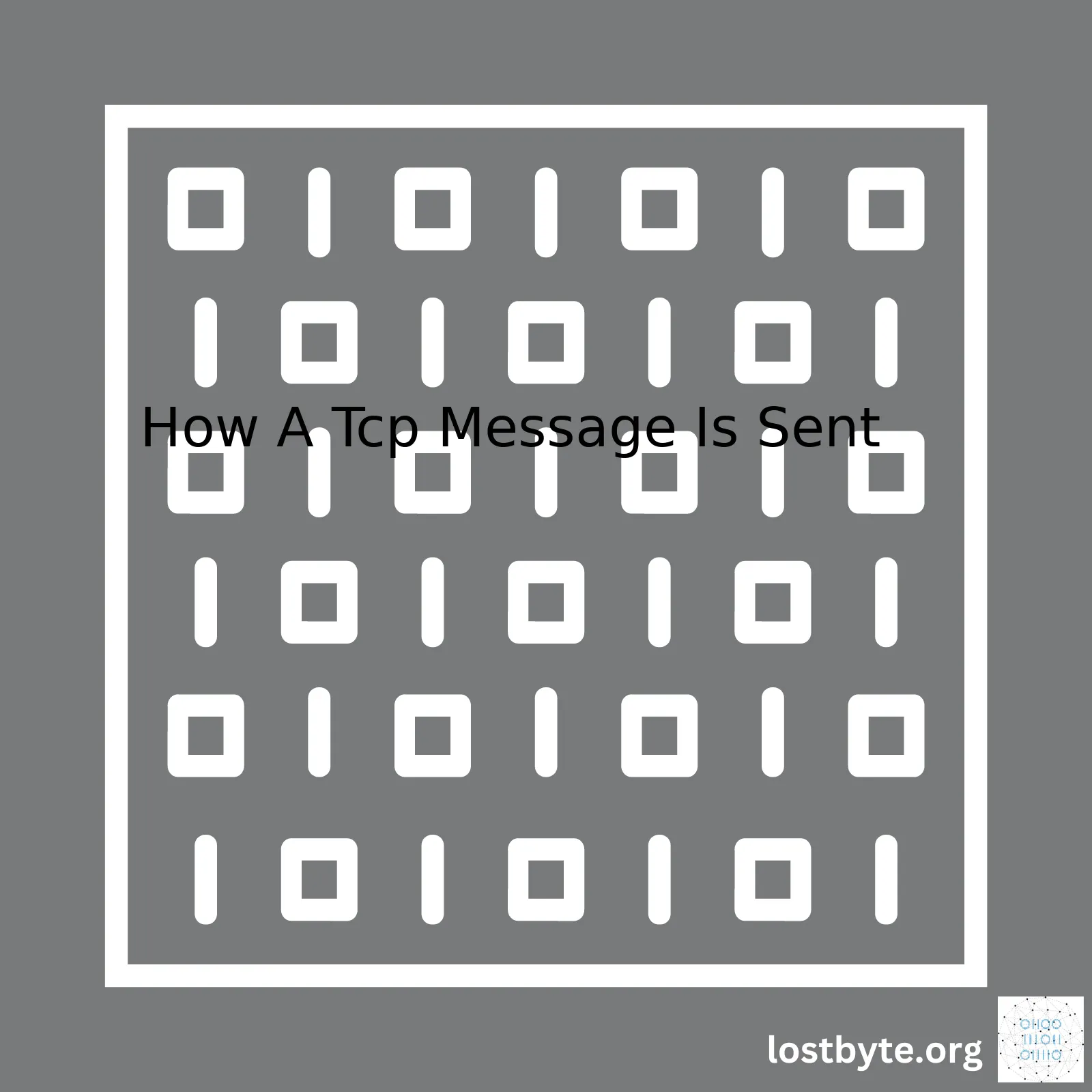
To make it easier to understand, I’ll outline the entire process with a table first:
Step | Details |
---|---|
Connection setup with three-way handshake | The sender initiates the connection by sending a SYN packet. The receiver then acknowledges this with a SYN-ACK packet. Finally, the sender sends an ACK packet back to the receiver, confirming the connection. |
Data transmission | The sender starts transmitting data in small blocks known as ‘segments.’ These segments are encapsulated in IP packets and transmitted over the network. |
Acknowledgement | The receiver sends ACKs (Acknowledgements) for each segment it receives. If the sender does not receive an ACK due to packet loss or delay, it re-transmits the segment after a timeout period. |
Connection termination | Once all the data has been transmitted and acknowledged, the sender or receiver can initiate termination of the connection using a FIN packet. Similar to the connection setup, this also requires a three-way handshake. |
When a TCP message is sent, the foremost step is the execution of a ‘three-way handshake’ which establishes a reliable connection between the sender and receiver. This involves sending synchronization (SYN) and acknowledgment (ACK) packets between both parties.
