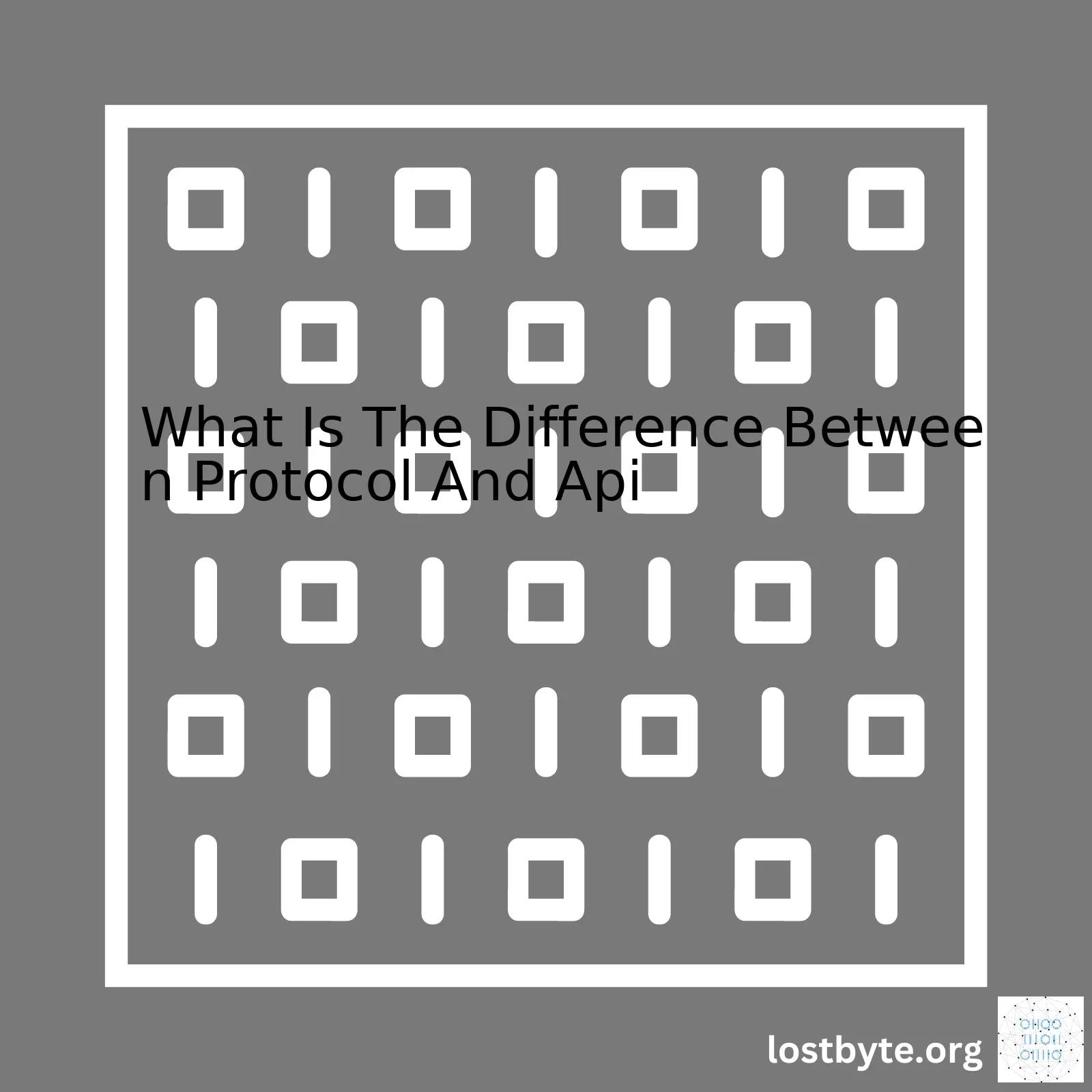
Protocol | API |
---|---|
A protocol is a standard or set of rules that define how data should be formatted, transmitted and received. It gives devices on network understand each other when they communicate. Protocols cover a broad scope including error handling and data compression. | An API (Application Programming Interface), in contrast, is a set of defined methods of communication between software components. It allows different software applications to interact with each other. APIs are used across all types of programming languages and can be customized to suit specific needs. |
Protocols and APIs may seem similar because they both facilitate communication, but they perform these tasks at different levels and have unique features. To illustrate, let’s take HTTP (Hypertext Transfer Protocol) as an example. HTTP is a protocol used for transmitting hypertext over the Internet. It has a standardized set of rules for how requests and responses should be structured, with methods such as GET, POST, PUT, DELETE etc. When you type a URL into your web browser, this actually sends an HTTP command to a web server directing it to fetch and transmit the requested page.
