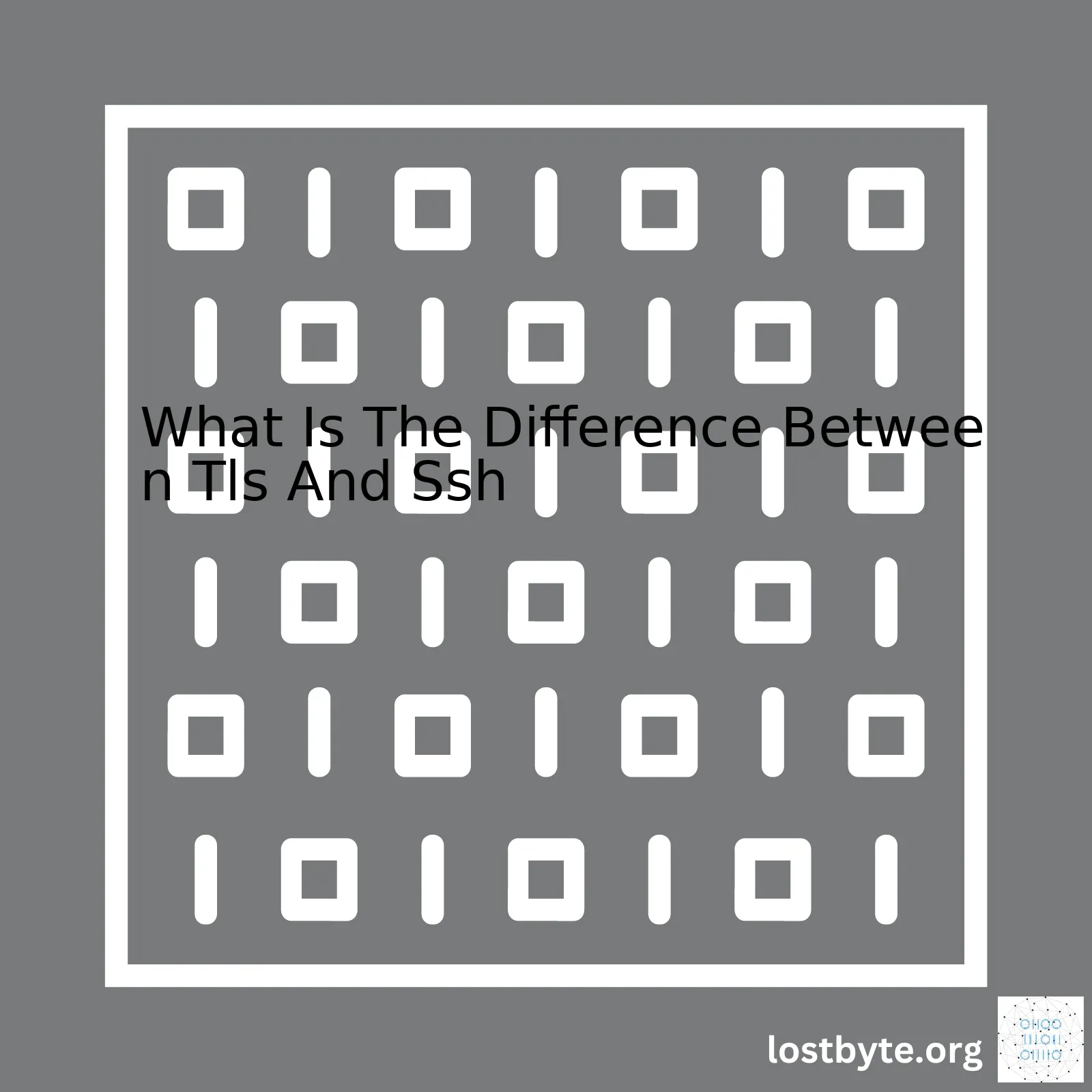
TLS (Transport Layer Security) | SSH (Secure Shell) |
---|---|
Protocol that provides privacy and data integrity between two communicating applications. | Network protocol that gives users, particularly system administrators, a secure way to access a computer over an unsecured network. |
Used for web browsers and other applications that require data to be securely exchanged over a network and ensures that a connection to a remote server is genuine by encrypting the data that is being transmitted. | Often used by system administrators to control web servers remotely. |
Certificates (from trusted third parties) are widely used to confirm the identity of the server for client software. Thereby ensuring the client will trust the data it receives from the server. | It authenticates using key pairs, with a private and a public key. The private key remains a secret and a public key can be disseminated. Anyone can encrypt a message using the receiver’s public key, but only the holder of the private key can decrypt the message. |
The Transport Layer Security (TLS) and the Secure Shell (SSH) both offer ways to protect sensitive data when transmitted over unsecured networks. Though they serve similar functions, each has its unique characteristics and areas of use.
TLS secures a multitude of everyday internet usages – it guards your data during online transactions, ensures email exchanges remain confidential, and verifies that the websites you visit are what they claim to be (GlobalSign Blog). Unlike SSH, TLS applications interchange cryptographic keys using certificates issued by trusted authorities – this is what makes your browser deem a website “trustworthy”.
