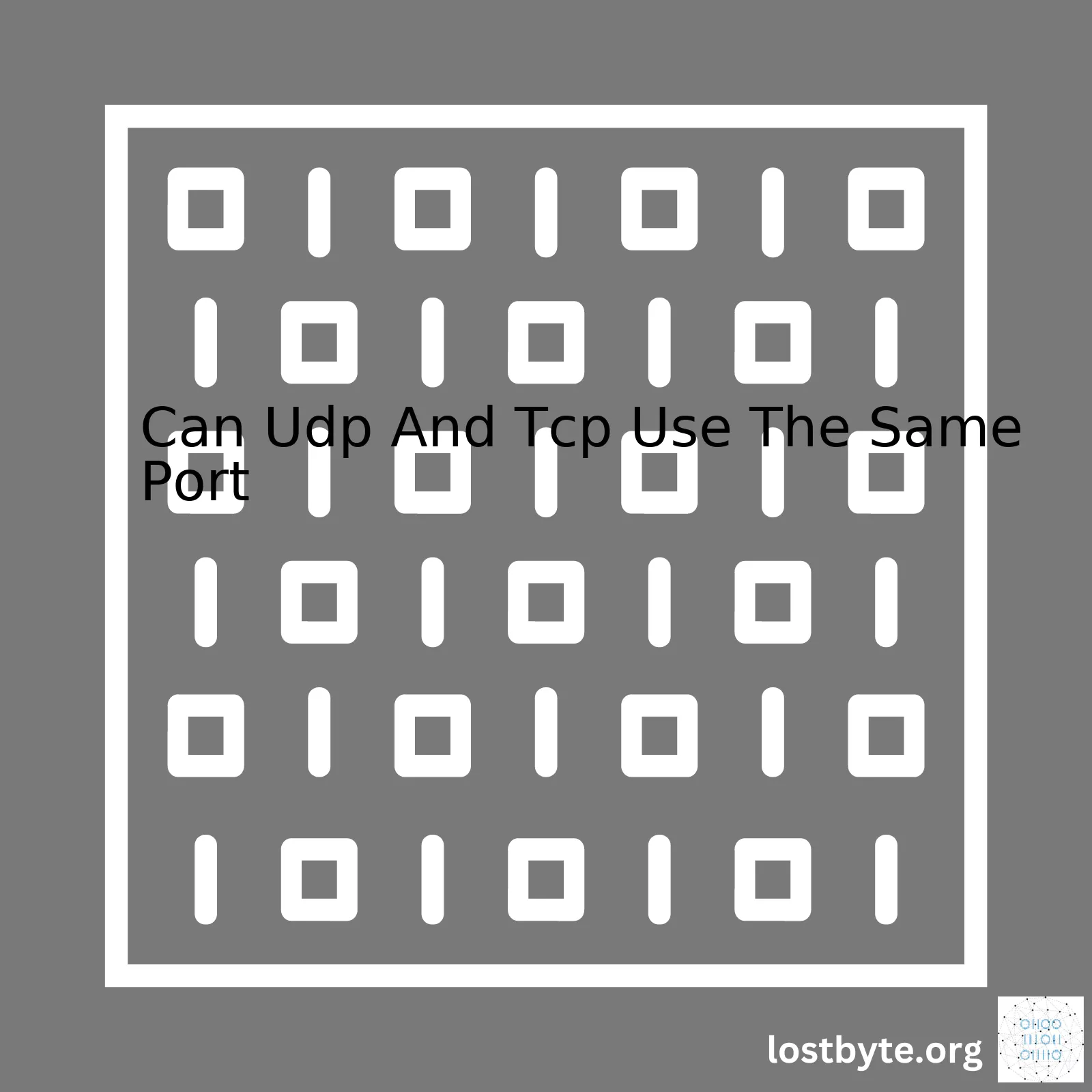
The question of whether UDP (User Datagram Protocol) and TCP (Transmission Control Protocol) can use the same port can be broken down into a simple statement: Yes, they can. The reason lies in how these two protocols are defined and how the system interprets them. Specifically, the pair of IP address and port identifies a specific process running on a machine and listening for incoming connections or data. However, ports from TCP and UDP are in different namespaces which means they can share the same numerical value without conflicting.
