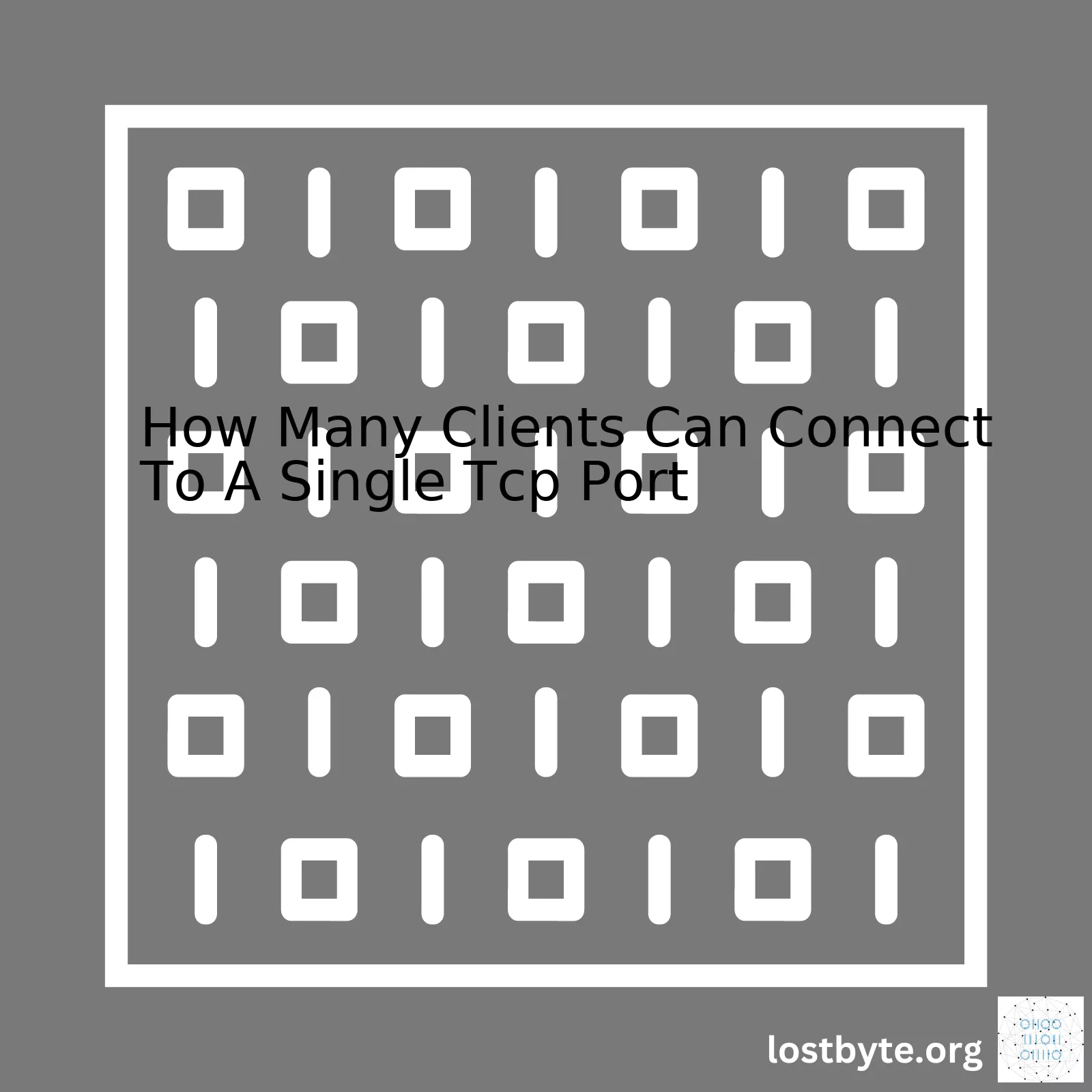
TCP Ports | Clients Possible |
---|---|
Single TCP Port | Theoretically unlimited. However, practical limits are imposed by client-side port availability and server resources. |
The Transmission Control Protocol (TCP) itself does not limit the number of clients that can be connected to a single TCP port. In fact, a TCP port, once opened by a server, can receive connections from an arbitrary number of clients.source
However, there are two primary considerations that limit the number of connections:
- Client-side Port Availability: Each client connection is recognized by a unique combination of source IP, source port, destination IP, and destination port, with the port numbers ranging from 0 to 65535. Since ports below 1024 are reserved, each client has around 61000 available ports for new connections. Hence, a single client can technically establish up to around 61000 simultaneous connections with a server.source
- Server Resource Constraints: Although theoretically unlimited, the actual number of clients that a server can handle simultaneously is limited by its available system resources (CPU, Memory, Network I/O). Serving too many clients at once may degrade the performance of the server or push it into resource exhaustion.source
Hence, while the answer depends on multiple variables like the client’s port availability, server’s resources, and the application’s nature, TCP does not impose any built-in limit to prevent multiple clients from connecting to a single port. The most optimal approach is to monitor the server continuously and upscale or downscale your server depending upon the usage. That way, you can make sure that the server can efficiently handle the incoming traffic.
