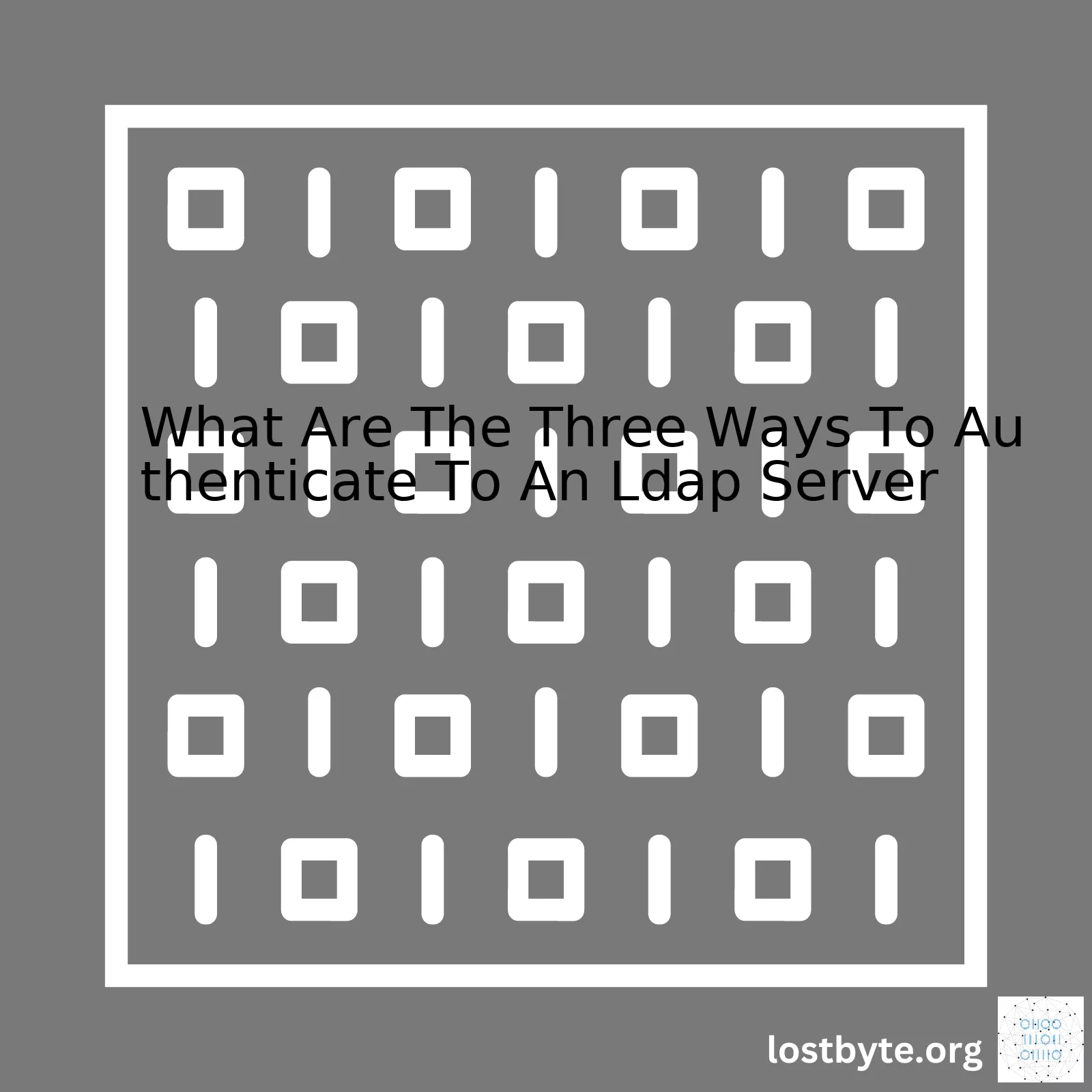
Method | Description |
---|---|
Anonymous Binding | This is a minimalist approach to LDAP authentication, where the user does not provide any credentials. It has limited access privileges and usually is restricted to the public portions of the directory tree. |
Simple Authentication | In simple authentication, the user provides a distinguished name (DN) and a password that is sent over the network in clear text format. It may pose security concerns and is advisable only over secure connections. |
SASL (Simple Authentication and Security Layer) | SASL facilitates authentication protocols in internet protocols. Unlike the above two, it supports data integrity and confidentiality control, making it more secured. |
The first among these is anonymous binding. This method does not require the user to provide any apparent credentials. However, this anonymity comes at the cost of accessibility – privileges are typically restricted to the LDAP Server’s public portions. While feasible for limited access or read-only scopes, it sits at the lower end of the security spectrum.
