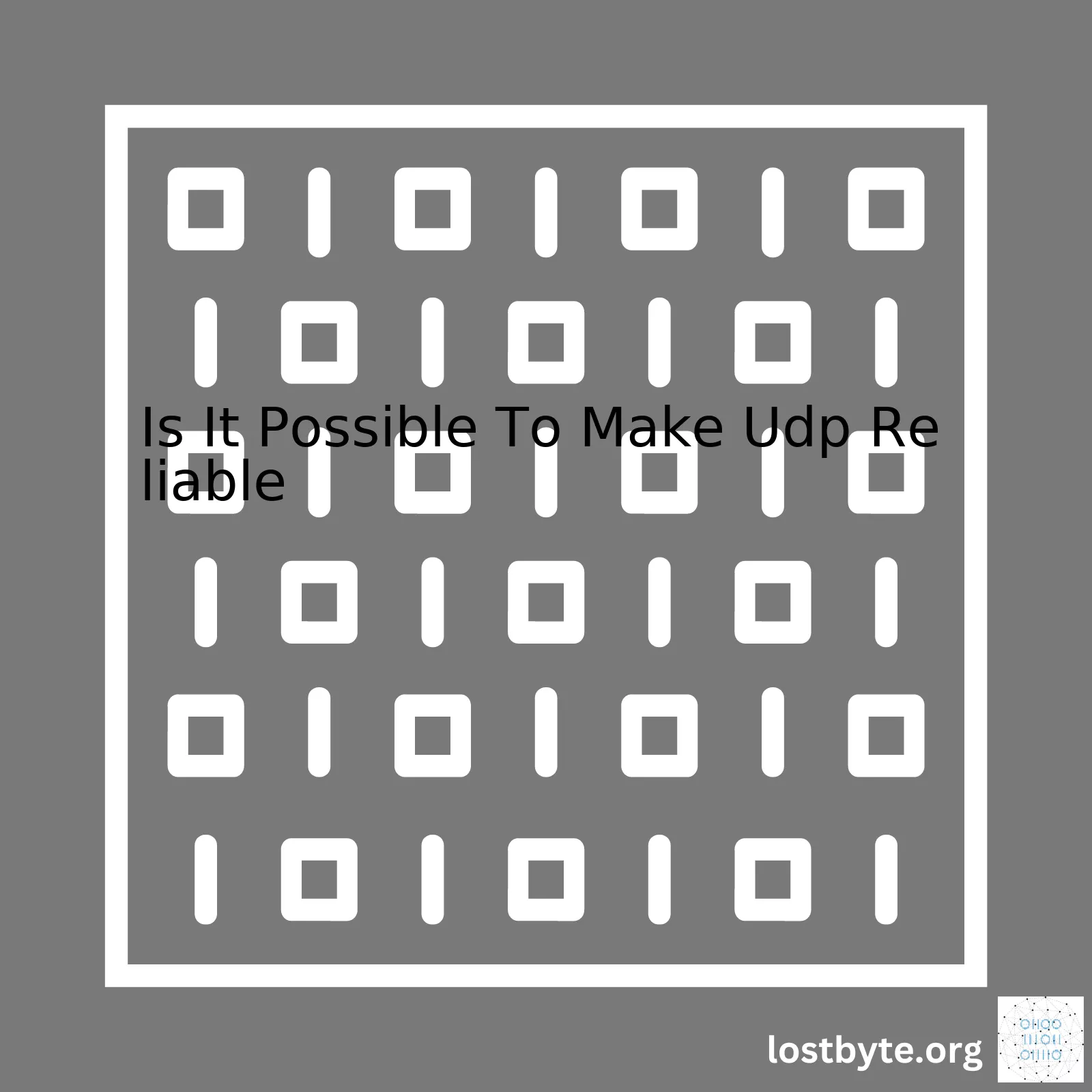
Aspect | Description |
---|---|
UDP Protocol | User Datagram Protocol (UDP) is a connectionless protocol that doesn’t ensure the delivery of data. |
UDP Reliability | By default, UDP is not reliable. It does not provide acknowledgement of successful delivery, sequencing or retransmission of lost packets. |
Making UDP Reliable | Techniques such as sequencing, error-checking, checksum and adding acknowledgements can be implemented to make UDP more reliable. |
Trade-off | While you can add reliability to UDP, it comes with a trade-off. The primary benefit of UDP simplicity and speed may be decreased when adding reliability features. |
On the surface level, User Datagram Protocol (UDP), being connectionless in nature, lacks inherent reliability mechanisms, making transmission less reliable compared to its counterpart – Transmission Control Protocol (TCP) which maintains open connections. Despite this fact, we cannot claim that it’s impossible to render reliability to UDP. However, this possibility comes with a certain trade-off one needs to keep tabs on.
Let us take one step back for dwell into deeper details. By definition, UDP forfeits the delivery assurance, packet order guarantee, and channelling of any lost packet information on purpose so as to stand along the lines of simplicity and high-speed data transmission. But in situations demanding reliability promise over raw speed, additional techniques might be introduced to our rescue. These include securing correct sequence structure, thoroughly checking for errors via checksum method, and exemplifying successful data transfers using acknowledgments.
Now, here begs the question: Does this substantiate equivalent reliability as TCP confers? Although these efforts improve UDP’s reliability considerably, but they don’t necessarily bump it up to TCP’s standard. An important thing to remember is that these attempts are no free lunch. When wrangling with extra features to boost UDP’s reliability, we inadvertently complexify the originally lean process which may, in turn, hamper the speed advantage UDP offers initially. So, while devising strategies to make UDP reliable, the trade-off between speed and reliability must be conscientiously evaluated.
