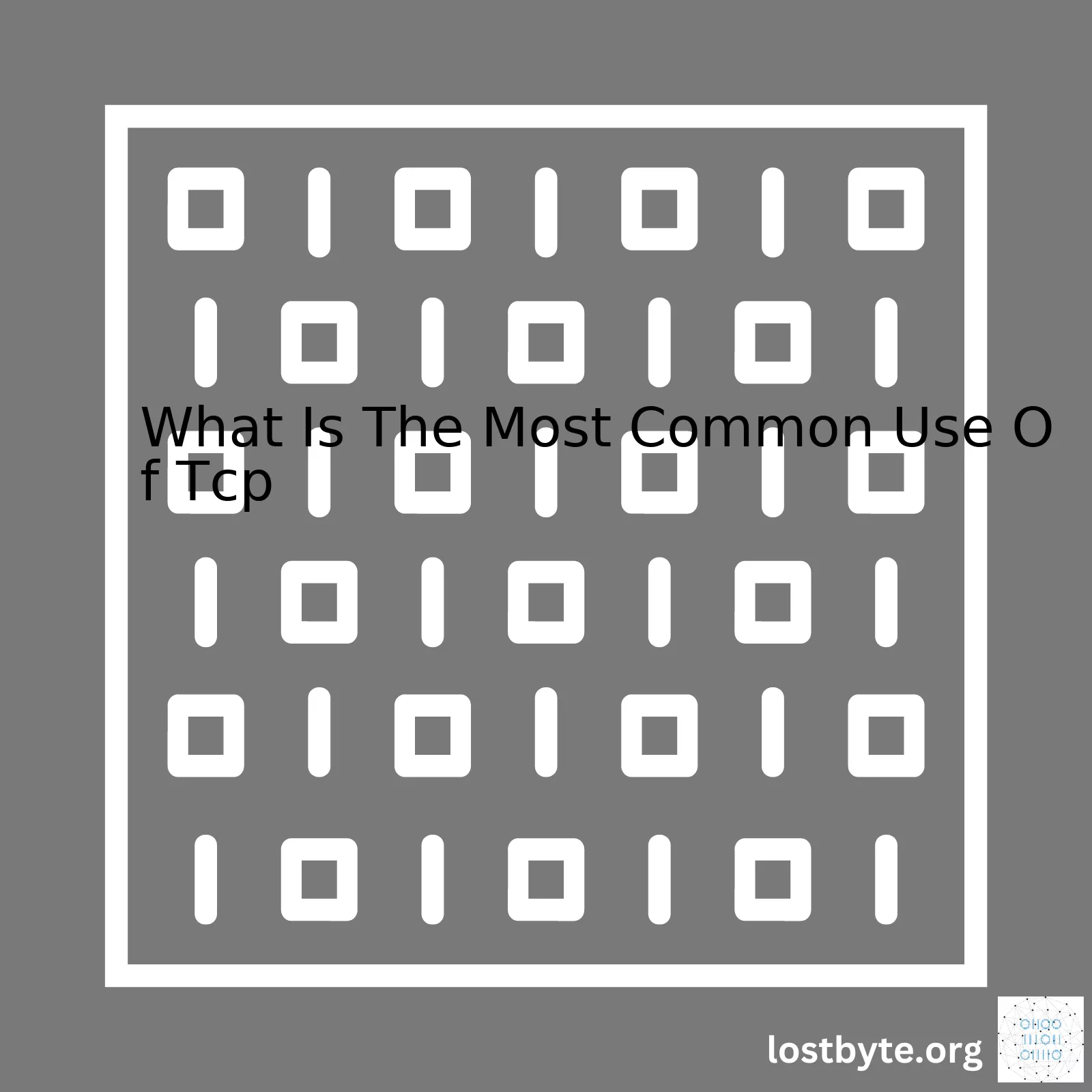
Common Uses | Description |
---|---|
Email Protocols (SMTP, IMAP, POP) | TCP is used to send and retrieve emails. |
File Transfers (FTP) | This protocol provides reliable file transfers due to the TCP’s ability to check for errors and correct them. |
Web Browsing (HTTP, HTTPS) | TCP ensures that data packets are received in the correct order when browsing the web or loading a webpage. |
Secure Shell (SSH) | TCP is used by SSH for remote administration, secure file transfer, and command-line login. |
The Transmission Control Protocol or TCP is one of the main protocols used in the world of internet networking. Its common uses can be categorized into four broad sectors – email protocols like SMTP, IMAP, and POP; file transfers via FTP; web browsing through HTTP/HTTPS, and the Secure Shell (SSH).
