TCP: Reliable but Robust
Transmission Control Protocol (TCP) is a standard for transmitting data over networks that provides error checking and correction. Its key features include:
- Acknowledgement of packets
- Flow control mechanism
- Error checking and recovery
The entire delivery process with TCP is similar to sending a registered mail. The sender knows about the successful transmission only when the acknowledgment is received.
Here is an example of establishing a TCP connection
// Server side
Socket socket = new Socket();
socket.bind(new InetSocketAddress(5000));
// Client side
Socket socket = new Socket();
socket.connect(new InetSocketAddress("192.168.1.1", 5000));
UDP: Fast but ‘Fire-and-Forget’
On the contrary, User Datagram Protocol (UDP) is like passing notes in a classroom. It doesn’t acknowledge if a packet has been delivered, nor does it provide any tracking information.
UDP boasts the following features:
- No built-in error correction mechanism.
- Rapid data transfer since there are no acknowledgements.
- Best suited for broadcast messages.
Check out how a UDP connection can be set:
xxxxxxxxxx
// Server side
DatagramSocket datagramSocket = new DatagramSocket(5000);
// Client side
DatagramSocket datagramSocket = new DatagramSocket();
datagramSocket.send(new DatagramPacket(data, data.length, InetAddress.getByName("192.168.1.1"), 5000));
Which One is More Efficient?
To find which one – TCP or UDP, is more efficient, we need to first define what “efficiency” means in our context. If ‘efficiency’ is defined by fast and voluminous data transfer without worrying about data loss or errors, then UDP is more efficient. It removes the mechanisms of re-sending lost packets or acknowledging successful deliveries, which reduces network burden and speeds up the overall data transmission rate. It’s most appropriate for live broadcasts or gaming where missed frames or information don’t significantly degrade quality.
On the other hand, if ‘efficiency’ implies ensured delivery without data corruption, TCP is more efficient. Though taking longer, it guarantees that every single packet will reach its destination in the correct order, making it ideal for database operations, email exchanges, file transfers, and web browsing.
One needs to choose between speed and reliability when it comes to choosing between TCP and UDP. The choice, thus, should be driven by the specific requirements of your application. For a thorough comparison, you might want to check this Lifewire article.
Transmission Control Protocol (TCP) and User Datagram Protocol (UDP) are both transport protocols used to send data packets over the internet. This comparison has taken an interesting standpoint from the effectiveness perspective of TCP and UDP but primarily focusing on the distinguishing features of TCP.
1. Connection-oriented:
TCP is a connection-oriented protocol, meaning that it establishes a connection before transmitting data.
xxxxxxxxxx
TCP_request() // Requesting connection
TCP_accept() // Accepting connection request
. Hence, guaranteeing reliable delivery of packets as TCP retransmits lost or out-of-order packets.
2. Error checking:
TCP performs extensive error-checking, ensuring every packet sent is acknowledged by the recipient
xxxxxxxxxx
send_data()
ack_received()
. When there’s no acknowledgement, TCP automatically resends the data. While such a feature bolsters reliability, it also creates overhead that might not be applicable in real-time applications.
3. Flow control:
A vital characteristic of TCP lies in its flow-control mechanism. Unlike UDP that sends datagrams without considering the recipient’s condition, TCP adjusts the rate at which data is sent based on network congestion. This is done through a sliding window mechanism
xxxxxxxxxx
sliding_window_algo() //Implementing flow control
. Such a feature minimizes potential traffic jams but may slow down data transfer.
4. Ordered delivery:
TCP guarantees ordered delivery of data packets as each data segment header includes a sequence number
xxxxxxxxxx
assign_sequence_number() //Assigns unique sequence numbers
. This nature is essential where order of data matters – for instance in displaying webpages with graphics or streaming videos.
In contrast, TCP’s efficient sibling UDP is connection-less, delivers unordered data, does minimal error-checking and is void of flow-control. Absence of these features make UDP faster and more efficient in terms of overhead but less reliable than TCP. Use cases for each largely depend on application requirements: reliability over speed or speed over reliability.