Category | TCP | UDP |
---|---|---|
Port Availability | Can share the same numerical port value with UDP | Can share the same numerical port value with TCP |
Namespaces | Has separate namespace from UDP | Has separate namespace from TCP |
Data Packet Delivery | Guaranteed delivery but slower due to connection setup and teardown for each packet sent | No guaranteed delivery but faster than TCP as it doesn’t require acknowledgment of each packet received |
To exemplify this, both a TCP and UDP service can bind to localhost (127.0.0.1) at the same port number, let’s say 8080, without any conflicts. This is because even though the numerical values of the ports are the same, the protocols differentiate them. Without going too deep into networking principles, you can think of it as having one post office box that accepts both standard mail, represented by TCP, and courier packages, symbolized by UDP. They arrive at the same physical location, but the handling of them differs drastically based on their nature.
// TCP server using port 8080 var net = require('net'); var server = net.createServer(); server.listen(8080, '127.0.0.1'); // UDP server also using port 8080 var dgram = require('dgram'); var server = dgram.createSocket('udp4'); server.bind(8080, '127.0.0.1');
While both TCP and UDP can technically utilize the same port without conflict, it is essential to remember that each protocol serves a distinct purpose and should not be confused or combined. Knowing when to use TCP versus UDP is critical to ensuring efficient and reliable network communication.
For more detailed information about TCP and UDP protocols, I refer to sources like Guru99 and Wikipedia.
Sure, let’s delve into the complex world of UDP and TCP protocols. Now, these two are key internet protocols that we’d often use without even realizing it. Yet, there’s a burning question to address: Can UDP and TCP use the same port? For the uninitiated, ports in context of computer networking, are communication endpoints.
Understanding UDP
UDP
, or User Datagram Protocol, is what we refer to when we’re talking about connectionless protocols. The simple, transmission-based protocol doesn’t call for any initiation of a handshake process or confirmation of data receipt. Here are key attributes:
- Doesn’t require an established connection.
- Packet delivery isn’t guaranteed but quicker.
- No congestion handling.
Understanding TCP
On the other hand, the Transmission Control Protocol,
TCP
, involves making an established connection before transferring data. This complexity allows it to offer guaranteed packet delivery and manages packet order, helping you avoid data corruption during transmission. Key elements include:
- Connection-orientated protocol. A handshake process initializes the communication.
- Packets are checked for errors.
- It caters to congestion control, flow control and reliability.
Can UDP and TCP Use The Same Port?
Now let’s tackle the main question. Essentially, UDP and TCP are independent protocols; hence they may use the same port number without any conflict1. Our operating system differentiates between active sessions using the combination of protocol (TCP/UDP), source IP, destination IP, source port and destination port. This lets TCP and UDP connections coexist peacefully without interfering with one another – even on the same port.
To highlight this further, let’s use a coding example as follows where we create a TCP server and a UDP server running on the same port2.
import socket # Creating a TCP server tcp_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM) tcp_socket.bind(('localhost', 55000)) tcp_socket.listen(1) # Creating a UDP server udp_socket = socket.socket(socket.AF_INET, socket.SOCK_DGRAM) udp_socket.bind(('localhost', 55000)) # Further implementation
In this script, we successfully bind a TCP and a UDP server to the same port without any issues because the operating system treats them as distinct processes based on the specified protocol.
In closing, TCP and UDP can work side by side using the same port numbers due to their independent nature. That said, they serve unique roles in network communication, so choose the one fitting your application’s needs.
The internet comprises numerous protocols interacting to facilitate data transfer. Two of these quintessential protocols are the User Datagram Protocol (UDP) and the Transmission Control Protocol (TCP). These two play key roles in the transportation layer, with significant but differentiating functionalities.
