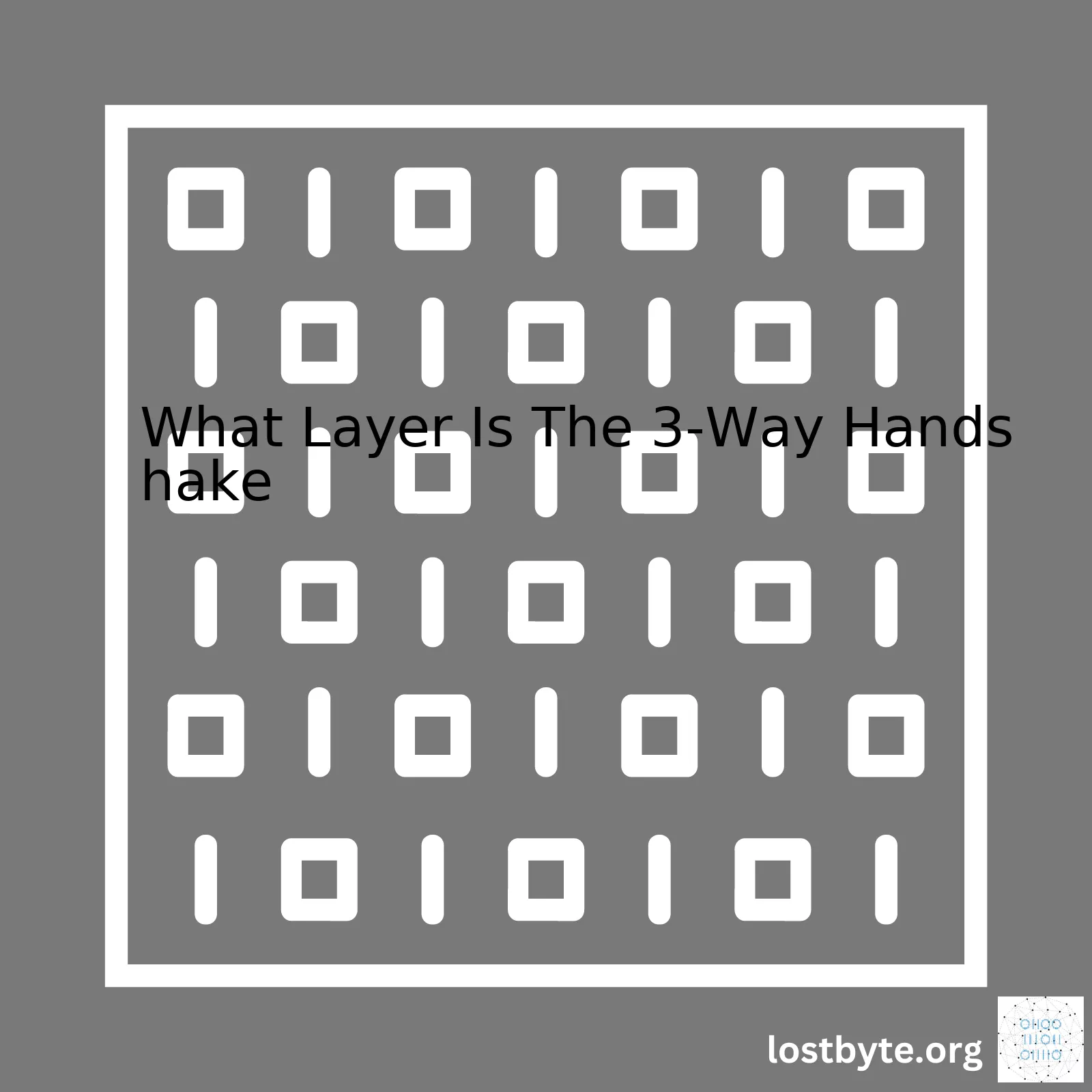
TCP/IP Network Model | |
---|---|
Layers | Functions |
Application Layer | User interface, comprehensive support protocols |
Transport Layer | Connection Establishment (3-Way Handshake), Reliable Transmission |
Internet Layer | Routing, Addressing |
Network Interface Layer | Data link control, Hardware details |
A three-step process of SYN, SYN-ACK and ACK makes up the “3-way handshake”. The client sends a SYN packet to the server requesting a connection, followed by the server acknowledging this request with a SYN-ACK packet. Finally, the client completes the handshake by sending an ACK packet back to the server.
These exchanges allow both ends to agree upon initial sequence numbers, which are essential for organizing packets and managing the orderly transfer of data. They also provide assurance that both recipients are ready to send and receive data, eradicating the risk of lost data or system overload.
Additionally, the completion of the 3-way handshake signals the beginning of the ‘connected’ state in a TCP/IP socket.
Client --------SYN----------> Server
Client <------SYN/ACK------- Server
Client --------ACK----------> Server
Reading about network protocols and connection establishment can sometimes feel abstract. However, an understanding of these processes is essential for many aspects of life today – from basic web browsing to much more complex tasks such as running a WebRTC application. I encourage you to dive deeper into the IOC model, where the 3-way handshake takes place, to further your grasp on the fascinating world of networking and coding.Understanding the 3-Way Handshake in the context of networking relates directly to the Transport layer of the OSI (Open Systems Interconnection) model. The Transport layer, also known as Layer 4, carries out this sequence for allowing a client and server to exchange data reliably over a network connection.
The term “3-Way Handshake” refers to the process involved in establishing a TCP (Transmission Control Protocol) connection between two network devices. This exchange involves three distinct steps: the SYN message, SYN/ACK message, and ACK message.
