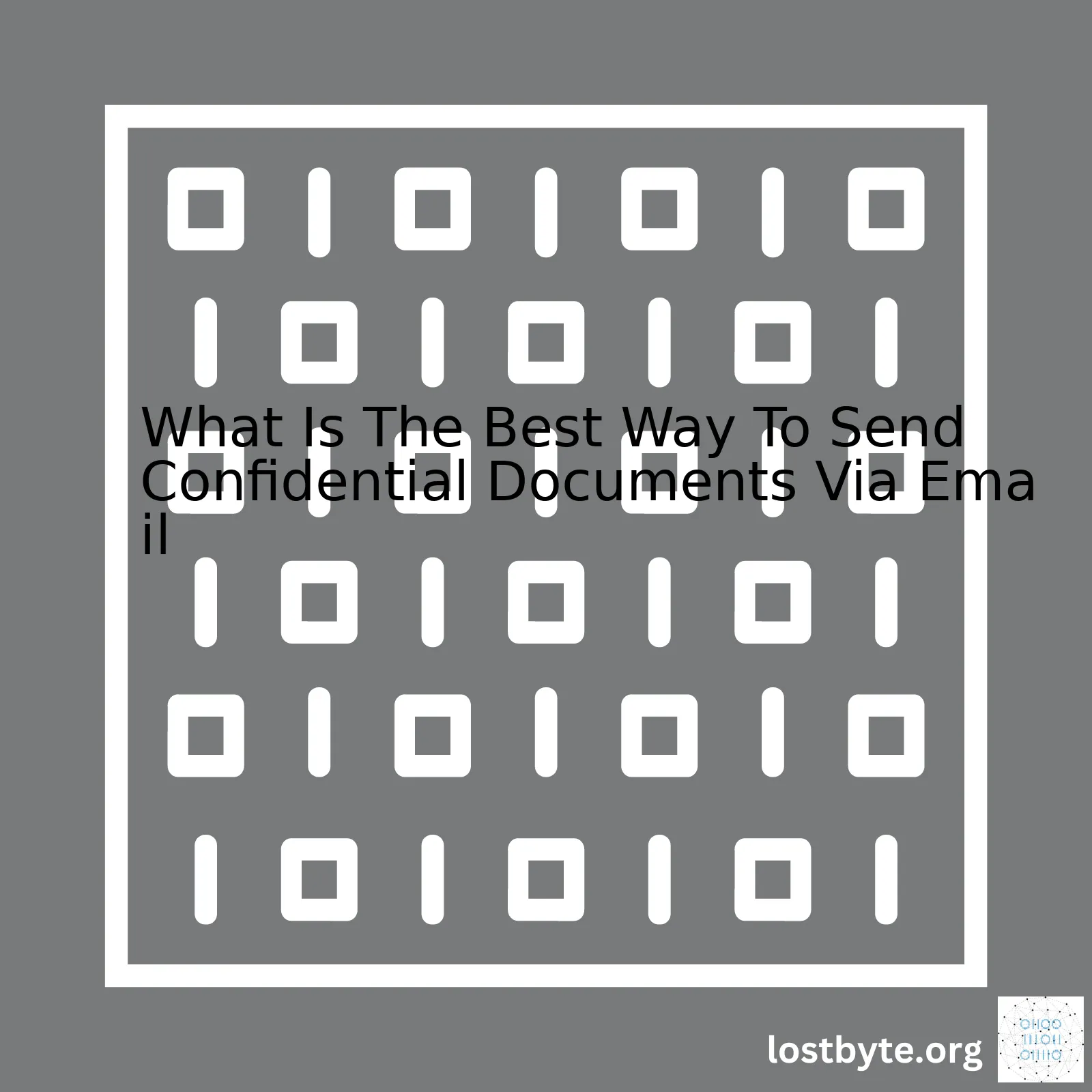
Method | Security Level | Ease of Use |
---|---|---|
Email Encryption | High | Intermediate – Requires understanding encryption protocols |
Password Protected Files | Medium | Easy – Most software provide this feature |
Secure Cloud Services | High | Easy – User-friendly interfaces and accessible across multiple devices |
Personal Document Management Systems | Very High | Advanced – Requires investment into infrastructure |
When it comes to transferring sensitive information such as confidential documents via email, security is a paramount factor. You may want to take preventative measures to ensure your data’s safety and privacy. Here are some top-tier methods that tech experts suggest: Email encryption, password protected files, secure cloud services, and personal document management systems.
Email Encryption: This involves encoding the content of the email so that only the person with the correct decryption key can read it. Tools like PGP (OpenPGP) enable end-to-end encryption of emails, protecting the confidentiality while in transit.
//An example code on how to use OpenPGP
const openpgp = require('openpgp');
const passphrase = 'your pass phrase';
(async () => {
const { keys: [privateKey] } = await openpgp.key.readArmored(yourPrivateKey);
await privateKey.decrypt(passphrase);
const { data: decrypted } = await openpgp.decrypt({
message: await openpgp.message.readArmored(encryptedMessage),
privateKeys: [privateKey]
});
console.log(decrypted); // "Hello, World!"
})();
Password Protected Files: Adding a password to a document adds an extra level of security. If the email is intercepted, the interceptor would need to know the password to open the document. Many commonly used systems like Microsoft Word or Adobe Acrobat allow users to add passwords to their files.
Secure Cloud Services: These platforms (like Google Drive, Dropbox, Box, etc.) allow you to upload the document, then share a link to the document via email. The document itself is never sent through email, making interception impossible. Some services even let you set expiration dates for the links and revoke access at any time.