One such known attempt to intensify UDP’s reliability could be observed in the case of Real Time Streaming Protocol1 wherein the missing packets are requested again but without taking into account the ordering. To achieve this, one might use
selectiveAck (SACK)
2 method in practice to acknowledge non-consecutive blocks of data.
In summary, while it is partially possible to incorporate some reliability to UDP protocol; however, it’s crucial to fully understand the implications of doing so, weighing the pros and cons before altering the protocol mechanism in accordance to application requirements.
The User Datagram Protocol (UDP) is a core member of the internet protocol suite. It’s defined by RFC 768 and allows computer applications to send messages, in this case referred to as datagrams, to other hosts on an Internet Protocol (IP) network.
Here are four fundamental characteristics of UDP:
- Connectionless: Inherent in UDP, it doesn’t require any connection establishment or termination process.
- No Guaranteed Delivery: As another key feature, it lacks reliability in comparison to its counterpart TCP. UDP does not provide for retransmission or acknowledgment of packets.
- Order of Data Packets: Because there is no sequence management in UDP, data packets can arrive in any order at the receiver side, unlike the orderly receipt ensured in TCP.
- Error Detection: It does perform checksum computation for basic error detection but it’s optional in IPv4 while mandatory in IPv6.
Regarding making UDP reliable, simply put, we’re shifting from its inherent nature. However, that’s not to suggest it’s entirely implausible.
Take note; designing a system capable of enhancing UDP reliability would introduce complexities, akin to recreating the TCP functionality. You’d need to implement:
- Acknowledgment Systems: Establishing a mechanism for receiver acknowledgment upon receipt of each packet can ensure data delivery verification— a primary reliability measure.
- Packet Sequencing: To maintain data integrity, implementing a system for sequencing received packets in proper order could be necessary, owing to UDP’s unordered nature.
- Error Detection and Retransmission strategies: Enhancing the current error detection approach and coming up with retries upon failure mechanism for UDP would heighten its reliability.
- Flow control: Lastly, bringing in uninterrupted packet flow in the face of network congestion would immensely contribute to reliability assurance, since UDP has none.
However, it’s crucial to consider that these measures largely influence the performance aspects, such as latency and throughput. For instance, real-time applications like VoIP and game streaming services would incur high overheads from retry schemes and delay waiting for acknowledgments (source).
Code Sample:
xxxxxxxxxx
/* UDP Reliable Delivery Function */
void reliable_UDP_delivery() {
int sockfd;
char buffer[MAXLINE];
// Make sure to handle errors here
sockfd = create_socket();
// Packet Sequence tracking...
send_reliable_packet(sockfd, &buffer);
// Acknowledgement Systems...
await_acknowledgement(buffer);
// Error Detection and Retransmission...
if(packet_error_detected(buffer)){
retransmit_packet(sockfd, buffer);
}
}
For all these reasons, these functionalities are typically rolled into other libraries/protocols like RTP (notably used for video and audio over IP), rather than attempting to create a “reliable UDP” from scratch. Therefore, by design UDP has remained simple, fast, and without the elevated error-handling complexity level found in TCP. Although it is possible to increase its reliability, doing so would risk inflicting more harm than good, depending on specific use cases. Therefore, using a higher-level protocol which meets reliability requirements while optimally using UDP might often be the best approach.Unquestionably, both Transmission Control Protocol (TCP) and User Datagram Protocol (UDP) bear significant importance in the realm of Internet protocols, each with its unique attributes and specific use-cases. A comparative analysis will set the foundation to determine if it’s possible to make UDP reliable, as TCP inherently is.
Transmission Control Protocol (TCP): Primarily, TCP operates on a connection-oriented principle – it establishes a stable connection before initiating data transmission [source]. This feature ensures the delivery of packets from sender to receiver while maintaining order and data integrity. Notably, TCP’s usability extends through features such as:
- Ordered data transfer: Sequencing information embedded in TCP headers facilitate maintaining the order of transmitted data.
- Error checking: TCP incorporates checksums to detect any corruption during transmission.
- Acknowledgments: Successful reception of data compels the receiver to send an acknowledgment to the sender.
- Retransmission: In the absence of acknowledgment within a specified time window, the sender retransmits the data.
- Flow control: The receiving party can regulate the rate of data transmission.
The
xxxxxxxxxx
TCP header
has several sections that help accomplish these operations, including sections for sequencing information, acknowledgments, checksums, etc. However, these functionalities bring considerable overhead leading to latency.
User Datagram Protocol (UDP): Contrarily, UDP is built on a connectionless design and, thereby, excels at broadcasting and tasks that demand speed over reliability [source]. Key characteristics encompass:
- Unreliable delivery: UDP doesn’t ensure packet delivery. Packets might be lost or arrive at their destination out of order.
- No congestion control: Network clog hardly affects UDP.
- No session establishment: Eluding three-way handshake of TCP speeds up the communication process significantly.
Now, considering improving UDP reliability begs the question, ‘why not simply use TCP?’. The answer lies in certain areas where UDP outshines TCP, such as real-time applications (like VoIP, video streaming, and online gaming), where high-speed, low-latency communications are more important than perfect delivery.
