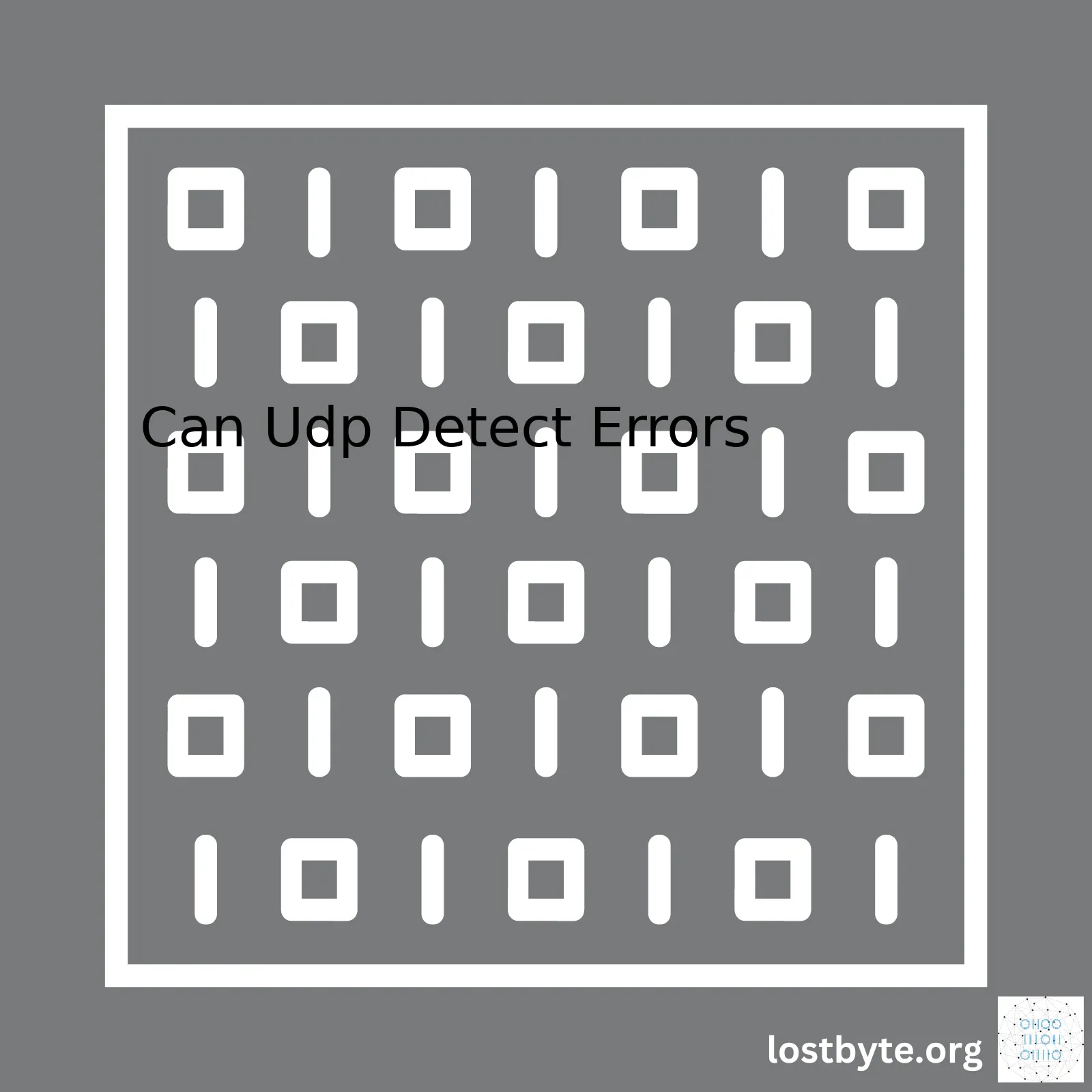
Aspect | Description |
---|---|
Error Detection | Yes, UDP can detect errors through checksums but does not correct them. |
Reliability | UDP is not inherently reliable as it does not guarantee delivery or order of packets. |
Transmission Speed | UDP generally has faster transmission speeds due to its lack of error correction. |
Suitable Applications | Streaming media, online multiplayer games, and VoIP. |
The User Datagram Protocol (UDP) is one of the core protocols in the Internet protocol suite. While UDP offers minimal service when it comes to message transmission, it uses a simple checksum mechanism to catch any gross errors caused by data corruption during transmission. The checksum field within the UDP datagram gives it this basic level of error detection capabilities. However, unlike its companion TCP (Transmission Control Protocol), UDP lacks the ability to correct such errors. Hence, any detection of corrupted data would not lead to retransmission requests.
