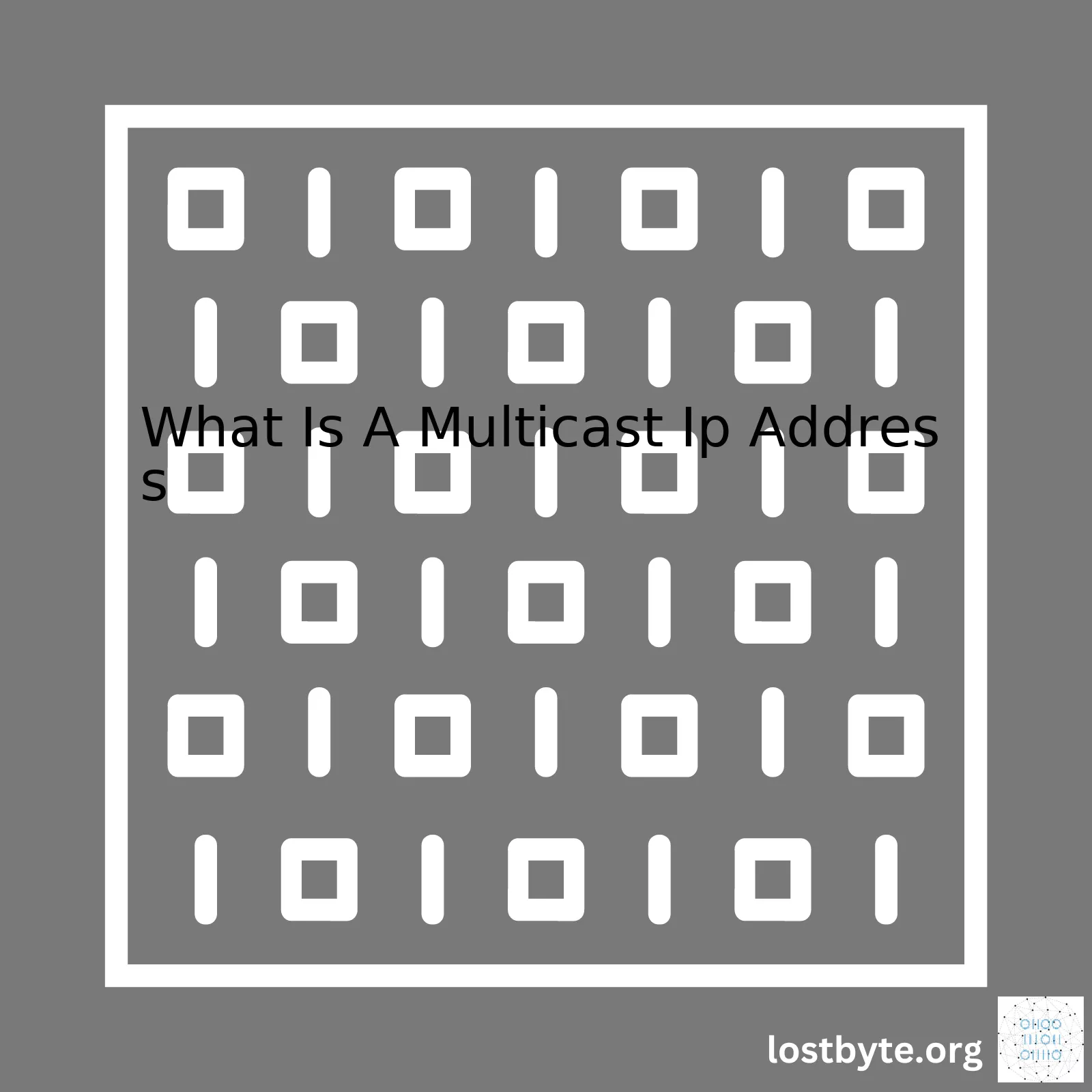
Term | Description |
---|---|
Multicast IP Address | An identifier for a group of hosts that have joined a specific multicast group and are capable of receiving certain transmissions. |
A Multicast IP Address distinguishes itself from unicast and broadcast addresses through its operational mechanism: it’s designed to deliver packets to multiple destinations, simultaneously. We could consider multicast as a kind of one-to-many communication where a source sends out data to an ensemble of destinations at once.
