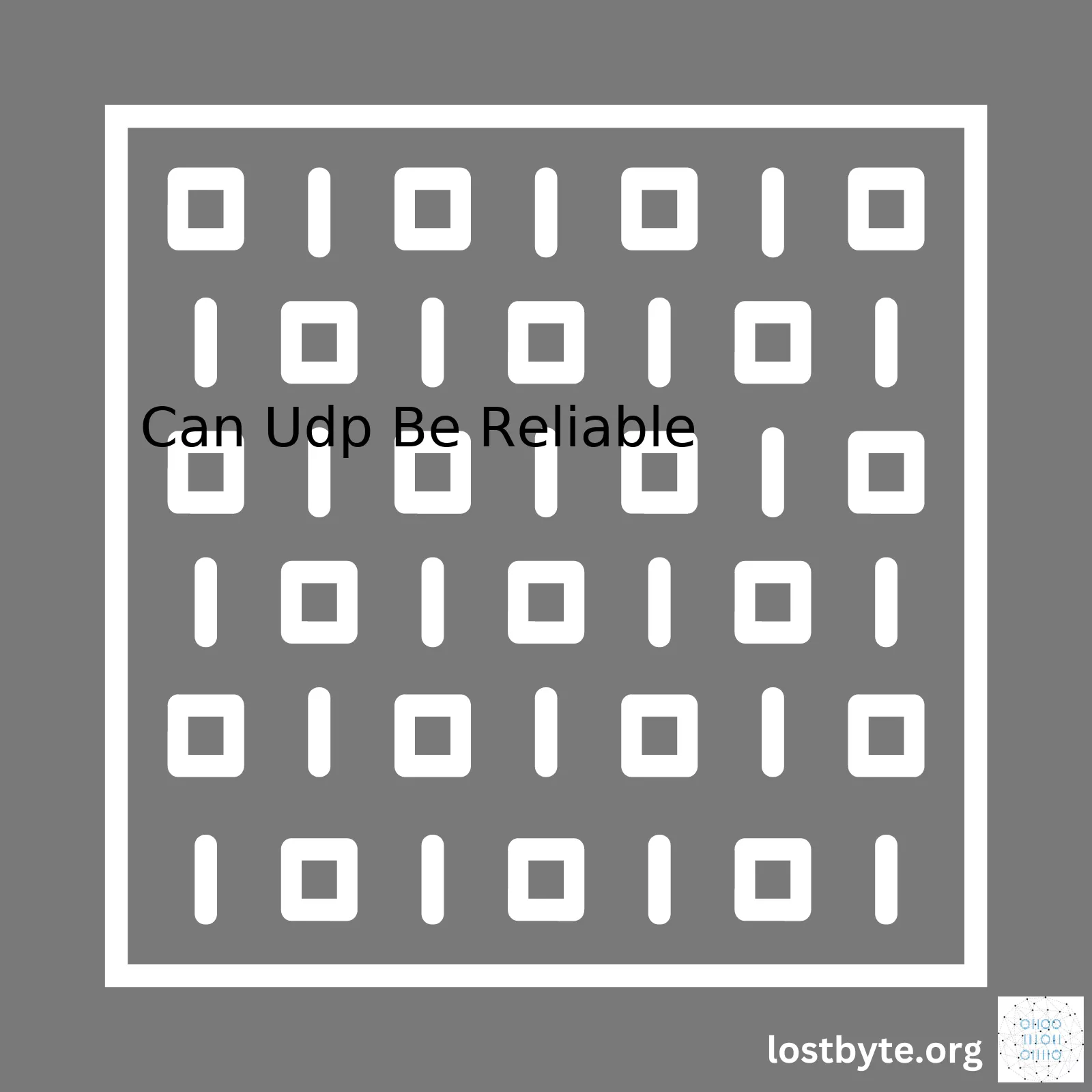
Let’s go ahead and generate a table summarizing the key points around the reliability of User Datagram Protocol (UDP).
Feature | UDP |
---|---|
Data Transfer Method | Connectionless |
Error Checking | Minimal, only checks data integrity |
Acknowledgement | No explicit acknowledgement of data packets received |
Reliability | Low |
Fast Transmission | Yes |
Packet Ordering | No guarantee on ordering |
The UDP is often perceived as unreliable because it uses a connectionless method for transferring data. This means information is sent without establishing a connection, making it susceptible to being lost or arriving in different order at the receiving end. Unlike TCP/IP, UDP doesn’t utilize error checking or packet sequence tracking, which results in a low reliability quotient.