Here is the simplified representation of the client-server networking model:
TCP Server:
server_sock = socket(AF_INET, SOCK_STREAM)
server_sock.bind((server_ip, server_port))
server_sock.listen(backlog)
while True:
client_sock, client_address = server_sock.accept()
TCP Client:
client_sock = socket(AF_INET, SOCK_STREAM)
client_sock.connect((server_ip, server_port))
In this code snippet, the server creates a socket, binds it to a specific IP address and port, and starts listening for incoming connection requests. On receiving a request, it spawns a new socket for every client. Concurrently, the client also creates a socket and sends a connection request to the server’s IP address and port.The Transmission Control Protocol (TCP) is a standardized Internet protocol that’s particularly important to developers. TCP helps establish and maintain network connections while transmitting information between clients and servers. As a professional coder, being knowledgeable about TCP can improve your ability to optimize network conductivity, troubleshoot issues, and provide secure solutions.
For the question of how many clients can connect to a single TCP port, it’s crucial to note that, technically, there isn’t any limit regarding the number of clients that one TCP port can handle. However, this doesn’t mean there will be no problems when you scale up. Numerous factors such as server resources, the Operating System (OS), and the TCP/IP stack itself could all limit the number of concurrent TCP connections significantly.
Factors impacting TCP connections
– Connection Limitations Imposed by the OS: The maximum number of TCP connections varies across different platforms, server resources, and OS configurations. For instance, Windows Pro supports a maximum of 16777216 (2^24) outbound TCP connections, whereas Linux’s limits are determined by certain parameters that could be adjusted when necessary.
– Server Resource Constraints: CPU power, memory allocation, and network bandwidth can all put practical constraints on the number of clients that one TCP port can handle. Each connection uses some amount of these resources, so the number of simultaneous connections a server can support would be limited by its resource availability.
– Port Exhaustion: Although the theoretical limit for TCP ports is quite high (65535 available ports), normal usage might run out of ports due to the TIME_WAIT state, which is a delay imposed before freeing up a TCP port after disconnection to ensure no data packets are left in the network.
To better analyze and manage these factors, professional coders often use tools like netstat or ss command in Unix/Linux or Process Explorer in Windows.
Just to illustrate, let’s consider a situation where we have reached the maximum number of TCP connections set by our OS. On a Linux system, you could check this by running:
xxxxxxxxxx
sysctl net.ipv4.ip_local_port_range
If the output shows you’ve reached your current limit and need to increase it, you can adjust those values for a larger range:
xxxxxxxxxx
echo '1024 61000' > /proc/sys/net/ipv4/ip_local_port_range
In summary:
– There’s no defined number of clients that can connect to a single TCP port; it’s based on various factors.
– The key determinants are the limitations imposed by the OS, server resources, and potential port exhaustion.
– Identifying and monitoring these factors can help sustain more TCP connections per port, but at a certain point, you’ll have to balance performance.
More about TCP and its configurations here.
In computer networking, a TCP port refers to an endpoint of communication in the TCP/IP protocol suite. The TCP (Transmission Control Protocol) is one of the main protocols in the IP (Internet Protocol) suite and ensures that the bytes sent from a server are properly delivered to a client without any missing parts.
A TCP Port can be visualized as a docking station where data arrives from the internet and is then sent to specific applications within your computer. Every application on a device has a unique port number used to communicate with it. For instance, web servers typically use port 80 for HTTP traffic or port 443 for HTTPS.
An interesting question to address here is how many clients can connect to a single TCP port? This question could have saved some headaches to anyone maintaining a server and trying to optimize the performance. In general, multiple clients can connect to a single TCP port simultaneously. However, they each need to initiate a unique connection using a different client-side port.