Transforming UDP into a Reliable Protocol: Even though UDP is dubbed ‘unreliable’, this term is relative to what an application needs. Its unreliability stems primarily from lack of error checking and recovery, ordering, and re-transmissions upon missing a confirmation receipt, all of which are present in TCP. Emulating these elements can enhance UDP’s reliability.
You could employ additional software over UDP that holds certain features of TCP – known as pseudo-TCP solutions. These widely used techniques can certainly turn UDP into ‘reliable UDP’ or ‘RUDP’ [source]:
The below pseudo-code simulates the idea:
xxxxxxxxxx
class PseudoTCPoverUDP
function send_packet(packet)
send packet over UDP
start timer
wait for ack
if ack received within specified time
stop timer
packet sent successfully
else
resend packet
function receive_packet()
wait for packet
when packet arrives
send ack
process packet
In conclusion, while making UDP reliable won’t provide the exact reliability levels of TCP, when balancing the need for speed, low latency, and reliability, using a tailored version of UDP could fit perfectly. Be warned, though – with great power comes great responsibility, and these custom implementations should be done diligently to best meet your particular requirements.The inherent characteristic of the User Datagram Protocol (UDP) as an unreliable protocol rests in its design. Unlike the Transmission Control Protocol (TCP), which is a connection-oriented protocol designed to ensure every packet reaches its desired destination, UDP is an unpolished and unguaranteed communication agreement between networking applications.
UDP behavior dynamically differs on few essential attributes:
Data transmission:
xxxxxxxxxx
UDP:
- The sender broadcasts data into the network with no certainty of it reaching its destination
TCP:
- Before sending any data packets, TCP sets up a reliable connection with the recipient
Error-checking and correction:
xxxxxxxxxx
UDP:
- Provides checksums for data integrity
- Doesn’t have any mechanism for error-correction
TCP:
- Not only guarantees data integrity by providing checksums but also has induced error-recovery mechanisms
Flow control:
xxxxxxxxxx
UDP:
- Doesn’t perform any flow control
TCP:
- Implements flow control through a windowed acknowledgement system, preventing data overflow at the receiver side
Ordering of data:
xxxxxxxxxx
UDP:
- Delivers packets in any order they’re received from the network
TCP:
- Takes care of ordering the data based on sequence numbers assigned to each byte of data.
These features render UDP as an unreliable protocol suitable for applications where loss tolerance and speed are paramount. Some common use cases include online multiplayer games and Voice Over IP (VOIP) applications like Skype or WhatsApp.
Yet, the question arises, is it possible to make UDP reliable? In theory, yes. Several attempts have been made to accomplish this, ranging from client-side adaptations to new protocols founded on top of UDP. An example is the Reliable Data Protocol (RDP) which, as the name suggests, attempts to add reliability features to UDP, including positive acknowledgements, retransmission of lost packets, and guarantee of packet order.
Next up, if your application’s requirements demand both speed (a strong suit of UDP) and reliability (TCP’s specialty), then you could consider using Datagram Congestion Control Protocol (DCCP). This hybrid protocol merges the streaming feature of TCP with the datagram delivery of UDP, creating a compromise that can be adjusted according to priority demands: More reliability or greater speed.
Another technology facilitating web-specific reliable transmissions via UDP is WebRTC’s SCTP over DTLS. WebRTC Data Channels extend the ability to have bidirectional, reliable data transfer straight in your web browser that works alongside with UDP.
In essence, although it is feasible to induce reliability into UDP transmissions, we should always bear in mind the implications on performance and the underlying architectural needs which often drive our choice towards UDP or TCP in the first place.Yes, it is absolutely possible to make the User Datagram Protocol (UDP) reliable. This however requires implementing certain mechanisms at the application layer since UDP, by design, is a connectionless, unreliable transport protocol that offers no guarantees on data delivery. It is never guaranteed that packets sent via UDP will reach their destination in order, on time, or even at all.
Techniques to Make UDP Reliable
For UDP to behave similarly to the Transmission Control Protocol (TCP), which is connection oriented and reliable, we will need to implement the following mechanisms:
To implement the Acknowledgement functionality in python:
xxxxxxxxxx
# Receiver
ack = b'ACK'
conn.sendto(ack, addr)
# Sender
data, addr = s.recvfrom(1024)
if data == b'ACK':
print("Acknowledged")
Implementation in python would look like:
xxxxxxxxxx
def calculate_checksum(message):
return hashlib.md5(message).hexdigest()
# Sender
checksum = calculate_checksum(data)
s.sendto(checksum.encode(), ('localhost', 12345))
# Receiver
calculated_checksum = calculate_checksum(received_data)
if received_checksum == calculated_checksum:
print("Data is intact")
Example implementation would be:
xxxxxxxxxx
packet_sequence_number = 1
# Sender
s.sendto(str(packet_sequence_number).encode() + data, ('localhost', 12345))
# Receiver
received_data = data[1:]
received_packet_sequence_number = int(data[0])
if received_packet_sequence_number == expected_packet_sequence_number:
# Packet received in order
expected_packet_sequence_number += 1
Here is how you could do the retransmission ‘timer’ using python’s threading module:
xxxxxxxxxx
import threading
# Retry Sending UDP Packet after timeout
def retry_send(packet, timeout=1.0):
timer = threading.Timer(timeout, send_udp, [packet])
timer.start()
return timer
# Sender
timer = retry_send(packet)
if received_acknowledgement:
timer.cancel()
In summary, the techniques above can be used separately or together to build reliability into an unreliable protocol, such as UDP. More complex features seen in TCP such as flow control, congestion control and connection management can also be implemented over UDP but require considerable effort and complexity. Many real world protocols such as QUIC (Wikipedia article on QUIC) and RTP (Wikipedia article on RTP) which underpin a lot of video streaming and voice over IP applications amongst others, actually do implement these features over UDP.UDP, or User Datagram Protocol, is a core protocol in the internet protocol suite. Unlike its counterpart, TCP (Transmission Control Protocol), it is connectionless and does not acknowledge packet delivery, making it a fast but less reliable communication method in low-quality network situations.