Below are the precise details of how these steps work:
• SYN message – A client (like your web browser) sends a SYN (synchronize) packet to a server it wants to connect with. This packet contains key information that the server needs, like the client’s initial sequence number.
xxxxxxxxxx
Client → SYN packet→ Server
• SYN/ACK message – Once the server receives the SYN packet, it responds with a SYN/ACK (synchronize/acknowledge) packet. This packet not only acknowledges the client’s request but also tells the client some important details about the server, such as it’s initial sequence number.
xxxxxxxxxx
Client ← SYN/ACK packet ← Server
• ACK message – Finally, the client sends back an ACK (acknowledge) packet, which simply confirms receipt of the SYN/ACK packet from the server. Following this acknowledgement, both the client and server have exchanged enough information to send actual data packets between each other.
xxxxxxxxxx
Client → ACK packet → Server
It’s interesting to note that a 3-Way Handshake is crucial within the context of making a secure connection in today’s internet use, ranging from surfing web pages to downloading data. Without it, reliable data transmission would face impediments.
Here is a simple diagram showing the concept visually.
To sum up, the 3-way handshake primarily pertains to Layer 4 or the Transport layer of the OSI model. It plays a fundamental role in transmitting data securely and ensuring the efficient operation of our daily internet activities.
The 3-way handshake protocol is a fundamental cornerstone of establishing connections in network communications, particularly in connection-oriented services such as the Transmission Control Protocol (TCP)
Specifically, when talking about the 3-way handshake, we are usually referring to how it’s used within the context of the TCP/IP model. This model describes a set of rules for how computers send and receive data over the internet or an intranet.
The TCP/IP framework is composed of four stacked layers. Each layer has a specific function and role to play during internet communication:
- Application Layer
- Transport Layer
- Internet Layer
- Network Interface Layer
The function of the 3-way handshake resides at the Transport Layer.
TCP 3-way Handshake Explained
This three-way handshake process establishes both ends of the connection to ensure they are prepared for transmission. The mechanism works roughly as follows:
- SYN: A SYN (synchronize) packet is first sent from one node (let’s refer to this as Node A) to another (Node B). It contains an initial sequence number (ISN).
- SYN-ACK: Upon receiving the SYN packet, Node B creates a session for the new connection and responds with a SYN-ACK (synchronize-acknowledge) acknowledgment packet that includes its own ISN.
- ACK: Lastly, Node A sends an ACK (acknowledgment) packet back to Node B, acknowledging the receipt of the SYN-ACK packet, thus confirming the successful establishment of the connection. This concludes the 3-way handshake.
To illustrate this, I’ll include a simple analogy. Think of the 3-way handshake as a phone call initiation: Node A dials Node B’s number (SYN), Node B answers and acknowledges the call (SYN-ACK), then Node A confirms reception (ACK), establishing clear communication lines.
Relation to the Transport Layer
The Transport Layer lies in the center of the TCP/IP model, facilitating communication between hosts. This is the ideal layer for the 3-way handshake method to exist, primarily because of two roles the Transport Layer plays.
- End-to-end connectivity: This layer facilitates full-duplex data streaming. Thus, ensuring the devices involved have established a solid communication line before transmitting data is critical—which is what the 3-way handshake process guarantees.
- Reliability: By incorporating mechanisms like acknowledgment packets and sequence numbers in a 3-way handshake, delivery reliability is significantly improved.
I hope this gives you a detailed idea about the role of TCP/IP in a 3-way handshake and its relevance to the Transport Layer. For further depth on this topic, you might want to check out some networking books or online resource materials focusing on internet protocols and data communication, such as this tutorial.
xxxxxxxxxx
// Pseudo implementation of a 3-way TCP handshake
function threeWayHandshake() {
sendPacket('SYN');
waitPacketType('SYN-ACK');
sendPacket('ACK');
}
In this pseudo-code, ‘sendPacket’ is a function defined elsewhere that sends a packet of the type passed to it, while ‘waitPacketType’ waits until a certain type of packet is received. A real-world implementation would diversify depending on your networking environment and the programming language you’re using.
The three-way handshake is an essential process in computing networks that occurs at the Transport Layer (Layer 4) of the OSI Model. The handshake establishes a connection between two network nodes utilizing protocols like TCP/IP to guarantee data transfer reliability, order, and integrity.
What’s Transport Layer?
The Transport Layer provides end-to-end communication services for applications within a layered architecture of network components and protocols. Its primary responsibilities include:
- Connection-based data transport: ensure data pieces arrive at their intended destination.
- Data reliability: confirmation or retries if any error occurred during transmission.
- Flow control: managing the rate of data transmission, avoiding sender-overloading the receiver with data.
- Multiplexing: allowing multiple application processes to be conducted simultaneously across the network.
The 3-Way Handshake Mechanism
The functional stages of the 3-way handshake mechanism are:
1. SYN: The client sends a SYN (synchronization) packet to inquire whether the server is open for new connections.
xxxxxxxxxx
packet_1 = Client_SYN
2. SYN-ACK: Upon receipt of the SYN, the server responds with a SYN-ACK (synchronization acknowledged) packet asserting its openness to connect.
xxxxxxxxxx
packet_2 = Server_SYN-ACK
3. ACK: Lastly, the client sends an ACK (acknowledgment) back to the server confirming receipt of SYN-ACK, thus establishing a successful connection.
xxxxxxxxxx
packet_3 = Client_ACK
These interaction phases facilitate secure connections and synchronize sequence numbers before data transmission begins.