Following the successful connection, data transmission takes place. The data is segmented into small, manageable blocks that are encapsulated within Internet Protocol (IP) packets. For each packet transmitted, there’s a sequence number attached that assists in the ordering of data at the receiving end.
The backbone of TCP lies in its error control and flow control mechanisms. As data segments are received, an acknowledgment (ACK) message is returned to the sender. In case a packet gets lost or damaged during transmission, it will not be acknowledged, triggering a timeout at the sender’s end. This will result in the retransmission of that specific packet, ensuring reliability of data transfer.
Once the data is fully transmitted and acknowledged, the connection is terminated through another three-way handshake involving finish (FIN) packets.
The TCP methodology provides a dependable transport layer protocol designed to offer seamless and secure data transmission over networks. This systematic approach of setting up connection, segmenting data, acknowledging packets and terminating connection efficiently ensures that all data is accurately delivered without any errors.[source]
Here are some simple Python
socket
library snippets demonstrating simple TCP data transmission:
Server side:
import socket # Create a TCP/IP socket sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM) server_address = ('localhost', 12345) sock.bind(server_address) sock.listen(1) while True: connection, client_address = sock.accept() data = connection.recv(1024) print(f'received {data}') connection.close()
Client side:
import socket # create a TCP/IP socket sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM) server_address = ('localhost', 12345) sock.connect(server_address) try: message = b'This is a test message.' sock.sendall(message) finally: sock.close()
In these snippets, we’ve used Python’s built-in
socket
library, which provides a standard interface to communicate over TCP/IP networks. The server listens for incoming connections, while the client initiates a connection, sends data, and then terminates the connection.Undeniably, TCP (Transmission Control Protocol) represents an essential protocol in the vast world of Internet communication, providing an imperative bidirectional conversation between two computers. Here, we will analyze how a TCP message is sent and delve into all the stages it takes to ensure successful transmission.
To initiate with, consider a computer or server wanting to transmit a message to another device using TCP. It begins by establishing a TCP connection via a method called ‘The Three-Way Handshake.’ As part of this technique, it follows these steps:
- SYN: The initiating client sends a
SYN
packet to the targeted server, declaring its desire to open a connection.
- SYN-ACK: Once obtained, the server acknowledges the request by sending a packet named
SYN-ACK
.
- ACK: On receiving the
SYN-ACK
, the original sender replies with an
ACK
message. This final exchange completes the setup and ensures both devices are ready for TCP transfers.
With the handshake complete, the intricacy of data transmission comes into play. TCP, known for its reliable delivery, splits the entire data into multiple packets of manageable size to enhance communication efficiency. Afterward, each packet is marked with a sequence number, ensuring that eventual reassembling maintains the original order.
Next, data transfer ensues. The client sends groups of packets at a pre-agreed rate termed as the window size. Each time the server receives a packet, it sends back an acknowledgement (ACK). If an ACK for a particular packet isn’t received within a specified time-out period, then the client assumes that packet was lost in transit, and thus, resends it. In addition to quality control, this principle also serves as a base to avoid network congestion.
On completion of the data transfer, it’s necessary to properly terminate the TCP connection avoiding any accidental data loss or undefined behavior. This process also involves a four-way handshake process, ensuring that both sides simultaneously close the connection when they have no more data to send.
One critical thing to remember about the TCP is that it’s not just raw data being exchanged. Within the data packets, there’s a segment header containing the source port, destination port, sequence number, acknowledgment number, offset, flags, window, checksum, and urgent pointer. These values govern the standards of TCP communication and guarantee effective, error-free interaction between connected devices.source
Below is a rudimentary Python code snippet showing how to create a TCP client using Python’s socket module:
import socket def create_tcp_client(server_ip, server_port): # Create a socket object s = socket.socket(socket.AF_INET, socket.SOCK_STREAM) # Connect to the server s.connect((server_ip, server_port)) # Send data s.sendall(b'Hello, Server') # Receive response data = s.recv(1024) # Close the connection s.close() print('Received', repr(data))
As expected, understanding how a TCP message is sent provides valuable insight into network communication protocols, their optimization process, and principles. By unveiling these layers, professionals can design innovative software solutions to handle networking tasks more effectively and efficiently.Sure, the transport of a TCP (Transmission Control Protocol) message over a network rides on its unique anatomy. For data to be transported successfully from one node to another in a reliable, error-free manner, a well-structured TCP message is key. This entire data transporting procedure is often defined as ‘TCP Message Sending’.
