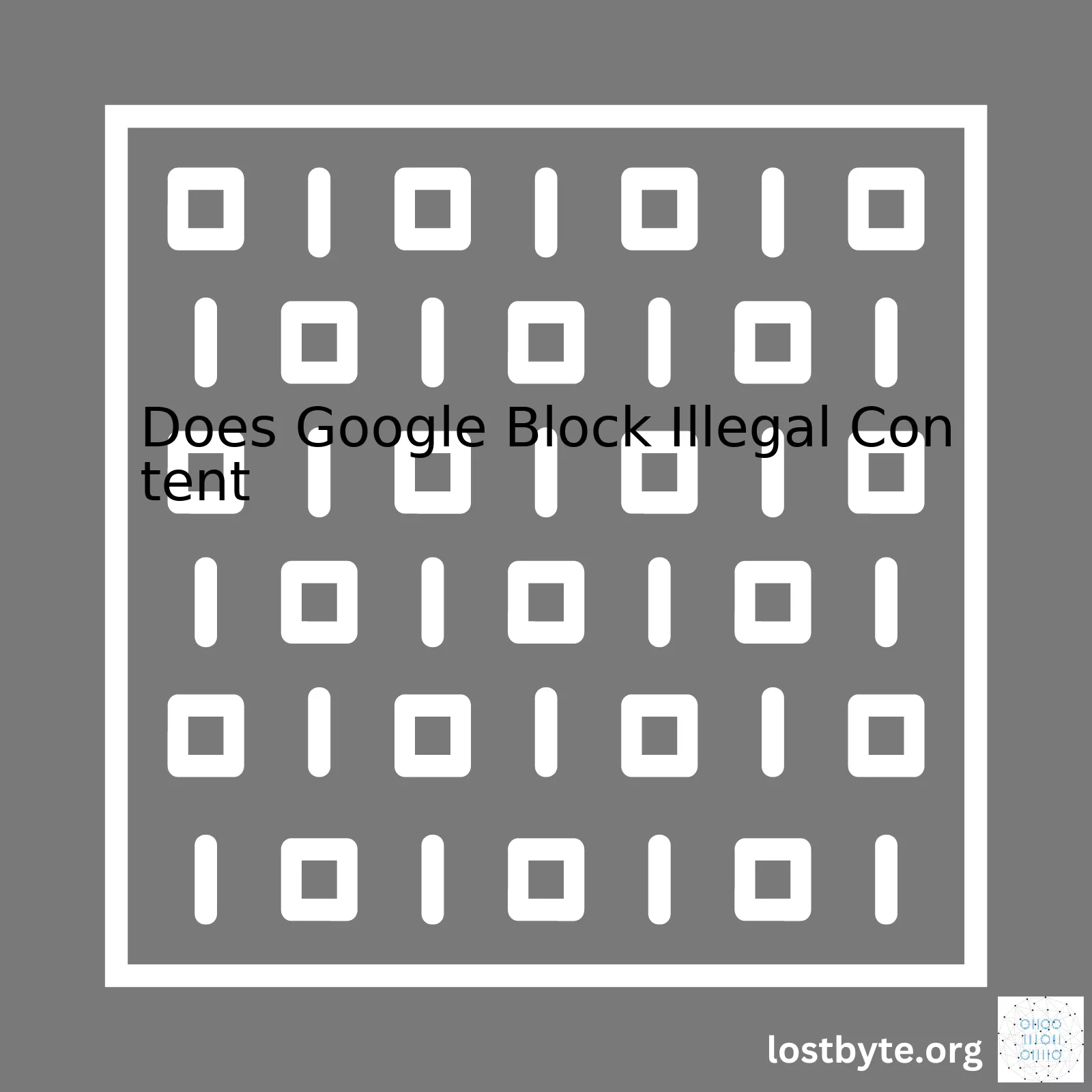
Policy | Description |
---|---|
Copyright Infringement | Google removes specific URLs reported for copyright infringement. |
Child Sexual Abuse Material (CSAM) | Google has zero tolerance towards CSAM. Any reports related to it are immediately reported to relevant authorities. |
Explicit Content Involving Minors | It’s prohibited by Google to show sexually explicit content featuring minors. |
Violent or Graphic Content | Google prohibits promotion of violence or graphic content intended to shock or disgust viewers. |
Hateful Content | Google doesn’t support content that incites hatred against any individual/group based on race, religion, etc. |
The Google search engine is programmed with a strict content policy in place targeting illegal activities. It uses advanced algorithms and user reports to detect and remove unlawful content. However, with the continual addition of new online domains and content, complete eradication is not always instantaneous but involves diligent and real-time efforts.
