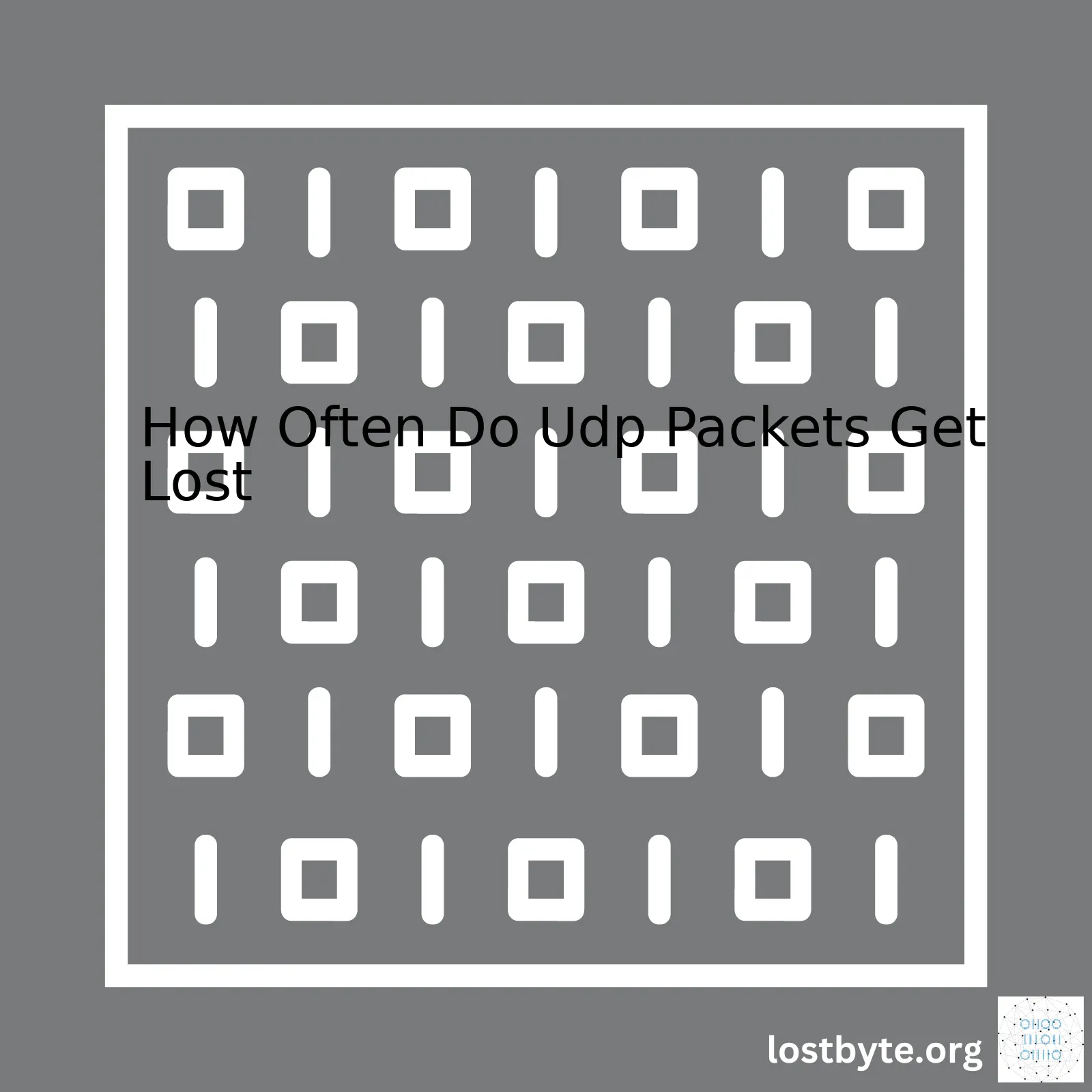
Factors | Description |
---|---|
Network congestion | When a network is congested with high traffic, it leads to higher packet loss. |
Physical interference and hardware issues | Interference like bad weather or hardware issues can cause UDP packets to get lost. |
Routers | Routers decide the priority of UDP packets. Sometimes low-priority packets are discarded causing packet loss. |
Incomplete Sequences | If UDP packets arrive in an unordered sequence, some might be overlooked, resulting in packet loss. |
The User Datagram Protocol (UDP) is a connectionless protocol which, unlike TCP, does not guarantee delivery by retransmitting lost packets. As such, how often UDP packets get lost is largely contingent on several factors.
Network congestion is perhaps one of the most common reasons for packet loss. A network experiencing high traffic levels may lead to packet overflows resulting in discarding some packets. It’s somewhat akin to a highway during rush hour. If the road gets too busy, cars will have to slow down or even stop completely.
Another possible cause is physical interference and hardware issues. Issues such as bad weather conditions, radio interference, or malfunctioning hardware components can disrupt packet transit and cause packet loss.
Routers also play a vital role in retaining or discarding UDP packets. They make the decision regarding which packets take precedence based on various factors. When the buffer space becomes limited, routers may decide to discard some lower priority packets, leading to packet loss.
Moreover, UDP supports packet delivery without a necessary order, which means incomplete sequences can often result in packet loss. For example, if packets 1, 2, and 4 of a sequence reach the destination but packet 3 does not, the sequence could be disregarded.