On the contrary, SSH is specifically designed to give system administrators a secure way to access and manage system resources remotely (TechTarget SearchSecurity). Instead of relying on external entities for authentication as in TLS, SSH uses key pairs which comprise one public and one secretive private key. This makes SSH an ideal choice for administering systems located in insecure environments.
Understandably, deciding whether to use TLS or SSH comes down to scrutinizing their main differences while also considering industry best practices.
Here is an example of establishing a secure connection via SSH:
ssh username@hostname
And here’s an exemplar of common secured connections over https utilizing the force of TLS:
xxxxxxxxxx
HTTPS://www.securewebsite.com
In light of this information, your specific business needs, and the type of data and services involved, the appropriate choice could be made between TLS and SSH.Understanding the basics of Transport Layer Security (TLS) and Secure Shell (SSH) while highlighting their differences is paramount for coding professionals, network administrators, and cybersecurity experts handling secure internet communication.
xxxxxxxxxx
TLS
and
xxxxxxxxxx
SSH
are protocols meant to provide privacy and data integrity between two communicating applications and deliver security features directly to the application layer. They were specifically designed to defend against eavesdropping, tampering, or message forgery.
TLS
•
xxxxxxxxxx
TLS
, a successor of Secured Socket Layer (
xxxxxxxxxx
SSL
), guarantees encryption, decryption, and checking of all transmitted data at a very high standard. This is achieved by encoding network connection segments above the transport protocol.source.
• It establishes a highly secured connection through a process called TLS Handshake. In this phase, server and client agree on the protocols and cryptographic algorithms to use, authenticate the identity of the server, and generate shared secrets.
• It operates directly under application protocols such as HTTP, SMTP, FTP, providing them with secured connections.
SSH
•
xxxxxxxxxx
SSH
, also known as Secure Socket Shell, is a protocol whose main function is to secure remote login from one computer to another.source.
• It provides strong password authentication and secure encrypted data communication over insecure networks, protecting against IP spoofing, routing spoofing, and other network-level attacks.
• SSH operates at the session layer, allowing applications to spontaneously establish sessions without manual intervention.
TLS | SSH | |
---|---|---|
Security Position | Sits above Transport Protocol | Operates at Session Layer |
Main Function | Encrypts Segments of Network Connections | Remote Login from One Computer to Another |
Common Use | HTTP, SMTP, FTP | Secure Remote Logins Over Unsecured Networks |
The main difference between
xxxxxxxxxx
TLS
and
xxxxxxxxxx
SSH
lies in their operation layer and function. While TLS serves more generalized purposes, like securing browser content, send encrypted emails, and perform secure file transfers, SSH concentrates more on administering systems and executing tasks remotely over an unsecured network.
Sample usage of
xxxxxxxxxx
TLS
: An HTTPS service:
xxxxxxxxxx
import http.server, ssl
server_address = ('localhost', 4443)
httpd = http.server.HTTPServer(server_address, http.server.SimpleHTTPRequestHandler)
httpd.socket = ssl.wrap_socket(httpd.socket,
server_side=True,
certfile='path/to/cert.pem',
keyfile='path/to/key.pem',
ssl_version=ssl.PROTOCOL_TLS)
httpd.serve_forever()
Sample usage of
xxxxxxxxxx
SSH
: A remote command execution:
xxxxxxxxxx
from paramiko import SSHClient
ssh = SSHClient()
ssh.load_system_host_keys()
ssh.connect('myserver.com')
stdin, stdout, stderr = ssh.exec_command('ls -l')
files_list = stdout.read().splitlines()
for file in files_list:
print(file)
ssh.close()
In summary,
xxxxxxxxxx
TLS
and
xxxxxxxxxx
SSH
are both crucial components in maintaining the security and integrity of data in network communications. While they share similar security measures, understanding their unique roles and differences in operation will enable more efficient and safe use of them.The Transport Layer Security (TLS) has some key components integral to its workings. These components include:
• Handshake Protocol: Before the transfer of data between two systems, it devises encryption algorithms to be used and carries out authentication.
• Change Cipher Spec Protocol: It necessitates the change in cipher depending upon the communication requirement.
• Alert Protocol: Transmits an alert message whenever a problem is detected.
• Records Protocol: Handles organization of data into blocks, compression, encryption, and transference.
Now, let’s contrast these components with SSH (Secure Shell). First, it’s crucial to remember that TLS and SSH are used for different purposes even though they both provide secure and encrypted communications over the internet.
TLS vs SSH:
• Purpose: While TLS is widely utilized to secure web browser sessions, mail protocols, and VPNs, SSH is created for secure remote login and command execution on remote systems.
• As opposed to the TLS Handshake Protocol, SSH uses a different session establishment process that involves a server listening for connection requests from a client, which further interact to establish a secured channel.
• Unlike the Change Cipher Spec Protocol in TLS, SSH includes choosing a cipher within the initial setup and does not entail any separate protocol for changing ciphers.
• The TLS’ Alert Protocol isn’t applicable for SSH as when errors occur in SSH communications, the connection is simply terminated.
• While the TLS Recording Protocol structures data and handles compression before transport, SSH deploys binary packets for secure transportation which is fundamentally similar but structured differently.