Contrastingly, let’s say we’re dealing with Facebook’s Graph API, which lets us connect our application with various Facebook services – that involves retrieving user data, posting on their wall, sending messages, and so forth. This API specifies how software components should interact and enables developers to create, read, update, or delete data through that API.
There is a degree of overlap in that APIs often use protocols (e.g., HTTP) as part of their operation. However, the key distinction lies in their purpose: protocols like HTTP govern the network communication rules, enabling information exchange across devices, while APIs like Facebook’s Graph API provide a scaffold for building software applications that interact with other systems, using those network protocols. The two concepts thus provide complementary functionalities: one sets the communication ground rules, the other leverages those rules to enable meaningful interactions between systems.
For further reading on Protocols and APIs, the [MuleSoft] article provides an in-depth perspective on APIs. Concurrently the [SearchUnifiedCommunications] article serves useful information about protocols. As a coder, understanding the difference and relationship between these protocols and APIs will help in designing and developing robust applications.
// Sample code showing usage of Facebook's Graph API. // NOTE: This is a conceptual example and might not work correctly in a real-world context. const FB = require('fb'); FB.api( "/me", function (response) { if (response && !response.error) { /* handle the result */ console.log(response); } } );
In the above code snippet, we make use of Facebook’s Graph API to retrieve information about the logged-in user. The ‘FB.api’ function allows for sending HTTP requests to the Facebook API following the guidelines that the Facebook API provides. The HTTP protocol ensures that these requests and responses follow a set pattern so that every party involved in the process knows what to expect.Protocols and APIs are two very important concepts in the world of software development. Their functionalities are quite essential, both to client-server communication and for application integration. However, while they have certain similarities, it’s crucial to clarify that they are decidedly different concepts.
What is API?
An API (Application Programming Interface) is simply a set of rules and protocols designed to allow different software applications to communicate. APIs provide a specification of software components in terms of their operations, inputs, and outputs. These functions can then be called by other applications, thereby enabling interface and integration between different software systems.
For instance, consider a website like Facebook. To develop a mobile application, developers will require the Facebook API. This API provides methods to perform operations such as retrieving user information, posts, friends lists etc – essentially allowing controlled access to Facebook’s data.
APIs are present all around us. Every time we use an app like Facebook, send an instant message, or check the weather on our phone, we’re making use of API.
import requests def get_weather(city): response = requests.get(f'https://api.openweathermap.org/data/2.5/weather?q={city}&appid=') return response.json() print(get_weather('London'))
In this simple snippet of code, you see how APIs work. By sending a GET request to the ‘openweather’ API endpoint with the appropriate query parameters, you fetch weather data related to London.
What is Protocol?
In the field of networking, a protocol represents standard rules that dictate how data is transmitted over the internet or any other network. In essence, these are conventions or sets of rules implemented to achieve uniformity and understanding in technology development and usage.
Consider HTTP (HyperText Transfer Protocol), for example. It’s a protocol which governs the transfer of hypertext (any text that contains links to other texts) across the network. Almost every function carried out on the world wide web follows the standards set by HTTP. This consistency allows global users to experience seamless internet browsing.
Another commonly employed protocol is FTP (File Transfer Protocol), used for transferring files between computers on a network.
A demonstration of HTTP protocol:
When you type
http://www.google.com
in your browser,
– Your computer sends an HTTP GET Request to the Google server.
– The server processes the request and sends back the appropriate HTML file in an HTTP Response.
– Your browser then interprets the HTML file to display the Google homepage.
Difference between API and Protocol
Despite their similarities, one key difference between API and Protocol is their level of abstraction. APIs act at a high level of abstraction, offering a programming interface to interact with a software component. On the other hand, protocols operate at a lower level, stipulating the rules governing network communication.
Their uses also vary significantly:
– APIs are majorly used to connect different software applications, enabling data exchange and functionality sharing.
– Protocols, are mostly seen managing communications over networks.
