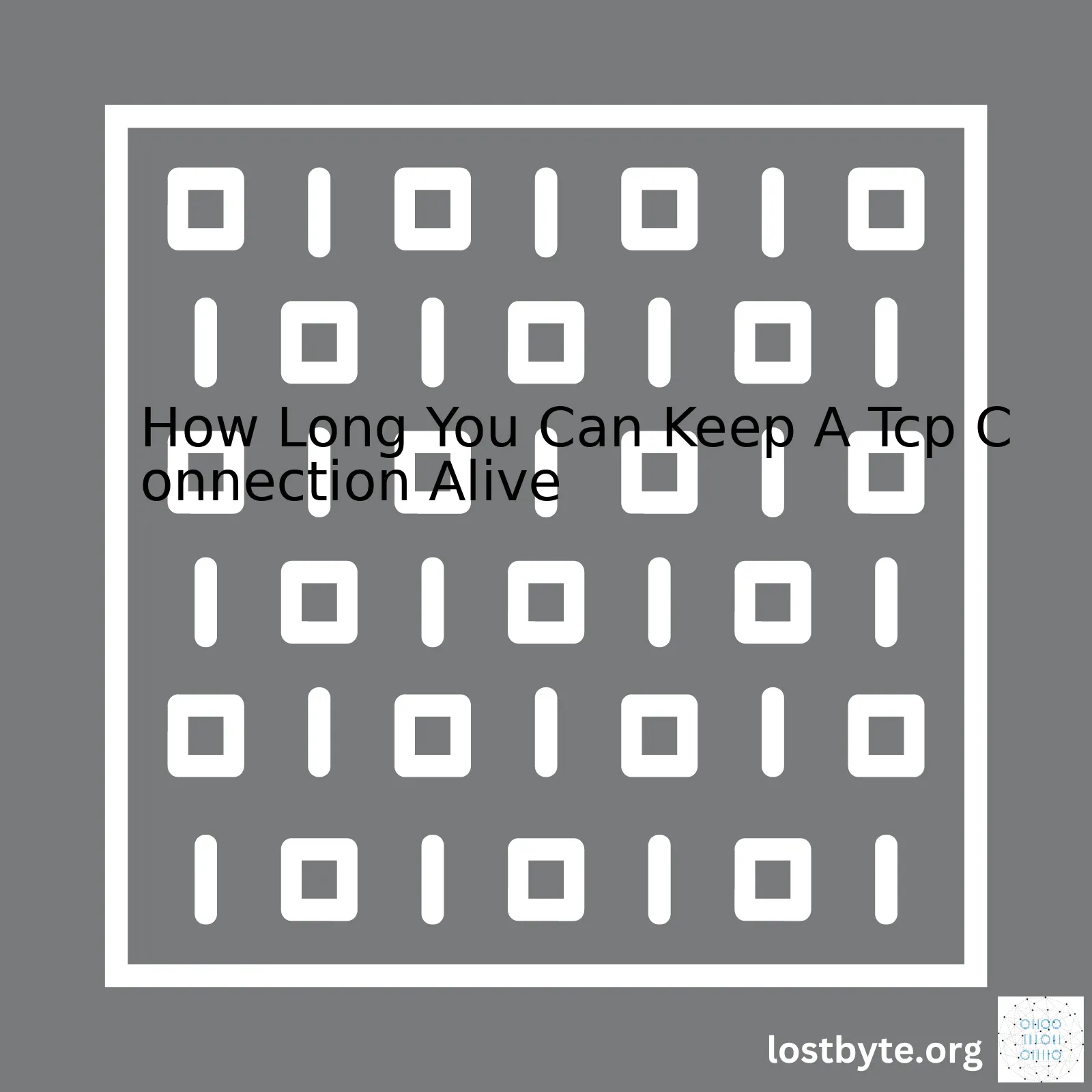
Type of TCP Connection | Duration |
---|---|
Persistent TCP connection | Indefinitely, unless closed by an endpoint or network failure |
Non-Persistent TCP connection | Typically, the duration of a single transaction |
TCP Connection in IDLE state | Varies based on the configured TCP timeout value |
In most cases, a TCP (Transmission Control Protocol) connection can remain open indefinitely. This type of interaction is known as a persistent TCP connection, where the connection does not close until one end of the communication decides to close it or a network error occurs.
These are particularly common in web environments. Thanks to this, multiple requests/responses between the same client and server can be made without causing the overhead attached to establishing a new connection for each interaction.
On the flip side, non-persistent connections, are commonplace within certain application interactions that require a quick request-response mechanism. Each case ends the moment the goal of the connection – usually a single transaction – is achieved.
