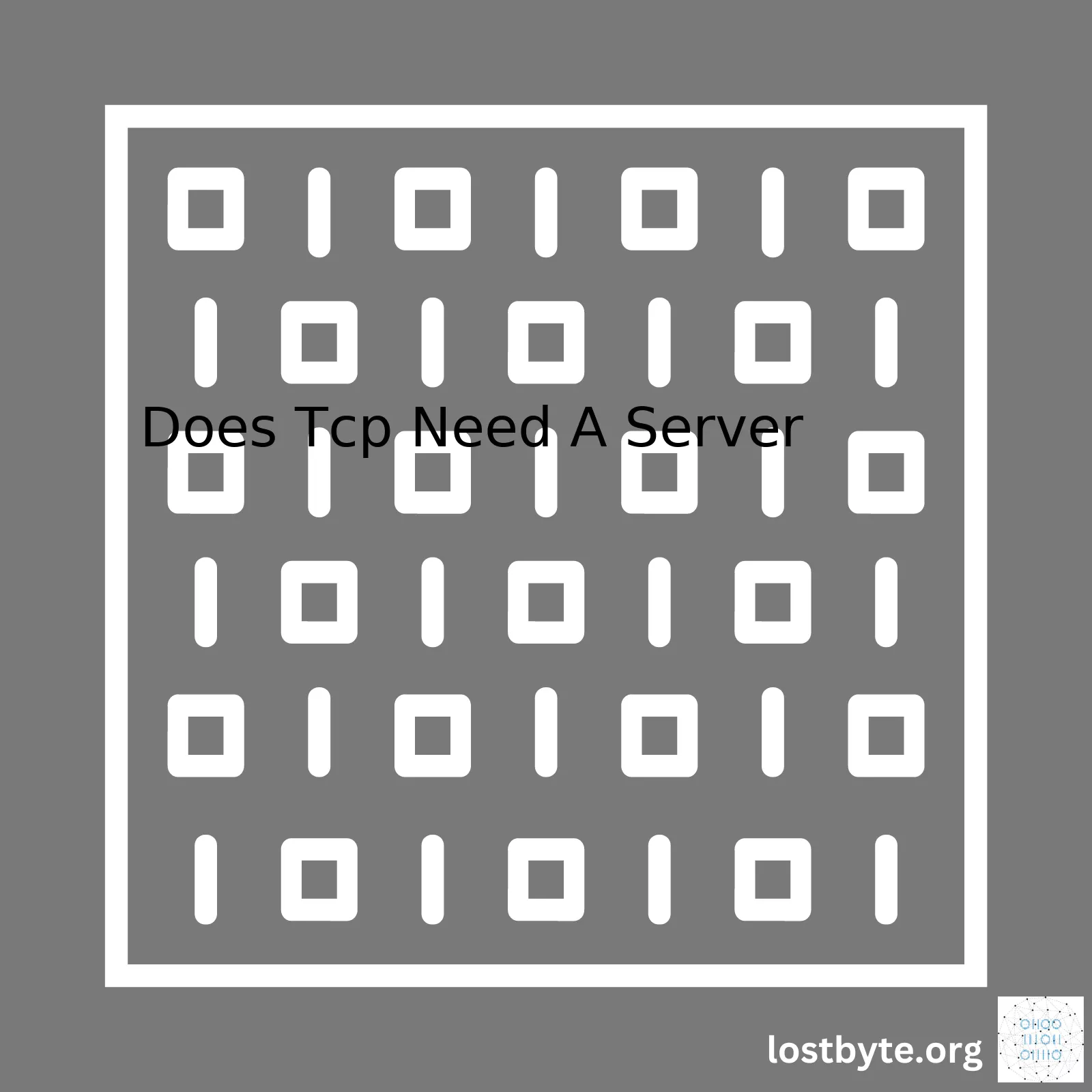
TCP | Requires a Server? |
---|---|
Yes | A TCP protocol operates based on a client-server model where a server is indeed required. |
No | However, it’s important to note that ‘server’ and ‘client’ in this context do not necessarily represent physical machines. These terms are more about the roles these entities play during data transmission. |
The Transmission Control Protocol (TCP) is one of the main protocols in the Internet protocol suite. It is particularly known for providing reliable, ordered, and error-checked delivery of a stream of octets between applications running on hosts communicating over an IP network.[1] The concept of server and client is present here, but it doesn’t necessarily mean two separate machines or devices. Instead, it refers to the roles that these two entities play.