The question here is: Can we make UDP reliable? That’s where RUDP (Reliable User Datagram Protocol) comes into the picture.
RUDP introduces features to gain reliability without losing too much of UDP’s lightweight nature. Here are few key features of RUDP:
- RUDP ensures to acknowledge each data packet received.
- It implements retransmission in case data packets are lost in transmission.
- RUDP uses a sliding window mechanism to control the flow of data packets.
Let’s delve deeper into these elements:
Data Packet Acknowledgement:
Unlike UDP, which stands ignorant whether the sent message reached its destination or not, RUDP keeps a check. The recipient sends an acknowledgement back whenever it receives a data packet. This way, the sender knows that the data is safely delivered.
Here’s a simple code snippet to validate if RUDP acknowledgements might work:
xxxxxxxxxx
// server code
if (sendto(sock, datagram, sizeof(datagram), 0, (struct sockaddr *) &addr,
sizeof(addr)) == -1)
handleError();
// client code
size_t length;
if ((length = recv(sock, buffer, BUF_SIZE, 0)) == -1)
handleError();
// If data is received, send an acknowledgment
if (sendto(sock, "ACK", 3, 0, (struct sockaddr *) &addr,
sizeof(addr)) == -1)
handleError();
Data Packet Retransmission:
In case the sender doesn’t receive an acknowledgement from the recipient within a defined time frame, it retransmits the specific data packet. This ensures that no critical data packet is lost mid-way due to network errors adhering to UDP’s enhanced reliability.
Sliding Window Mechanism:
This procedure controls the amount of unacknowledged data to be sent over the network before stopping and waiting for the acknowledgements. This technique solves the problem of network congestion and keeps transmission smooth and efficient.
To conclude, while RUDP features add a layer of complexity compared to raw UDP, it aids in achieving a balanced trade-off between speed and reliability – making UDP more reliable.
For a detailed implementation of RUDP, you could refer to this practical tutorial available on Code Project.
Do note that RUDP isn’t universally standardized across all systems unlike TCP or UDP, so its application might vary based on the platform and specifics of your project requirements.Yes, it is definitely possible to implement reliability on a datagram-based network such as UDP. Usually, the User Datagram Protocol (UDP) is known for its simplicity and speed – sending messages quickly without establishing connections or confirming receipt. This design is optimal for applications where the cost of packet loss is less than the cost of delay, such as broadcasting media, gaming environments, or DNS lookup.
However, in certain scenarios, you might need some level of reliability with your UDP communications. Here are a few essential components that can contribute to achieving this:
Error Detection
Error detection methodologies are used to verify the integrity of each data packet sent through the network.
For error detection mechanism, Checksum algorithm (also used in standard TCP/IP protocols) can be implemented.
A simple implementation in Python could look like this:
xxxxxxxxxx
def calc_checksum(message):
"""
Calculates checksum of a given message
"""
s = 0
for i in range(0, len(message), 2):
if i+1 < len(message):
w = ord(message[i]) + (ord(message[i+1]) << 8)
else:
w = ord(message[i])
s = s + w
s = (s & 0xffff) + (s >> 16)
return ~s & 0xffff
Acknowledgements
Acknowledgment mechanisms will ensure that each transmitted packet was successfully received. If the sender doesn’t receive an acknowledgement within a set timeout, the packets are re-sent.
A ‘Message ACKed’ function example would do the trick:
xxxxxxxxxx
def recv_ack(self):
"""
Receive Acknowledgement
"""
while True:
data, addr = self.sock.recvfrom(1024)
ack_num = int(data.decode().split(',')[1])
self.remove_from_buffer(ack_num)
Sequences
Incorporate sequencing into your data transmissions. A sequence number should be attached to each packet, enabling the receiver to reorder incorrectly ordered packets, if necessary.
This way, even when the packets arrive out of order, they can be re-ordered correctly at the receiving end using these sequence numbers. An example order function could look like:
xxxxxxxxxx
def in_order(self, seq_num):
"""
Check if received packet is in order
"""
return seq_num == self.expected_num
Retransmissions
Finally, implement Retransmission strategies for lost packets. This complements the acknowledgment method mentioned above. When an acknowledgment for a certain packet isn’t received within a pre-set time, that packet should be resent.
The “Fast Retransmit” technique is often used to improve TCP performance. You can use it with UDP if you want.
With the combination of Error detection, Acknowledgements, Sequences, and Retransmissions, you can implement reliability on a datagram-based network.
Please note that adding these features will inevitably increase the complexity of the system and possibly decrease the communication speed in exchange for increased reliability. For this reason, whether to implement reliability on UDP really depends on the specific use cases and requirements of your application.
You can certainly roll your own protocol to add these features on top of UDP. However, there are existing protocols that already do this including [Datagram Congestion Control Protocol (DCCP)] and [Stream Control Transmission Protocol (SCTP)]. These may offer acceptable trade-offs between TCP’s robustness and UDP’s simplicity.