Stage | Description |
---|---|
SYN | Client sends synchronization request to server. |
SYN-ACK | Server acknowledges and responds to client’s request. |
ACK | Client confirms acknowledgment, establishing the connection. |
Overall, the 3-way handshake’s integral role at the Transport Layer deems it indispensable for reliable, efficient network communication. It guarantees data integrity and delivery order, while also implementing flow control and multiplexing. Ensuring comprehensive understanding of this process enables better grasp of network functionalities and connection protocols.
Additionally, appreciating the 3-way handshake reinforces awareness of cyber threats that may exploit such connection establishment methods. For instance, reflective distortion of this process can result in a SYN flood attack (Cloudflare), emphasizing the need for meticulous attention to network security aspects.
For detailed code examples and exploration of the 3-way handshake in various networking scenarios, feel free to dive into this comprehensive resource by the Internet Engineering Task Force: (IETF RFC 793).During a TCP/IP network connection process, there’s an indispensable protocol known as the 3-Way Handshake process. This technique plays a crucial role in establishing a reliable end-to-end communication. It falls into layer 4, otherwise known as the Transport Layer of both the OSI and TCP/IP models.
Understanding the stages of this three-way handshake will provide a profound insight into how connections are established between computers on a network.
Stage One: SYN Package
The handshake kicks off with the ‘SYN’ (synchronize) packet sent by the client to the server. This packet communicates to the server that the client wants to establish a connection. The client independently sets a sequence number (let’s say X), which will be used throughout the communication session.
xxxxxxxxxx
Client ---------------SYN(X)-----------------> Server
Stage Two: SYN-ACK package
On receiving the SYN packet from the client, the server acknowledges this request by sending back a ‘SYN-ACK’ packet. The ACK part carries the acknowledgment number (X+1). At this stage, another sequence number is also generated by the server, let’s denote it Y.
xxxxxxxxxx
Client <--------------SYN(Y), ACK(X+1)-------- Server
Stage Three: ACK package
Finally, the client takes its turn and sends an ACK (acknowledgment) packet to the server. This contains the incremented server's sequence number (Y+1).
xxxxxxxxxx
Client ------------------ACK(Y+1)--------------> Server
At the end of these three stages, both the client and the server have agreed and confirmed each other's initial sequence numbers. This way, a full-duplex communication link is established for further data transmission over the network.
Notably, all this action happens at the Transport Layer (Layer 4), specifically using the Transmission Control Protocol (TCP). The TCP cares about ensuring that the packets get from the sender to the recipient reliably and in the right order. The 3-way handshake is one of its core strategies to meet these objectives.
For more details about the 3-way handshake process along with a precise explanation of transport protocols like TCP and UDP, refer to Cisco's networking academy study material here.
To visualize the different steps in the 3-way handshake, here is a basic table:
Step | Action |
---|---|
1 | Client sends SYN packet to server |
2 | Server responds with SYN-ACK packet |
3 | Client sends ACK packet to server |
And here's how we might represent the three-way handshake in Python using the socket module:
xxxxxxxxxx
import socket
# Create a socket object
s = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
# Define the server address and port
server_address = ("localhost", 12345)
# Stage 1: Send SYN packet
s.connect(server_address)
# Stage 2 and 3 handled automatically by TCP/IP stack!
# Perform data transmission here...
# Close the connection
s.close()
This code simply outlines the part the programmer has control over - initiating the handshake (the SYN packet). The rest of the handshake process (stages 2 and 3) are handled by the underlying TCP/IP implementation.The 3-way handshake in TCP/IP networking, specifically involving the SYN, SYN-ACK, and ACK packets, is a requisite protocol for establishing connections between computers that happens at the transport layer.
Firstly, let's examine these three distinct certificate types:
• SYN: Short for "synchronize", this packet is dispatched by a client who wishes to initiate a connection with a server. The SYN flag's bit is set to 1 in the TCP header of the initial packet, indicating the intent to establish a new connection.1
• SYN-ACK: This is the response from the server back to the client after receiving the SYN packet. The bytes received do not contain any actual data, instead it serves as an acknowledgment of the SYN request, hence the name "SYN-ACK". The SYN and acknowledgment flags in the TCP header are both set to 1, signifying that it's ready to synchronize and acknowledging the prior message.2
• ACK: Finally, the "ACK" or Acknowledgment packet marks the conclusion of the 3-way handshake. It's sent from the client back to the server, once more reaffirming receipt of the SYN-ACK packet. Once both sides have exchanged these messages, they're primed to transmit data.3
xxxxxxxxxx
Client:SYN ------->
Server:<------ SYN-ACK
Client:ACK ------->
In the context of TCP/IP model layers, the 3-way handshake operates at the transport layer—specifically layer 4.4 Remember that the primary function here is transmission management, accounting for functions such as segmentation, multiplexing, flow control, error detection, and correction. Naturally, the SYN, SYN-ACK, and ACK packets which make up the 3-way handshake fall into this category, forming the foundation for reliable and virtual point-to-point connections.5
In conclusion, the 3-way handshake is crucial to network communications and fundamental to the transfer of data in TCP/IP networking. Understanding how it operates provides valuable insight into harnessing its full potential and safeguarding against common threats when programming within the constraints of the transport layer.
Decoding the significance of sequence numbers in reliable communication specificaly involves examining an integral part of the Transmission Control Protocol (TCP) often termed TCP Three-Way Handshake process, which takes place at the Transport Layer.
To set light upon why this mechanism is so pivotal and how it pertains to reliable communication, let's begin by understanding what precisely this term 'Three-Way Handshake' denotes.