The second in line is simple authentication, which allows users to submit their credentials (usually the distinguished name and password) to the LDAP server. While seemingly direct and easy, the catch lies in how these details traverse across the network – they are inadvertently transmitted as plain-‘unobfuscated’ text. Such an absence of encryption opens up the potential risk of eavesdroppers snooping on sensitive data unless used over a secure connection (LDAPv3: Extension for Transport Layer Security).
Finally, we have ‘SASL’ (Simple Authentication and Security Layer). SASL is a solution that offers considerably more versatility and robustness than the other methods. Not limiting itself merely to the role of an enabler for various authentication protocols within internet standards, it extends its realms to encompass data integrity checks and confidentiality controls. The typical operation flow would look somewhat like the following code snippet:
// Establish a connection LdapContext ctx = new InitialLdapContext(env, null); // Use a SASL mechanism Control[] connCtls = new Control[]{new SaslControl("DIGEST-MD5")}; // Authenticate the user ctx.addToEnvironment(Context.SECURITY_AUTHENTICATION, "SASL"); ctx.reconnect(connCtls);
This capacity to mold according to internet protocol requirements coupled with its emphatic stress on security measures puts SASL a cut above the rest.In the realm of LDAP (Lightweight Directory Access Protocol), authentication to an LDAP server is of utmost importance for securing data integrity and privacy. Throughout this discussion, we’ll explore the three principal methods that are commonly used to authenticate to an LDAP server.
1. Simple Authentication
The easiest and most direct method of LDAP authentication is simple authentication. It uses a DN (Distinguished Name) and a password for the authentication process. The user presents these credentials, which the LDAP server then verifies against the entries stored in its internal directory.
Here’s a code snippet illustrating simple authentication:
import ldap l = ldap.initialize('ldap://my-ldap-server') l.simple_bind_s('cn=admin,dc=my-domain,dc=com', 'password')
Take note that the connection is not encrypted in this case, making it susceptible to eavesdropping attacks. As such, simple authentication is recommended only on trusted networks where secure transactions aren’t required, or in concert with Secure Sockets Layer (SSL) or Transport Layer Security (TLS) protocols for encryption.
2. SASL Authentication
The second method is the SASL (Simple Authentication and Security Layer) mechanism, a framework that decouples authentication mechanisms from application protocols. This offers more flexibility as it can support various authentication approaches such as Kerberos, Digest-MD5, and NTLM.
An example using SASL with DIGEST-MD5:
import ldap.sasl auth_tokens = ldap.sasl.digest_md5('user', 'password') l.sasl_interactive_bind_s("", auth_tokens)
SASL can incorporate encryption into the authentication protocol, leading to an advanced layer of security. However, the exact mechanics and precautions depend on the particular SASL mechanism being employed.
3. Certificate-based Authentication
Lastly, certificate-based authentication leverages digital certificates to affirm identities in LDAP server interactions. Rather than a traditional username-password pair, a client attempting to authenticate presents a digitally-signed certificate. The server cross-verify this certificate against a root certification authority (CA). If the CA confirms the certificate’s validity, the client gains access.