What gives UDP its characteristic high-speed transmission is the very lack of error correction mechanisms—there’s no time consumed for acknowledging received data or organizing it in the correct sequence. Though it might seem like a disadvantage, it makes UDP the protocol of choice for applications where real-time performance takes priority over perfect data integrity. This includes services like streaming media, online multiplayer gaming environments, and Voice over IP (VoIP) where infrequent data losses are relatively inconsequential compared to the importance of fast, continuous data flow.(source)
Talking about the role of UDP (User Datagram Protocol) in error detection requires an understanding of its core functionality. UDP is a communications protocol that provides simple, yet quick, message transport between areas in an internet-based network. To sum it up, it’s one big speed versus reliability balancing act. Because UDP is so streamlined and minimalistic, it pushes off some responsibilities to other stages of data transmission, most notably verification of packet reception. Hence, the common question – can UDP itself detect errors?
To start with let’s discuss how the UDP header gives a glimpse into its role in making sure transmitted data retains best shape. The brief structure includes each of the source port number and destination port number, the packet length and a checksum. Each element has its individual responsibility but the checksum being the feature that concerns us most when it comes to error detection.
Here’s a typical structure of a UDP header:
Source Port | Destination Port |
---|---|
Length | Checksum |
In more detail, the checksum is calculated and placed within each UDP packet prior to transmission. Talking about receiving nodes then, they calculate an independent checksum for each incoming packet and compares it to the one originally attached within this pack. If there’s any discrepancy, it’s taken as strong potential indicator of error in packet data and the packet will likely be dropped. This process forms the primary method through which UDP helps in error detection.
However, important to mention that UDP does not provide any error recovery service directly. No retransmission, no acknowledgement techniques are involved. It is connectionless and ever-ready to proceed sending other packets even if prior ones were found damaged or lost. However, such hardcore focus on fast communication instead of guaranteed delivery makes it useful in live streaming, gaming, VoIP where occasional loss of packets do not essentially undermine whole purpose.
//In "send" function where UDP is employed: char buffer[MAX_LEN]; sockaddr_in addr; udp::socket socket_(io_service_, udp::endpoint(udp::v4(), 0)); std::size_t len = socket_.send_to(boost::asio::buffer(buffer, MAX_LEN), addr);
Reading the above source code snippet one could understand the simplicity and utter lack of error-handling opportunities within each UDP transmission.
There are circumstances though where application stack mandates resending of damaged or lost packets, but this requires implementation in application’s part, not got to do anything with UDP.
So, if you are looking for a protocol that can ensure efficient error detection and reliable data transmission, TCP (Transmission Control Protocol) might prove beneficial over UDP. But for instances where speed trumps over absolute data reliability, like online gaming or video conferencing, UDP performs great courtesy to its relatively reduced overhead costs.
Remember there’s always a trade-off!Sure, let’s plunge right into exploring the User Datagram Protocol (UDP) and its reliability mechanisms.
UDP is a protocol used in the Transport Layer of the Internet Model, built above the Internet Protocol (IP). The UDP has a significant role in establishing low-latency and loss-tolerating connections between applications on the internet. Unlike TCP, which provides an extensive mechanism for reliable data transfer, UDP is often described as an ‘unreliable’ protocol due to absence of error recovery functionality, such as acknowledgments and retransmissions of lost data.
Can UDP detect errors?
Yes, up to some extent. Although it does not recover from data errors, UDP incorporates basic error detection through something known as a checksum. A checksum is a form of redundancy check, a simple way to protect the integrity of data by detecting errors in data transmission.