Consider audio streaming, gaming or VoIP calls; these areas favor UDP due to minimal delay requirements even if some packets get dropped. However, file transfers, emails or webpage displays use TCP because they need all content delivered accurately.
Find more information about TCP and UDP differences on this online visualization link.
Remember, there’s no definitive answer on whether TCP or UDP is more efficient as it boils down to specific needs of an application – Speed or Accuracy?
Transmission Control Protocol (TCP) and User Datagram Protocol (UDP) play key roles in data transmission across the internet. While they share commonalities, they differ significantly which is why we need to consider their unique characteristics to know which one is more efficient for a particular situation.
Unique Characteristics of TCP and UDP
The User Datagram Protocol (UDP) Wikipedia tends to be faster and more efficient for short communications because it lacks the error-checking mechanisms of TCP. This makes it suitable for video streaming, where occasional lost packets are less important than maintaining the flow of data.
On the other hand, Transmission Control Protocol (TCP) Wikipedia guarantees delivery by providing error checking and recovery features that ensure all packets reach their destination. It’s reliable and perfect for applications that require high reliability, like web servers and databases.
Taking this into consideration, here are some unique characteristics of both protocols:
Characteristic | TCP | UDP |
---|---|---|
Error checking | Yes | No |
Delivery Guarantee | Yes | No |
Order Delivery | Yes | No |
Speed | Slower | Faster |
Suitable For… | Email, Web Browsing, File Transfers | LIVE Video/Audio Streaming, Games |
So Which Is More Efficient: TCP or UDP?
Efficiency depends on what you mean by ‘efficient.’ If efficiency pertains to speed, then UDP is the clear winner. Without the burden of ensuring ordered, reliable packet delivery, UDP-based applications can pump packets onto the network as fast as the bandwidth will allow.
xxxxxxxxxx
/* Commented Sample Code Showing Usage of UDP Socket */
/* Create UDP Socket */
socket(AF_INET, SOCK_DGRAM, 0);
/* Send Data - (Fast but unreliable) */
sendto();
However, if your measure for efficiency factors in reliability, then TCP’s rigorous error checking makes it a better option. Even though TCP requires more bandwidth compared to UDP due to its control mechanisms such as handshake process, retransmission, etc., it ensures accurate data delivery from source to destination.
xxxxxxxxxx
/* Commented Sample Code Showing Usage of TCP Socket */
/* Create TCP Socket */
socket(AF_INET, SOCK_STREAM, 0);
/* Send Data - (Slow but Reliable) */
send();
Remember, each protocol serves different purposes in network communication. Efficiency must therefore align with the specific needs of your application.
The comparison of efficiency between Transmission Control Protocol (TCP) and User Datagram Protocol (UDP) requires a deep understanding of their underlying functionalities. Both protocols have both strengths and weaknesses making them preferred choices in particular situations.
Transmission Control Protocol (TCP)
xxxxxxxxxx
TCP
, part of the Internet Protocol suite, is a connection-oriented protocol facilitating reliable data transfer between two network devices.
- Reliability: TCP ensures data integrity, maintaining an error-checking mechanism that retraces lost or erroneous packets and retransmits them. Its use of sequence numbers helps to assemble packets in their original order at the receiving end.
- Connection-oriented: Before transmitting any data, TCP establishes a connection using a three-way handshake (SYN, SYN-ACK, ACK). This stablishment guarantees the delivery of packets.
- Flow Control: TCP manages a suitable rate of information flow by controlling its sender from overloading the receiver.
- Congestion Control: TCP has a built-in congestion control mechanism. On detecting network congestion, it reduces the data sending rate to alleviate the situation, ensuring efficient network utilization.
However, these features also pose a negative impact on the speed, making TCP slower compared to UDP.
User Datagram Protocol (UDP)
xxxxxxxxxx
UDP
, another member of the Internet Protocol suite, represents a simpler, connectionless Internet protocol.