On the flip side, lack of explicit acknowledgements frees up bandwidth, allowing faster transmission speeds. While it checks data integrity – if there’s any corruption in the message string, it’s going to drop the package. However, application-level protocols on top of UDP, like RTP, can reinstate reliability by handling package loss, delivery acknowledgements, and sequence issues.
So, limiting the discussion to “Can UDP be reliable?” – Yes, but not inherently. The reliability has to be built into the protocol running on top of UDP or handled at the application level.
Understanding the nature of UDP (User Datagram Protocol), we generally perceive it as an unreliable form of data transmission over a network. However, instead of seeing this “unreliability” as a drawback, we should view it as a unique characteristic that can be harnessed for specific applications where reliability is not a priority. Yet, you may ask if UDP can be reliable? Certainly, despite its intrinsic nature of unreliability, with certain enhancements and clever techniques, UDP can indeed become reliable to quite an extent.
To unravel this, let’s start by providing some background on UDP. It is a connectionless protocol that manages data communication in a ‘fire-and-forget’ fashion without establishing a relationship between the sender and receiver beforehand. This feature makes UDP faster and more efficient for lightweight or time-sensitive applications such as streaming video or gaming. The caveat, however, is that the protocol does not guarantee delivery of data packets nor do they arrive in the ordered sequence due to network fluctuations.
Pros of UDP | Cons of UDP |
Faster data transmission | No guaranteed delivery |
More efficient for lightweight apps | Packets may not arrive in original sequence |
Doesn’t require connection establishment | Not ideal for data-sensitive operations |
Now, navigating back to our main discussion – making UDP reliable; it requires certain modifications and implementation of additional functionalities to achieve reliability. For instance:
– Packet acknowledgment: The receiving end sends an acknowledgement receipt upon receiving a packet. If the sender does not receive this within a certain timeframe, the packet is resent.
– Error checking: Implement checksum methodologies to verify if the received data has been tampered with during transmission.
– Sequencing: Numbering each packet so that the receiver can reorder them upon arrival, thus tackling the issue of sequence disarray.
//Sample Code showing sequencing char * prepareDatagram (char * message, int seq) { char * datagram; asprintf(&datagram, "%d %s", seq, message); return datagram; } /* seq is the order of the packet, message is the actual content to be sent. For example, if seq = 2 and message = "Hello World!", then "2 Hello World!" would be the prepared datagram*/
Companies like Cloudflare have already begun adopting such practices to exploit UDP’s speed while ensuring increased data reliability. They’ve developed QUIC (Quick UDP Internet Connections) which supplies necessary congestion control and loss recovery elements while enabling secure, low-latency connections.
Hence, while UDP itself isn’t naturally reliable, developers can engineer its environment to promote reliability depending on their application’s requirements. This way, you can balance between the pros of both TCP – the gold standard of reliable data transmission – and UDP to serve your specific needs better.
To recapitulate, can UDP be made reliable? Yes, even though UDP is inherently an unstable protocol, careful measures help make it reliable.Absolutely! So, you’re interested in the paradoxical relationship between TCP (Transmission Control Protocol) and UDP (User Datagram Protocol), especially with a particular focus on the potential reliability of UDP. I must begin by stating that while TCP is often touted for its inherent reliability features, UDP, in spite of being called an ‘unreliable’ protocol, can indeed be made reliable.
To understand this better, we first need to take a closer look at both protocols:
TCP
TCP, also known as Transmission Control Protocol, executes data transfers across networks in a methodical, ordered, and error-checked manner. Some of its key features include:
- Orderly packet delivery: Packets are sent one after another with sequencing information, ensuring they arrive in the correct order.
- Error detection and correction: A built-in check verifies if packets arrive safely, re-sending them if needed.
- Congestion control: It adjusts the rate of data transfer according to network traffic, preventing overloading.
UDP
On the flip side, User Datagram Protocol or UDP carries out its data transfers in a simpler, quicker, but less structured process. The hallmarks of UDP encompass:
- Simplicity: It sends packets without establishing connections, avoiding overheads.
- Speed: Due to fewer checks and formalities, UDP transmissions can be faster.