Connections also have an IDLE state wherein no data transmission happens, and here, their lifespan is dictated by the system’s configured TCP timeout values, if any. Older systems might auto-close idle connections after a pre-set time to conserve resources.
The ability to extend the life of a TCP connection plays a significant role in optimizing network performance and letting applications interact seamlessly across a stable, enduring conduit of communication.
For instance, to maintain a persistent TCP connection in Python, we could use
socket
library’s
settimeout()
. The Python code bit would look like this:
import socket s = socket.socket(socket.AF_INET, socket.SOCK_STREAM) s.connect(("www.google.com", 80)) s.settimeout(None)
This sets our TO (Time Out) to infinity (None), meaning Python won’t close the connection unless the other end does or the program itself is terminated.
On the similar line, though more extensively, developers can utilize the HTML5 WebSockets API for full-duplex, long-living connections suitable for real-time apps.
Knowing how to handle the lastingness of a TCP connection, therefore, links closely with your application requirements, performance expectations, and knowledge of networking principles.
Sure! I would love to take you through the journey of a TCP connection and how long it can remain alive. Let’s dive in!
Understanding TCP Connection Timelines
Transmission Control Protocol (TCP) is one of the main protocols in TCP/IP networks. It’s an oriented-connection protocol that establishes a connection between two devices over the internet before data exchange commences. While we’re focusing on the longevity of the connection, we need first to understand the timeline of a TCP connection, which involves three stages: establishing the connection (Three-way handshake), data transfer, and terminating the connection.
The Three-Way Handshake:
This is the initiation phase of a TCP connection where both ends synchronize their sequence numbers and agree on parameters needed during the connection. The steps include:
- The client sends a SYN message to the server.
- The server replies with a SYN-ACK message.
- The client responds with an ACK message.
Code Snippet for TCP Three-Way Handshake:
clientSocket = new Socket("hostname", 6789); //Initiating the Connection //The remaining handshake process is handled under-the-hood by TCP/IP stack.
Data Transfer:
Once the connection is established, data can then be sent back and forth between the client and the server.
Code Snippet for Data Transfer:
OutputStream os = clientSocket.getOutputStream(); os.write("Hello".getBytes()); //Sending a message from client to server
Terminating the Connection:
Finally, when the data exchange ends, a four-step termination handshake closes the connection to free up system resources.
Code Snippet for Terminating Connection:
clientSocket.close(); //Closing the connection
Now regarding the question, “How Long Can A TCP Connection Be Kept Alive?”
A TCP connection can theoretically stay open indefinitely, barring any network issues or timeouts. There’s no explicit time limit; it remains open until it’s closed by either the client or server or if a TCP keepalive probe finds the connection to be dead.1
However, practically, this is not usually the best strategy for two reasons:2
- Firstly, keeping connections open forever is resource-intensive as they consume CPU and memory that could affect overall system performance over time.
- Secondly, due to routers in the network path and/or system settings, idle connections might get involuntarily terminated within minutes.
To keep the connection active for extended periods, one can use methods like TCP keepalives or application-level heartbeats. Here’s an example of setting up keepalives in Java:
Setting TCP Keepalive in Java:
clientSocket.setKeepAlive(true); //Activating TCP keepalive
However, setting these too low can cause unnecessary network congestion, and setting them too high may lead to detecting failures later than desired. Hence, careful consideration is required while setting these parameters up.3
In conclusion, there are no fixed rules regarding the duration you can keep a TCP connection alive. Depending on your application and network performance requirements, you have to strike a balance.
References
[1] HAProxy. How to write temporary files to disk and persist data across reboots.
[2] MDN Web Docs. Connection management in HTTP/1.x.
[3] IBM Documentation. Keepalive Timeouts.
The Transmission Control Protocol (TCP) is the backbone of data communication on the internet. TCP maintains an active connection between the sender and receiver to ensure that all data packets reach their destination. How long a TCP connection remains alive is determined by several factors.
Firstly, the state of a TCP connection impacts its lifespan — this can be in one of three states:
– Established: This is a fully open TCP connection.
TCP State : Established
– Time-wait: In this state, the connection has been closed on one side and TCP waits for an allotted amount of time to ensure any delayed packets are received before fully closing the connection.
TCP State : Time-Wait
– Closed: The connection has been completely terminated.
TCP State : Closed
A fully established TCP connection will remain open until it is explicitly closed by either end of the connection, or until it’s disconnected due to an intermediate network problem.