Now, let’s dig a little deeper. Devices (hosts) interested in a specific multicast transmission will ‘join’ a multicast group. These groups are identified by multicast IP addresses RFC 1112 . These addresses fall in the IPv4 range of 224.0.0.0 – 239.255.255.255, a reserved segment under the Class D IP range.
joiningParticularGroup() { // Initialize the multicast address for a group. InetAddress mcastaddr = InetAddress.getByName("230.0.0.1"); // Create a MulticastSocket MulticastSocket mcast = new MulticastSocket(2300); // Join the Group mcast.joinGroup(mcastaddr); }
The code snippet above collects how a host would join a particular multicast group using Java. Line by line, it defines the desired multicast group address, creates a MulticastSocket for joining the group, and finally solicits membership to the group with `mcast.joinGroup(mcastaddr);`.
This potential to simultaneously communicate data to many hosts allows network resources to be managed more efficiently while also ensuring robust data delivery mechanisms. This ability is commonly used in routing information protocol (RIP) computerworld RIP , Internet Group Management Protocol(IGMP) sciencedirect IGMP and many other system architectures.A Multicast IP address is a unique network address that directs packets to specific subsets of the network, notably a group of interested receivers, rather than all systems. This enables efficient data distribution and a network ban on unnecessary traffic, permitting high-bandwidth transmissions across the network with significantly lower network load. It exists between the concepts of unicast addresses, that refer to one sender and one receiver, and broadcast addresses, spanning one sender and all possible recipients.
In internet protocol version 4 (IPv4), multicast addresses range from
224.0.0.0
to
239.255.255.255
. Network devices that require communication with multiple nodes simultaneously employ these addresses. Prominent examples include audio and video streaming like Internet radio or IPTV, and other real-time communication broadcasts.
Schematic Representation:
Imagine a music band performing in a concert hall. Here’s how different types of IP addresses could be represented:
– Unicast: The band performs for a single person.
– Broadcast: The band plays for everyone in the stadium irrespective of their interest in music.
– Multicast: The band performs for those who are interested and have bought tickets to attend the concert.
Such an analogy helps paint a picture of how multicast IP addresses function in the IT realm.
How Multicast IP Works
The essential mechanism involved revolves around ‘listening’ and ‘joining a multicast group.’ Devices interested in receiving specific information ‘listen’ to a pre-specified multicast IP address. By ‘joining’ this group, the device indicates its interest in receiving the data sent to that address. Key things to note here include:
- The sender only transmits the information once, regardless of the number of listeners.
- The network infrastructure takes up the task of replicating and routing the packets to all members interested in that multicast address. It does not disturb others outside this scope.
- New members can join the group at any time, and members can leave whenever they want. The sender generally remains unaware of who joins or leaves.
Network protocols such as Internet Group Management Protocol (IGMP) manage the dynamic membership of a multicast group efficiently and robustly.
Below is a Python [source code](https://gist.github.com/ibeex/3257877) snippet illustrating a multicast. However, it’s crucial to remember that supporting multicast delivery largely depends on the networking hardware and software in use, and setting it up might be complex.
import socket MCAST_GRP = '224.1.1.1' MCAST_PORT = 5007 IS_ALL_GROUPS = True sock = socket.socket(socket.AF_INET, socket.SOCK_DGRAM, socket.IPPROTO_UDP) if IS_ALL_GROUPS: # on this port, receives ALL multicast groups sock.bind(('', MCAST_PORT)) else: # on this port, listen ONLY to MCAST_GRP sock.bind((MCAST_GRP, MCAST_PORT)) sock.setsockopt(socket.IPPROTO_IP, socket.IP_ADD_MEMBERSHIP, socket.inet_aton(MCAST_GRP) + socket.inet_aton('0.0.0.0')) while True: print(sock.recv(10240))
Key takeaways:
Please understand that Blackbox testing including multicast requests may involve several external variables – like routers, switches, and more which make this testing type difficult. Also, refer to your organizational policy before playing around this facet since it involves networking at a wider scale.
Here is the official Cisco guide to IGMP configuration.Undeniably, the concept of multicasting plays a critical role in networking, particularly when dealing with the distribution of data to multiple recipients efficiently. To fully understand this significance, we’ll delve into what multicasting is and its functionality, grounding our discussion on the central piece: the multicast IP address.