Personal Document Management Systems (DMS): More secure than most other options, DMS is ideal if transmitting confidential documents is routine in your line of work. It enables you to control who can view the document, track activity related to the document, and maintain versions of the document. However, setting up DMS infrastructure requires significant investment, both in terms of money and time.
Each method offers a varying degree of security and ease of use. So when choosing, consider what best suits your needs in terms of time, resources, technical expertise and the importance of the documents being transferred.
Recognizing the significance of confidentiality in email communications can be crucial, particularly when it comes to transmitting sensitive documents. It’s not just about protecting data, but also about retaining credibility, ensuring compliance with regulations, and safeguarding your professional stature. Propagation of confidential information might lead to undesired exposure, which could trigger significant corporate problems. As we delve into this subject more deeply, let’s focus on how best to send confidential documents via email.
Encrypt Your Messages
One of the most vital defenses for your confidential emails is encryption. It transforms your content into a secret cipher that only somebody with the suitable decryption key can decode. You can use tools like “Office 365 Message Encryption” or “Google’s Confidential Mode”.
To turn on Google’s Confidential mode:
xxxxxxxxxx
Click Compose.
In the bottom right of the window, click Turn on confidential mode .
Set an expiration date and passcode.
Click Save.
Make sure to source reputable tools to handle your encryption needs. These would typically come directly from your email provider, such as Microsoft’s own encryption for Office 365, or from respected cybersecurity organizations.
Use Secure Email Providers
Certain email providers are dedicated to giving secure, encrypted email services. They typically use end-to-end encryption, which means even they can’t read your emails. Services like ProtonMail, Tutanota, and Hushmail are recognized for their emphasis on privacy and security.
Password Protect Documents
Before sending off a confidential document, password-protecting it adds another layer of protection. This way, even if the email falls into the wrong hands, accessing the file itself will still require a password. Softwares like Microsoft Word or Adobe Acrobat allow you to easily do this.
For example, in Word:
xxxxxxxxxx
Click File > Info > Protect Document > Encrypt with Password.
Type in a password then press OK.
Reenter your password and click OK again.
Email Less Sensitive Information
Minimize risks by emailing less susceptible data. If feasible, aim to transmit sensitive information through internal secure systems, such as company-owned databases or authorized data sharing tools.
Always Double-Check Recipients
Mistakes happen! Always double-check recipients before hitting the send button. One simple error could result in confidential documents being sent to the wrong person, resulting in potential data breaches.
By following these practices, you can ensure that your confidential documents remain secure while transferring via email. Being aware of the importance of confidentiality and employing appropriate measures to enforce it is crucial in the current digital environment. Knowing the best ways to send confidential documents via email aids in this endeavor, offering peace of mind to both sender and recipient alike.
Please remember: while these are all efficient methods to ensure the safe delivery of confidential information, no system is fully foolproof. Continue to stay updated on the latest cybersecurity developments to make sure you’re using the most score strategies.When addressing the issue of sending confidential documents via email, encryption methods come handy in safeguarding your information. There are various ways to encrypt emails and they all contribute to minimizing the risk of unauthorized access during transmission.
To begin with, there’s a traditional method known as Secure/Multipurpose Internet Mail Extensions (S/MIME). S/MIME encrypts your emails using private and public key pairs. The sender uses their private key to digitally sign the email. By doing so, it assures the recipient that the content has not been tampered with during transit. Additionally, if the sender would like to encrypt the message, they can use the public key of the receiver, ensuring only the intended receiver will be able to decrypt it with their private key[1].
Here is an example showing what S/MIME encoding might look like in python.
xxxxxxxxxx
from M2Crypto import BIO, Rand, SMIME, X509
# Make a MemoryBuffer of the message.
buf = BIO.MemoryBuffer(data)
# Seed the PRNG.
Rand.load_file('randpool.dat', -1)
# Instantiate an SMIME object.
s = SMIME.SMIME()
# Load private key and cert.
s.load_key('key.pem', 'cert.pem')
# Encrypt the buffer.
p7 = s.encrypt(buf)
# Output p7 in mail-friendly format.
out = BIO.MemoryBuffer()
s.write(out, p7)
# Save the PRNG's state.
Rand.save_file('randpool.dat')
Next is Pretty Good Privacy (PGP), another useful email encryption method. PGP uses both symmetric and asymmetric encryption to achieve its goal. Symmetric encryption is fast but requires secure key transfer whereas asymmetric encryption does not require secret key transfer but is slower. PGP combines the advantages of the two; the document or message is encrypted using a random symmetric key, and then the symmetric key itself is encrypted using the recipient’s public key.
Here is a Python code snippet for PGP encryption using GNUPG[2].
xxxxxxxxxx
import gnupg
def encrypt_data(data):
gpg = gnupg.GPG(gnupghome='/path/to/keyring/directory')
encrypted_data = gpg.encrypt(data, recipients=['reciever@domain.com'])
return str(encrypted_data)
data = "Confidential Information"
print encrypt_data(data)
In addition, there are modern platforms, such as ProtonMail and Tutanota, that offer end-to-end encryption services. These platforms ensure your email content is encrypted before leaving your device and can only be decrypted once it reaches the recipient’s device.