- Unreliable: Unlike TCP, UDP does not provide any guaranty about packet delivery. Packets may arrive out of order, go missing, or duplicate during transmission.
- No Connection Setup: UDP doesn’t require pre-establishing a connection for transmitting data. It uses datagrams (independent packets).
- No Congestion Control: UDP lacks congestion control, causing potential overload and consequent inefficiency during heavy data transfers.
However, despite these downsides, UDP’s simplicity gives it speed. It comes in handy when you need fast, less reliable transmission such as live audio/video streaming.source
TCP | UDP | |
---|---|---|
Reliability | High | Low |
Speed | Slow | Fast |
Ordering of Data Packets | Yes | No |
Uses Handshaking | Yes | No |
Congestion Control | Yes | No |
In sum, choosing TCP vs UDP boils down to the degree of reliability, order preservation, and data integrity required by your application. For applications focusing on speedy data transmissions where a negligible loss can be tolerated – like streaming services, online gaming, or Voice over IP (VoIP),
xxxxxxxxxx
UDP
becomes more efficient. However, if your application necessitates robustness, precision, and accuracy, such as web applications, mail services, or file transfers, then
xxxxxxxxxx
TCP
will serve as a more efficient choice.source
If we want to examine TCP (Transmission Control Protocol) and UDP (User Datagram Protocol) in terms of efficiency and reliability, we must dive deep into their functionalities, especially how they handle error checking. So let’s break it down.
TCP is known for its robustness and reliability because it offers error checking and correction features essential for applications that require high data integrity. The underlying mechanism involves sequence numbers and acknowledgment messages. In essence, each packet transmitted over a TCP connection carries a sequence number, which allows the receiving node to reorder the received packets and send an acknowledgment number back to the sender. If a packet is lost during transmission or arrives with errors (detected via a checksum),
xxxxxxxxxx
TCP
flags it and requests retransmission. Here’s a simplified example:
xxxxxxxxxx
// TCP sends
SEND packet 1 --> WAIT for ack... <-- ACK 2 RECEIVED -- Continue to send next packet
// packet not acknowledged, TCP resends
SEND packet 3 --> WAIT for ack... <-- NO RESPONSE -- RESEND packet 3
On the other hand, UDP doesn’t offer any such mechanism. It has no inherent error-checking or recovery function. UDP just shoots out the data and hopes it gets there. It isn’t concerned with delivery order, either. This might sound unreliable, but it makes UDP quite lean and efficient for certain protocols and applications. A simple representation would be:
xxxxxxxxxx
// UDP simply sends without waiting for acknowledgments
SEND packet 1 --> SEND packet 2 --> SEND packet 3
Now, to answer the question “Which is more efficient, TCP or UDP?”, we need to specify the context. Efficiency can mean different things in different scenarios. Here’s a quick breakdown:
- For applications where data integrity and delivery order are crucial – like HTTP, FTP, or email where losing a single packet could corrupt the whole message – TCP’s overhead and error-correction mechanisms enhance efficiency, despite consuming more resources.
- Conversely, for tasks such as streaming audio/video, real-time gaming, live broadcasts, or DNS lookups, UDP’s 'fire-and-forget' method is far more efficient. Some data loss or out-of-order packets won’t significantly impact the overall experience, and the system’s performance won’t degrade due to repeated retransmissions.source
This table summarizes the main points effectively:
TCP | UDP | |
---|---|---|
Error Checking | Yes | No |
Recovery Mechanism | Yes (Resend) | No |
Efficient for high-integrity applications | Yes | No |
Efficient for real-time applications | No | Yes |
In conclusion, TCP and UDP have their own strengths and weaknesses, and the choice between TCP vs UDP efficiency entirely depends on the particular requirements of the application in use.When it comes to connectivity and data transmission over a network, TCP (Transmission Control Protocol) and UDP (User Datagram Protocol) are crucial protocols in the internet protocol suite. Despite their shared purpose - transferring data between systems -, these two differ in functionality, efficiency, and overall suitability for specific tasks.
