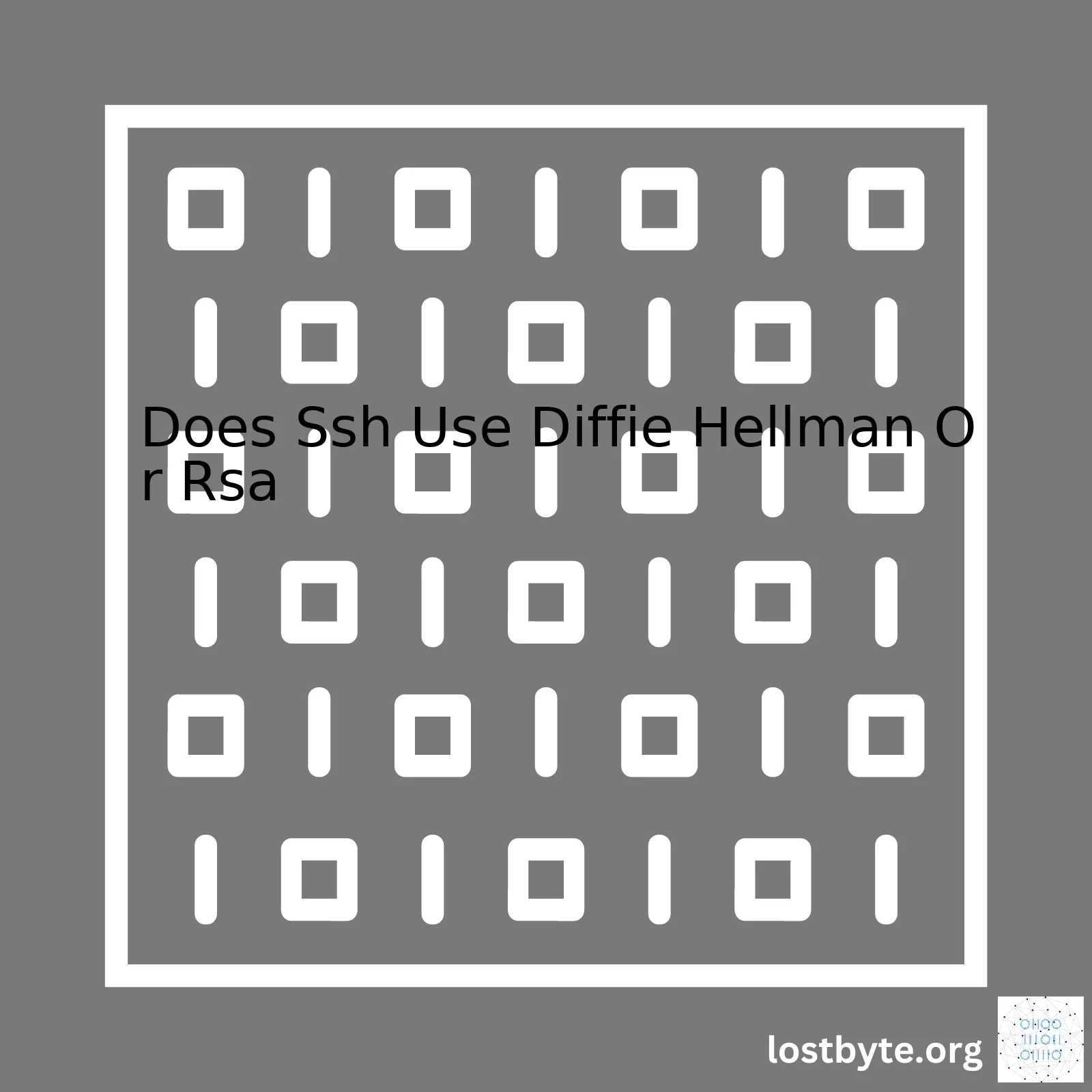
Protocol | Usage in SSH |
---|---|
Diffie-Hellman | Used during the SSH handshake for key exchange |
RSA | Used as a method for authenticating identity |
In analyzing whether SSH (Secure Shell) uses Diffie-Hellman or RSA (Rivest-Shamir-Adleman), it’s critical to note that these cryptographic tools are not mutually exclusive, but rather work together to enhance secure communications. SSH makes use of both protocols at different stages of the communication process.
At the beginning of an SSH session, the participants perform a protocol handshake. Here, the Diffie-Hellman algorithm plays a powerful role. The strength of this algorithm lies in enabling two parties, over an insecure channel, to establish a shared secret key known only to them. This secret key then serves as the basis for symmetric encryption of the actual data transmission, keeping the exchanged data confidential.
