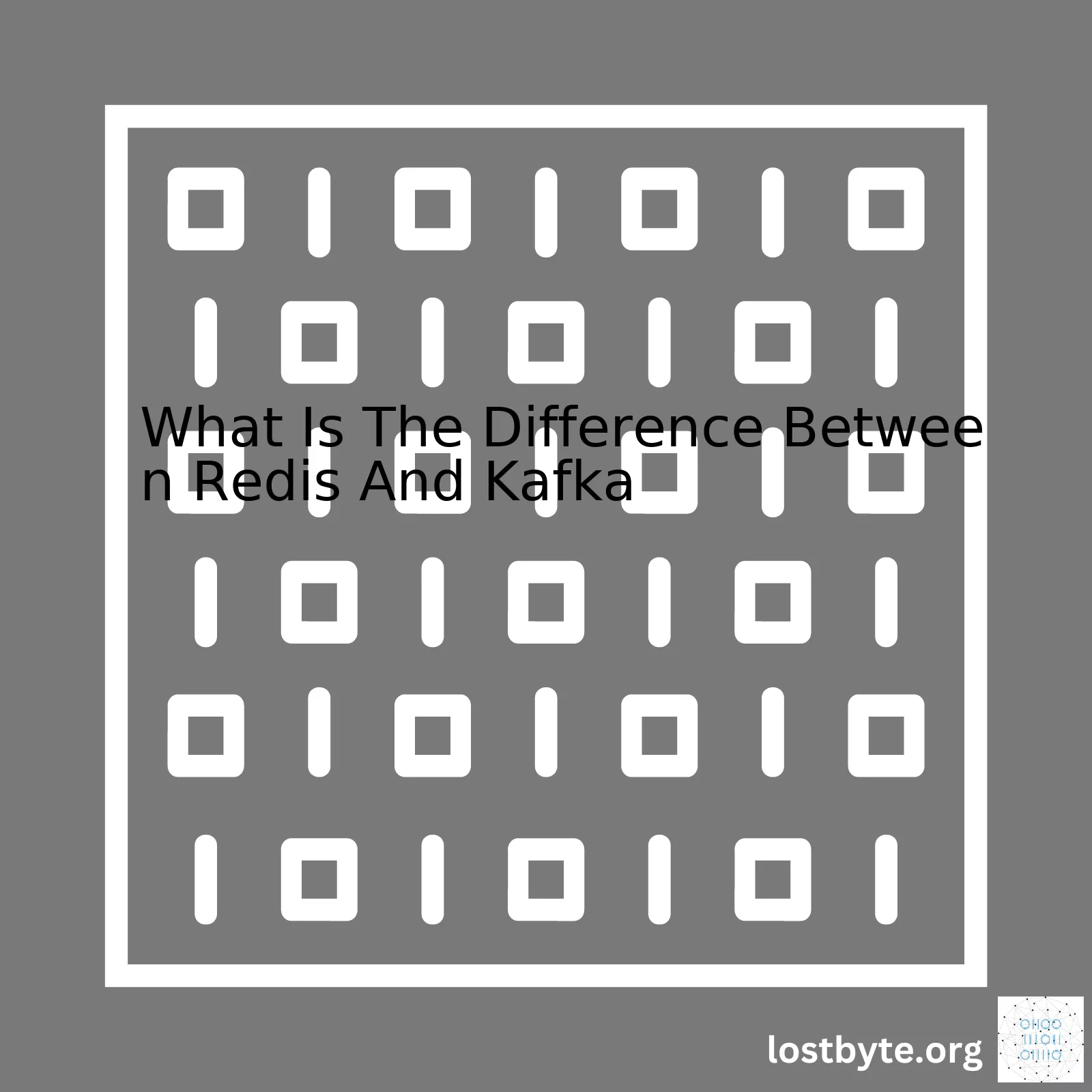
Features | Redis | Kafka | |
---|---|---|---|
Data Model | Key-Value Store | Log-based Message Queue | |
Persistence Options | Snapshotting & Append Only File (AOF) | Write-Ahead Log (WAL) | |
Clustering Options | Master-Slave Replication | Distributed and Partitioned | |
Message Delivery Semantics | At most once / At least once | At least once | |
Language Support | Multiple via client libraries | Multiple via client libraries |
Now, let’s dive deeper into these features:
Redis, originally standing for REmote DIctionary Server, is an open-source, in-memory data structure store that acts as a database, cache, or message broker. The primary feature of Redis is its key-value store system. Hence, it usually performs better at fetching a single key-value pair compared to Kafka.