For email protocols like SMTP, IMAP, and POP, TCP is used for sending and retrieving emails. Each of these protocols performs different tasks with Simple Mail Transfer Protocol (SMTP) used for email transmission, while Post Office Protocol (POP) and Internet Message Access Protocol (IMAP) are used for email retrieval.
When it comes to transferring files, FTP which stands for File Transfer Protocol makes use of TCP. What makes TCP advantageous for FTP is its ability to check for any inconsistencies or errors during the transmission process. It then appropriately handles these errors, ensuring that the file transfer is accurate and consistent.
In terms of web browsing, TCP is key to the operation of HTTP and HTTPS protocols. Whether you’re simply browsing the web or loading complex web pages, TCP ensures that all data packets are received as intended, in the correct sequence, regardless of their path over the network. This attribute is why almost all web traffic utilizes TCP.
Finally, TCP also plays a significant role in Secure Shell (SSH) connections. SSH uses TCP for remote administration, secure file transfers, and command-line logins. It provides a secure channel between local and remote computers, where TCP assures the reliability of this connection.
Overall, TCP helps to maintain the integrity and consistency of our daily online activities, from reading and sending emails to browsing and downloading from the web. By checking and correcting any error that might occur during transmission, TCP serves as an indispensable part of the internet infrastructure.
For more detailed information about TCP and its various applications, you might find resources like the Geek for Geeks and Cloud flare Glossary helpful.The Transmission Control Protocol (TCP) is an integral part of our digital communication today. It is a set of rules used to send and receive messages at the Internet Protocol (IP) level. The primary function of TCP is to ensure that every byte sent from a source machine gets to the destination machine safely and in the correct order. Furthermore, it establishes a connection using what is known as a ‘three-way handshake’.
Three-Way Handshake:
The three-way handshake sets up a connection by allowing both the client and the server to exchange SYN(synchronize) and ACK(acknowledgment) packets before actual data transmission begins. This process can be presented visually in the following table:
Step | Description |
SYN | The initiating computer sends a SYN to the receiving computer, asking if it’s open for new connections. |
SYN-ACK | If the receiver has open ports that can accept and initiate new connections, it responds with an acknowledgment receipt in the SYN-ACK packet. |
ACK | The initiating computer responds back with an ACK, and this completes the three-way handshake. |
Once the connection is complete, the two machines can exchange data seamlessy.
Common use of TCP:
The most common use of TCP is in transmitting data over the internet, or any network, in a reliable and sequenced manner through applications like HTTP (Hypertext Transfer Protocol), SMTP (Simple Mail Transfer Protocol), FTP (File Transfer Protocol), among others. Below is how you can use TCP with Java for usages like HTTP:
// Setting up a simple TCP client in java Socket tcpClient = new Socket("example.com", 80); // Connecting to example.com on port 80 (default HTTP port) OutputStream out = tcpClient.getOutputStream(); PrintWriter writer = new PrintWriter(out, true); writer.println("GET / HTTP/1.1"); // Sending an HTTP GET request writer.println("Host: example.com"); // Specifying the host writer.println("Connection: Close"); // Closing the connection after the request is processed writer.println(); // Empty line indicates end of request headers InputStream in = tcpClient.getInputStream(); BufferedReader reader = new BufferedReader(new InputStreamReader(in)); String responseLine; while ((responseLine = reader.readLine()) != null) { System.out.println(responseLine); } reader.close(); writer.close(); tcpClient.close();
This code snippet outlines how TCP allows for smooth and standardized communication on the web. As you can see, TCP’s reliability and built-in error checking make it perfect for the needs of internet communication, especially in a world that relies heavily on accurate data.
The Transmission Control Protocol, often abbreviated as TCP, is a core part of the internet protocol suite. TCP operates at a higher level compared to Internet Protocol (IP), providing reliable, ordered, and error-checked delivery of stream data between applications running on hosts that are connected via a network. A TCP connection is established through a three-way handshake and used for transmitting chunks of data (“segments”) from one application to another over a network.
Common uses of TCP include email transmission, web browsing, file transfers among others, but the most prevalent use is likely in the area of web browsing.
- Data Streaming:
Offering a reliable, ordered stream of data, TCP is ideal for delivering these types of content:
- Web pages (HTTP, HTTPS)
- Video and Audio streaming
These days, our lives are filled with multimedia content such as web pages, songs, films, etc., which need proper sequencing for users to understand. Because TCP provides a reliable and sequenced way of data streaming, it’s tremendously crucial in this regard.
// Establishing a standard TCP connection using Go conn, err := net.Dial("tcp", "golang.org:80") if err != nil { // handle error } fmt.Fprintf(conn, "GET / HTTP/1.0\r\n\r\n") status, err := bufio.NewReader(conn).ReadString('\n')
To establish a typical TCP socket connection, connect to a host and send a simple HTTP request as portrayed above.