/* Example pseudocode demonstrating the Diffie-Hellman Key Exchange */
Alice_and_Bob_public = generate_diffie_hellman_params()
Alice_secret = generate_random_secret()
Bob_secret = generate_random_secret()
Alice_public_share = calculate_public_share(Alice_secret, Alice_and_Bob_public)
Bob_public_share = calculate_public_share(Bob_secret, Alice_and_Bob_public)
Alice_final_secret = calculate_final_secret(Alice_secret, Bob_public_share, Alice_and_Bob_public)
Bob_final_secret = calculate_final_secret(Bob_secret, Alice_public_share, Alice_and_Bob_public)
assert Alice_final_secret == Bob_final_secret // They now share a common secret
On the other hand, RSA is typically used in SSH for user authentication. Once the ephemeral keys have been exchanged using Diffie-Hellman, RSA steps in. The client combines its private key with the server-provided random challenge, creating a digital signature. The server can validate this by using the public key corresponding to the client’s private key—the one it has on record. If the server successfully verifies the signature, it means the client demonstrated possession of the correct private key, thereby confirming their identity.
xxxxxxxxxx
/* Example pseudocode demonstrating RSA Authentication */
challenge = server_provides_random_challenge()
signature = create_signature(private_key, challenge)
isAuthenticated = verify_signature(signature, public_key, challenge)
if isAuthenticated:
allow_access()
else:
deny_access()
When you decide between using Diffie-Hellman or RSA within SSH, remember that they do not replace each other but instead, they synergistically contribute toward the overall security architecture.
For more details on how SSH utilizes these cryptographic algorithms, I recommend checking out resources such as this SSH Protocol Architecture document from IETF. It details the inner workings of SSH communications and gives an even deeper look into how Diffie-Hellman and RSA play important roles in SSH.To understand how SSH operates and whether it uses Diffie-Hellman or RSA, we need some context about these cryptographic protocols.
Secure Shell (SSH) is a cryptographic network protocol that permits a secure channel between two systems over an insecure network. It’s primarily used to access login sessions from remote machines, but it also supports other secure network features like secure file transfers and network component tunnelling.
As part of its data encryption strategy, SSH employs two distinct types of key-based cryptography:
1. Symmetric Encryption (also known as Secret Key Cryptography)
2. Asymmetric Encryption (also known as Public Key Cryptography).
While symmetric encryption uses one shared key to both encrypt and decrypt information, asymmetric encryption employs two linked keys: one public and one private. Each can decrypt what the other has encrypted.
Now, let’s delve into Diffie-Hellman and RSA.
Diffie-Hellman
The Diffie-Hellman Key Exchange (DH) is a method for securely exchanging cryptographic keys over a public network. It allows for the negotiation of a shared secret key over an insecure communication channel without prior knowledge of each other’s keys. The key created by the Diffie-Hellman exchange can then be used as a symmetric encryption key.
An example of Diffie-Hellman can be shown in the python code below:
xxxxxxxxxx
import secrets
p = 23
g = 5
alice_secret = secrets.randbelow(1000)
bob_secret = secrets.randbelow(1000)
A = (g**alice_secret) % p
B = (g**bob_secret) % p
alice_shared_key = (B**alice_secret) % p
bob_shared_key = (A**bob_secret) % p
print(f"Alice's Shared Key: {alice_shared_key}")
print(f"Bob's Shared Key: {bob_shared_key}")
RSA
On the other hand, RSA (Rivest-Shamir-Adleman) is an algorithm used by modern computers to encrypt and decrypt messages. It is an asymmetric cryptographic algorithm because it uses a pair of keys: a public key for encryption and a private key for decryption.
Here is an example of how RSA works in Python:
xxxxxxxxxx
from Crypto.PublicKey import RSA
key = RSA.generate(2048)
private_key = key.export_key()
file_out = open("private.pem", "wb")
file_out.write(private_key)
file_out.close()
public_key = key.publickey().export_key()
file_out = open("public.pem", "wb")
file_out.write(public_key)
file_out.close()
Does SSH Use Diffie Hellman Or RSA?
The answer is: SSH uses both Diffie-Hellman and RSA, but for different purposes. Here is how they work together:
The RSA algorithm is used for Authentication. When a client connects to an SSH server, the server presents an RSA public key to the client. The client checks if this key has been seen before and if so, continues with the connection.