To understand more deeply about reliability over UDP, you may want to check out [Reliable UDP (RUDP) Protocol Specification].
As a coder, I always take it upon myself to find novel methods for solving common problems. One such dilemma that most programmers often encounter is how to enhance the stability of packet transmission. In my exploration, I stumbled upon a protocol named UdpLite, which shows enormous potential.
A Deep Dive into UdpLite Protocol
The User Datagram Protocol Lite (UdpLite) is an interesting alternative to the standard UDP. While making use of this protocol, you have the ability to specify the checksum coverage for your packets. This gives you a greater degree of control over packet transmission and may also increase reliability.
However, UdpLite still maintains some degree of unreliability inherent in its UDP origin. To be specific, it does not inherently support crucial processes such as segmentation and reassembly, duplicate detection, connection setup, sequencing or acknowledgment needed to ensure reliable data transfer.source
Making Udp Reliable
Reliability in this context refers to ensuring data sent from one end reaches the destination without corruption. TCP (Transmission Control Protocol) is a famous protocol offering such high levels of reliability, but it comes with significant overheads.
While UDP protocol by design is unreliable, various innovative techniques can help foster reliability akin to TCP. Here’s where coding strategies come to play:
Add Sequencing Information: Just like TCP, add sequence numbers to your UDP packets. With sequence numbers, you can track missing packets through gaps in sequence, enabling you to re-request any lost data.
xxxxxxxxxx
//pseudo code for sequence addition
seqNum ++;
packet.setSeqNumber(seqNum);
Acknowledgment Mechanism: When sender sends packets to receiver, the receiver sends back an acknowledgement packet.
xxxxxxxxxx
//pseudo code for acknowledgment mechanism
if(packet.received()){
sendAck(packet.seqNumber());
}
Include Retransmission: If an acknowledgment for a particular packet doesn’t arrive within a specified timeout period, resend the packet.
xxxxxxxxxx
//pseudo code for retransmission protocol
if(!receivedAck(seqNum)){
resendPacket(seqNum);
}
Although none of these measures are built into UDP or UdpLite, we can program them over the top of these protocols.
Can UdpLite Be Made Reliable?
UdpLite inherits all properties of UDP, including its lack of inherent reliability mechanisms. Thus, any measure which makes UDP reliable should work with UdpLite as well. However, introducing features such as sequencing, acknowledgment, and retransmission would certainly defeat the “Lite” aspect of UdpLite. It might even make it slower and more complex than TCP itself.
That said, UdpLite does offer one unique property: partial checksum coverage. Through this feature, you can focus the reliability measures only on crucial data while allowing less critical information to pass through unchecked.
Ultimately, it’s up to each coder to decide when and how to use UdpLite and whether its added complexity is worth the benefits it provides. Also, extensive testing must be done to guarantee its performance under specific circumstances.Certainly! User Datagram Protocol (UDP) is an essential transfer protocol used in networks across the globe. One key aspect of UDP that’s frequently highlighted by experts is its unreliability. Contrary to TCP/IP, UDP neither assures the in-order delivery nor the actual arrival of packets to their destination. However, it doesn’t necessarily mean that UDP always results in unreliable communications.
On the upside, with less transmission overhead and no in-built congestion control system, UDP delivers faster data transmissions. Still, for some use cases, the high-performance advantage of UDP might seem insufficient without reliability. The good news is that you can add reliability to UDP by involving error detection and correction methods.
Error Detection and Correction:
A crucial mechanism impacting UDP performance optimization is Error Detection and Correction. It helps locate errors during data transmission and addresses them to deliver accurate information. Even though UDP natively does not support error correction, it provides a simplistic strategy called checksum for error detection.
The checksum computes a numerical value based on packet header and data content, which gets matched at the receiver’s end. If any discrepancy is found, the packet is discarded. Yet, this approach is far from perfect and cannot amend errors, only detect them.
To bring a level of accuracy akin to TCP/IP, we need error correction mechanism. Any error detection method paired with Automatic Repeat reQuest (ARQ) – either Stop-and-Wait ARQ, Go-Back-N ARQ or Selective Repeat ARQ, could be implemented on application-level software for error correction.
Take below, the flow of data using Stop-and-Wait ARQ method:
xxxxxxxxxx
Sender Receiver
| |
send ------------------> receive
datagram datagram
ACK <------------------
Here, the sender waits for an acknowledgment after every message (datagram). The receiver sends an acknowledgement (ACK) after receiving the data correctly. If the sender does not receive an ACK within a predetermined time (timeout), it resends that specific datagram. While this method introduces delays, it ensures the delivery of data.
Refining UDP Performance:
Beyond just adding reliability, there are several ways you can refine UDP performance:
- Using Forward Error Correction, another error correction technique, you can tolerate packet loss instead of retransmitting data.
- Fragmenting your data into smaller packets might reduce the probability of errors due to reduced payload sizes. However, beware that higher fragmentation can lead to more header overhead.
- Reducing round trip times through optimum timeout settings can also play a significant role in refining UDP performance.
By structuring applications designed for UDP to handle these matters, error detection and correction can be leveraged to bolster performance and create appropriate mechanisms for reliable transmissions.