Here’s a tabular comparison for easier visualization:
API | Protocol | |
---|---|---|
Definition | A set of rules for building software and applications. | A set of rules that governs the data communication mechanisms over the network. |
Level | High-level abstraction for interacting with software components. | Low-level rules for network communication. |
Use | Connects different software applications enabling data exchange and sharing of functionalities. | Manages data communications over the network. |
To round up, it’s safe to say that both API and protocol serve equally crucial roles in the computing world. APIs are the connectors linking disparate software systems, bringing about interconnectivity across platforms. Protocols, on the other hand, ensure stability and efficient networking communication within the digital space.
While an API could embody protocols since it defines how components should interact, including considerations for network communication, a protocol cannot substitute an API since its responsibility is at a lower level, closer to the infrastructure that facilitates digital communications.
References:
1. RedHat — What is an API?
2. TechTerms — Definition of ProtocolThinking about the Internet or any computing system, we can compare it to an intricate web of technologies interacting and communicating with one another. In this bustling hub, two components—an API (Application Programming Interface) and a protocol—are integral facilitators of interactions. They might seem similar on the surface, but they actually cater to different layers of communication.
Protocol
A protocol defines a standard set of rules that computers follow to facilitate data transmission. It governs how data is sent, received, and interpreted across networks. For example, the Hypertext Transfer Protocol (HTTP)—a protocol widely known—circumscribes the rules for transferring or ‘serving’ hypertext (e.g., web pages) over the internet. An HTTP transaction involves a client (browser) sending a request to a server and then awaiting its response. Here’s a simple example of an HTTP request:
GET /index.html HTTP/1.1 Host: www.example.com
This command says “Get me the index.html file using HTTP version 1.1 from the host at www.example.com.” This set pattern of composing messages is part of the HTTP protocol specification.
API
On the other hand, an API serves as a contract between different software applications. It specifies how software components should interact. It’s like a menu in a restaurant – the interface (menu) outlines what dishes (functions) you can request from the kitchen (software application).
For instance, Google Maps API allows developers to embed Google Maps on webpages using JavaScript or Flash. When calling the Google Maps API, the caller doesn’t need to be aware of all the complexity behind rendering maps, calculating routes, etc. Instead, they just have to follow the API guidelines to request specific operations.
Consider the following code snippet where the Maps API is used to create a map:
var map; function initMap() { map = new google.maps.Map(document.getElementById('map'), { center: {lat: -34.397, lng: 150.644}, zoom: 8 }); }
Differences Between an API and a Protocol
Now are APIs and protocols fundamentally different? Yes, they are!
• Purpose: Protocols define the rules for data exchange over networks. They operate at lower network layers and regulate information flow at a more fundamental level. On the other hand, APIs simplify access to functionalities of a software system at a high level.
• Examples: Examples of protocols include HTTP, FTP (File Transfer Protocol), and SMTP (Simple Mail Transfer Protocol). These focus on the transmission of data between systems. Examples of APIs include the Google Maps API, Twitter API, and Amazon Product Advertising API. These provide predefined ways of interacting with software applications.
• Levels of Abstraction: Protocols usually operate at lower levels of abstraction, dealing with raw data transfer, while APIs work at higher levels, hiding the underlying details from the developer, enriching their programming experience.
In summary, both protocols and APIs play important roles in facilitating efficient digital communication. Moreover, these two concepts frequently intertwine. I’m sure you’ve come across mentions of the “Twitter API over HTTP”–an example of an API utilizing a specific protocol (HTTP). Comprehending the difference between them provides a broader understanding of how data flows across systems, allowing you to select the appropriate tool during development.
Additional reads for a better understanding of these subjects can be found here:
Developer Mozilla – HTTP Overview,
Google Maps APIs | Google Developers.The terms API (Application Programming Interface) and protocol often cause confusion as they are both fundamental components of software development. Let’s provide a concise breakdown to demystify these two.
API: The Hero Behind the Operation
API is like the backstage manager of a concert – not immediately visible, but incredibly crucial for the operation to run smoothly. APIs are essentially sets of rules that dictate how software components should interact and communicate with each other. They define methods and data formats that a program can use to perform its tasks. In essence, an API provides a structure for programmers to follow when developing complex, interactive applications.
For instance, when using an application on your mobile device, the API would manage the communication between the frontend that you interface with, and the backend where all the data processing happens. Every time you interact with the app – such as sending a message or uploading a photo – this request is passed to the backend via the API, showcasing the pivotal role APIs play in running applications.