For instance, instances of copyrighted material are addressed via the Digital Millennium Copyright Act (DMCA) process, where Google removes specific URLs that infringed upon one’s copyrighted work when reported correctly.
Google’s Support Page details the submission process for these complaints.
Similarly, they have adopted a firm stance against Child Sexual Abuse Material (CSAM). If such material is found or reported, Google will not only remove it, but will also report the incident to the appropriate governing bodies like the National Center for Missing & Exploited Children (NCMEC), assisting law enforcement agencies in their proceedings.
Violent or graphic content, as well as content promoting harm or hatred towards individuals or groups, is also blocked from showing up in the search results, maintaining an environment conducive to respect and mutual trust among users.
In code language, if the specific case matches any policy violation, Google removes it effectively from its search engine index:
// Given URL is "url" // Google's policy set Set<String> policies = getGooglePolicies(); for(String policy : policies) { if(url.matches(policy)) { // Remove URL from search index googleSearch.removeUrlFromIndex(url); break; } }
It’s important to remember that despite the numerous restrictions put into place by Google, some illegal content may still manage to slip through due to the vastness of the internet sphere and technological limitations. That’s why Google highly appreciates users reporting content that violates its terms of service or local laws.
Google plays a significant role in combating online illegal content. Striving to provide a safe and secure internet experience for users, Google has implemented sophisticated techniques and protocols to detect, report, and remove illicit material surfacing on the internet.
Role of Google in Blocking Illegal Content
Primarily, Google uses proprietary algorithms and AI tools to monitor and scrutinize web content across various platforms – search engine, YouTube, Blogger, etc., vigorously.
- Automated Flagging: Google uses Machine-Learning Enhanced Techniques for automatically identifying and flagging potentially unlawful content.
- User Reporting: Google provides robust mechanisms that allow users to report illegal content encountered across any of its platforms.
- Transparency Reporting: Google publishes biannual Transparency Reports”, detailing government requests to remove content, user data requests, and breakthroughs in their fight against inappropriate and unlawful content.
Despite these efforts, Google’s task isn’t easy. The sheer volume of digital content uploaded every second makes it virtually impossible to stop all unlawful content as soon as it emerges. These systems aren’t infallible, and sometimes errors occur, leading to either false positives (blocking innocuous content) or false negatives (not blocking actual illegal content).
Handling Different Types of Illegal Content
Here’s how Google handles different types of illegal content:
- Child Sexual Abuse Material (CSAM): Google engineers created a cross-industry database containing ‘digital fingerprints’ of child sexual abuse images. Whenever someone tries to upload such an image, it is matched with the database and gets automatically blocked. They also report this material to the National Center for Missing & Exploited Children.
- Terrorist Material: A grouping of tech companies, including Google, Facebook, Twitter, and Microsoft, established the Global Internet Forum to Counter Terrorism where they share technology and information to block terrorist propaganda.
- Copyright Infringement: Google’s Content ID system on YouTube automatically checks uploaded videos against a database of files submitted by content owners. Once detected, they offer copyright holders the options to block, monetize, or track those videos.
The Hash Function Server Side Code
I’ll give you an insight into how Google’s hashing (i.e., fingerprinting) technique works through an analogous Python server-side code. Note that this is very simplified for demonstrative purposes: google’s algorithm would be much more complex.
def hash_check(file, database): file_hash = hashlib.sha256(file).hexdigest() if file_hash in database: return "Illegal content detected. Upload failed." else: return "Upload successful."
What Happens When Illegal Content is Detected?
When automated systems or reports from users identify potential illegal content, this fact launches an investigation. If the content is found illegal, Google takes the following actions:
- Blocking: Stops the display of such content across all its services.
- Reporting: Sends these illegal URLs to the appropriate legal authorities for further action which may include criminal investigations.
- Delist: Ensures that these pages do not appear in Google Search results.
While I’ve provided an overview of Google’s measures to combat illegal content, it’s crucial to understand the challenges involved. Illegal content creators often use advanced techniques to bypass detection systems, while privacy concerns can constrain what Google can surveil. Hence, Google collaborates closely with law enforcement, NGOs, and other businesses to continually enhance their anti-illegal-content armor.
I trust this provides a clear portrayal of Google’s efforts in fighting against online illegal content, although it’s worth remembering that despite all measures, no system is foolproof. However, Google’s continued efforts towards improving safety measures maintains their commitment to providing a secure online environment.As a professional coder, I’m quite familiar with Google’s policies relating to the protection of intellectual property rights and how it tackles illegal content. When content breaches these rules, Google adopts several strategies, including search result filtering, disablement of accounts, and in some instances, content blocking.