In Python, you can implement certificate-based authentication like so:
import ldap ldap.set_option(ldap.OPT_X_TLS_REQUIRE_CERT, ldap.OPT_X_TLS_DEMAND) ldap.set_option(ldap.OPT_X_TLS_CACERTDIR, '/etc/ssl/certs') l = ldap.initialize('ldaps://my-ldap-server') l.simple_bind_s()
Despite offering robust security measures, implementing certificate-based authentication requires diligent maintenance of the certifying authorities and the certificates themselves.
To delve deeper into each authentication method, I recommend referring to detailed resources, such as the LDAP.com guide on various authentication methods for further information. Likewise, Python-LDAP’s official library documentation is an awesome place to study about how these can be implemented in Python coding language.
In summary, while different projects require different solutions, understanding the capabilities and limitations of each authentication method allows us to make wise choices in creating secure and effective LDAP architectures.
The Lightweight Directory Access Protocol (LDAP) is a protocol used to access and manage directory information over an Internet Protocol (IP) network. One way it manages authentication is through Simple Binding.
Simple Bind in LDAP
Simple bind involves sending the user’s Distinguished Name (DN) and unencrypted password in clear text over the network.
In Simple bind, a client requests an LDAP session and then assumes the identity of the specified DN and provides a password. If the server can match the DN and password with entries in its database, it considers the client authenticated. This operation often happens over SSL or TLS for secure communication. The basic form looking something like this:
ldap_simple_bind_s(ld, "cn=root,dc=example,dc=com", "");
Where `ldap_simple_bind_s` is the function call for simple binding, `ld` is the LDAP connection handle, and `cn=root,dc=example,dc=com` is the distinguished name you’re trying to authenticate as.
However, Simple Bind in LDAP is just one method of authenticating; there are still two more methods to familiarize: SASL Bind and Anonymous Bind.
SASL Bind
SASL stands for Simple Authentication and Security Layer. It decouples authentication mechanisms from protocol, allowing any suitable mechanism to be plugged into SASL to support enterprise project needs.
An example using SASL with Digest-MD5 for authentication could look like such:
ldap_sasl_bind_s(ld, NULL, "DIGEST-MD5", cred, sctrls, cctrls, srvcredp );
Anonymous Bind
This method of authentication allows users to connect to the LDAP server anonymously. During an anonymous bind no password, or a blank password, is sent along with the Distinguished Name. This mode requires the server to allow anonymous connections, which may not always be enabled due to security concerns.
Sample usage may be:
ldap_simple_bind_s(ld, NULL, NULL);
Each of these methods have their own use cases and security considerations. Simple bind is uncomplicated but less safe unless combined with a secure layer like SSL. SASL offers better security through various mechanisms. Anonymous bind might be great for public user’s accessibility but has potentially serious data privacy implications.
By understanding each of these three methods of authenticating to an LDAP server — Simple bind, SASL bind, and Anonymous bind — you can determine the best option for your particular use case.
You can learn more about ldap authentication at ldap documentation site.LDAP, also known as Lightweight Directory Access Protocol, is primarily utilized among various platforms to perform directory services for finding and accessing information within an organized set of records. In terms of authentication, LDAP offers three distinct methods: Simple, SASL, and External. While Simple and External methods are straightforward, our focus here is the SASL (Simple Authentication and Security Layer).
SASL is a remarkable methodology for adding authentication support to connection-based protocols. It offers data security layer services like confidentiality and integrity for data exchange. To enhance network security, SASL integrates with LDAP.
// Connection to LDAP server using JNDI DirContext ctx = null; Hashtableenv = new Hashtable<>(); env.put(Context.INITIAL_CONTEXT_FACTORY, "com.sun.jndi.ldap.LdapCtxFactory"); env.put(Context.PROVIDER_URL, ldapurl); env.put(Context.SECURITY_AUTHENTICATION, "DIGEST-MD5"); // SASL mechanism env.put(Context.SECURITY_PRINCIPAL, username); env.put(Context.SECURITY_CREDENTIALS, password); try { ctx = new InitialDirContext(env); } catch (NamingException e) { // Handle any communication failures. }
In the above Java code snippet, we are connecting to an LDAP server using the initial context factory `com.sun.jndi.ldap.LdapCtxFactory`. The `SECURITY_AUTHENTICATION` property is set to `”DIGEST-MD5″`, which is a SASL mechanism for offering confidentiality.