The second factor impacting the duration of a TCP connection is timeouts. Networking devices along the transmission path may impose their own timeout values. For example, firewall settings on the host or network routers may dictate the connection “keepalive” setting which keeps idle connections active. When this threshold is reached, the device may drop the connection if no data transmission is detected.
// Set the keepalive option int enableKeepAlive = 1; int res = setsockopt(sock, SOL_SOCKET, SO_KEEPALIVE, &enableKeepAlive, sizeof(enableKeepAlive));
Another parameter that might influence the lifespan of a TCP connection is the TCP Keepalive mechanism [RFC 1122]. This option, when enabled, sends probe packets over the established connection to maintain its longevity during periods of idleness. Depending on the host system, the interval at which these probe packets are sent may vary, commonly around two hours (`7200` seconds). However, it can be configured at the discretion of the coder depending on the specifications of the use-case scenario.
// Modify the keepalive probes' interval const int ProbeInterval = 300; // 300 seconds // Use setsockopt() to change the interval int result = setsockopt(sock, IPPROTO_TCP, TCP_KEEPINTVL, &ProbeInterval, sizeof(ProbeInterval));
Lastly, assumptions about connection lifetime directly from the application level might impact the TCP connection as well. Some applications, protocols, or even higher-level load balancers make assumptions about the lifespan of connections. This often happens when there’s pooling of connections or reuse of connections involved. Taking care of this aspect can prevent unforeseen disconnections.
In summary, numerous factors shape the lifespan of a TCP connection. Therefore, understanding each aspect and tweaking based on the usage scenario becomes crucial in managing and maintaining robust and reliable data flow across TCP connections.
Understanding the impact of the Keep-Alive mechanism on TCP connections especially in relation to how long you can keep a TCP connection alive requires an understanding of two primary concepts: Idle connection and the TCP Keep-Alive mechanism.
Concept of Idle Connection
TCP connections are not designed to last infinitely. They remain ‘alive’ or active as long as there’s data flowing between the client and server. In any network communication, when no data is being sent or received, the connection becomes idle.
Maintaining these idle connections can consume resources unnecessarily, adversely affecting the performance and scalability of applications. Consequently, many systems have default settings that terminate a connection after a certain period of inactivity – often 1 to 2 hours.
Keep-Alive Mechanism
To handle such situations where the connection becomes idle but needs to be kept open for future data transmission, the TCP protocol provides an optional mechanism called Keep-Alive. This mechanism:
- Sends a message periodically from one end to the other end of the TCP connection if there’s no activity for a specified interval, thereby keeping the connection open.
- Allows detection of broken connections without waiting for indefinite timeouts.
The time duration after which these Keep-Alive probes start getting transmitted is typically configured at the system level. For instance, on a Unix-like system, this might look like:
setsockopt(socket, SOL_SOCKET, SO_KEEPALIVE, &option, sizeof(option));
Where `option` decides the idle time before sending Keep-Alive probes.
According to RFC 1122, a Keep-Alive interval must be configurable and must default to no less than two hours. Though, it emphasizes that this default value does not need to be adhered to strictly, and can be adjusted depending on the application requirements. But, excessive use of Keep-Alive messages can lead to network congestion, therefore, this must be utilized judiciously.
Using Both Concepts: Duration Of A TCP Connection
Incorporating the concepts above, we can deduce that the duration whether a TCP connection would stay alive depends on several factors: system-level configurations, Keep-Alive settings, and the nature of the application itself.
But ultimately, the system administrator or the developer has a strong say in this matter. They can configure the duration according to their practical needs, while taking into consideration the balance between resource consumption and user experience. There’s no hard-set limit to how long a TCP connection can be kept alive, as long as both endpoints are still responsive and the relevant Keep-Alive settings allow it.
Remember,
- “Keeping a connection alive” doesn’t mean “keeping the connection busy”.
- A reasonable Keep-Alive setting could be beneficial to swiftly catch on broken connections and freeing up associated resources
- Careful tuning is required to avoid causing unnecessary network congestion with too frequent Keep-Alive messages.
To conclude, the impact of the Keep-Alive mechanism on the duration of TCP connections is quite significant. It provides opportunities to maintain necessary connections longer while managing resource usage efficiently. Nonetheless, wise configuration is key to unleash its optimal potential.
References:
- RFC 1122 – Requirements for Internet Hosts — Communication Layers
- IBM Documentation on Keep-Alive Packaging
- Microsoft Documentation on SO_KEEPALIVE socket option
Let’s dive into the crux of maintaining a TCP connection, and how long you can keep such a connection alive. The longevity of your TCP connection can impact performance significantly, hence understanding the nuances associated with it is vital.