A machine could be a server at one point and become a client at another depending on which application is doing the interaction. To establish a TCP connection, one side must listen on a specified port for an incoming connection while the other initiates the connection. In this scenario, the system listening for connections is often referred to as the ‘server’, while the one initiating the connection is the ‘client’.
Here’s a simple example in Python to illustrate the code behind it:
#Server Code
import socket
s = socket.socket()
print("Socket successfully created")
port = 12345
s.bind(('', port))
print("Socket binded to %s" %(port))
s.listen(5)
print("Socket is listening")
while True:
c, addr = s.accept()
print('Got connection from', addr)
c.send('Thank you for connecting')
c.close()
#Client Code
import socket
s = socket.socket()
port = 12345
s.connect(('127.0.0.1', port))
print(s.recv(1024))
s.close()
The first part represents the server, listening for connections at port 12345. Once a connection is established, it sends a thank you message to the client for connecting. The second part is the client, attempting to connect to the server on local host at the same port number; once the connection is established, it receives the server’s welcoming message and prints it out.
One of the powerful aspects of modern Internet communication is the use of protocols like TCP (Transmission Control Protocol), which establishes a reliable, ordered, and error-checked delivery of a stream of packets on the internet. Without these kinds of protocols, we would experience lost data, slow connections, and disorderly messaging.(source).
When looking at the question “Does TCP need a server?” the answer fundamentally hinges on our understanding of how TCP works.
TCP follows a client-server model for communication, but this doesn’t mean it ‘requires’ a server in the conventional sense. What it needs are at least two endpoints: one to send data and another to receive that data. We often refer to these as the client and the server, but in truth, both are more or less equals in the protocol’s design, and their roles can be reversed as well.
The Basics of TCP
TCP is connection-oriented, meaning it needs to establish a connection before transmitting data. This process begins with a three-way handshake:
xxxxxxxxxx
1. SYN: Client sends a synchronize packet to the server.
2. SYN-ACK: Server responds with synchronize acknowledgment.
3. ACK: Client replies back to acknowledge.
This handshake ensures that both client and server are ready to transmit and receive data and have agreed upon the sequence numbers that will be used to correctly order the packets, enhancing reliability.
Importance of TCP
TCP, being connection-oriented and having features like error-correction and congestion control mechanisms, works best in scenarios where reliability is paramount:
xxxxxxxxxx
• File transfers
• Email communications
• Secure shell connections (SSH)
If you’re streaming video or gaming online and real-time data is more important than getting every single bit of data right, then something like UDP may be more appropriate(source).
TCP | UDP |
---|---|
Connection-oriented | Connection-less |
Error-checking | No Error-checking |
Sequencing of data | No sequencing of data |
Congestion control | No Congestion control |
Acknowledgement | No Acknowledgement |
In conclusion, whether TCP requires a server or not depends on your perspective. What it necessarily demands is two endpoints for proper communication: One to send and one to receive data. These are conventionally referred to as the client and server within TCP, even though their roles could potentially interchange over time if necessary.Many often struggle to figure out whether TCP (Transmission Control Protocol) or UDP (User Datagram Protocol) is a better fit for an application’s needs. So, let’s dig deep and differentiate these two network protocols, but with a primary focus on one question: Does TCP need a server?
TCP | UDP |
---|---|
TCP necessitates the establishment of a connection before data can be sent. This connection is initiated by what we refer to as the “client,”with the recipient end being referred to as the “server.” It’s important to remember that the terms client and server don’t necessarily relate to different physical machines, but rather to distinct roles within the exchange. The server is essentially the listening end of the conversation and it waits for incoming connections. | On the other hand, UDP doesn’t require a server since it operates without setting up a connection. Instead, it sends data in discrete packets, known as datagrams, across a network. With this protocol, there’s no concept of client or server, just communicating endpoints. |
Other Key Differences
While TCP requires a dedicated server, and UDP does not, there are other key differences between these two protocols as well:
Data Transfer: With TCP, you’re assured that your data will reach its destination in the exact order it was sent since the protocol arranges data packets in sequence. Conversely, UDP offers no such guarantee, as data packets can be received in any order.