Some key points to note about APIs:
- They simplify the process of coding: Programmers can utilize existing APIs instead of coding everything from scratch. This significantly streamlines the programming process.
- Versatility: APIs come in different types (such as Web APIs, Operating Systems APIs, and Database APIs), each catering for specific uses.
- Promotes Integration: APIs stimulate seamless interaction between different software components, thereby fostering software integration.
A snippet of
API Code Example:
import requests response = requests.get('https://api.example.com/data') data = response.json() print(data)
Protocol: The Rulebook of Communication
Protocol, on the other hand, can be thought of as the language or rulebook that systems adhere to when communicating with each other. Protocols outline the syntax, semantics, and synchronization of communication. They also determine the way error messages should be handled, which offers reliability. A good example of a protocol used in API communication is HTTP (Hyper Text Transfer Protocol).
Some essential characteristics of protocols:
- Uniformity: Protocols ensure uniformity and standardization, so devices and systems manufactured by different companies can engage in meaningful inter-operations.
- Error Detection: Most communication protocols have error detection for reliable messaging functions. These mechanisms help ensure data integrity over the transmission.
- Efficiency: Protocols streamline system communication making operations more efficient.
APIs and Protocols: What is the Difference?
The primary difference between API and protocol lies in their function; API’s main objective is to connect software components together and facilitate their interaction. On the other hand, the protocol provides the rules of how this interaction will happen.
While an API entails the code that enables software programs to communicate, a protocol is the set of rules guiding the interaction between programs. You could think of the protocol as the language spoken between computers, and then API as the interpreter that allows the computers to understand each other even if they do not use the same internal software architecture.
Code has APIs inside it. But within those APIs, there will be protocols. Basically, without protocols, the API couldn’t function correctly, as it wouldn’t have a foundation of rules to build an interface upon.
So, while these terms can sometimes seem interchangeable, and they certainly work closely together, they do distinctly unique things and have critical roles in the functionality of any software ecosystem.
More on HTTP protocolCertainly. When talking about the world of software development, understanding the roles and differences between a Protocol and an API (Application Programming Interface) is essential. Let’s compare these two concepts.
Definition
APIs are a set of rules that allow different software applications to communicate with each other. They expose certain functionalities of a program or service which enable developers to use parts of it in their applications. This interaction makes it possible to not only extract data but also perform operations on it.1.
On the other hand, a Protocol is a well-documented set of rules and procedures followed to reciprocate, transfer, or communicate data between multiple devices over a network. The protocol sets the groundwork for how data is transmitted and received on a network2.
Usage
APIs have diverse usage across the globe. They are predominantly used in building software applications. A good example would be a mobile application using the Google Maps API to display location data. They are foundational for ‘machine-to-machine’ interactions.
Protocols have extensive usage in networking to establish a connection, authenticate users, maintain the connection, and ensure that data is transferred correctly. Examples include HTTP (HyperText Transfer Protocol), FTP (File Transfer Protocol).
Flexibility
APIs offer flexibility as they can be tailored to meet different needs and requirements of the developer. You could add, modify, or delete a functionality based on what your application needs to do.
Protocols provide less flexibility because they’re a standard set of rules which must be strictly adhered to for successful data transmission and communication without any issues.
Communication Means
APIs allow a program to interact with another program. An API defines methods and data formats that a program can use to communicate with the other programs provided by software components like libraries or operating systems.
Protocols define how to transport messages in a system network, with emphasis on the points, nodes, routers which ensure correct delivery, including error checking and correction.
Code Examples
Here’s an example of how an API might be utilized:
fetch('https://api.example.com/items') .then(response => response.json()) .then(data => console.log(data)) .catch(error => console.log('Error:', error));
In this JavaScript example, we’re utilizing the Fetch API to make a GET request to ‘https://api.example.com/items’. The then method is used to parse the response into JSON, and that data is outputted to the console.
And here’s an example involving the FTP protocol transferring a file:
ftp -n ftp.example.com quote USER myusername quote PASS mypassword cd /files put myfile.txt quit
This script uses the FTP protocol to connect to a server located at ‘ftp.example.com’, navigate to a ‘/files’ directory, upload a file called ‘myfile.txt,’ and then close the connection.
To summarize, while APIs and protocols carry out different tasks, both are crucial in effectively delivering information from one point to another. Protocols facilitate reliable communication over a network, whereas APIs allow for interaction between different software components.