To counter these challenges and ensure as few packet losses as possible, methods like using wired connections for critical applications, ensuring good quality networking hardware, and congestion management techniques like queuing disciplines on routers can be employed.networkworld.com
Here’s a simple Python script that uses the socket library to create a client-server communication trip illustrating how UDP works. The loss of data or messages manifests how this communication protocol doesn’t inherently guarantee data delivery.
import socket # Create a UDP Server srv_sock = socket.socket(socket.AF_INET, socket.SOCK_DGRAM) srv_sock.bind(('localhost', 9090)) while True: data, addr = srv_sock.recvfrom(4096) print("received message: %s" % data) # Create a UDP Client cli_sock = socket.socket(socket.AF_INET, socket.SOCK_DGRAM) cli_sock.sendto(b'Hello, World!', ('localhost', 9090))
UDP, or User Datagram Protocol, is one of the core protocols in the Internet protocol suite. Unlike TCP (Transmission Control Protocol), UDP is connectionless and does not abide by the process of establishing a connection before transmitting data. This essentially means that UDP sends packets without knowing whether the recipient is ready to receive or even exists.
Understanding the frequency of UDP packet loss entails delving into the characteristics and workings of UDP itself. Let’s break this down:
Features of UDP:
- – No Guaranteed Delivery: UDP doesn’t confirm whether the packets have been delivered or not.
- – Connectionless: It doesn’t establish a connection before sending data.
- – Header Size: The header size of UDP is small, only 8 bytes.
- – Flow Control & Congestion Control: UDP does not provide these, unlike TCP.
Due to its features, UDP is often used in time-sensitive applications like streaming services, online gaming, VoIP and broadcast or multicast transmission where speed takes precedence over accuracy.
Now, how often do UDP packets get lost? The answer to this question isn’t concrete as it depends on multiple factors including network conditions, server congestion, and glitches in intermediate devices.
Network Conditions:
UDP packet loss becomes frequent in unstable network conditions, such as poor Wi-Fi signals or overtaxed ISP networks.
Server Congestion:
If the recipient server is overloaded with traffic, UDP packets may be dropped, resulting in packet loss.
Intermediate Devices:
Routers or firewalls can cause packet loss if they are inefficient or malfunctioning.
To visualize packet loss, oftentimes tools like “Ping” or “Traceroute” are used. They send UDP packets to a specific IP address and then report back the success/failure rates.
$ ping -c 4 www.example.com
This command will send 4 ICMP Echo requests (similar to “phantom” UDP packets) to www.example.com and display how many were successful versus lost.
Conceptualizing packet loss in the context of User Datagram Protocol is imperative for recognizing the efficiency trade-offs in UDP’s quick-and-dirty approach to data transmission. If guaranteed packet delivery is critical, then TCP would be the more appropriate choice over UDP despite the former’s higher overheads.
For further insights, refer to the RFC 768: User Datagram Protocol.
Resources:
Lifewire: How to Use Ping Network Utility,
IPTrends: An Introduction to Monitoring Network Traffic
- UDP, which stands for User Datagram Protocol, is a commonly used protocol in the realm of internet communications. Designed to send short messages known as datagrams but lesser-known for its unreliability, it poses an intriguing question: How often do UDP packets get lost? The answer is not straightforward and depends on various factors.
- UDP transmission happens without establishing a connection between the communicating systems. Hence, it provides no guarantee that the data sent would reach the intended recipient. It delegates the task of ensuring data receipt and error checking to the application using the protocol, meaning packet loss in UDP is possible, although the frequency can be significantly varied.
- The three key factors that affect the likelihood of UDP packet loss include network congestion, poor transmission quality, and system resource limitations:
- To view this loss within your code, we could opt for libraries like scapy in Python that permits crafting, sending, capturing, and analyzing network packets with a few lines of code.
- This rather simple script sends a single UDP packet to the destination IP address. If there’s no response (indicating the packet was lost), it prints “Packet lost”. Otherwise, it confirms the packet being received. Keep in mind; this script doesn’t take into account whether or not the packet arrives at the correct software application, only that it landed somewhere in the specified IP address.
- Packets getting lost is indeed a concern when dealing with UDP, so if you are working on applications where data integrity is critical, consider using protocols with built-in error-checking and re-transmission capabilities. TCP (Transmission Control Protocol) is a recommended alternative in these situations. Nonetheless, UDP is excellent for real-time applications like VoIP, gaming, or streaming where speed is desired over accuracy.
- It’s important to continually monitor UDP packet loss when designing and deploying systems. Developers must ensure the reliability of their underlying network infrastructures, strategically choosing the right time to use UDP as packet loss has wide-ranging implications on application performance and user experience.
Factor | Explanation |
---|---|
Network Congestion | When the network path taken by the UDP packets is overloaded with traffic, it might become incapable of handling additional packets, causing them to be dropped. The higher the network traffic, the more likely it is for UDP packets to be lost. |
Poor Transmission Quality | Lower-quality transmissions, such as those over wireless or long-distance networks, are more prone to errors. Environmental interference and distance from the source can cause UDP packets to be lost during transmission. |
System Resource Limitations | If the receiving system is occupied with other high-resource tasks, it may drop UDP packets if it can’t process them quickly enough. |
from scapy.all import * packet = IP(dst="destination_ip") / UDP() sent = sr1(packet) if sent == None: print("Packet lost") else: print("Packet received")
There are a variety of reasons why UDP packets might get lost, primarily due to the very nature of User Datagram Protocol (UDP) itself.
UDP is a connectionless protocol. This means it doesn’t ensure a reliable communication link between the sender and receiver and thus does not guarantee the delivery of packets. With this in mind, let’s delve into how often these UDP packets are lost.