TCP: Robust but Heavy
TCP is designed for accuracy and reliability at the expense of speed. As a connection-oriented protocol, it ensures full data delivery through a series of checks and balances:
- Handshake Method: A three-way handshake method (SYN, SYN-ACK, ACK) establishes the connection between sender and receiver.
- Error Checking: TCP checks for errors at every step by employing a checksum formula.
xxxxxxxxxx
111if(Checksum==Received_Checksum){ accept packet;} else { discard packet;}
- Acknowledgment System: The receiver acknowledges each packet received with an ACK back to the sender.
- Flow Control: TCP uses a sliding window flow control to manage the amount and speed of data sent. This avoids overwhelming the receiver.
- Ordering: Packets may arrive out of order, so TCP numbers them sequentially to ensure correct assembly at the destination.
- Retransmission: If a certain time elapses with no ACK from the receiver, the sender assumes the packet was lost and retransmits it.
While all these mechanisms create a robust framework that guarantees complete data transmission, they come with a cost in terms of bandwidth usage, latency, and processing power - making TCP less efficient for certain applications.
UDP: Lightweight But Risky
Contrary to TCP, UDP is a connectionless protocol, meaning it sends data without any assurance of its arrival. This provides several advantages:
- No Handshake Protocol: UDP does not require connection establishment, thus reducing overhead and speeding up transmission times.
- No Acknowledgement: With no need for the receiver to send acknowledgment packets, network traffic congestion is reduced.
- No Sequential Numbering: UDP sends data as soon as it receives it, eliminating any delay caused by packet sequencing.
- No Retransmission: Lost packets are left to higher-level protocols or the application itself to handle, creating a faster, more streamlined service.
However, such liberties mean that packet loss, out-of-order arrival, and error detection remain unhandled at the protocol level. This makes UDP less reliable but more efficient than TCP when it comes to real-time applications like streaming and gaming where speed matters more than completeness and sequence of data.
So, Is TCP More Efficient Than UDP? It depends on how you define "efficient." If maintaining data integrity and order is your highest priority, TCP's robustness outweighs the extra resources it consumes, thus, being more 'efficient' for your needs. Conversely, if speed and lightweight operation are vital, UDP's lack of protocol-level checks - despite risking data quality - makes it the more 'efficient' choice.
For a thorough examination of TCP vs UDP, I recommend checking the tutorial available at Guru99.First off, it is important to understand both TCP (Transmission Control Protocol) and UDP (User Datagram Protocol) in order to make an accurate comparison.
TCP - Transmission Control Protocol
TCP is a connection-oriented protocol that provides reliable, ordered, and error-checked delivery of a stream of bytes between applications running on hosts communicating over an IP network.
Some of the key features of TCP include:
- Acknowledgements for packets received
- Retransmission of lost packets
- Error detection via checksums
- Flow control - the rate at which data is transmitted is managed to prevent packet loss and network congestion
The downside to all of these features is the "chattiness" and overhead associated with TCP. Several round-trips are required before any application data can be sent, and this adds to latency.
UDP - User Datagram Protocol
On the other hand, UDP is a simpler, connectionless Internet protocol. With UDP, computer applications can send messages, in this case referred to as datagrams, to other hosts on an Internet Protocol (IP) network without requiring prior communications to set up special transmission channels or data paths.
Key features of UDP include:
- No guarantee of delivery
- No protection from duplicates
- Datagrams can arrive out of order
- No throttling mechanism, i.e., sender can transmit as fast as desired
UDP's strengths lie in its simplicity and efficiency. There isn't a need for extended session setup and teardown phases, and there is no state kept between packets. This means your application can send as fast as your OS/network will allow.