Being proficient with both protocols and APIs provides immense power to create efficient, data-driven, and interactive solutions.
.
Resources:
1. Redhat – What are Application Programming Interfaces?
2. Introduction to Protocols in Computer Network – GeeksforGeeksThe term ‘protocol’ in systems communication refers to a set of rule-based guidelines that enables computers to correspond with each other across a network. These protocols are essential for successful data transfer and they determine how data transmitted between computing devices is formatted, sent, received, and responded to. One well-known example is the HyperText Transfer Protocol (HTTP), which forms the foundation of any data exchange on the Web, and facilitation of these exchanges is often undertaken via APIs.
On the other hand, an Application Programming Interface (API) is a set of pre-defined rules that allows different software applications to interact with each other. It’s essentially a way for different software programs to communicate and share data. APIs provide a standard way of accessing any application’s functionality or database, thereby allowing different software systems to communicate even if they were not specifically designed to do so.
Differences Between Protocols and API
Protocols | APIs |
---|---|
Used to format, send, receive, and respond to data. |
Primarily used to define functions that access features or data of an operating system, application, or service. |
Categorized as formal language that specifies sequence of actions. |
Specifies how software components should interact. |
Represents communication between/affecting hardware components. |
Pertains to software-to-software interface, not user interaction. |
Typically works at a lower level of a system. |
Works at a high level, allowing software components to communicate at a process level. |
In terms of practical applications, there is significant overlap in theory and practice. For instance, the workings of many APIs inherently include adherence to specific protocols. A good example of this comes in the form of HTTP APIs such as the REST API. REST is an architectural style used for networked hypermedia applications, primarily used in web services development. With REST, you can use standard HTTP methods to create, read, update, and delete resources through their representations.
Here’s an example of some simple API usage:
GET /employees/1
This piece of code makes a GET request to the /employees endpoint of the API, asking for the data of the employee with ID 1. This request would be handled by the server, which would return the appropriate response, likely in JSON format.
Essentially, while protocols like HTTP inform how the server and client communicate, APIs like REST structure what information actually gets sent and the prerequisites for sending it.
Further reading:
In working with modern applications, we constantly encounter APIs and protocols. APIs or Application Programming Interfaces are sets of rules that specify how one component should interact with another. On the other hand, a protocol is an established set of rules for data exchange over a network.
So, what’s the difference between Protocol and API? The primary distinction lies within their functionality and usability.
Application Programming Interface (API)
An API acts like a communication interface between two software components. It is a software intermediary enabling two applications to communicate with each other. Practically speaking, if you are using a mobile app like Facebook or Twitter – every time you send a message, upload a photo, or refresh your feed, you’re using an API.
Here’s an example of an API in Python:
import requests def get_weather(city): base_url = "http://api.openweathermap.org/data/2.5/weather" params = { 'q': city, 'appid': 'your_api_key' } response = requests.get(base_url, params=params) return response.json()
This piece of code utilises the OpenWeatherMap API to acquire real-time weather updates for a specific city.
Protocol
On the other side, a protocol outlines how algorithms should be used to achieve a specific task. In essence, protocols define the methods and properties to facilitate communication between different devices on a network. Protocols have been designed to perform various tasks such as retrieving web pages (HTTP), file transfer (FTP), and email transmission (SMTP).
For example, the HTTP (Hypertext Transfer Protocol) which is used to fetch information from servers to be displayed in the user’s web browser is given as:
GET /index.html HTTP/1.1 Host: www.example.com
Above, a basic HTTP GET request is shown. This protocol is responsible for fetching the specified URL (in this case, “index.html”).
One frequent confusion among developers is understanding HTTP as an API. But in reality, it isn’t. HTTP is a protocol. A Web API uses HTTP as its underlying communication protocol thus leveraging the built-in capabilities of the HTTP protocol methods such as GET, POST, DELETE, etc.
So in conclusion:
- APIs exist in the application layer: They allow for interaction between software applications. These can be systems calls, library routines, or window systems, essentially helping different software components communicate.
- Protocols exist in the transport (or internet) layer: They support communication between devices over a network. Protocols such as TCP/IP, UDP, HTTP, FTP help in delivering messages across the network from one system to the other.