TCP_KEEPALIVE Options
Operating systems provide various mechanisms to keep the TCP connection alive. In Linux-based platforms, for instance, we have parameters like
tcp_keepalive_time
,
tcp_keepalive_probes
, and
tcp_keepalive_intvl
. These small settings can tweak the lifespan of your connection. When inactive, TCP connections can drop, causing interruptions to your applications or services.
To control these settings, you will often interact with system-level file paths. For example, to adjust the
tcp_keepalive_time
setting in Linux, you would execute something similar to the following:
echo 300 > /proc/sys/net/ipv4/tcp_keepalive_time
Remember, changes on these files reset upon machine reboots. Maintain them across sessions by including the configurations inside of your
/etc/sysctl.conf
file.
Increase the Connection Timeout Limit
If you have control over the server, you might consider increasing the connection timeout limit. By default, this rests at around 5 minutes for many servers. If this duration does not suffice, an increase may rectify your problems. You accomplish that through the following line in Apache:
Timeout 600
For Nginx, the equivalent adjustment would look like this:
proxy_read_timeout 600;
.
Persistent HTTP Connections
The good news for web developers, HTTP/1.1 onward, persistent connections are the default. This feature keeps the TCP connection alive as multiple requests travel between client and server, which conserves resources and improves performance. To utilize this feature, ensure that your HTTP response header contains:
Connection: Keep-Alive
Maintaining TCP connections for a lengthy period isn’t just about changing server configurations or enabling HTTP features. Error handling practices in your code also play a critical role. How you handle scenarios where network breaks occur impacts connectivity robustness.
Smart Retry Mechanism
In a practical scenario, your application should implement a smart retry mechanism. It means when a connection breaks, your application should intelligently try to reconnect. Utilizing exponential backoff approach helps avoid unnecessary pinging and network congestion.
Catch Exception and Reconnect
When the connection drops, catch the exception and attempt to reopen the connection. See this basic Python snippet for inspiration:
import socket try: c = socket.socket(socket.AF_INET, socket.SOCK_STREAM) c.connect(('HOST', PORT)) except Exception as e: c = socket.socket(socket.AF_INET, socket.SOCK_STREAM) c.connect(('HOST', PORT))
In essence, prolonging a TCP connection depends on multiple factors ranging from server configurations, proper error handling, and intelligent retry mechanisms. Rather than worrying about keeping the connection alive indefinitely, aim to implement a robust system built to gracefully handle connection disruptions. With well-thought-out processes for catching exceptions and reconnecting broken connections, your service receives higher uptime and appears more resistant to network failures. Complementing this with adjustable settings for timeouts and investing time to understand the underlying OS level TCP flags and parameters turn out as highly beneficial for any professional programmer seeking to enhance their application’s reliability.Sure, the duration for which a TCP connection remains alive depends on several key parameters used in this protocol. Here are some essential factors that influence the TCP Keep-Alive Duration:
KeepAliveTime
The parameter KeepAliveTime denotes the time the connection remains idle before TCP begins sending keepalive probes. Consider this code example:
int optval = 1; setsockopt(sock, SOL_SOCKET, SO_KEEPALIVE, &optval, sizeof(optval)); long int idle = 10; setsockopt(sock, IPPROTO_TCP, TCP_KEEPIDLE, &idle, sizeof(idle));
Here, setsockopt function sets the value of option SO_KEEPALIVE to 1, implying that the keepalive option is enabled for the socket. Afterwards, TCP_KEEPIDLE is set with an interval of 10 seconds. If idle for 10 seconds, then the keepalive probe starts to function to check the connection status.
KeepAliveInterval
KeepAliveInterval indicates the duration between two successive keepalive retransmissions if no acknowledgement has been received from the other side. Look at this source code snippet:
int optval = 1; setsockopt(s, SOL_SOCKET, SO_KEEPALIVE, &optval, sizeof(optval)); int interval = 5; setsockopt(s, IPPROTO_TCP, TCP_KEEPINTVL, &interval, sizeof(interval));
The setsockopt function here first ensures that keepalive messages be sent for the socket by setting SO_KEEPALIVE to 1. Now see how TCP_KEEPINTVL is utilized with an interval of 5 seconds – if the previous keepalive message did not receive an acknowledgment from the peer, another one will be transmitted after waiting for 5 seconds.