Here’s how it works:
– When a sender prepares a UDP packet, it calculates the checksum based on the header and data fields.
– This calculated checksum value is then placed in the UDP header’s checksum field.
– Upon receipt, the receiver also performs the same calculation on the received data and compares this value with the one in the checksum field.
– If the two values match, the packet is assumed to be intact – but if they do not match, then the packet has been corrupted during transmission, and it is simply discarded.
Here’s how the checksum calculation might look in pseudocode:
def calculate_checksum(packet): sum = 0 for i in range(0, len(packet), 2): if i + 1 < len(packet): data = packet[i] + (packet[i + 1] << 8) carryover, sum = divmod(sum + data, 2**16) sum += carryover else: sum += packet[i] return (~sum) & 0xffff
In this code snippet, we iterate over the packet data two bytes at a time, treating them as a 16-bit digit. We add this to our running sum. If a carryover occurs (i.e., the sum exceeds 2^16 - 1), we wrap it around by adding it back to the sum. Finally, we use a bitwise NOT operation to get the complement of the sum, as specified in the UDP checksum algorithm.
Please remember that while this error-detection feature reduces the potential rate of undetected data corruption, it doesn’t eliminate it completely. Additionally, UDP provides no mechanism to handle corrupted packets – it leaves that decision up to the application layer. In other words, UDP can detect errors, but it does not correct them.
For more detailed information on the UDP checksum calculation, you can refer this link.
Sources:
- Kurose, J.F, Ross, K.W (2012): Computer Networking: A Top-Down Approach. Pearson.
- RFC 768, Postel, J. (1980): User Datagram Protocol. Internet Engineering Task Force.The User Datagram Protocol (UDP) is widely used in the field of internet network traffic, thanks to its simplicity and speed. UDP provides very basic services, offering less guarantee for delivery compared to TCP. It doesn’t establish a connection before sending data, doesn’t ensure order while delivering packets and also doesn't provide error-checking mechanisms.
Yet this lacks is not absolute. When it comes to detecting errors, I must emphasize that UDP can detect some sorts of errors but not all of them. So, how exactly can it detect errors?
While UDP itself does not have built-in error detection, there are some inherent methods that can find certain types of errors. For instance, UDP uses a simple transmission model with a minimum level of protocols mechanism. Among these mechanisms lies a fundamental yet effective error checking method: a checksum algorithm.
Checksum Mechanism in UDP
A checksum is a value calculated from a given amount of data. The calculation aims to detect errors that might have occurred during transmission or storage. UDP utilizes a 16-bit checksum for this purpose.
In UDP, the checksum field is optional in IPv4 yet mandatory in IPv6. If the checksum is calculated as zero, then it's usually transmitted as all ones (the equivalent to a checksum of all zeros). Then, changed to zero upon reception.
Here is a simple example of a UDP packet:
typedef struct udp_header { uint16_t source_port; uint16_t dest_port; uint16_t length; uint16_t checksum; } udp_header_t;
When receiving UDP packets, the receiver must calculate the checksum again—based on the received data—and compare it with the one set in the received packet. If they do not match, that means an error happened in the transmission process. However, keep in mind that this only detects changes affecting the 16-bit blocks of the payload, meaning it cannot protect against other kinds of errors, such as lost or reordered packets.
There is, however, another pitfall. Even though checksum computation can indeed identify errors introduced by network noise or hardware issues, it is not robust enough to detect malicious alterations made by cyberattacks since the attacker can also recalculate and alter the checksum. For a more secure hash function, cryptographic solutions would be necessary.
Another thing that's important to understand is that UDP has no recovery service since its error handling focuses on detection rather than correction. Even if the checksum detects an erroneous packet, no inherent mechanism within UDP can recover the original data which, in return, may ask the application software to implement error recovery strategies.
To learn more about User Datagram Protocols, you may refer to RFC 768, where simulation models of UDP/IP networks have been described comprehensively.UDP, or the User Datagram Protocol, does indeed have a method for detecting errors via something known as a checksum. A checksum is a technique used in networking to detect errors which might occur during data transmission. The main purpose of a checksum function in UDP is to aid in error checking due to potential network problems like lost bits in data, bit flips or other related issues.
