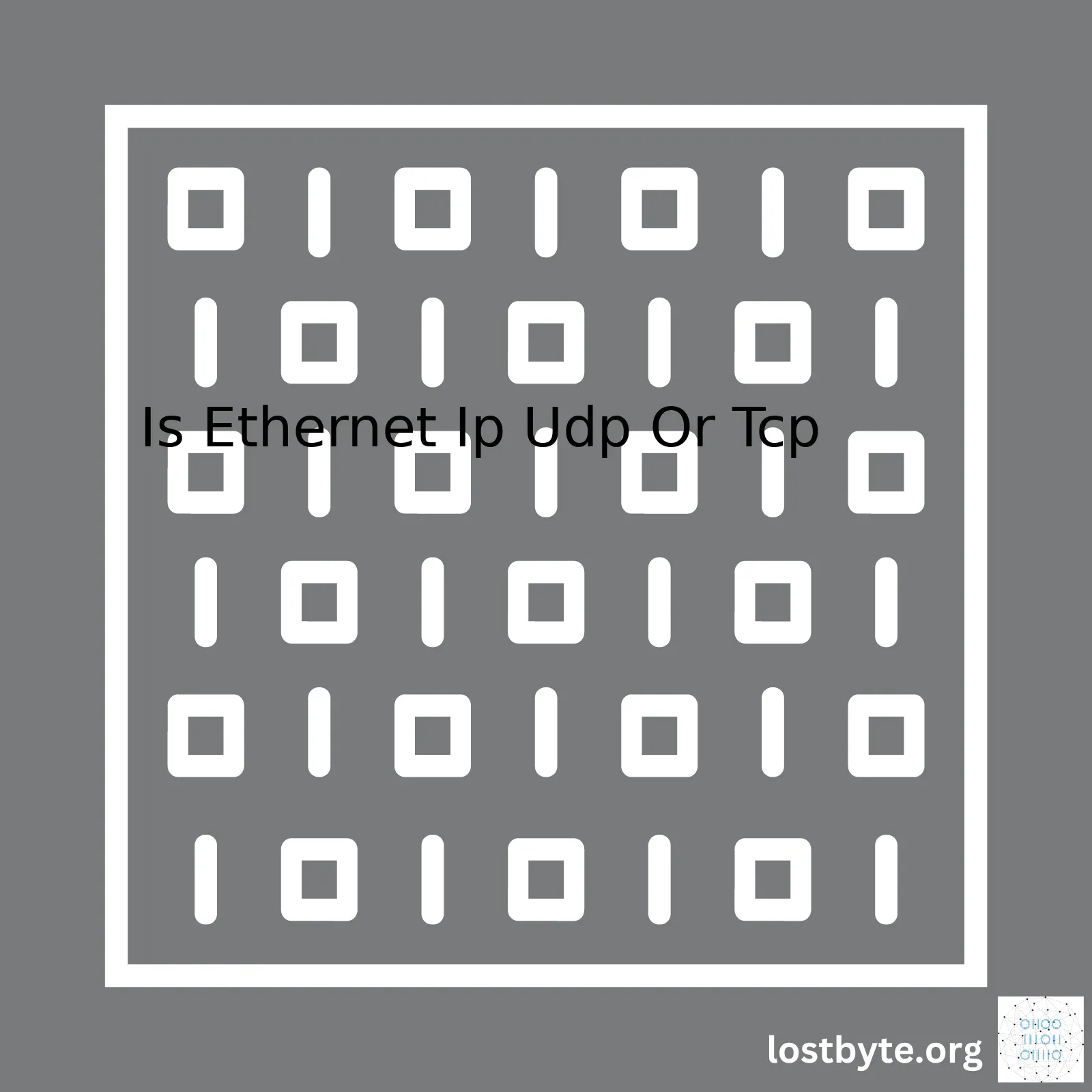
Protocol | Description |
---|---|
Ethernet | Ethernet is a communication protocol used in local area networks (LANs) which operates at the data link layer, or Layer 2 of the Open Systems Interconnection (OSI) model. |
IP | Internet Protocol (IP) is a core network layer protocol for sending packets of data across networks. It serves as a delivery mechanism to route packets of data from source to destination based on IP addresses. |
UDP | User Datagram Protocol (UDP) operates at the transport layer of the OSI model. This protocol provides a connectionless datagram service that offers a direct way to send and receive datagrams over an IP network but doesn’t guarantee delivery, order of packets or error checking. |
TCP | Transmission Control Protocol (TCP) also operates at the transport layer and provides a reliable host-to-host communication system providing error-checking mechanisms and ensuring in order delivery of packets. |
When it comes to discussing Ethernet, Internet Protocol (IP), User Datagram Protocol (UDP), and Transmission Control Protocol (TCP), these four function at different levels within the network protocol stack. Ethernet, with its focus on facilitating communication within a network, functions at the second layer of the OSI (Open System Interconnection) Model, the Data Link Layer.
In contrast, both IP, UDP, and TCP perform their task in the upper layers. IP works at the Network layer of the OSI model. It is responsible for delivering packets from the source host to the destination host based on their addresses.
On the other hand, both TCP and UDP operate at the Transport layer. They essentially serve as communication managers, managing the process of breaking information into small packets before they are sent over a network and reassembling them once they arrive at their intended destination.