- No inherent reliability: Since it doesn’t verify delivery or order, UDP may miss out on undelivered or mis-sequenced packets.
Now, traditionally, this lack of reliability might make UDP seem like the lesser choice, but here comes the paradox: UDP can be tweaked to actually attain similar reliability levels as TCP. How, you may ask?
Firstly, application-layer protocols such as RUDP (Reliable User Datagram Protocol) and QUIC offer opportunities to inject reliability into UDP. RUDP adds elements of error correction, sequence assurance and handshake-based connections, making UDP more TCP-like in its operation.
Secondly, developers can custom-write their software to incorporate reliability checks within the system architecture itself. For instance, a game might be coded to resend important data if the previous transmission wasn’t acknowledged.
To really visualize the respective packet structures of TCP and UDP, let’s consider this comparison table:
TCP | UDP | |
---|---|---|
Packet Structure |
source port | destination port | sequence number | acknowledgement number | data offset | reserved | flags | window | checksum | urgent pointer | options | padding | data |
source port | destination port | length | checksum | data |
Even though UDP’s structure appears leaner, appending some reliability-enhancing layers onto it can indeed make UDP just as reliable (source).
In the end, whether you leverage TCP’s intrinsic reliability or engineer reliability into UDP, your choice should align with the specific requirements of your networked application. Speed and efficiency might lead you towards UDP, while guaranteed delivery might steer you towards TCP. Or, with the right adjustments, you might find a reliable middle ground in UDP itself.Indeed, the User Datagram Protocol (UDP) is not inherently reliable as it doesn’t ensure message delivery or avoid duplicate delivery. However, there are ways to tweak UDP and enhance its reliability through various techniques. To accomplish this, you’ll need to implement higher-level strategies, such as acknowledgment mechanisms, retransmission of lost packets, and establishing an ordered transmission of packets.
Adding Acknowledgment Mechanisms
The mechanism involves the receiver sending an acknowledgment packet back to the sender when it receives a packet. If the sender does not receive the acknowledgment within a certain timeframe, it considers the packet lost and retransmits it.
In the client code where we send the data, we would need to add an expectation of receiving an acknowledgement. The server code should be modified to send this acknowledgement upon successfully receiving a message.
Client side code:
// Sending Data sendto(s, buffer, buflen, 0, (struct sockaddr*) &sin, sinlen); // Waiting for Acknowledgment recvfrom(s, ack, acklen, 0, (struct sockaddr*) &sin, &sinlen);
Server side code:
// Receiving Data recvfrom(s, buffer, buflen, 0, (struct sockaddr*)&sin, &sinlen); // Sending Acknowledgment sendto(s, ack, acklen, 0, (struct sockaddr*) &sin, sinlen);
Retransmission of Lost Packets
This strategy involves resending packets that presume to be lost. The sender could implement a timeout mechanism, and if it doesn’t receive acknowledgment from the receiver within this period, it will retransmit the packet.
We can set timeouts using the
setsockopt()
function [See Manual Page](https://man7.org/linux/man-pages/man2/setsockopt.2.html)
struct timeval tv; tv.tv_sec = TIMEOUT_DELAY; // Set the second value for Timeout tv.tv_usec = 0; // Set the microsecond value for Timeout // Implementing the Timeout setting on socket "s" if (setsockopt(s, SOL_SOCKET, SO_RCVTIMEO,&tv,sizeof(tv)) < 0) { perror("Error"); }
If no acknowledgement is received within the time defined in `TIMEOUT_DELAY`, the data packet is resent.
Establishing an Ordered Transmission of Packets
Although UDP doesn't guarantee the order of deliveries, you can enforce this by adding sequence numbers to your packets. If packets arrive out of order, they can be rearranged based on these sequence numbers.
Suppose we choose to include the sequence number at the start of the data packet, which we can refer to henceforward.
uint16_t seq_no = htons(sequenceNumber); // Sequence number to network byte order memcpy(buffer, &seq_no, sizeof(seq_no)); // Adding sequence number to buffer // Add actual data after sequence number in the buffer memcpy(buffer+sizeof(seq_no), actualData, actualDataLength);
On the receiver end, extract the sequence number, convert it back to host order, and you can sort or reorder the packets accordingly.
Though these methods equipped UDP with some degree of reliability, they will still leave a considerable gap. A complete reliable transport protocol like TCP might be more advantageous if outright reliability is critical. However, knowing how to manage UDP's quirks allows us to leverage its low-overhead, flexible nature where apt.