A more straightforward method to consider would be the use of password-protected files. Many data processing platforms including Microsoft Office, Adobe Acrobat and WinRAR support creating password-protected files. This ensures that the document can’t be opened without the correct password. Here is an example from the Microsoft website on how you can do it in Word:
xxxxxxxxxx
Document = Application.Documents.Open ('C:\\....docx');
Document.Protect (
Type:=Microsoft.Office.Interop.Word.WdProtectionType.wdAllowOnlyReading,
NoReset:=false,
Password:="password",
UseIRM:=false,
EnforceStyleLock:=false);
Document.Save();
Document.Close();
Bear in mind, though reliable, these methods are not foolproof and users should follow best practices such as keeping software up-to-date, using strong passwords, and verifying the recipients’ identities.
Sources:
[1] RFC 8551 – Secure/Multipurpose Internet Mail Extensions (S/MIME) 4.0 Message Specification
[2] GNU Privacy Guard Tutorial (GnuPG)If you’re keen on sending confidential documents via email, the best method to ensure that your sensitive information remains private is to use secure email providers. In light of emerging cyber threats, many email providers have upped their privacy and security measures to protect user data.
Why Use Secure Email Providers?
Email is often an easy target for hackers due to its inherent vulnerabilities. Sending a confidential document via a regular email provider isn’t much different from sending a postcard – easily intercepted and readable by anyone who gets their hands on it. On the other hand, secure email providers use encryption which effectively scrambles the message, making it unreadable by unauthorized parties.
There are several reputable and efficient secure email providers in the market today:
Each service boasts powerful security features, including end-to-end encryption (meaning only you and your recipient can read emails), two-factor authentication, and zero-knowledge architecture (your data isn’t stored by these services).
How To Send Confidential Documents Via Email
Complementing the use of secure email providers with the right practices further enhances the safety of your confidential documents. Let’s walk through one way to send these files:
- Create your document and save as a PDF – this format is universally accepted and more secure than.docx files.
- Protect your PDF document by adding a password.
You can add a password to your document using most PDF editors:
xxxxxxxxxx
// Example how to set a password using Adobe Acrobat Pro
1. Open your PDF document in Adobe Acrobat Pro
2. Click on File > Properties
3. Click on the Security tab
4. Choose "Password Security" in Security Method
5. Check "Require a password to open the document", enter your desired password and confirm it
6. Click OK, OK again and then save your document
With your document now encrypted, you may proceed to send it via your chosen secure email provider. However, you should pass on the decryption key (password) to your recipient through other secure channels such as over the phone or face-to-face meeting.
These measures will ensure your confidential documents don’t fall into the wrong hands, preserving the integrity of your data and your professional relationships. A combination of using secure email services along with best practices in handling and transferring confidential information goes a long way in securing your communication.When it comes to sending confidential documents via email, there are certainly best practices to adhere to, while some actions should be completely avoided. This practice is crucial to ensure the confidentiality and security of sensitive information.
Firstly, let’s talk about what you should do when sending confidential documents via email:
• Encrypt Your Document: Encryption can be likened to transforming your document into a secret code. Only persons with the right decryption key, usually in the form of a password, will be able to decipher and access the actual contents of the document. In Microsoft office, for instance, this can be achieved by accessing
xxxxxxxxxx
File > Protect Document > Encrypt with Password
• Send Documents Via Secure Email Providers: Reputable email providers have sophisticated security algorithms to protect information in transit over their networks. Gmail, for instance, has an option called Confidential mode that allows you to set an expiration date for messages or revoke access at any time source.
• Confirm Recipient’s Email: Always verify that you have the correct recipient email address before hitting send. A single digit or letter mistake could send your confidential document to the wrong person.
Conversely, here’s what you should not do when sending confidential documents through email:
• Don’t Send Without Prior Permission: Ensure the recipient is expecting the document. Sending sensitive documents without prior warning could lead to them being overlooked or opened in insecure environments.
• Don’t Neglect Two-Factor Authentication (2FA): 2FA adds an extra layer of security by requiring at least two types of identification before you can access your email account. Not using 2FA makes you more susceptible to hacking threats.
• Avoid Using Public Wifi: Public networks often lack proper security measures, making them popular targets for hackers. As such, refrain from sending confidential emails when connected to public wifi.
In summary, ensuring the safety of sending sensitive documents via email revolves around authenticated encryption, secure email service providers, verification of recipient details, and obtaining appropriate permissions for data transmission. Simultaneously, avoid potential security traps, including circumventing 2FA, using public networks for email transmissions, and sending sensitive content without prior acknowledgement from its intended receiver.
These deliver best practices on how to transmit confidential files safely through email, therefore maximizing their security and confidentiality throughout the entire process.The best way to send confidential documents via email is by password-protecting them. This level of security mitigates various risks such as data breaches, hacks, and unauthorized access related to sensitive information.
Why Password-Protected Documents?
Password protection is one layer of defense strategy which adds an additional barrier between your confidential document and cybercriminals. Essentially, if someone aside from the intended recipient attempts to open the document, they’ll be required to input the correct password.
This process involves necessary encryption. When you set a password, the data in the file becomes encrypted, meaning it’s transformed into an unreadable format that only can be decrypted (made readable) again by entering the correct password.
xxxxxxxxxx
File -> Info -> Protect Document -> Encrypt with Password
Enter your chosen password twice for confirmation, and then click ‘OK’. Now, whenever you or anyone else tries to open the document, they’ll need to enter this password.
Best Practice Sending Confidential Documents Via Email
When thinking about how to send these confidential, password-protected documents via email, always remember:
- Never include the password in the same email as the document. If possible, share the password through a different communication channel entirely.
- Avoid sending highly confidential information during high-risk periods, like when using public Wi-Fi networks.