The 3-Way Handshake is a networking method used for establishing connections in TCP/IP based network systems. This connection ensures that data packets reach their intended destinations without errors or packet loss, making way for reliable communication.
Diving deep into the mechanics of this dependable connection gives us these steps:
* Step 1 - SYN: The client wants to establish a connection with the server and sends a segment with SYN(Synchronize Sequence Number)
* Step 2 - SYN-ACK: The server acknowledges the client's SYN request and responds with its own SYN request
* Step 3 - ACK: The client receives the server's SYN request and acknowledges it, thus completing the handshake
The sequence numbers hold intrinsic value in sorting out two particular concerns involved with TCP:
Data Sequencing
In TCP, the sender could transmit several packets at a single time for efficiency, each stamped with different sequence numbers. Hence, if the data packets arrive in an incorrect order, TCP can rearrange them using these sequence numbers.
Error Checking & Retransmission
If a packet gets lost during transmission, the receiver will understand this because it won’t receive the expected sequence number within the anticipated timeframe. It is then able to send a re-transmission request to the sender.
Code-wise, analyzing sequence numbers looks something like this (ethereal output of a captured packet):
xxxxxxxxxx
Frame 4 (62 bytes on wire, 62 bytes captured)
Ethernet II, Src: Compaq_59:6c:de (00:50:8b:59:6c:de), Dst: Cisco_d4:0a:f1
(00:10:7b:d4:0a:f1)
Internet Protocol, Src Addr: 192.168.1.102 (192.168.1.102), Dst Addr:
172.16.120.50 (172.16.120.50)
Transmission Control Protocol, Src Port: 3110 (3110), Dst Port: 23 (23),
Seq: 256590566, Ack: 2591832009, Len: 0
Here are some HTML tables illustrating the SYN, SYN-ACK, and ACK as well as sequence numbers.
Packet Type | Description |
---|---|
SYN | Client wants to establish a connection and sends a segment with a sequence number |
SYN-ACK | The server acknowledges the client's SYN, responds with its own SYN |
ACK | Client receives the server's SYN, responds with an acknowledgement. |
Field | Value |
---|---|
Source Port | 3110 |
Destination Port | 23 |
Sequence Number | 256590566 |
Acknowledgement Number | 2591832009 |
Length | 0 |
Finally, remembering the 3-way handshake happens at the Transport Layer of the OSI model or the Internet layer of the TCP/IP model is fundamental. Underlining where the three-way handshake function is essential since these models provide an understanding of how different network protocols interact and work together to provide network services (source).
Session establishment on the internet typically leverages a process called a 3-Way Handshake, which is the backbone of the Transmission Control Protocol (TCP). TCP operates at the Transport Layer of the Internet Protocol Suite, more colloquially known as TCP/IP. The Transport Layer is Layer 4 in the Open Systems Interconnection (OSI) model, a theoretical framework for understanding network interactions.
Understanding the 3-Way Handshake
A 3-Way Handshake functions with three primary steps: SYN, SYN-ACK, and ACK, which stand for synchronize, synchronize-acknowledge, and acknowledge respectively - thus forming the foundation of a TCP connection.
Step | Description |
---|---|
SYN | The client or initiating host sends a SYN request to the host that it wishes to establish a connection with. |
SYN-ACK | The server or receiving host sends back a synchronization acknowledgment by adjusting the sequence number. |
ACK | The client sends an acknowledgment message back to the host, completing the handshake and establishing the connection. |
Spotlight on the Transport Layer and TCP 3-Way Handshake
TCP being categorised within the Transport layer (Layer 4) of the OSI stack makes this layer responsible for the provision of end-to-end communication services for applications. It is tasked with handling the error-free transmission of segments between points on a network. This layer bears the responsibility for flow control (ensuring that data flows at a rate where it can be processed) and sequencing (ensuring that packets transmitted across the network arrive in order).
xxxxxxxxxx
Pseudo-code overview of the TCP 3-way handshake process:
1. c = Socket() // Client opens a TCP socket
2. c.SYN(s) // Client sends a SYN request to server s
3. s.SYN_ACK(c) // Server responds with SYN-ACK
4. c.ACK(s) // Client acknowledges with an ACK
5. The connection is established
In context of networks, TCP's 3-Way Handshake proves essential because it establishes a connection that is reliable, ordered and error-checked, which are critical attributes when transmitting data across the web. The initiating device requires assurance that its packets will reach their destination, while the receiving device requires assurance that it will receive these packets in order. For coders, developers and network administrators, understanding this mechanism is vital to troubleshooting and ensuring clean data transfer across all digital communications.
The Transmission Control Protocol (TCP) Three-way Handshake is an essential process in network communication. It occurs in the Transport Layer (Layer 4) of the Internet Protocol Suite, often referred to as the OSI model.
The importance of understanding error detection measures and flow control in connections becomes evident when delving into the workings of TCP and its Three-way Handshake. This technique ensures data transfer is successful, secure, and error-free - a vital requirement for the seamless operation of web applications, file transfers, emails, and more.
Error Detection Measures
Error detection plays a significant role in ensuring the integrity of data transmissions. A core mechanism used by TCP for error detection is the checksum - a simple yet effective computational method. In this method, the sending device calculates a value based on the packet's content and includes it in the packet header before transmission.
xxxxxxxxxx
Data = "Hello"
CheckSum = sum(ASCII values of 'H','e','l','l','o')
Packet = Data + CheckSum
Upon receipt, the receiving device performs the same calculation on the incoming data and compares it with the received checksum. If there is inconsistency, an error is detected, triggering re-transmission.