If authenticated successfully, the client and server will use the Diffie-Hellman Key Exchange to generate a random, one-time use symmetric key that both sides know, but never transmitted. This is used for the actual data encryption of the SSH Connection.
Therefore, when you connect through SSH to a server, you are using both RSA and Diffie-Hellman at different stages for different purposes – RSA for authentication and Diffie-Hellman for establishing the encrypted connection.
References :
Definitely, the Secure Shell (SSH) protocol engages both the Diffie Hellman and RSA algorithms in its core operation. For every SSH connection, two primary security functions come into play. The major ones are:
– User authentication
– Key exchange
But let’s dig deep in order to understand in what context and how these protocols are used with SSH.
How SSH uses RSA for User Authentication:
RSA is primarily involved in the user authentication procedure. SSH employs RSA keys as part of its public key infrastructure for confirming the identity of a client to an SSH server.
Here’s how RSA works within SSH:
– Firstly, the client generates an RSA key pair by choosing a random number and using it to encrypt a message.
– Then, the matching decryption key is left on the SSH server.
When a client looks to initiate a connection, the server challenges it by sending a unique number. The client encrypts this number with their private RSA key and sends it back to the server. If the server can decrypt the reply using its stored RSA key and get the original number, this verifies the client’s identity.
This simplistic code highlights how an RSA key pair might be generated and deployed:
xxxxxxxxxx
$ ssh-keygen -t rsa
$ cat ~/.ssh/id_rsa.pub | ssh username@hostname 'cat >> .ssh/authorized_keys'
It is always great to reference from reliable sources. You can understand more about SSH RSA user authentication by checking out this RFC document.
How SSH uses Diffie Hellman for Key Exchange:
Diffie-Hellman comes into effect during the SSH key exchange act, which executes at the commencement of every new SSH session.
This process delineate hereafter:
– Both the client and server generate temporary pairs of Diffie-Hellman keys (private and public).
– They then mutually swap their DH public keys.
– Afterward, they independently employ their own DH private key and the counterparty’s DH public key to compute a common shared secret.
This extra step provides an additional security layer, often known as “forward secrecy”. Even if a third party gets hold of the long-term RSA private key of an SSH user, they still wouldn’t manage to decipher the keys or data directly transmitted over any specific SSH connection as these are protected with the temporary DH keys.
Following python-esque pseudo-code demonstrates the basic mechanism behind Diffie-Hellman key exchange method:
xxxxxxxxxx
def dh_key_exchange(public_key1, private_key1, public_key2):
shared_secret1 = compute_shared_secret(private_key1, public_key2)
shared_secret2 = compute_shared_secret(private_key2, public_key1)
return shared_secret1 == shared_secret2
Diffie Hellman mechanism in SSH has further variants – most notably,
– Classic Diffie-Hellman key exchange (“dh-group1-sha1”)
– Elliptic-Curve Diffie-Hellman (“ecdh-sha2-nistp256”).
More granular details on How SSH Uses Diffie-Hellman can be found in this RFC document.SSH (Secure Shell) protocol uses both Diffie-Hellman and RSA algorithms, depending on the context and the phase of establishing a secure connection. To answer this question in great detail, let’s first explain how the SSH protocol operates.
SSH initiates its operation by identifying the server to the client using RSA or another digital signature algorithm. This process ensures that the computer you’re connecting to is legitimately who it says it is. This is typically done via RSA keys. As part of this identification phase:
- The server shows its public key.
- The client then uses this public key to encrypt a random number and sends it back to the server.
- Only the server, with its private key, can decrypt this random number. Thus, proving its identity.
After completing this identification phase successfully, SSH then moves into the key exchange phase. At this point, it uses the Diffie–Hellman key exchange algorithm to create a shared secret between the client and the server without ever transmitting the secret across the network.
The steps involved are as follows:
- Both machines (the client and the server) agree on a large prime number, which doesn’t need to be secret.
- Each machine then picks another private prime number and generates a public piece of data (not necessarily a number).
- The public data is exchanged between the two machines, and they use it to compute a shared secret number.
- This shared secret can then be used as the key for a symmetric encryption algorithm, which is much faster than any available asymmetric algorithm.
In essence, the RSA is mainly used for authentication while the Diffie-Hellman Key Exchange enables each party to create a shared secret key which can be used further for symmetric encryption, thus maintaining a secure communication channel.
Therefore, we see that RSA and Diffie-Hellman work hand-in-hand in SSH connections. Authentication security is ensured through RSA while session privacy and integrity are achieved using Diffie-Hellman.