In coding terms, setting up a TLS connection might look like this:
xxxxxxxxxx
final SSLContext sslContext = SSLContext.getInstance("TLS");
sslContext.init(keyManagerFactory.getKeyManagers(), trustManagerFactory.getTrustManagers(), new SecureRandom());
final SSLSocketFactory sslSocketFactory = sslContext.getSocketFactory();
On the other hand, here’s a simple representation of how you might set up an SSH connection:
xxxxxxxxxx
JSch jsch = new JSch();
Session session = jsch.getSession(user, host, port);
session.setPassword(password);
session.setConfig(config);
session.connect();
As you can see, while both TLS and SSH use encryption to protect data during transmission, they differ significantly in their design, intended use, and implementation processes. Understanding the fundamental aspects of both will ensure you choose the right protocol for your specific needs. More info found on SSL/TLS Learning Center and RFC 4253.Beside being vital for system administrators managing servers, understanding the working mechanism of the Secure Shell (SSH) is beneficial even for regular internet users. So let’s delve into detail without further ado.
Secure Shell, commonly called SSH, offers cryptographic communication for network services over an untrusted network. Furthermore, it uses a protocol method that ensures privacy and confidentiality of data exchanged between two systems through encryption.
The distinct underlying mechanism that makes this possible includes:
- Authentication: Before a secure connection is set up, clients are authenticated by the server using multiple methods, with password authentication and public-key authentication being the most frequent ones.
- Channel Establishment: When authentication succeeds, a new secure channel is established that allows the flow of encrypted data.
- Data Transfer: Data transfer ensues within this secure channel, offering integrity and confidentiality to the transmitted information.
Now, you may ask, how does this differ from Transport Layer Security (TLS)? This question arises quite frequently since TLS, like SSH, also provides a mechanism for transmitting data securely over the internet.
While there are similarities between these two protocols, there are also significant differences. They include:
- Design Usage: SSH was designed to create a secure channel between local and remote hosts, often used for terminal session, file transfers or port forwarding. On the other hand, TLS is typically seen protecting web applications as HTTPS when interacting with the world wide web.
- Authentication Approach: With SSH, only the client verifies the server’s identity taking advantage of public-key cryptography method. This compares to TLS where both parties, client and server, can authenticate each other’s identities, leading to mutual verification.
- Certificate Handling: TLS mostly relies on X.509 certificates issued by trusted Certificate Authorities for verification, which SSH ordinarily doesn’t require. SSH employs a “trust on first use” model where initially connected host keys are generally assumed to be correct.
To highlight the comparison even better, consider this simple analogy:
Think of SSH as a pipe connecting two systems, allowing secure flow of data, whereas think of TLS as a safety jacket worn by the data while being transmitted over the internet.
For example source code of an SSH connection could look like this:
xxxxxxxxxx
ssh -i ~/.ssh/private_key.pem user@IP
On the contrary some programming languages such as Python make use of TLS when making a request:
xxxxxxxxxx
requests.get("https://www.google.com")
Remember, both TLS and SSH have their own roles and serve different purposes in different scenarios. By understanding how they function and what they are intended for, you can make better decisions on which one to use depending on your specific needs.
For a deeper insight, I suggest reading the original documentation of SSH from the Open Group here and for the official RFC document for TLS 1.3, click here.The significant role of TLS (Transport Layer Security) in data protection is to provide end-to-end security across internet communication. It secures our data by encrypting the information being transmitted over the network. Therefore, making it unfeasible for unauthorized parties to gain access or manipulate the data within the transmission.
On a practical level, here’s how TLS works:
• An application that wishes to protect its data begins a TLS session by sending a ‘client hello’ message to a server.
xxxxxxxxxx
# Simulated Python code
class Client:
def __init__(self):
self.message = "Client hello"
def send_message(self, server):
server.receive_message(self)
• The server, on receiving this call, responds with a ‘server hello’ message and the process of establishing a TLS encrypted connection starts.
xxxxxxxxxx
# Simulated Python code
class Server:
def receive_message(client)
if client.message == "Client hello":
return "Server hello"
From a functional point of view, TLS supports cipher suites, which are pre-configured sets of cryptographic algorithms that can be used together to secure a network connection. Additionally, TLS supports certificate-based authentication, where servers (and optionally clients) prove their identity using digital certificates.
Next, let’s comprehend SSH (Secure Shell). SSH is a protocol that also provides secure remote login and other secure network services. Its main capabilities are remote command-line, login, and remote command execution. However, any data sent over an SSH connection is encrypted, offering excellent protection against common network-level attacks.
But then, how does SSL differ from TLS? Here’s a side-by-side table illustration:
| | TLS | SSH |
|——————————-|————————————|————————————–|
| Encryption | Uses asymmetric encryption | Uses both symmetric and asymmetric |
| Authentication | Certificate-based | Public RSA key |
| Use cases | Secure web browsing (HTTPS) | Secure file transfers, remote logins |
| Vulnerabilities | Dependent on implementation | Fewer known vulnerabilities |
In essence, while both TLS and SSH provide mechanisms for protecting data during transmission, the ways they operate and their use cases bring about the key differences.
It’s worth noting that TLS is primarily employed to secure web browser sessions, email, VoIP, and instant messaging. On the other hand, SSH is commonly utilized for securely accessing remote systems, managing network devices, and connecting to Git repositories.