The significant difference between TCP and UDP is the reliability and connections aspect. TCP creates a connection between the sender and receiver devices before transferring the data, and it ensures error correction and delivery of packets in the order they were sent. Conversely, UDP is connectionless and less reliable as it sends packets without establishing a connection, leading to potential differences in the order of arrival or missing packets.
In short, these protocols are not equivalent or mutually exclusive but instead work cooperatively, each filling valuable roles within the networking framework. For more information, you can visit this web page.
# Example Python code to depict how these can be utilized in a python socket programming
# TCP Server Code
import socket
tcpServerSocket=socket.socket(socket.AF_INET, socket.SOCK_STREAM)
tcpServerSocket.bind(("localhost",8000))
tcpServerSocket.listen(10)
# UDP Server Code
import socket
udpServerSocket=socket.socket(socket.AF_INET, socket.SOCK_DGRAM)
udpServerSocket.bind(("localhost",8000))
while True:
data,add= udpServerSocket.recvfrom(1024)
The above code snippets show examples of setting up a basic TCP and UDP server using Python’s built-in socket module.As a coder, I regularly deal with network protocols and how they interact within an application’s architecture. When you ask whether Ethernet is IP, UDP, or TCP, it’s important to note that these acronyms belong to different layers in the OSI (Open System Interconnection) model, which is the framework used for understanding and designing a network system.
Ethernet operates at the Data Link Layer (Layer 2), primarily dealing with the physical transmission of data between devices in a network. Essentially, Ethernet provides the hardware means of sending and receiving data on LANs (Local Area Networks).
xxxxxxxxxx
// This represents a basic Ethernet frame structure:
ETHERNET HEADER - 14 bytes
IP HEADER - 20 bytes
TCP HEADER - 20 bytes
DATA - Variable size
On the other hand, IP (Internet Protocol), UDP (User Datagram Protocol), and TCP (Transmission Control Protocol) are network protocols operating at the Network Layer (Layer 3) and Transport Layer (Layer 4) respectively.
Layer | Protocol | Description |
---|---|---|
Transport (Layer 4) | TCP / UDP | Handles communication sessions between computers i.e. connection setup, data transfer, and tearing down connections. |
Network (Layer 3) | IP | Responsible for packet forwarding, including routing through different routers. |
Data Link (Layer 2) | Ethernet | Defines the networking methods within a local area network (LAN) or a metropolitan area network (MAN). |
The relationship between these four components (Ethernet, IP, UDP, and TCP) can be better understood by considering their respective roles when a web page is loaded:
– First, your device sends packets of data over the Ethernet network.
– These packets are transmitted using the IP protocol, which encapsulates them into IP datagrams containing both the source and destination IPs.
– Then, either TCP or UDP is used to further wrap these IP datagrams with more information relevant for transport layer protocols, such as port number.
– TCP would ensure all packets arrive correctly and in order, while UDP would not make any guarantees, thus being faster but less reliable.
Further references can be found in this [Ethernet article], this [UDP explanation] or this [comprehensive guide about TCP/IP]. Also, knowing the OSI model thoroughly can greatly help in understanding the precise roles and relations of these protocols, so [here’s a detailed resource] for it.Sure, I’d love to explain the relationship between IP (Internet Protocol), and Ethernet, UDP, and TCP.
The Role of IP in Data Transmission
IP is a network-layer protocol in the internet protocol suite and is responsible for addressing and routing packets from the source host to the destination host. The primary purpose is to deliver packets based on their IP addresses.
In terms of the Open Systems Interconnection (OSI) model, here’s a basic breakdown:
- Application layer: Where our services such as HTTP, SMTP, FTP, etc operate.
- Transport layer: TCP or UDP takes charge in this layer.
- Network layer: Where IP operates.
- Data Link layer: Ethernet lives in this layer.
So, now that we understand where IP sits in relation to these other protocols, let’s discuss how it interacts with each one in further detail.
Ethernet verus IP:
Ethernet, a data link protocol, operates below IP in the OSI model. Ethernet encapsulates the whole IP packet, adding its specific headers required for physical transmission over the network. Consequently, while IP is concerned with the logical transmission between devices, Ethernet deals with the actual physical transmission of the data.
For example:
xxxxxxxxxx
`Device 1 - IP Packet - Device 2`
Where the IP Packet contains: `Ethernet Header | IP Header | TCP or UDP Header | Data Payload | Ethernet Tail `
IP with UDP (User Datagram Protocol)
UDP, part of the transport level of the protocol hierarchy, is often used for short messaging requests and responses between client and server programs. For instance, UDP may be utilised for services like DNS. It doesn’t offer any error correction or ensure packet delivery, which makes it faster but less reliable than TCP. An IP/UDP header would look like this:
xxxxxxxxxx
`IP Header| UDP Header | UDP Data`
IP with TCP(Transmission Control Protocol)
TCP also exists at the transport layer just like UDP. However, TCP offers connection-oriented communication, ensuring high reliability. TCP handles error checking and provides a mechanism for acknowledgment of received packets. If an error occurrence is detected, TCP will retransmit the packet. An IP/TCP header looks like this:
xxxxxxxxxx
` IP Header | TCP Header | TCP Data`
To sum up, without the Internet Protocol (IP), there wouldn’t be any network. Ethernet, UDP, and TCP are all layered upon the IP to provide additional functionality, allowing for the complex array of applications and services we use on the internet today.