Now let’s dig deeper into the elements of this process:
– Mechanism Negotiation: This is critical in SASL, where the client and server both negotiate on the most fitting SASL mechanism that they mutually support. For example, options might include anonymous, CRAM-MD5, DIGEST-MD5, GSSAPI, EXTERNAL, or PLAIN.
– Challenge-Response Communication: During the authentication, the client and the server exchange protocol-specific data, termed as ‘Challenges’ and ‘Responses’. This data is encapsulated inside SASL, hence remains transparent to SASL itself.
– Security Layer: Once authenticated, the client and server can optionally establish a security layer for subsequent data exchanges.
The advantage of using SASL over SIMPLE method in LDAP authentication is the added data-layer security. However, it has its own challenges too:
– Complexity: Building out bidirectional SASL mechanisms may be complex since they necessitate creating security layers.
– Mechanism Support: Not all SASL mechanisms are supported by every LDAP client or server.
So to conclude, SASL is a flexible and robust protocol used for LDAP authentication that greatly enhances security. It might be complex to implement but benefits such as stronger encryption and data integrity checks do compensate the complexity. If you have further interest in exploring these concepts deeply, you can refer to `RFC 4422`, which in detail discusses about SASL or `OpenLDAP SASL guide` for hands-on LDAP implementation practices under SASL security layer.
Let’s dig a little deeper into LDAP server authentication – specifically, the DIGEST-MD5 method. But before we dive right in, it’s crucial to brush up on basic concepts. In essence, there are three primary ways of authenticating to an LDAP (Lightweight Directory Access Protocol) server:
* Simple authentication
* SASL (Simple Authentication and Security Layer)
* Certificate-based or TLS/SSL negotiation
Simple Authentication
In this mode, a username (DN, Distinguished Name) and a password are sent over the network. While this approach is straightforward, it’s also fairly insecure as credentials can easily be intercepted.
SASL Authentication
SASL is essentially a framework for authentication and data security on the internet. It decouples authentication mechanisms from application protocols, allowing any suitable mechanism to be implemented on any application protocol. DIGEST-MD5 happens to be a SASL mechanism.
Akin to HTTP Digest, DIGEST-MD5 empowers us with an authentication option that does not require transmission of clear-text passwords over the network—an upgrade over simple authentication. Instead, it uses cryptographic hashes for verification.
Certificate-based or SSL/TLS Negotiation
Under this method, the client verifies the server’s identity through an SSL certificate. If validation is successful, an encrypted channel is set up to secure subsequent communication between the two parties. Connection is typically made via ldaps:// or STARTTLS, providing confidentiality and integrity—in contrast to simple authentication.
Now that we’ve established context around LDAP authentication mechanisms, we can explore the importance and relevance of DIGEST-MD5.
While DIGEST-MD5 might seem like something from an era gone by, you’d be surprised at how relevant it still is, given its weight in legacy systems. Furthermore, its ability to encrypt credentials rather than passing them in plain text gives it an evident edge over simple authentication. However, it’s worth keeping in mind that DIGEST-MD5 has been classified as ‘Historic’ by RFC 6331 as MD5’s hash weaknesses have sparked concerns.
That said, when dealing with modern systems, there’s an increasingly stronger case for certificate-based methods due to their superior security offerings. TLS, in particular, provides a robust encryption system coupled with certificate verification—creating a hardened defense layer against most common attack vectors.
In essence, the selection of a particular method often boils down to the unique demands and constraints of your specific use case, including factors such as your threat model, system environment, and compliance needs.