Causes of UDP Packet Loss
Cause | Description |
---|---|
Unreliable Network Infrastructure | Packet loss often occurs due to issues with the underlying network infrastructure. It could be because of faulty hardware, bad network cables, or outdated networking equipment that cannot keep up with current data traffic load. |
Network Congestion | This happens when too many users are accessing the same network resources concurrently. Data traffic cannot be handled optimally, leading to packet delay or total loss of packets during transmission. |
Buff Overflow at Receiver Side | If the receiving application is slow to process incoming packets, they may amass in the buffer until it gets full. Once it exceeds capacity, further incoming packets will be dropped, thus causing packet loss. |
How Often Do UDP Packets Get Lost?
The frequency of UDP packet loss is highly dependent on the stability of the network and its overall functionality and can greatly vary from one situation to another.
While there is no definitive rate given the variability of factors influencing packet loss, researches like “Loss Patterns of Real-Time Video Over the Internet” by Bolot et al. delineate that losses often occur in bursts rather than randomly. In high load conditions, packets were observed to have a loss rate of as much as 20%.
Remember, these values fluctuate depending on various uncontrollable external factors such as:
- The state of your network: If the network is congested with heavy data traffic or the physical infrastructure is not well-maintained, it can lead to higher packet loss rates.
- The capability of your system to receive and process packets: A slower system with limited buffer space could result in an overflow, meaning more packets will end up being discarded.
With these caveats, it’s clear that maintaining healthy network infrastructure, proper system settings for receiving packets, and efficient management of data traffic can significantly minimize UDP packet loss.
# An example of handling UDP in Python import socket def send_udp_packet(host, port, message): try: udp_socket = socket.socket(socket.AF_INET, socket.SOCK_DGRAM) udp_socket.sendto(message.encode(),(host, port)) print(f"Sending message '{message}' to {host}:{port}") except Exception as e: print("Error:", e) finally: udp_socket.close() send_udp_packet('localhost', 12345, 'Hello UDP')
In this snippet, we create a socket object in Python using
socket.socket()
. The
socket.AF_INET
parameter specifies the address family for IPv4. The
socket.SOCK_DGRAM
parameter specifies the type of socket (UDP in this case). The
sendto()
function transmits the message through the specified host and port. Keep in mind, this approach lacks any form of error checking or retransmission mechanism if there is packet loss.
References:
- UDP and TCP Traffic Classification and Prioritization
- Loss Patterns of Real-Time Video Over the Internet
When exploring the frequency of loss of UDP packets, it is imperative to understand several factors that may contribute to these instances. One of the most fascinating aspects of the User Datagram Protocol (UDP), which is a part of the Internet Protocol suite used to transmit short messages known as datagrams, but doesn’t guarantee delivery, order of arrival, or integrity of data, like its counterpart Transmission Control Protocol (TCP) offers.
Firstly, bear in mind that UDP packets are particularly susceptible to packet loss. Packet loss happens when one or more packets of information traveling across a computer network don’t reach their destination. This could be due to various reasons:
Factor | Description |
---|---|
Network congestion | This occurs when the load on the network—also known as the amount of traffic—is greater than the network’s capacity. |
Insufficient hardware | If the networking hardware such as routers and switches are not capable enough to handle the network traffic, packets can get lost. |
Software bugs | Bugs in the network software—particularly errors in discarding redundant packets or misidentifying their sequence—can also prompt packet loss. |
Changes in the network topology | If there are changes in the physical layout or configuration of the network without updating related adjustments, UDP packets can easily get lost in transit. |
Security Threats | Packets can be maliciously captured, altered, or rerouted by attackers, causing loss of UDP packets. |
However, with a
ping
command, you can immediately diagnose whether you’re experiencing packet loss.
For example:
$ ping -c 5 www.google.com
Here,
-c 5
instructs the program to send 5 ping requests to www.google.com.
In terms of how often UDP packets get lost, it hugely depends on the quality of your network or internet connection. For reliable networks, the loss may be relatively rare; However, for unstable or congested networks, TCP would be better suited as it provides mechanisms such as error detection and correction methods that ensure data integrity.
Understanding both the influential factors and different protocols’ strengths and weaknesses can lead to more knowledgeable decisions regarding the choices and maintenance of your network environment. You can reduce the chance of loss by frequently monitoring your system, upgrading networking equipment as needed, patching software regularly, securing your network, and addressing potential issues early before they escalate. Check out this comprehensive guide about how UDP and IP are used in LTE E2E data transmission to add context and depth to your understanding of these principles.As a professional coder, my job is to demystify complex topics and one of these issues revolves around network congestion and how it contributes to the loss of UDP (User Datagram Protocol) packets. The crux of this subject matter pertains to the frequency of UDP packet loss.
Network congestion happens when a network node or link carries data that exceeds its transmission capacity. This bottleneck can lead to packet loss or delay of packets. Consequently, in scenarios where network traffic is overwhelming, the likelihood of losing UDP packet elevates.
UDP, unlike its TCP (Transmission Control Protocol) counterpart, doesn’t involve any form of acknowledgement for received packets. Its “fire-and-forget” nature means it doesn’t ensure reliable delivery of packets. If any packet stands lost during this process, UDP neither resends the packet nor recovers it from loss. This makes it particularly susceptible to drops during periods of high network congestion.
To paint this into context, look at this code segment representing a simple UDP communication:
import socket UDP_IP = "127.0.0.1" UDP_PORT = 5005 MESSAGE = "Hello, World!" sock = socket.socket(socket.AF_INET, socket.SOCK_DGRAM) sock.sendto(MESSAGE, (UDP_IP, UDP_PORT))
In the above Python code snippet, it’s clear there’s no mechanism for guaranteeing packet arrival. The program simply sends a UDP message without an assurance of delivery.