Persistence options in Redis include snapshotting and AOF (Append Only File), which provide different balances between performance and durability.
As for clustering, Redis uses master-slave replication. In case of a server failure, a replica slave node takes over the master role to ensure smooth service.
Moreover, message delivery semantics in Redis is typically “at most once” or “at least once”, implying it tries to guarantee no message loss but may deliver some messages more than once.
Kafka on the other hand, developed by Linkedin, is a distributed event streaming platform capable of handling trillions of events a day. Unlike Redis, Kafka adopts a log-based distributed and partitioned messaging system, making it highly reliable and scalable.
In terms of data persistence, Kafka uses a method known as Write-Ahead Log (WAL), wherein every write operation is recorded in the log before it is applied.
Kafka works on a clustered model with data distributed and replicated across networked brokers. Kafka’s distributed design ensures that it’s able to handle large volumes of read and write traffic without going down.
Finally, Kafka supports “at least once” message delivery semantics, indicating each message is guaranteed to be processed, albeit potentially multiple times.
Both Redis and Kafka offer comprehensive language support, meaning they can work seamlessly with multiple programming languages such as Java, C++, Python, etc., primarily through client libraries.
These qualities make Redis best suited for high-performance tasks such as caching, session storage, or acting as a message queue or chat server. Conversely, Kafka is ideal for real-time data processing, building real-time analytics from this stream data, activity tracking, gathering metrics from many different locations, or passing messages between services.
Despite seemingly different in nature, Redis and Kafka both have unique capabilities that serve to enhance data accessibility and ease of use for developers. However, it’s essential to understand what sets them apart to maximize their effectiveness when developing applications.
-
What is Redis?
-
What is Kafka?
-
Redis Vs Kafka: The Main Differences
Redis, which stands for Remote Dictionary Server, works primarily as an in-memory data structure store1. Although most widely recognized as a database, cache, and message broker, its flexibility allows for a range of applications:
Feature | Description |
---|---|
Data Structures | Redis supports a variety of data structures such as strings, hashes, lists, and more. |
Speed | As an in-memory data store, Redis provides high-speed read and write operations. |
Persistence | While known for memory storage, Redis also offers on-disk persistence, balancing speed with durability. |
You can use Redis for caching, session storage, pub/sub and other related use cases where you need instantaneous access to your data, but remember, the data size should be within your server’s memory limits.
On the other hand, Kafka – originally developed by LinkedIn – operates as a distributed event streaming platform. It lets you publish, subscribe, store, and process streams of records in real time2. Here are some important aspects of Kafka:
Feature | Description |
---|---|
Distributed System | Kafka runs as a cluster that spans multiple servers, even across different data centers. |
Fault-Tolerant | Kafka effectively replicates data to prevent record loss. |
Scalability | Kafka supports massive message flows with minimal latency, enabling high-volume data stream handling. |
Kafka’s primary purpose is to handle real-time data feeds. It’s used heavily for tracking website activity, aggregating logs from different sources, processing streams of data and more.
While both technologies may seem similar – especially since they’re often used for dealing with real-time data – they differ significantly in functionality, structure, and usage.
# How Redis and Kafka could feature in the same system setup: from redis import Redis from kafka import KafkaProducer producer = KafkaProducer(bootstrap_servers='localhost:9092') r = Redis(host='localhost', port=6379, db=0) producer.send('my-topic', r.get('key'))
When it comes to scalability, Redis is traditionally single-threaded – it can take advantage of only one CPU core. There has been development to allow for multi-threaded Redis, but it still hasn’t come close to the level of Kafka, which can seamlessly scale over various machines3.
Likewise, for data retention, Kafka has a clear edge. It can hold large amounts of data (i.e., terabytes) for a configurable amount of time whereas Redis, being an in-memory data store, is restricted by the physical memory available on your server.
In essence, the best way to leverage these technologies will depend on the specific requirements of your project. While Redis excels in fast data retrieval Ideal for caching), Kafka proves beneficial in situations that require effective log analysis or Real-Time Analytics.
Yes, certainly! Let’s break this down.