Here, I would like to attach a table for better comprehension:
Algorithm | Purpose |
---|---|
RSA | Used for authenticating the server’s identity to the client |
Diffie-Hellman | Used for exchanging keys to maintain a secure communication channel |
Finally, it’s worth noting that modern SSH implementations offer a variant of Diffie-Hellman that provides “perfect forward secrecy.” That means even if a session key were compromised, it would not lead to the compromise of past or future session keys.
Now, understand that this is just a basic overview. If you want to dive deeper into these cryptographic mechanisms, consider reading source materials like books about digital cryptography (link here) or relevant online documentation such as the RFCs that define the SSH protocol, such as RFC 4251, 4252, 4253.
For a brief demonstration on how to generate RSA and Diffie-Hellman keys:
xxxxxxxxxx
#Generating RSA Key
ssh-keygen -t rsa
#Generating Diffie-Helman Keys
openssl dhparam -out dhparams.pem 2048
Remember, the prime objective of such an elaborative step-by-step approach adopted by SSH is to ensure the greatest level of security during a remote connection. After all, in the world of cybersecurity, one can never be too careful!
Secure Shell (SSH) is a cryptographic network protocol that leverages different encryption methods to secure data communications over untrusted networks. Two significant operations underlie the security offered by SSH: Key exchange and server authentication. Here’s where RSA (Rivest-Shamir-Adleman) and Diffie-Hellman come into play.
A key exchange algorithm, such as Diffie-Hellman, is used for securely establishing a shared secret between two entities (like your local machine and an SSH server), which have never communicated before. This shared secret acts as the symmetric key for subsequent communication during the session.
xxxxxxxxxx
// Establish a connection
ssh -i /.ssh/id_rsa @
On the other hand, RSA comes into the picture in the SSH process through server-side authentication and, in certain configurations, for client-side authentication too. RSA or any other asymmetric cryptography method like ECDSA or Ed25519 is used in making public-private key pairs. The server holds the private key secretly and shares its public key.
For server authentication, the client verifies the host against a locally stored list of servers’ RSA keys. In cases where RSA-based user authentication is implemented(e.g., public key authentication), the client signs random data with their private key, and the server uses the related public key to verify the signature.
xxxxxxxxxx
// Generate RSA key pair
ssh-keygen -t rsa -b 4096 -C "your_email@example.com"
// Copy the public key to the server
ssh-copy-id -i ~/.ssh/id_rsa.pub @
RSA | Diffie Hellman | |
---|---|---|
Usage in SSH | Used for server and optionally client authentication | Used to securely establish a shared symmetric key |
Type of Cryptography | Asymmetric | Asymmetric |
Key Size | Usually 1024, 2048, or 4096 bits | Usually 1024, 2048, or 3072 bits |
So to conclude, SSH leverages both RSA and Diffie-Hellman in its operations: RSA for user and host verification ensuring you are connecting to the right server and not a malicious one and Diffie Hellman for securely creating a shared secret key between client and server for symmetric encryption of the actual transmission.
You can find further riches on this deep dive into cryptography in SSH at RFC 4253 – The Secure Shell (SSH) Transport Layer Protocol.
SSH, which stands for Secure Shell, is renowned for its role in providing secure access to remote systems. It uses different cryptographic algorithms to ensure confidentiality and integrity of data during transmission. SSH primarily uses public key cryptography, but it’s crucial to note that SSH can either be based on Digital Signature Algorithm (DSA) or the Rivest-Shamir-Adleman (RSA)[1]. However, I’d like to shed more light on how SSH utilizes Diffie-Hellman protocol for key exchange.
Let’s take a small detour to unwrap the workings of the Diffie-Hellman protocol. The brilliance of Diffie-Hellman lies in rendering feasible secure communication over a public channel. It does so by producing a shared secret between two parties, each having an associated pair of a private key (known only to itself) and a public key (open to other parties).
Now, let’s dive into how SSH makes use of this protocol through some concrete steps:
- The client initiates a connection with the SSH server.
- Both the SSH client and server generate temporary Diffie-Hellman key pairs.
- The client sends its public Diffie-Hellman key to the server.
- The server receives this public key, and then it creates a shared secret using its own Diffie-Hellman private key and the client’s public key.
- The client also calculates the same shared secret using its Diffie-Hellman private key and the server’s public key.
- This shared secret, which is now available to both the client and the server, becomes the basis for generating symmetric encryption keys used in that particular session. This way, both parties avoid transmitting the actual encryption key and reduce the risk of exposure.