For instance, GitHub uses SSH as one approach to authenticating connections to remote repositories.
Indeed, understanding these protocols’ functionalities not only aids in enhancing data security but forms a fundamental basis for many security practices we employ today. Appropriately choosing between TLS and SSH fosters greater protection in respective applications.SSH and TLS are both protocols used for ensuring secure communications between devices in a network environment. They both stand as a pillar to online communication, enabling us to transfer sensitive data securely.
Secure Socket Shell (SSH)
This is a network protocol that provides a secure channel over an unsecured network. The typical use of SSH is for managing networks and systems remotely; this means that you can command and control complete servers and machines from a separate location. We primarily use SSH where the shell (command window) needs to be secured while communicating.
xxxxxxxxxx
ssh username@hostname
The features of SSH include:
- It provides strong password authentication
- It shields against IP spoofing, throttling of connection, and routing of information
- Prevents against DNS spoofing.
Transport Layer Security (TLS)
On the flip side, we have TLS. It ensures privacy and data integrity between computer applications. When one application wants to send its data safely to another application, it uses TLS.
An example would be when a user enters their credit card number into a web form on a site that they’ve navigated to from their web browser:
xxxxxxxxxx
https://secure-website.com/
The notable features of TLS include:
- Encryption: Ensures no eavesdropping from an attacker
- Authentication: Guarantees you’re communicating with the right server and not a pretender
- Data Integrity: Protects data from being modified without your knowledge
Differences Between TLS and SSH
Both SSH and TLS provide secure communication, but they differ in multiple aspects:
SSH | TLS | |
---|---|---|
Purpose | Used for secure remote login and command execution | Used for encrypting network connections above the transport layer |
Use Case | Frequently used in client-server architecture | Commonly used in web browsing, email, instant messaging, and VOIP |
Cipher Suite Negotiation | Yes | Yes |
While understanding the difference is crucial, it’s equally essential to know that these protocols play a vital role in keeping internet communication secure and running smoothly. Their differences aren’t about superiority but more about purpose and the specific use case scenario.
Needless to say, both SSH and TLS are critical components of modern connected systems, each excelling in its particular context.
To further understand and weigh their implications, you could refer to the Internet Engineering Task Force’s published standards for both SSH and TLS.While SSL, TLS, and HTTPS are all cryptographic protocols designed to secure communication over a computer network, each has its unique characteristics. However, when it comes to SSH (Secure Shell), it stands in its own category as an entirely different protocol. I shall explain these concepts initially before honing in on the difference between TLS and SSH.
SSL (Secure Sockets Layer)
Founded by Netscape in the mid-90s, SSL was one of the first popular security protocols for internet communication. It establishes a secure link between two machines, typically a web server and a client (i.e., web browser). Although SSL is largely deprecated due to vulnerabilities and replaced with TLS, people often still refer to TLS as SSL out of habit.
Example of code usage:
xxxxxxxxxx
ssl_sock = ssl.wrap_socket(sock, server_side=True,
certfile="localhost.pem", keyfile="localhost.key")
TLS (Transport Layer Security)
TLS is the successor to SSL. It provides encrypted data transmission of messages to maintain privacy and data integrity between applications. The latest versions like TLS 1.3 offer many improvements over SSL and early TLS versions, including better performance and more robust security.
Example of code usage:
xxxxxxxxxx
context = ssl.create_default_context(ssl.Purpose.CLIENT_AUTH)
context.load_cert_chain(certfile="mycertfile", keyfile="mykeyfile")
server = context.wrap_socket(server, server_side=True)
HTTPS (Hyper Text Transfer Protocol Secure)
It’s indeed HTTP running over an SSL/TLS connection. When a website uses HTTPS, the data sent between it and its visitors is encrypted. Thus, making it a widely accepted standard for safe financial transactions and personal data entry.
When you analyze these protocols we can tell that they perform specific functions for securing network communication. But, none of them serve the function of SSH.
So, what distinguishes TLS from SSH? Revealingly, the primary difference lies in their application and use-case scenarios.
TLS vs. SSH
TLS serves to establish a secure communication path between local and remote systems mainly for transporting HTTP traffic, thus protecting the transmitted data between the server and client.
Contrarily, SSH is devised for securely executing commands across the same types of networks. It provides a secure method for logging into another computer over a network, executing commands on a remote machine, and transferring files from one machine to another. Moreover, unlike TLS, SSH enables authenticated and encrypted connections between untrusted hosts over an insecure network.
Here is a sample code snippet for creating an SSH client using python’s paramiko package:
xxxxxxxxxx
import paramiko
ssh = paramiko.SSHClient()
ssh.set_missing_host_key_policy(paramiko.AutoAddPolicy())
ssh.connect('hostname', username='user', password='passwd')
So, if your goal is securely transmitting HTTP data over a network, then you would lean towards utilizing TLS. Alternatively, if you need a secure way to perform administrative tasks on a remote server, then SSH is unquestionably the appropriate choice.
Whether it’s managing remote servers (SSH) or securing browser-server communications (HTTPS/TLS), understanding these protocols gives us highly valuable tools enabling secure digital interactions across seemingly unsafe distance! For more detailed information about these concepts, please visit Cloudflare Learning Resources.