Estimating how often UDP packets get lost is a multi-faceted task. Varied factors come into play such as:
– Network congestion
– Available bandwidth
– Link quality
– Network hardware
– And more
A study titled “Characterizing Residential Broadband Networks” published on IEEE demonstrated that typical packet loss rates vary widely ranging from less than 1% up to 20% due to these aforementioned network conditions.
Packet loss in some networks may be too much uneventful, especially if it’s low-volume, non-critical traffic. However, for applications requiring real-time data like video streaming or online games, even a small proportion of packet loss could significantly tarnish the user experience. For instance, losing one-in-ten of all audio packets in Voice over IP (VoIP) might render your call unintelligible.
UDP Usage | Consequence of Packet Loss |
---|---|
Video Streaming | Lower resolution, pixellation |
Online Gaming | Lagging, jarring motion |
VoIP | Poor audio quality, dropped calls |
Adopting a Quality of Service (QoS) protocol could help prioritize certain types of traffic, which decreases the chance of packet loss for critical applications that use UDP. Nevertheless, it’s important for users and network engineers to appreciate that UDP throughput isn’t always guaranteed and measures should be taken to mitigate packet loss in environments where data needs to be reliably transferred.Analyzing the frequency of lost User Datagram Protocol (UDP) packets provides intriguing insights about network performance and reliability. An inherent nature of UDP is its connectionless state, making it susceptible to packet loss. This attribute is especially significant in environments where network traffic is high or bandwidth is unreliable.
import socket server_address = ('localhost', 8080) sock = socket.socket(socket.AF_INET, socket.SOCK_DGRAM) data = b'Test data' sent = sock.sendto(data, server_address) # Check for lost packets try: data, server = sock.recvfrom(4096) except socket.error: print('Packet Lost') finally: sock.close()
The Python script given above illustrates a basic way of checking UDP packet transmission. However, it only implements a rudimentary form of error checking. A more robust application would count the number of packets sent versus received to get a percentage of lost packets.
On a broad spectrum, several factors influence how often UDP packets get lost, which include:
– Network congestion: If the network is saturated with data traffic, packets may drop regularly.
– Wireless connections: These are particularly unstable, leading to increased packet loss compared to wired connections.
– Faulty hardware or software: Damaged routers or switches or improper configuration might lead to consistent packet dropping.
– Distance: Transmission over long distances without proper routing infrastructure can cause packets to get lost frequently due to latency in signal propagation.
It’s worth noting that the loss frequency varies drastically based on these conditions – it could range from minimal (less than 1%) to significant (over 30%) losses under extreme circumstances. Therefore, continuous monitoring and frequent analysis are highly advised to ensure optimal network performance.[source]
Likewise, understanding how losing UDP packets impacts applications helps make informed decisions about when to use UDP over TCP. For instance, Real-time streaming content, online games, or Voice over IP (VoIP) make use of UDP despite its potential for lost packets, because it has lower overheads and latency as compared to TCP.[source] But in these cases, some packets getting lost is preferable than waiting for packets to be re-transmitted as it would delay the real-time services thus lowering the user experience.
Below is an illustrative table summarizing the effects of losing UDP packets on different types of applications.
Application | Average UDP Packet Loss | Impact of Packet Loss |
---|---|---|
Online Gaming | 2% – 5% | Minor gameplay glitches |
Voice over IP | 1% – 3% | Slight voice lag or distortion |
Video Streaming | 2% – 15% | In low bitrates video Frames Especially, pixelated scenes or frozen frames |
Thus, while each lost UDP packet indeed indicates an imperfection in the network, they’re often a trade-off we accept for other benefits such as lower latency or bandwidth optimization. Like most things in tech, it usually boils down to picking the right tool for the job.Definitely. When we talk about packet loss in the context of network communications, we’re referring to instances during data transfer where packets fail to reach their intended destination. The User Datagram Protocol (UDP) is especially known for experiencing certain levels of packet loss given that it’s a connectionless protocol commonly used in time-sensitive applications, such as video streaming or online gaming.
For coding and understanding purposes, here’s how you can create a simple UDP server with Python:
import socket localIP = "127.0.0.1" localPort = 20001 bufferSize = 1024 UDPServerSocket = socket.socket(family=socket.AF_INET, type=socket.SOCK_DGRAM) UDPServerSocket.bind((localIP, localPort)) while(True): bytesAddressPair = UDPServerSocket.recvfrom(bufferSize) message = bytesAddressPair[0] address = bytesAddressPair[1] print("Message from Client: ", message)
Given this, weather conditions may actually contribute to an increase in UDP packet loss. The physics behind this phenomenon lies within the impact climate changes have on physical infrastructure that supports data transmissions, particularly when dealing with wireless connections such as Wi-Fi or Cellular Data (source).
Aspects influenced by weather impacting UDP Packet loss can be categorized as follows:
– Rain and Snow: These weather conditions greatly affect microwave radiation transmission, which is used by some data communication protocols, including satellite Internet connection, leading to a potential increase in lost UDP packets.
– Wind: High winds may cause physical damage to outdoor networking gear; for instance, wind can move directed antennas from their distanced alignment and cause packet loss.
– Temperature: Tech devices are susceptible to extreme temperatures. Network equipment, like other electronics, can overheat or freeze, causing inconsistent performance and packet loss.
– Sunlight: Intense sunlight can cause heat spikes and interfere with the normal functionality of networking equipment, indirectly leading to packet breakdown.
However, keep in mind that while weather conditions do affect UDP packet loss, they may not necessarily always have a significant enough impact for the end-user to notice. Most modern systems and networks are designed to provide built-in resilience against problems like packet loss with error correction methods and redundancies.