User Datagram Protocol (UDP)
UDP is a connectionless protocol, meaning it isn’t necessary for a connection to be established before data is sent. Differentiating features of UDP include:
- It prioritizes simplicity and speed over data reliability
- It doesn’t require a handshake, so messages are immediately sent without undergoing verification
- Data packets sometimes get lost during transmission due to its lack of error-checking mechanisms
import socket UDP_IP = "127.0.0.1" UDP_PORT = 5005 MESSAGE = "Hello, World!" print("UDP target IP:", UDP_IP) print("UDP target port:", UDP_PORT) print("message:", MESSAGE) sock = socket.socket(socket.AF_INET, # Internet socket.SOCK_DGRAM) # UDP sock.sendto(MESSAGE, (UDP_IP, UDP_PORT))
Transmission Control Protocol (TCP)
In contrast, TCP functions based on establishing a strong connection before sending any data. TCP’s salient features comprise:
- TCP guarantees delivery of data packets due to its error-checking and recovery attributes.
- It organizes data packets so they are transmitted sequentially.
- It manages data packet size by splitting data into smaller packets if necessary.
import socket TCP_IP = '127.0.0.1' TCP_PORT = 5005 BUFFER_SIZE = 1024 MESSAGE = b"Hello, World!" s = socket.socket(socket.AF_INET, socket.SOCK_STREAM) s.connect((TCP_IP, TCP_PORT)) s.send(MESSAGE) data = s.recv(BUFFER_SIZE) s.close() print ("received data:", data)
Can UDP and TCP Use The same Port?
To answer this query, we need to adopt a technical perspective. A port can be utilized by either TCP or UDP, but not simultaneously as per their design principles. Here’s why:
– Ports are just virtual gateways enabling applications to share information via TCP or UDP.
– The port used by an application is differentiated by the IP address and the transport protocol; hence, technically speaking, there could concurrently exist a UDP and a TCP connection open that leverage the same local IP and port.
– It equates only to mere coincidence if a TCP connection coincides with a UDP connection using similar IP addresses and ports, since these connections don’t communicate or have anything common between them.
So ultimately, UDP and TCP are entirely distinct on a protocol basis and physically individual from the perspective of records held within a machine for routing purposes – thereby permitting flexibility to utilize the same port number across these protocols independently.
For elaborate details on how UDP and TCP work, I highly recommend checking out this thorough comparison article.
Absolutely, both the User Datagram Protocol (UDP) and Transmission Control Protocol (TCP) can use the same port number. However, it’s critical to understand that TCP and UDP are distinct transport protocols. Consequently, even if they use the same port numbers, they are handled as different streams of data.
Look at it this way: when a device or program communicates over the internet, it sends a series of packets via TCP or UDP. The destination port number tells the receiving system which application should receive the packet. A computer can differentiate between TCP and UDP traffic going to the same port because each protocol has a distinctive header.
Therefore, having a TCP server on port 8080 and a UDP server on port 8080 is totally feasible. Remember that the combination of IP, protocol (UDP or TCP), source port, and destination port uniquely identify each network stream, so there’s no overlap or conflict.
Here’s an analogy for clarity: think of TCP and UDP as two separate mail services such as FedEx and UPS, and consider the ports as the suite numbers in a building. FedEx delivery (TCP) and UPS delivery (UDP) can both deliver packages to Suite 8080 without any confusion because they are separate ‘services’ despite using the same ‘suite.’
Consider the following simple
Python
example for creating a socket for UDP and TCP:
For a TCP socket:
import socket s = socket.socket(socket.AF_INET, socket.SOCK_STREAM) s.bind(("localhost", 8080)) s.listen(1)
And for a UDP socket:
import socket s = socket.socket(socket.AF_INET, socket.SOCK_DGRAM) s.bind(("localhost", 8080))
Now those two can run simultaneously without any issues since they use different protocols.