Here’s a bit of code to illustrate this process:
xxxxxxxxxx
import socket
# Create a TCP/IP socket
s = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
# Bind the socket to the port
server_address = ('localhost', 10000)
print('starting up on {} port {}'.format(*server_address))
s.bind(server_address)
# Listen for incoming connections
s.listen(1)
while True:
# Wait for a connection
In the above code, the server is bound to a specified port that listens for in-coming messages by calling the
xxxxxxxxxx
listen()
method. The number argument denotes the maximum number of queued connections which defaults to zero when unspecified.
Now, let’s talk numbers. Each TCP connection is uniquely defined by four variables termed a socket pair:
- Server IP Address
- Server Port Number
- Client IP Address
- Client Port Number
The practical limit of clients that can connect depends on how many outbound ports are available to the clients, since the server’s inbound port remains constant. As per the specification stated in RFC 793, there are 65,535 TCP Ports, excluding Port 0, which is reserved. Restrictions on the number of simultaneous incoming connections primarily depend on the operating system’s limitations rather than the protocol itself. When we consider ephemeral ports (the client-side temporary ports assigned automatically by the OS), the most common range typically includes around 16,384 ports.
To summarise—while theoretically a large number of clients can connect to a single server-side TCP port simultaneously, the actual limitations lie in terms of the operational parameters determined by factors such as the sockets implementation, available system resources, and ephemeral port range.
For detailed and further readings on TCP/IP networks and related subjects, you can refer to these online resources: TCP – Wikipedia, RFC 793 – Transmission Control Protocol Specification.Transmission Control Protocol (TCP) is a fantastic backbone of the internet, facilitating communication between client and server applications. TCP behavior is best understood by exploring how it sets up connections, aptly termed the TCP three-way handshake.
This starts with the initiating device sending a synchronized (SYN) message to the receiver, typically the server. This SYN message has an initial sequence number for reliable packet tracking.
Next, the recipient acknowledges this SYN message by returning an acknowledgement (ACK) along with its own SYN message – hence a SYN/ACK message. The ACK corresponds to the initiating device’s SYN initial sequence number plus one, confirming receipt. Similarly, the SYN portion from the server also contains an initial sequence number for the packets it will be transmitting.
Finally, the initiating device acknowledges the server’s SYN/ACK message, echoing back the SYN sequence number provided by the server incremented by one in an ACK message.
xxxxxxxxxx
Device A ------SYN-----> Device B
Device A <-----SYN/ACK--- Device B
Device A ------ACK-----> Device B
Now we have a stable connection and data exchange can proceed. Importantly, each end of the connection keeps track of its own send sequence and acknowledgment numbers, updating them as conversations progress to ensure proper delivery and sorted traffic. Consequently, lost packets can be retransmitted based on missing sequence numbers.
Speaking about multiple clients connecting to a single TCP port, the `SO_REUSEADDR` socket option permits more than one `socket()` to bind to the same IP address and port. However, this doesn’t mean many clients can concurrently have an active connection on the same exact source TCP port.
Multiple concurrent connections on the same server TCP port are allowed because each client connection to that server port will have its unique client-side TCP port. TCP connections are uniquely defined by 4 things: {client IP, client port, server IP, server port}.
When a `listen()` call is made on a server socket, it specifies how many pending client connection requests the OS will queue before refusing new ones but doesn’t cap how many connections the server can handle simultaneously.
The number of concurrent active connections a server can handle is primarily restricted by:
– Operating system limitations: Each active connection consumes a file descriptor, which is typically capped in Linux systems. Modifications are possible but should heed performance and security impacts.
– Server’s resource availability: Each connection may add processing load on the application, consume RAM, cause network bottlenecks etc., thus testing and tuning appropriate to the specific scenario is crucial.
75% of web servers use either Apache or Nginxsource. Both technically support hundreds of thousands of concurrent connections. However, in practice, the optimal configuration varies and heavily depends on the purpose of the server, content type, user behavior, and hardware capabilities.
In summation, a multitude of technical and practical factors determine how many clients can maintain an active connection to a single TCP port, rendering the question contingent on the specifics of any given situation. It’s a balance act of system architecture, application design, infrastructure resources, and network management. Ports are an integral part of client-server communication in computer networking. They serve as endpoint connections in the network transport system, allowing distinct network services or processes to share a single physical network connection simultaneously. This sharing is made possible with the help of the transport layer protocols like TCP (Transmission Control Protocol) and UDP (User Datagram Protocol).