Kafka
Apache Kafka is a distributed stream-processing software platform developed by LinkedIn and donated to the Apache Software Foundation. It’s written in Scala and Java. The project aims to provide a unified, high-throughput, low-latency platform for handling real-time data feeds.source
Some characteristics of Kafka include:
- High-throughput: Kafka can handle millions of messages per second.
- Productivity: This platform allows developers to work on a single codebase that could process streams of data in real-time or batches.
- Fault-tolerant: Kafka stores streams of records safely and reliably. Each piece of data (message) within Kafka is replicated across multiple Kafka brokers. Even if one broker fails, there are backups available.
Add a bit of code
Here’s an example of how you might publish and consume messages with Kafka in Python using Confluent’s `kafka-python` library.
from kafka import KafkaProducer from kafka import KafkaConsumer producer = KafkaProducer(bootstrap_servers='localhost:9092') producer.send('sample', b'Sample message') consumer = KafkaConsumer('sample', bootstrap_servers='localhost:9092') for message in consumer: print (message)
Redis
In contrast, Redis is an open-source key-value data structure store, used as a database, cache, and message broker. It supports various types of data structures, including strings, hashes, lists, sets, sorted sets with range queries, bitmaps, HyperLogLogs, and geospatial indexes with radius queries source
Notable features of Redis include:
- In-memory storage: Redis stores its dataset entirely in memory, providing exceptional performance.
- Data types: A variety of rich data types assists developers to solve problems more effectively.
- Persistence: Redis uses different strategies to persist data on disk without sacrificing in-memory capabilities.
Add a bit of code
Here’s an example of how you might use Redis in Python using the `redis-py` library.
import redis r = redis.Redis(host='localhost', port=6379, db=0) r.set('foo', 'bar') print(r.get('foo'))
Comparison between Kafka and Redis
Depending on your use case, you may find one or the other more suitable. However, there are particular differences between Redis and Kafka:
- Scalability: Apache Kafka is designed to handle data at a much larger scale than Redis, thanks to its distributed nature.
- Latency: While both are capable of handling real-time data, Redis’ in-memory storage capability means it can often provide lower latency.
- Data Types: Redis supports multiple data types, whereas Kafka primarily works with message-based streams of records.
- Persistent Data: Kafka duplicates and retains messages until a specified duration elapses, which provides stronger data persistence guarantees. On the other hand, Redis offers optional durability and data persistence but usually prioritizes speed and performance.
I hope that helps! Happy coding!Undoubtedly, comparing Redis and Kafka is like comparing apples and oranges, given that they are designed to address different areas of data storage. However, it’s important to drill down into their fundamental differences in terms of structure, performance, and data persistence, to get a thorough understanding of how these data storage and processing technologies vary.
Redis:
Redis, an abbreviation for Remote Dictionary Server1, is an open-source, in-memory data structure store, used as a database, cache, and message broker.
In terms of structure, Redis stores data in multiple formats, such as strings, lists, hashes, sets, and sorted sets with range queries. One unique feature is that Redis has built-in commands to handle these data types, simplifying the process of manipulating them. For example, you can add or remove elements from a list or a set using simple commands. Here is some indicative code using Node.js:
var redis = require('redis'); var client = redis.createClient(); // Strings client.set('name', 'John Doe'); client.get('name', function(err, reply) { console.log(reply); }); // Lists client.lpush('fruits', 'apple'); client.lrange('fruits', 0, -1, function(err, reply) { console.log(reply); });
Data in Redis is stored in memory, making data operations incredibly fast, but volatile. To achieve data persistence, Redis offers two primary methods: RDB (Redis DataBase file) and AOF (Append Only File). RDB takes snapshots of your dataset at specified intervals while AOF logs every write operation received by the server.
Kafka:
Kafka, on the other hand, is a distributed event streaming platform used by thousands of companies for high-performance data pipelines, streaming analytics, data integration, and mission-critical applications2.