The differentiation between TCP and UDP traffic on the same port is also facilitated by the operating system’s networking stack. Information about the protocol used (UDP or TCP, from the IP layer) is maintained along with the datagrams throughout their lifetime within the computer’s network stack. Because of this differentiation mechanism, applications can listen to the same port number on both UDP and TCP without any port conflict.
That said, having both TCP and UDP servers listening on the same port might not be common or practical, but technically, it’s entirely possible.
If you’re interested in delving deeper into how TCP/IP works, I’d recommend picking up a copy of “TCP/IP Illustrated”. It provides comprehensive and detailed coverage on TCP/IP protocols, ideal for anyone involved in networking development, administration, and education.Unpacking the Network Architecture: Understanding Ports in Relation to TCP and UDP Using the Same Port
Transmission Control Protocol (TCP) and User Datagram Protocol (UDP) are fundamental elements of network architecture. They both utilize ports as interaction endpoints where network communication begins and terminates, allowing cummunication even if multiple applications are running.
Understanding TCP and UDP
TCP is a connection-oriented protocol that involves setting up a clear link between the sender and receiver before data transfer. It ensures that data arrive at their destination without errors and in order. Conversely, UDP is a connection-less protocol which does not guarantee delivery by losing the meticulous processes of handshaking and error-checking typical to TCP. Instead, it is often used for tasks requiring minimum latency like online gaming or streaming.
A Little More on Ports
In terms of network topology, ports act as doors through which information enters or exits a device or system. Ports are numbered and associated with specific protocols to ensure data ends up in the right application. Internet-assigned port numbers range from 0 to 65535, and are categorized into three types:
Port Number Range | Type |
---|---|
0 – 1023 | Well-known ports |
1024 – 49151 | Registered ports |
49152 – 65535 | Dynamic or private ports |
Let’s move onto the underpinning question: Can TCP and UDP use the same port?
When TCP and UDP Share the Same Port:
Given that TCP and UDP are two distinct protocols, they can indeed employ the same port number without conflict since they manage their connections differently. The combination of IP address, protocol (TCP or UDP), and port number define a unique socket—a place of interaction for application processes. Consequently, TCP and UDP could successfully use the same port without leading to a ‘race condition’ as they are distinguished by their respective protocols.
To illustrate, you might surf a website via an HTTP request on TCP port 80 while simultaneously transmitting a DNS query – using UDP port 80 – to translate a domain name. Your operating system differentiates these connections despite using the same port, attributing them to separate instances happening over TCP and UDP respectively.
For example, you may have two connection tuples that look something like this:
(192.0.2.1:12345 -> 203.0.113.1:80/TCP)
and
(192.0.2.1:12345 -> 203.0.113.1:80/UDP)
Each tuple is separate, despite sharing ports and IP addresses, thanks to the differentiation of protocols. However, bear in mind that utilizing the same port across different protocols does not usually occur in common practice, as certain port numbers are conventionally used for well-defined services.
References:
TCP Protocol |
UDP Protocol |
Port Numbers
TCP and UDP using the same port is possible in a network because TCP and UDP packets will be distinguished based on the protocol type. Here’s an interesting fact: both TCP and UDP can use ports within the range of 0 to 65535 [source](https://stackoverflow.com/questions/27687867/is-it-possible-that-tcp-and-udp-sockets-have-same-port). However, the system recognizes them as separate entities because they’re processed separately.
Let’s take you through some crucial points about this concurrency:
– **TCP (Transmission Control Protocol) and UDP (User Datagram Protocol) are two core Internet protocols.** TCP offers error checking and sequencing of packets, providing reliable communication. On the other hand, UDP offers minimal packet overhead, resulting in faster transmission but with less reliability.
var server = require('net').createServer(); var udp_client = require('dgram').createSocket('udp4'); server.on('connection', function(sock) { /* handle incoming connections */ }); udp_client.on('listening', function () { /* handle incoming datagrams */ });
This example signifies how TCP and UDP can share the same port in Node.js. The TCP server is set up to listen for incoming connections while the UDP client listens for incoming UDP datagrams.