For a server application, a particular port – usually a well-known number like 80 for HTTP or 443 for HTTPS – is set aside and “listened to” to facilitate communication between it and its clients. Each client that initiates communication with the server does so by connecting to this designated port.
Let’s talk about the question at hand: How many clients can connect to a single TCP port?
Technically, a vast multitude of client devices can establish individual connections with a single TCP server port. The crucial aspect that makes this possible is the concept of a socket. A socket is created whenever the client establishes a TCP connection to the server.
Here’s how it works:
A unique combination of the following constitutes a socket.
- Client IP address
- Client port number
- Server IP address
- Server listening port number
Each time a client reaches out to the server’s listening port, a new TCP connection is established with a unique client-side port and thus opening a new socket for that connection.
In a perfect world scenario where there are no restrictions on the total number of outgoing ports from all the client machines and also no limitation on the computing capability of the server, the theoretical maximum count of simultaneous sockets, and hence clients, would be:
Number Of Clients = (Total Client Machines) x (Max Outgoing Ports Per Machine)
However, real-world scenarios impose some limitations:
- Outgoing Port Limitations: The number of outgoing ports per client machine is limited to approximately 60,000 due to the effective range of usable ports.
- Server Capacity: The server should have enough resources to handle and maintain multiple open connections.
Examine the following Python example for a TCP server which handles multiple clients:
xxxxxxxxxx
import socket
server_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
server_socket.bind(('localhost', 12345))
server_socket.listen(5)
while True:
client_socket, addr = server_socket.accept()
print(f'Got Connection From {addr}')
message = 'Thank you for connecting'+ '\r\n'
client_socket.send(message.encode('ascii'))
client_socket.close()
This server listens on port 12345 and accepts connections from any client. Each connection is handled as its own socket.
Given these constraints, we see how numerous client devices can establish individual connections with a single server port. However, keep in mind that scaling and managing many thousands of connections simultaneously presents various engineering challenges related to the server’s computing resources and the network’s bandwidth.
As a coder, when designing systems for extensive client-server communication, you may need to employ strategies like load balancing or clustering servers or use advanced non-blocking I/O models depending upon your service requirements to ensure smooth performance and scalability.Certainly, to understand how many clients can connect to a single TCP port, first we need to grasp the working process of the Transmission Control Protocol (TCP). TCP is one of the main protocols in the Internet protocol suite. It’s essentially a set of rules for how device connections should be established, maintained and terminated across networks.
When a client wants to communicate with a server (an email server, for instance), it initiates a TCP connection or what’s commonly referred to as a TCP handshake. The client will send a request to the server on a specific port using the server’s IP address.
Here’s a basic code representation of establishing a connection (in Python) to help you visualize:
xxxxxxxxxx
import socket
s = socket.socket()
host = "localhost"
port = 8000
s.connect((host, port))
In this model, when a setup request has been accepted by the server, a dedicated communication route is created between the two endpoints – a unique combination of four parameters: ServerIP, ServerPort, ClientIP, ClientPort forms what’s called a socket. So even though multiple clients might connect to the same server port number, they’re distinctly identified by their unique ClientIP and ClientPort combination.
So now comes the answer to your question – How many clients can connect to a single TCP port?
Theoretically, there is no actual limit to the number of clients connected to one TCP port. The limit isn’t positioned on the part of the TCP protocol itself but due to other factors such as:
– System resource limits (like memory, CPU),
– Network bandwidth,
– OS-imposed limitations.
For a fun fact, since client connecting ports range from 1024 to 65535 (a total of 64511 ports), if all ports are used under ideal conditions, that means a single server can establish around 64K different connections per client IP. But remember, real-life performance highly depends on the factors mentioned above.
Finally, modern servers employ a bunch of techniques like load balancing, clustering or implementing high-level protocols like HTTP/2 to manage a large number of concurrent connections better.
Sources:
– [Transmission Control Protocol](https://en.wikipedia.org/wiki/Transmission_Control_Protocol)
The Transmission Control Protocol, or TCP, is a widely used protocol in networking for sending and receiving data. The maximum number of clients that can connect to a single TCP port on a server is influenced by various factors such as Operating System limits, hardware configuration and application design. This topic may seem technically complex, but I’m here to break it down for you.