- Ensure the person receiving the document knows how to handle it securely on their end. Educating them on the importance of not sharing the password, deleting the document once done, etc., is crucial.
- For an added layer of security, you could consider sharing the document over an encrypted email service like ProtonMail (source) or Tutanota (source), where both the content and attachments of your emails are encrypted.
No system is invincible but exercising caution with regard to who has access to your password and when to send these types of documents can significantly increase protection. Having two-factor authentication for your email account also provides an extra layer of defense.
Conveying the Importance of Security
Finally, making others aware of the critical role that cybersecurity plays in our day-to-day digital interactions can help to foster an environment where data privacy is taken seriously. Encourage colleagues and counterparts to practice the same measures and to stay informed on emerging cybersecurity issues.
Overall, password-protecting confidential documents and choosing secure methods of delivery ensure that you’re upholding your part in safeguarding any sensitive information shared via email. It’s a best practice everyone should consider adopting. For more information on securing your files, take a look at this online guide on ‘How To Password Protect a MS Word Document’ (source).Digital signatures play a fundamental role in the secure exchange of documents. They provide authenticity, integrity and non-repudiation in electronic communications and transactions. A document signed digitally assures the recipient that it has not been tampered with during transmission and that it indeed originates from a verified sender.
xxxxxxxxxx
# Example of how to apply digital signature in Python
from cryptography.hazmat.primitives import hashes
from cryptography.hazmat.primitives.asymmetric import padding
signature = private_key.sign(
data_to_be_signed,
padding.PSS(mgf=padding.MGF1(hashes.SHA256()),
salt_length=padding.PSS.MAX_LENGTH),
hashes.SHA256()
)
To send confidential documents securely via Email, it is best practice to use encryption in conjunction with digital signatures. Encryption transforms data into an unreadable form, only decipherable by whoever holds the decryption key. This defends against unauthorized access while the document traverses through the potentially insecure public network infrastructure.
Using encryption and digital signatures together contributes to both data privacy and verification. The signature validates the sender’s identity and the originality of the message; the encryption shields the document contents from unauthorized view. Both these measures are crucial when dealing with sensitive or confidential information.
xxxxxxxxxx
# Example of how to combine digital signature and encryption in Python
from cryptography.hazmat.primitives.ciphers import Cipher, algorithms, modes
from cryptography.hazmat.primitives import hashes, serialization, padding
from cryptography.hazmat.primitives.asymmetric import rsa
# Generate a new RSA private key and encrypt a document
private_key_sender = rsa.generate_private_key(public_exponent=65537, key_size=2048)
public_key_sender = private_key_sender.public_key()
padder = padding.OAEP(mgf=padding.MGF1(algorithm=hashes.SHA256()),algorithm=hashes.SHA256(),label=None)
encrypted = public_key_sender.encrypt(data_to_be_encrypted, padder)
# Apply the digital signature
signer = private_key_sender.signer(padding.PSS(mgf=padding.MGF1(hashes.SHA256()),salt_length=padding.PSS.MAX_LENGTH),hashes.SHA256())
signer.update(data_to_be_signed)
signature = signer.finalize()
Generating different keys (one for signing, one for encryption) will ensure if either of the two keys is compromised, the damages imposed will be limited. While encryption prevents unauthorized reading of your confidential document, signing validates the legitimacy of your encrypted email so recipients can trust the content they receive.
But how to disseminate this encrypted and signed email?
Adding another layer of security, Secure/Multipurpose Internet Mail Extensions (S/MIME) protocol ensures secure transmission of your encrypted and digitally-signed email over the internet. Moreover, many popular email clients such as Outlook, Apple Mail, Thunderbird, and others, already support S/MIME.
An alternative safeguarding solution would be using secure email providers dedicated to preserving confidentiality. Examples include ProtonMail and Tutanota, which offer end-to-end encryption by default—encrypting all your emails even before they leave your device.
Remember, out front tools that simplify secure email like Virtru can take care of encryption and digital signature hassle for you if you’d rather not get into complex coding.
To most effectively send confidential documents via email, employ precision tools designed for this purpose: combined Digital Signatures and Encryption.Email tracking technology holds great potential in the arena of confidential document transfer. Constraining our discussion to the most effective way to send such sensitive information via email, it’s important to understand how email tracking influences this process.
Email Tracking: A Crucial Player
xxxxxxxxxx
Email tracking
is an integral component of contemporary digital communication. It allows users and organizations to obtain valuable information such as whether an email has been opened, the number of times it’s been accessed, and even the geographical location at which it was viewed (source).
In the context of confidential document sharing, email tracking enhances transparency and establishes a foundation for ensuring the safe transit of delicate information. Put merely this form of tracking gives you real-time data about the message journey—right from the click of your ‘send’ button to the recipient’s ‘open’.
However, this doesn’t imply that we should start sending confidential documents by attaching them straight away to emails with some tracking enabled. Security and protection of delicate information during transmission require more robust measures.
Confidential Document Transfer: Best Practices
To ensure secure transfer of confidential documents over email, making use of encrypted email services is one way forward. Confidentiality, after all, must be saved from prying eyes, and encryption offers just that.
xxxxxxxxxx
End-to-end encryption
, specifically, ensures that the data (in this case, the confidential document) can only be deciphered using a unique key accessible solely by the sender and the intended recipient (source). Even if someone intercepts the message, the contents stay unreadable without the decryption key.