– **Distinct Sockets**: When a service gets a message over a socket, it checks not only the port number but also the underlying protocol. This dual check is the reason why both protocols can comfortably share the same port without interference. They act independently on different “sockets,” facilitating the concurrent processing of data.
– **Handling Concurrent Requests**: Two types of data (TCP/UDP) can arrive concurrently at the same port. The nature of the protocol (TCP being connection-oriented and UDP being connection-less) means they’ll handle these requests differently. For instance, TCP establishes a reliable communication pipeline between the sender and receiver before data transfer, ensuring no loss of information. UDP, however, sends the data without prior connection establishment, prioritizing speed over reliability.
socket.bind({ address: 'localhost', port: 8000, exclusive: true });
In the sample code above written in Node.js, TCP and UDP use the same port by binding them to the same local address and port. The `exclusive: true` option prevents port sharing.
– **Potential Benefits**: Using identical ports has its upsides. It reduces the need for additional open ports when services can share the same one efficiently, reducing potential security risks. This approach works particularly well where linked services predominantly use one protocol but occasionally resort to the second for specific functions.
While all this suggests compatibility, do bear in mind that implementing TCP and UDP on the same port is highly circumstantial and might not always be the optimal solution. It may work in specific instances – like the FTP protocol, which employs TCP port 21 for command transmission and TCP port 20 for actual data exchange – but might not necessarily perform optimally across all networks.
Protocol | Error Checking | Sequencing | Reliability |
---|---|---|---|
TCP | Yes | Yes | High |
UDP | No | No | Low |
In summary, TCP and UDP staying apart or sharing a common port purely depends on the design considerations. Consider the requirement, weigh the pros and cons, evaluate the trade-offs to make the best use of these protocols. TCP and UDP are two robust transport layer protocols in the Internet Protocol Suite often used in various networking applications. While they both use port numbers for connection purposes, their manner of operation is significantly different.
The concept of ports is crucial to both TCP and UDP protocols as it helps establish communication between applications. A port number helps identify a specific process to which an internet or network message is to be forwarded when it arrives at a server. System ports range from 0 – 1023, user-registered ports from 1024 – 49151, and dynamic or private ports from 49152 – 65535.
At first glance, you might think that TCP and UDP using the same port might encounter conflicts, but the reality is a bit more nuanced. TCP and UDP are identified uniquely using a combination of IP address and port number, creating what we call a socket. Importantly, these protocols are separate from one another, resulting in unique sockets even if they share the same port number.
For example, let’s consider an application listening on UDP and another on TCP at port X:
> UDP_App: (_) -- [192.1.2.3:4000]
> TCP_App: (_) -- [192.1.2.3:4000]
In this case, even though the port number (4000) is the same, the protocol is different for each application (UDP vs TCP), so there isn’t any conflict. Each of these makes a distinct socket, facilitating smooth parallel operations without causing overlap or collision in data handling.
However, there are certain constraints that arise when both TCP and UDP operate on the same port, which are the result of inherent characteristics and operational differences of the protocols themselves:
- Packet Delivery: UDP delivers information in packets. If there’s a glitch in the network leading to packet loss or corruption, UDP does not have mechanisms to detect or recover it. On the other hand, TCP is equipped with error-checking mechanisms to ensure safe delivery by retransmitting lost packets.
-
Connection Establishment:
TCP requires a handshake protocol to establish a communication session before transmitting data, which ensures a reliable connection. In contrast, UDP tends to be connectionless, meaning it can send packets without establishing a connection, making it faster but less reliable. - Order of Data: TCP maintains the sequence of transported data, while UDP may send data out of order. This fundamental difference impacts applications that require data in a specific sequence.
- Usage Constraints: Not all applications can handle both TCP and UDP. For some multimedia streaming or online gaming applications, speed is more critical than reliability. So UDP would be preferred. However, web browsing or email services that prioritize data integrity would likely choose TCP.