Multicasting, generally speaking, refers to the phenomenon of single-source communication intended for a group of destinations over a network. Instead of one-to-one (unicast) or one-to-all (broadcast) communication, multicasting allows for one-to-many or many-to-many interactions. It serves as a practical and effective method specifically when supplying the same data stream (packet/s) to numerous receivers.
One of the cornerstones of multicast networking is the RFC 1112 designated IP address range known as a multicast IP address.
// example of a multicast IP address 224.0.0.9
A multicast IP address essentially signifies a specific group of hosts bound by common interests or tasks within a network. Ranging from 224.0.0.0 through to 239.255.255.255, these addresses guide routers to deliver the message packets to all devices subscribe to the given multicast group.
It’s important to note, however, that not all 28-bit set are used in practice. As documented in RFC 5771, certain sections of the range are either reserved or allocated for different purposes, such as:
Address Range | Purpose |
---|---|
224.0.0.0 – 224.0.0.255 | Reserved for network protocols on a local network link. |
224.0.1.0 – 238.255.255.255 | Allocated for globally scoped addresses |
The use of multicast IP addresses can be quite beneficial in various contexts:
– Efficiency: By dispatching copies of messages only where there are interested receivers, it reduces unnecessary network traffic.
– Scalability: Multicasting alleviates the strain on the source node by delegating the replication tasks to the routers. A large set of receivers can therefore be served without impacting the sender’s performance.
Certain real-time applications, including IPTV or live-streamed events, greatly benefit from multicasting, ensuring that every participant can enjoy the service simultaneously and without delay.
Indeed, if we consider an unicast or broadcast alternative to these scenarios, the negative impacts on the network would be severe. Thus, the inclusion of multicast IP addresses in computer networking has been instrumental; it boosts efficiency, scalability, and overall user experience.A multicast IP address is an identifier for a group of hosts that have joined a specific multicast group. The hosts can belong anywhere within the network and receive the data addressed to that group’s IP address.
Differentiating between Unicast, Multicast, and Broadcast transmissions provides us insightful understanding to mechanisms applied by these systems. Let’s dissect each method:
* Unicast: With Unicast, data is sent from one sender to a single recipient. Therefore, it implies a one-to-one connection between the sender and receiver. This type of communication is primarily used over the Internet where information is sourced from one point (for instance, a client computer) and sent to another point (say, a server). Transmissions using a unicast system utilize TCP/IP protocols, had long been essential for accessing the web or email servers. In simplest terms, when you’re sending an email or browsing a website, you’re typically using unicast communication.
/* Imaginary representation of Unicast transmission */ sender.transmitTo(receiver, data_packet);
* Broadcast: In Contrast to Unicast, Broadcast involves sending a single data packet which is then received by all nodes in a network. This process does not target individual hosts but rather, it sends out information to everyone within reachable distance. It’s somewhat an “all hands on deck” approach in disseminating data where every node possesses a measure of the transmitted data. Although this technique can be useful in certain scenarios – like software updates, its indiscriminate nature could potentially lead to excessive network traffic.
/* Imaginary representation of Broadcast transmission */ sender.transmitToAll(network_nodes, data_packet);
* Multicast: A multicast IP address lays right between the two extremes of Unicast and Broadcast. Through this method, information can be sent to multiple designated recipients at once. But unlike Broadcast, data isn’t sent to all hosts – only those registered to a particular multicast group. Its selective sharing mechanism helps maintain a balance in data distribution, making it favorable for applications such as live streams or online gaming where similar data is to be shared among many users.
/* Imaginary representation of Multicast transmission */ sender.transmitToGroup(multicast_group, data_packet);
To summarize, the multicast IP address serves as a “middle ground,” providing a system that effectively broadcasts information without posing excessive strain on the network resources. By gaining a deeper understanding of how Unicast, Broadcast, and Multicast work, it’s easier to see their individual advantages and potential use cases within our networks.