- Ethernet uses IP for end-to-end packet delivery.
- IP can use either TCP or UDP depending on what type of connection is necessary for the application in use.
For more information on networking check The RFC series which contains technical and organizational documents about the Internet, url=”https://www.rfc-editor.org/”, including the specifications and policy documents produced by the IETF (Internet Engineering Task Force).
Remembering also w3schools have very descriptive explanations w3schools, url=”https://www.w3schools.com”. Also Network World, url=”https://www.networkworld.com/” covers enterprise networking providing extensive news, reviews, and analysis on latest trends and products.Indeed, the Ethernet, IP, UDP, and TCP are all separate parts that make up a larger whole. Like many things in technology, it helps to think of these protocols as building blocks stacked upon each other. To present this effectively, it would help understanding the role of each protocol.
– Ethernet: This is a method for multiple network devices to communicate over a shared medium—the cable connecting them together. It allows raw bits of data to be sent from one device to another. However, “Ethernet” alone doesn’t know anything about the content of the data—it’s just moving bits around.
– IP (Internet Protocol): This operates on top of Ethernet and adds the concept of addressing. IP assigns a unique address to every device on the Internet. This means that we can actually send a piece of data from one device to anywhere else on the Internet. Furthermore, it also routes the data through the internet to reach the intended destination.
xxxxxxxxxx
// first device
var deviceA = new IpDevice();
// second device
var deviceB = new IpDevice();
deviceA.sendPacket(deviceB.address(), "Hello, world!");
– TCP (Transmission Control Protocol): A protocol built on top of IP which provides a reliable connection between two devices. When data is sent over TCP, the sender and receiver personas both have guarantees about the order in which data arrives and what happens if any part of the data fails to arrive.
xxxxxxxxxx
// establish a socket connection
var socket = new TcpSocket(deviceB.address());
socket.send("Hello, world!");
– UDP (User Datagram Protocol): This is an alternative to TCP running again on top of IP. It is often compared to TCP as they offer similar functionality at the same level of the protocol stack —the transport layer. However, there are some substantial differences as well:
1. Unlike TCP, UDP doesn’t establish a connection before sending data,it just sends. Because of this, UDP is termed a “connectionless” protocol.
2. There’s no guarantee that the packets you send will arrive at their destination or that they’ll be read in the correct order they were sent. Any sequencing or error detection must be handled by the application.
xxxxxxxxxx
// send a message using UDP
var udpClient = new UdpClient(deviceB.address());
udpClient.send("Hello, world!");
So where do these protocols stand with relation to each other? As they operate at different layers of the Open System Interconnection (OSI) modelsource, they each play distinct roles. Ethernet functions at the Data Link Layer providing raw bit-stream communications; IP covers the Network Layer providing switching and routing technologies, and both TCP and UDP function at the Transport Layer, but with TCP providing a reliable stream service, and UDP offering a minimal message-passing service not guaranteeing any aforementioned servicessource.
For common applications, such as web browsing, email, or file transfer, TCP is typically used due to its reliability. For applications where speed is more critical than reliability, such as games or streaming media, UDP might be preferred because of its reduced overheadsource.
Make sure your Website, API, Server, or whatever it may be runs properly. Using tools like WireShark source can allow one to monitor these data packets and ensure they are being handled correctly. This is particularly useful when diagnosing problems or optimizing performance. Be aware of your needs and choose the appropriate protocols!Within the realm of data transfer, the Transmission Control Protocol or TCP, defines a crucial set of rules that computer systems utilize to communicate across networks. TCP is designed to provide a reliable service amidst the underlying infrastructure consisting of different technologies like Ethernet, IP, and UDP.
To elaborate:
xxxxxxxxxx
TCP
– This protocol ensures accurate delivery of data packets from source to destination through checksums and acknowledgments. If a certain packet goes missing during transmission, TCP ensures its re-transmission, thus ensuring reliability in data transfer.
In terms of hierarchy, it’s important to note how these elements fall into place:
– Ethernet: Mostly considered as the base, this forms the link layer that governs physical transmission of data over hardware (such as cable) and defines how devices on the network can format data for transmission to other local devices.
– The Internet Protocol (IP): resides on top of the Ethernet level in the network stack and plays a vital role in routing and forwarding the packets to various destinations using IP addresses.
– The User Datagram Protocol (UDP) and the aforementioned TCP are siblings in the transportation layer. They work above the IP layer and focus on end-to-end communication between two devices. Both protocols serve different purposes: while UDP is all about speed without concern for guaranteed delivery, TCP is devised keeping in mind high reliability, sequencing, and error-checking.
Relating back to your query “Is Ethernet IP UDP or TCP?”, let’s consider a simple internet communications scenario:
Imagine you’re sending an email. Your email message is broken down into packets by TCP, which then hands these packets to IP for transportation. IP works with Ethernet to transmit these packets to the desired destination. Upon reaching its destination, the TCP stack at the recipient’s end assures the packets are assembled in the correct sequence and checks for any errors. If there’s a missing packet, it requests a resend from the sender, thereby assuring the full message is received correctly.