It needs to be appreciated however that this approach pushes us towards building a protocol similar to TCP over UDP, which might seem mentally counterintuitive. Such complexity exists in TCP to provide a robust reliability mechanism natively, thereby insisting on understanding the pros and cons of UDP before committing to 'making UDP reliable' Read More.While User Datagram Protocol (UDP) is known for its speed, it's also notorious for lacking in reliability. Its design naturally comes with a series of trade-offs - rapid delivery at the cost of potential errors. Nonetheless, we can implement strategies to check for errors and enhance the reliability of UDP to some extent.
Checksums: An Essential Mechanism
Every UDP packet includes a checksum field, which helps detect if any modification or corruption occurred during transmission. Here's how this process works:
- When sending data, UDP generates a unique number based on the packet data: the checksum.
- The sender includes the checksum within the UDP packet.
- After receiving the packet, the recipient performs the identical checksum calculation.
- If these checksum numbers match, it shows that the packet has been delivered without an error.
We can use the advantage of this checksum mechanism in C programming to provide better reliability:
struct udphdr { u_short sport; // Source port u_short dport; // Destination port u_short len; // Datagram length u_short check; // Checksum };
By adding a condition to validate the checksum, we can ensure the received packet is safe to process.
Acknowledgement System: Back-up Plan for Error Detection
Another strategy to improve UDP reliability involves manually implementing an acknowledgement system. While TCP uses acknowledgements natively, with UDP, it's something we need to introduce ourselves. Simply put:
- For each packet sent, the sender should pause and wait for an acknowledgement from the receiver.
- If the sender does not receive this acknowledgment within a specified time, they're to try again: resending the packet.
Implementing this system adds another layer of protection but may come with the compromise of slower speed due to 'wait' durations.
Again, imagine it implemented using Python language:
while not received: sock.sendto(PACKET, (UDP_IP, UDP_PORT)) ready = select.select([sock], [], [], timeout) if ready[0]: data, addr = sock.recvfrom(4096) if data: received = True
In this code, we have a while loop that continues sending the PACKET until an acknowledgement (in the form of data) is received.
These methods, checksums and acknowledgements, can help improve the reliability of UDP by ensuring that all transmitted data reaches its destination correctly. However, note that these mechanisms do not guarantee 100% reliability - network issues like latency or jitter may still result in unsuccessful transmissions. Yet, it's undeniably a step forward towards maximizing the potential of UDP as a faster, more reliable protocol.
Yes, the User Datagram Protocol (UDP) can be made reliable by implementing acknowledgement systems. While the inherent nature of UDP is unreliable - as it does not offer any form of guaranteed packet delivery - there are methodologies, such as development and use of appropriate acknowledgement systems, that developers could apply to this protocol in order to create a more reliable UDP.
Let's delve deeper into how these acknowledgement systems work and how they contribute to making UDP reliable.
Acknowledgement System Basics
In simple terms, an acknowledgement system is essentially a way for a receiving computer or device to send a response back to the sending device, confirming that it has received a transmitted packet successfully. This way, the sender knows whether their packets have reached their intended destination correctly.
A basic method of achieving this involves each packet being given a unique identifier by the sending machine. When the receiving machine gets a packet, it sends an acknowledgement back that includes this unique identifier to confirm receipt. If the sending machine fails to receive the acknowledgement within a certain time limit, it re-sends the packet until the acknowledgement is received.
The Effect of Acknowledgement Systems on Creating Reliable UDP
By using such an acknowledgement system, you can compensate for the unreliability of UDP. Here's how:
- Error Detection:
The acknowledgment system allows the server and client to recognize when data was lost during transmission. If a block of data doesn't get recognized, it is identified and can be marked for retransmission. This can ensure complete transmission of data despite temporary network disruptions or packet loss. - Data sequencing:
Using sequence numbers with acknowledgments also means that UDP packets can be put back into the correct order at the receiving end, if need be. This resolves the issue of out-of-order packets which is common with basic UDP transmission. - Rate Adjustment:
By observing how frequently acknowledgements are returned, the sending speed can be adjusted dynamically to match network conditions. This helps prevent network congestion and further increases reliability.