The structure of a TCP message, also known as a ‘Segment’, comprises several components:
The TCP Header
The TCP header is a critical part of the segment that accompanies the actual data payload. It contains important fields including source and destination port numbers, sequence numbers, acknowledgment numbers, among others. Below is a visual representation of the TCP Header.
Source Port | Destination Port |
---|---|
Sequence Number | Acknowledgment Number |
Data Offset | Reserved |
Flags | Window Size |
Checksum | Urgent Pointer |
Options | Padding |
You might ask why these header fields matter in sending TCP messages. Here’s how they’re instrumental in TCP message transmission:
✔ Source Port and Destination Port: These port numbers help identify the specific applications or processes communicating over a network.
✔ Sequence Number: This number is used by servers to assemble packets in the right order when they arrive out-of-order due to network issues.
✔ Acknowledgment Number: The receiver uses this field in TCP to inform the sender about which packets it has received without corruption or loss. It lets the sender know if any packets need to be retransmitted.
✔ Data Offset, Reserved, Flags, Window Size and Checksum: All these fields together manage the control information like data synchronization, delivery acknowledgment, data flow control, etc; effectively bolstering the reliability of TCP communication.
Data Payload
The data payload carries the actual message or data that is to be transmitted from the sender to the receiver. ASCII format is commonly used for encoding the data during transfer.
Here’s a simple diagram representing the construction of a TCP Segment.
---------------- | TCP Header | ---------------- | Data Payload | ----------------
The process of sending a TCP message consequently involves the formation of these TCP segments with proper header values followed by their transmission through the Internet Protocol (IP). The receiving endpoint uses the header details from each segment to construct the original message ensuring data integrity and correctness.
Remember though, TCP doesn’t operate alone. Instead, it works in combination with various other protocols within the internet protocol suite to ensure smooth and reliable delivery of messages across networks. Examples include HTTP, HTTPS, FTP and SMTP; all of who owe their efficient functionality to the robustness of TCP.
For further understanding about TCP internals, you can refer to this IETF document.When discussing the TCP (Transmission Control Protocol) messaging process, we mustn’t sidestep the importance of a fundamental component: the Three-Way Handshake. Emphasizing its brilliance, the Three-Way Handshake is a cornerstone in establishing a reliable and ordered flow of data packets between network devices.
So, why is this three-step dance so vital? Let’s delve deeper:
It Establishes the Connection:
TCP is connection-oriented, which means that it sets up a dedicated connection before transmitting data. The ‘SYN’ packet kicks off the handshake; it carries the initial sequence number from the sender to the receiver and specifies the sender’s willingness to establish a connection. Upon receiving the ‘SYN,’ the recipient sends back a ‘SYN-ACK,’ acknowledging the establishment readiness. Finally, the original sender responds with an ‘ACK,’ thereby solidifying a successful connection attempt.
Here’s a snapshot of a TCP Connection establishment using three-way-handshake:
Sender | Receiver |
---|---|
Sends SYN | Receives SYN |
Receives SYN-ACK | Sends SYN-ACK |
Sends ACK | Receives ACK |
Session Synchronization:
The Three-Way Handshake isn’t merely a networking formality – it serves to synchronize the TCP communicating entities, especially regarding sequence numbers. These play a pivotal role in sequencing the order of data packet transmission, ensuring no missing or out-of-order packets disrupt communication. This synchronization helps maintain orderly and reliable communication over TCP networks, thereby drastically reducing potential data corruption or loss.
Flow & Congestion Control:
Congestion can easily disrupt data transmission over a network, leading to delayed or dropped packets. TCP has built-in mechanisms that control the rate of data transmission based on network capacity. Because of the feedback loop established during the handshake (with ‘SYN’, ‘SYN-ACK’, and ‘ACK’), both sender and receiver agree on parameters like window size, specifying how many bytes can be sent before requiring acknowledgment. This method adds a layer of robustness to TCP, adding its resilience amidst varying network conditions.