Note: It is essential to mention that this protocol involves significant computations, elevating its performance cost. Therefore, these keys are generated per session basis, not for each message.
But where does RSA fit into all of this?
Well, the authentication step within the SSH protocol is where RSA shines. The client will prove its identity to the server using an RSA digital signature[2]. Here’s how this works:
xxxxxxxxxx
ssh-keygen -t rsa
This command generates an RSA key pair comprising a private key (id_rsa) and a public key (id_rsa.pub). You store the private key on the client machine, whereas you add the public key to
xxxxxxxxxx
.ssh/authorized_keys
file on the SSH server. During each login attempt, the client creates a digital signature using its RSA private key. At the receiving end, the server confirms this signature using the related RSA public key[3].
So in essence, SSH utilizes both Diffie-Hellman and RSA – RSA for authenticating user identity, and Diffie-Hellman for the secure exchange of symmetric encryption keys.
Secure Shell (SSH) is a protocol that provides secure shell services for remote network services. Underneath its shell, it employs several cryptographic algorithms to ensure the confidentiality and integrity of data as they traverse over insecure networks. The most common ones being RSA and Diffie-Hellman.
RSA and Diffie-Hellman serve different purposes in SSH. Here’s how each interacts within the context of an SSH session:
RSA and Diffie Hellman in SSH
RSA algorithm is primarily used in authentication while Diffie-Hellman is utilized in key exchange.
- RSA: Unlike most public key cryptosystems, the RSA algorithm has dual functions; it can carry out encryption and digital signature applications. In SSH, the client begins by presenting the server with its public key. If the server recognizes this key (meaning the corresponding private key is authorized), then the client proves its identity by encrypting some message with its private key, which can be decrypted with the public key. It lies in the proof that the client has access to the associated private key without actually revealing the private key itself during transit over the network.
- Diffie-Hellman: As a step-up from RSA in terms of security, SSH also employs the Diffie-Hellman key exchange protocol to establish a shared secret between two parties (in this case, the client and the server) over an insecure network. This shared secret can later serve as the symmetric encryption key for further communication, making it significantly more arduous for eavesdroppers to decode, even if they intercept all the transmitted messages.
An Example
To illustrate, here is an example sequence in setting up an SSH session:
Firstly, the client and server agree on the versions of SSH they’re going to use. When agreed, both sides initiate the key exchange process using Diffie-Hellman. They start off by publicly sharing their open numbers, but keep their private numbers to themselves.
xxxxxxxxxx
Client: "Let's use these values (client_public_key, g, p)"
Server: "Okay, I'll use my private number and we'll get this shared_secret"
Remember, this whole process stays secure because the Diffie-Hellman problem – computing the shared_secret knowing only the public keys – is difficult to solve unless you know at least one of the private numbers.
Next, the client authenticates itself to the server using an RSA type key. Typically, the client will have generated a pair of keys (public and private) and stored the private key in some secure location, while adding the public key to the server’s authorized_keys file.
xxxxxxxxxx
Client: "I'd like to authenticate myself with this RSA public key..."
The server checks if this key is within its authorized_keys store. If it exists, then the server knows that this client is possibly authorized, and hence, proceeds to challenge the client.
xxxxxxxxxx
Server: "If you are the legitimate owner, sign this random_number with your RSA private key!"
The client signs the number with its private key and sends back the encrypted message.
xxxxxxxxxx
Client: "Here you go! (encrypted_random_number)"
The server decrypts the message with the public key it has on file. If it matches with the initial random_number, then the client was able to successfully prove that it had the right private key and thus is authenticated.
And voila! An SSH connection is established and now the client and the server can engage in secure communication using the symmetric key derived from the shared_secret established in the Diffie-Hellman key exchange process.
In short, SSH uses both RSA and Diffie Hellman. RSA facilitates authentication (proving your identity to the server) and Diffie Hellman deals with confidentiality (keeping the information secret from potential eavesdroppers).
Encrypting the entire session using RSA would be quite slow due to the complex mathematic calculations involved in public key cryptography. By using Diffie-Hellman to negotiate a shared symmetric key, SSH makes sure the bulk of the data transfer during the session happens quickly and efficiently while still maintaining a strong level of security.
If you are interested in learning more about these cryptography systems, check the link here for RSA, and this link here for Diffie–Hellman.
The Secure Shell Protocol (SSH) is a secure remote login protocol that utilizes encryption to protect network communications from compromise. Both RSA and Diffie-Hellman play crucial roles in the SSH public key infrastructure but serve different purposes.