Operating System Limits
The operating system plays a huge role in determining how many client connections on a single TCP port are possible. These limits typically involve the total number of file descriptors (sockets included) the OS allows per process/user.
In UNIX/Linux systems, you can check this limit using the following command:
xxxxxxxxxx
ulimit -n
For instance, if the output from the command above is 1024, this means only 1024 active connections are allowed. Note, however, that adjusting these limits often requires administrative privileges and it might pose potential security and stability risks(source).
Hardware Configuration
RAM and CPU also influence the number of clients that can connect to a single TCP port. For each connection, the server needs to store some state information and this consumes memory. If the server doesn’t have enough resources, additional client connections start getting denied. Also, handling multiple concurrent connections can put stress on the CPU too.
Application Design
The way the server application running on the host machine has been designed significantly affects the maximum number of client connections. For instance, if an application uses a synchronous style (one which waits for an operation to complete before proceeding with the next operation), it likely won’t be able to handle as many client connections as an application designed using an asynchronous or multi-threaded approach. Asynchronous applications can operate on multiple requests simultaneously, thereby improving the potential number of clients that can connect.
Let’s consider a common server-side technology: NodeJS. In Node, being single-threaded and event-driven, it allows high throughput via asynchronous programming, hence more concurrent connections.
xxxxxxxxxx
const http = require('http');
const requestHandler = (request, response) => {
console.log(request.url);
response.end('Hello Node.js Server!');
}
const server = http.createServer(requestHandler);
server.listen(3000, (err) => {
if (err) {
return console.log('something bad happened', err);
}
console.log(`server is listening on 3000`);
});
Ports Availability
The dynamic port range varies based on the platform. For example, the IANA suggests use of ports 49152 to 65535 for dynamic or private ports (source). So even while maintaining uniqueness of IPs and ports, theoretically speaking, a server can only maintain approximately 64K simultaneous connections with a client from a specific IP and destined to a particular port.
These were some key elements influencing how many clients can connect to a single TCP port. However, remember each case is unique and dependent on specific requirements and challenges.TCP, short for Transmission Control Protocol, is one of the main transport layer protocols utilized in the Internet Protocol Suite. When it comes to connections involving a single TCP port, there are a few aspects we need to dive into:
Concept of ‘Connection’
In TCP, a connection refers to a “four-tuple” that contains
– Source IP address
– Source port
– Destination IP address
– Destination port
A client connecting to a server doesn’t merely use a single port on the server but also uses a port on its own side – an ephemeral or temporary port that it randomly chooses.
Understanding the limit
Going by the ‘four-tuple’ concept, theoretically, every client can establish a new connection to the same server port as long as it uses a new source port for each new connection. Here’s the kicker: most operating systems typically allow 65535 ports per IP address (excluding reserved ones) which you can check using below
xxxxxxxxxx
command:
shell
sysctl net.ipv4.ip_local_port_range
On counting the TCP possible connections, with IPv4, there are circa ~281 trillion potential combinations allowed!
However, practically, the actual number might be smaller due to factors like:
– System resource constraints such as memory and CPU.
– Network bandwidth limitations.
– Settings in OS TCP/IP stack, for instance, Linux has certain kernel parameters that place limits on TCP connections.
Amping up the limit
To accommodate more connections, professionals often design their servers around concurrent connections. Strategies involve:
– Connection pooling at application level.
– Load balancing, redirecting incoming TCP connections to multiple backend servers.
– Scaling horizontally by adding more servers as demand increases.
For tweaking system level settings related to TCP connections, check this [DigitalOcean Tutorial](https://www.digitalocean.com/community/tutorials/how-to-tune-your-ssh-daemon-configuration-on-a-linux-vps) out.
Usage of ports
To illustrate how ports are used, consider this scenario where a browser makes simultaneous requests to a server.
xxxxxxxxxx
Let Server IP = s_ip, Server Port = s_port
Client IP = c_ip. The browser picks random Client Ports - c_port1, c_port2 for two requests:
For request 1:
Source -> (c_ip, c_port1)
Destination -> (s_ip, s_port)
For request 2:
Source -> (c_ip, c_port2)
Destination -> (s_ip, s_port)
The above snippet shows that, even though connections are to the same server port, they’re considered distinct because the client-side source ports differ.