So, how often do UDP packets get lost? Well, there is no definitive answer to this query due to its sensitivity to a plethora of variables, including network quality, congestion, distance between hosts, and naturally, weather conditions. In an ideal/non-congested network, one could observe minimal UDP packet loss. However, real-life conditions are rarely ideal, thus resulting in intermittent packet losses. Maintaining high-quality equipment, optimizing network layout, employing redundancy measures, and keeping networking software up to date could all assist in minimizing occurrences of UDP packet loss.The link quality factor refers to the measure of signal strength and signal-to-noise ratio (SNR) in a network. With reference to User Datagram Protocol (UDP) packets, it plays a critical role in dictating their loss or safe transmission across the network.
UDP, by design, is a connectionless protocol, implying that it essentially skirts around techniques such as congestion control and error recovery, unlike its ‘elder brother’ – Transmission Control Protocol (TCP). Consequently, UDP packets are significantly more prone to getting lost during transit.
What primarily triggers packet loss? Well, for UDP, these could be:
– Network congestion: When a network becomes overloaded with traffic, packets often tend to get lost.
– Poor link quality: The scenario we’re presently discussing, poor signal strength can easily result in packet loss.
– Hardware failures: If there’s an issue with the router, switch, or any other device on the network this could lead to packet loss too.
How often do UDP packets get lost? This boils down to the quality factor of the link in place. Now, you might find yourself questioning, “What exactly measures Link Quality?” Here are the key contributors:
– Signal Strength: How strong is the signal reaching your device? Low signal strength means high chances of information getting damaged along its path and eventually leading to packet loss.
– SNR (Signal to Noise Ratio): It defines the ratio between a signal power and the background noise level. An elevated SNR translates to a better link and thus fewer lost UDP packets.
Now let’s assume we have good signal strength but what if the noise level is also high? Even then, your valuable UDP Packets will get lost. Thus, even though increasing signal strength is essential, simultaneously curbing noise level is equally imperative.
Let’s see a python script that uses the scapy library to send a UDP packet.
from scapy.all import * data = "Hello, World!" a = IP(dst='192.168.1.2')/UDP(dport=12345)/data send(a)
In this example, we are creating a simple packet with the string “Hello, World!” inside it and sending it to IP address ‘192.168.1.2’. However, due to the above-mentioned contributors, the delivery of this packet may fail, causing it to get lost.
To combat the packet losses in UDP, what one can really do? Strategies like sending supplementary redundant packets, introducing application-level acknowledgments, and retransmissions can be of assistance. One of the popular user-space solutions is UDT (UDP-based Data Transfer Protocol). It provides reliability controls and congestion control, thereby increasing the efficiency in the transfer of large amounts of data over high-speed wide area networks.
In conclusion, ‘How often UDP packets get lost?’ is an entity subjected vastly to the quality factor of the used link but it can be mitigated with appropriate strategies and checks in place.Without doubt, the delivery of packages is an essential aspect that impacts the efficiency of any network communication. UDP (User Datagram Protocol), known for its connectionless nature and simplicity, plays a crucial role in this. However, UDP doesn’t guarantee the successful delivery of packets to the destination. Instances of lost packets are commonly witnessed when using UDP, but how frequent are these instances?
Truly, the frequency of lost UDP packets is influenced by several factors. Among all, software bugs stand out as one influential element. It’s imperative to understand this relationship as a step towards enhancing your overall coding and network management practices.
Software Bugs Impact on Lost UDP Packages
At its core, a software bug is a glitch or fault in a computing program, causing it to yield unexpected responses or behaviors. When such bugs infiltrate UDP-based applications, they may spur packet loss patterns, either by generating erroneous data streams or mishandling UDP sockets.
Erroneous Data Streams: A software bug could lead to the generation of massive, irrelevant data transmitted via UDP. This could cause traffic congestion, eventually leading to overloading of routers and discarding (loss) of some packets.
Mishandling UDP Sockets: A key aspect of writing UDP programs involves the proper handling of network interfaces, ports, and connections using sockets. Software bugs might impair socket operations, leading to non-receipt of packets at their destined endpoints.
//Incorrect usage of UDP Socket, can cause packet loss public class UDPServer { public static void main(String args[]){ DatagramSocket aSocket = null; try{ aSocket = new DatagramSocket(6789); byte[] buffer = new byte[1000]; //Continuous listening - bug present! while(true) { DatagramPacket request = new DatagramPacket(buffer, buffer.length); aSocket.receive(request); //No break condition - potential packet loss due to overflow! } } catch (SocketException e){ System.out.println("Socket: " + e.getMessage()); } catch (IOException e){ System.out.println("IO: " + e.getMessage()); } finally { if(aSocket != null) aSocket.close(); } } }
Source code above demonstrate how incorrect usage of UDP socket can lead to packet loss. The server is designed to continuously listen to incoming packets without a pause or break. If the rate of incoming packets exceeds the processing speed of the application, some packets might be lost.
Such software errors may trigger occasional or frequent packet losses; the frequency majorly depends on the extent of the bugs and how they distort the relevant software functions. Additionally, environmental factors like network congestion, latencies, equipment failures, and network settings can also contribute to packet loss frequencies.
Embracing strategies like regular patching, meticulous code writing and review, use of error detection and debugging tools like IntelliJ IDEA, SonarQube among others would significantly minimize the risks of introducing bugs hence lessening UDP packets losses. After all, reducing the extent and impact of these abnormalities is beneficial to enhancing the integrity, reliability, and performance of our networking affairs.