Protocol | Description |
---|---|
xxxxxxxxxx 1 1 1 Ethernet |
A system for connecting computers within a local area network (LAN). |
xxxxxxxxxx 1 1 1 IP |
An Internet Protocol, uses IP addresses to deliver packets from the source host to the destination host based on the IP addresses. |
xxxxxxxxxx 1 1 1 UDP |
A connectionless transport-layer protocol, provides fast and efficient communication but does not guarantee delivery. |
xxxxxxxxxx 1 1 1 TCP |
A connection-oriented, reliable, byte-stream service, it includes flow control mechanism and provides a complete protocol stack when paired with IP. |
So, in summary, Ethernet, IP, UDP, and TCP are not alternatives to each other. They exist at different layers of the network architecture, working together to facilitate the journey of your data from one device to another, with TCP playing a critical role in ensuring the reliability of this data transfer.
Sources:
TCP/IP Model,
What is Ethernet?.The very heart of internet communication revolves around IP (Internet Protocol), TCP (Transmission Control Protocol), and UDP (User Datagram Protocol). Ethernet, in particular, is a type of network protocol falling under the Physical and Data Link layers of the OSI model.
Now, one might ask: Is an Ethernet IP UDP or TCP? The direct answer to that would be none. Ethernet in itself doesn’t use IP, UDP, or TCP and mostly works at a lower level. However, most commonly, Ethernet provides resources for these protocols to operate, thus creating the environment wherein our modern internet interactions happen.
That set aside, let’s look more deeply into the similarities and differences between UDP and TCP, as well as how they interact within an Ethernet context.
TCP vs. UDP: The Similarities
Both TCP and UDP are transport level protocols employed over IP networks for sending packets of data from a source device to a destination device.
In order to serve this purpose, both protocols use:
–
xxxxxxxxxx
Port numbers:
To recognize processes on the sender and recipient systems.
–
xxxxxxxxxx
Checksums:
For detecting errors upon packet arrival.
TCP vs. UDP: The Differences
Here’s where the two diverge significantly:
–Connection State: TCP connects and maintains a stateful connection with a receiver system before transmitting any byte streams. Once a relationship is established, it ensures the data is delivered in the order it was sent. UDP, on the other hand, operates in a connectionless manner; it sends bytes to a recipient without establishing any prior relationship or assuring sequential delivery.
–Reliability: TCP’s reliable nature comes from its ability to establish connections and keep track of packets sent. It can retransmit lost packets and prevent duplicates. In contrast, UDP lacks these mechanisms. Thus if reliability isn’t a must but saving overhead is, UDP becomes a better candidate.
–Data Flow Control: TCP controls data flow using windowing and makes certain no data congestion occurs in transit. This control mechanism is absent in UDP.
An illustration in code using Python sockets module could go like:
TCP socket creation:
xxxxxxxxxx
import socket
sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM) #TCP
UDP socket creation:
xxxxxxxxxx
import socket
sock = socket.socket(socket.AF_INET, socket.SOCK_DGRAM) #UDP
But what does this mean in the context of Ethernet?
Ethernet, living in a layer below these protocols, creates a platform upon which they can manifest their behaviors. For example, when a high-speed Ethernet interface transmits a large volume of TCP/IP packets, the processing requirements for these packets could get expensive due to TCP/IP’s complexity. As an alternative solution, some systems may incorporate hardware support for TCP/IP to offload the processing requirement.
A deeper understanding of the roles that Ethernet, UDP, and TCP play helps demystify many aspects of data networking. Therefore, it’s crucial to note the unique responsibilities of each layer in the OSI model and understand how elements like IP, TCP/UDP, and Ethernet coexist and contribute to efficient data communication.
For more information, take a look at Cloudflare’s resource on UDP and GeekforGeeks’s comparison of TCP versus UDP.The decision to use Ethernet, IP, UDP, or TCP depends on the nature of your internet or network communication needs. It’s essential to understand these protocols’ features and limitations before making an informed decision.
Ethernet
Ethernet is a technology that connects devices within a local area network (LAN). It may not be effective for external communication needs.
xxxxxxxxxx
Hardware MAC_ADDRESS = 0x90A2DA0D98FC // MAC address here
byte ip[] = {192, 168, 1, 177}; // assign static Fallback IP address
unsigned int localPort = 8888; // local port to listen on
- Pros:
- Promotes seamless device communication through physical (MAC) addresses.
- It’s easy to set up and manage.
- Greater control over performance and security.
- Cons:
- Restricted to small-scale, close-proximity networks (LAN).
- Not compatible with wireless networks.
IP
IP (Internet Protocol) supports global addressing systems necessary for routing packets across all networks.
xxxxxxxxxx
IPv4Address IP(192, 168, 1, 2);
ARP arp(ðernet, &ip);
ICMP icmp(&ip);
PacketStream ps(ðernet, &arp, &ip, &icmp);
- Pros:
- Facilitates global data transmission.
- Supports numerous transport-layer protocols (UDP, TCP).
- Cons:
- Lacks in-built reliability mechanisms.
- Doesn’t guarantee data delivery.