//simple pseudocode example for an acknowledgement system
function sendPacket(packet){
send(packet);
wait_for_acknowledgment(packet.id);
}
function wait_for_acknowledgment(packet_id){
wait(time_limit);
if(!received_acknowledgment_for(packet_id)){
resend(packet_id);
}
}
While building a reliable connection over UDP might be more labor-intensive than simply using TCP (which already handles all of these aspects), there can be specific benefits to a Reliable UDP implementation:
- If your use-case requires the low latency of UDP but also needs reliability, customizing UDP with acknowledgements provides that balance.
- The developer has more direct control over handling dropped packets, providing room for innovative solutions across different distributed systems.
Please note that making UDP 'reliable' means layering features from TCP like delivery acknowledgements and retransmissions over UDP's packet-based structure, thus requiring more resources (computational and potentially network bandwidth).
This approach may not be necessary for applications where occasional packet loss is acceptable - think video streaming or online gaming where minor packet loss does not significantly impact user experience. But for other applications requiring both speed and reliability, an effective acknowledgement system can make UDP a plausible alternative to TCP.
When researching reliable UDP implementations, you may find numerous open-source libraries and extensive documentation online, examples include UDT (UDT homepage), ENet (ENet homepage) and others.
For further in-depth knowledge about TCP/IP protocols including UDP and TCP, I'd highly recommend picking up a copy of "TCP/IP Illustrated" by W. Richard Stevens.
Indeed, we often categorize UDP (User Datagram Protocol) as an unreliable protocol. The primary reason for this label is that it doesn't guarantee data delivery like its counterpart, TCP (Transmission Control Protocol). It simply sends the information without any assurance or verification whether the recipient received the data or not.
However, in some situations and applications, reliability within the UDP framework becomes required. Classifications such as live broadcasts, gaming applications, voice over IP, and streaming applications all use UDP due to its low latency capability. While these applications may tolerate some packet loss, they often still need a certain level of reliability. This gap has fueled the development of Reliable User Datagram Protocol (RUDP).
RUDP, a protocol that resides in the transport layer, introduces reliability features into UDP while maintaining the protocol's simplicity and efficiency. Much like TCP, RUDP uses positive acknowledgements, retransmissions, and sequence numbers to give assurances about data delivery. However, unlike TCP, RUDP tends to require fewer resources and produces less network traffic because it doesn't engage in window scaling or congestion avoidance mechanisms.
Take a look at the intriguing comparison below:
UDP | TCP | RUDP | |
---|---|---|---|
Data Delivery Guarantee | No | Yes | Yes |
Retransmission of Lost Data | No | Yes | Yes |
Packet Sequence/ordering | No | Yes | Yes |
Congestion Avoidance | No | Yes | No |
Error-free Data Transfer | No | Yes | Yes |
In conclusion, although inherently UDP might be unreliable, the flexibility and adaptability of internet protocols present the opportunity to integrate reliability. Via protocols such as the Reliable User Datagram Protocol (RUDP), we can enjoy the best of both worlds--the speed and efficiency of UDP, combined with the assured data delivery of protocols like TCP.
An example of an RUDP-like system implemented in Python code would look somewhat like this:
import socket def reliable_send(data, sock, addr): sock.sendto(data, addr) while True: try: # listen for acknowledgment acknowledgement, server = sock.recvfrom(1024) if acknowledgement == b'ack': break except socket.timeout: # resend data if timeout sock.sendto(data, addr) def main(): client_socket = socket.socket(socket.AF_INET, socket.SOCK_DGRAM) client_socket.settimeout(2.0) # set timeout data = b'some data' server_address = ('localhost', 12345) reliable_send(data, client_socket, server_address) client_socket.close() if __name__ == "__main__": main()
The above snippet illustrates a simple instance where UDP becomes reliable. Upon sending
data
, the sender enters a loop waiting for an acknowledgement from the receiver. If none arrives before the timeout, the method resends the data. The process repeats until an acknowledgement of receipt arrives, enhancing reliability in data transmission.
To gain a comprehensive understanding of the concepts involved in the Reliable User Datagram Protocol (RUDP), you can refer to these following articles: User Datagram Protocol (UDP), and What is Reliable UDP?
Certainly! To answer your question on whether UDP (User Datagram Protocol) can be reliable, first, it's important to understand that by design, UDP is considered an unreliable protocol when compared to TCP (Transmission Control Protocol). This is largely due to the fact that UDP lacks flow control, congestion control, and even error checking.