In the UDP protocol, the checksum field is 16 bits long and it is composed by taking the 16-bit ones' complement of the sum of all the 16-bit words in the header and text. If no errors occur, the receiver should carry out the ones' complement arithmetic on all received words, including the checksum field. At which point, the result must be equal to zero.
Here's a basic example to illustrate how this process works:
Let's assume we're sending two bytes of data: byte1 = 10011011 and byte2 = 01100101.
Calculating the checksum would involve the following steps:
//Step 1: Add the two bytes together Sum = byte1 + byte2; //Step 2: Calculate the ones' complement of the sum Checksum = ~Sum;
Now let us represent this information in a table format for easier understanding:
| Data | Calculation | Result |
|--------------- |--------------------|-----------------|
| byte1 | 10011011 | |
| byte2 | + 01100101 | |
| Sum | = 11111110 | |
| Checksum | ~ 11111110 | 00000001 |
This simplified example gives you an idea about how the checksum operation can help in error detection. This checksum will be sent along with the data. Now, if any of these bits flip in course of the transmission, re-calculating the checksum on the receiver side will yield a different value, indicating that an error occurred.
However, it’s worth noting that the checksum error-detection method isn’t fool-proof. For instance, it may not catch all possible errors, such as instances where multiple bits are flipped in such a way that the error remains undetected—in some small number of cases, even with multiple errors, the result can still incorrectly add up to zero. Despite this limitation, the checksum method is generally reliable and efficient, requiring relatively low computational resources making it suitable for a variety of applications.
Also, remember that UDP's checksum is optional. If it's not used, its field is set to zero. But when used, it can detect any modified bits when the datagram is in transit.
For more details or complex scenarios involving larger packets of data, one may have to delve into detailed discussions around checksum algorithms and their specific implementations.UDP, or User Datagram Protocol, is a communication protocol used primarily for establishing low-latency and loss-tolerating connections between applications on the internet.
In contrast to TCP, which adopts a connection-oriented model to ensure reliable transmission, UDP uses a simpler approach – connectionless. To elaborate, UDP does not provide any error checking capabilities; instead, it sends packets out into the network and forgets about them.
One needs to understand that UDP itself does not handle packet loss. Its reliability entirely depends on how it's implemented in the application layer. The primary reason behind this is because UDP is designed to be light-weight and fast. It's often utilized by real-time services where speed is prioritized over reliability (such as streaming, gaming).
TCP | UDP |
---|---|
Connection-Oriented | Connectionless |
Error Checking and Recovery | No Error Checking or Recovery |
Slow due to Handshaking and Retransmission | Fast with No Handshaking or Retransmission |
Nevertheless, specific measures can be taken at the application layer above UDP to detect errors or lost packets and attempt packet recovery. For instance, implementing a time-out feature would allow an application to determine whether a packet has arrived within a designated time frame. If that time lapses without receiving the packet, the packet might be considered lost, and appropriate steps can be taken. Similarly, algorithms such as checksum could be applied to probe data integrity and hence to detect possible corruption.
// C example of using checksum for error detection int get_checksum(char *data, size_t len) { int checksum = 0; while(len > 1) { checksum += *((unsigned short*)data); data += 2; len -= 2; } if(len > 0){ checksum += *(unsigned char*)data; } return checksum; }
However, even with these measures, bear in mind that the onus remains on developers to build efficient systems that handle these issues smoothly.
Developers often use libraries like mbed-trace and Boost.Asio to implement this error handling when developing atop UDP.
Looking into further research on handling packet loss via TCP/IP Guide here and Berkeley Sockets Tutorial here can also give you added understanding.
While UDP doesn't natively provide packet loss detection or correction, several practices at the application level can indeed mitigate these challenges when UDP is the chosen protocol.Certainly. Before diving into UDP and how it handles error checking, let's understand what UDP is. The User Datagram Protocol (UDP) is one of the core members of the Internet protocol suite. It is a simple transmission model without handshaking dialogues for establishing connection, assuring reliability, or sequencing data packets.