Type | Description | Use Case |
---|---|---|
Unicast | Data sent from one sender to a single recipient. | Emails, Website Browsing |
Broadcast | Data packet received by all nodes in a network. | Software Updates |
Multicast | Information sent to multiple designated recipients at once, but not to all hosts. | Live Streams, Online Gaming |
It’s important to keep in mind that both Broadcast and Multicast require network infrastructure support to function correctly. Devices connected to the network must be configured to process or ignore multicast messages, and likewise, network equipment like routers must facilitate the broadcast / multicast protocol, or it cannot be utilized. Additionally, unintended misuse of both broadcasting and multicasting can often result in what is known as “broadcast storms” which create an extremely high amount of network traffic, potentially crippling network functionality. Therefore, even though they are strong tools, their usage requires careful consideration and planning.A multicast IP address, typically ranging from 224.0.0.0 to 239.255.255.255, is a crucial component inside the network layer of the internet protocol suite. Its primary use is in the delivery of broadcast content over an IP network. Essentially, a multicast IP address is a unique type of Internet Protocol address dedicated towards network segments, permitting for messages to be sent to multiple locations simultaneously instead of just a single host.
This incredible functionality offered by multicast IP hinges on a concept known as group communications. This method allows data traffic to be replicated on demand and then relayed to the group of destination computers concurrently, within that specific multicast group address.
When it comes to in-depth comprehension of how multicast IPs work, one can split the process into two distinct segments:
Group Concept: In this process, several hosts create a “group”. This could be anything from a couple of devices within the same local area network (LAN) to millions of hosts scattered across the world.
≶network≫192.0.2.0 0.0.1.255 area 0 ≶neighbor ip-address bfd≫
Data duplication: When using multicast IP addressing, the source VM or server generates a singular data packet for each piece of content it needs to transfer. It sends this single packet to the multicast IP address, leaving the responsibility of data duplication and distribution up to the communication equipment such as routers. Through this process, bandwidth can be saved – a part imperative for the seamless execution of high-bandwidth, real-time applications like IPTV.
≶debug ip packet≫ ≶show ip mroute≫
Given the versatility and efficiency offered by multicast IP addresses, they see common utilization in a wide range of applications, including video streaming services and even some protocols like Domain Name System (DNS).
However, use of multicast IP addressing also incurs complications such as requiring specific routing support and often being blocked by default by many company firewalls. Despite this, when applied in the right scenarios such as private streaming, multicast IP addressing offers a far more efficient solution than standard unicast addressing.A multicast IP address is a single address that refers not to one host, but to multiple hosts that opted to listen to traffic sent to this address. The use of such an address allows for efficient data distribution, as it permits the sending out of information to numerous recipients at the same time.
Deep diving into the structure, a multicast address is comprised of two main sections:
1. Multicast Group Field:
This is the part of the address that designates the multicast group. It consists of 28 bits and determines which group of hosts will receive the data packets distributed to this address.
Consider the example below where we are showcasing how prefix is set up in IPv4:
1110 28 Bit ↓ Multicast Prefix (FIXED) 11100000.00000001.00000011.00000111 ↑ Multicast Group Field
2. Fixed Prefix Field:
The fixed prefix field signifies that the address being used is a multicast address. In IPv4, the first four MSBs (Most Significant Bits) are set to 1110. This designation means any address ranging from 224.0.0.0 to 239.255.255.255 can be a multicast address.
In contrast, an IPV6 Multicast Address holds additional functionality; it comprises the following fields:
• Flags
• Scope
• Group ID
These enriched structure aids IPv6 in hosting node-specific multicast operations and a more flexible range of multicast groups.
IPv6 multicast addresses have the first eight bits set to ‘1111 1111’.
FF03::1 - Node-local scope all-nodes address FF02::1 - Link-local scope all-nodes address
In both IPv4 and IPv6, as portrayed by these examples, the address structure draws a clear line between unicast and multicast addresses. This distinction allows network devices to quickly differentiate and make appropriate action.
Achieving a deeper comprehension of multicast address structure unlocks the ability to effectively configure networking devices, enhance data distribution efficiencies, and optimize multicast operation capabilities.
For further reading, you could take a look on RFC 4291, Section 2.7 dedicated to “IP Version 6 Addressing Architecture”.Indeed, the fundamental technology that allows multicasting to happen is a Multicast IP address. Constructed specially to facilitate one-to-many communication, these addresses are defined in the Internet Protocol version 4 (IPv4) and version 6 (IPv6) standards.