Diffie Hellman: The Diffie-Hellman algorithm, named after Whitefield Diffie and Martin Hellman, is a method of generating a shared secret between two parties over an insecure network, and thus establishing an encrypted communication channel. It’s widely used during the initial key exchange in the SSH handshake.
xxxxxxxxxx
# A typical Diffie-Hellman Key Exchange:
# Alice and Bob generate their respective pairs
A = g**a mod p; B = g**b mod p
# Alice sends A (public key) to Bob, and Bob sends B to Alice
exchange(A, B)
# Alice computes s = B**a mod p (Bob's public message raised to her own private value)
# Bob computes s = A**b mod p (Alice’s public message raised to his private value)
s_a = compute_s(B, a); s_b = compute_s(A, b)
# If all goes according to plan, s_a should equal s_b
assert s_a == s_b
RSA: RSA (Rivest-Shamir-Adleman) is another critical cryptographic algorithm frequently used in SSH. While not typically used like Diffie-Hellman for key exchange, its main role lies in user authentication and host verification.
xxxxxxxxxx
# A very basic implementation of RSA:
def generate_keypair(p, q):
# n is the modulus for the keys
n = p * q
e = find_e(n, phi)
d = find_d(e, phi)
return ((e, n), (d, n))
generate_keypair(61, 53)
# Once you have keys generated by something like this,
# You can use them to encrypt & decrypt messages
encrypted_msg = encrypt(public_key, "Hello World!")
decrypted_msg = decrypt(private_key, encrypted_msg)
Comparative Analysis of Diffie-Hellman versus RSA:
While comparing these two algorithms, they both have their unique benefits and are used for different purposes in SSH:
• Key Generation: In Diffie-Hellman, new keys are generated for every session and aren’t stored for any future sessions. With RSA, a single pair of keys is used indefinitely, raising more potential risk if a key is compromised.
• Encryption/Decryption: Diffie-Hellman is solely used for key exchange and doesn’t offer an encryption/decryption facility. On the contrary, RSA can encrypt and decrypt data.
• Authentication: RSA shines brighter than Diffie-Hellman, as it allows digital signatures for authenticating the sender, ensuring better integrity.
So, when considering the question: “Does SSH use Diffie Hellman or RSA?”, the optimal answer is both. The SSH protocol employs Diffie-Hellman to establish a shared secret key between the client and server for the session and uses RSA for authentication, maintaining the integrity of the communication.
For further understanding, feel free to delve into the official documentation of the RFC 4253 – SSH Transport Layer Protocol standard.Secure Shell (SSH) is a cryptographic network protocol for secure communication over an insecure network. A critical aspect of Secure Shell is its key management, which involves generating, distributing, and storing cryptographic keys. SSH makes use of two main types of key algorithms: RSA and Diffie-Hellman.
When we talk about RSA and SSH, we need to understand that RSA has two broad usages: key generation/exchange and authentication.
For key exchange, though widely used in the past, RSA does not see much usage in newer versions of OpenSSH, like OpenSSH 7.0 upwards, due to inherent security issues. Instead, SSH now uses the Diffie-Hellman algorithm, more specifically, an elliptic curve version called ECDH, for key exchange or session key establishment.
Key Exchange | RSA | Diffie-Hellman |
---|---|---|
Usage in SSH | Rarely used for modern versions of SSH | Used by default (ECDH) |
Security | Vulnerable to certain attacks | More secure than RSA for this purpose |
Let’s look at RSA’s continued relevance in SSH: authentication. Once a secure connection has been established using some variant of Diffie-Hellman, RSA is often used to authenticate the server to the client, using a digital signature. In the authorization process, the server identity is verified by the client through RSA.
Authentication | RSA |
---|---|
Usage in SSH | Commonly used |
Security | Considered secure for this purpose |
To illustrate how this works, let’s break it down:
- The client requests a connection from the server.
- The server provides its public key.
- The client verifies it against known hosts.
- A session key is negotiated via ECDH.
- The server uses its private RSA key to sign the handshake, proving its identity.
You can create an RSA key pair using commands such as:
xxxxxxxxxx
$ ssh-keygen -t rsa
And then distribute it to the clients you want the server to be recognized by:
xxxxxxxxxx
$ ssh-copy-id user@host[1]
From these highlights, it’s clear that both RSA and Diffie Hellman have critical roles in SSH key management, with Diffie-Hellman (specifically ECDH) mostly handling the safe creation of a shared session key, while RSA sees primary use in authenticating the server to the client. Thus, when exploring SSH key management, recognizing the distinct roles and functions of these algorithms proves crucial.