Additionally, two-factor authentication or 2FA adds another layer of security, demanding user verification before email access—a vital element noting the consequence of the theft of confidential information online.
Technology | Role in Safe Email Communication |
---|---|
Email Tracking | Offers real-time data about email’s lifecycle; whether an email has been opened, how many times, where was it seen. |
End-to-End Encryption | Makes content of an email readable only for sender and recipient; Provides protection from unwanted interceptions, ensuring confidentiality. |
Two-Factor Authentication | Secures access to email; Requires user verification before email login, preventing unauthorized intrusion. |
However, one should note that these powerful tools are as potent as their responsible usage. It is crucial to keep software updated, routinely change passwords, and educate users about phishing schemes (source)— embracing secure email practices for the safe transfer of confidential documents.
So to recap, while email tracking empowers users with information about the life-cycle of an email, neither does it offer document confidentiality nor protect against access from malevolent agents. The fusion of end-to-end encryption—with additional security layers like two-factor authentication alongside responsible behaviors—is the ideal framework for transferring confidential documents via email safely.For email users, privacy is an essential aspect to consider – especially when dealing with sensitive or confidential documents. One valuable but somewhat overlooked tool for preserving privacy is utilising the BCC (Blind Carbon Copy) field in emails. The BCC field is a hidden component which prevents recipients listed here from being visible to others. This functionality makes it an excellent tool for distributing information while ensuring other recipients remain unaware of who else has received the message.
So, how can this feature help protect the confidentiality of your documents?
At first glance, sending a document as an attachment via email appears to be the simplest way, but there are inherent risks involved, such as accidental exposure of your client’s or contact’s email addresses to everyone on the email thread if not using BCC. By including these contacts within the BCC field, you ensure that only the sender knows who else has received the email attachments.
However, while the BCC field helps maintain a base level of privacy, it isn’t the most secure method when it comes to transmitting highly confidential data via email. Here’s where encryption becomes paramount. Encryption converts your readable text or files into encoded text that can only be decoded with the right key, hence adding a layer of security to protect your documents from unauthorised access.
Here’s how a simple email encryption process may look:
xxxxxxxxxx
// Encrypt the document
encryptedDoc = encrypt(doc, public_key)
// Send the encrypted document
sendEmail(encryptedDoc, receiver_email)
Moreover, services like Google’s Confidential Mode are useful tools. With Gmail’s confidential mode, the recipient gets a link to view the mail instead of getting the actual content. The original content and attachments remain in the sender’s mailbox, protected by Google’s security. Furthermore, the sender can put an expiration time or manually revoke the recipient’s access anytime.
In addition, secure file transfer services such as ShareFile1, WeTransfer2, or Dropbox3 are excellent resources for sending confidential documents. These platforms provide additional layers of protection like password-protected links and expiry dates on shared files.
Service | Features |
---|---|
ShareFile | Password-protected links, file access control, and tracking |
WeTransfer | Sends large files, password protection for ‘Plus’ accounts, file expiration policy |
Dropbox | File sharing with password protection and expiration, audit logs, |
To sum up, while BCC offers some degree of privacy, for better safety measures with confidential documents, combining the use of BCC with encrypted email services or file transfer platforms provides comprehensive protection. It would be best to understand each tool’s benefits and limitations, and use them appropriately according to the nature of the documents and their relative confidentiality levels.Sure. Ensuring the security of sensitive and confidential information is vitally important, especially when that information is sent via email. There are several powerful technologies designed to help make this process safer, such as Secure/Multipurpose Internet Mail Extensions (S/MIME) and Pretty Good Privacy (PGP) protocols.
Understanding S/MIME
xxxxxxxxxx
S/MIME stands for Secure/Multipurpose Internet Mail Extensions. It's a protocol that adds digital signatures and encryption to standard MIME data. S/MIME uses asymmetric cryptography, meaning it relies on pairs of keys – one public, one private – for encryption and decryption.
You can use S/MIME to encrypt your emails so only a specific recipient can read it. The public key of the recipient is necessary in order to achieve this. Consequently, anyone who intercepts or tries to open the email without this key won’t be able to understand the encrypted content.
Demystifying PGP
xxxxxxxxxx
Pretty Good Privacy (PGP), meanwhile, provides a slightly different function but with the same end goal of secure communication. PGP also uses a public and private keypair system to enable encryption and decryption. It protects email communication via cryptographic privacy and authentication methods.
PGP works by creating a unique hash, or digital fingerprint, of the email and then encrypting this hash using the sender’s private key. This forms what we call a “digital signature.” By doing so, the validity and integrity of the email can be confirmed by the recipient once the email is decrypted with the sender’s public key.
Choosing Best Method For Sending Confidential Documents
When it comes to securing your email communications, both S/MIME and PGP have their own set of strengths.
• S/MIME is typically integrated into larger systems and sits directly inside web-based clients like Outlook. This makes it incredibly straightforward to use on a day-to-day basis.
• PGP, on the other hand, allows you to manually verify signatures for given messages and gives a feeling of hands-on control over your email security.
Therefore, while either method could be suitable, choosing between S/MIME and PGP comes down to your specific needs and circumstances. It’s about evaluating the options at hand and selecting the one that best fits your requirements. Businesses might prefer the seamless integration provided by S/MIME while independent users might enjoy the fine-tuned control available through PGP.
In any case, the evolving nature of cybersecurity threats emphasizes the need for keen awareness and proactive measures when handling and sending confidential documents. Therefore, adopting an approach that uses technological aids like PGP or S/MIME becomes not just beneficial, but essential in our digital age.