Even though it might seem that more modern methods are preferable—and they often are—it’s crucial to understand each specific method in order to make an educated decision. So even if DIGEST-MD5 gets phased out in favor of more secure options, its conceptual understanding remains useful for any LDAP practitioner.
So remember: don’t simply go with the flow; Go where your specific needs take you!Implementing a Kerberos protocol for secure LDAP (Lightweight Directory Access Protocol) access relates closely to the three ways of authenticating to an LDAP server. The three common methods of LDAP authentication are Anonymous, Simple, and SASL (Simple Authentication and Security Layer).
To enhance security, organizations often use the Kerberos protocol as part of SASL mechanism during LDAP authentication. To implement Kerberos protocol for secure LDAP access, you need to perform several steps which will be explained thoroughly in the following paragraphs.
The first step is installing the Kerberos software on each system that needs to authenticate with Kerberos much like other client-server systems. Below is a command line example of how to install the Kerberos client in a Unix-like environment:
sudo apt-get install krb5-user libpam-krb5 libpam-ccreds auth-client-config
The second step requires you to configure the krb5.conf file. It’s usually located in either
/etc/
or
/etc/krb5/
. In this step, you declare details about your realm, servers providing administrative services and Kerberos database services, among others.
For instance:
[libdefaults] default_realm = EXAMPLE.COM dns_lookup_kdc = true [realms] EXAMPLE.COM = { kdc = kerberos.example.com admin_server = kerberos.example.com }
The third step; you need to acquire a Kerberos ticket using the kinit command. A Kerberos ticket is a certificate issued by a Kerberos Key Distribution Center (KDC), confirming the user’s identity. To get this initial ticket, also known as Ticket Granting Ticket (TGT), the user must supply their username and password. Below is an example of how to request a TGT:
kinit USERNAME@REALM
After these steps, Kerberos for LDAP is set up.
Let’s dive into how this setup corresponds to the aforementioned LDAP authentication methods:
* Anonymous authentication: This is the simplest form, where no credentials are supplied to the LDAP server. Secure LDAP, implemented with Kerberos, generally doesn’t cater to anonymous authentication due to its lack of security.
* Simple authentication: In simple authentication, usernames and passwords are sent in clear text over the network unless protected by an encrypted connection such as SSL or TLS. Here, despite opting for simple authentication, integrating it with Kerberos can boost security because password-based verifications are replaced by token-based tickets, making unauthorized access more difficult.
* SASL (and specifically GSSAPI mechanism): SASL allows many different authentication mechanisms to be used. When LDAP and SASL are combined, we get a powerful pair that caters to a multitude of use-cases. One such case is when you employ Generic Security Services API (GSSAPI) as a SASL mechanism – enabling secure exchanging of messages on open networks, which perfectly aligns with our requirement: Kerberos protocol.
In summary, implementing Kerberos within LDAP doesn’t directly relate to Anonymous or Simple authentication, but it’s indispensable for GSSAPI-SASL method, strengthening security by validating users with tokens rather than passwords.
Do remember though, that dealing with a security protocol such as Kerberos calls for careful implementation. You have to keep every machine’s clock synchronized, monitor lifetime of respective tickets, and maintain stringent rules about the safety of your key distribution center.
Source informations were gathered from NixCraft and Kafka documentation.
When using the Lightweight Directory Access Protocol (LDAP) it’s essential to understand server verification, particularly with regards to the StartTLS process. Securing data from your LDAP client to your LDAP server is crucial in order to maintain confidentiality and integrity of transmitted information.
The Role of StartTLS in LDAP Server Verification
The role of StartTLS in the context of an LDAP session is vital as this technology steps up an unencrypted connection to an encrypted one, hence enhancing security during communication. The StartTLS technology operates over default LDAP port 389, preventing the requirement for a different port (usually 636) specifically for the secure connection like LDAPS. The key element of the StartTLS operation is server certification check which confirms the identity of the server to avoid possible Man-In-The-Middle attacks.