To gain a deeper understanding of SSH key management, consider delving into resources such as the [OpenSSH man page](https://man.openbsd.org/OpenBSD-current/man1/ssh-keygen.1) and complimentary online cryptography courses.
Remember, maintaining strong and secure key management practices is critical when dealing with sensitive data and communications in computer programming.
Sources:
– [OpenSSH man page](https://man.openbsd.org/OpenBSD-current/man1/ssh-keygen.1)
– [RFC 4253 – The Secure Shell (SSH) Transport Layer Protocol](https://tools.ietf.org/html/rfc4253)
– [StackExchange Cryptography – Why RSA is used in SSH for signing and verifying](https://crypto.stackexchange.com/questions/27459/why-rsa-is-used-in-ssh-for-signing-and-not-for-encryption)
– [IBM Community – Understanding SSH Key Pairs](https://www.ibm.com/cloud/blog/understanding-ssh-key-pairs)
[1]: This will copy your public key to the host ‘user@host’ to allow the host to recognize your machine.
Yes, Secure Shell (SSH) does make use of the Diffie-Hellman key exchange algorithm, which is essential in setting up a secure communication channel. Essentially, SSH primarily uses RSA for creating and validating digital signatures but it can also support DSA and ECDSA.
When establishing an SSH connection, this involves several important steps:
1. Version Exchange: The client initiates the session by sending the protocol version string to the server.
2. Algorithm Negotiation: The client follows up by sending a key-exchange-init message with its choice of algorithms for key exchange, hostkey type (RSA, DSA, ECDSA), encryption, etc.
3. Key Exchange: Depending on the algorithm agreed upon in the previous step, either RSA or Diffie-Hellman procedure will be used to securely exchange keys. In most cases, Diffie-Hellman is used as it’s safer for ephemeral key exchanges.
When employing Diffie-Hellman within an SSH context, it’s termed as Ephemeral Diffie Hellman (DHE). Its functionality revolves around generating a one-time key for every SSH session.
Here’s a basic implementation of how an SSH connection setup would look like in Python, utilizing the Paramiko library which, internally, employs Diffie-Hellman for key exchange:
xxxxxxxxxx
import paramiko
ssh = paramiko.SSHClient()
ssh.set_missing_host_key_policy(paramiko.AutoAddPolicy())
ssh.connect('hostname', username='username', password='password')
In this code snippet, Paramiko handles all the complexities of SSH including the key exchange part. It’s worth noting that while both Diffie-Hellman and RSA are used in SSH, they serve different purposes. RSA is primarily used for authentication and assurance of data integrity while Diffie-Hellman is used for key exchange and maintaining secrecy of communication.
Key exchange parameters in SSH can be altered based on requirements, allowing usage of either RSA or Diffie-Hellman where needed. Thus, to answer your question succinctly – Yes, SSH does use both Diffie-Hellman and RSA depending on the specific stage of secure communication setup.
For detailed understanding on SSH protocol workings, refer online resources like [RFC 4253](https://tools.ietf.org/html/rfc4253): The Secure Shell (SSH) Transport Layer Protocol which provides a comprehensive overview about the inner workings of SSH.SSH (Secure Shell) protocol can utilize both RSA and Diffie-Hellman algorithms in establishing secure communication channels. However, their application differs based on the specific part of the SSH session.
The SSH handshake protocol involves two major parts:
1. Authenticating the server to the client
2. Establishing a shared symmetric encryption key.
RSA is typically used in the server authentication phase where the server presents a public key, and the client decrypts it with the stored public key to ensure that it’s dealing with the legitimate server. In this scenario, RSA ensures that only the valid server can read the information since only it possesses the corresponding private key.
An example of how RSA authentication works can be illustrated below:
xxxxxxxxxx
/* Server sends its public key */
Server: "My public key is xxx"
/* Client encrypts a random string using the public key and sends it back, which can only be decrypted by the owner of the private key */
Client: Encrypt("random string", xxx)
/* Server returns the decrypted message to prove ownership of the matching private key */
Server: Decrypt(Encrypted Message)
Diffie–Hellman, or some variant like Elliptic Curve Diffie-Hellman (ECDH), on the other hand, is used for creating a shared symmetric key for the session without directly sending it over the network. This process adds another layer of security.