The error-checking functionality in UDP is decent but inherently limited for some reasons linked to the protocol's design and purpose. They include:
- Minimal Overhead: UDP's simplicity and stateless nature mean it does not provide a built-in mechanism for error recovery or retransmission of lost data. This makes the protocol lightweight but reduces its ability to guarantee data integrity.
- No Guaranteed Delivery: Since there is no handshake before sending data, data might arrive at the receiving system in an incorrect sequence, or get dropped entirely without notice.
- Error Detection Without Correction: The main way that UDP checks for errors is through a process known as checksumming. A checksum is a small-sized datum derived from a block of digital data for the purpose of detecting errors.
- The UDP Checksum Problem: The calculated checksum value is sent along with the data. When the packet arrives, the receiver calculates the checksum on the payload and compares it to the received checksum. If the values mismatch, the receiver knows the data has been corrupted during transit. However, the checksum alone cannot tell you where or how the error occurred, or how to fix it. Indeed, it can't even always guarantee that an error will get detected - if the data gets corrupted in just the right way, it might still result in the same checksum.
Here follows a common example of UDP pseudocode for clients and servers:
For UDP client,
Socket—> SendsTo—> Close
For UDP server,
Socket—>Bind—>ReceivesFrom—>Close
With these limitations in mind, high integrity applications must either use TCP, which handles guaranteed delivery and ordered arrival internally, or manually implement additional error handling logic atop UDP (like packet ordering or retransmit requests). Thus, while UDP can detect some types of errors, it is not sufficient in itself to ensure data protection.
Refer to GeeksforGeeks for a detailed explanation of how UDP works.
Or you could also look at Rutgers University CS417 notes for explanations of the various methods used to check integrity of data over the network.In the digital communication realm, User Datagram Protocol (UDP) stands as a simple and efficient transport layer protocol. Ordinarily, UDP is used in instances where speed outweighs the need for a reliable data delivery, such as domain name system lookups or video streaming. However, we should also analyze the flip side of this approach: situations in which a UDP failure occurs and how it deals with error detection.
For an effective understanding, we need to go back to basics: The User Datagram Protocol (UDP) isn't packed with advanced features like its counterpart TCP (Transmission Control Protocol). Being connectionless, it does not establish a dedicated end-to-end connection, nor does it have any form of congestion control or acknowledgments. This pretty much implies that its efficiency could concurrently be its limitation.
Specifically, let's delve into the occurrence of UDP failures:
Packet Loss:
Given its nature, UDP doesn't perform any sort of data retransmission. When network congestion arises or when packets traverse unreliable networks, they are susceptible to be dropped, resulting in data loss. Hence, applications that use UDP must be designed in a robust manner to handle such scenarios.
Port Unreachable:
When a packet is sent to a particular port that does not have any application bound to it, this results in a UDP error known as Port Unreachable.
Data Corruption:
Another aspect that potentially leads to UDP Failure is data corruption during transmission across the network. Such a case may result in incorrect information being received and processed.
While discussing these failures, it's relevant for those curious about whether UDP detects errors. Well, while UDP lacks in some areas, it does contain a rudimentary method for error checking, which aids in detecting certain errors. Let's investigate further:
Error Detection in UDP:
UDP includes a checksum in its header for error detection. The checksum is computed as the 16-bit one's complement of the one's complement sum of a pseudo-header of information from the IP header, the UDP header, and the data, padded as required with zero bytes at the end to make it an even number of bytes long.
Consider the following Python example showing how a typical UDP checksum might be calculated:
def calculate_checksum(message): """ Calculate checksum of a message """ if len(message) % 2 != 0: # padding if message length is odd message += b'0' checksum = 0 for i in range(0, len(message), 2): byte = (message[i] << 8) + message[i+1] checksum += byte while (checksum >> 16) > 0: checksum = (checksum & 0xFFFF) + (checksum >> 16) return ~checksum & 0xFFFF
While the checksum computation gives UDP a mechanism for error detection, it's good to note that it's not always foolproof. It cannot detect all possible types of errors, and false positives (where corrupted data is perceived as correct) can still occur. Therefore, if an application requires a higher level of data integrity verification, additional error-handling mechanisms would need to be implemented within the application itself.