Content Filtering
Google utilizes a system known as Content ID, implemented primarily on YouTube, which matches user-uploaded content against a database of files submitted by content owners. This algorithm automatically checks every upload, ensuring all content respects the copyrights of original creators.
Below is an interlude about using the YouTube Data API:
POST https://www.googleapis.com/youtube/v3/videos { "id": "Ks-_Mh1QhMc", "status": { "privacyStatus": "public", "embeddable": true, "license": "youtube" } }
This snippet uses the YouTube Data API to set the privacy status and license of a retrieve video.
Disablement of Accounts
If there is repeated infringement of intellectual property rights, Google has the authority to disable the offending account. This policy applies across all Google products.
Blocking Search Results
Google employs its search algorithms along with manual reviews to de-rank or remove web addresses (URLs) that are flagged for hosting infringing content. For example, upon receipt of valid DMCA notices, search results linking to alleged infringing sites will be removed from Google’s index.
Code-wise, let’s think about uploading a file using Google Drive API. Here’s a Python snippet:
from googleapiclient.http import MediaFileUpload from googleapiclient.discovery import build drive_service = build('drive', 'v3') file_metadata = { 'name': 'My Report', 'mimeType': 'text/plain' } media = MediaFileUpload('files/report.txt', mimetype='text/plain', resumable=True) created = drive_service.files().create(body=file_metadata, media_body=media, fields='id').execute() print('File ID: %s' % created.get('id'))
This code uploads a report.txt file to Google Drive. Remember, if this text violates copyright law, Google’s system can block the file completely.
In practice, Google doesn’t typically “block” content outright, but rather works to ensure that it does not get distributed or displayed via their services. Users or businesses who misuse Google’s platforms to disseminate unauthorized intellectual properties do so at the risk of having such content taken down and their access revoked.Google’s commitment to safe browsing entails a comprehensive system that focuses on the identification and blocking of illegal or prohibited content. These efforts are centered on providing users with a secure online environment, which makes it increasingly difficult for malicious actors to compromise user safety or violate any laws.
One of the ways Google accomplishes this feat is through their Safe Browsing technology. This checks websites against Google’s lists of unsafe web resources, which includes social engineering sites (like phishing and deceptive sites), unwelcome software, and other dangerous sites. Specifically, if a site hosts malware, unwanted software or engage in phishing, Google acts swiftly to block access to it.
Consider this piece of Python code, using Google Safe Browsing API:
# Import necessary modules import requests # Define the API key and the URL you want to check apiKey = "your-api-key" urlToCheck = "http://example-url-to-check/" # Check the given URL against the Google Safe Browsing database response = requests.get( 'https://safebrowsing.googleapis.com/v4/threatMatches:find?key=' + apiKey, json={ "client": { "clientId": "mycompany", "clientVersion": "1.5.2" }, "threatInfo": { "threatTypes": ["MALWARE", "SOCIAL_ENGINEERING"], "platformTypes": ["ANY_PLATFORM"], "threatEntryTypes": ["URL"], "threatEntries": [{"url": urlToCheck}] } } ) # Print the response (any matches will be in the `matches` field of the JSON response) print(response.json())
Further, Google has implemented machine learning algorithms to detect and block illegal content. These AI-driven models have been trained with vast amounts of data and are capable of identifying patterns associated with illegal content. Content that flags up these patterns is then reviewed by experienced teams at Google and subsequently blocked, reported, or removed if deemed as harmful.
Moreover, Google also expedites its safe browsing protocol by encouraging user reports concerning potential security threats. Users can report malicious sites via the Report a Malware Page platform, leading to swift action being taken for identified security risks.
Crucially, Google isn’t only committed to blocking such content but also to equip individuals with the necessary knowledge about staying safe online. The company provides useful information and guidelines including how to avoid scams, protect your data, identity, and more through a resource called Google Safety Center.
Thus, Google soldiers on with aggressive strides to evade illicit or damaging content from reaching users, leveraging technology, analytics, manual oversight, and public collaboration. Prohibited content remains an exigent issue; however, Google underlines effective countermeasures to ensure platform integrity and user safety. While imperfections might appear at times, continuous improvements in their algorithmic detection and response mechanisms underline a firm resolve towards a safe browsing experience.
Yes, Google does block illegal content and has mechanisms in place for censorship. This is not only a legal obligation, but part of Google’s ethical responsibilities as the world’s most-used search engine. The company has designed systems to regulate and filter out certain types of content, although this process is nuanced and complex.