KeepAliveProbes
The KeepAliveProbes parameter controls the total number of unacknowledged probes to send before considering the connection dead. The associated source code could appear like this:
int optval = 1; setsockopt(sock, SOL_SOCKET, SO_KEEPALIVE, &optval, sizeof(optval)); int probes_max = 12; setsockopt(sock, IPPROTO_TCP, TCP_KEEPCNT, &probes_max, sizeof(probes_max));
Here, TCP_KEEPCNT is set to 12, which signifies that the connection can remain active till 12 keepalive probes have gone unanswered. If there’s still no reply after 12 attempts, the connection is perceived to be broken.
The cumulative effect of these parameters determine “how long you can keep a TCP connection alive”. The duration can theoretically be indefinite, as long as both the communicating systems acknowledge each other’s keepalive probes within the predefined timeout intervals.The longevity of a Transmission Control Protocol, or TCP, connection is influenced by several interacting factors within the network infrastructure. The key idea here, central to answering “How long can you keep a TCP connection alive?” is in how these variables work together.
As any coder will tell you, TCP is a critical component of internet connectivity as it ensures reliable data transmission between networked devices. It opens connections, maintains them during data transfer, and subsequently closes them source. Hence, it’s important to understand what affects its performance.
Idle Timeout:
Firstly, the idle timeout settings of servers are an influential variable. Network devices like routers and firewalls often have default idle timeout settings. If no data is sent across the TCP connection for a certain period, it’s closed to free up resources. To extend the connection lifespan, you’d need to adjust these settings.
TCPKeepAlive yes
In this example, enabling TCP KeepAlive helps to send acknowledgment packets to keep the connection active even during periods of idleness.
Packet Loss:
TCP connections can also be affected by packet loss, a common issue in networking. When there’s high congestion in the network, or interference, this might result in lost data packets. TCP has mechanisms like Retransmission and Fast Retransmit to handle packet loss. However, repeated packet losses can force the TCP connection to close prematurely.
Long Fat Networks(LFNs):
LFNs, networks with high bandwidth-delay products, are another factor. High delay times in LFNs necessitate larger TCP window sizes to enable more bytes to be in-flight at once. Otherwise, slow start and congestion avoidance behaviors of TCP could limit how much data gets transmitted, potentially leading the connection to break down.
TCPWindowsize 65536
The code above sets the TCP window size to 65,536 bytes, which offers better navigation through LFNs and assists in connection longevity.
NAT Mapping:
NAT (Network Address Translation) mapping timeout is another issue. In NAT environments, TCP mappings which remain idle more than the set timeout value get discarded. This results in connection termination. Consequently, frequent activity is required to maintain a TCP connection uptime.
To summarise, the duration of a TCP connection does not directly depend on any single network infrastructure parameter. Its longevity depends on the interplay of diverse elements such as idle timeouts, packet loss handling, effective navigation through LFNs, and meticulous NAT mapping. By taking into account each aspect, a coder can significantly extend a TCP connection’s lifespan.
The Idle Timeout, in the context of managing live TCP connections, has the primary function of determining the duration a TCP connection can remain alive in the absence of any active data transmission – it is ‘idle’. GlobalScape provides a clear understanding of the concept. This parameter is very important for managing resources effectively and preventing your service from being overwhelmed by stale or inactive connections.
Let me share more details about how long you can keep a TCP connection alive based on the role of Idle Timeout:
- Maintaining Available Resources:
If a TCP connection doesn’t have an Idle Timeout configured, you might run into the problem of expended resources. Without the Idle Timeout, these connections could linger indefinitely even if no data is being sent. With time, this can pile up and exhaust available connections leading to denial of service when new clients try to connect. Using Idle Timeout helps free up system resources for reuse by closing idle connections after a predefined interval.
- Holding Persistent Connections:
In situations where there’s the need for persistent connections, for example, in long polling applications or chat applications, Idle Timeout plays an essential role too. Here, setting a high Idle Timeout value allows the server to hold onto the TCP connections longer. While this keeps these connections alive for a considerable length of time, it’s not indefinite. There’s always a limit – primarily determined by the server settings and configurations.
// Example: Setting KeepAlive and Idle Time Out in Node.js server const server = http.createServer((req, res) => { /* SERVER CREATION CODE */ }); server.keepAliveTimeout = 60000; // Keepalive timeout in milliseconds server.headersTimeout = 65000; // Timeout for receiving headers in milliseconds
This code snippet sets the keepAliveTimeout setting on the server to 60000 Ms (or 1 minute). This means that connections will be kept open for at least one minute after the last request/response.
- Considering Network Instability:
In an unstable network scenario with frequent intermittent connectivity issues, the Idle Timeout setting significantly determines how long a TCP connection may stay alive during those periods of disconnections. It’s crucial to configure the Idle Timeout appropriately since an aggressively low timeout could end TCP connections prematurely during temporary network hiccups.