As far as structure goes, Kafka stores streams of records in categories called topics. Data within these topics are partitioned and then replicated across multiple nodes for fault tolerance. You can produce or consume data from a specific point in a topic allowing replayable events. The equivalent Java producer API looks something like this:
import org.apache.kafka.clients.producer.*; Properties props = new Properties(); props.put("bootstrap.servers", "localhost:9092"); props.put("key.serializer","org.apache.kafka.common.serialization.StringSerializer"); props.put("value.serializer", "org.apache.kafka.common.serialization.StringSerializer"); Producerproducer = new KafkaProducer<>(props); ProducerRecord record = new ProducerRecord<>("test-topic", "key", "value"); producer.send(record); producer.close();
While Redis is primarily in-memory storage, Kafka guarantees durability through persistent storage. Once data is written to a Kafka topic, it’s stored for a set amount of time – even after being consumed. This makes Kafka suitable for scenarios where you would need stream processing and maintaining the sequence of events.
Difference:
The key variance between Redis and Kafka lies in their design philosophy and use cases. If you require real-time processing, analysis, and caching with atomic counters, Redis should fit the bill. In contrast, if your project involves lengthy message queues, log aggregation, and streaming data pipelines, Kafka may be the better choice. It’s not about choosing one over the other, but understanding which tool suits your requirements best.
Both Redis and Kafka are robust, open-source software solutions widely adopted in the tech industry, but they serve different goals. They each have unique methods of handling data processing which lead to their individual advantages and use-cases.
Redis Data Processing
Redis (Remote Dictionary Server) is essentially an in-memory data structure store, used as a database, cache, and message broker.[1]. It supports a plethora of data structures like strings, hashes, lists, sets, sorted sets, and more.
SET mykey "Hello" GET mykey
This simple example shows how data can be stored and retrieved using Redis (using a string in this case). In terms of data processing, Redis is renowned for its speed. This is because it holds the entire dataset in memory, allowing for fast read and write operations.
Kafka Data Processing
Apache Kafka is a distributed stream-processing platform.[2] Its primary role involves building real-time streaming data pipelines that can move data between systems or applications. An excellent way to visualize Kafka’s processing model is by thinking about it as a system of record keeping for events:
ProducerRecordrecord = new ProducerRecord<>("CustomerCountry", "Precision Products", "France"); producer.send(record);
This code snippet shows how you can send a simple message to Kafka from a producer, which would contain key-value pairs. Kafka stores streams of records in categories named topics. Kafka clusters store streams of records in a scalable, fault-tolerant way, making it optimum for big data use-cases and real-time analytics both.
Now let’s look at the major differences in terms of data processing technique:
Criteria | Redis | Kafka |
---|---|---|
Storage Mechanism | In memory. | Persistent on disk and in memory. |
Latency | Ultra low latency due to in-memory nature. | Slight delay due to commit logs in the disk storage system. |
Throughput | High throughput with an optimized single-threaded architecture. | Very high throughput suitable for event-streaming and big data processing. |
Data Model | Key Value Store. | Record/Event Streams. |
Use-case | Caching, Pub/Sub messaging, Queuing, and highly transient data. | Event driven architectures, long-term event storage, activity tracking and metrics. |
In summary, there’s not necessarily a ‘winner’ here—Redis and Apache Kafka excel in different areas. If you require rapid access to small pieces of data, suitable for caching and transient data then Redis could be your go-to service, whereas if your application demands handling large volumes of data in real-time, ability to replay past messages and fault tolerance, Kafka would make a better fit. The choice really boils down to your project-specific needs.
When we look at Redis and Kafka, both are powerful tools with unique capabilities that provide so many services. However, the most noteworthy differences lie in their data handling protocols and fault tolerance capabilities.
Redis, a well-established open-source key-value store, is an in-memory store utilized for multiple purposes including caching, pub/sub, counting, and rate limiter to name just a few. The most notable feature of Redis is its replication which is used for redundancy and reads through either asynchronous or synchronous replication. Redis employs asynchronous replication where writes operations are performed in one server (master) and replicated to other servers (slaves). If the master falls down, then one of the slaves gets promoted as the master, ensuring that the system remains intact source. Understanding this functionality clearly shows us how effective Redis at fault tolerance due to its master-slave model.
redis-cli -h master_host -p master_port SLAVEOF NO one
On the other hand, Kafka, a distributed streaming platform featuring high-throughput, low-latency platform for handling real-time data feeds does things differently from a fault tolerance perspective. Kafka’s fault tolerance is architecture-based, thanks to a concept called topic-partition replicas source. In fact, it works by creating copies (replicas) of each partition within a cluster across various nodes. One node acts as a leader coordinating read and write requests for certain partitions, while others serve as followers backing up the data. A follower becomes a leader if the leader fails, without compromising the consistency and availability of the messages inside the Kafka Cluster.