- File Transfers:
With FTP or File Transfer Protocol, TCP plays an essential role in transferring files from source to destination in a reliable and ordered manner. It ensures the integrity of transferred data. Any corruption or loss of data would result in a problematic file that’s either missing information or isn’t usable.
// Here is a basic sample of establishing an FTP transfer using Python from ftplib import FTP ftp = FTP('example.com') ftp.login(user='username', passwd='password') ftp.cwd('/specific_directory/') filename = 'file_to_download.txt' localfile = open(filename,'wb') ftp.retrbinary('RETR ' + filename, localfile.write)
This simple script connects to an FTP server, logs in, changes into a specific directory, and then downloads a file. It showcases how you can make use of TCP in an application.
Component | Description |
---|---|
Source port | Identifies the sending port |
Destination port | Identifies the receiving port |
Sequence number | A unique identifier assigned to each byte transmitted |
Acknowledgement Number | Indicates the next expected TCP octet |
Data Offset | Specifies the size of the TCP header |
Window size | Notifies the receiver of the amount of space available to receive data |
There are many more areas where TCP shines, but undoubtedly, its dominant role in web browsing and streaming media content displays the sheer importance of TCP in everyday applications that influence our life on the internet. It’s hard to imagine daily life without the dependability and efficacy of TCP. You can delve deeper into this subject by reading this informative guide on TCP-Transmission Control Protocol.
Sure, let’s dive right into understanding the working mechanism of the Transmission Control Protocol (TCP). TCP is an essential part of our digital lives and powers the internet as we know it. This protocol ensures that data sent over the network reaches its destination in the correct sequence and without errors.
Let us breakdown the working mechanism of TCP:
TCP Connection Establishment – The Three-Way Handshake:
To maintain reliability, TCP establishes a strong connection using a process called a three-way handshake. In this process:
- The client sends a SYN packet to the server requesting connection.
- Upon receipt, the server sends a packet back with both SYN and ACK flags set, acknowledging the request.
- In return, the client sends an ACK packet, and the connection is considered established.
This can be illustrated in a table:
Step | From | To | Flag(s) |
---|---|---|---|
1 | Client | Server | SYN |
2 | Server | Client | SYN, ACK |
3 | Client | Server | ACK |
Transmission and Reception of Data:
After the connection, next step is data transmission. In TCP, sender sends a series of bytes. These bytes are encapsulated into packets for transmission. Each byte is assigned a unique sequence number.
When the data is received at the recipient end, TCP implements mechanisms like error checking, acknowledgment of received data segments, ignoring duplicate packets, and the ability to handle retransmission requests.
TCP Connection Termination:
Lastly, when the data transfer is completed, TCP doesn’t leave the connection hanging and appropriately moves onto its termination. It effectively closes down the connection to ensure no unnecessary use of system resources.
In light of the common use of TCP, it acts as the foundation for numerous applications and protocols that form the basis for our everyday internet usage. Most notably, HTTP (HyperText Transfer Protocol) and HTTPS (HTTP Secure), the protocols used for transferring web pages between servers and clients, are built on top of TCP.
GET /index.html HTTP/1.1 Host: www.example.com
This sample HTTP GET request uses TCP for the reliable transportation of this request from the client to the server. Similarly, email protocols such as SMTP (Simple Mail Transport Protocol), IMAP (Internet Message Access Protocol), and POP3 (Post Office Protocol version 3) also utilize TCP technology for reliable communication.
Given the vastness and importance of TCP in the world of network communication, diving deeper into the subject will certainly prove beneficial. For further insights into TCP, check out the original Request for Comments (RFC) document that fully describes TCP online at IETF RFC 793.First, let’s understand what TCP (Transmission Control Protocol) really does. TCP builds a connection for data to be sent over a network, ensuring the packet of information reaches its destination successfully. It establishes a mechanism that helps in error-checking and pinging back a request for re-transmission in case of lost data. Computers and servers use TCP to manage the applications they operate.
Role of TCP in Data Transfer
Here are some specific ways TCP impacts data transfer:
• Connection Establishment – TCP ensures that a connection has been established between sending and receiving devices, initializing data transmission through a three-way handshake mechanism. In brief, the sender sends a signal, the receiver responds affirmatively to this init signal, and finally, the sender acknowledges receipt of this agreement.
Here’s an example of how this is achieved in Python using the socket library:
import socket s = socket.socket(socket.AF_INET, socket.SOCK_STREAM) s.connect(("www.google.com", 80))
• Error-Checking – TCP checks for errors during data transfer. When the recipient gets a packet, it performs a checksum calculation, which it compares with the value sent in the header. If there’s a mismatch indicating an error, a retransmission is requested.