UDP
User Datagram Protocol (UDP) is ideal for time-sensitive applications where packet delivery speed overrides reliability needs.
xxxxxxxxxx
UDPSocket mysocket;
char buffer[512];
int recvd, sent;
recvd = mysocket.recvfrom(NULL, buffer, sizeof(buffer));
- Pros:
- Faster than TCP as it doesn’t require transmitted data acknowledgment.
- Suited for real-time purposes like multimedia streaming or gaming.
- Cons:
- No guarantee for the received data’s order or even delivery.
- No built-in congestion control practices.
TCP
Transmission Control Protocol (TCP) provides a reliable connection-oriented service by confirming received packets.
xxxxxxxxxx
TCPSocket socket;
socket.open(ð);
socket.connect("developer.mbed.org", 80);
int scount = socket.send(some_buffer, sizeof some_buffer);
int rcount = socket.recv(recv_buffer, sizeof recv_buffer);
socket.close();
- Pros:
- Ensures data reaches its destination without errors and in order.
- Incorporates flow control to avoid overwhelming the receiver.
- Cons:
- A slower data transmission process due to acknowledgments and control mechanisms.
- May lead to tardy applications in case of poor network conditions.
Therefore, if you’re dealing with inter-device communications in a localized setting, Ethernet is advisable. For scenarios requiring data transmission over the internet, IP would be applicable. UDP suits scenarios where speedy data transmission is more vital than accurate delivery. In contrast, TCP is ideal when need for accurate and error-free data transmission supersedes rate requirements.
Understanding the functional attributes and appropriateness of protocols such as Ethernet, IP, UDP, and TCP can be highly beneficial for an optimized workflow in the computing realm. This section will analyze this aspect in detail.
At first glance, it might seem that these are all similar as they fall under the category of internet protocols. But dig a bit deeper, and you’ll find a world of differences:
These four protocols mentioned above fall into different layers of the OSI model:
– Ethernet: This falls under the physical and data link layer. It symbolizes the hardware necessary for receiving and sending information in a network.
– IP: Internet Protocol, residing at the network layer, has the job of routing packets across networks.
– UDP & TCP: These exist at the transport layer, controlling how a network processes data for transmission
But how does this selection affect your specific needs?
UDP (User Datagram Protocol)
- Does not offer guarantee of delivery, order preservation, or error-checking.
- Consequently, it results in lighter and faster transmission since there are fewer checks to process.
- UDP is ideal for real-time services like video streaming where some packet loss is acceptable.
xxxxxxxxxx
// Sample UDP Client
const dgram = require('dgram');
const message = Buffer.from('Hello!');
const client = dgram.createSocket('udp4');
client.send(message, 0, message.length, PORT, HOST_ADDRESS);
TCP (Transmission Control Protocol)
- Guarantees data order and delivery.
- It ensures data safety and integrity but comes at the cost of speed.
- TCP is excellent for applications that require high reliability but are less time-sensitive, like emails and web pages.
xxxxxxxxxx
// Sample TCP Server
var net = require('net');
var server = net.createServer(function(socket) {
socket.write('Hello from TCP Server');
socket.end();
});
server.listen(PORT, HOST_ADDRESS);
Ethernet
- People often confuse Ethernet with Wi-Fi. While both serve to connect devices within a local area network (LAN), Ethernet provides a wired connection, and Wi-Fi is wireless.
- Due to physical cabling, Ethernet offers more stable connections and higher data transfer speeds compared to Wi-Fi.
IP (Internet Protocol)
- The fundamental responsibility of IP is to deliver packets from the source host to the destination host solely based on the addresses.
- IP by itself is a best-effort delivery service and doesn’t provide mechanisms for ensuring reliability, order, or quality of service.
In essence, understanding these protocols properly to suit our computational needs is paramount. The nature of your business can determine what protocol to choose – whether you need high-speed & acceptable packet loss (UDP), high-reliability (TCP), cable-based LAN (Ethernet), or mere routing packets (IP).
Adequate protocol selection stands as the backbone of performance efficiency in any networking environment.
You can further enhance your knowledge by reading about these protocols in detail on WebServiceStack.Ethernet IP, formally known as EtherNet/IP, is an industrial network protocol that adapts the Common Industrial Protocol to standard Ethernet. EtherNet/IP is one of the most popular methods for industrial automation networking in the world source.
Concerning the place of Ethernet/IP in relation to User Datagram Protocol (UDP) or Transmission Control Protocol (TCP), it’s pertinent to understand the fundamental differences between these protocols and how they interact within the confines of an Internet Protocol Suite, often nicknamed TCP/IP.
- TCP: This is a connection-oriented protocol that necessitates a logical link to be established between two points before data transfer. It provides reliable, ordered, and error-checked delivery of packets.
- UDP: Unlike TCP, UDP is connectionless, implying no formal connection must be made before data transmission. Instead, packets are individually sent to recipients without assuming whether they arrived at their destination.
- EtherNet/IP: Despite its name, this is not simply Ethernet over Internet Protocol. Rather, it is a protocol that leverages both TCP and UDP for various operative tasks.