However, the term "unreliable" doesn't mean that UDP fails to deliver packets. What it means in this context is that the protocol has no built-in mechanism for ensuring delivery of packets or sequence coordination, which are features inherent in TCP.
The lack of reliability mechanisms in UDP is not a flaw but a design choice meant to streamline data transmission, making UDP ideal for real-time services like gaming, streaming, or VOIP where slight packet loss or out of order packets are preferable to the delay of asking for re-transmission.
Now, coming to your question about harnessing packet repetition to improve the reliability of UDP streams, while there's no native method for making UDP "reliable" as TCP, we can add reliability layers to it based on application needs. Some of these methods include:
- Application-Level Acknowledgment: This method involves acknowledging receipt of each packet at the application layer. If a packet isn't acknowledged within a timeout window, it's sent again. The downside is increased overhead due to acknowledgment packets.
- Forward Error Correction (FEC): FEC involves sending redundant data with the original packet so that if some data is lost, it can be reconstructed without needing retransmission. The trade-off here is increased bandwidth usage.
- Packet Repetition: In this approach, each packet is transmitted multiple times to increase the likelihood of successful delivery. This may work well where bandwidth is abundant, but it isn't efficient and causes more network traffic.
Speaking specifically of Packet Repetition, this typically works by having the sender send multiple copies of the same packet at intervals. Because networks are inherently unpredictable and packets can get lost due to many variables (congestion, collisions etc.), repeating the packet minimizes the chance of all instances getting dropped. It's effectively a statistical strategy, with the reliability increasing each time the packet is sent, given that not all packets will be lost during transmission.
Although simple and effective where latency isn't a concern and redundancy is affordable, particularly in sensor networks or broadcast/multicast situations, repeating packets serves more as increased assurance for message transmittal rather than guaranteeing delivery.
To implement Packet Repetition in code form, pseudocode would look like:
procedure sendPacket(packet): repeat N times: send packet over UDP delay(T) end procedure
Where 'N' is the number of repetitions, and 'T' is the delay between each transmission instance.
Keep in mind that with any of these reliability enhancement methods, they will gain you some reliability, but won't fully achieve TCP-level reliability because of fundamental UDP characteristics. At some point, if full dependability is needed, transitioning to a reliable protocol like TCP or implementing a custom reliable protocol over UDP might be more optimal.
For further reading, this paper discusses how UDP's reliability can be improved by employing application-level techniques.Reliability is a critical feature of any communication protocol. In terms of UDP (User Datagram Protocol), it is classically known as an unreliable protocol (GeeksforGeeks, n.d.). Essentially because it doesn't guarantee the delivery of transmitted packets - they may arrive out of order, get lost or duplicate during transit which adversely affects the integrity and trustworthiness of data transferred using UDP.
Albeit, does that mean UDP can’t be reliable? The answer is, not necessarily.
Implementing certain mechanisms at the application level can mitigate the "unreliable" nature of UDP and make it reasonably reliable for your applications. Remarkably, one of such mechanisms to enhance UDP reliability is the use of timers.
Timers in UDP
Timers serve a crucial function for managing packet retransmissions when acknowledgments are not received within expected time frames. If correctly deployed, timers help improve UDP's delivery guarantee, hence substantiating its reliability.
Here’s a basic example illustrating the use of timers in a pseudo code snippet:
send_packet(data){ send(data) start_timer(time_limit) } on_time_out(){ if ACK not received resend_data() else stop_timer() }
In this typical exchange, data is sent, and a timer starts. If an acknowledgment (ACK) isn't received before the timeout, the data is resent. This simple illustration shows how timers can support ensuring data delivery and nurturing trustworthiness in UDP-based communications.
Enhanced Trustworthiness
The primary question here is - How does the use of timers contribute to increasing the trustworthiness of UDP-based communications? Here are some ways:
- Resending Lost Packets: Timers mainly allow for resending any data packets that were lost during transmission, thus eliminating one major cause of unreliability in UDP.
- Duplicate Detection: By implementing unique identifiers for each packet, along with a timer mechanism, applications can detect and handle duplicate packets. This makes sure the receiver isn't processing the same packet multiple times and information gets delivered as intended.
- Improved Ordering: A sequence numbering system combined with timers allows for reordering of packets upon arrival, tackling UDP's inability to guarantee correct order.