References:
1. [S/MIME](https://docs.microsoft.com/en-us/windows/win32/seccertenroll/about-smime)
2. [PGP](https://www.openpgp.org/)While many might consider Fax Over Internet Protocol (FoIP) as a relic of the past, it’s actually still a trusted and secure method of sending sensitive data over the internet. When it comes to posing a question about the best way to send confidential documents via email, FoIP offers an answer that is both effective and reliable.
Here’s how FoIP works: Instead of sending a fax over a traditional voice line, FoIP allows businesses to send and receive faxes over the internet. This makes it an incredibly flexible solution, integrating with digital platforms like your email. Because all the communicated data is being sent over the internet and not on apart phone lines, it’s akin to sending an email.
xxxxxxxxxx
//Sample pseudo code for using a FoIP service
FAX_IPaddress = "XXX.XXX.XX.X"
DOCUMENT_Path = "/folder/my-confidential-document.pdf"
fax_service.Send(FAX_IPaddress, DOCUMENT_Path)
One of the benefits is saving cost. While the primary use of utilizing fax was to transmit documents over long distances, FoIP eliminates the need for expensive fax lines and international charges.
This technology doesn’t just offer cost and efficiency advantages, but security ones too, coming back to the point of sending confidential documents via email. Since a FoIP transmits data over the internet, the transmission can be encrypted. This is a higher security level than traditional faxing, where anyone who has access to the telephone line could potentially intercept the document.
Moreover, you don’t have to worry about the physical storage or loss of the document since everything is stored digitally, thus providing yet another level of security. Imagine an email box full of faxes, accessible anywhere and backed up into the cloud!
Just think of this table:
FoIP Advantages |
Cost-efficient |
Efficiently integrates with digital platform |
Encrypted transmission enables secure document sharing |
Digital storage avoids risks of physical storage loss |
In conclusion, FoIP provides a secure yet straightforward solution for sending critical and personal documentation through an easy system that users are familiar with – their email interface.
Many providers out there (such as [eFax](https://www.efax.com/), [RingCentral](https://www.ringcentral.com/), etc.) offer robust FoIP services that include features like high volume faxing, worldwide coverage, mobile faxing and more. They often include APIs that allow to code custom solutions and integrations with other systems. It’s simply a modern method built from a trustworthy, old tech.
When dealing with confidential data, however, the final verdict lands on the intersection of convenience and security. And FoIP definitely marries the two effectively and assertively, proving to be an ‘old but gold’ solution for sending confidential documents via email.Sharing confidential documents through email can often put the transmitted information at risk because traditional emailing systems are not designed to protect the privacy of the information contained within. However, there are ways to ensure your valuable data remains secure, and one pivotal method is using a Virtual Private Network (VPN).
A VPN connects your device to another network over the Internet while encrypting all data that you send or receive. It masks your IP address making it much harder for anyone trying to track your online activities. The encryption everyone is talking about here is an essential part of achieving this level of safety.
This encryption is quite helpful when you’re sending emails that contain sensitive information. Using a VPN creates a data tunnel between your local network and an exit node in another location. With these measures in place, while your data is in transit, it’s secure from prying eyes.
However, Please note that using a VPN isn’t whole solution for safe emailing. Here’s why:
- Not all VPNs Are Equal: There’s a vast difference in the quality and security standards among various VPN providers. Premium solutions offer optimal security features like top-level encryption, no-logs policies, DNS leak protection, and support for OpenVPN (the best VPN protocol). Make sure that the chosen VPN comes with industry-standard encryption algorithms – AES (Advanced Encryption Standard) with a 256-bit key.
- Email servers: Most email servers store messages after they’ve been sent. If these servers aren’t secured adequately, your secret information might remain exposed there unless deleted.
- The recipient’s devices: Even if you use a VPN, once the recipient reads the email on their device, your message is potentially exposed. If their device is compromised, your confidential information could be, too.
With these points in consideration, the best way to send confidential email would be to combine using a VPN with other data safeguarding methods such as:
- Email Encryption: For advanced protection, you can also opt for end-to-end email encryption. Tools like OpenPGP can be used to encrypt the content of your emails to keep them private.
- Password Protected Documents: If you’re sending a report or file as an attachment, consider using tools like Adobe Acrobat to password-protect your documents. This adds an extra layer of security while sharing files via email.
- Secure Email Providers: Consider using a trusted secure email service. These providers prioritize your privacy and will assist by offering encrypted email options.
Here’s a simple Python example how VPN connection can be established:
xxxxxxxxxx
# Import necessary modules
from getpass import getpass
from vpn_connect import init_connection
# Get VPN details from user
hostname = input('Enter VPN server: ')
username = input('Enter username: ')
password = getpass('Enter password: ')
# Establish VPN connection
connection = init_connection(hostname, username, password)
print('VPN connection successful.')
In conclusion, always use a reputable VPN alongside other security methods such as email encryption and secure email providers when dealing with confidential information via emails. These combined actions will create a strong fortress that keeps your sensitive data well protected and obscures your digital footsteps from malicious entities lurking online.When it comes to transferring confidential documents online, we often have several options at our disposal; key among them are cloud services and direct mailing. It’s paramount to take an in-depth look at these methods, contrasting their strengths, weaknesses, and how they operate in a secure way.