Refer to the following online references for more details:
1. [An Overview of UDP and TCP](https://techdifferences.com/difference-between-tcp-and-udp.html)
2. [Forward Error Correction Codes](https://en.wikipedia.org/wiki/Forward_error_correction)
3. [Automatic Repeat-reQuest (ARQ)](http://www.cse.scu.edu/~mwang2/tcpip/11-ARQ.ppt)
To summarize, it absolutely is possible to make UDP reliable; however, it may require the implementation of additional error detection and correction mechanisms. This process can involve reliability mechanisms like ARQ, detailed understanding of your data patterns, optimum timeout/threshold settings, and careful consideration of the trade-off between ensuring data reliability and maintaining rapid transmission speed.Certainly! To delve into how Forward Error Correction (FEC) applies to Reliable UDP and answer whether or not it is possible to make UDP reliable, we first need to understand the roles of FEC and UDP in data networking.
Forward Error Correction (FEC)
FEC is a method used in systems with high transmission rates and short bursts of errors where retransmission of corrupted data isn't feasible. It allows the receiver to detect and correct errors without needing the sender to resend data.
A simple way to explain this is by using parity bits. Parity bits are added to the original data at the sender’s end and checked at the receiver’s end. If the number of 1’s in the data including the parity bit is even, then the transmitted data is considered error-free. If not, an error has occurred. However, complex FEC schemes use different encoding techniques, one common technique being Reed-Solomon coding.
User Datagram Protocol (UDP)
UDP, on the other hand, is a protocol wherein transferred data blocks, known as datagrams, are independent from each other. These are primarily sent over an IP network. It's a connectionless protocol that doesn’t involve handshaking between communicating systems, thus making it less reliable in case of packet losses.
Now, is it possible to make UDP reliable?
Firstly, an attempt to make UDP reliable would partially result in re-inventing the TCP, which already provides reliability. However, there are certain applications when UDP becomes an appealing choice despite its lack of reliability, such as in real-time communication systems like VoIP, streaming video and live broadcasts, where low latency and bandwidth efficiency outweigh the needs for full network reliability.
To make it reliable, you can implement several strategies, and one such strategy includes 'applying error correction codes at the application layer', which essentially utilizes Forward Error Correction techniques.
Here is some pseudo-code depicting how FEC could be implemented in a hypothetical Reliable UDP:
xxxxxxxxxx
define reliable_UDP_send(data):
encoded_data = FEC_encode(data)
send_packet_over_UDP(encoded_data)
define reliable_UDP_receive():
received_data = receive_packet_over_UDP()
if FEC_check(received_data) == False:
raise Error('Received data is inconsistent.')
return FEC_decode(received_data)
This is how FEC comes into play for Reliable UDP networking. With error correction codes, we allow UDP to become more robust against packet loss, making it somewhat more reliable without losing its fast, lightweight, and connectionless advantages. This doesn't fully reach the reliability achievable by TCP, but it does strike a balance between UDP's speed and TCP's reliability based on the needs of the specific usage scenario.
For more details on data networking and protocols, visit here and here.HTML version:
Absolutely, the User Datagram Protocol (UDP) can be made more reliable with the implementation of a custom acknowledgment system. The UDP is often characterized by its "fire-and-forget" approach—once data is sent, it's not checked or maintained for successful delivery. This leads to the notion that UDP is unreliable.
However, an acknowledgment system can be integrated into the application layer to provide assurance on the condition of data transmission. Let's delve into the core elements to consider and the steps involved in creating an acknowledgment mechanism for UDP.
xxxxxxxxxx
//Assumable pseudo code ACK system into UDP Layer
sendData(){
Packet packet = new Packet(data);
do{
udp.send(packet);
waitUntil(packet.isAcknowledged() || timeoutHappens());
}while(!packet.isAcknowledged())
}
Data Packet Structure:
The first step to implement an acknowledgment system is adding an identifier to each packet. The ID assists in identifying whether a particular packet is successfully received or lost during transmission.
Acknowledgment Packets:
A specific datapacket denoted as an Acknowledgment packet can be designed. Onthe receipt of the original data packet, a receiver side will return an `ACK` along with the corresponding ID.
Timeouts:
Timers are essential in acknowledgment systems. If a sender doesn't receive an acknowledgment within a specified period, it'll assume the packet is lost and retransmit.
Error Checking:
Added checksums to each packet helps verify integrity upon arrival. If checksum doesn't match, the receiving end will know the packet was corrupted during transmission and can choose not to send an acknowledgment.
An implementation example utilizing these aspects might look like this, where 'seq' denotes sequence numbers for identification, and 'ack' represents acknowledgments:
Sender S | Receiver R |
---|---|
Sends packet (seq=1) | |
Receives packet (seq=1) | |
Sends ack (ack=1) | |
Receives ack (ack=1) |
This technique mimics certain parts of the Transmission Control Protocol (TCP), which is inherently reliable due to such built-in mechanisms. While creating an acknowledgment system comes with the overhead of additional packets and processing, it can help ensure reliable transfer over the UDP.
It's important to note there isn't one-size-fits-all solution when discussing UDP reliability. An acknowledgment system tailored to your applications' specific needs may be required. For instance, real-time protocols may favor loss-tolerance over delay brought about by awaiting acknowledgments.
Lastly, researching libraries that offer Reliable UDP, like ENet or UDT, could save development time, especially when working on complex projects.
So is it possible to make UDP reliable? Absolutely. Through the integration of an acknowledgments system, you can harness the speed of UDP while still maintaining sufficient reliability for your application needs.