While mitigating packet loss entirely is nearly impossible given the design and workings of UDP, understanding how software bugs contribute to such losses would pave way to cleaner codes, well-optimized applications, and fewer packet loss incidents. Utilizing professional network monitoring and troubleshooting tools can offer real-time insights about packet losses, aiding developers and network managers optimize their UDP-based applications better.Faulty networking hardware can often result in data packet loss. This is because a substantial proportion of the data transmitted over networks utilizes the User Datagram Protocol (UDP). UDP stores messages in packets that it sends individually, potentially using different routes across a network to their destination. If any part of this network is compromised – for example, by faulty networking hardware – these packets may be lost.
Understanding Packet Loss in Networking
Packet loss refers to cases where one or more packets of data traveling across a computer network fail to reach their destination. This can occur when the network is overloaded with traffic, interference from external sources happen, or quite commonly due to faulty networking hardware. Packets get discarded when devices along the way become overwhelmed and cannot accommodate all ingress traffic.
What is UDP?
Unlike TCP (Transmission Control Protocol), UDP User Datagram Protocol does not provide acknowledgment of packets received or retransmission of lost packets. It’s a connectionless protocol used in applications that require efficient communication, sacrificing reliability. Examples of such applications include online multiplayer games, streaming videos, or VoIP calls. In these scenarios, if all parts of a message don’t arrive, it’s generally better to skip a packet and move on to the next rather than waiting for a resend, thus avoiding lags.
Does Faulty Hardware Lead to UDP PACKET Loss?
Faulty networking hardware like routers, switches, or modems can cause packets to get lost. Corrupted software, outdated firmware, or physical damage are some common factors impacting network gadgets that lead to packet loss.
Influence of Faulty Hardware on Data Transmission
Hardware Not Faulty | Hardware is Faulty | |
TCP | Packets almost always reach their destination thanks to TCP’s built-in error checking and correction. | Lost packets get re-transmitted after the receipt acknowledgment fails. Resulting in slower transmission rates. |
UDP | Faster transmission because no overhead error-checking and lost packets aren’t resent. | Since UDP lacks an acknowledgment mechanism, once the packets are dropped, they’re gone. Resulting in unrecoverable information loss. |
Examining Your Network for Faulty Hardware
To determine whether your network’s hardware is causing packet loss, you could investigate the following indicators:
• Frequent disconnects or slow network speeds might suggest an issue with your modem or router.
• If certain areas in your home or office have poor connectivity, your network may lack the necessary range and you might need to consider mesh or add a WiFi extender.
• Updates failing to install, signaling network interface card is malfunctioning.
• Cable wear and tear – ensure to check Ethernet cables regularly as physical damage can cause network issues.
Debugging Packet Loss
Running a ping test offers insights into potential packet loss. The command `ping` followed by a web address or IP sends a series of test packets out to the specified address.
$ ping google.com
Returned data will show if any packets failed to make the return trip, representing the extent of packet loss.
Unfortunately, if UDP packets do get lost as a consequence of faulty networking hardware, there’s not much that can be done to recover them as UDP doesn’t support packet retransmission. The solution is to troubleshoot and replace any faulty hardware to prevent future packet loss.
Remember, your network’s performance is heavily reliant on the condition of its hardware; maintaining the integrity of every piece ensures reliable data transfer. Regular check-ups, prompt updates, and replacing damaged parts will nip potential packet loss issues right in the bud!A buffer overflow, in the context of networking, refers to an instance where more data is put into a fixed-length buffer than it can handle. Specifically when working with User Datagram Protocol (UDP), this can contribute significantly towards packet loss.
To illustrate how this happens, here’s an HTML-friendly step-by-step breakdown of the process:
– When data packets arrive faster than they can be processed: Too much data vying for a limited amount of space often sends some data into oblivion. This situation primarily develops when UDP traffic rate exceeds the receiver’s processing capacity.
– When excess data overflows the allocated buffer: The exceeding data isn’t stacked somewhere else or given a ticket to wait its turn. Instead, an overflow causes the excess data to swamp and overwrite other information in adjacent memory locations, thus contributing to missing UDP packets.
– When subsequent packet loss leads to unrecoverable data: Given that UDP doesn’t have a built-in mechanism for guaranteeing delivery, if packets are dropped or lost along the way due to buffer overflow, that information is permanently gone. Unlike TCP, UDP doesn’t have a recovery protocol for retrieving lost or corrupted data. Consequently, buffering issues can degrade the overall performance of UDP applications.
Let’s delve into a simplified code snippet to conceptualize how a buffer overflow might occur:
char buffer[500]; packet = recvfrom(sockfd, buffer, 800, flags, src_addr, addrlen);
In this simplistic scenario, we’ve created a fixed-size array ‘buffer’ to store data from incoming UDP packets. If a larger UDP packet (in bytes) tries to fit into smaller allocated buffer, it’ll result in a buffer overflow and potential packet loss.
Now addressing the question: How often do UDP packets get lots? It heavily depends on multiple factors:
– Network Congestion: Severe network traffic congestion increases the likelihood of these instances happening since buffering queues rapidly fill up during peak times, leading more frequently to overflow situations.
– Buffer Size: The size of the queue buffer also influences this frequency. A smaller buffer filling up quicker results in a higher chance of overflowing, hence a greater probability of losing UDP packets.
– Application Behavior: Some applications could possibly be designed not to handle data as expediently as it arrives. In such scenarios, even if the network communication operates perfectly and no congestion exists, data can still go missing merely because it wasn’t collected quickly enough from the buffer by the application.
As you can see, buffer overflow does indeed directly contribute to missing UDP packets, which is contingent upon diverse circumstantial parameters. Overall optimization of programs and appropriate allocation of buffer sizes should substantively reduce this occurrence, enhancing reliable data transmission via UDP. For more intricate details regarding buffer overflow, consult references [such as this](https://owasp.org/www-community/vulnerabilities/Buffer_Overflow).