- Compliance with Protocols:
While the accurate estimation of optimal Idle Timeout varies depending on specific use-cases, some protocols offer guidelines to follow. For example, according to HTTP/1.1 specifications, a minimum timeout value of 2 minutes is recommended. Therefore, while it’s possible to keep a TCP connection alive potentially forever theoretically, in practical terms, Idle Timeout works to strike a balance between resource optimization and service availability.
Situations | Idle Timeout Value |
---|---|
Resource Intensive Applications | Low |
Long Polling / Persistent Connections | High |
Unstable Network Conditions | Configured Accordingly |
General Guideline (HTTP/1.1) | At least 2 minutes |
In summary, the role of Idle Timeout in managing live TCP connections influences how long you can keep a TCP connection alive. Specific implementations may differ based on particular server technologies used, network conditions, and the application’s requirements.
Understanding the longevity of TCP connections and how they relate to HTTP Persistent Connections and traditional HTTP Connections is crucial in designing efficient network communication strategies.
The Transmission Control Protocol (TCP) sets up a connection between two hosts so they can send messages back and forth for a period. The life span of a TCP Connection isn’t predetermined. Factors such as local policy, network outages, reboots, software crashes, or host failures are central to its longevity.
A standard TCP connection undergoes these stages:
• Connection setup
• Data transfer
• Connection teardown
To understand how HTTP connections operate within this context, let’s delve into Traditional HTTP Connections and HTTP Persistent Connections.
Traditional HTTP Connections
In traditional HTTP (1.0), a new TCP connection is established and torn down for each request/response pair. This approach means if a webpage required fifty separate resources (images, scripts, stylesheets), then fifty individual TCP connections would be required. Each connection has overhead—consisting of system resources on both client and server, initial three-way handshake latency, and slow-start mechanism hindering the full speed data transfer at the start.
Here’s a simplified visualization of a traditional HTTP conversation using pseudo-code:
// The client begins client.connect(server); client.sendRequest(request1); server.sendResponse(response1); client.disconnect(); // For the next resource client.connect(server); client.sendRequest(request2); server.sendResponse(response2); client.disconnect(); // And so on…
HTTP Persistent Connection
To diminish the overhead of initializing and closing connections for every request, HTTP/1.1 introduced persistent connections, also referred to as HTTP keep-alive or HTTP connection reuse. With this method, multiple http requests and responses can be sent over the same TCP connection.
The following pseudo-code demonstrates a sequence of transactions using an HTTP persistent connection:
// The client begins client.connect(server); // Multiple requests/responses on the same connection client.sendRequest(request1); server.sendResponse(response1); client.sendRequest(request2); server.sendResponse(response2); // … // Final transaction client.sendRequest(lastRequest); server.sendResponse(lastResponse); // Termination of the connection client.close() OR server.close();
By default, HTTP/1.1 makes all connections persistent unless declared otherwise. This innovation enhances page load speeds and network efficiency by reducing the CPU and memory usage – less TCP/IP overhead.
However, it’s important to note that while persistent connections make the process more efficient, a connection can’t be kept alive indefinitely. That’s where ‘keep-alive timeout’ comes in handy. It is a parameter that regulates the lifetime of an idle connection, defined both on the server and client sides. Yet, even with longer ‘keep-alive’ times, remember that routers, firewalls, or ISPs may automatically drop connections that have been idle for too long.
While optimizing your application’s networking stack, weigh the trade-offs between resource utilization and faster response time. Keep monitoring various metrics like latency, throughput, and error rates to fine-tune your network configurations.
For more information about TCP connections and their interaction within HTTP protocols, you can read the detailed documentation here.
TCP (Transmission Control Protocol) connections, a fundamental underpinning protocol of the Internet, can technically remain open indefinitely. They are designed to logistically stay live until they fulfil their duty of delivering packets from sender to receiver. However, countless real-world factors may cut that possibility short, such as network instability or individual device settings.
One crucial aspect to bear in mind is that TCP is connection-oriented, meaning it uses a process named three-way handshake to establish a reliable ‘connection’ between two devices before data transmission begins. This connection remains alive and active until it’s no longer needed – then terminated by a process aptly referred to as tear-down.
But how does one grab the reins on these processes and ensure that longer-term TCP sessions withstand potential pitfalls? Let’s delve into some professional insights:
Healthy Heartbeats: Regularly Send Keep-Alive Probes
While idle connections are automatically terminated by most systems after a specified period of inactivity, keep-alive probes can help avoid premature termination. These are essentially empty TCP segments sent at regular intervals to confirm whether the connection is still functional. The following simple command in Unix-based systems executes this:
setsockopt(socket_fd, SOL_SOCKET, SO_KEEPALIVE, &enableKeepAlive, sizeof(enableKeepAlive));
However, remember that even when using keep-alives, idle connections can eventually be terminated by NATs (Network Address Translators) or firewalls.