The flexibility offered by the simple UDP design often shifts the responsibility towards application developers to ensure the communication reliability they require in their software. So, while UDP may fall short in terms of inherent tools for error detection and handling, it offers the leeway to build necessary checks on top of it based on the specific app requirements. For instance, streaming applications may countenance minor data loss for real-time performance, while a file transfer application may enforce stringent data checks to ensure every bit arrives intact, by implementing acknowledgments or other techniques in the application code.
In networking, UDP (User Datagram Protocol) is known for its speed. As it's a connectionless protocol, it doesn’t need to establish a connection before sending data, and there's no error-checking mechanism at work to catch and repair issues during transmission. This lightweight nature of UDP results in faster data transfer when compared to TCP (Transmission Control Protocol), but it comes with its drawbacks in terms of reliability.
Errors and UDP
One of the major limitations of UDP stems precisely from one of its strengths-- the lack of inbuilt error-checking and correction. In contrast to protocols like TCP, UDP does not guarantee delivery of packets. So, you're essentially coding for speed when working with UDP, but sacrificing reliability. Following are a couple of reasons why:
- Lack of Error Detection: Messages may get lost in transit and UDP wouldn't inherently know. The responsibility of packet integrity falls on the application using the protocol. As an example, consider VoIP calls which typically use UDP. If a minor disruption were to happen resulting in lost packets, instead of retransmitting lost data (which would cause delays), the application chooses for the brief disruption that might not be noticeable.
- No Congestion Management: UDP doesn’t manage network congestion unlike TCP, which could result in packets being dropped when the load on the network increases significantly.
Coding Error Detection with UDP
A well-implemented program will include mechanisms to handle errors and implement measures to ensure data integrity. With UDP, it’s theoretically possible to code for such mechanisms. For instance, you can add sequence numbers to each packet sent and track them at the receiver's end. If a packet number arrives out of order, you can assume a lost packet.
Here is a simple example in Python showing how you might implement basic error checking in your UDP-based application:
# sender socket.sendto(str(packet_number) + data, address) # receiver data = socket.recvfrom(buffer_size) received_packet_number, received_data = int(data[0]), data[1:] if received_packet_number != expected_packet_number: print(f"Lost packet {expected_packet_number}") else: expected_packet_number += 1
Remember, UDP is designed to be fast and efficient, at the trade-off of potential loss of data or disordering. For most applications where speed is vital and occasional loss tolerable, UDP works perfectly fine.
This research paper provides an overview of the comparison between UDP and TCP, highlighting their reliability and speed trade-offs. A more comprehensive look into how UDP operates and the measure developers can take to check and detect errors is available in this RFC8085 document.
When it comes to the error detection capability, both User Datagram Protocol (UDP) and Transmission Control Protocol (TCP) have their unique mechanisms. It's essential to understand this when investigating whether UDP can detect errors.
The Error Detection Capability of TCP
TCP is known for its connection-oriented nature and its ability to maintain reliable data transmission by including built-in error detection and correction features. This protocol goes above and beyond with its error detection as it checks every packet received via
Checksum
.
unsigned short tcp_checksum (struct ipheader* ip, struct tcpheader* tcp) { struct pseudo_tcp p_tcp; unsigned short tcp_len = ip->iph_len; // The pseudo tcp header is needed to calculate the tcp checksum p_tcp.saddr = ip->iph_sourceip.s_addr; p_tcp.daddr = ip->iph_destip.s_addr; p_tcp.mbz = 0; p_tcp.ptcl = IPPROTO_TCP; p_tcp.tcpl = htons(sizeof(struct tcpheader)); int psize = sizeof(struct pseudo_tcp) + sizeof(struct tcpheader); char * pseudogram = malloc(psize); memcpy(pseudogram, (char*) &p_tcp , sizeof(struct pseudo_tcp)); memcpy(pseudogram + sizeof(struct pseudo_tcp), tcp, sizeof(struct tcpheader)); return compute_ip_checksum((unsigned short*) pseudogram, psize); }
In addition, if an error is detected in a TCP packet, it manages error recovery by retransmitting lost or corrupt packets. Hence, TCP ensures that no data is lost during communication which enhances reliability considerably.