The key takeaway here is designing our applications with thoughtful consideration for handling incoming data intelligently. Adopting techniques like dynamic resizing of buffers or offloading data for later processing rather than letting it stagnate in the buffer could help mitigate buffer overflow—playing a significant role in minimizing instances of UDP packet loss.
When dealing with the topic of unreliable connection points leading to package drops, it’s critical to tackle this in relation to UDP packets. UDP, or User Datagram Protocol, does not offer an in-built recovery system for lost packets. Therefore, getting a grasp on how often UDP packets get lost and how we can address these issues becomes essential.
First off, let’s quickly differentiate between TCP (Transmission Control Protocol) and UDP (User Datagram Protocol). Both of these are communication protocols used for sending data over the internet, but they operate in different ways.
– TCP is like a phone call – establishing a connection first, ensuring the recipient is there to receive the data before proceeding.
– UDP, however, works more like mailing a letter – sending data without prior ‘handshake’, hence no guaranteed delivery.1
Since UDP doesn’t guarantee delivery of every packet, packet losses might be prevalent. So, how often do UDP packets get lost?
It’s tricky to put an exact figure on it because packet loss greatly depends on network conditions, congestion, hardware performance, among other variables. In stable networks, the typical packet loss rate can be as low as 1% or even less. However, in congested or poor network conditions, the percentage can climb significantly higher.2
To detect and possibly recover lost UDP packets, here’s where coding steps in. Strategic programming measures might include:
1. Implement a sequence number system for UDP packets: With this, you’ll be able to order packets correctly and identify if any are missing. This involves assigning each packet a unique sequential number upon transmission. The receiver end then checks this sequence to determine if any packets got lost during transit.
// Pseudo code for sequence numbering class UdpPacket { int sequenceNumber; ... // Other packet properties }
2. Use acknowledgments (ACKs) and time-outs: If you design your application to wait for an acknowledgment before sending the next packet, it will know whether the previous packet was delivered successfully.3
// Pseudo code to show ACK usage function sendPacket(packet) { ... // Code to send packet wait for ACK or timeout if (ACK received) { send next packet } else { resend current packet } }
3. Lastly, ensure error checking mechanisms are incorporated: Mechanisms such as Cyclic Redundancy Check (CRC) or checksum can help detect data corruption which might have resulted from the associated packet loss.
// Pseudo code demonstrating CRC usage class UdpPacket { int crcValue; ... // Other packet properties boolean checkIntegrity() { return calculateCrc(data) == crcValue; } }
A key point to remember is that while these techniques can help manage lost packets, they cannot completely eliminate packet loss. They can also make your UDP-based applications heavier, reducing the speed advantage of UDP over TCP. Hence, choosing between UDP and TCP should be considered on a case-by-case basis, depending on the specific needs of your software functionality.
Lastly, it’s worth mentioning that some smaller-scale platforms may use specialized protocols like RTP (Real-Time Transport Protocol) or QUIC (Quick UDP Internet Connections), which combine the best features of TCP and UDP, providing both speed and reliability.4
References:
Platform Link | Explanation |
---|---|
TCP vs UDP: Understanding the Difference | Understanding the difference between TCP and UDP. |
Network Engineering StackExchange post about packet loss | Different views on packet loss rates in typical networks. |
Acknowledgment strategy in Selective Repeat Protocol | Explains how ACK method works. |
What is the Quick UDP Internet Connections (QUIC) protocol? | An overview of the QUIC protocol which combines the benefits of TCP and UDP. |
Certainly, I’d be happy to explain how packet sniffers can influence the loss of UDP packets data.
Let’s begin with understanding what UDP and Packet Sniffers are:
User Datagram Protocol (UDP)
is an Internet Protocol which allows computer applications to send messages, in this case referred to as datagrams, to other hosts on an Internet Protocol (IP) network (Wikipedia).
A
packet sniffer
, also known as a network analyzer or protocol analyzer, is software that can intercept and log traffic on a digital network. The danger with such tools is they have legitimate uses but can also be used inappropriately for malicious purposes.
How do packet sniffers relate to the loss of UDP packets?
Networks are known to be noisy places. Packets collide, get routed inefficiently, vanish, or are purposely ignored. Even despite this, most data gets to where it needs to go. This seeming magic is predominantly thanks to TCP/IP’s corruption recognition checks and transmission control system.
However, UDP lacks these sophisticated mechanisms to ensure reliable delivery. So when a packet sniffer enters the scene, it further exacerbates the situation by possessing the ability to trigger unintended packet losses. Here’s how this happens:
– Overloading the Network: When sniffing on a busy network, the added load can consume enough extra bandwidth or cause enough additional collisions to result in packet losses elsewhere.
– Missing Packets: A passive sniffer, particularly one running on a machine doing heavy network input/output (I/O), can miss packets. Essentially, incoming packets may be lost if the sniffer cannot process them quickly enough.
– Usage of Promiscuous Mode: Some sniffers operate in ‘promiscuous mode’, allowing them to see all traffic on a LAN segment. However, receiving a glut of unexpected packets can trigger excessive processing or resource exhaustion leading to packet loss.