Despite the constraints and operational differences, TCP and UDP can coexist on the same port number because the system views them as entirely separate protocols. However, the choice between the two boils down to the specific requirements of the application in question.
More details about these protocols can be found in RFC 793 for Transmission Control Protocol and RFC 768 for User Datagram Protocol.There’s a common misunderstanding that Tranmission Control Protocol (TCP) and User Datagram Protocol (UDP), as two different Internet protocols, cannot share the same port number on the same host. In reality, this is not true. TCP and UDP are indeed two different protocols, with considerable differences in their mechanism, each having its exclusive headroom for port numbers.
Here are some fascinating insights about TCP and UDP protocol duality w.r.t to ports:
Different Protocols, Different Tables: The operating system keeps separate tables to track UDP and TCP connections. So, TCP and UDP requests are processed differently because their ‘port’ data is stored separately. This means that a UDP and a TCP connection can use the same port without any conflict since they will have distinct entries in their respective tables.
In simple terms, when a packet arrives at the device, the OS uses a combination of the transport protocol and port number to decide how to handle incoming packets. Here’s a small illustration of what I meant:
// For instance, these two would be treated as different services TCP 127.0.0.1:3000 UDP 127.0.0.1:3000
Simultaneously Running Services: It’s entirely possible to have a UDP-based service and a TCP-based service running simultaneously on the same port. They do not hinder each other because different network stacks process them.
For example, DNS operates on both TCP and UDP on port 53. Even HTTP/3 is planning to overlap UDP and TCP ports.
No Data Overlap: One more point to highlight here is that the data sent via a UDP socket associated with a port won’t ever be received by a listener for TCP traffic on the same port, or vice versa. That’s just not how the internet stack works.
To understand this better, you must realize that even though both TCP and UDP use ports, they are different in their essence. TCP is like a package delivery service that ensures your package gets from one place to another accurately and reliably. On the other hand, UDP does not offer such reliability but is faster instead.
A real-life analogy could be envisioning ports as ‘apartments,’ TCP and UDP as different ‘mail carriers.’ Even if they share the same apartment number (port), the mail (data) they deliver stays separate because they work independent of each other.
In an interesting read titled Can TCP and UDP traffic run on the same port? discussed on StackOverflow, various network experts and coders alike shared similar views on protocol duality and its feasibility.
This brings us to dispel the misconception and affirm that yes, TCP and UDP can indeed use the same port number, working coherently without interference. Protocol dualism is challenging due to the inherent difference between TCP and UDP, but always possible.
Transmission Control Protocol (TCP) and User Datagram Protocol (UDP) are both transport layer protocols that are used to facilitate data communications in a network. Even though they serve similar functions, the way they handle data is very different, making each more ideal for specific situations more than the other. Despite these differences, it’s interesting to note that TCP and UDP can actually use the same port number. But before we dive into that, let’s briefly understand what TCP and UDP are and the difference between them.
TCP is a connection-oriented protocol meaning before any communication happens, a connection must be established between the sender and receiver. This guarantees that packets sent will all arrive at the destination intact and in order. On the flip side, UDP is connectionless. It sends packets without establishing a connection thus reducing latency but doesn’t guarantee the order or the arrival of packets.
Now, coming back to your question, “Can TCP and UDP use the same port?” Here’s the answer: Yes, they can. This is because TCP and UDP each maintain their own separate list of port numbers. Therefore, a TCP connection and a UDP datagram might share the same number, without causing any conflicts. Below are some real-life scenarios where both TCP and UDP utilize the same port:
DNS
The Domain Name System (DNS), uses both TCP and UDP on port 53. As per the DNS specifications (RFC 1035), smaller requests/responses or queries generally use UDP while larger ones like zone transfers use TCP.