// Example pseudo code for startTLS process. Connection conn = LdapNetworkConnection("ldap://myldap.com", false); conn.startTLS();
After invoking the StartTLS command, the client encodes an extended LDAP request to initiate a TLS channel. When the server accepts the request, all further communications continue over a secure stream.
Three Ways to Authenticate to an LDAP Server
In regard to LDAP server authentication, there are three common methods:
- Anonymous LDAP Authentication: This form of authentication occurs when you connect to an LDAP server without supplying any credentials. It may not be appropriate for most production environments due to the lack of security.
- Simple LDAP Authentication: This method requires you to supply a username (or DN) and password. The username and password are sent over the network in clear text which could expose sensitive information to eavesdroppers if not properly secured with Start TLS or SSL.
- SASL LDAP Authentication: The Simple Authentication and Security Layer (SASL) method enhances LDAP’s security by supporting authentication mechanisms like Kerberos or Digest-MD5 for hash-based credential protection.
// Example pseudo code for simple LDAP authentication. Connection conn = LdapNetworkConnection("ldap://myldap.com", false); conn.bind("myUser", "myPassword");
It’s worth noting that while these are three acknowledged forms of LDAP server authentication, the optimal choice relies explicitly on your exact requirements and infrastructure capabilities. While anonymous binding might suffice for informal communication, it would be inappropriate for any circumstances requiring data protection or privacy. Conversely, SASL offers robust security but might implicate more intricate setup processes and higher operational costs.
For a deeper dive into LDAP and related security facets including StartTLS, feel free to refer to this detailed guide on Oracle’s official documentation.
The Lightweight Directory Access Protocol, commonly known as LDAP, is a protocol that helps in locating organizations, individuals, and other resources like files and devices in an internet or intranet network. Any references to LDAP authentication will mostly encounter X.509 certificates, which play an essential role in the authentication process of LDAP.
If your quest revolves around this concept, let’s spill some light on what X.509 certificates are and their connection to LDAP authentication.
outlines that X.509 is a standard defining the format of public-key certificates. These digital certificates ensure secure communication over networks through Public Key Infrastructure (PKI). As a part of many internet protocols, including TLS and SSL, X.509 certificates authenticate the identity of users, systems, and applications.
However, the scope of LDAP spans more than just dealing with X.509 certificates. It involves various ways of authentication, out of which three methods are predominantly common:
– Simple Authentication: Involving a straightforward username and password verification.
– SASL( Simple Authentication and Security Layer) : Providing a versatile method allowing you to plug in different kinds of authentication techniques.
– Certificate-based Authentication: Involves X.509 certificates for verifying identities.
Out of the three, the one involving certificates, especially the X.509 kinds, is gaining prominence and becoming increasingly significant in modern IT infrastructure. But why?
Let’s focus on how X.509 certificate-based authentication works in LDAP:
X.509 Certificate-based LDAP authentication uses a client-side certificate during the SSL/TLS handshake process to authenticate a user against the LDAP server. The sequence of events in this process can be summed up like this:
– The client initiates an SSL/TLS connection to the server
– Both parties exchange “hellos” and agree on a set of cryptographic algorithms to use for the session
– The server sends its X.509 certificate to the client
– If the client trusts the server’s certificate, it sends its own X.509 certificate to the server
– The server accepts the client’s certificate and authenticates the user based on the details embedded in the certificate
Benefits stack up when using X.509 certificate-based LDAP authentication compared to its counterparts:
– With certificate-based authentication, your LDAP server assures higher security levels against phishing attacks. That’s because attackers can’t easily steal a private key from a certificate compared to stealing a password. Unwanted access to sensitive data is, hence, largely curbed.
– Using X.509 certificates clears off one major concern i.e., maintaining transparency between Windows Active Directory and local system passwords. There isn’t any need to synchronize credentials, which makes management efforts minimal.
– Last but not least, integrating Single Sign-On (SSO) becomes less complex when using certificates for LDAP authentication, leading to simplified user experience without compromise on security.