For instance, here’s an interesting source code example illustrating the use of a simple python packet sniffer using raw sockets:
import socket s = socket.socket(socket.AF_INET, socket.SOCK_RAW, socket.IPPROTO_IP) s.bind(("YOUR_INTERFACE_IP", 0)) s.setsockopt(socket.IPPROTO_IP, socket.IP_HDRINCL, 1) s.ioctl(socket.SIO_RCVALL, socket.RCVALL_ON) while True: print(s.recvfrom(65565))
In summary, UDP’s lack of guaranteed delivery coupled with the potential impacts presented by a packet sniffer increases the likelihood of UDP packet loss. Although some packet drop is inevitable in some environments, controlling and monitoring your network traffic is crucial for maintaining good performance. Recommended steps include always securing your network, monitoring for unusual activities, and considering the use of more efficient protocols, like TCP, where appropriate.The fundamental difference between TCP (Transmission Control Protocol) and UDP (User Datagram Protocol) stems from their inherent design methodologies, with TCP being a connection-oriented protocol and UDP being a connectionless one.
Let’s delve deeper into these protocols, especially focusing on the reliability aspect and how often UDP packets get lost.
TCP |UDP | Reliable, as it |Unreliable, as it doesn't provide ensures packet delivery |packet delivery guarantee. | Includes error-checking mechanism |Doesn't include an intrinsic for data integrity, and re-sends |error-checking system. Hence, corrupted or lost datagrams. |more prone to packet loss. | Includes flow control to prevent |Doesn't include flow control which network congestion. |can lead to network congestion and |subsequent packet loss.
Now let’s discuss why UDP can experience packet loss and how often it happens.
1. As mentioned before, UDP is a connectionless protocol. It means it does not need to create and maintain a dedicated end-to-end connection like TCP. It sends datagrams to the destination without ensuring whether the recipient is ready to receive them or not, or even if the packets will reach at all. Because of this nature, UDP packets can get lost more frequently, especially in situations where network conditions are less than ideal.
2. Unlike TCP, UDP does not have an inbuilt mechanism to acknowledge receipt of data packets. TCP users would receive acknowledgments of packet receipt. If they don’t, TCP assumes that the packet was lost and sends it again. This mechanism significantly contributes to TCP’s reliability. In contrast, since UDP does not have an inherent acknowledgment system, it might not recognize when a packet gets lost, therefore not resend the packet.
3. Network congestion is another contributing factor for packet loss. With TCP, an inbuilt flow control mechanism prevents sending too much data at once, easing up network congestion. However, UDP lacks this feature, leading to possible network congestion and subsequent packet loss if there is too much data sent over the network simultaneously.
It’s difficult to exactly pinpoint how often UDP packets get lost as it primarily depends upon the network conditions. In a high-speed, low-latency network, UDP packets can transmit efficiently with minimal loss. Still, in congested networks with low bandwidth and high latency, UDP packet loss could be significant.
Worth noting is that despite these discrepancies, UDP finds utility in applications that prioritize speed over data integrity, like live streaming, online gaming, etc., where occasional loss of some data packets doesn’t hugely spoil user-experience, but lags surely do.
For more in-depth information, you may want to explore references such as [Computer Networks by Andrew S. Tanenbaum](https://www.amazon.com/Computer-Networks-5th-Andrew-Tanenbaum/dp/0132126958/) or advanced networking coursework available online. While learning, keep in mind that no protocol is universally ‘better’ than the other. The choice largely depends on application requirements. Even TCP and UDP’s shortcomings contribute to their suitability for different use-cases, including the matter of packet loss.Digging deep into the phenomena, “How Often Do UDP Packets Get Lost,” really depends on a couple of key factors. Some variables at play include the quality of the network and the load it is under.
The
UDP
or User Datagram Protocol, by definition, does not guarantee that packets will be received in the order they were sent, or even that they will arrive at all. This inherent lossy nature partly explains why some UDP packets may get lost during transmission.
However, this doesn’t mean that every single
UDP
packet is doomed to disappear into the ether. When you send a UDP packet, there’s still an incredibly high chance it will reach its destination intact, unless the network is exceptionally congested or there are hardware issues.
If your application is running over a stable network, chances are, very few UDP packets will actually get lost. However, if the network is unstable or heavily burdened, packet loss can drastically increase.
Also, unlike its counterpart, TCP (Transmission Control Protocol), UDP does not have any form of error checking mechanism or handshake agreements to establish a connection before sending data. This means packets can get ‘lost’ in the sense that they might be delivered out of order; however, missing packets won’t cause delays as
UDP
doesn’t involve re-transmission of lost packets like TCP.
// Example of UDP server import java.net.*; public class UDPServer { public static void main(String args[]) throws Exception { DatagramSocket serverSocket = new DatagramSocket(9999); byte[] receiveData = new byte[1024]; byte[] sendData; while(true) { DatagramPacket receivePacket = new DatagramPacket(receiveData, receiveData.length); serverSocket.receive(receivePacket); // More code goes here } } }
In the example above, a crude representation of how UDP works is shown using Java. Notice here that regardless of whether the packet reaches its destination, the sending process is continued — this demonstrates the non-deterministic nature of UDP.
It’s also worth mentioning that how often UDP packets get lost can depend on the specifics of your algorithm. For example, if it needs to handle large volumes of data quickly, like live video streaming or online gaming, the occasional lost packet could theoretically go unnoticed but if accuracy is paramount, packet loss could pose a more significant issue.
While answering the question definitively would require in-depth network analytics and monitoring for packet delivery outcomes, my analysis provides a general sense of just how often UDP packets might get lost. Also, let me point out that despite potential packet loss, the advantages of a speedy delivery make UDP a preferred choice for real-time applications.
For further reference, you might want to review the details of the UDP protocol at IETF RFC 768. Understanding the underlying design of UDP can help give context to why packet delivery isn’t guaranteed and, consequently, why packet loss rates vary so much.