With these points made, while protocols are more concerned with data transportation following certain rules, APIs are more about action and taking control of the system. More informatively speaking, we can say that APIs are like the control panel of an application/microservice, whereas protocols ensure the commands delivered via that panel reach their destination reliably. Therefore, both APIs and protocols play a crucial role in software interactions today.
Even when they might seem similar at first glance, API and protocol both have distinctive functions aiding different processes. To serve our everyday digital needs, these two elements work together harmoniously, making interactions smoother and services more reachable.
When understanding the digital world, two essential concepts that emerge are Protocols and APIs (Application Programming Interfaces).
The Protocol
In computing terms, a protocol is comparable to a conversation conductor or a set of rules and standards used for data communication across different devices. These protocols dictate how this electronic dialogue should take place, ensuring successful data transactions. For instance, the Hypertext Transfer Protocol (HTTP), which is commonly used in internet browsing, dictates the format and transmission mode of the request-response cycle in web communications.
// A common HTTP request structure GET /index.html HTTP/1.1 Host: www.example.com
The process operates with a client (usually a web browser) sending an HTTP request to a server, awaiting an HTTP response, much like ordering a meal at a restaurant.
The API
An API, on the other hand, acts as a go-between, communicating between different software applications. The API specifies the paradigm for making requests for specific data or functionalities from one program to another. It’s kind of a menu for developers offering a list of functional dishes they can “order” (call) and use in their own programs.
// A popular PHP API request example $curl = curl_init(); curl_setopt_array($curl, array( CURLOPT_URL => "http://api.example.com/data", CURLOPT_RETURNTRANSFER => true, CURLOPT_TIMEOUT => 30 )); $response = curl_exec($curl); $err = curl_error($curl); curl_close($curl);
This piece of code might be an API query where we reach out to ‘api.example.com’ and ask it for ‘data’. This could be anything, from requesting users’ information to weather forecasts from a certain location – depending on the capabilities of the API.
So, What’s Their Relationship?
In essence, the key difference lies in their roles. A protocol orchestrates how information travels, while an API establishes what is transmitted and its representation. They work together in numerous ways.
- A protocol can be seen as a delivery pathway, while an API works like the specific content in the package navigating down this pathway. When building a house, for instance, a protocol could comprise the rules and guidelines for moving construction materials from point A to B safely (traffic rules, speed limits, weight restrictions). An API, conversely, would be the blueprint, dictating how those materials are used to build the house once delivered.
- APIs often use protocols during operations. In most cases, APIs actually function atop protocols. APIs use protocols to determine specifics for delivering a particular message or data packet. API calls frequently happen over HTTP protocol, establishing a connection policy for seamless interaction between servers and clients.
It’s important, however, to remember that not all APIs are going to operate over the network or utilize network protocols. Those that do are typically referred to as Web APIs.
To understand this better, take a look at the table below showing the relationship of these two concepts concerning web development.
Protocol | API |
---|---|
Lays down the rules for data communication | Defines methods and data formats that an application can use to communicate with other applications |
Primarily implemented in the network layer of a network model | Implemented in the application layer of a network model |
Examples: HTTP, FTP, POP3, SMTP | Examples: REST API, GraphQL API, SOAP API |
Understanding these differences can help comprehend your next online interaction better and become a more adept developer if you’re exploring that path.
Before diving into the specifics of case studies on protocol usage, it’s important to establish a clear understanding of two key terms: protocols and APIs. A protocol is a set of rules or standards used by systems to communicate with each other. An essential part of computer networking, it defines how information should be structured, formatted, transmitted, and acknowledged. Examples of protocols include HTTP (Hypertext Transfer Protocol), FTP (File Transfer Protocol), and POP3 (Post Office Protocol 3).
On the contrary, an API (Application Programming Interface) serves as an interface between different software programs, making it possible for them to interact with each other. It exposes certain functions and methods which allows third-party applications to utilize the features or data of an existing program, without revealing the underlying source code. An example of API is Google Maps API that can be integrated into any website, yielding location-driven functionality.
Hence, while both are communication enablers, their purpose and use-case scenarios differ significantly. Protocols dictate ‘how’ the interaction occurs, whereas APIs determine ‘what’ can be exchanged during the interaction.
Let us now consider some case studies to examine the application of protocols and compare those with APIs:
Case Study A – Email Service
Email services provide an excellent example of protocols at work.
-
SMTP (Simple Mail Transfer Protocol)
: Used for sending emails.