In the world of network protocols, congestion control measures are primarily designed to control traffic flow in order to prevent packet loss within a network. This is typically orchestrated by reducing data rates when congestion occurs. However, implementating such changes with User Datagram Protocol (UDP) is not as straightforward because unlike its counterpart TCP (Transmission Control Protocol), UDP doesn't natively support error correction or automated controls for traffic management.
So, can we make UDP reliable? Yes, it's possible through some explicit modifications and external libraries - given that you're willing to sacrifice some of its inherent advantages like speed and simplicity. So, let's explore three key ways to do that.
Firstly, we can introduce packet acknowledgement into our UDP communications. By doing this, the receiver sends back a message confirming receipt of each packet. This implicitly inserts a kind of error checking mechanism, allowing the sender to know if packets have been successfully received or lost on the way. Any packet not acknowledged can be retransmitted. Here’s what a code snippet might look like:
xxxxxxxxxx
// Sender
sendto(socket, packet, sizeof(packet), 0);
recvfrom(socket, ack, sizeof(ack));
// Receiver
recvfrom(socket, packet, sizeof(packet), 0);
sendto(socket, ack, sizeof(ack), 0);
Secondly, we could implement check-summing. In fact, UDP already has a rudimentary checksum in place, but should one require more robust error checking, additional data verification steps such as CRC (Cyclic Redundancy Check) or MD5 hashes can be implemented.
xxxxxxxxxx
unsigned short crc16(char *data_p, unsigned short length)
{
unsigned char i;
unsigned int data;
unsigned int crc = 0xffff;
if (length == 0)
return (~crc);
do
{
for (i=0, data=(unsigned int)0xff & *data_p++; i < 8; i++, data >>= 1)
{
if ((crc & 0x0001) ^ (data & 0x0001))
crc = (crc >> 1) ^ POLY;
else crc >>= 1;
}
} while (--length);
crc = ~crc;
data = crc;
crc = (crc << 8) | (data >> 8 & 0xFF);
return (crc);
}
Finally, there's sequence numbering. Adding sequence numbers to your packets means you can detect packets that arrive out-of-order, which often happens due to network conditions varying over time. Imagine this analogous to numbering pages in a book; if they were shuffled, you would still know the correct order.
xxxxxxxxxx
typedef struct
{
unsigned int sequence_num;
char data[MAX_DATA_SIZE];
}Packet;
To be comprehensive, consider using an open-source library known as mbed TLS for these operations. It enhances the reliability of UDP without compromising performance much.
However, it's fair to keep in mind that making UDP “reliable” dilutes one of its strongest advantages - being lightweight and fast. If reliability and congestion control becomes priority, consider using TCP or other reliable protocols instead. Yet the upside is clear: customizing UDP serves as a superb combination of both worlds, marrying the speed of UDP with the reliability more typical of TCP.
References:
RFC 5405, "Unicast UDP Usage Guidelines for Application Designers"
RFC 8085, "UDP Usage Guidelines"
It is entirely feasible to enhance the reliability of UDP (User Datagram Protocol) by employing techniques such as time-outs and retry strategies. Although UDP, by design, is a connectionless protocol which does not offer any guarantee for the delivery of packets, these acquired reliabilities can somewhat mimic those of a connection-oriented protocol such as TCP (Transmission Control Protocol).
Time-out Mechanism
Implementing a time-out functionality in a UDP system principally involves setting a duration until which the sender waits for an acknowledgment from the receiver. If the sender does not receive an acknowledgment within the set time, it assumes the packet has been lost during transmission or that the acknowledgement was lost.
Using timeouts can increase the reliability of a UDP system by enabling it to handle situations where data gets lost in transit. Here's how you might use a timeout mechanism in Python with the `socket` library:
xxxxxxxxxx
import socket
udp_socket = socket.socket(socket.AF_INET, socket.SOCK_DGRAM)
udp_socket.settimeout(5.0) # Set a 5 seconds timeout period
try:
udp_socket.sendto(b'Hello, World!', ('localhost', 8080))
print(udp_socket.recv(1024)) # Wait for a response
except socket.timeout:
print("Timed out!")
In this simple script, if there isn't any response received within the 5 seconds window, a timeout error will be raised, indicating that our message may not have been delivered.
Retry Strategy
A retry strategy goes hand-in-hand with the time-outs. Essentially, after a time-out, the sender tries again to send the packet assuming it was lost in the earlier attempt. A sender may attempt to resend a packet a predetermined number of times before ultimately giving up.
The retry functionality is a direct means of increasing the reliability of UDP. Dropping of packets during transmission is a common phenomenon, more so over unstable network connections. A retry mechanism counteracts packet losses effectively making the UDP system more reliable.
Adding a retry strategy to your previous code could look something like this in Python:
xxxxxxxxxx
MAX_RETRIES = 5
for attempt in range(MAX_RETRIES):
try:
udp_socket.sendto(b'Hello, World!', ('localhost', 8080))
print(udp_socket.recv(1024)) # Wait for a response
break # Break the loop if response is received
except socket.timeout:
print(f"Attempt {attempt + 1} failed, retrying...")
In this adapted script, the code will continuously retry sending the message for five times whenever a timeout occurs.
UDP and its purpose
However, it's notable to take into account that implementing these mechanisms defies the fundamental principles and intended purpose of UDP. The protocols such as UDP exist as they are optimally suitable for specific applications even without the embedded security and reliability functions present in others such as the TCP. For instance, real-time services like gaming or streaming video greatly benefit from the 'simplicity' of UDP where speed is favoured over absolute reliability.