References:
1. RFC 8446 – The Transport Layer Security (TLS) Protocol Version 1.3.
2. RFC 4251 – The Secure Shell (SSH) Protocol Architecture.Secure Shell (SSH) protocol offers a secure network communication system. Developed in early the 90s, SSH aims to replace insecure remote shell programs such as Telnet and rlogin.
The key features of SSH include:
- Authentication: SSH provides a secure method to authenticate users. For instance, it can use public-key cryptography where a pair of keys (public and private) are created. The public key is kept on the server, and when connection occurs, the server sends an encrypted message that can be deciphered only using the private key.
- Encryption: All data transmitted between client and server is encrypted ensuring confidentiality and integrity of all data exchanged.
- Integrity: SSH ensures that data is not tampered in transit by incorporating message integrity check. Any change in the data during transmission would cause the verification process to fail.
xxxxxxxxxx
#Example Usage of SSH
ssh user@host
On the contrary, Transport Layer Security (TLS), formerly known as Secure Sockets Layer (SSL) operates at a different layer of the networking model compared to SSH.
TLS is primarily used for securing data sent over HTTP which means it’s used for securing data in a web browser session. It provides:
- Privacy: By encrypting communications between the client and the server, eavesdroppers cannot decode what’s being discussed.
- Integrity: Ensures no one tampers the data while in transit.
- Authentication: It verifies if you’re truly communicating with who you believe you are.
TLS uses asymmetric and symmetric encryption. In Asymmetric encryption, two mathematically linked keys are used, one for encryption and one for decryption. This is quite slow so it’s only used at the start of the TLS session. The rest of the session uses symmetric encryption, which uses a single key for both encryption and decryption that has a lower overhead.
xxxxxxxxxx
#Example Use of Python requests with TLS
import requests
response = requests.get('https://example.com')
While both SSH and TLS provide secure communication via encrypted tunnels, they are used for different types of network traffic. SSH is ideal for command-line interface access to remote systems, whereas TLS excels more for recent applications like safeguarding web transactions.
In terms of security, both rely on similar cryptographic principles but has clear functional differences. Notably, TLS only provides server authentication as standard whereas SSH typically requires mutual authentication.
Hence, SSH arguably will ensure more secure services in environments necessitating extra scrutiny at the point of entry. However, both have their place and play crucial roles in today’s Internet communications ecosystem.
For further reading Click Here
On a fundamental level, both Transport Layer Security (TLS) and Secure Shell (SSH) are cryptographic protocols that provide communication security over a network. They encrypt data to prevent unauthorized access during transmission, making them key tools in the realm of internet communications and data protection. Despite their similar uses, TLS and SSH differ significantly in their method of encryption, data authentication, and use cases.
Encryption Method
TLS
The process of establishing a TLS connection involves several steps:
- A client sends a “client hello” message with a list of supported cipher suites.
- The server responds with a “server hello” message, choosing the strongest mutually supported cipher suite.
- An asymmetric, or public-key encryption is carried out in order for the client and server to establish a shared symmetric key.
Knowledge of this symmetric key provides the ability to decrypt the information, hence it’s kept entirely private between the client and the server.Code Example:
xxxxxxxxxx
151// A high-level look at a simplified TLS handshake
2Client: Hello, these are the cipher suites I support.
3Server: Hi, let's use this particular cipher suite that we both understand.
4Client and Server: Let's establish a common key for subsequent data encryption.
5
SSH
SSH does utilize symmetric encryption as well, involved parties both store key pairs (a public key and a private key), but the encryption process is slightly different.
- The client initiates the session by sharing its version information, which includes the SSH protocol version and the name of the software.
- The server also shares its version number then, client and server exchange their public keys.
- A shared piece of random data, known as a secret, is created merging the two public keys.
- This combined data forms an encryption key used for channel encryption and decryption.
Code Example:
xxxxxxxxxx
151// A high-level look at a simplified SSH handshake
2Client: Hello, this is my software version. Here's my public key.
3Server: Great, this is my software version. This is my public key.
4Client and Server: With these public keys, we'll generate a secret for our symmetric encryption.
5
Protocol Encryption Method TLS Follows a handshake protocol to establish a common symmetric key, which is subsequently used for data encryption and decryption. SSH Uses both public and private keys. During the handshake phase, the client and server exchange their public keys and combine to generate a unique symmetric key for the session. Data Authentication
TLS employs certificate-based authentication. The server presents a digital certificate to prove its identity to the client. This certificate is digitally signed by a trusted Certificate Authority (CA).
In SSH, on the other hand, host authentication is done using either passwords, public key mechanisms or a combination of both. Password-based authentication is self-explanatory whereas public key mechanism entails an exchange of public keys during the initial handshake, authenticating each to the other.
Use Cases
TLS is widely used in securing web traffic. It guards your interactions on websites, particularly where sensitive information is being transferred, like online banking sessions or e-commerce checkouts.
SSH, however, is primarily employed ensuring security for remote logins to computer systems. System administrators use SSH to manage servers, databases, and networks remotely. Programmers use it to securely access git repositories.
Despite having similar goals – keeping your data safe from prying eyes, TLS and SSH have evolved to serve very different functions within the sphere of secure communications.