Here is a brief illustration of the Diffie-Hellman algorithm at work:
xxxxxxxxxx
/* Both parties agree on a global large prime number p and a base g */
Global: Agreed Prime - p, Base - g
/* Both parties generate their secret numbers a and b then compute A and B respectively */
Client: A = (g^a) mod p
Server: B = (g^b) mod p
/* They exchange A and B. The secret shared key can now be computed as: */
Client: Shared Secret Key = (B^a) mod p
Server: Shared Secret Key = (A^b) mod p
In conclusion, the answer to whether SSH uses RSA or Diffie-Hellman is: it actually uses both, but for different purposes. RSA is used to verify server authenticity, while Diffie-Hellman (or its variant) is employed to securely establish a shared secret key.
For deeper explanations with more thorough examples, you may want to check SSH Protocol Documentation by OpenSSH[1] and RFC 4251[2].SSH, short for Secure Shell, is a cryptographic protocol used to securely access remote systems. The most popular SSH key exchange methods are RSA and Diffie-Hellman (DKH). Now, let’s talk about the advantages and disadvantages of using DKH with your SSH protocol, as well as looking at whether it uses Diffie Hellman or RSA.
?
Using Diffie-Hellman With Your SSH Protocol: Advantages
- Perfect Forward Secrecy: One significant advantage of using DKH with SSH protocol is that it provides Perfect Forward Secrecy (PFS). This means even if a hacker manages to compromise one session key, they cannot use it to decipher past or future sessions. This adds an extra layer of security compared to RSA.
- Ephemeral Keys: Every time an SSH session is initiated using the Diffie-Hellman algorithm, a new session key gets generated. By continuously changing the keys, it makes it difficult for anyone to track your data.
- Greater Security: Generally speaking, DHK offers greater security than RSA when both keys are at the same length. Thus, for similar levels of security, DHK requires less computational resources.
Using Diffie-Hellman With Your SSH Protocol: Disadvantages
However, there are also downsides to consider:
- Computationally Intensive: One of the major drawbacks of using Diffie-Hellman within the SSH protocol is that it can be computationally intensive, leading to slower connection times.
- No Signature Algorithm: Diffie-Hellman itself doesn’t offer any sort of signature algorithm. This isn’t necessarily a disadvantage, but it does mean that SSH has to combine Diffie-Hellman with DSA or other algorithm to authenticate the server to the client.
Does SSH Use Diffie-Hellman or RSA?
Both! That might surprise you, but SSH actually uses both RSA and Diffie-Hellman. How exactly?
Here’s how:
xxxxxxxxxx
ssh-keygen -t rsa
This command line will generate RSA keys for authentication.
Then, the SSH protocol uses Diffie-Hellman during the SSH handshake to create the symmetric key used to encrypt the actual SSH session.
So, SSH makes use of both of these cryptographic methods to best protect data transmission – RSA for initial authentication, and Diffie-Hellman to ensure the security of the ongoing session.
Just remember, either algorithm has pros and cons. It’s essential to understand what kind of security aspects you prioritize before you choose which one to use with your SSH protocol.
References:
– Diffie-Hellman Key Exchange (ssh.com)
– Configure ASA SSH with AES and Diffie-Hellman Group 14 Compatibility (cisco.com)
Both RSA and Diffie-Hellman are pivotal when SSH (Secure Shell) sets up secure connections, but their uses differ greatly. Let’s delve deeper into their roles within the context of SSH.
Role of RSA in SSH:
RSA is a public-key encryption algorithm that belongs to the broader set of asymmetric cryptography systems. This algorithm holds a unique place in SSH for the sheer purpose of authentication. RSA works on the principle of factorizing huge numbers into their primes which counteracts the attempts of intercepting data by attackers. A vital characteristic of RSA in this scenario is its provision of digital signatures which verifies identity and maintains data integrity.
Role of Diffie-Hellman in SSH:
Conversely, the Diffie-Hellman key exchange protocol comes into play while creating shared secrets, integral for symmetric key encryption. That explains why SSH primarily embraces Diffie-Hellman instead of RSA during the setup of secure communications; it provides ‘forward secrecy’, keeping past data confidential even if the private keys get compromised later.
xxxxxxxxxx
// An example of how Diffie-Hellman is used in SSH:
Server and Client agree using Diffie-Hellman to an encryption level.
They both prepare public-private key pair.
They then swap public keys.
Now both sides can generate an identical key for future communication,
As they own each other’s public key and their own private key.
Lastly, kindly bear in mind that protocols are subject to customization according to specific security requirements or performance. Some flavors of SSH might choose to incorporate the Elliptic Curve version of Diffie-Hellman or potentially utilize DSA instead of RSA for authentication. It’s all about finding the balance between speed, compatibility and protection.source.