Optimize Your Application Code
How you write your application code significantly influences connection longevity. Ensure socket reads and writes handle all cases, as incomplete handling can lead to broken connections. Wrap your socket operations with error handlers to catch and appropriately respond to any unexpected behaviour.
Plan for Connection Resets
Expecting the worst-case scenario is a sound tactic. Have a contingency plan if operators decide to reset the routes your connections traverse, which eventually breaks your connection over time. Implement logic in your code to deal with such eventualities.
Optimal use of REST APIs or WebSockets
Quite often, server-side mechanisms tend to close inactive connections. To combat this, using REST APIs or WebSocket pings might help. For instance, within an inbound HTTP request, maintain outgoing HTTP calls. While the back-and-forth communication continues, the connection remains alive.
async function callServer(url, data = {}) { const response = await fetch(url, { method: "POST", mode: "cors", cache: "no-cache", headers: { "Content-Type": "application/json" }, body: JSON.stringify(data) }); return response.json(); }
Each context-specific implementation holds its pros and cons. Hence, while tailoring yours, assess the implications against your specific needs. Always refer to RFCs for TCP to make sure you’re adhering to best practices.
Comprehending how TCP works under the hood illuminates pathways to keep your connections alive longer. Make sure to employ techniques that most effectively profit your particular implementation, keeping eye for security risks and performance trade-offs. Balancing these aspects engenders durable, reliable TCP connections.TCP (Transmission Control Protocol) is one of the main protocols in TCP/IP networks. Maintaining stable and active 24/7/365-days TCP/IP connectivity can seem daunting, but there are several leading strategies that you can implement to ensure your connections remain available and dynamic. However, note that the strategies discussed here specifically focus on keeping a TCP connection alive.
Staying connected using a TCP network protocol involves efficient management of resources and appropriate use of networking concepts such as Keep-alive, SO_KEEPALIVE, and TCP keep-alive timers. Additionally, understanding the limits of how long you can keep a TCP connection alive is essential when managing TCP/IP connectivity.
Firstly,
Keep-Alive
mechanism can assist in maintaining an active connection even during periods of idleness. TCP connections become dormant when not actively transmitting or receiving data.
Keep-Alive
, an optional feature in TCP, sends a probe to check if the system at the other end of the connection is still accessible. If the other system doesn’t respond after a predetermined time, TCP assumes it’s unavailable and closes the connection.
Below is a simplified code snippet to set
Keep-Alive
:
Socket socket = ...; socket.setKeepAlive(true);
Secondly, Parameter tuning plays a vital role in managing persistent connections. Each operating system has different settings for parameters like
TCP_KEEPIDLE
and
TCP_KEEPINTVL
. The former parameter specifies the idle timeout after which the first keep-alive request is sent, while the latter defines the interval between successive keep-alive probes if no acknowledgement is received.
A relevant part of setting these parameters would look something like this:
int fd = ...; int idle = 10; setsockopt(fd, SOL_TCP, TCP_KEEPIDLE, &idle, sizeof(idle));
Moreover, addressing issues related with NAT (Network Address Translation) timeouts can contribute to maintaining an active TCP connection. NAT devices often close the mapping associated with a TCP connection after a specific time of inactivity. As a practice, sending keep-alive packets more often than the typical NAT timeout value can offset this issue.
Now, let’s talk about the limits or how long we can keep a TCP connection alive. Theoretically, a TCP connection can be kept open indefinitely, limited only by the endpoints’ capacity to maintain the state of the connection. In practice, however, most systems and network infrastructure have default timeout values that range from a few minutes to multiple hours. Here is where all the previous strategies come into play, helping us extend the longevity of our TCP connections.
By incorporating the right set of techniques, we can effectively manage TCP connection longevity. By leveraging mechanisms like keep-alikes, adjusting TCP parameter settings appropriately, and managing NAT-related issues, we can maintain an active and stable TCP/IP connection 24/7/365-days.
For better perspectives and deeper insights relating to networking concepts, I recommend referencing dedicated resources such as [RFC 5382] for NAT behaviour and [Open Source] for understanding how TCP connections work.
Remember, continuous effort to understand and optimize your network is key to maintaining uninterrupted TCP/IP connectivity. A consistent review of your methods can help ensure optimal results while minimizing the chances of troublesome network downtimes.