java -jar kafka-topics.jar --alter --topic test-topic --replication-factor 3 --zookeeper localhost:2181
Redis | Kafka | |
---|---|---|
Fault Tolerance | Uses Asynchronous replication. If the master fails, a slave is upgraded. | Follows the Leader-Follower Mechanism.A follower is chosen as the leader should the current leader fail. |
Data Handling | In-memory data structure tool.Perfect for caching and realtime use-cases. | Distributed streaming platform.Great for high-throughput and low-latency needs. |
To sum up, while both Redis and Kafka offer admirable solutions for data management, their distinct forms of operation put them at odds in terms of when and where they may be suitably applied. All in all, in understanding the difference between Redis and Kafka regarding their respective fault tolerance strategies, you must have a clear vision about your system demands.
Assessing scalability in a database management scenario typically involves comparing different solutions based on their processing capabilities, performance under load, and flexibility to accommodate relative growth. Today, I’ll focus on comparing two popular technologies: Redis, an open-source, in-memory data structure store, and Apache Kafka, an open-source, distributed event streaming platform.
Redis Scalability
To understand Redis’s scalability, it is necessary to delve into what Redis offers:
- Redis provides data operations in-memory, meaning the primary dataset is stored in memory rather than disk. This greatly improves speed.
- Unlike traditional databases which become slower as data grows, Redis maintains its speed.
- It uses various mechanisms like replication, sharding, and persistence to ensure data is available even if a node fails or there’s a network partition.
Scalability with Redis is achieved through primarily two ways:
1. Replication:
In this strategy, Redis creates copies of datasets across multiple Redis nodes to impart distributive qualities to incoming payloads. When using replication, write operations go to a master node and then are duplicated asynchronously to one or more replica nodes.
2. Sharding:
It is another common method to distribute data across nodes. Each node stores its unique subset of hash slots. Therefore, by adding more nodes, the system capacity can be increased.
Despite these benefits, however, Redis has some limitations:
- Relying heavily on RAM can become expensive as you scale up.
- While Redis does offer some on-disk solutions, they’re not as flexible or performant as competitors like Kafka.
Kafka Scalability
Kafka, on the other hand, was designed from the ground to scale out rather than scale up:
- Kafka accomplishes this via a distributed and partitioned transaction log, with multiple brokers (servers) that can handle terabytes of traffic without flinching.
- The design allows it to efficiently manage and process streams of records in real time—meaning Kafka can handle high message throughput for both publishing and subscribing.
Apache Kafka scales messaging in three essential ways:
1. Partitioning:
Topics are divided into partitions to allow for parallel consumption. A single partition can only be consumed by a single consumer group to prevent duplicate processing.
2. Brokers:
A Kafka cluster consists of multiple brokers. This setup distributes message delivery to multiple consumers in the same consumer group to ensure quick processing.
3. Replication:
Apache Kafka allows topic replication across several brokers to deal with possible broker failure.
So when might Kafka be a better choice? If you have high-scale streaming, log aggregation, or analytics workloads, Kafka is capable of delivering messages reliably and quickly (millions per second), and it can retain large amounts of data with very little overhead.
In Summary
From the above comparison, we can summarize a few key points:
– Both Redis and Kafka serve different purposes and functions, and they excel under different use cases.
– Computational expense, retention periods, and requirements for data persistence should all weigh heavily in decision-making.
– Kafka’s strength lies in its robust, scalable distributed system capabilities, making it an excellent choice for handling high-volume, real-time data feeds.
– Redis comes in strong as an excellent caching solution, supporting numerous data structures that are quite handy when considering horizontal scaling with unique key-value pairings.
In terms of scalability, both Redis and Kafka provide exciting possibilities. They each have their inherently designed mechanisms to handle scale, but it ultimately depends on the specific needs of your application.
For further insights on Kafka and Redis and how they stack against each other, you can refer to the official documentation for both Redis and Apache Kafka.
When it comes to event sourcing, the primary purpose is to manage and store state transitions or changes as a sequence of events. Two of the most popular tools used for managing these events include Redis and Apache Kafka. Although these two technologies are aimed at serving similar purposes, they come with distinct features, offering different kinds of utilities that cater to specific needs and scenarios.