In the case of TCP Three-way Handshake:
- SYN packet (Step 1 & Step 2) and ACK packet (Step 3) should arrive without errors.
- If the checksum validation fails for any of these packets, the handshake will fail, ultimately causing failure in establishing the connection.[source]
Flow Control
Flow control is another critical component for meaningful communication over networks. It controls the data rate for reliable transmission, preventing the sender from overwhelming the receiver with too much data at once.
In TCP, one principal method for flow control is the sliding window system. This mechanism determines the quantity of data segments that might be sent before receiving an acknowledgement from the recipient.
Using TCP Three-way Handshake as an illustration:
- In Step-2 of the handshake, along with SYN-ACK packet, the receiver sends window size.
- This window size informs the sender how many bytes can be sent without flooding the receiver's buffer.[source]
To summarize, both error detection measures and flow control have high significance in handling TCP connections, enhancing their efficiency, integrity, and reliability. Without these, the three-way handshake, which forms the foundation of all TCP connections, would not function correctly or effectively. Therefore, these concepts are invaluable for everyone working within networking or involves communication protocols.The three-way handshake protocol is an integral part of the Transmission Control Protocol (TCP) which operates at the transport layer (layer 4) of both the seven-layer OSI model and the five-layer TCP/IP model. This protocol allows for the establishment of a reliable connection-oriented communication between two network hosts. The three steps involved in this process are:
- Sending a SYN (synchronizing) packet
- Responding with a SYN-ACK (synchronization acknowledged) packet
- Sending an ACK (acknowledgement) packet in return
These steps can be represented in pseudo code as follows:
xxxxxxxxxx
(function() {
const sender = new TCPSender();
const receiver = new TCPReceiver();
// Step 1: Sender sends SYN packet to Receiver
sender.sendPacket('SYN');
// Step 2: Receiver responds with a SYN-ACK packet
receiver.receivePacket('SYN');
receiver.sendPacket('SYN-ACK');
// Step 3: Sender sends an ACK packet to receiver
sender.receivePacket('SYN-ACK');
sender.sendPacket('ACK');
})();
Please note that this represents a simple demonstration of the protocol’s working, while an actual implementation might involve more details like handling time-outs, sequence numbers, etc.
This process enables the reliable transfer of data across networks, as it ensures that the receiving end is ready and capable of accepting data before transmission begins. Major popular real-time implementations of the three-way handshake protocol can be found in connections using HTTP(S), FTP, SMTP, and more.
For instance, a visit to a web page initiates this process in the background as follows:
- Your browser (client-side) sends a SYN packet to the server hosting the webpage.
- The server acknowledges the request by issuing a SYN-ACK packet back to your browser.
- Finally, your browser sends an ACK packet back to the server, confirming the successful establishment of a connection.
This happens unseen every single time you click on a link or type a URL into your address bar! The power mid-protocol binding helps in mitigating several potential issues including retransmissions, out-of-order delivery, and congestion control.
To check the details of this process, network utilities like Wireshark (source) can capture and display all these packets transmitted over your network.
Let's illustrate what a typical three-way handshake process may resemble through Wireshark:
xxxxxxxxxx
// typically displayed information
No. Time Source Destination Protocol Info
1 0.000000 192.168.1.2 93.184.216.34 TCP 49152 > http [SYN] Seq=0 Win=8192 Len=0 MSS=1460
2 0.005225 93.184.216.34 192.168.1.2 TCP http > 49152 [SYN, ACK] Seq=0 Ack=1 Win=5792 Len=0 MSS=1460
3 0.005375 192.168.1.2 93.184.216.34 TCP 49152 > http [ACK] Seq=1 Ack=1 Win=8208 Len=0
Addressed above, understanding the real-time implementation of the three-way handshake protocol adds another layer to our comprehension of what's really happening when we interact with networked devices and services.
Remember, this existing protocol plays an essential role in our digital lives, supporting the backbone of internet usability from commercial applications like online banking, emails, social media, browsing websites down to modern IoT implementations. Hence, knowing such foundational interaction directly corresponds to diverse fields like troubleshooting, performance optimization, security enhancements, and more.Sure, let's explore the data security implications during a three-way TCP Reset attack (RST attack), and how to prevent them. We're going to relate this back to which layer of the OSI model the three-way handshake process occurs.
Data Security Implications
A three-way TCP Reset Attack (RST attack) can have serious implications for data security:
- A successfully executed RST attack can disrupt or terminate an established TCP connection, leading to potential data loss.
- This type of attack can prevent access to important websites or online services by disrupting the underlying TCP connections. In turn, this could impact ecommerce transactions, user interactions, or other essential activities.
- It may be used as part of a larger Denial-of-service (DoS) attack, aimed at overwhelming a server with traffic until it is no longer operational.
Now, on to discuss the prevention strategies.
Prevention Strategies
The following are proven strategies that can help prevent a three-way TCP Reset Attack:
- Packet Filtering: Packet filters inspects packets as they are transmitted across a network. Potentially harmful packets — like those that might be used in a RST attack — can be identified and stopped based on their IP address, packet size, or other characteristics.
xxxxxxxxxx
// Sample packet filtering code:
if (packet.getTCP().isRst()) {
return false;
}
- Firewalls: A firewall meticulously analyzes incoming and outgoing packets to keep out malicious traffic like that from a potential RST attack. Firewalls can stop these attacks by blocking ints sustaining traffic once detection has occured.
xxxxxxxxxx
// Sample firewall rule code:
add rule ip filter FORWARD 'tcp.flags & (SYN|RST) == SYN|RST drop
- Monitoring and Alerts: Effective system monitoring and alert mechanisms can notify relevant personnel when an unexpected number of TCP resets or unusual traffic patterns are detected, indicating a possible ongoing attack. Intrusion Detection Systems (IDS) and Intrusion Prevention Systems (IPS) can also be a fruitful approach for it.
xxxxxxxxxx
// Sample IDS alert trigger code:
if (rstCount > THRESHOLD) {
alert('Potential RST attack detected');
}
In addition to the above, regular vulnerability scans and updates to devices protecting the network will ensure readiness for newer threats, including evolved versions of three-way TCP Reset attacks.