The Internet Assigned Numbers Authority (IANA) has specifically reserved certain IP address ranges for multicasting:
– For IPv4: The range from 224.0.0.0 to 239.255.255.255
– For IPv6: The prefix ff00::/8 is reserved
Protocols Supporting Multicasting
For multicasting, several protocols have been designed and implemented to ensure efficient and accurate delivery of information. Some of the most important multicast protocols include:
IGMP (Internet Group Management Protocol) | IGMP is used by hosts and adjacent routers to establish multicast group memberships. It essentially acts as an intermediary between your device and the network’s multicast group. |
---|---|
PIM (Protocol Independent Multicast) | PIM is a group of multicast routing protocols that provide one-to-many and many-to-many distribution of data over the internet, irrespective of the underlying unicast routing protocol. |
MSDP (Multicast Source Discovery Protocol) | MSDP is used between routers to share information about active multicast sources. It ensures robustness in case of failure of any single path through source redundancy. |
All these protocols play varying but complementary roles in creating a fertile environment for multicasting to effectively take place.
Take, for instance, the IGMP protocol. Here is a simple illustration of how it works:
# IGMP Join Request Group_A.Member_1 ==IGMP_JOIN_REQ==> Router Router ==IGMP_JOIN_ACK==> Group_A.Member_1
In this snippet, you can observe a member machine (Member_1) sending a join request to be part of a multicast group (Group_A). The router acknowledges this request and accepts the machine into the group.
This example may be oversimplified, but it succinctly highlights how IGMP manages group memberships on a local network level. On broader levels, protocols like PIM and MSDP work to connect various multicast groups distributed across the internet.
The Role of Multicast IP Address
While the protocols mentioned above do their part in multicasting, none of it would make sense if not for the unique construct of a Multicast IP address. These special IP addresses enable routers to identify and correctly forward multicast traffic to all recipients who have expressed interest in it, forming the basis for one-to-many and many-to-many real-time communication over the internet.
As with any explanation of networking protocols and technologies, the details can get quite complex and intricate. However, I hope that this broad overview offers a starting point for understanding the crucial roles of Multicast IP addresses and supporting protocols in enabling multicasting over the internet. If interested, I urge you to delve further into each of these fascinating topics – you never know what nuggets of knowledge you might uncover!
Bear in mind that the proper functioning and efficiency of multicast routing are heavily reliant on tuning the aforementioned protocols to align with your network’s specific needs.A Multicast IP Address is essentially a group of destination addresses for network devices. Often utilized in multicast networking, these special IPs help in the efficient delivery of data packets across multiple interfaces at the same time. It makes it possible to distribute live video feeds or software updates simultaneously to many users without overtaxing the server or the network.
Typically, Multicast IP addresses lean on Internet Group Management Protocol (IGMP) as its underpinning technology and adopts the address range from 224.0.0.0 to 239.255.255.255 based on IANA (Internet Assigned Numbers Authority) classification1. Here’s noteworthy information how IGMP interconnects with Multicast IP Addresses:
IGMP working with Multicast IP Addresses:
IGMP acts as the key protocol that brings multicast communication to life. If we visualize the series of actions taken in multicasting, they are much like passing the baton in a relay race where IGMP plays an imperative role.
– First off, IGMP communicates to the local device (often, a router) about a host’s membership status in a multicast group. This means acknowledging which Multicast IP Address or ‘group’ it belongs to.
– Afterward, the request escalates up the chain; each device relays the message further until reaching the origin server. The server then broadcasts data to the given address and every member associated with this Multicast IP Address receives the package of data.
To illustrate it better let’s consider an example – when using IPTV (Internet Protocol Television) service, your tv box sends IGMP Join/Leave Group messages to your router, specifying the Multicast IP Addresses that refer to the channels you want to watch. Your router then instructs (using protocols like PIM or DVMRP) other routers about the new status, while the servers broadcasting those channels send the data packets directly to the multicast address specified, delivering the same data to all hosts subscribed to those addresses.