Redis
Redis, which stands for Remote Dictionary Server, is an open-source, in-memory data structure store. It can work as a database, cache, and message broker. Here are some of its highlighted features:
– Redis provides data replication, Lua scripting, LRU eviction, transactions, and different levels of on-disk persistence.
– It supports multiple data structures such as strings, hashes, lists, sets, sorted sets with range queries, bitmaps, hyperloglogs, geospatial indexes, and streams.
In an event sourcing type system, you can use Redis as a temporary storage where you sequentially add new events to it using time-stamped logs before processing them. The code for adding an event might look like this:
EVENTS_KEY = 'events' event_data = {"id": "123", "type": "purchase", "value": 100.0, } redis.rpush(EVENTS_KEY, json.dumps(event_data))
In the above code, a JSON object representing an event is continuously added to a list, keyed by ‘events’.
Kafka
On the other hand, Apache Kafka is a distributed streaming platform used for building real-time data pipelines and streaming applications. It’s designed to handle data streams from many different sources and deliver them to multiple consumers. Some important features of Kafka are:
– Kafka lets you publish and subscribe to streams of records (similar to a message queue or enterprise messaging system).
– It can store streams of records in a fault-tolerant way.
– It helps process streams of records as they occur.
Kafka’s robust durability and low latency make it particularly excellent for managing high volume, high throughput, real-time event sourcing systems. Adding an event to Kafka topic could look like:
producer = KafkaProducer(bootstrap_servers='localhost:9092') event_data = {"id": "123", "type": "purchase", "value": 100.0} producer.send('events', value=event_data) producer.flush()
The above code writes a single message containing an event to a topic named ‘events’.
Redis vs. Kafka : Key Differences
There is more than one key difference between Redis and Kafka when it comes to event sourcing, summarized as below:
– Performance: Redis offers high-performance capabilities due to its in-memory nature, making it suitable for low-latency applications that require rapid access to data. On the contrary, Kafka is highly scalable and capable of handling larger volumes of data and distributing them across environments, so it’s perfect for high-throughput requirements.
– Data Persistence: In Redis, developers have to manage data persistence manually, while Kafka automatically handles record retention for defined durations.
– Fault Tolerance: While both platforms offer fault tolerance, Kafka’s distributed design provides a higher level of resiliency since information is replicated across different brokers within a cluster.
So, whether to select Redis or Kafka essentially depends on what your specific use case is. If you’re looking for high performance and speed and do not need to deal with large volumes of concurrent data flows, Redis would be a better choice. However, if scalability, large data volumes, and guaranteed delivery matter most, then Apache Kafka would be the obvious winner.
Keep in mind the unique aspects and strengths of both Redis and Kafka to leverage their utilities effectively. Remember to determine your specific needs to ensure you make the right technological choices in your event sourcing strategy.Let’s dive into a deep analysis of two popular message queuing systems: Redis and Apache Kafka. By doing so, we’ll be able to draw clear distinctions between these two technologies, enlightening us on their unique characteristics and optimal use cases.
Redis:
Redis, an acronym for Remote Dictionary Server, is popularly known as an in-memory data structure store that can be used as a database, cache, or message broker(Redis). It places emphasis on performance, featuring milliseconds data access which makes it perfect for high-speed transactions.
One key component of Redis pertinent to our discussion is Redis Pub/Sub (Publish/Subscribe). A fundamental pattern in messaging systems, pub/sub allows services to communicate asynchronously, with the sender (publisher) pushing messages to channels and receiver(s) (subscribers) listening to those channels. Here’s a basic setup:
Publisher -> Redis (Channel 1) -> Subscriber 1 -> Subscriber 2
Apache Kafka:
This open-source stream-processing software platform developed by LinkedIn and later donated to the Apache Software Foundation operates on real-time feeds(Apache Kafka). Kafka enhances the ability of handling real-time data feeds through a publish-subscribe model significantly.
A Kafka cluster maintains feeds of messages in topics, essentially categorised “channels” where data is stored. Producers send messages (push data) to topics and consumers read messages (pull data) from topics. For the sake of illustration, observe the example below:
Producer 1 -> Kafka (Topic 1) -> Consumer 1 Producer 2
-> Consumer 2
So, what are the primary differences between Redis and Apache Kafka?