Scenario | Source | Destination |
---|---|---|
Request 1 | (Client IP, Client Port 1) | (Server IP, Server Port) |
Request 2 | (Client IP, Client Port 2) | (Server IP, Server Port) |
Remember client-server architecture primarily aims to service multiple clients concurrently, often exploiting this very feature of TCP alongside addressing & porting, irrespective of underlying hardware & software capacity constraints.
To elaborate further, each connection to a TCP port requires a file descriptor on the host machine’s server. Operating systems typically have limits set around the number of file descriptors available. This effectively creates a cap on the number of connections that can be established, as every TCP connection needs its own separate file descriptor. So, even if the theoretical maximum is 2^16 (or 65,536) concurrent connections, you’re likely going to hit your OS limit before you reach this.
xxxxxxxxxx
//Here is an example of how you might increase limit in Unix-based systems:
$ ulimit -n 4096
The above line would set the file descriptor limit to 4096. Remember, it’s imperative to remain cautious while adjusting these numbers. Setting this limit too high can have adverse effects, such as consuming excessive resources.
Yet, let’s remember that the OS isn’t the only potential bottleneck. The application might also impose constraints or counters with thresholds. Software like Nginx or Apache have their limitations and settings which need adjustments too.
Nginx Configurations | Apache Configurations |
---|---|
xxxxxxxxxx 1 5 1 worker_processes auto; //This will scale the number of worker processes automatically. 2 events { 3 worker_connections 5120; //This will increase the number of connections. 4 } 5 |
xxxxxxxxxx 1 6 1 StartServers 2; //This will start with two server processes. 2 MinSpareThreads 25; MaxSpareThreads 75; //These will govern spare threads. 3 ThreadLimit 64; ThreadPerChild 250; //These dictate thread limits. 4 MaxClients 200; //This tops the number of simultaneous requests. 5 MaxRequestsPerChild 4000; //Limits requests during process's life. 6 |
Remember, both Nginx and Apache configurations can be located in /etc/nginx/nginx.conf and /etc/httpd/conf/httpd.conf paths respectively in most distributions of Linux.
Another crucial point to drill home here has to do with memory management. Simply increasing connections without scaling server memory accordingly will result in disaster. As connections rise, so does memory consumption. Verify adequate memory exists before cranking up those numbers! A tool like vnstat could prove essential for monitoring bandwidth usage and memory allocation.
I hope this detailed analysis was comprehensive enough to give a clear understanding on improving our server’s configuration to handle more clients connecting to a single TCP port. Now, get tweaking!
There’s a common misconception out there that one might run into trouble when trying to connect more than 65,536 clients to a single TCP port because of the limitation on the number of available ports. This isn’t entirely accurate. To clarify this point, we first need to understand what a TCP connection is made of.
A TCP connection is uniquely identified by four elements:
xxxxxxxxxx
<source IP:source port, destination IP:destination port>
With all these combinations available, you can theorize an absolute maximum of:
xxxxxxxxxx
{{2^16}^2 * {2^32}^2}
or
xxxxxxxxxx
{{4294967296}^2 * {65536}^2}
That’s an extremely large number of possible connections.
But there are practical limitations to this.
- One client machine: A single client machine will be limited by its own ephemeral port range. This typically restricts a single client machine to a maximum of
xxxxxxxxxx
11128,000 to 65,000
outbound connections per IP address.
- One server’s single listening port: From the server’s perspective, since it uses just one listening port, no such restriction applies, and the number of allowed inbound connections from multiple client machines can certainly exceed
xxxxxxxxxx
11165,535
.
The real bottleneck begins to show up not because of available TCP ports but due more to available system resources. Managing tens of thousands of open connections may consume significant memory and CPU time for the “housekeeping” tasks (managing connection state, buffers, etc.). That’s where strategies like load distribution across multiple servers come in.
To distribute the connections among several servers you can use a Reverse Proxy Server.
Here’s a simple approach using Nginx as a reverse proxy server to balance load across two backend servers:
xxxxxxxxxx
http {
upstream backend {
server backend1.example.com;
server backend2.example.com;
}
server {
listen 80;
location / {
proxy_pass http://backend;
}
}
}
In this example, incoming HTTP requests are distributed between
xxxxxxxxxx
backend1.example.com
and
xxxxxxxxxx
backend2.example.com
.