The 3-Way Handshake and OSI Model
Let's connect all the discussed concepts back to the OSI Networking model. The three-way handshake — a foundational process that establishes the parameters of a TCP connection between two computers — happens at the Transport Layer of the Open Systems Interconnection (OSI) model. This is the fourth layer of the model, which manages node-to-node communication and controls transmission procedures.
So, how does this relate to our discussion on RST attacks? Well, performing checks and security measures at the transport layer would directly counteract these types of attacks. Any preventative measures — such as implementing firewalls, effective sys-log monitoring, Intrusion Detection System( IDS ), IPS, and packet filtration — should be placed in this 4th layer itself for optimal performance.
To understand this better, here is a reminder of the 7 layers of the OSI model and what functions they perform, tabled below:
Layer | Name | Functions |
---|---|---|
7 | Application | Email, Web browser, Network games, etc., |
6 | Presentation | Encryption, Decryption, Data compression, etc., |
5 | Session | Establishes, Manages and Terminates connections between applications. |
4 | Transport | Transmission control protocol (TCP), Establishes, maintains and ends virtual circuits for information transport. |
3 | Network | Handles routing and transferring data from one network to another. |
2 | Data Link | Transfers data between network entities and provides error detection. |
1 | Physical | The connection to the transmission medium. It specifies electrical/optical, mechanical, and functional interface to the physical medium. |
Reference : OSI Model - Wikipedia
In conclusion, understanding the implications of a Three Way TCP Reset (RST) attack, its prevention methods, and the relevant OSI Networking layer is integral to maintaining secure data transmission over networks. The most effective way to prepare for and prevent such attacks necessarily begins at the Transport layer where the 3-way handshake originates, reducing the likelihood of a successful TCP RST attack.The 3-way handshake is an integral aspect of the TCP/IP network model, predominantly occurring at the transport layer. This handshake process focuses on starting a session between the server and client, ensuring seamless data transmission. Essentially, this three-step pillar involves:
xxxxxxxxxx
1. A SYM message from the client to the server.
2. The server responding with a SYN-ACK message.
3. The client replying with an ACK response, subsequently subjecting the server to respond accordingly.
Perhaps one of the most significant threats that the 3-way handshake faces is the SYN Flood attack. Operating as a kind of Denial of Service(DoS) attack, the attacker sends a huge volume of SYN packets to the target’s system and strategically abandons the process at the second step of the handshake. How does this affect the system?
- The server continues to wait for a response from the client's end, inevitably leading to queues' congestion.
- Over time, authentic users struggle to establish fresh sessions, causing service denial. Consequently, it can cause substantial downtime and potentially severe damage to online services or web applications.
Mitigating this SYN flood threat requires comprehensive and innovative strategies. Incorporating tools such as firewalls proves beneficial—firewall configure rules limit the number of simultaneous SYN_RECV connections or SYN packet rates, thus reducing the chance of server saturation.
Another technique frequently used to mitigate SYN flood attacks is SYN cookies. This method doesn't use the memory space to store information about each SYN packet received; instead, it embeds the necessary details into the TCP sequence number included in the SYN-ACK.
Implementing these defensive protocols contributes to protecting businesses from catastrophic downtimes triggered by DoS attacks. Nonetheless, caution must be exercised owing to the ever-evolving nature of cyber threats. Continuous auditing and rigorous debugging stand crucial towards realizing ideal systems hygiene.
Developers can also make use of rate limiting, which will restrict the amount of incoming connection requests to a level your server can handle.
A pseudo-code could look like :
xxxxxxxxxx
if incoming_connections > your_limit
drop_packet()
else
establish_connection()
Apart from just mitigating SYN flood attacks, adopting a multilayered security architecture also helps deter security issues that might exploit other TCP/IP Model layers vulnerabilities. Comprehensive knowledge of all these meticulously interweaved strategies ameliorates our approaches towards providing robust cybersecurity solutions.
Sources:
The Three-Way Handshake definition,
What is SYN Flood Attack,
Preventing SYN Flood Attacks.
Simultaneous opening functions, referred to as the TCP (Transmission Control Protocol) three-way handshake, is a critical aspect of establishing a connection between two devices within a network. Explained in detail within the RFC793 document1, this function becomes evident at Layer 4 - Transport layer of the OSI model.
The Open Function & How These Functions Interchange
The 'Open' function in Transmission Control Protocol (TCP) is initiated by sending a segment with the SYN control bit set and implicitly advising that the Simplex connection should move from CLOSED state to SYN-SENT state or LISTEN state to its SYN-RECIEVED state
Following is the snippet for initiating the open call:
xxxxxxxxxx
Socket.open()
{
//client sends a syn packet to server
sendSynPacket();
state = STATE.SYN_SENT;
}
SYN
Once the open call has performed its duties, it makes use of the Synchronize (SYN) flag, TCP's control bit used when first establishing connections. The SYN can be perceived as a request sent From node A to B, declaring the sender's initial sequence number and requesting a connection.
xxxxxxxxxx
Socket.sendSynPacket()
{
//creates syn packet
Pack syn = createSynPack(localIp, remoteIp, localPort, remotePort);
send(syn);
}
ACK
Following this, an Acknowledgement (ACK) is then received from the receiving system and the acknowledgement number field is set to one more than the received sequence number.