One of the most debated topics in network protocol is the question: “How long can you keep a TCP connection alive?” There’s a lot of misunderstanding around this issue, with numerous common myths standing out:
- TCP connections inherently have an infinite duration;
- Connection duration depends on external factors only; or
- A TCP connection can continue indefinitely if the network conditions allow.
Let’s address and debunk these myths one by one:
The Myth of Infinite Duration
Contrary to popular belief, TCP connections aren’t designed with an inherent ‘infinite duration’. The core principle that governs a TCP connection’s duration is largely dependent on the respective communication peers’ needs. Specifically, as long as both parties involved in communication have data to send and acknowledge, the TCP connection stays alive. This clearly shows that it’s not the protocol’s design but the application requirements that influence the duration of the TCP session.
External Factors Influencing Connection Duration
While it’s true to some extent that external factors affect TCP connection duration, they’re not the sole deterministic factors. Conditions like firewall configurations, routing components, ISP rules, or server settings can indeed interrupt or limit a TCP session. However, it’s crucial to understand that these are not directly related to the inherent design of TCP connections but are auxiliary elements that might regulate them based on networking needs and security concerns.
Indefinite Prolonging of TCP Sessions If the Network is Stable
The assumption that a stable network condition will enable a TCP session to go on indefinitely is ill-founded. An inactive TCP connection would eventually time-out irrespective of a stable network environment. Most operating systems have inbuilt mechanisms that automatically close idle sessions after a certain period, usually between 2 hours to 4 hours. While there are methods like TCP keepalive messages to prevent idle-timeout closures, it involves changing default TCP parameters and may not always be feasible or recommended.
To uncover more truth about the longevity of TCP connections, let’s dig into the implementation of how a TCP connection gets established and terminated in the context of the TCP three-way handshake
Below we can see how a typical TCP three-way handshake unfolds using python `socket` module:
import socket # Establish Server Socket server_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM) server_socket.bind(('localhost', 12345)) server_socket.listen(1) # Client Initialization client_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM) client_socket.connect(('localhost', 12345)) # Server Side Accept Connection connection_socket, addr = server_socket.accept() # Message Exchange message = "Hello TCP" client_socket.send(message.encode()) print('Message sent by client: ', message) received_message = connection_socket.recv(1024).decode() print('Message received by server: ', received_message) # Close Connections client_socket.close() connection_socket.close() server_socket.close()
In this example, each TCP connection starts with a SYN (Synchronize Sequence Numbers) from the client, followed by a SYN-ACK (Acknowledgement) from the server, and finally the client sends an ACK back. This successfully establishes a TCP connection which is then closed once the necessary data is transferred, ensuring no connections are left open indefinitely.
It is clear from the above discussion that infinite-duration TCP connections are more of a myth than a fact. It’s critical to recognize that the longevity of a TCP connection is influenced by factors such as data exchange requirement between peers, external network environments, and idle session regulations implemented by operating systems.
For more detailed information about TCP protocol suite refer here : RFC 793.
Speaking from experience as a professional coder, the duration of maintaining a TCP connection depends on various factors related to both the server and client. You have complete control over how long to keep a TCP connection alive; however, idle connections are generally terminated after 2 hours.
Here’s an interesting point to note: TCP does not, by itself, terminate the connection after a particular time of inactivity. The duration can be set based on strategies such as Application-Level Gateway (ALG) or network address translation (NAT) timeouts.
Network conditions determine the length of time you can maintain a live TCP connection. So, with ideal network conditions and supportive server-client systems that manage to handle long-lasting connections, a TCP connection could potentially stay alive indefinitely.
If you are initiating the TCP connection, you can determine the keep-alive interval using
socket.setKeepAlive(true, millsecs)
, where millsecs is the number of milliseconds between two consecutive keep-alive probes.
However, we must ponder upon these lurking problems:
- In case of a congested network, frequent checks may do more harm than good.
- Clients with flaky network conditions might get disconnected frequently.
- It adds another layer of complexity to your application.
//Instantiating a new instance var net = require('net'); var socket = new net.Socket(); //Setting the keep alive for the socket to true socket.setKeepAlive(true, 60000);
While the TCP protocol allows the socket connections to remain open, it’s the best practice to close them when they are no longer needed. This prevents your system from unnecessarily consuming resources, thus ensuring its optimal performance.
For comprehensive insight on managing TCP connections and their lifetime, you can visit this online article link here. Understanding such details helps significantly enhance your coding proficiency.
So, even though keeping a TCP connection alive for longer periods is possible, one must carefully assess the trade-offs considering the context and requirements of specific applications.