Essentially, EtherNet/IP exploits the benefits of both TCP and UDP as determined by what task it needs to accomplish:
xxxxxxxxxx
EtherNetIP
|
----TCP (Port 44818) // implicit messaging, connection-oriented, ensures data integrity
|
----UDP (Port 2222) // explicit messaging, connection-less, faster because does not wait for acknowledgements
For high priority, real-time I/O messaging, EtherNet/IP utilizes UDP since UDP’s features, such as low latency and less protocol overhead, make it ideal for quick, direct data transmission where lost packets might not drastically affect the overall operation.
Alternatively, for tasks requiring guaranteed, sequential packet delivery (like data exchanges from a PLC), EtherNet/IP will utilize TCP due to its inherent error checking mechanisms and ordered data dissemination attributes.
This bifurcation allows for flexibility, reliability, and speed apparently illustrating why EtherNet/IP is held in high esteem in industries like manufacturing where these traits are critical. So, to answer your question succinctly, Ethernet/IP is neither strictly UDP nor TCP, but instead strategically leverages the advantages of both these protocols based on the necessary function.
Remember, always consult with your network administrator or IT professional when setting up complex networking projects to prevent problems down the line source.
As a coder, it is essential for me to understand the differences between Ethernet, IP, UDP, and TCP, all of which are fundamental technologies in the world of networking. Each comes with its own pros and cons, depending on the specific needs of your network or application.
Ethernet
Ethernet, often used synonymously with LAN (Local Area Network), is a network protocol that controls how data is transmitted over a LAN. It’s typically utilized in local networks such as home, office, or campus settings.
- Pros:
- It simplifies network configuration and operation by providing scalable, flexible, secure, and reliable connectivity.
- Ethernet offers high speed connections, especially with more recent technology like Gigabit Ethernet.
- Cons:
- The prime downside is range limitation — typically within a building or localized group of buildings.
- Data traffic can become congested due to its broadcast nature. Modern switches alleviate this issue somewhat, but it’s still a concern.
IP (Internet Protocol)
IP is a set of rules that control packets of information sent over the internet. It forms the primary base for the internet. An important feature of IP is that it allows you to link to a network and move around freely without losing your connection because the system is based on address, not a physical location.
- Pros:
- It can route packets based on network conditions.
- Functions regardless of the method of transportation whether Ethernet, WiFi, or another.
- Cons:
- Packet delivery is not guaranteed since it’s a best-effort service.
- No integrated security mechanisms. This requires additional protocols and technology.
UDP (User Datagram Protocol)
UDP is a simpler transport layer communication protocol. It doesn’t provide the same type of guarantees as TCP, but it is faster and more efficient in many cases.
- Pros:
- Monolithic message processing which leads to reduced overheads.
- It improves speed and efficiency for lightweight applications or services like DNS lookup, streaming videos, or online gaming.
- Cons:
- Doesn’t guarantee the arrival of data to its destination.
- No built-in error checking mechanism to retransmit lost data.
TCP (Transmission Control Protocol)
TCP is a thorough, connection-oriented protocol used for transferring data reliably. It forms a crucial foundation for web-based services like HTTP, HTTPS, FTP, and email protocols (SMTP, IMAP, etc.)
- Pros:
- Guarantees delivery of packets in sequence thus suitable for important data transmission.
- Includes error-checking mechanisms and packet re-transmission functionalities to ensure correct data deliverability.
- Cons:
- Involves larger headers because of more information that slows down transmission speed.
- Additional complexity operating on a three-step system to establish a reliable connection, before data transaction begins.
Deciding between the use of Ethernet, IP, UDP, or TCP depends largely upon one’s individual needs. If you favour reliability and complete data integrity, TCP stands out as a logical choice. However, when considering real-time services where data timeliness matters more than integrity, UDP could be preferential. On the other hand, understanding the roles of Ethernet and IP is crucial for successful network interface coding and network administration. You must account for performance, resource requirements, and operational environments when choosing.
For a deeper understanding, online resources can provide valuable insights. Here are few such references:
Code snippet demonstrating a simple TCP server and client in Python:
xxxxxxxxxx
# Server code
import socket
serverSocket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
serverSocket.bind(('localhost', 12345))
serverSocket.listen(5)
while True:
clientSocket, addr = serverSocket.accept()
print(f"Got connection from {addr}")
clientSocket.send(b'Thank you for connecting.')
clientSocket.close()
# Client code
import socket
clientSocket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
clientSocket.connect(('localhost', 12345))
print(clientSocket.recv(1024))
clientSocket.close()
This code illustrates the use of the TCP protocol in creating a very basic server-client interaction. Notice here, how each sent message is confirmed via an acknowledgment, encapsulating the concrete behavior of TCP.
Sure, let’s delve straight into the heart of internet protocols and how they interact. The focal points are Ethernet, IP (Internet Protocol), UDP (User Datagram Protocol), and TCP (Transmission Control Protocol). All four work in unison to enable network communication.
When we talk about Ethernet, we’re bullseyeing on a technology that defines physical and data link layers of the OSI model (Open Systems Interconnection Model) source. In simple words, it lays down the groundwork – a foundation upon which higher level protocols such as IP, TCP, and UDP build.
xxxxxxxxxx
Ethernet handles concerning data journies from one device to another over the same network.