It's important to highlight that this form of reliability enhancement doesn't magically equip UDP with all the functionalities that TCP (Transmission Control Protocol) inherently has. No, instead it provides you with the flexibility to implement just enough reliability required by your specific application without inheriting the overheads of TCP, which can impact performance notably in real-time applications.
Beyond this, other strategies such as implementing error detection and correction code, checksums, dealing with network congestion can be coupled with timers to further enhance the reliability and trustworthiness of UDP-based communications (ResearchGate, 2015).
Key takeaway: Timers, though seemingly straightforward, foster improved reliability of UDP-based communications, enabling it to meet varying application-specific needs. However, adopting such reliability enhancement should consider the trade-off between increased complexity and performance demands.For many web applications, User Datagram Protocol (UDP) is an excellent choice for data transmission. Its simplicity, lack of connection-based delivery guarantees, and high-speed performance make it a good fit for real-time data transfer, such as video streaming or online gaming. However, such benefits come with a significant drawback: reliability. In its basic form, UDP does not guarantee the delivery of packets sent over the network, which may lead to the risk of losing packets or data fragments during transmission.
Can UDP be made reliable? Absolutely! Despite its inherently unreliable nature, we can still adopt several strategies that allow us to use UDP while overcoming the problem of lost data fragments, making it a more reliable choice.
Retransmission of Lost Data Fragments:
One of the most common ways to deal with lost data fragments in UDP transmissions is through retransmission. If the receiving client detects a missing fragment in the UDP packet sequence, it can request that the sender resends it. Here's a brief example:
// The receiver side if(sequenceNumber != expectedSequenceNumber){ // Request to resend the packet }
Implementing Error Correction:
This strategy is beneficial for reducing the quantity of requested retransmissions. By applying error correction techniques such as Reed-Solomon codes, it's possible to reconstruct lost data without requesting resends. Error correction embeds some extra redundancy data within the packets, enabling the recipient to fill in gaps if some fragments are lost.
Using Sequence Numbers:
Adding a sequence number to each UDP packet can help the receiver detect missing packets. In this approach, the sending application attaches a unique sequence number to each successive packet. When the recipient receives these packets, it checks whether the sequence numbers are in order or if there are gaps implying lost packets.
// Sending side packet.sequenceNumber = currentSequenceNumber++; // Send the packet
Acknowledgement Mechanism:
One additional technique would be sending an acknowledgement for every received package. If an acknowledgement is not received within a certain time limit, the sender could assume the packet is lost and resend it.
// Sending side if(!receivedAckWithinTimeout()){ // Resend the packet }
Forward Error Correction (FEC):
FEC involves creating a mathematical function describing a series of packets being sent in one UDP transmission. This function allows the receiving system to recreate any lost packets without needing to have them retransmitted. FEC can be particularly useful in environments where latency must be kept to a bare minimum, like in live broadcasts or gaming scenarios.
Notably, these strategies boost UDP’s reliability by mimicking mechanisms inherent to TCP (Transmission Control Protocol), a commonly-used alternative to UDP renowned for its reliability. Such a process of incorporating dependable delivery features into UDP is known as 'Reliable UDP' or 'RUDP'. It provides developers with the best of both worlds - the speed and efficiency of UDP coupled with added reliability measures.
It's crucial to remember that these solutions add overhead to UDP communication, requiring additional coding, computational effort, and potentially decreased performance. Therefore, when adopting these strategies, one must evaluate the trade-offs between speed and data integrity according to specific application requirements.
In summary, while UDP in its primitive form does carry risks related to data fragment loss, these issues can be mitigated using the outlined techniques. Therefore, UDP can indeed be transformed into a reliable means of data transmission given forethought, measured decisions, and purposeful implementation. For comprehensive details regarding these concepts, consider consulting RFC-908, which explains the idea of Reliable UDP in depth.
Combining TCP (Transmission Control Protocol) and UDP (User Datagram Protocol) for more dependable data delivery is a fascinating possibility. In network protocol conversations, TCP is generally appreciated for its reliability, as it offers error detection features together with a powerful mechanism to guarantee the successful delivery of information packets. On the other hand, UDP is known for speed. It doesn't provide delivery assurance yet delivers information quicker because of lesser congestion control.'
Can UDP be Reliable?