Redis | Kafka |
---|---|
Data resides in memory, making it faster for read/write operations. | Kafka stores data on disk, permitting longer retention periods. |
High availability and persistence come through Redis Sentinel and Redis Cluster. | Kafka brokers ensure high availability and fault tolerance. |
Redis Pub/Sub has no built-in partitioning; thus, scalability is handled manually. | Kafka caters for automated partitioning, promoting scalability across numerous consumers. |
Persistence can be achieved via point-in-time snapshots or append-only files(AOF). | Persistence is attained via a commit log mechanism that facilitates fast and efficient retrieval. |
These differences highlight distinct applications for both systems. Redis offers high-speed transactions ideal for caching, real-time analytics, pub/sub apps, among others. On the flip side, Kafka shines with stream processing, tracking website activity, aggregating logs from different sources, and more.
In summary, while Redis and Kafka may seem similar due to their publish-subscribe models, they have unique architectural characteristics that make them suitable for different use-cases. The decision rests upon individual project needs, infrastructure, and architectural considerations. Ultimately, appreciating their differences aids in shaping informed decisions. Happy coding!Sure, let’s dive into it.
Redis
Redis
is a popular choice when dealing with in-memory data structures store tasks. It acts as a database, cache, and message broker. Redis allows you to work with a diverse range of data types like strings, hashes, lists, sets, sorted sets with range queries, bitmaps, hyperloglogs, and geospatial indexes with radius queries.
It has built-in replication, Lua scripting, LRU eviction, transactions, and different levels of on-disk persistence and also provides high availability via Redis Sentinel and automatic partitioning with Redis Cluster.
Kafka
On the other side,
Kafka
designed by Apache Software Foundation, is a distributed event streaming platform that can publish, subscribe, store, and process streams of records in real-time. The prime objective of Kafka is to provide a unified, high-throughput, low-latency platform for handling real-time data feeds.
Kafka maintains streams of records in categories called topics. Producers write data to topics and consumers read from topics.
The Differences – Kafka vs Redis
The key differences stem primarily from their different areas of working strength. While both can be leveraged for real-time processing, they do provide the unique set of services.
Data Structure:
Redis supports more complex data structures such as lists and sets in addition to simpler key-value pairs. Kafka, on the other hand, operates purely on a flat log structure which is an unchangeable, ordered record of messages.
Persistence:
Kafka persists all events on the disk for a configurable amount of time (which could potentially be indefinite), whereas Redis’ key-value store can disappear if it runs out memory because it was originally designed as an in-memory cache. A Persistence operation for Redis requires a snapshot of the entire state in memory, which can be expensive in terms of resources.
Scalability:
Kafka is more horizontally scalable and designed explicitly for a distributed environment whereas Redis achieves much of its performance through single-threaded operations and minimizes network hops for any operation. In terms of scalability and distributed data processing, Kafka might have the advantage due to its innate nature meant for distributed environments.
Usage:
Generally, Redis should be chosen if you need to deal with cache functionalities, message queuing with complex data, or real-time analytics. But if you need to deal with a massive stream of events in real time or perform continual real-time processing of real-time data, Apache Kafka would likely be the better choice.
You can refer to the official documentation for a more detailed understanding:
Redis
Apache Kafka
Sample code snippet:
To give you insight into how you might use both technologies in your application development, here are some basic code snippets.
Publishing a message to Redis pub/sub:
const redis = require('redis'); let publisher = redis.createClient(); publisher.publish("sample-channel", "Hello World!");
Publishing a message to Kafka:
const kafka = require('kafka-node'); let Producer = kafka.Producer; let client = new kafka.KafkaClient({kafkaHost: 'localhost:9092'}); let producer = new Producer(client); let payload = [{ topic: 'sample-topic', messages: 'Hello World!' }]; producer.on('ready', function () { producer.send(payload, function (err, data) { console.log(data); }); });
Reference:
Redis use case
Apache Kafka use case
From my experiences and learnings as a professional coder, it’s undeniable that both Redis and Kafka have developed a robust reputation for exceptional performance in their respective uses. However, there are considerable differences when it comes to the support networks provided for both technologies.
Redis’s Support Network
Redis is supported by an active, burgeoning community. There are numerous online platforms and forums where developers can seek help, share their insights and discuss any challenges they might encounter – such as GitHub, StackOverflow, or the official Redis website with a dedicated community page redis.io/community.