This snippet marks the receipt of ack packet and changes the status to ESTABLISHED
xxxxxxxxxx
Sockets.onPackReceived (Pack pack)
{
if(pack.isAck && state == STATE.SYN_SENT)
{
//server recieved acknowlgement to the sent syn packet
state = STATE.ESTABLISHED;
}
}
The Role of SEQ and ACK Numbers
Both the SEQ and ACK numbers are paired with a second SET control bit, working together to complete the three-way handshake process using a synchronized sequence.
This process involves:
- Step 1: Sending a SYN message containing a random sequence number A from node A to B.
- Step 2: Responding with an ACK signal back to node A, confirming receipt of SYN message.
- Step 3: Node B now initiates its own SYN message to node A, containing another random sequence number B.
- Step 4: Finally, node A responds with an ACK, acknowledging it received node B's SYN message.
Each data segment has 32 bits allocated for both the SEQ and ACK fields. The sequence number identifies the order of bytes sent from each device so that the data can be arranged in the correct order, whereas the acknowledgement number acts as a receipt notifying the sender that the respective byte has been successfully accepted.
Upon completion, we achieve a fully connected and synchronized communication line between the two nodes where they continue to update and acknowledge each other's sequencing throughout the exchange of information. A table summary better illustrates the sequence of actions during the handshake.
Step | Node A | Node B |
---|---|---|
Step 1 |
xxxxxxxxxx 1 1 1 state = SYN-SENT; seg.seq = x; |
|
Step 2 |
xxxxxxxxxx 1 1 1 state = SYN-RECEIVED; seg.ack = x + 1; seg.seq = y |
|
Step 3 |
xxxxxxxxxx 1 1 1 state = ESTABLISHED; seg.ack = y + 1 |
The three-way handshake method provided by TCP allows for a reliable, ordered and error-checked delivery of a stream of bytes from one program on one computer to another program on another computer, making this handshake an essential part of layered network architectures and their corresponding protocols such as HTTPs.As an experienced coder, understanding the difference between active-open connections and passive-open connections in terms of the OSI (Open Systems Interconnection) model is almost as crucial as writing effective lines of code. This technical terminologies can sometimes seem like they're shrouded in complexity, but in reality, they're quite straightforward.
The OSI model is composed of seven layers, with each playing a distinct role in network communications. The 3-way handshake, an integral part of establishing TCP (Transmission Control Protocol) connections, occurs at the Transport Layer of the OSI model, or layer 4.
An Active-Open connection exists when a system initiates a connection actively. It sends a SYN packet to request a connection with another system on a specific port. The system on the receiving side will then respond with an acknowledgment packet, also known as a SYN-ACK. Finally, the initiating system will reply with an ACK, completing the three-way handshake.
Here's an example demonstration shows the creation of an Active-Open connection:
xxxxxxxxxx
socket = socket(AF_INET, SOCK_STREAM, 0);
connect(socket, (struct sockaddr*)&server_address, sizeof(server_address));
On the flip side, a Passive-Open connection refers to those instances where a system is ready to accept incoming connections, rather than initiating them outwardly. The system waits for incoming SYN packets to commence a connection. If such a message arrives, the same three-way handshake process transpires from its perspective.
Here's a demonstration creating a Passive-Open connection:
xxxxxxxxxx
listener = socket(AF_INET, SOCK_STREAM, 0);
bind(listener, (struct sockaddr*)&server_address, sizeof(server_address));
listen(listener, 5);
socket = accept(listener, NULL, NULL);
To further visualize this, let me provide you with another way of representing it.
Active Open Connection | Passive Open Connection |
---|---|
|
|
To wrap up, it's important to remember that neither the active-open nor passive-open state inherently designates the client or server role within a communication. Both systems involved in a transaction can simultaneously have active-open connections to different ports.
Finally, if you want to understand more about the intricacies of these processes and their roles in layer 4 of the OSI model, online resources like ["TCP/IP Guide"](http://www.tcpipguide.com) can offer deeper dives into the matter. Whether you need to make sense of the SYN flooding attack, review window scaling options, or simply better comprehend the nature of your network connections, such well-documented resources can be invaluable.
The TCP three-way handshake is a process used to create a TCP connection between two network hosts. As the name suggests, it involves three steps: SYN (synchronize), SYN-ACK (synchronize-acknowledge), and ACK(acknowledge). This handshake happens at the Transport Layer of the OSI Model.
There are notable challenges with the TCP three-way handshake that you should be aware of:
- SYN Flood Attacks: A SYN flood attack involves an attacker sending a burst of SYN requests to a target's system in an attempt to consume enough server resources to make the system unresponsive to legitimate traffic.source
- IP Spoofing: An attacker could fake the source IP address during the handshake process to create a false connection or to induce the hosts into sending data into a void. This spoofing action can be quite harmful given that the trust is usually based on IP addresses.source
- Port Scanning: Although not a problem with the handshake itself, the TCP three-way handshake can be abused via port scanning - a popular reconnaissance technique where attackers send requests to multiple ports and analyze responses to find out which services are available and ripe for exploitation.source
Such issues arise mainly due to the trust-oriented design of the TCP three-way handshake. The Transport Layer has less control over who sends the data and relies on Internet Protocol (IP) security mechanisms to ensure the authenticity and integrity of the data packets.