Congestion Handling Difference Between TCP And UDP
TCP’s built-in congestion handling mechanisms can slow the rate of output to match what the network path can sustain. When losses are detected,
xxxxxxxxxx
TCPcc
algorithms in modern operating systems back off the rate of output, then try to increase it again until the network’s capacity is once more detected.
On the other hand, UDP doesn’t have any inherent congestion avoidance, and so the sending host could potentially flood the network. Because of this, congestion on a network servicing UDP traffic has the potential to degrade quicker than a network servicing TCP traffic.
Efficiency Comparison
Answering which one is more efficient - TCP or UDP - really depends on what you define as "efficient". If by efficient, you mean, speed, then
- For real-time communications where speed is critical - like VoIP, online gaming, and live streaming -, UDP's 'send and forget' style is typically more efficient because it doesn't care whether packets arrive in the correct order or even at all.
- But, if your main concern is reliability over networks with unstable conditions, TCP's built-in error correction might function more efficiently.
It is also crucial to point out the impact on applications. For example, web browsing or email would not function properly using UDP due to the fact they require the reliable delivery that TCP offers. Here, TCP would be the 'more efficient' choice.
Thus, rather than thinking about efficiency in a broad sense, it may be helpful to consider what the specific needs and constraints of your particular network or application are. Efficiency can then be evaluated in terms of how well either TCP or UDP meets these requirements.
Sources:
1. Webopedia: TCP/IP
2. IETF: UDP
3. Microsoft QoS DocumentationTo answer your question about the efficiency of TCP vs UDP in a real-time context, it's crucial to dive into an analysis of different scenarios where they're used.
When we mention TCP (Transmission Control Protocol) and UDP (User Datagram Protocol), we are talking about two of the main protocols from the transport layer in networking.
The Pros and Cons of TCP
TCP is by far the most frequently used protocol due to its reliability. It guarantees that data packets will arrive in the order they were sent, and if any get lost along the way, the protocol will resend them. This makes it the preferred choice for transferring important data such as files or web pages. However, this all-round dependability comes at a cost: latency. TCP needs several exchanges between client and server to establish a connection before actual data transmission starts. That's called the "three-way handshake".
xxxxxxxxxx
> Client - SYN -> Server
> Client <- SYN-ACK - Server
> Client - ACK -> Server
The above example shows the 'three-way handshake' in sequence. This built-in robustness means increased overheads and slower delivery times.
The Pros and Cons of UDP
On the other hand, UDP is an example of simplicity. The underlying philosophy behind UDP is 'send and forget.' This protocol doesn't wait for acknowledgments before sending the next packet and doesn't reorder them when they are received. If traffic congestion results in lost packets, too bad – UDP doesn't know or care. Therefore, it’s much faster than TCP and works great in real-time scenarios where speed is prized over absolute precision.
For example, let's consider use cases like live broadcasts or multiplayer gaming:
- Live streaming video: If you're watching a football match happening halfway across the globe, you want the action relayed to you in real time. A delay of just a couple of seconds can take away from the excitement, especially when everyone on social media is reacting instantly. Using TCP can result in buffering and delayed playback due to packet retransmissions.
UDP shines here due to its blistering speed. Sure, you might notice slight pixelation occasionally when some packets are dropped, but overall, the user experience is much more seamless.
- Video conferencing services: Numerous users communicating simultaneously can't afford to be slowed down by a focus on packet delivery perfection. Timely, good-enough data arrival through UDP ensures smooth conversations free from lags.
- Online Gaming: The success of games like Fortnite and PUBG is partly due to the real-time interaction between players worldwide. Apply TCP’s strict reliability standards here, and the game would lag whenever there's packet loss or delay. Instead, using UDP allows for instantaneous reactions and thrilling gameplay.
In Summary...
To answer the original question - Is TCP more efficient than UDP or vice versa? - that highly depends on what you prioritize: speed or reliability. For most applications where overriding importance is given to the fast delivery of data rather than its perfect receipt, UDP often turns out to be the more "efficient" choice. Yet, when data integrity is paramount, TCP shall hold the efficiency crown.