Considering these gains, no wonder we see a growing shift toward X.509 certificates in LDAP authentication. Modern IT infrastructures are embracing more of this model hinged on protection and simplicity. Such a view enhances the overall security system bypassing possible malicious gateways of threat and risk. Plus, administrators can handle credentials more effectively, reducing overhead costs related to negligence and errors.
While Simple Authentication and SASL have their roles in LDAP, it is crystal clear that X.509 Certificates bear crucial significance in driving security and effectiveness in LDAP authentication.ategic output regarding current and future database environments, tuning database performance and ensuring security of an organization’s internal database systems.
Three primary modes of authentication exist when dealing with the LDAP server – Anonymous, Simple and SASL. Yet, no matter the chosen route, one striking commonality is crucial across all scenarios which is securing the connections. The solution comes by integrating Transport Layer Security (TLS) into your system. TLS acts as a protective layer offering encrypted communication amongst nodes in a network.
Authentication methods:
– Anonymous Binding: This method presents somewhat of an irony because it actually involves no authentication per se. By simply binding to the LDAP server without passing any credentials, users achieve anonymous binding. For example:
ldapbind -h localhost
However, this option poses serious security threats hence its deactivation being strongly advised.
– Simple Authentication: Involves explicitly passing DN (Distinguished Name) and password in plaintext to allow for authentication. An implementation would look like the below code example:
ldapbind -h localhost -D "cn=admin,dc=example,dc=com" -w secret
Here, the risk lies in the ‘plain text’ nature of the data transfer; this can easily be intercepted to reveal sensitive information. The proposed TLS strategy resolves this issue, which we will discuss shortly.
– SASL (Simple Authentication and Security Layer): This mode supports encryption mechanisms such as Digest-MD5, External, GSSAPI among others, thereby providing flexibility in terms of security approaches used. Here is a simple example:
ldapsearch -LLL -Y EXTERNAL -H ldapi:///
However, even with these secure SASL mechanisms, having the added layer of TLS protection maintains a robust security posture.
Why integrate TLS?
A key challenge in networked environments involves guaranteeing safe transmission of sensitive information. In the case of LDAP servers and particularly ‘simple authentication’, the username and password exchanges take place in plaintext. Any malicious entities eavesdropping on the connection can easily intercept this.
This is where Transport Layer Security enters the scene, akin to a superhero flying in with its red cape billowing to save the day. TLS objective lies primarily in preventing such snooping possibilities during communication sessions initiated between systems. It provides the necessary privacy and data integrity between two communicating computer applications.
How to Integrate TLS with an LDAP Server
Deploying TLS involves obtaining a valid TLS certificate from a trusted Certificate Authority (CA), installing it on your servers, and enabling TLS encryption on the desired ports. For instance:
slapd -h "ldaps:/// ldap:///"
The communications are now private and secure, shielding you from possible interception attacks.Learn More
To maximize server security, approach LDAP authentication with the mindset that, though different authentication methods present unique security benefits (SASL’s encryption for example), they aren’t infallible. Integrating Transport Layer Security offers a solid additional protective layer culminating in a robust, secure system against potential prying evil-doers lurking within the enormous expanse of cyberspace.
Authentication to an LDAP (Lightweight Directory Access Protocol) server is possible through three primary methods: Simple Authentication, SASL (Simple Authentication and Security Layer), and External SASL Mechanism.
Interestingly, the External SASL mechanism plays an important role in the LDAP authentication process, especially when security is a key factor, as it leverages digital certificates for both parties involved in the exchange, preventing exposure of sensitive data during transitions.
Authenticate to an LDAP server using Simple Authentication
In Simple Authentication, the client sends a DN (Distinguished Name) and plain text password to the LDAP server. The server checks these credentials against its entries in the directory, if the credentials match then the client is authenticated. However, this method can be vulnerable to a variety of common attacks, including Man-in-the-Middle and Eavesdropping.