Keywords In Detection:
The automated systems Google uses can detect certain types of illegal content, mostly through the use of specific flagged keywords or phrases that are often associated with such material. This means if a web page associated with unlawful activities includes any of these specific keywords, Google’s algorithm would likely flag it during a search query.
GoogleAlgorithm(){ // Snippet of mechanism Google use for keywords detection performSearch(query:String){ flaggedWords = ["illegal content example1", "illegal content example2"] for word in flaggedWords{ if query.contains(word){ return blockContent(); // Blocking mechanism } } return provideSearchResults(); } }
URL Filtering:
Google also censors certain URLs believed to be hosting illegal content from appearing in search results. Building a large database helps them exclude such links from queries. Websites blacklisted in this manner could be reevaluated and removed from the blacklist if they no longer contain prohibited content.
GoogleDatabase(){ // Hypothetical example of how Google might handle URL filtering validateURL(URL: String){ blacklistedSites = GoogleDB.loadBlacklistedSites() if blacklistedSites.contains(URL){ return blockURL(); // The act of blocking the site } return validateContentOfSite(); } }
It’s important to note that, due to the staggering amount of requests Google processes every second, automatic tools may sometimes yield false positives, which might also cause certain farm-free content to get censored unexpectedly. Therefore, Google works on constantly refining their detection and blocking algorithms.
Community Flagging:
In addition to the automated methods, a significant portion of flagged content comes from users leveraging community flagging tools provided by Google. These features allow anyone to report suspicious activities related to search results, emails (in Gmail), videos (on YouTube), etc.
YouTube’s user-based reporting system, for instance, allows users to flag videos they believe violate Community Guidelines. Content flagged by users is reviewed by human moderators, who then make the final decision whether to allow or block said content.
The Legal Aspect:
Google is obliged to censor illicit content within its search engine according to regional laws. For example, European Union privacy law now compels Google to censor some search results upon requests under the ‘Right to Be Forgotten’ regulations (
General Data Protection Regulation) . Authorities also send direct requests to Google asking to remove explicit materials, copyright infringements, among other illegal artifacts.
Thus, Google bears a tremendous responsibility to ensure their platform remains free from harmful and illicit content. While this task is challenging given the vast amount of information processed daily, the giant continues to leverage automation and machine learning alongside human moderation to meet these standards.
The Digital Millennium Copyright Act (DMCA) is a law introduced by the United States in 1998 with the intent to promote innovation and preserve intellectual property rights online. It provides a legal protection for owners of copyrighted content on the internet by way of DMCA takedowns.
A DMCA takedown is when a copyright owner requests an online service provider to remove their copyrighted material from a website that is using it without their permission. The Online Service Provider (OSP) must respond promptly to these requests, or risk being held liable for copyright infringement themselves.
Google, as OSP, adheres to the provisions of the DMCA. When Google receives DMCA complaints, it reviews and if validated, delists the infringing pages from its search results. This is not necessarily because the content is ‘illegal’, but because it infringes copyright, which is a civil matter rather than a criminal one.
However, Google does block certain types of content deemed illegal according to its own policies and local laws where it operates – such as child exploitation imagery, terrorist content, hate speech or gratuitous violence. But it is important to remember that this is separate from their role in handling DMCA takedown requests.
For example, to understand how Google responds to DMCA take downs:
// A complainant sends a DMCA notice to Google. Complainant sends DMCA Notice-> Google // Google assesses the notice. Google Assess validity of the Notice // If valid, Google removes the reported URLs from their search results. If Valid -> Remove URLs from Search Results
Separately,
// Google algorithm detects illegal content; disallowed by Google policy. Google detects illegal content // Google delists or blocks the content. Google Delistes/Blocks content
Understandably, Google’s method must strike a balance between upholding user’s right to freedom of expression, and protecting the rights of copyright holders. Hence, the implementation of DMCA in Google’s policy enables rightful owners to take action against unauthorized use of their copyrighted materials while maintaining a relatively free, open web.
Moreover, Google provides transparency through its Copyright Transparency Report , where they provide details about the volume and nature of copyright-related takedown notices received.
Table providing a visual difference:
DMCA Takedowns | Illegal Content Blocking | |
---|---|---|
Trigger | Received complaint from copyright owner | Detected by Google algorithm or flagged by users |
Violation type | Civil (Copyright Infringement) | Can be Criminal (depends on the content) |
Result | Delisting of specific infringing URLs | Blocking or delisting of offending page/site |