To sum up, while there are strategies to make UDP more reliable, including timeouts and retry strategies, these should be used judiciously, keeping in mind the potential impacts on performance, particularly in latency-sensitive applications.
For more information on UDP, check out the official Internet Engineering Task Force (IETF) specification.Application Layer Protocols refers to protocols that are used by networked applications as they communicate over a network. Examples of these application layer protocols include Hypertext Transfer Protocol (HTTP), File Transfer Protocol (FTP), Post Office Protocol (POP), and Simple Mail Transfer Protocol (SMTP) among others. These protocols use a combination of mechanisms to deliver data reliably over an inherently unreliable system such as the Internet. Acknowledgements, retransmissions and error-detection codes are commonly employed strategies for ensuring reliability.
Protocol | Description |
---|---|
HTTP | Hypertext Transfer Protocol is used in web browsers to access websites and communicate with web servers. |
FTP | File Transfer Protocol is used for transmitting files between computers on a network. |
POP | Post Office Protocol is used by local email clients to retrieve emails from a remote server. |
SMTP | Simple Mail Transfer Protocol is primarily used for sending messages between email servers. |
User Datagram Protocol (UDP) is another protocol used frequently, particularly in real-time scenarios like video conferencing where high speed is paramount and some data loss is acceptable. UDP does not come with built-in error checking and recovery services. Data packets that arrive out of order, corrupted or not at all must be handled by the application. Hence, UDP on its own, is considered unreliable.
However, it potentials to make UDP reliable. It's a matter of incorporating certain features of Transmission Control Protocol (TCP), which ensure reliability, into UDP. Here's how:
Packet Acknowledgment: The receiver sends acknowledgement packets back to the sender. This ensures the sender that the packet has been successfully received.
xxxxxxxxxx
SENDER RECEIVER
Send Packet ------>
<------ Send Acknowledgement
Sending windows: Rather than transmitting one packet at a time, the sender can transmit a "window" of packets simultaneously. If all acknowledgements are received, the sender moves the window forwards. If an acknowledgment is missed, the sender will repeat the whole window.
xxxxxxxxxx
SENDER RECEIVER
Send Packets 1,2,3 ------->
<------- Received Packets 1,2,3
Timeouts and retransmissions: If no acknowledgment is received within a certain amount of time, the sender assumes the packet has been lost and resends it.
xxxxxxxxxx
SENDER RECEIVER
Send Packet ------>
Time Out
Resend Packet ------>
These methods are associated with overheads and may increase latency, but they do grant UDP a greater degree of reliability. Thus, it’s possible to leverage the speed of UDP and still maintain a certain level of data integrity. However, you'll need to consider whether or not this suits your specific use case.
For some developers, creating custom solutions makes sense. For instance, if you're working on a real-time game or live streaming service where latency is critical, you might choose to build reliability features atop UDP.
On the other hand, projects like QUIC, a Google initiative that aims to create a more reliable and faster transport layer protocol based on UDP, are interesting to look at.
In summary, while UDP doesn’t provide reliability mechanisms out of the box, it's absolutely possible to incorporate those capabilities depending on the use cases and requirements.With the application of certain techniques, it is indeed plausible to enhance the reliability of User Datagram Protocol (UDP). UDP, by its very nature, it is an unreliable and connectionless protocol. However, if our application requires assured data delivery which entails retransmission of lost packets, in-order delivery of messages, congestion control, and error checking, we need to incorporate additional protocols or utilize a reliable UDP library at the application layer.
Below are methodologies to leverage reliability on top of UDP:
- Arq Techniques
xxxxxxxxxx
Automatic Repeat Request (ARQ)
is a protocol used in telecommunications to request for retransmissions of lost or corrupted data. Some common methods are Stop-and-wait ARQ, Go-Back-N ARQ, and Selective Repeat ARQ.
- Using Reliable UDP Libraries
Protocols like Real-time Transport Protocol (RTP), and Real-Time Streaming Protocol (RTSP) have been developed to provide reliability to UDP traffic. Libraries such as UDT (UDP-based Data Transfer), RUDP (Reliable UDP), and ENet provide reliability along with many other features.
- Development of Custom Application-Level Protocol
Developing a custom protocol encompassing sender acknowledgments, packet numbering (for sequencing), checksum validation, and perhaps even some form of windowing is another way to make UDP reliable.
Here's how a checksum validation works:
xxxxxxxxxx
def udp_checksum(data):
s = 0
n = len(data) % 2
for i in range(0, len(data)-n, 2):
s += ord(data[i]) + (ord(data[i + 1]) << 8)
if n:
s+= ord(data[i + 1])
while (s >> 16):
s = (s & 0xFFFF) + (s >> 16)
s = ~s & 0xffff
return s
However, every method has its pros and cons. While these methods can bestow reliability upon UDP, they cannot transform UDP into TCP. Each protocol was designed with specific purposes and use-cases in mind, hence attempting to turn one protocol into another may defeat the underlying principles and advantages each offers. It's crucial to consider the needs and requirements of your specific project when deciding to add reliability to UDP, and always be aware that performance overhead may ensue due to the addition of extra operations.
Finally, one must ensure that the SEO keywords like 'Making UDP Reliable', 'Reliable UDP', 'UDP', 'ARQ Techniques' and related terms, should ideally feature prominently throughout your discourse in order to cement the understanding that you are indeed addressing the topic of making UDP reliable in the eyes of search engines. For further insight, please refer to this article (source).