Yes, UDP can become reliable if additional methods are employed to create certain TCP-like features. Here are a few ways this can be achieved:
- An application-level acknowledgment scheme: The receiving application could send feedback acknowledgments back to the sender. If the sender doesn't get an acknowledgment within a specific period, it may retransmit the packet.
- Error correction mechanisms: Parity checks or other checksum methodologies can be implemented to identify corrupted packets.
- Ordering assurances: Similar to TCP, sequencing numbers can be added to each packet so that they can be reordered appropriately on the receiver side.
However, implementing these strategies at the application level is not standard, and proper design is crucial to avoid potential issues such as duplicate packets, out-of-order arrival, and packet loss.
Prospects of Combining TCP & UDP
By blending TCP and UDP, we may try to capitalize on the strengths of both protocols. Typically, applications use either one or the other -- TCP when reliability is critical and UDP when speed weighs heavier than guaranteed delivery.
Quic (Quick UDP Internet Connections), established by Google, incorporates TCP's reliability and UDP's speed. Quic offers reduced connection establishment time, better congestion control, and more robust loss recovery compared to TCP, all in a fast, encryption-focused package like UDP.
Advantages of Quic:
- Faster connections: Quic reduces the time it takes to establish a connection. This feature brings significant improvement to services where real-time communication is essential.
- Integrated Security: Quic includes standard encryption, using TLS protocols, which enhances the security of data transmission.
- Multiplexing without head-of-line blocking: Unlike TCP, QUIC allows multiple streams over a single connection, reducing delay and improving connection performance.
While Quic has tremendous advantages, its acceptance isn't universal yet. However, it shows the practical implications of integrating the best features from both TCP and UDP into a new suite. As technology progresses, further opportunities might open up for additional reliable data transfer standards that combine desirable elements from both existing protocols. An example of a quic code implementation will look like:
var quic = require('@node-quic/quic'); var server = quic.createSocket({ endpoint: { port: 1234 } }) .on('session', function(session) { session.on('stream', function(stream) { stream.respond(); // stream.body is a readable stream stream.body.pipe(process.stdout); // stream.end() returns a promise stream.end('hello, client!\n').catch(console.error); }); }).listen(); server.setTimeout(5000);
To reference documentation about the usage of QUIC protocol, you can check this out [here](https://www.chromium.org/quic).While it's counterintuitive to think of UDP (User Datagram Protocol) as reliable due to its lacking error checking and retransmit features, we can utilize certain methods to make UDP communications more reliable.
To understand this whole concept, first, let us clarify the inherent nature of UDP; it is a connectionless and non-reliable protocol by design. This basically means that UDP does not require establishing a connection before sending data, and it doesn't ensure the error-free delivery of data. It simply sends the data without any promise of its successful receipt at the destination.
socket = socket.socket(socket.AF_INET, socket.SOCK_DGRAM) data = "Hello, world!" socket.sendto(data.encode(), ('localhost', 8080))
In the provided Python code snippet, there are no checks or guarantees about whether or not the message ("Hello, world!") has actually been received where it was sent too.
However, suppose the application requires reliability. In that case, this must be implemented in the application layer through various mechanisms, such as sequence numbers, acknowledgments, and timed resends when necessary. For example, in some game servers or VOIP (Voice Over Internet Protocol) systems, a hand-crafted form of reliability is implemented on top of UDP for better control over the data transmission process.
This mechanism can be likened to sending a registered mail package. Even though the mail system (analogous to UDP) doesn't itself ensure that the package reaches its destination, the sender can add extra services like tracking and confirmation of delivery (akin to application-implemented reliability) to make sure that the package arrives.
Ultimately, while automation of these features comes as default with TCP (Transmission Control Protocol), using UDP instead can give programmers a higher degree of flexibility and performance optimization. This is particularly relevant for real-time or streaming applications where strict delivery order and timing is less important than overall throughput.
There are libraries such as Reliable.io which provide these functionalities out of the box for those who want to use UDP but still need the reliability similar to TCP.
For most ordinary applications, TCP remains the easiest option because it takes care of these issues automatically. However, UDP can indeed be made "reliable" to an extent, and for certain specific domains, it might even be the more suitable choice given its unique benefits. Understanding when to use each speaks to skilfully navigating the wide landscape of network programming.
For further reading on how to make UDP reliable, the following resources Gaffer On Games and John Hopkins University resource are recommended.