• Data Resending – In common internet communications, packets often get lost on the way because of unstable connections or jammed networks. TCP addresses this problem by performing acknowledgments for every chunk of data; if an acknowledgment isn’t received within a specified period, the data gets resent.
• Flow Control -TCP uses ‘windows’ to enable efficient flow control by dictating how much data can be sent before having to wait for an acknowledgement. This functionality helps prevent the recipient from being overwhelmed by too much data.
The most common use of TCP
The most common use of TCP is undoubtedly in web browsing, specifically HTTP and HTTPS traffic. These protocols transporting web requests and responses from browsers to servers and vice versa run over TCP.
Protocol | Port Number | Description |
---|---|---|
HTTP | 80 | Standard protocol for transmitting unencrypted web content. |
HTTPS | 443 | Secured version of HTTP, widely used for securing web transactions. |
Whenever you type a URL into your browser, an HTTP or HTTPS request gets delivered via a TCP connection. As an older, more complex and robust transport protocol, TCP provides reliable, ordered delivery of a stream of bytes from one program on one computer to another program on another computer.source
For instance, when loading a webpage, if any packets were dropped (which happens frequently), TCP would ensure they were resent and put in the correct order before rendering the page. Without reliable data transmission, modern Internet experience would not be possible.
There is also widespread use in email communication (SMTP, IMAP, POP all use TCP), FTP for file transfers, and Secure Shell (SSH) for secure remote logins among other applications.Understanding TCP (Transmission Control Protocol) reliability is crucial considering its widespread use in internet traffic, especially, when it’s used for data transfer where accuracy is paramount. The most common use of TCP, beyond any doubt, is the HTTP (Hyper Text Transfer Protocol), which powers our daily use of the World Wide Web source.
To provide a deeper insight:
TCP Reliability Features
TCP achieves reliability through a host of complex mechanisms such as:
• Error Checking: Every TCP segment has a checksum field to verify data integrity.
• Acknowledgement Scheme: This scheme ensures that every packet sent is acknowledged by the receiver. If acknowledgment isn’t received within a predetermined timeframe, the sender resends the packet.
• Sequencing: Each TCP segment is assigned a sequence number. This allows out-of-order packets to be rearranged by the receiver.
• Flow control: This feature prevents speedy senders from overloading the network or the receiver.
These TCP features are inherently designed to assure data transfer integrity.
TCP and HTTP – A Relationship of Reliability
As stated earlier, one of the most common uses of TCP is to support HTTP, the fundamental protocol for data transfer on the web. It’s HTTP that makes the opening of web pages possible on your browser. Here is how it uses TCP:
Firstly, the HTTP client (like your web browser) opens a TCP connection to the HTTP server (the server hosting the website). Next, the client sends an HTTP request through the TCP connection, asking for a specific data like a web page. After receiving the request, the server sends back an HTTP response carrying the requested data, using the same TCP connection.
Let’s illustrate this with a simplified source code example in Go programming language:
package main import ( "net" "fmt" ) func main() { // Dial TCP conn, _ := net.Dial("tcp", "google.com:80") // Send an HTTP request fmt.Fprintf(conn, "GET / HTTP/1.0\r\n\r\n") // Receive and print HTTP response buff := make([]byte, 1024) n, _ := conn.Read(buff) fmt.Println(string(buff[:n])) }
This simple program demonstrates opening a TCP connection with Google’s website at port 80 (standard HTTP web server port), sending an HTTP GET request for the homepage, and reading the response.
It’s key to highlight that if there’s an error in transmission, thanks to TCP’s reliability features, the faulty package will be retransmitted. This is why when you type “www.google.com” into your browser, you almost certainly get Google’s homepage, instead of some random junk.
In summary, TCP reliability is crucial for maintaining a consistent and reliable internet experience, given its unique ability to provide accurate data delivery. Its most commonly seen through the use of HTTP, ensuring that webpages load correctly, giving us the internet we know and use today.There are a plethora of protocols running our digital world and TCP (Transmission Control Protocol) is one of the most essential among them, playing a key role in ensuring that data transferred across digital networks reach its destination accurately. This protocol primarily functions on the principle of connection-oriented communication, meaning TCP establishes a connection first, transmits the data and then terminates the connection.
How TCP Works To Ensure Delivery of Information:
Fascinatingly, TCP establishes this much-needed error-free channel for data exchange by leveraging three crucial strategies: Sequence Numbers, Acknowledgement (ACK), and Retransmission.