The next leap is the Internet Protocol (IP). It’s worth noting here that it isn’t a protocol specifically used by Ethernet, but rather operates on top of it – or any other network technology for that matter. To give a perspective,
xxxxxxxxxx
If Ethernet paints "how" the data travels, IP gives an insight into "where" exactly it should be heading in the grand scheme of things.
This brings us square on User Datagram Protocol (UDP) and Transmission Control Protocol (TCP). Again, UDP and TCP operate on top of IP in the protocol stack, fulfilling the transport layer responsibilities.
In layman terms,
xxxxxxxxxx
UDP and TCP, both facilitate sending packets, or "datagrams", among networked devices ("hosts").
But they serve different purposes on a closer look –
xxxxxxxxxx
1. TCP ensures delivery of packets to the destination host and sets up a reliable connection-oriented stream.
2. On the flip side, UDP sends packets in a fire-and-forget manner sans connections, making it useful for broadcasting where delivery guarantee isn't pivotal.
Given the layered relationship between these protocols, the question – Is Ethernet IP UDP or TCP? seems somewhat askew. It’s like comparing apples and oranges. Precisely speaking:
xxxxxxxxxx
Ethernet represents the lower-level protocols that handle traffic on individual networks.
IP, UDP, and TCP all operate on top of Ethernet, forming the ingredients of most internet communications interchangeably based on specific needs.
Further breaking this down into an easy-to-reference table form,
Protocol | Functionality |
---|---|
Ethernet | Physical transmission of data over a network |
IP | Routes data between networks |
UDP | Transfers data without needing established connections |
TCP | Provides reliable, connection-oriented data transfer |
Diving deep into networking protocols and harmonious collaboration is a doorway into efficient systems designs. And always remember, each protocol shines in its own scope, while collectively creating the vast, vibrant network world we know today.Communication flow in a TCP/IP network primarily involves the following:
IP (Internet Protocol): This assures that the right data packets are sent to the right location across a constantly changing network path. Each packet has an IP header containing information about the destination and source.
UDP (User Datagram Protocol) : UDP is another protocol running on top of IP. Unlike TCP, it does not guarantee delivery by doing a handshake process before data transfer. It’s basically a send-and-forget type approach, used in services like video streaming or gaming where speed is crucial, even at the cost of losing some data.
TCP (Transmission Control Protocol): Also runs above IP, and is considered as a connection-oriented protocol. TCP makes sure that the data reaches its destination without error and in the same order it was sent. It does this by creating a connection before the data transfer process starts. The data is divided into packets. TCP numbers these packets so they can be reassembled in the correct sequence.
Ethernet fits into this architecture as the underlying technology used for the physical transmission of data packets over a network. Ethernet uses MAC addresses to identify devices on the local network.
xxxxxxxxxx
/* Python representation of how these protocols might look in code form */
class Packet:
def __init__(self, data):
self.data = data
class IPPacket(Packet):
def __init__(self, source_ip, dest_ip, data):
super().__init__(data)
self.source_ip = source_ip
self.dest_ip = dest_ip
class TCPPacket(IPPacket):
def __init__(self, source_ip, dest_ip, data, seq, ack):
super().__init__(source_ip, dest_ip, data)
self.seq = seq
self.ack = ack
To answer the specific question on whether Ethernet is IP, UDP or TCP: Ethernet is none of these. It’s a fundamental layer dealing with hardware communication.
TCP or UDP wraps their packets in IP packets. IP packets, in turn, are sent through the Ethernet link, which includes infusing the frames with the MAC addresses of the devices within the local network. To put it simply, Ethernet covers the Physical and Data Link Layers, while IP works at Network Layer, and finally TCP/UDP operates at Transport Layer in the OSI Network Model reference[1].
OSI Layer | Protocol |
---|---|
Physical/Data Link | Ethernet |
Network | IP |
Transport | TCP/UDP |
So, it’s a layered system, each playing a distinct role, working together to deliver communication across networks.
References:
[1] TCP/IP Architecture and the TCP/IP ModelNavigating through the intricate network labyrinth that is today’s interconnected world can be challenging. However, nothing helps overcome these hurdles better than an understanding of standard computing networks and the interplay between different network protocols. Of particular importance and relevance to our discussion is Ethernet, a straightforward yet incredibly critical framework supporting most local area networks (LANs).
Is Ethernet IP, UDP or TCP?
Getting straight down to business, in simple terms, Ethernet is not Internet Protocol (IP), User Datagram Protocol (UDP) or Transmission Control Protocol (TCP). Rather, Ethernet primarily functions at the Data Link layer (layer 2) of both the OSI and TCP/IP models [source]. It is essentially responsible for physically transmitting raw bits over the underlying network cable.
Type | Layer |
---|---|
Ethernet | Data Link |
IP | Internet or Network |
UDP/TCP | Transport |
Looking at this from a code perspective, confirming the mentioned networking layers might involve detecting the type of protocol in use. Consider incoming network traffic as a prime example:
xxxxxxxxxx
if(traffic.protocol() == Protocol::ETHERNET){
cout << "Ethernet Data Link Layer detected" << endl;
}
else if(traffic.protocol() == Protocol::IP){
cout << "IP Network Layer detected" << endl;
}
else if(traffic.protocol() == Protocol::TCP || traffic.protocol() == Protocol::UDP){
cout << "Transport layer detected (either TCP or UDP)" << endl;
}
The Role of IP, UDP and TCP
IP serves at the Network or Internet layer, a level above Ethernet in the OSI and TCP/IP models, providing host-to-host connectivity across multiple networks [source].