To further differentiate between TLS and SSH, please refer to this table:
Parameter TLS SSH Data Authentication Uses Certificate-based Authentication Implements either password or public key mechanisms Use Cases Primarily used in securing web traffic Often used for secure remote logins to computer systems While the concepts of SSH and TLS might seem complex initially, digesting them bit by bit makes one realize how essential these protocols are for internet security. Whether you’re making an e-commerce purchase over a safe website (protected under TLS) or remotely logging into servers securely (using SSH), both have proven to be critical cogs in the secure data transmission machinery.
Your curiosity about the difference between TLS and SSH, particularly in regards to their performance efficiency, is an interesting point of conversation. As a coder, I have frequently utilized both protocols for a multitude of frameworks, so allow me to provide a solid comparison.
TLS (Transport Layer Security) and SSH (Secure Shell) are cryptographic protocols designed to ensure secure communication over computer networks. While they share a common purpose, their operational functions and performance efficiency swing them in different directions.
TLS is typically associated with securing web browser sessions, safeguarding sensitive data transfers, and securing email communications among others. It operates above TCP, permitting HTTP to function securely as HTTPS.
xxxxxxxxxx
141/* hypothetical code snippet showing a pseudo implementation of TLS */
2TLS.init(serverCert);
3TLS.send_secure(message);
4
SSH on the other hand, is a protocol most commonly used in remote login applications. Securely managed by network administrators to control servers and automate processes remotely, it’s a go-to tool in the scripting realm.
xxxxxxxxxx
141/* hypothetical code snippet showing a pseudo implementation of SSH */
2SSH.init(sshKey);
3SSH.login(server_address);
4
Performance Efficiency: TLS vs SSH
Analyzing performance efficiency involves assessing several distinct but interconnected variables: speed, computational resources, and security strength.
While direct benchmarks are hard to establish due to the number of factors involved (like software implementations or hardware spec), we can make some educated assumptions based on the protocol designs and typical usage scenarios.
– TLS often provides commendable performance for HTTP traffic, especially when HTTP/2 is in play. With its capacity to handle multiple requests concurrently within one connection, fewer TCP connections are needed, which economizes on CPU cycles and reduction in latencies due SSL/TLS Handshake.
– SSH can be slightly laggy compared to TLS in instances where small amounts of data are transferred at high frequencies – for instance, typing in a remote shell session. This is because SSH packet sizes and TCP/IP ratios aren’t optimized for such use cases.
– In terms of encryption overhead, both protocols are quite efficient and don’t impose significant computational costs, especially with modern hardware which accelerates cryptographic functions.
Remember, these differences are minimal and are usually imperceptible during typical uses. Remember also that TLS and SSH were designed with somewhat different use-cases in mind, and shine their best within those scopes.
The major aspect that sets them apart is their set of features: SSH excels in granting secure direct access to systems via a command-line interface, while TLS shines in providing a secure channel for application-level protocols like HTTP, SMTP etc.
For additional comparisons and insights, you might refer to valuable online resources like [IBM developer](https://developer.ibm.com/topics/security/) blog for articles related to security protocols’ tools and technologies or [OpenSSL’s documentation](https://www.openssl.org/docs/) for comprehensive information about TLS and SSH implementations.When talking about network security, two significant protocols often come up are Transport Layer Security (TLS) and Secure Shell (SSH). These are Internet standards utilized for protecting data transmitted over networks. Both of these protocols address different kinds of security vulnerabilities, but there are certain common risks they mitigate. In order to understand this, we need to first briefly explain what TLS and SSH are:
– **Transport Layer Security (TLS)**: This network protocol secures connections between web servers and browsers in a bid to prevent eavesdropping, tampering, and message forgery. With its predecessor being Secure Sockets Layer (SSL), TLS is used largely in a wide array of applications like email, VoIP, and instant messaging.
xxxxxxxxxx
191# Sample code that sets up a basic TLS connection
2import socket, ssl
3context = ssl.SSLContext(ssl.PROTOCOL_TLSv1_2)
4context.load_cert_chain(certfile="mycertfile.pem", keyfile="mykeyfile.pem")
5serversocket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
6ssl_sock = context.wrap_socket(serversocket, server_side=True)
7ssl_sock.bind(('localhost', 443))
8ssl_sock.listen(5)
9
– **Secure Shell (SSH)**: This cryptographic network protocol enables secure remote login and other secure network services operation over an insecure network. It provides encrypted channel for administrators and end users to log into systems remotely.
xxxxxxxxxx
191# Sample code that illustrates the use of SSH with python's paramiko library
2import paramiko
3ssh = paramiko.SSHClient()
4ssh.set_missing_host_key_policy(paramiko.AutoAddPolicy())
5ssh.connect('localhost', username='username', password='password')
6stdin, stdout, stderr = ssh.exec_command('ls')
7print(stdout.readlines())
8ssh.close()
9
Now, let’s discuss the common vulnerabilities addressed by both of these protocols:
– **Data protection**: Both TLS and SSH provide encryption, which ensures that all data transmitted over networks are secure from undesired entities who may intercept it. The exact level of encryption depends upon their configurations.
– **Authentication**: They both utilize certificates or keys to authenticate communicating parties. This mitigates the risk of Man-in-the-Middle (MitM) attacks where an attacker poses as intended sender and receiver in a communication link.