Furthermore:
- The source code for Redis is readily accessible on GitHub github.com/redis/redis, which allows developers across the world to contribute to improving its functionality and fixing bugs. This creates a decentralized, open-source environment encouraging growth and technological advancement.
- There are also plenty of learning resources available, including tutorials, documentation, whitepapers, and webinars that developers can leverage to expand their understanding.
- For more serious users who desire guaranteed response times or require assistance with complicated issues, there are commercial support plans available from Redis Labs.
Here is an example of how to use Redis in Python:
import redis r = redis.Redis(host='localhost', port=6379, db=0) r.set('name', 'John Doe') print(r.get('name'))
Kafka’s Support Network
Just like Redis, Kafka also has a strong presence on similar platforms, like StackOverflow, GitHub, and its own Apache-based website with an actively engaged community where professionals can connect and collaborate kafka.apache.org.
The details of Kafka support:
- A lot of back-end support where anyone can improve Kafka functionality is present through full code access on Github github.com/apache/kafka.
- Apache Kafka technology has robust documentation covering all aspects of Kafka concepts, APIs, implementation details and so forth. There are books, blog posts, webinars, freely accessible online.
- Apache software foundation provides more hands-on interactions and community events to foster knowledge sharing, innovation, and camaraderie amongst Kafka users.
- In addition, Confluent offers a complete platform built around Kafka with commercial support services for enterprise-level application.
An example of using Kafka in Python looks something like this:
from kafka import KafkaProducer producer = KafkaProducer(bootstrap_servers='localhost:9092') producer.send('sample-topic', b'Hello, World!') producer.flush()
Understanding these various considerations is crucial in choosing whether Redis or Kafka, each offering unique value propositions and drawbacks, would be best suited to meet a project’s requirements. Moreover, it sheds light on the critical role that active support networks play in enhancing user experience and driving technological optimizations.From the perspective of a professional coder, it’s essential to comprehend the core differences between two powerful technologies such as Redis and Kafka. Both these technologies operate in an entirely different field but are immensely efficient and reliable in solving problems within their field of competence.
Redis is a high-speed storage system used for caching, message broker and database tasks, featuring a data structure server. It augments the speed because of its ability to store data in memory, not on disk. One can effortlessly perform operations like pushing a new element to a list or incrementing the value in a hash. Additionally, Redis supports varied data structures such as strings, hashes, lists, sets etc (source).
// JavaScript code representation for redis to save data const redis = require('redis'); let client = redis.createClient(); client.on('connect', function() { console.log('Connected to Redis...'); client.set('framework', 'AngularJS'); });
On the other hand, Kafka provides a horizontally scalable messaging system, essentially used for building real-time data pipeline patterns. This distributed event streaming platform sees wide application in big data and streaming applications. Common use-cases include stream processing, website activity tracking, metrics collection and monitoring, log aggregation. Kafka keeps longer records and allows the possibility of reprocessing the past events (source).
// Producer and consumer example in Apache Kafka // PRODUCER Properties props = new Properties(); props.put("bootstrap.servers", "localhost:9092"); props.put("key.serializer", "org.apache.kafka.common.serialization.StringSerializer"); props.put("value.serializer", "org.apache.kafka.common.serialization.StringSerializer"); Producerproducer = new KafkaProducer (props); producer.send(new ProducerRecord ("testTopic","Hello, World!")); producer.close(); // CONSUMER Properties props = new Properties(); props.put("bootstrap.servers", "localhost:9092"); props.put("group.id", "testGroup"); props.put("key.deserializer", "org.apache.kafka.common.serialization.StringDeserializer"); props.put("value.deserializer", "org.apache.kafka.common.serialization.StringDeserializer"); KafkaConsumer consumer = new KafkaConsumer (props); consumer.subscribe(Arrays.asList("testTopic")); for (int i=0; i<100; i++) { ConsumerRecords records = consumer.poll(100); for (ConsumerRecord record : records) System.out.println(record.value()); }
Ultimately, both Redis and Kafka are fundamentally divergent. The primary variance lies in their core purposes and use cases, with Redis being more tilted towards fast, in-memory key-value storage, supporting different types of data structure, whereas Kafka exists to provide a single unified, high-throughput, fault-tolerant streaming platform. Their usage entirely depends upon the specifications required by a project and neither can replace the other wholly due to their unique offerings.