In terms of code, here’s a basic command in a Cisco Router adding a static route for a multicast group :
Router(config)# ip mroute 0.0.0.0 0.0.0.0 Serial4/0 225.1.2.3
In this scenario, ‘225.1.2.3’ would be the Multicast IP Address used for specific group communication.
Thereby, IGMP orchestrates the symphony of data being sent out on a Multicast IP Address to recipients part of the respective multicast group. Essentially, without IGMP, multicast communications and therefore Multicast IP Addresses would not work as seamlessly as they do.
Endeavoring to learn more about TCP/IP Fundamentals? Explore comprehensive resources2. Seeking hands-on networking practice? Consider interactive platforms3.
As always, it’s important to remember that a proper understanding of different IP address types and the nuances of IGMP protocol can form bedrock skills for any network enthusiast or professional aiming to excel in the world of networking. By delving deep into the relationship between Multicast IP Addresses and IGMP, one can gain fascinating insights into networked communication models, thereby enhancing their problem-solving abilities within the field.A multicast IP address is a unique type of internet address that allows for the delivery of information to multiple points simultaneously instead of just one. Here’s how it’s relevant for several real-world applications:
A multicast IP address is essentially an efficient communication protocol used for transmitting information over a network. Instead of just sending data to one endpoint like unicast, or to all possible endpoints like broadcast, multicast allows for the dissemination of news to many endpoints at once.
The main appeal of a multicast IP address lies in its efficiency, which can be quite beneficial for a variety of real-world applications such as:
– Video Conferencing: A multicast IP address facilitates more reliable and expedited data transfer during video conferencing among large groups. For instance, if there’s a company-wide meeting with dozens or even hundreds of participants, the video and audio data could be routed to all members using multicast, rather than sending individual streams to each participant.
– IP Television (IPTV): IPTV providers use Multicast IP addresses to distribute content, offering a good user experience by saving bandwidth and reducing network congestion. Rather than sending separate streams of content to each viewer, multicast allows the content to be sent in a continuous stream, which users ‘tap into’ to view real-time broadcasts.
How it works: 1. An IPTV provider sends out a multicast stream containing TV Channel 1 2. Multiple subscribers can join this multicast stream and start watching - everyone sees the same. 3. If a second viewer tunes into the channel, no additional stream is needed; they simply join the existing multicast group.
– Software Distribution: Companies often need to roll out software updates or patches to multiple machines simultaneously. Using multicast technology, the network can send the update once, and have it received by all pertinent systems, concurrently.
Essentially, any application where the same data needs to be delivered to multiple recipients benefits from a multicast IP address due its reduction in bandwidth usage and server load.
While the effectiveness of multicast IP addressing is clear, it’s essential to note that all elements of a company’s infrastructure, including switches, routers, and firewalls, need to support multicast protocols for it to work correctly. Also, proper planning and management are required to prevent potential issues like packet duplication or network flooding.
If you’re interested in reading further on the topic, Cisco provides an excellent comprehensive resource on how multicast works.
Remember: whether it’s ensuring smooth, high-quality video conferencing, delivering IPTV, or distributing software updates, multicast IP addressing plays an inevitable role. Its ability to scale effectively makes it instrumental to the functioning of modern networks.In the context of internet operations, multicast IP addresses are a special type of network address used to transmit data, usually audio or video streaming, to multiple endpoints concurrently. Multicast is a logical union of one-to-many and many-to-many real-time communication over an IP infrastructure in a network.
While regular unicast IP addressing allows one-to-one communication between two specific points, multicast uses a single sender and can carry information to multiple, concurrent receivers, saving network resources as the data is only transmitted once no matter how many endpoints are receiving it.
Multicast IP addresses are class D Internet Protocol (IP) Addresses, which range from 224.0.0.0 to 239.255.255.255. These addresses are reserved for multicast groups, where common examples include the all-hosts group of 224.0.0.1, to which every network-enabled device automatically belongs when turned on.
Examples of Multicast IP: Internet Assigned Numbers Authority (IANA): 224.0.0.0 - 224.0.0.255 (Reserved for network protocols) 224.0.1.0 - 238.255.255.255 (Globally scoped addresses)
The primary challenge associated with Inter-AS (Autonomous System) MVPN (Multicast Virtual Private Network) implementation is using multicast IP addresses effectively among distinct autonomous systems. Due to the disposition of multicast IP addresses, managing them to perform resourcefully across different networks is a complex task.