Nevertheless, it's noteworthy to remember that these are generic principles and the actual reality can get way more complex. More and more systems are starting to employ custom transports built on top of UDP to add a controllable level of reliability ('reliability spectrum'). One such example is the QUIC protocol being developed by Google.
Remember, at the end of the day, the "better" protocol entirely depends on the specific requirements of the task at hand. Efficiency can only be determined once those parameters have been identified and weighed up against each other.Before delving into the specifics of whether Transmission Control Protocol (TCP) or User Datagram Protocol (UDP) is more efficient, it's worth establishing an understanding of what these protocols are. Both TCP and UDP are transport layer protocols used for sending bits of data — known as packets — over the internet.
TCP is reliable; it guarantees the delivery of data to the destination router. It provides error-checking mechanisms and packet sequence alignments to ensure accurate data transmission [techtarget]. However, it comes with a high overhead cost due to its complexities and established connection requirements.
UDP, on the other hand, has lower overhead because it does not provide the sophisticated features that TCP offers. No connection is needed before sending data and there's no guarantee of order or even delivery of sent packets. Hence, UDP is considered inherently faster but less reliable [techtarget].
The efficiency of TCP versus UDP ultimately depends on what you mean by "efficient". If your top priority is speed and you can afford data loss, then UDP shines here because of fewer processing requirements. This is suitable for applications like streaming where minor data loss might not be noticeable.
For example, considering video streaming,
xxxxxxxxxx
createSocket(AddressFamily.InterNetwork, SocketType.Dgram, ProtocolType.Udp)
might be preferred because missing some pixels may not ruin the viewing experience, though consistently losing lots of data does degrade quality.
However, if reliability and order preservation of communication is key, TCP is a better choice. For instance, when downloading a file, you'll want all parts of it to arrive, in the right order. In python, creating a socket would look like:
xxxxxxxxxx
createSocket(AddressFamily.InterNetwork, SocketType.Stream, ProtocolType.Tcp)
In different network conditions, these protocols behave differently:
Network Conditions | TCP Efficiency | UDP Efficiency |
---|---|---|
High Latency | Poor; due to TCP congestion-control algorithm, which scales back packet-sending rate at every lost/late ACK | Better; UDP isn't affected by high latency as it doesn't use ACKs for communication |
Low Bandwidth | Decent; flows well if data rate doesn't exceed available bandwidth | Fair; Uses considerably less bandwidth due to lesser headers than TCP |
Unstable Connection | Good; handles re-transmissions and guarantees ordered-delivery of packets | Poor; risk of multiple packet losses with no recovery mechanism |
To summarize:
• TCP is preferably used under moderate network conditions and applications requiring high reliability, and guaranteed packet delivery.
• UDP is ideally used in adverse network conditions and multimedia applications where speed triumphs perfect delivery.
Without context, it's tricky to pinpoint one protocol as more efficient over another. Efficient use of TCP or UDP relies heavily on the specific needs of your application, and understanding how they perform under different network conditions.
Adopting a hybrid approach: using reliable UDP libraries (example: UDT or QUIC), or application-aware TCP techniques could get the best of both worlds – something fast, enabling both speed and reliability [hpbn]. This method can allow for dynamically adjusting between TCP/UDP-like behavior based on network conditions, user preferences, and application requirements. Such real-time adjustments can maximize network-efficiency without compromising too much on reliability or speed.As a professional coder, it's critical to understand the underpinnings of network communication, particularly when making decisions on whether to use Transmission Control Protocol (TCP) or User Datagram Protocol (UDP). These are two primary protocols used for data transmission in network applications. To decide which one is more efficient, we need to take into account the characteristics of both TCP and UDP.
xxxxxxxxxx
// Using TCP
ServerSocket serverSocket = new ServerSocket(port);
Socket clientSocket = serverSocket.accept();
PrintWriter out =
new PrintWriter(clientSocket.getOutputStream(), true);
BufferedReader in = new BufferedReader(
new InputStreamReader(clientSocket.getInputStream()));
...