DNS over UDP: QUERY - Client -> Server : Standard query 0x1234 A www.test.com REPLY - Server -> Client : Standard query response 0x1234 A 192.0.2.10 DNS over TCP: [Three-Way Handshake here] QUERY - Client -> Server : Standard query 0x5678 AXFR test.com REPLY - Server -> Client : Standard query response 0x5678 AXFR multiple replies ... [Additional conversation as required] ... - Server -> Client : Last reply, terminates connection
HTTP and HTTPs
HTTP operates on TCP port 80 and HTTPS utilizes TCP port 443. For instance, QUIC, which stands for Quick UDP Internet Connections, is an experimental protocol developed by Google. QUIC supports a set of multiplexed connections between two endpoints over UDP, and it was designed to provide security protection equivalent to TLS/SSL, along with reduced connection and transport latency. Thus, it’s possible to see UDP being utilized on the traditionally TCP port 443.
Standard HTTP over TCP: - Client -> Server : GET /index.html Standard HTTPS over TCP: - Client -> Server : CONNECT www.example.com:443 QUIC (HTTPS over UDP): - Client -> Server : STREAM frame containing GET /index.html
In summary, while TCP and UDP are distinct protocols servicing different use cases, they can indeed use the same port numbers. However, this does not create a conflict as they maintain separate lists for port numbers, acting independently of each other.
TCP (Transmission Control Protocol) and UDP (User Datagram Protocol) are transport layer protocols in the TCP/IP model and both can use identical port numbers. However, their performance varies greatly due to their underlying mechanisms and purposes.
TCP:
It’s a connection-oriented protocol and offers:
– Reliable transmission of packets.
– Ordered delivery of packets.
– Error-checking functionality with acknowledgments and retransmissions.
– Flow control & congestion control.
All these features lead to overhead costs and delay in communication but ensure the reliability of data transfer.
import socket SERVER = "localhost" PORT = 8080 client = socket.socket(socket.AF_INET, socket.SOCK_STREAM) client.connect((SERVER, PORT)) client.sendall(bytes("This is from Client",'UTF-8'))
UDP:
On the contrary, UDP is a connection-less protocol and offers:
– No guarantee of packet delivery and no acknowledgment scheme.
– No order of delivery.
– It lacks the congestion control feature.
– Low latency and overhead compared to TCP.
Due to its simplicity and speed, UDP is ideal for real-time applications like video streaming or gaming where dropped packets are preferable to delayed packets.
import socket msgFromClient = "Hello UDP Server" bytesToSend = str.encode(msgFromClient) serverAddressPort = ("127.0.0.1", 20001) bufferSize = 1024 # Create a UDP socket at client side UDPClientSocket = socket.socket(family=socket.AF_INET, type=socket.SOCK_DGRAM) # Send to server using created UDP socket UDPClientSocket.sendto(bytesToSend, serverAddressPort)
Regarding your inquiry “Can Udp And Tcp Use The Same Port?”, the answer is YES. From an OS perspective, each unique socket (the combination of source IP, source port, destination IP, destination port, and protocol) defines a unique connection [RFC793]. This means that a TCP and UDP connection operating on the same port number (i.e., 53 for DNS) are different because they use different protocols.
Below table further compares the two protocols regarding their performance:
| | TCP | UDP |
|———————-|:—————————:|:——————————:|
| Connection | Connection-oriented | Connection-less |
| Reliability | Yes, ensures reliable transmission | Unreliable |
| Delivery Order | Guaranteed | Not guaranteed |
| Control Features | Flow & Congestion control | No controls |
| Speed | Slow due to controls & header size | Fast due to low overhead |
| Ideal Applications | Web browsers, email, file transfer | Video streaming, gaming, VoIP |
When developing network applications, understanding the underlying protocols is crucial for optimizing data transmission. User Datagram Protocol (UDP) and Transmission Control Protocol (TCP) both play a significant role in data transfer. However, they serve unique objectives.
Before we dig into whether UDP and TCP can share ports, let’s understand these protocols individually.