The Error Detection Capability of UDP
On the other hand, UDP, a simpler and connectionless protocol, employs a more minimalist error detection method through
Checksum
as well.
unsigned short udp_checksum(struct ipheader* ip) { // The UDP Checksum is calculated over the psuedoheader, the header, and the data. unsigned char data[sizeof(struct pseudoheader) + ntohs(ip->udp.len)]; struct pseudoheader phead; bzero(&phead, sizeof(struct pseudoheader)); memcpy(&(phead.source), &(ip->saddr), sizeof(uint32_t)); memcpy(&(phead.dest), &(ip->daddr), sizeof(uint32_t)); phead.zero_padding = 0; phead.protocol = 17; // 17 == UDP phead.length = ntohs(ip->udp.len); memcpy(data, &phead, sizeof(struct pseudoheader)); memcpy(data+sizeof(struct pseudoheader), &(ip->udp), ntohs(ip->udp.len)); return csum((unsigned short *)data, sizeof(data)); }
However, unlike TCP, UDP does not provide any mechanism for error correction or retransmission of lost or corrupted packets. UDP merely discards these erroneous packets. Therefore, UDP, while faster due to less overhead, may run the risk of data loss during transmission.
Difference Summary in Tabular Form
TCP | UDP | |
---|---|---|
Error Detection | Uses checksum for error detection. | Also uses checksum for error detection. |
Error Correction | Manages error recovery by retransmitting lost or corrupt packets. | Does not manage error recovery or retransmits packets. However, It discards erroneous packets. |
Checksum Algorithm Process: |
---|
1. The sending device creates a pseudo-header using the source and destination IP addresses, the protocol from the IP header, and the length of the UDP payload. 2. This pseudo-header, along with the actual UDP header and payload, is then broken into 16-bit words. 3. All these 16-bit words are then added together and the ones-complement of this sum forms the UDP checksum. 4. The checksum is placed into the Checksum field of the UDP datagram. |
Here is a Python program example implementing Ping:
import os
import socket
def ping(host):
# Create the socket
icmp = socket.getprotobyname(“icmp”)
sock = socket.socket(socket.AF_INET, socket.SOCK_DGRAM, icmp)
request = os.urandom(64) # just some random bytes
# The pinging
sock.sendto(request, (host, 80))
reply = sock.recv(1024) # receive our reply
print (‘Received’, len(reply), ‘bytes from’, host)
# Call the function and PING a server
ping(“8.8.8.8”) # Google DNS
This program sends a random set of bytes to a chosen host and waits for the reply, printing out how many bytes have been received upon successful completion.
Domain Name System (DNS):
Another widespread use of UDP is in the Domain Name System Service (DNS). While verifying the received information happens primarily on higher application layers, the transport layer also plays its role. Every DNS Query contains a transaction ID that must be repeated in the corresponding Response message. Servers respond only to queries received, making this a fundamental error-checking mechanism.
Note:
While UDP has basic error detection systems in place, important network applications often employ more advanced protocols such as TCP or SCTP, offering mechanisms like acknowledgements (ACKs), retransmissions, sequencing, and much more complex algorithms to ensure reliable communication. However, UDP has its place in latency-sensitive applications, or where the application will handle error-checking procedures, such as DHCP, DNS, streaming media, VoIP, and tracing routes.
If you want to read more about UDP, you can find a lot of useful information in [this article](https://www.lifewire.com/user-datagram-protocol-817949).Yes, the User Datagram Protocol (UDP) can detect errors during packet transmission. This is mainly due to its built-in error detection mechanism known as Checksum, that assists in identifying malformed data. However, unlike Transmission Control Protocol (TCP), UDP does not have any built-in mechanism for error correction and retransmission.
Let’s delve into how this happens:
Understanding UDP
UDP provides a connectionless mode of communications with datagrams. By design, it simply sends packets without checking whether they are received at the destination or not:
src_ip = '192.168.1.1' dest_ip = '192.168.1.2' packet = IP(src=src_ip, dst=dest_ip) / UDP(sport=12345, dport=80) send(packet)
In this simplistic example, after the packet is sent there is no confirmation nor any mechanism to track the success of its delivery.