As starts with cloud services, such as Google Drive, Dropbox, or OneDrive. These platforms provide space on the internet where you can upload files and share them with specific individuals through generated links. It’s like having a remote hard disk readily accessible from any part of the world.
xxxxxxxxxx
//sample pseudo code for sending a document via Google Drive
UploadFileToGoogleDrive(file)
GetShareableLink(file)
SendLinkToRecipient(email, link)
Some of its strengths include:
- Can handle large files.
- Allows simultaneous accessibility and editing by multiple users.
- Keeps track of file versions.
Despite being heavily fortified against cyber threats, these platforms have vulnerabilities. The sharing links might fall into the wrong hands leading to unauthorized access. Also, storing sensitive information on third-party platforms brings about data sovereignty issues.
Switching gears to direct mailing – typically done through email platforms like Gmail, Outlook or Yahoo Mail. Here, the document is attached directly to the mail and sent to the recipient.
xxxxxxxxxx
//sample pseudo code for sending a document via email
ComposeEmail(recipient, subject, message)
AttachFileToEmail(file)
SendEmail()
Direct Mailing benefits include:
- Ease and speed of use.
- No need to rely on third-party storage platforms.
On the dark side, there are size limitations on the files that can be attached. Sensitive data may also get compromised if either the sender’s or recipient’s email account is hacked.
Considering these points, what then would be the best approach to sending confidential files over the Internet? While both have their pros and cons, the merits of using encrypted emails stand out when dealing with sensitive files. Encryption works by encoding information so only authorized parties can access it, ensuring data remains confidential during transmission. Online tools such as ProtonMail offer end-to-end encryption services, building a secure tunnel between sender and recipient.
xxxxxxxxxx
//sample pseudo code for sending an encrypted email
ComposeEmail(recipient, subject, message)
EncryptEmail()
AttachEncryptedFileToEmail(file)
SendEmail()
With this being said, the actual choice of method largely depends on the user’s exact needs, the sensitivity of the documents to be shared, and the level of trust established with the receiving party. Nonetheless, security should always be your North Star in selecting a digital file transfer method. Practice good cybersecurity hygiene regardless of the medium used. This could mean strong password practices, awareness of phishing attempts, and utilization of two-factor authentication.
For further reading on topics discussed:
How to share files from Google Drive?
What is end-to-end encryption?
Email security is crucial when you’re transferring confidential data through electronic channels. One might often wonder, does my antivirus program or firewall affect the safety of my emails? Let’s delve into this and unravel how firewalls and antivirus programs impact email security, touching briefly on the best practices for sending confidential documents via email.
Antivirus Programs and Email Security
Antivirus software enhances email security by scanning incoming and outgoing emails for malware, viruses, or suspicious links. Here’s how:
- Malware Protection: A diligent antivirus will constantly scan your email to inspect every attachment for malware. If it detects any malicious threats, it benightedly blocks them from accessing your files.
- Phishing Safeguard: Antivirus software can also identify phishing scams disguised in seemingly innocuous emails, preventing theft of personal information.
xxxxxxxxxx
Example:
if(file.scanForVirus()){
blockFileAccess();
}
Despite these benefits, some cons associated with antivirus programs include false positives and the potential slowing down of your system due to extensive virus scans.
Firewalls and Email Security
Firewalls act as your computer’s first line of defense against unauthorized external influences, including harmful emails. Here’s how they work:
- Regulating Data Flow: Firewalls control data flow between networks, blocking unauthorized access while ensuring necessary data reaches you in a secure manner.
- Filtering Content: They filter content based on set rules, rejecting potentially harmful or flagged content.
xxxxxxxxxx
Example:
if(!verifyNetworkAccess()){
blockDataFlow();
}
On the downside, firewalls may cause accessibility issues if not configured properly and could lead to legitimate emails being blocked due to stringent rules.
Sending Confidential Documents Via Email
In order to ensure that confidential documents reach their destination securely via email, one must consider the following steps:
- Encryption: Encrypting email contents will make sure only authorised recipients who have a decryption key can open it. Secure/Multipurpose Internet Mail Extensions (S/MIME) is a common protocol used for this purpose.
- Password Protect Files: Add another layer of security by password-protecting your confidential document and securely sharing the password with the recipient.
- Use Reliable Email Providers: Trusted email providers usually have strong built-in encryption methods and provide better protection to your content.
Going forward, we need to balance the pros and cons of using firewalls and antivirus programs for our email security. Both these tools can significantly enhance your protection online, but remember they are just a part of an entire security ecosystem. Always use end-to-end encryption, reliable email platforms, and additional security protocols to send confidential documents through email in a secure way.
From a coder’s perspective, using file compression techniques can help safeguard your sensitive documents while sending via email. Compressing files comes with dual benefits – it not only reduces the file size but also gives an added layer of security. To understand how you can leverage these techniques for confidential document safety during email transmission, we shall delve into three key aspects involving Compression, Encryption, and Email Transmission.
1. Compression
Compression is a useful technique for minimizing file size, leading to faster transfer rates while emailing. File compression tools such as 7-Zip and WinZip are commonly used.
Let’s see how you can compress a file using the 7-zip software.
xxxxxxxxxx
Right Click -> 7-Zip -> Add to archive...
While performing this step, you can choose various compression levels depending upon your requirements. More the level of compression, smaller the resultant file size, however, at the expense of more computation time.
2. Encryption
Encryption is the process of encoding information so that unauthorized users can’t access it. However, the authorized recipient can decrypt that information using the correct encryption key. Some compression tools like WinRAR and 7-Zip allow you to encrypt the compressed file with a password. You can use AES-256 encryption, which provides a high level of security. Here’s how you can add a password to your zip file using 7-Zip:
xxxxxxxxxx
Right Click -> 7-Zip -> Add to archive... -> Enter Password & Confirm -> OK
Remember, the strength of your encryption depends heavily on the quality of your password. Always ensure it’s strong enough by combining uppercase and lowercase letters, numbers, and special characters.