-
IMAP (Internet Message Access Protocol)/POP3
: Used for receiving emails.
In this scenario, the email server and client utilize these protocols to send and retrieve emails effectively.
Contrastingly, if a developer wants to build an application that has access to a user’s email account, they would use an API, like Gmail API, provided by the email service. This API can fetch unread emails, send emails, and perform all operations an email client can.
Case Study B – Web Browsing
Web browsing primarily involves the HTTP/ HTTPS protocol. When you enter a URL in your browser:
- The browser sends an HTTP request to the server.
- The server processes this request and responds with HTML, CSS, JavaScript files.
- The browser interprets these files and presents the webpage.
Here, HTTP is the protocol defining this sequence of actions.
In contrast, should a developer want to build a third-party app requiring weather updates from a weather site, they’d need to use the Weather Site’s API. The API will provide data according to the calls allowed by its interface.
Hopefully, these examples clarify the distinctions and operational mechanics amongst the APIs and Protocols. Recognizing this difference offers a comprehensive understanding of how apps and services communicate, thus nurturing more effective and accurate programming decisions. Partaking in deep analysis of such case studies provides further insight for budding developers and programmers into network communication nuances and techniques.Diving straight into the investigation, let’s begin with understanding what APIs (Application Programming Interfaces) and Protocols are. In a nontechnical parlance, think of an API as a waiter in a restaurant taking your order. This waiter is responsible for conveying your request to the kitchen and then bringing back the dish you asked for.
As for protocols, they can be treated like the set of rules or the language that the waiter and the cook follow to understand each other. HTTP (Hypertext Transfer Protocol) may serve as an example – just like English, it’s used by clients (browsers) to communicate with web servers.
However, this generalized summary does not do justice to understanding our topic in its entirety. To delve further into API and Protocol, we get to the crux of the differences.
Difference between API and Protocol
API | Protocol |
---|---|
APIs refer to a set of rules and procedures that software applications can follow to accomplish a specific task. | Protocol refers to a set of rules that govern how data is transmitted over a network. |
Functionality provided by an API includes procedures, functions, routines, or commands used by programmers to develop software or facilitate interaction between distinct systems. | Protocols govern the way networks send and receive packets of data, ensuring reliable communication. |
Hereafter, let’s consider two real-world examples that underline the use of APIs and Protocols: Weather Application and Emailing System:
1. A Weather Application:
Take a mobile app; say we’re examining weather forecast applications. These apps utilize APIs to fetch data from remote weather stations or services.
fetch(weatherAPI_endpoint) .then(response => response.json()) .then(data => /* use this data to populate your weather app */);
The actual process involved here uses HTTP protocol to send a GET request to the weather server, fetch the data in JSON format, and then the API helps in manipulating the received data to suitably present it on the application interface.
2. An Emailing System:
Consider how SMTP (Simple Mail Transfer Protocol) is used to send e-mails. On hitting ‘Send’, your email client communicates with the SMTP server using a series of commands outlined by the SMTP protocol.
MAIL FROM:RCPT TO: DATA Subject: Hello World! . QUIT
In this scenario, a preset API would enable developers to simply institute ‘MAIL FROM’ field, ‘RCPT TO’ field, etc., rather than writing whole sets of instructions manually. APIs make it smooth to work with different protocols for numerous functionalities across diverse domains.
While APIs and Protocols might be intertwined in usage, discerning their characteristics brings clarity as we continue exploring technical frameworks. Utilizing APIs allows for an enhanced integration, proficient code reutilization, better security, and efficient adaptivity with changing trends. Equally important is understanding the role of protocols that ensure reliable communication in exchanging the data across networks.
Remember, mastering both these concepts makes it much easier to maneuver the modern world of programming as they comprise essential components in software development.
Source 1
Source 2If we consider the digital world as a large organization, APIs (Application Programming Interfaces) and protocols are essentially the communication system that support its daily operations. Protocols define the rules for communication among diverse elements in a network while API is more like a middleman which facilitates the interaction between two software programs.
Understanding Protocols
In the realm of digital communication, a protocol can be compared to a language. It’s a set of standardized rules that allows computers to communicate. These rules outline specific sequences, methods, and data formats that each participating node on a network must follow in order to communicate without encountering any conflict.
Key elements of protocol include:
- Syntax: It defines how exactly the data should be structured, encapsulated, and represented.
- Semantics: It outlines the rules for sending and receiving data packets.