In terms of working with these challenges, mitigating strategies exist at various layers including but not limited to the Transport Layer. For instance, SYN cookies can be applied as a countermeasure against SYN flood attacks, IPSEC can help protect against IP spoofing, and Intrusion Detection Systems (IDS) can help detect unusual port scanning activities etc.source
Here is an example of a simple TCP Handshake using
xxxxxxxxxx
socket
library in Python:
xxxxxxxxxx
import socket
s = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
s.bind(("localhost", 9999))
s.listen(1)
print("Listening on port 9999... ")
while True:
conn, addr = s.accept()
print('Connected by', addr)
data = conn.recv(1024)
if not data: break
conn.sendall(data)
conn.close()
In this code, we're creating a simple server that listens on port 9999 for any incoming connections. When a client connects, it waits for the client to send some data and then it sends back that same data. This repeats until the client stops sending data.
Name | Description |
---|---|
SYN | A synchronize message to initiate a communication |
SYN-ACK | An acknowledgement message for receiving a SYN |
ACK | An acknowledgement message sent from the initiating party after receiving a SYN-ACK |
In the world of cybersecurity, understanding these above potential challenges associated with TCP's Three-way handshake is crucial and helps in building and securing robust systems while identifying vulnerabilities in the early stages of software development practice.
Emerging trends and developments in connection protocols, particularly regarding the transport layer of the OSI model where the process of a 3-way handshake occurs are becoming more evident with advancements in Information Technology.
The Transport layer of the OSI (Open Systems Interconnection) model, The layer that is home to the infamous 3-way handshake, plays a critical role in data communication between hosts. It uses protocols like TCP and UDP and offers services such as connection-oriented communication, reliability, flow control, and multiplexing.
xxxxxxxxxx
TCP Server TCP Client
--------- -----------
Listen<----------->SYN(Synchronize sequence numbers)
SYN+ACK ------------> SYN
------------------->ACK(Acknowledgment)
Substantial improvements have been made among these traditional protocols, leading to the emergence of advanced features aimed at boosting performance and security. Let's look at some of the recent trends and developments:
QUIC (Quick UDP Internet Connections): As an emerging trend in connection protocols, QUIC is developed by Google. It aims to improve web browsing speed by reducing latency and providing better encryption for secure connections. QUIC replaces TCP in the transport layer and uses UDP instead to achieve faster transmission, consequently enhancing the user experience.
For further insights, you can read [online](https://blog.cloudflare.com/the-road-to-quic/).
Using QUIC, you would perform the handshake this way:
xxxxxxxxxx
Client ----------> SYN [ClientHello||EarlyData]
Server ----------> SYN+ACK [ServerHello...EncryptedExtensions...Finished] + 1-RTT Key (avoiding the usual second round trip in TLS handshake)
Client ----------> ACK [Finished]
TLS 1.3 Protocol: Another prominent update in connection protocols is the newly introduced TLS 1.3 protocol. It is aiming to enhance privacy and performance compared to its predecessor, TLS 1.2. Significant changes include shortened handshake time and simplified negotiation process, thus speeding up data transmission.
When using TLS 1.3, the 3-way handshake will look a bit like this:
xxxxxxxxxx
Client ----------> SYN [ClientHello...Pre_shared_key]
Server ----------> SYN+ACK [ServerHello...EncryptedExtensions...Finished]
Client ----------> ACK [Finished]
There are also other enhancing developments coming up like Multipath TCP (MPTCP), LEDBAT for buffering issues, TCPinc for encryption purposes, etc.
Remember that while older-generation protocols may still dominate today's digital landscape, staying informed about these emerging trends provides me a competitive edge in the ever-evolving field of technology. It is essential not only for improving my craft but also contributing to the collective growth of our digital society.
Here is a table summary:
Protocol | Description | Used In |
---|---|---|
QUIC | Reduces connection establishment time, resilient to packet loss, secure and congestion control. | Primarily used by Google websites |
TLS 1.3 | Better privacy, simpler and faster than TLS 1.2 for encrypted communications. | HTTPS servers and other TLS-dependent services. |
Multipath TCP | Combining multiple internet connections | Situations where multiple end-to-end paths are required |
Diving deep into the fascinating topic of the 3-way handshake, it's clear that to fully grasp its essence, knowing the layer in which it operates is vital. The answer lies in the Transport Layer; this network layer, a crucial link in the OSI model, is where the 3-way handshake unfolds.
The Transport Layer, or Layer 4 of the OSI model, accommodates the vigorous nature of the 3-way handshake protocol. This layer carries out a significant role in delivering communication services directly to the application processes on a network. Its functions hugely impact the data flow over the network.
xxxxxxxxxx
The simple steps of TCP’s 3-way handshake process:
1. SYN: The active open is performed by the client sending a SYN to the server.
2. SYN-ACK: In response, the server replies with a SYN-ACK.
3. ACK: Finally, the client sends an ACK back to the server.
Therefore, the integral constituent element in the initiation of a TCP connection, such as setting up connection-oriented communication, carrying out end-to-end communications, and troubleshooting - all fall under the purview of the Transport Layer.
OSI Model | Role |
---|---|
Application Layer | Interaction with software applications |
Presentation Layer | Translation of data between network and application |
Session Layer | Managing communication sessions |
Transport Layer (3-way Handshake) | Reliable transmission of data segments |
Network Layer | Routing of different networks |
Data Link Layer | Transfer data between network entities |
Physical Layer | Physical connection between devices |
So, optimize your understanding of networking by acquainting yourself with these concepts. To improve SEO understanding make sure to study about '3-way handshake' and 'Transport layer'.