– **Integrity check**: They both ensure data integrity by validating that the information sent is indeed received as it was sent without any alteration during transmission. They employ robust hashing algorithms to achieve this.
Both TLS and SSH are highly customizable in terms of their settings and the encryption methods used, making both flexible enough to meet a variety of needs while maintaining a high level of security. However, while both address some similar security concerns, it’s important to remember that they’re used differently. TLS is mostly used to secure web traffic and generally runs on top of HTTP (resulting in HTTPS) but can be applied to many other types of traffic, too. Meanwhile, SSH is used for managing and executing commands on remote systems – typically over a terminal. In general, one isn’t a substitute for the other, and it’s not uncommon to see TLS and SSH used in conjunction within a single system for maximum security.From a technical perspective, there are key differences between TLS (Transport Layer Security) and SSH (Secure Shell). Both of them serve distinct purposes in the realm of network and internet security. While TLS is predominantly used to secure data transmission over networks like that of web servers, SSH offers a secure method of remote login from one computer to another.
Understanding the Differences
xxxxxxxxxx
111SSH
offers a higher level of control on both sides of the connection. It’s primarily employed for managing systems remotely, keeping the communication encrypted. On the contrary, when you connect to an HTTPS server or SMTP server with STARTTLS, you’re most likely using
xxxxxxxxxx
111TLS
which sets up an encrypted tunnel for safe data transmission.
Every coin has two sides, so do TLS and SSH. One offers security when interacting with web servers, while the other secures remote connections. Talk about safety in diversity! Here’s an extensive comparative analysis:
# Advantages of Using TLS Over SSH
- Data Integrity & Confidentiality: TLS assures better protection of data transferred between servers and clients through encryption. This makes it nearly impossible for hackers to interpret the data.
- Interoperability: TLS is widely accepted and implemented across applications and systems, ensuring high levels of compatibility.
- Certificate-Based Authentication: Owing to Public Key Infrastructure (PKI), websites can establish their authenticity, leading to increased trust among users.
- Administrative Control: SSH is extensively used by sysadmins to control servers remotely. Its secure nature makes it perfect for these tasks.
- Tunneling Capability: With SSH, you can establish a secure “tunnel” through which unsecured data can travel safely.
- User Authentication: You get robust user authentication, designed specifically for human users instead of machine-level interactions.
- Protocol Design: In terms of interoperability, TLS has a widely accommodating design. It supports a variety of cipher suites (different combinations of authentication, encryption, message authentication code (MAC), and key exchange algorithms), allowing implementers to choose which ones to support based on their requirements.
- Versions: Over time, multiple versions of TLS have been released. Servers can support multiple versions to maintain compatibility with older clients and vice versa. This kind of backward compatibility is crucial in ensuring interoperability.
- Compatibility: The broad range of cipher suites and versions supported by TLS make it highly versatile across various environments making it more interoperable.
- Usage: SSH tends to be adopted for administration tasks, rather than broader communication needs that TLS might cater to, impacting its scope for interoperability.
- Limited Flexibility: Unlike TLS, SSH doesn’t support the wide variety of cipher suites. While some SSH libraries support different algorithm options, this selection is more limited than TLS.
- Purpose-Built: SSH was built specifically to create secure command-line sessions between computers. While it can be used in other ways, it is less flexible than TLS, limiting its overall interoperability.
- Its typical use case is securing web traffic via HTTPS.
- It works on the Application layer of the OSI model.
- It supports numerous types of encryption algorithms and cipher suites depending on the level of security needed.
- TLS does not provide any file transfer capability or command execution, unlike SSH.
- SSH operates on the transport layer of the OSI model, one step below TLS.
- Provides secure ways to manipulate files and execute commands remotely.
- Possesses full shell functionality along with tunnelling abilities.
- SSH uses only symmetric-key cyphers for encryption.
# Advantages of Using SSH Over TLS
It’s evident that when dealing with securing data on sites or online transactions, leveraging TLS could be a better option due to its architecture designed for such proactive use cases. However, for system administrative purposes, SSH would provide a more fortified solution owing to its structural design, primarily oriented towards secure administrative tasks. Well, the choice between TLS and SSH may entirely depend on the particular needs of your project and the security requirements.
For documentation on how to use TLS and SSH, follow these hyperlinks:
For TLS (RFC 5246) and for SSH (RFC 4253).Moving Forward
Remember, whether you choose TLS or SSH, each protocol carries its strengths. They work diligently in safeguarding our sensitive data from potential threats, hence making the world of internet a safer place. Going forward as a coder, it is imperative to adapt and align with the continually changing tech environment and make decisions accordingly! Choose wisely, code wisely!