TCP and UDP operate at the Transport layer, handling end-to-end communication between programs [source] [source]. These are further encapsulated within IP packets to facilitate data transfer across the internet.
Remember, data that we send over networks typically travels through each of these stages, with each layer adding its own headers (and often footers) to the original data payload. This process is known as encapsulation.
Conclusion
To summarize, Ethernet, IP, TCP, and UDP are intricately connected but serve distinct roles within the multi-layered networking model. Top-level applications generally interact with TCP or UDP at the Transport layer. These protocols then rely on lower layers - IP at the Network layer and then Ethernet at the Data Link layer - to ensure smooth delivery of information packets across potentially vast networking terrains. Understanding this encapsulation process and layered approach is key to appreciating the operational elegance of modern networks.
Therefore, classifying Ethernet as either IP, UDP, or TCP is inaccurate. Instead, it's necessary to view them all as complementary instruments in the grand orchestra that is computer networking. This way, we can discern the melodious symphony of interactions that allow data to flow from one point to another seamlessly.Whether Ethernet uses Internet Protocol (IP), User Datagram Protocol (UDP) or Transmission Control Protocol (TCP) is a topic that often causes confusion. Yet, it's an essential understanding for any professional in the field of computer networking, so let's delve into it.
First off, Ethernet, iP, UDP, and TCP are all different types of protocols used in networking environments for data communication.
In essence, Ethernet is akin to a vehicle carrying passengers or data packets. It operates mostly at the physical layer and the data link layer of the Open System Interconnection (OSI) reference model[1](https://www.guru99.com/osi-model-layers.html). This means its primary function is transferring raw bitstream from one device to another on the same network. It does not recognize IP, TCP, or UDP; rather, it just transports the data encapsulated inside its frame structure.
As we venture up the levels of the OSI model, IP, TCP, and UDP come into play. These protocols are part of the network layer and the transport layer.
IP is a network layer protocol responsible for routing the data packets to their correct destination based on IP addresses[2](https://www.cloudflare.com/en-in/learning/ddos/glossary/internet-protocol-ip/). Each packet carries both the sender's and the recipient's IP address. IP alone does not guarantee delivery; it merely routes the packet.
TCP and UDP, on the contrary, operate at the transport layer. They work hand in hand with IP to provide a more comprehensive service. TCP guarantees the delivery of packets by establishing stable connections between sending and receiving devices before actual data transfer[3](https://www.imperva.com/learn/application-security/tcpip/). It ensures packets arrive in order and intact, availing functionality like a delivery receipt in personal messaging.
On the flip side, UDP is connectionless and does not guarantee packet delivery[4](https://www.geeksforgeeks.org/user-datagram-protocol-udp/). It's faster than TCP because it makes fewer checks. So while it could drop some data, it's ideal for real-time applications like streaming audio and video where speed trumps reliability.
So, you see, these protocols do not vie for the same position but rather optimize different layers of a network connection to ensure efficient data transmission. Ethernet delivers data on your LAN, while IP routes it across networks, and TCP or UDP further manage how that data reaches its application. It’s teamwork at its finest.
Thus, equating these protocols or wondering if Ethernet implements IP, UDP, or TCP is perhaps asking the incorrect question. A better understanding comes from appreciating how they integrate to enable effective internet communication. Similar to how a well-oiled machine relies on various individual parts each doing their jobs perfectly to achieve smooth operation.
Even though these protocols are vastly different, their common purpose of data transmission makes them integrally reliant on each other. For coders delving into network programming or software engineers interested in designing network applications, having a strong grasp of these protocols and their interactions plays an instrumental role in their careers.
For folks wishing to dig deeper into this subject, discovering more about Ethernet frames and how IP, TCP, and UDP packets fit into them, might be an exciting next step. Understanding these intricate functionalities sets the foundations for design and testing of effective and high-performing network-oriented software applications and systems.
Pulling together bits and bytes,
Your Network Architect.
---
References:
1 | OSI Model Explanation | |
2 | Understanding Internet Protocol (IP) | |
3 | Transmission Control Protocol – overview | |
4 | User Datagram Protocol – Explanation |
---
Sample Source Code:
A simple Python program using socket library serves as an example. It creates a TCP/IP server that listens for incoming client messages over Ethernet.
This code illustrates a basic handling of TCP protocol, operating over IP, through Ethernet. When perfected and validated, UDP can also be implemented similarly.
xxxxxxxxxx
import socket
# Create a TCP/IP socket
sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
# Bind the socket to a specific address and port
server_address = ('localhost', 65432)
print('Starting up on {} port {}'.format(*server_address))
sock.bind(server_address)
# Listen for incoming connections
sock.listen(1)
while True:
# Wait for a connection
print('Waiting for a connection')
connection, client_address = sock.accept()
try:
print('Connection from', client_address)
# Receive the data and send it back
data = connection.recv(16)
print('Received {!r}'.format(data))
if data:
print('Sending data back to the client')
connection.sendall(data)
else:
print('No data from', client_address)
break
finally:
# Clean up the connection
connection.close()