- Timing: It stipulates speed for sending and receiving data and synchronization.
Examples of protocols used in computer networks include TCP/IP, HTTP, FTP, and SMTP. Each has its own rules and is applicable to specific situations.
Below is an example of how a HTTP Get Request protocol works:
GET /index.html HTTP/1.1 Host: www.example.com
Here, ‘GET’ is the method defined by HTTP protocol to retrieve information, ‘/index.html’ is the file to be fetched, and ‘HTTP/1.1’ specifies the version of the protocol.
Understanding APIs
APIs, while serving as interfaces that allow different software entities to interact, do not inherently specify how communication occurs. They come into play when one application wants to utilise the functionality of another. This interface includes specific commands, functions, protocols and objects that an application can use to interact with the operating system, another service or software component.
For instance, if you’re running an online store, your programmer wouldn’t need to figure out how to process credit cards, remember user preferences or even work out complex product sorting algorithms. All that heavy lifting is done by APIs which simply let your site know whether a transaction was successful or not and receive data accordingly.
Consider this Python code snippet showing how a Twitter API might be used:
import twitter api = twitter.Api(consumer_key='consumer_key', consumer_secret='consumer_secret', access_token_key='access_token', access_token_secret='access_token_secret') status = api.PostUpdate('I love python-twitter!') print(status.text)
Here, the third party (Twitter) handles all the underlying processes of posting a tweet, while the first party (the Python program) just sends a request through the API and waits for the response.
Difference Between Protocols and APIs
Both APIs and protocols are fundamental to our digital communications, aligning diverse systems and applications to effectively share information. Despite their similar purpose, they differ in their functions and usage:
- While protocols set the rules of communication, APIs are the interfaces that allow this communication.
- Protocols can operate independently but APIs generally work in conjunction with other software components.
- A protocol applies universally across all interactions of a certain type, whereas an API typically provides access to a specific software application or service.
The impact of protocols and APIs on the digital world is undeniable. From letting us update statuses on social media to enabling credit card processing on e-commerce sites- these ‘invisible heroes’ behind the scenes make it possible for us to live in a seamlessly connected world. Any piece of software we use daily synonymous with a web link MDN Web Docs can function effectively on the internet thanks to complex layers of APIs and underlying protocols working together harmoniously. Authentication, data exchange, and real-time updates are few amongst the many operations underpinned by APIs and protocols alike.Diving deep into the world of technology, it’s essential to distinguish between two fundamental terms – Protocol and API. While both terms might seem interchangeable at first glimpse, they serve strikingly different purposes in software development.
Let’s take a sail through and bring some clarity on this topic.
Protocol is essentially an agreed-upon set of rules that define how data communication occurs in computing systems. These are like instructions or blueprint for network devices to interact, thereby establishing successful data transmission. Examples include HTTP, TCP/IP, SMTP–each designed to handle specific types of communication for varied purposes spanning web browsing, emails, and more. A protocol informs how packets should be formatted and addressed to seamlessly travel across the network.
Here’s a sample representation depicting typical protocol structure with its key components:
Data Unit | Key elements |
---|---|
PDU (Protocol Data Units) | Source/Destination address, Error detection, Control information |
Coming back to API or Application Programming Interface, think of it as a messenger that enables different software applications to communicate and share data amongst each other. APIs lay down the groundwork on how exactly this interaction should take place. They provide predefined classes, procedures, functions that work as building blocks to develop a software application. For instance, social media platforms like Facebook or Twitter use APIs that allow third-party websites to integrate their functionalities within those sites.
Look at the following example, demonstrating how you can get user details using Twitter’s API:
#!/usr/bin/python import twitter api = twitter.Api(consumer_key='consumer_key', consumer_secret='consumer_secret', access_token_key='access_token', access_token_secret='access_token_secret') print(api.VerifyCredentials())
In simpler terms, if we compare both these concepts to real-world logistics: a Protocol would relate to traffic laws dictating rules for vehicles to move around while an API could be likened to shipping labels that carry destination details, content type, etc., enabling delivery of the parcel to the right place.
Creating a clear distinction between Protocol and API brings a newfound lucidity in understanding the interoperability of software components—each playing a significant role in ensuring smooth digital communication. This understanding results in effective application development processes, ultimately fostering superior digital experiences.
Do refer to Search App Architecture and Techopedia for further insights on Protocols and APIs.