Some of these difficulties encompass:
– Delivery efficiency: Achieving full multicasting potential requires that transmitted data packets follow an optimal path to all subscribers, minimizing replication of packets across identical network segments.
– State scalability: As the size of the multicast group grows, tracking states for each receiver can lead to enormous routing tables garnished with additional complexity for routers handling multicast traffic.
– Inter-AS multicast routing: It involves forwarding multicast traffic among various ASes, transforming it into a more complex situation than unicasting due to the need for inter-AS multicast distribution trees, identifying a multitude of possible paths for each source.
Mitigation strategies used to overcome these challenges often involve deploying specialized multicast routing protocols, like Protocol Independent Multicast (PIM) to manage the delivery paths, and Multicast Source Discovery Protocol (MSDP) to share information about multicast sources between routers in different ASes.
Therein resides the importance of understanding both the complexities involved in implementing Inter-AS MVPNs and the basic functionality of multicast IP addresses, as they provide foundations for developing efficient network solutions and strategies particularly in scenarios where simultaneous data delivery to multiple endpoints is required.
Hyperlink references:
“IP Multicast” https://tools.ietf.org/html/rfc1112
“Source-Specific Multicast for IP” https://tools.ietf.org/html/rfc4607A multicast IP address is a unique network technique that hands out data to multiple particular destinations at once, instead of just a single destination. It performs this task using the most efficient strategy among sending multiple individual data streams or broadcasting the content across an entire set of network nodes. In other words, it’s like addressing a group email rather than single ones – you send out one copy, and multiple people get access simultaneously.
These IP addresses fall in the range of 224.0.0.0 to 239.255.255.255. Routers use these “class D” addresses to identify multicast groups on a network. It’s a functional attribute widely used for streaming media, multi-player gaming, and video conferencing.
However, as with any IP-based technology, using multicast IP addresses brings about some security concerns, which we’ll review:
#### Unauthorized Access
Malicious actors could potentially gain unauthorized access because multicast traffic isn’t person-to-person but one-to-many. They could tune into certain multicast channels, intercepting secure data flowing through it without detection. This issue intensifies when unencrypted data transmits, making it even more vulnerable.
#### Distributed Denial-of-Service (DDoS) Attacks
Multicast technology may also make servers susceptible to DDoS attacks. A hacker can send packets to a multicast IP address, which then disseminates from the router to all devices connected to that designated IP address. This act floods the network causing service disruptions.
#### Traffic Control Limitations
Where conventional IP routing affords administrators control over routing paths, multicast doesn’t. Routing protocols like
Distance Vector Multicast Routing Protocol (DVMRP)
determine multicast pathways. Administrators likely have limited influence on the propagation, making traffic management slightly challenging.
To counter these challenges, there are best practices organizations can follow:
– Encrypt Sensitive Data: To keep unwanted eyes off sensitive information, encryption is crucial. For instance, Internet Group Management Protocol (IGMP) instances should utilize IGMP version 3 that inherently supports Source-Specific Multicast (SSM). This function offers increased control over the multicast source, thereby reducing unsanctioned access risk.
– Good Firewall Practices: Ensure firewall settings are stringent. Don’t allow traffic from any arbitrary source to infiltrate your networks.
– Effective Network Monitoring: Adequate monitoring systems must be in place to track unusual traffic spikes, specifically on multicast IP, as they may indicate the onset of a DDoS attack.
For further read about securing multicast networks, you can review the draft document by the Internet Engineering Task Force (IETF).
In a nutshell, <p> While multicast IP addresses present several benefits for network efficiency and bandwidth usage, understanding potential security risks associated with it is vital. Mitigating these risks requires adopting strong encryption, rigorous firewall settings, and proactive network monitoring. </p>
Exploring these points in depth underscores the importance of blended technology methodologies – leveraging the strengths of different technologies while taking precautions to mitigate their weaknesses.When dealing with multicast IP addresses, one might encounter a number of common troubleshooting issues. Overcoming these obstacles requires a solid understanding of what exactly a multicast IP address is and how it functions.