Error Checking:
Finally, every TCP header carries a checksum, providing a mechanism for error detection. During the handshake phase, the systems exchange these headers, checking for errors. If an error occurs during transmission, the connection establishment fails, preventing further incorrect transmissions.
For a quick reference illustrating a simple Three-Way Handshake implementation in Python, check out Python TCP Implementation. Again, remember that proper understanding and utilization of this hands-on guide requires a fair grasp of basic python syntax.
Overall, it’s clear to see: TCP messaging isn’t a hit-or-miss affair. It’s a meticulously orchestrated festival, where the Three-Way Handshake makes sure everyone’s in tune before the grand symphony begins. Remember, without this, TCP messaging would lose its reliability, efficiency, and speed. So when you send your next TCP packet, take a moment to appreciate the elegant little dance of network synchronicity happening under the hood.TCP – Transmission Control Protocol, is a crucial aspect in the landscape of computer network communications. It plays its role by defining how data communicates between different devices on the internet. TCP carefully scrutinizes and oversees that all packets are safe, ordered, and uncorrupted.
Why Being TCP Aware?
Understanding TCP segments can equip us with valuable insights into aspects like performance optimization, network troubleshooting, and securing your applications from potential vulnerabilities. I’d say it’s an extremely useful thing to do, especially for a programmer or network administrator.
Let’s dive into the TCP world.
Field | Description |
---|---|
Source Port | Indicates the source port# |
Destination Port | Indicates the destination port# |
Sequence Number | For sequencing the order of sent packets |
Acknowledgment Number | For acknowledging received packets |
Data offset | Specifies the size of header part |
Reserved | Reserved for future use |
Control Bits/ Flags | Various control bits like SYN, ACK, FIN, etc. |
Window | Indicates the window size acceptable |
Checksum | To check for communication explicit errors |
Urgent Pointer | Pointing urgent data if URG flag is set |
Options & Padding | Additional options and padding to ensure segment end |
TCP Data Packaging: How A TCP Message Is Sent?
Here is how the process goes:
1. To send a message over TCP, first, the data is broken down into small chunks, called ‘segments.’
2. Each segment contains a header (
byte[0-19]
) and the actual data (also known as the payload).
3. The encapsulation takes place at the sending device where each TCP segment gets wrapped up within an IP packet before its journey towards the receiver.
4. At the receiver’s end, the IP layer decapsulates the packet, obtaining the original TCP segment. Then data is ordered according to the sequence numbers and delivered upwards to the application layer.
Take a look at the illustrative code below which opens a socket connection to handle a TCP transmission:
import socket def send_tcp_message(message, server_address): sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM) sock.connect(server_address) try: sock.sendall(message) finally: sock.close()
In everyday programs and systems, this process happens countless times without us even noticing. It’s quite interesting to perceive what transpires behind just a simple click or press of a button.
By comprehending how data packaging and transmission occurs via TCP segments, we’re not just becoming more technically sound professionals but also building our foundation for complex network communications and security concepts.
It’s always great to have this technical prowess under your belt in the fast-paced and ever-evolving field of IT and programming. With this understanding, you can now create, debug and optimize network applications more effectively.
Some valuable resources for further reading:
* RFC 793: Transmission Control Protocol
* Wikipedia: TCP Segment structureSure, let’s take a deep-dive into how TCP (Transmission Control Protocol) messaging works, where sequencing and acknowledging packets come into play.
When sending data over the Internet, it’s broken down into smaller units called packets. For TCP, each packet is assigned a unique sequence number that identifies its position in the overall data stream. This process ensures that the receiving system can reassemble the packets in the correct order.
**Sequencing Packets in TCP**
In TCP, the sequence number for the first byte of data is chosen randomly when a connection is established between two systems. Afterward, the sequence number for each subsequent packet is incremented according to the length of the data in the last packet.
TCP follows these steps to sequence packets:
– A packet’s sequence number is essentially the cumulative count of all preceding bytes (visible to TCP) in the same TCP stream.
– When a data segment isn’t the first of its TCP stream, its sender gets the sequence number by summing the sequence number used in the previous data segment and the length of data in that previous segment.
– The receiver uses this sequence number to order the received segments correctly and to eliminate any duplicate segments.
Here is an example demonstrating how the sequence numbers increment in a TCP data stream:
code
TCP Stream: [packet1:100 bytes] -> [packet2:200 bytes] -> [packet3:50 bytes]
Sequence Numbers: [packet1:1] -> [packet2:101] -> [packet3:301]