/* Example of TCP connection */ Socket clientSocket = new Socket("hostname", 6789); DataOutputStream outToServer = new DataOutputStream(clientSocket.getOutputStream());
TCP establishes secure connections before transmitting data. It carries an overhead cost due to handshaking, ensuring that packets reach their destination in order and without errors.
/* Example of UDP connection */ DatagramSocket clientSocket = new DatagramSocket(); InetAddress IPAddress = InetAddress.getByName("hostname"); byte[] sendData = new byte[1024]; DatagramPacket sendPacket = new DatagramPacket(sendData, sendData.length, IPAddress, 9876); clientSocket.send(sendPacket);
On the other hand, UDP favors speed over perfection. It just shoots off data without ensuring if the recipient is ready or even available, reducing latency in real-time applications.
Can UDP and TCP Use the Same Port?
Absolutely. The most critical aspect to note here is that a port isn’t limited to a single protocol. Ports are merely entry points identified by integers between 0-65535 on a host for specific processes or services. TCP and UDP are independent protocols and operate on different layers of the networking stack. Thus, theoretically, they can share the same port number on a server or device – they don’t collide or interfere with each other.
The decision to use the same or separate ports for each protocol comes from the nature of the application. If an application employs hybrid TCP/UDP model benefiting from the strengths of each protocol, utilizing shared or different ports becomes a strategic consideration.
If a service uses TCP and UDP to manage distinct types of traffic, using the same port can efficiently split the bandwidth between the two protocols, aiding smoother operation. DNS servers are the fitting examples where the data transmission efficiency is indeed enhanced by adopting a hybrid model.
Both protocols used in synchronization:
1. The service begins by sending a DNS query via UDP through port 53.
2. If the request fails or the response is too large (>512 bytes), the service repeats the query using TCP. This serves as a reliable backup should any packet loss occur, maintaining resiliency while still offering dynamic, efficient response times.
Therefore, by choosing a strategy that capitalizes on the strengths of both protocols, data transmission efficiency can indeed be enhanced. For further reading, you could refer to this additional resource TCP vs. UDP..
In short, yes, both UDP (User Datagram Protocol) and TCP (Transmission Control Protocol) can use the same port number. In the context of computer networking, these two protocols serve different purposes but they coexist at the transport layer of the OSI model.
Let’s have a deep dive into why:
– TCP and UDP are distinct protocols: Despite often being discussed in parallel, TCP and UDP are unique in their logic and purpose—TCP is connection-oriented, guaranteeing that every data packet reaches its desired destination, while UDP is connectionless and does not provide such assurances. This fundamental distinction enables them to exist independently and concurrently operate on the same port number without conflict.
– How Systems Differentiate Between TCP and UDP: Under the hood, every piece of communication data, also known as a “packet”, is tagged with protocol information. As a simple example, imagine sending two letters through the mail: one by standard postal service (UDP) and one by registered mail with tracking (TCP). Both could be sent to the same address (port number), but the postal system (network stack) would handle them differently based on their type.
In available code libraries, when you are defining a socket using either TCP or UDP, you specify this within the parameters. For example, in Python’s socket library, creating a TCP socket might look like:
import socket s = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
And creating a UDP socket could resemble:
import socket s = socket.socket(socket.AF_INET, socket.SOCK_DGRAM)
In both samples, we’re creating sockets bound to the IP protocol (AF_INET), but TCP uses SOCK_STREAM while UDP utilizes SOCK_DGRAM. They will inherently avoid overlapping due to their distinct operational nature in the network stack.
– Choosing Between TCP and UDP: Understanding when to use TCP or UDP comes down to the requirements of your application. If data delivery with reliability and ordering is utmost important, using TCP may be necessary. However, if speed takes precedence over guarantees of packet arrival, you might opt for UDP. Games often use UDP for this very reason, sacrificing occasionally lost packets for real-time performance.
For more sophisticated reading, RFC 793 thoroughly explores TCP, whereas RFC 768 discusses UDP in depth. Acknowledging the requirements of your specific scenario will lead to an informed decision about whether to use TCP, UDP, or even both simultaneously on the same port.