A multicast IP address is a unique network address that directs packets to a specific subset of network nodes, as opposed to a single node (unicast) or all nodes (broadcast). Notably, this subset of nodes can be located anywhere within the network, allowing for efficient information distribution across wide network segments.source
One primary issue often has to do with routers not properly forwarding the multicast traffic. This is because not every router is configured to handle multicast IP addresses straight out of the box.
Let’s explore some code snippet solution.
router#conf t Enter configuration commands, one per line. End with CNTL/Z. router(config)#access-list 50 permit any router(config)#interface Serial 0/0/0 router(config-if)#ip pim sparse-mode router(config-if)#ip multicast-group 239.1.1.1 router(config-if)#ip multicast ttl-threshold 16 router(config-if)#exit router(config)#end router#
The above lines enable multicast on an interface and sets some essential parameters, overcoming most basic router-level roadblocks.source
Even when routers are correctly configured, data loss can still be an issue due to high packet drop rates. This can often be resolved by optimizing the buffer sizes on your switches and routers.
Another frequent problem involves devices on the network not receiving multicast streams. Often, this is simply because the device isn’t set up to join a multicast group. Here’s how to instruct a device to join a group:
import socket import struct MULTICAST_GROUP_IPV4 = '224.1.1.1' sock = socket.socket(socket.AF_INET, socket.SOCK_DGRAM, socket.IPPROTO_UDP) sock.setsockopt(socket.SOL_SOCKET, socket.SO_REUSEADDR, 1) mreq = struct.pack("=4sl", socket.inet_aton(MULTICAST_GROUP_IPV4), socket.INADDR_ANY) sock.setsockopt(socket.IPPROTO_IP, socket.IP_ADD_MEMBERSHIP, mreq)
Lastly, always ensure firewalls and security groups are configured to allow inbound and outbound traffic on the multicast addresses you’re implementing.source
Problem | Solution |
---|---|
Routers not forwarding multicast traffic | Configure routers to support multicast IPs |
Data loss due to high packet drops | Optimize buffer sizes on switches/routers |
Devices not receiving multicast streams | Instruct devices to join multicast groups |
Firewall restrictions | Allow inbound/outbound traffic on multicast addresses |
Overall, proper configuration along with a prime understanding of the nature and functional mechanisms of multicast IP addresses should effectively help in overcoming most common troubleshooting scenarios.Multicast IP addresses form a crucial part of our internet structure, allowing for efficient data transmission to multiple destinations. They are an integral part of network communication as we use it today and can significantly enhance the efficiency of bandwidth usage for multi-recipient applications.
I look forward to diving deeper into this topic. Specifically, I’d like to discuss how these addresses operate: essentially, a ‘membership’ model. Here’s what I mean by that: A multicast IP address designates a group of devices on a network. However, not just any device can receive the data sent to a multicast address. Only those devices that have specifically requested to join the relevant multicast group will receive the information transmitted to that multicast IP address.
To dive even more into this topic, let’s take a moment to understand the role multicast IP addresses plays in streaming media. For example, if a streaming service were to leverage multicasting for their live streams, they would transmit a single stream of data (the show or movie being streamed), which then is received and processed by all the appropriate ‘members’ (people watching the stream), effectively reducing their bandwidth needs.
//A crude representation of streaming using Multicast public void startStreaming(Show show){ ... multicastIP.send(show.getStream()); }
This strategy of data delivery forms the backbone of efficient content delivery networks around the globe. It’s quite evident how important Multicast IP addresses are, and it’s clear that understanding them is crucial for anyone working within networking, software development, cybersecurity, and numerous other fields. I strongly recommend tech enthusiasts and professionals continue researching about such intricate yet vital concepts in networking technology, whether that means online self-study, enrolling in courses, or utilizing comprehensive resources like The Internet Engineering Task Force IETF publications.
Remember, in our connected world, knowledge is power – the more you understand about the processes that enable our digital lives, the better equipped you’ll be to innovate, troubleshoot, or secure them. Happy learning! Remember:
– Multicasting boosts efficiency via one-to-many data transmission.
– Devices must ‘join’ a multicast group to receive the data.
– Streaming services can utilize multicasting to reduce bandwidth load.
For further reading, feel free to check out these detailed articles on multicast IP addressing from Techopedia and Wikipedia.