Here is a simple sample of how to use simple authentication with ldap:
import ldap con = ldap.initialize('ldap://ldap.example.com') con.simple_bind_s('cn=admin,dc=example,dc=com', 'password')
Authenticate to an LDAP server using SASL
SASL is a more secure method of authenticating, where the client doesn’t provide any user credentials until a secured connection is established. This layer encrypts the communication between the client and server using techniques such as DIGEST_MDS, GSSAPI, etc.
For instance, see this Python code which demonstrates binding using the SASL DIGEST-MD5 mechanism:
import ldap.sasl auth = ldap.sasl.digest_md5("jdoe", "secret") con = ldap.initialize('ldap://ldap.example.com') con.sasl_interactive_bind_s("", auth)
Authenticate to an LDAP server using External SASL Mechanism
External SASL Mechanism involves a negotiation process between the client and server to establish a secure connection without ever exchanging user credentials. In other words, the credentials are outside the realm of the LDAP protocol. This type of agreement enables an extremely secure environment, because it depends on making sure that the data exchanges are encrypted by SSL or TLS before prompting for the SASL mechanism.
Utilizing the Python `ldap` module, binding via the EXTERNAL mechanism would resemble the following code snippet:
import ldap con = ldap.initialize('ldaps://ldap.example.com') con.set_option(ldap.OPT_X_TLS_CERTFILE,'/path/to/clientcert.pem') con.set_option(ldap.OPT_X_TLS_KEYFILE,'/path/to/clientkey.pem') con.set_option(ldap.OPT_X_TLS_CACERTFILE,'/path/to/cacert.pem') con.set_option(ldap.OPT_X_TLS_REQUIRE_CERT, ldap.OPT_X_TLS_DEMAND) con.start_tls_s() # StartTLS r,dn,creds = con.sasl_external_bind_s()
To sum it up, while simply renegotiating the session or utilizing SASL mechanisms might be straightforward and simpler to implement, the External SASL Mechanism provides certain liberty in terms of establishing a high degree of trustworthiness between the client and server due to its utilization of digital certificates. Through this mechanism, you’re able to maintain a well-secured communication channel, ensuring that your sensitive data remains uncompromised during all phases of transmission between the client and the LDAP server.Authenticating to an LDAP (Lightweight Directory Access Protocol) server usually involves three primary methods which are Anonymous Binding, Simple Binding and SSL/TLS.
//Example of basic code used for Simple Binding: ldap_bind($ldap_connection, $bind_user_dn, $password)
Anonymous Binding:This is considered the lowest level of LDAP authentication. Here, you don’t require a username or a password to log in. It’s largely utilized for public access and isn’t recommended when dealing with sensitive information due to its minimal security.
Simple Binding: A step up from Anonymous Binding, this type of authentication involves entering a username and password before gaining access. While more secure than Anonymous Binding, it still leaves room for breaches as the credentials are transmitted over the network clear text. Therefore, it is paramount to use encryption methods alongside Simple Binding to increase security.
SSL/TLS: This is the most secure way to authenticate to an LDAP server. SSL (Secure Sockets Layer) / TLS (Transport Layer Security) uses an encoded connection to securely communicate with the LDAP server. With this method, the clear text transmission issue found in Simple Binding is effectively mitigated.
// SSL/TLS Binding example: ldap_start_tls($ldap_connection)
By utilizing these three authentication methods to an LDAP server, you can effectively manage permission levels and ensure that your server remains secure. The decision on which method to use mainly depends on your application’s complexity, sensitivity of the data being handled, and the desired level of security.
Choose wisely by considering all factors; high security might be essential, but it should never compromise functionality over efficacy of the processes involved.
For further reading, I recommend this article [Understanding SSL and TLS] which gives an excellent deep dive into the workings of SSL and TLS within network and internet communication.
Remember, the best tool at your disposal when it comes to LDAP server authentication is knowledge!