- Sequence numbers: These are unique identifiers assigned by TCP to each byte of data being transferred. When the recipient receives the data segments, they can refer to these sequence numbers to reassemble the data in the right order.
- Acknowledgements (ACK):
These are validation messages sent by the receiving end to confirm that data was received successfully. If the sender does not receive an ACK within a certain timeframe, it assumes that the package was lost or corrupted during transmission and resends the data. - Retransmissions:
If a packet gets lost or corrupted in transmission, TCP detects this via the absence of an expected ACK. The TCP protocol then automatically retransmits the missing or erroneous data to the receiver.
Common Use of TCP:
Content-rich websites – Sites that host a lot of dynamic content like Youtube, Netflix or Facebook, rely heavily on TCP to ensure a high quality user experience.
Let’s shed some light on why TCP becomes indispensable here: Each time you’re streaming a new video, you certainly don’t want frames to be skipped or the voice-over words to disappear intermittently, do you? Your Netflix binge-watching nights would be entirely ruined! That’s exactly where TCP comes into picture with its ability to establish reliable channels for data exchange, hence ensuring complete delivery of information.
A quick snippet of Python’s socket module which uses TCP:
import socket TcpSocket = socket.socket(socket.AF_INET, socket.SOCK_STREAM) host = socket.gethostname() port = 9999 TcpSocket.bind((host, port)) TcpSocket.listen(1) while True: clientsocket,addr = TcpSocket.accept() print("Received connection from %s" % str(addr)) msg = 'Thank you for connecting'+ "\r\n" clientsocket.send(msg.encode('ascii')) clientsocket.close()
This script sets up a server that listens for incoming connections, gracefully welcomes anyone who connects, and closes the connection thereafter.
To summarize, TCP ensures error-free data transmission across networks by implementing checks and balances in form of Sequence numbers, Acknowledgments and Retransmissions. Its most common use is observed in content-rich sites where incorrect delivery or loss of data could ruin the user experience. A great web browsing experience is often the courtesy of this humble protocol diligently doing its job behind the scenes.Sure, let’s delve into the world of Transmission Control Protocol (TCP) and the significance of Three-Way Handshake procedure.
The Transmission Control Protocol (TCP) is primarily used for transmitting data reliably across a network. In the OSI model, TCP sits in the transport layer and is responsible for delivering data from an application program on a computing system to an application program on another one. With TCP, data transmission is reliable because it employs built-in error checking and transmission control protocols.
Three-Way Handshake Procedure:
The Three-way Handshake is a cornerstone of the TCP suite: It establishes a connection between client and server before data transfer begins. This method fosters a connection-oriented communication between the two networks devices.
Here’s how this process works in three steps:
Client -> SYN -> Server Server -> SYN-ACK -> Client Client -> ACK -> Server
In the first step, the client sends a synchronize packet (SYN) to the server over an IP network, which is essentially asking to open a connection.
Second, the server acknowledges the reception of this SYN message by sending back a syn-acknowledgement packet (SYN+ACK) to the client.
Finally, upon receiving the SYN-ACK from the server, the clinet will send the acknowledgement packet (ACK) to the server. The connection between client and server then becomes established, and they are ready to exchange further data.
Most common use of TCP:
The most popular use of TCP protocol is sending and receiving data over the network via applications such as email communications (SMTP protocol), web browsing (HTTP and HTTPS protocols), sharing files (FTP protocol) among others. For instance, when you type www.google.com into your browser, your laptop uses a TCP/IP based request to get the webpage content from Google’s server. Here’s how TCP comes into play:
Browser (www.google.com) -> SYN -> Google's Server Google's Server -> SYN-ACK -> Browser Browser -> ACK -> Google's Server Browser <- HTTP response (Data Transfer) <- Google's server
To summarize, TCP is integral to the functioning of most internet services as we know them. It allows for data to be sent/received in an orderly, error-checked manner so that the associated applications can function seamlessly. The three-way handshake is a key part of establishing these connections, enabling reliable data transfer in TCP/IP network communications.
References:
Three-Way Handshake, Techopedia.
Transmission Control Protocol, Wikipedia.An intrinsic aspect of the intricacies of Transmission Control Protocol (TCP), which commonly application to ensure reliable and ordered delivery of a stream of bytes from one network application to another, is its sophisticated error handling capabilities. Network errors are as inevitable as unplanned downtime - unexpected glitches can account for lost packets, host disconnections, network congestion among others.