So, while you could theoretically push quite a large number of connections to a single server TCP port, the more effective strategy for managing high loads would be to distribute your connections across multiple servers using techniques like Reverse Proxy Servers or Load Balancers.
Optimizing client connections to your server becomes crucially important when dealing with a high number of concurrent users. You should note that a single TCP/IP socket connection is defined by duplicity, meaning it is determined by both the client IP/port and server IP/port. So, theoretically you can scale to have about 65k (actually, it’s 216-1=65535) open connections from a single IP address with unique ports for each connection.
TCP Connection: |
xxxxxxxxxx 1 1 1 (Client IP-address:port <--> Server IP-address:port) |
Best Practices Considered:
1. Re-use idle connections: It’s common sense but worth repeating – don’t create one connection per request. If you use HTTP/1.1 or higher, the TCP connection remains open by default after an HTTP request, which allows subsequent requests to re-use the existing connection. A connection pool should be used on the client-side for better control over this.
2. Use HTTP/2: HTTP/2 enables multiple requests and responses to be multiplexed over a single TCP connection that improves efficiency and throughput in applications. The switch to HTTP/2 may provide significant improvements in network usage and performance without application changes. Visit HTTP/2 Official Website for more information.
3. Utilize ‘Keep-Alive’: Keep-alive helps by reducing the need for new connections by keeping existing connections open for further requests.
xxxxxxxxxx
Connection: keep-alive
header tells both client and server to keep the underlying TCP/IP connection open for further requests/responses.
4. Tune the OS settings: Increase max file descriptor limit, maximum ephemeral (short-lived) port range, TCP FIN/TIME_WAIT, etc. Your operating system will put a hard limit on how many connections it can support, regardless of how much hardware you throw at it. Check your current limit using
xxxxxxxxxx
ulimit -n
and increase it if necessary.
5. Execution threads: Each connection requires a separate thread. More connections need more system threads, memory, and CPU utilization. To handle massive connections, consider techniques like Thread pool, Asynchronous I/O, I/O Multiplexing, etc.
6. Load balancing: Distribute the load among many servers by using a load balancer to route traffic. This solution spreads open connections across multiple servers, increasing the maximum simultaneous connections. Visit Amazon’s Elastic Load Balancing for comprehensive solutions.
In conclusion, it’s essential to understand that there are technical limitations to the number of clients that can connect to a single TCP port at once. Best practices are to ensure correct connection management, optimizing server resources, utilizing modern protocols & technologies, balancing loads and tuning system parameters accordingly.
Though there’s a common misunderstanding that only one client can connect to the server via a single TCP port, this is in fact not true. The beauty of TCP or Transmission Control Protocol lies in its ability to distinguish between connections using a combination of local IP, local port, foreign IP, and foreign port.
The magic happens within the concept of socket pairs. A TCP connection isn’t just tied to a single port on your machine to a single port on the remote machine. Instead, it encompasses a four-value set (
xxxxxxxxxx
srcIP:srcPort, dstIP:dstPort
). So, when you perceive it as a ‘client connecting to a port’, keep in mind that the client too is connecting from an IP and from a port.
You might wonder if we’d run into limitations with this method. Practically speaking, the limit relates to the resources available at hand rather than TCP port restrictions. However, we do need to consider the ephemeral port range, which varies depending upon the operating system. For example, in Windows, by default, it can be between 49152 and 65535. Thus, theoretically, even assuming we’re constrained by a single client IP, within this range we could have approximately 16,383 connections made to a server at a single source port.
To give you an idea of how code for setting up multiple clients may look like, see below:
In server – python example:
xxxxxxxxxx
import socket
serversocket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
serversocket.bind((socket.gethostname(), 80))
serversocket.listen(5) # become a server socket, maximum 5 connections
while True:
connection, address = serversocket.accept()
buf = connection.recv(64)
if len(buf) > 0:
print(buf)
In client – python example:
xxxxxxxxxx
import socket
clientsocket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
clientsocket.connect((socket.gethostname(), 80))
clientsocket.send(bytes('hello','UTF-8'))
Considering all these elements, it’s evident that congestion, data handling efficiency, server bottlenecks, and hardware limitations will potentially pose more significant challenges when dealing with large volumes of concurrent connections, beyond any constraints perceived due to TCP ports.
If you want to dig deeper, you can check out some insightful articles here and here.
Remember, optimal scaling and efficient resource allocation are key when dealing with high concurrency in your applications.