3. Email Transmission
Once your document is compressed and encrypted, you’re now ready to securely send it via email.
However, avoid sending the decryption key (password) in the same email as the attached encrypted file. Instead, communicate the password through another secure channel. It could be an encrypted text message, a separate email thread, or a trusted private messenger.
It’s worth noting that although encryption adds an excellent security measure to your files, it doesn’t replace the need for safe online practices. Always observe good cybersecurity habits — keep your computer updated, use anti-virus software and avoid downloading attachments from suspicious emails.
Extra Security: End-to-End Encrypted Email Providers
For an extra layer of security while transmitting confidential documents via email, consider using end-to-end encrypted email services like ProtonMail, Tutanota, or Hushmail. These services guarantee your content will stay unreadable until it reaches the intended recipient.
To recap, always compress and encrypt your confidential documents before sending them via email. And remember to communicate the decryption key through a separate secure channel to ensure maximum security. Remember to up your game in cybersecurity by being mindful of your digital environment and observing safe cybersecurity practices.
Not only does application of compression techniques optimise transmission speed and capacity, it also allows an effective approach towards maintaining the confidentiality of your documents when they are sent through email.
When sending confidential documents via email, security should be your top priority. If you’re thinking about third-party software solutions to enhance the privacy and protection of your mailed documents, there are several notable quality tools on the market that specialize in data encryption and secure file transfers. However, understanding that first, email is not 100% secure by nature might make you want to reconsider how confidential information is shared in your organization.
To aid discussions, I’ll break this down into selective methods you can use to add layers of security when dealing with sensitive documents:
– **Use Encrypted Email Services:** One way to go about it would be to use encrypted email services like ProtonMailand Tutanota. These services provide end-to-end encryption which ensures that only the sender and the recipient can read the content of the emails.
xxxxxxxxxx
/* Sample ProtonMail Usage */
var proton = require('protonmail')
proton.login('Your_Username', 'Your_Password')
proton.send({
to: 'Recipient_Email_Address',
subject: 'Subject Here',
body: 'Body Content Here'
})
– **Secure Document Delivery Services:** Another method is to use secure document delivery services such as DocSend or Citrix ShareFile. These tools offer features like read receipts, download restrictions, password protection, and other user access controls.
xxxxxxxxxx
/* Example usage of ShareFile API */
var sf = new ShareFileClient("https://secure.sf-api.com/sf/v3/");
var authRequest = new OAuth2PasswordGrantRequest
{
Username = "username",
Password = "password",
ClientId = "clientId",
ClientSecret = "clientSecret"
};
var oauthToken = sf.Authenticate(authRequest);
– **File Encrypting Before Sending:** Tools like GNU Privacy Guard (GPG) can encrypt files before they’re sent. An encrypted file can appear as a meaningless jumble of random characters until it’s properly decrypted, using the right decryption key. This means that even if intercepted, the files cannot be read.
xxxxxxxxxx
/* Encrypting files using GnuPG */
gpg -c confidential.txt
While these avenues offer increased security, take note that those are still susceptible to risks if used improperly. As an overarching principle, never forget the value of educating yourself and your teams on basic cybersecurity best practices.
Lastly, ensure to keep your third-party software solutions always updated. Doing so keeps your security mechanisms solid against newly discovered vulnerabilities which hackers might exploit.
For a thorough guide on achieving maximum email security, check this comprehensive resource from EFF (Electronic Frontier Foundation). Reading through it will impart a wealth of foundational knowledge and practical tips.Dealing with sensitive data in the form of confidential documents requires careful attention, particularly when it comes to transmitting these via email. Despite the presence of many mediums for speedy information transmission, email remains one of the most used methods due to its universal access and ease of use[source]. Striking a balance between speed of access and data security is crucial in maintaining the confidentiality of such document communications.
– Ensuring data protection starts before the sending process; this includes first protecting the contents of the documents by encrypting them. You can employ password protection or digital signatures to safeguard the integrity of your document. The very commonly used
xxxxxxxxxx
PDF
format for instance allows the usage of password based encryption.
–
xxxxxxxxxx
HTTPS
on mail servers is another vital element in document security. This protocol guarantees encrypted communication, ensuring that third parties can’t make out the content of your communication[source].
– Using a secure email service is an absolute necessity when handling confidential documents. Services like ProtonMail or Tutanota guarantee end-to-end encryption making sure that only the sender and receiver have access to the email’s content.
– Another strategy would be to avoid sending the document directly through e-mail. Instead, you could use services like Google Drive or Dropbox to store your document and then share the link securely via email. These platforms support robust document encryption and are equipped with extensive permission settings.
Here is a simple example of how to password protect a PDF using Adobe Acrobat:
xxxxxxxxxx
Step 1: Open the PDF in Acrobat.
Step 2: Click on the "Tools" pane, then "Protect", and "Encrypt".
Step 3: Select “Encrypt with Password”, then select your password settings according to your preference.
Note: Always remember to send the password separately to the recipient to maintain maximum document security.
To round up, dealing with secure digital communication can seem overwhelming, but with little careful vigilance and application of the right tools, you can successfully uphold a high standard of data security. It all boils down to understanding the processes involved and harnessing encryption technologies to your advantage. Communicate safely, everyone!