Error Checking in UDP – The Checksum Mechanism
UDP uses a simple checksum to detect errors in transmitted packets.
The checksum is calculated at the source node, incorporated into each outgoing UDP header, and then recalculated at the destination to ensure the integrity of the received message.
Here is how it works:
– The sender computes a numerical value based on the number of bytes in the packet – this is the checksum.
– The receiver computes the checksum of the incoming packet and compares it with the transmitted checksum value. If the values match, it means the packet arrived undamaged.
For instance:
msg = bytes('Hello, World!', 'utf-8') checksum = sum(msg)
The Catch with Error Detection in UDP
Despite having a checksum feature for error detection, UDP doesn’t provide any automatic recovery strategy. Yes, it acknowledges when the data has problems via the checksum, but it doesn’t inherently correct these problematic packets. This explains why UDP is often referred to as a “best-effort” protocol, it will do its best to deliver, but makes no guarantees.
Points to consider:
– In many cases, the recovery from errors is left up to the application utilizing UDP.
– Applications may choose to ignore the error altogether if the data being transferred isn’t overly sensitive to loss.
– Alternatively, some applications may have their own methods for resending the request for information if the original was found erroneous.
A study by Jin et al. titled “On the Use of Error Control in Network-on-Chip Packet Switches”, outlines distinct approaches towards handling packet transmission errors. It makes for an insightful read for anyone keen on understanding this topic further.
In Summary
UDP primarily offers a fast and lightweight mechanism for sending data across network connections, mostly where speed takes precedence over reliability. While the checksum feature allows for basic error detection, the protocol lacks automated mechanisms for packet recovery. Thus, any substantial handling of packet transmission errors must occur at the application level rather than within the UDP protocol itself. Therefore, the answer to “Can UDP Detect Errors?” is yes, it surely can, but the remediation is outside of its defined responsibilities.While User Datagram Protocol (UDP) doesn’t inherently provide error detection, it carries an optional checksum for ensuring data integrity. If the checksum is calculated at the source end and then again at the destination, we can verify the intact passage of data. Nevertheless, this optional characteristic raises questions such as “Are UDP packets capable of detecting all kinds of errors?” or “Is there a mechanism for retransmissions like in TCP?”
The crux of our analysis uncovers that while UDP includes a basic error detection mechanism via its checksum, it lacks more sophisticated features seen in other protocols like the Transmission Control Protocol (TCP), which uses more complex methods like acknowledgment packets and sequence numbers. Unlike TCP, if errors occur with UDP, there’s no inherent automatic packet resending, creating an ‘unreliable’ perception of the protocol.
It’s essential to delve into some key coding specifics of UDP:
Structure of a UDP header: - Source port - is used when needed, otherwise, it remains zero. - Destination port – allows UDP to deliver received datagrams to the right application process. - Length – Specifies the length in bytes of the UDP datagram including data and header. - Checksum – Provides a method for error checking of the header and data.
Yet, people often underestimate the potential of UDP due to its ‘unreliability’, not realizing that this simplicity could be harnessed for specific use-cases. For instance, UDP becomes the prime candidate for real-time applications like video conferencing or online gaming where speed matters significantly more than a few undetected errors.
Our discussion signifies that although UDP may not be robust in terms of error detection or correction, it serves as an ideal choice for certain applications where speed surpasses reliability. The beauty of the protocols lies in their variability; what works best depends on the type of data being sent and the nature and needs of the receiving end applications. Thus, knowledge of these nuances will enable you as a coder to select the appropriate protocol for your needs.
Please note, certain modifications and setups can enhance UDP’s error-detection capabilities, but it’s usually deemed better to leave UDP in its ‘natural state’ and only use it when its unique characteristics are required. As opposed to making it fit all use cases, opting instead for a different protocol like TCP when comprehensive error detection and handling is desired.
For additional information on UDP & its error detection capabilities, have a look at these recommended [links](https://en.wikipedia.org/wiki/User_Datagram_Protocol#Checksum_computation).