Reference:
– For TLS, see (Wikipedia)
– For SSH, see (Wikipedia)Interoperability is a significant aspect of any client-server communication protocol. It refers to the ability of diverse systems and organizations to work together (inter-operate). It ensures seamless communication between different software and hardware systems irrespective of their underlying architecture or platform. Let’s analyze how interoperability differs for Transport Layer Security (TLS) and Secure Shell (SSH).TLS (Transport Layer Security)
TLS, often used with HTTP to form HTTPS, provides secure communication over a computer network. Its main aim is to provide privacy and data integrity between two or more communicating applications.xxxxxxxxxx
114141// Example: Establishing a connection with GitHub's server using TLS in Node.js
2const https = require('https');
3https.get('https://api.github.com', (resp) => {
4let data = '';
5resp.on('data', (chunk) => {
6data += chunk;
7});
8resp.on('end', () => {
9console.log(JSON.parse(data));
10});
11}).on("error", (err) => {
12console.log("Error: " + err.message);
13});
14
SSH (Secure Shell)
On the other side, SSH is a cryptographic network protocol for operating network services securely over an unsecured network. Typical applications include remote command-line, login, and remote command execution.xxxxxxxxxx
123231// Example: Establishing a connection with a server using SSH in Node.js
2const sshClient = require('ssh2').Client;
3const conn = new sshClient();
4conn.on('ready', function() {
5console.log('Client :: ready');
6conn.exec('uptime', function(err, stream) {
7if (err) throw err;
8stream.on('close', function(code, signal) {
9console.log('Stream :: close :: code: ' + code + ', signal: ' + signal);
10conn.end();
11}).on('data', function(data) {
12console.log('STDOUT: ' + data);
13}).stderr.on('data', function(data) {
14console.log('STDERR: ' + data);
15});
16});
17}).connect({
18host: '192.168.100.100',
19port: 22,
20username: 'frylock',
21privateKey: require('fs').readFileSync('/here/is/my/key')
22});
23
In summary, the core difference in interoperability between SSH & TLS stems from their individual design and purpose. Both are designed to secure communications, but they do so in different contexts—TLS for a broad surface of application layer protocols (like HTTP, SMTP, etc.) and SSH for specific administration and shell use cases.
For a full description of how each protocol achieves its goal, you might want to start from the respective RFCs, i.e., RFC5246 for TLS and RFC4253 for SSH.While both TLS (Transport Layer Security) and SSH (Secure Shell) are cryptographic protocols designed to provide secure communication over the internet, they have different scopes of application based on their inherent features and characteristics.Transport Layer Security (TLS) is primarily used for securely transmitting web content. It’s probably best recognized in the HTTPS protocol that secures websites and user information between a client and server. A straightforward example of this could be e-commerce websites like Amazon or eBay. When you make a purchase, your credit card information needs to be transmitted from your browser to their servers securely. This is where TLS steps in and ensures that your sensitive details are not accessible to hackers and remains confidential.
Here’s what happens when you buy something from an online store:
– You click “Purchase” and your browser initiates a TLS handshake with the server.
– Your browser and the server exchange keys for encryption and authentication.
– Your payment details are encrypted using these keys and then sent over to the server.In an illustrative code snippet:
xxxxxxxxxx
161/* Establishing a TLS Connection */
2SSLContext context = SSLContext.getInstance("TLS");
3context.init(kmf.getKeyManagers(), tmf.getTrustManagers(), null);
4SSLSocketFactory sf = context.getSocketFactory();
5SSLSocket socket = (SSLSocket) sf.createSocket(host, port);
6
On the other hand, SSH (Secure Shell) is commonly used for securely executing commands on remote servers, often in system administration and development scenarios. An everyday illustration of SSH usage can be seen in software development processes that use Git repositories. Software developers connect to a remote Git repository via SSH to clone, fetch, pull or push changes to that repository securely, ensuring none of their source code is tampered during transportation.
Here is how using SSH in Git operations plays out:
– To clone a repository, a developer would trigger the SSH protocol by specifying the SSH URL of the repo.
– The developer’s machine would initiate an SSH session with the repository’s host.
– Their private key is used to confirm their identity, establishing a safe encrypted channel for further interactions.HTML code snippet to illustrate this:
xxxxxxxxxx
131/* Cloning a Git Repository via SSH */
2$ git clone ssh://git@github.com/username/repository.git
3
So, TLS and SSH serve different purposes, but the common thread is the security they provide for data in transit. Web applications widely employ TLS to protect user data, while SSH finds its niche in secure command execution and file transfers.
To engage more broadly with these topics check these references:
RFC 5246 (The Transport Layer Security) Protocol Version 1.2,
RFC 4253 (The Secure Shell – SSH Transport Layer Protocol),
Stackoverflow discussion of TLS/SSL.Understanding the differences between Transport Layer Security (TLS) and Secure Shell (SSH) is essential for anyone working in the cybersecurity field. Both are cryptographic protocols providing data-in-transit protection, but they differ in focus, use cases, and modes of operation.xxxxxxxxxx
111TLS
primary role is secure transmission over networks. It provides a safe passage for data traveling on the internet by encrypting it, thus preventing unauthorized access or tampering. Here are some key characteristics of TLS:
On the other hand,
xxxxxxxxxx
111SSH
was explicitly designed for remote login to computer systems – tailoring its feature set accordingly. Let’s delve into SSH’s critical aspects:
To sum up, both of these protocols serve essential purposes in the world of internet communications. Where one may fall short, the other picks up, completing a robust security framework necessary for today’s technology era. While their design principles are substantially different, they collectively strive towards a single, common goal – ensuring data safety and integrity while journeying across potentially hostile networks.
For more in-depth information about TLS and SSH protocol workings, please refer to “Acunetix”
or “SSL“.