TCP Checksum
An initial approach to error control within TCP is the use of checksums. A checksum is a simple mathematical computation applied on the outgoing packets. When packets arrive at their destination, the same summation procedure is conducted again; if the received answer aligns with the original one, data integrity is presumed to have been upheld. If not, the packet is dropped, triggering retransmission algorithms. Consider the following code:
def compute_checksum(message): sum = 0 for i in range(0, len(message), 2): w = ord(message[i]) + (ord(message[i+1]) << 8 ) sum = checksum_add(sum, w) return ~sum & 0xffff def checksum_add(sum, word): sum += word max_uint16 = 0xffff while sum > max_uint16: lo = sum & max_uint16 hi = sum >> 16 sum = hi + lo return sum
Acknowledgements
TCP employs an acknowledgement system where the recipient sends an 'ack' back to the sender to confirm successful receipt of data. In cases where a segment of the message is compromised, corrupted, or lost during transmission, no acknowledgement would be sent from the receiving end. Consequently, the sending machine initiates retransmission upon expiration of a set time limit (known as the 'timeout period').
Sequence Numbers
For effective error handling, TCP uses sequence numbers, allowing messages to be reassembled correctly even when received out of order. Each byte of data sent through TCP is assigned a unique sequence number. This ensures that data is delivered in the correct order, bolstering the capability of TCP in handling long streams of continuous data.
Windowing and Flow Control
Windowing is another significant aspect of TCP's error handling realm. It allows multiple packets to be sent before expecting an acknowledgment. TCP likes to bundle up as many packets that fit into the specified window size and send them together. If an error occurs during the transmission of a batch of packets, the entire batch must be retransmitted. Therefore, the choice of window size is strategic. An optimized selection should balance between high throughput and low retransmission rates. For this reason, TCP also implements dynamic window resizing techniques to adapt dynamically to the changing network conditions.[1]
There is indeed more functionality to TCP than what this brief dissection includes. However, it's clear that enabling reliable communication over unpredictable networks necessitates such robust error handling mechanisms. Let's now pivot to reflect on the most common use of TCP - web browsing.
Web Browsing and Tcp
Seeing that over 59%[2] of global internet traffic comes from web browsing, the utilization of TCP plays a monumental role here. All Hypertext Transfer Protocol (HTTP) traffic, a protocol used for transmitting hypermedia documents in the World Wide Web, runs atop TCP[3]. Hence, every time you explore your favorite online shopping platform, access your email, or watch Netflix, TCP is working tirelessly behind the scenes to ensure a smooth, reliable user experience.
Retained in HTML requests, TCP checks for network errors, manages defining the route from the browser to the server, and ensures arrival of all packets to the correct address in perfect sequence. Without TCP’s myriad of protocols maintaining reliability and accuracy, the World Wide Web, as we know it today, simply couldn't exist. So, next time you click for that next episode binge, take a moment to appreciate the untiring work of the unsung superhero – Transmission Control Protocol (TCP).The most common use of the Transmission Control Protocol (TCP) is in creating a reliable data transmission network for web browsers. When you type a URL in your browser's address bar and hit enter, your browser sends a request to the server where that web page resides. This communication is facilitated by TCP.
TCP in Web Browsers:
TCP is responsible for establishing connection with the destination server, ensuring its reliability and closing it once the transfer of data is completed. The way this works is fascinating:
- Your browser sends a TCP SYN (synchronize) packet to the server to initiate a TCP connection.
- If the server is available and ready for a new connection, it responds with a TCP SYN ACK (synchronize acknowledgment) message, agreeing to the communication.
- On receiving the SYN ACK message, your browser sends back an ACK (acknowledgment) message, thus completing what is known as a three-way handshake, which is the first step in establishing a TCP connection.
- Once connected, your browser sends a HTTP GET request for the required content. All data packets sent between your browser and the server have a sequence number, which aids in reassembling the packets at their destination.
- The server then responds with the content and each packet of content received by your browser comes with an acknowledgment. If any packet is missing or in error, your browser can ask the server to resend it.
- After all the data is transferred, either the client (your browser) or the server can decide to end the connection, at which point a TCP FIN (finish) packet is sent. The server/browser acknowledges this FIN packet signaling the end of this communication. This process is called four-way tear down.
Here is a simple representation of how an HTTP GET request can be made using Java’s in-built libraries:
import java.io.BufferedReader; import java.io.InputStreamReader; import java.net.HttpURLConnection; import java.net.URL; //... URL url = new URL("http://www.example.com"); HttpURLConnection conn = (HttpURLConnection)url.openConnection(); conn.setRequestMethod("GET"); BufferedReader rd = new BufferedReader(new InputStreamReader(conn.getInputStream())); String line; StringBuilder result = new StringBuilder(); while ((line = rd.readLine()) != null) { result.append(line); } rd.close(); //...
This does not include explicit handling of the TCP connection but it does implicitly, under the hood.