TCP delivers reliable, ordered, and error-checked delivery of byte streams between programs running on hosts communicating via an IP network. When sending data, TCP ensures that all packets reach their destination, without errors, in the same order they were sent.
xxxxxxxxxx
// Using UDP
DatagramSocket serverSocket = new DatagramSocket(port);
byte[] receiveData = new byte[1024];
byte[] sendData = new byte[1024];
DatagramPacket receivePacket = new DatagramPacket(receiveData, receiveData.length);
serverSocket.receive(receivePacket);
...
On the other hand, UDP prioritizes speed over reliability. It does not guarantee that packets will be delivered in any particular order, nor does it ensure that all packets sent will be received. This means that while TCP might offer more comprehensive service, UDP can be faster and less resource-intensive because it doesn't require as many system resources to operate.
When considering efficiency, it primarily depends on what kind of application you're building:
- For real-time applications like gaming or streaming where speed is crucial and packet loss can be tolerated, UDP is generally more efficient.
- For applications where reliability is paramount such as web browsers, email, and file transfers, TCP would be a more efficient choice as it ensures all data arrive correctly at the destination and re-transmits lost packets.
So, is TCP more efficient than UDP? Or vice versa? The answer isn't straightforward—it very much depends on the specific requirements of your project. Efficiency here is context-dependent rather than universally applicable.
There's a trade-off between reliable delivery (TCP) and fast, lightweight communication (UDP). Understanding this empowers developers to carefully consider the nuances of their projects when deciding which protocol is more appropriate (TCP vs UDP comparison table).
TCP | UDP |
---|---|
Reliable delivery | Faster, lighter weight communication |
Ensures data arrives in the same order it was sent | No order guarantees |
Re-transmits lost packets | Packets may be lost with no re-transmission |
Analyzing these factors will enable you to make an informed decision on whether using TCP or UDP connection is more beneficial in your situation. You can read more about these protocols at Mozilla developer resources1.The determination of whether TCP (Transmission Control Protocol) or UDP (User Datagram Protocol) is more efficient greatly depends on your unique application needs. Both protocols offer dedicated avenues for data transmission, each with their own strengths and weaknesses.
xxxxxxxxxx
// TCP is connection-oriented
Socket socket = new Socket(address, port);
DataOutputStream dout=new DataOutputStream(socket.getOutputStream());
// UDP is connectionless
DatagramSocket datagramSocket = new DatagramSocket();
byte[] buffer = "Hello World!".getBytes();
DatagramPacket packet = new DatagramPacket(buffer, buffer.length, address, 80);
datagramSocket.send(packet);
On one hand, TCP's reliable, connection-oriented nature ensures the order and integrity of transmitted packets making it ideal for data-intensive applications such as video streaming, file transfer, and web browsing where accuracy is critical.
Key TCP features:
- Ordered data transfer
- Retransmission of lost data
- Error-free data transfer
- Flow control
- Congestion control
On the other hand, UDP's connectionless architecture aids in faster data delivery due to fewer communication steps, which makes it suitable for applications where speed takes precedence over error checking and recovery like online gaming, VoIP, streaming media, and live broadcasts.
Key UDP features:
- Connectionless protocol
- Speedier data transfer due to lesser checks
- Suitable for real-time applications
- Low network latency
To determine which is more efficient - TCP or UDP, you need to consider the type, requirements, and tolerance levels of your application. For real-time, fast-paced applications, UDP could offer more efficiency due to its lower latency and fewer communication steps. However, if high fidelity, reliability, and error-free transmissions are key requisites, TCP’s features might provide the efficiency boost you're after in that scenario.
In summary, both TCP and UDP carry unique efficiencies within different contexts. By understanding these protocols' characteristics, we can intentionally use them to maximize the efficiency of specific real-world applications. It's not about choosing a one-size-fits-all solution but, rather, customizing the approach according to different application needs and circumstances.