Strongly linked to the Internet Protocol (IP), TCP forms the backbone of any data exchange over the internet. It's essential for all web-based applications and services, including email, file transfer (FTP) and the operation of content delivery networks (CDNs).
For more details about how TCP/IP works, you might find MDN’s guide very helpful; it dives deeper into how XMLHttpRequest uses TCP/IP to make requests and receive responses from servers.
In the broader sense, TCP is one of the main reasons we can enjoy a seamless, uninterrupted internet experience, long live TCP!
Sources:
When it comes to data communication and exchange on the internet, two of the protocols reigning supreme are HTTP - Hypertext Transfer Protocol, and TCP - Transmission Control Protocol. Particularly, they work hand-in-hand to surface the web pages you request using your browser. It's through this seamless integration between HTTP and TCP that vast amounts of digital data float around cyberspace every day. But how do they work together?
HTTP and TCP
are part of the same internet protocol suite, also known as the TCP/IP model - a conceptual model and set of communications protocols used in the Internet. Consecutively, HTTP resides in the application layer while TCP is in the transport layer of the suite.
Before delving into the detailed discussion about their coexistence, let's get fugacious understanding of these two.
Hypertext Transfer Protocol (HTTP)
HTTP is a protocol used for transmitting hypertext requests and information between servers and browsers. In simpler terms, it lets you surf around the internet by clicking on links which are essentially HTTP requests.
Transmission Control Protocol (TCP)
TCP shines in providing reliable, ordered, and error-checked delivery of a stream of bytes between applications running on network hosts communicating via the internet. TCP keeps track of what's sent and received by numbering the data packets, ensuring any lost data during transition gets retransmitted.
Now, let's dive into the interplay between HTTP and TCP – more specifically relating to the most common use of TCP.
In a typical browsing scenario, when you enter a URL in your browser, an HTTP request is created. This request needs to be sent over the internet to reach the server hosting the requested website. Here comes the role of TCP. Your web browser establishes a TCP connection with the server for transmitting this HTTP request.
Once the server receives this HTTP request via the TCP connection, it processes it and sends back the requested webpage as an HTTP response. The beauty here - the returning HTTP response also uses the same TCP connection to ensure its successful transmission back to your browser.
Here’s an example of a simple GET request:
GET /index.html HTTP/1.1 Host: www.example.com
The above HTTP request asking to retrieve a webpage ( www.example.com/index.html ) will be transmitted via TCP in client-server communication. Every click, every page load, every image viewed on a website all goes through such operations making TCP the most commonly used transport protocol on the Internet.
To put it simply:
• HTTP relies on TCP to transfer its request and response messages.
• TCP occupies itself with individual data packets - breaking them down at the sending end, transferring them via the internet, and assembling them at the receiving end.
• HTTP is unconcerned with these nitty-gritty details, focusing only on presenting the webpage data it has been given following TCP's packet transmissions.
Through this power-packed duo of HTTP and TCP, the most familiar and widespread use of TCP – web surfing, aka internet browsing, continues to morph into an increasingly sophisticated and reliable experience.TCP, short for Transmission Control Protocol, is a crucial part of the internet's foundation. The most common use of TCP is establishing and managing network communications by providing reliable, ordered, and error-checked delivery of a stream of bytes from one system to another. It's the protocol that major internet applications like web browsers, email services, and file transfer systems leverage on.
For a perfect illustration of how TCP works, think of it as a mail service. As a TCP sender, you have an essential package (data) that needs to be transferred to a particular receiver securely. Instead of sending this bulky package at once, you split it into smaller parcels (packets), each with a unique identifier (sequence number), kind-of like tracking numbers on a physical mail.
When these packets reach their destination, TCP takes care of rearranging them in their original order so the recipient receives exactly what was sent without any loss. If for any reason a packet doesn't arrive or arrives corrupted, TCP can request a retransmission.
To visualize the data transmission over a TCP connection, consider the following scenario as part of the Python code below:
from socket import * msg = b'Message to be transmitted' # Create new TCP/IP socket sock = socket() # Connect socket to server address sock.connect(('server.domain.com', 2883)) # Send message over the connection sent = sock.send(msg) # Make sure all the data has been sent while sent < len(msg): sent += sock.send(msg[sent:]) print('All data sent.') sock.close()
The above Python script assumes we're using TCP since when creating a new socket, SOCK_STREAM (the default type which stands for TCP) is used.
When it comes to practical applications, virtually almost every application that requires data to be delivered accurately and in sequence utilizes TCP. A prime example is HTTP (HyperText Transfer Protocol). When you visit a website, your browser (a client) sends HTTP requests to the web server hosting the site using TCP protocol.
According to the MDN Web Docs, here's a simplistic view of how a client-server communication happens:
* Client opens a TCP connection: The first stage involves a three-way handshake to establish a connection.
* Client sends request: The client then sends a request to the server over the connection just established.
* Server processes the request: Server processes the request and sends a response back to the client.
* Client processes the response: The client receives the response, processes it, often displaying the received information.
* Close the connection: Finally, close the connection. Alternatively, the connection can be kept open for further requests.
This process highlights a critical operation of TCP – reliability and order delivery of data. The 'stream-of-bytes' concept ensures large data messages are received intact and in order, despite being broken down into packets for transmission. Furthermore, TCP works hand-in-hand with IP (Internet Protocol), forming TCP/IP suite. IP handles addresses and routes the packets, while TCP assures their orderly and safe delivery.
With the explained fundamental principles and examples, it becomes apparent why TCP is essential for sending mails, transferring files, and loading websites—activities that demand high reliability and proper sequencing of information.Multi-homing is a significant function in TCP/IP protocols that provides several benefits within network architecture, particularly to ensure stability, reliability, and performance factors. It essentially allows a networked device to have multiple network interconnection points or IP addresses. At its core, the most common use of Transmission Control Protocol (TCP) is to establish reliable connections in an Internet Network where data can be transmitted securely and orderly.
Multi-homing functionality, when employed in the TCP/IP model, brings two consequential effects:
- Fault Tolerance: In an event where one connection fails, the device still stays connected through other lines which guarantees no interruptions in service availability.
- Load Balancing: When there are multiple parallel connections, the device can manage traffic by distributing data across all available networks, optimizing bandwidth utilization and preventing congestion.
In line with the most prevalent utilization of TCP, the above multi-homing characteristics render it more resilient, allowing secured data transmission even under challenging network circumstances. The introduction of multi-homing in TCP brings a higher level of redundancy that aids in mitigating failures in network connectivity. Essentially, it enables the TCP/IP protocol stack to maintain the connection steadiness that defines the fundamental requirement for TCP's mission-critical roles such as operating secure HTTP (HTTPS) websites, transferring files via FTP, sending emails over SMTP, and more.
To visualize how multi-homing works, let's see the below pseudo code:
class Device: def __init__(self): self.connections = [] # Holds all the IP addresses def add_connection(self, ip_address): self.connections.append(ip_address) def remove_connection(self, ip_address): self.connections.remove(ip_address)
In this code snippet:
- We've created a device object that would signify a networked device in real life
- This device holds multiple connections, representing multiple IP addresses
- We have provided methods to add and remove connections
This illustrates how a device manages multiple network interfaces, typical in a multi-homed setup.
Further, we can see a simple depiction of multi-homing in the form of a table:
Device | Connections (IP Addresses) |
---|---|
Device 1 | 192.168.0.2, 192.168.0.3 |
Device 2 | 172.16.0.2, 172.16.0.3, 172.16.0.4 |
Device 3 | 10.0.0.2 |
Each device can have one or more connections signifying a genuine multi-homing environment.
More detailed information about TCP/IP protocols, their operations, and uses could be found on the official Internet Engineering Task Force (IETF) documentation here.
Drilling down into the specifics, the most common use of TCP (Transmission Control Protocol) is web browsing and email transmission. Why so? This is due to the reliability which TCP offers in contrast with its counterparts such as UDP. Below are three primary areas incorporating TCP:
Data Transmission In Web Browsing
This is probably where most people encounter TCP, even if they don't realize it. Each time you load a web page, your computer is sending TCP packets to the server and the server is replying with TCP packets. It's more than just delivering the data; it also ensures that the data arrives intact and in order.
Protocol | Function |
---|---|
TCP | Guarantees the delivery of data and also guarantees that packets will be delivered in the same order in which they were sent. |
HTTP | Rides on top of TCP because it needs the guaranteed delivery that TCP provides. |
Email Transmission
E-mails are also commonly transmitted via TCP. Similar to web browsing, Email Servers use TCP due to its reliability and error correction capabilities. SMTP for outgoing mail and POP3 or IMAP for incoming mail, are all standard internet communication protocols used for email communication and they employ TCP.
File Transfer
TCP can also often be found in the exchange of files across the network. File Transfer Protocol (FTP) rides on top of TCP because it too requires reliable service. Given the importance of accurate file transfer, TCP plays a crucial role here.
# Example code showing opening of TCP socket in Python import socket server_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
With all this at hand, one can understand why TCP is indeed the backbone of the internet universe affecting us on a daily basis. For anything which requires 'reliable' and 'ordered' data delivery, TCP is the protocol to go. Feel free to dive deeper into the nuts and bolts of TCP on online resources like IETF's detailed rfc793 document.