Reliability: In ensuring reliable delivery, TCP establishes a firm handshake connection, which includes mechanisms for acknowledging received packets, checking their order, and retransmitting lost ones. Conversely, UDP operates on a connectionless communication model with no handshake dialogue, meaning it provides a less reliable service where packet delivery isn’t guaranteed.
Speed: As a connection-oriented protocol reliant on acknowledgments for data transmission, TCP is slower compared to UDP, which does not wait for acknowledgments and hence can transmit data faster.
Error Checking: Both TCP and UDP perform error checking. However, only TCP provides error recovery – if any packet is found to be lost during transmission, the receiver automatically requests the sender to resend it. UDP performs error checking but simply discards any packet that arrives damaged without requesting a retransmission.
Flow Control: With TCP, flow control ensures that a sender doesn’t overwhelm a receiver by transmitting more data than it can handle. UDP lacks this feature.
xxxxxxxxxx
11 1TCP Server Code Example
xxxxxxxxxx
TCP Server Code Example
A Python implementation of a simple TCP server might look something like this:
xxxxxxxxxx
import socket
s = socket.socket()
print("Socket successfully created")
port = 12345
s.bind(('', port))
print("socket binded to %s" %(port))
s.listen(5)
print("socket is listening")
while True:
c, addr = s.accept()
print('Got connection from', addr)
c.send('Thank you for connecting')
c.close()
In conclusion, whether TCP (which requires a server) or UDP (which does not) better serves the goals of your application largely depends on the specific needs of that application. For data that must arrive in a precise order and completeness, TCP would be the favorable choice despite its slightly slower speed. However, when speed is paramount and a bit of loss is acceptable – such as in real-time media streaming or gaming – UDP may be the preferred option.
Sources:
– Mozilla Developer Network (MDN)
– Mozilla Developer Network (MDN)
– Python Socket LibraryCertainly, I’d be glad to delve into the topic of how TCP (Transmission Control Protocol) operates in regards to server connectivity. A key question you may have is “Does TCP need a server?” and the short answer is – Yes. Nonetheless, matters demand a more thorough exploration for solid understanding.
TCP falls under the category networking protocols used to facilitate data transmission across networks like the internet. Primarily, TCP is founded on the client-server architecture model as it’s built around the idea of making requests from client systems and having responses sent over from servers. However, do note that because TCP itself is not directly in charge of the connection establishment, as per the OSI model, TCP is applicable to both client-based and server-based operations. Here is a schematic representation of the roles different layers in the OSI model play:
Layer | Responsibility |
---|---|
Application | Software application interface with network services. |
Presentation | Formats data to present to the application layer. |
Session | Inter-host communication. |
Transport | TCP falls into this. Handles error recognition and recovery. |
Network | Routes packets across network nodes. |
Data Link | Communication directly between network nodes. |
Physical | Physical media for transmitting data. |
To illustrate this, let’s suppose we are building a simple HTTP Server using Node.js. The server here will listen for any HTTP request from clients, process it, and give an HTTP response back.
xxxxxxxxxx
const http = require('http');
const server = http.createServer((req, res) => {
// Process Request
// Send Response
});
server.listen(port, hostname, () => {
console.log(`Server running at http://${hostname}:${port}/`);
});
In such a case, our Node.js application is acting as the server, while the TCP does the heavy lifting behind the scenes, segmenting our data, ensuring reliable delivery, reassembling it at the other end, etc.
What happens when there isn’t a server? Well, without a server, the client can send a TCP request but, no one would be available to acknowledge the request or respond to it. This means there will be no exchange of data or information. Client-server based models, p2p models, these all rely on TCP for communicating via the internet but at the core, there must be something that accepts the request for the protocol to work effectively.
However, in things like peer-to-peer networking (P2P), every node can act as both a client and a server. Many modern protocols like BitTorrent utilize such functionality. Still, even in peer-to-peer network settings, the ‘server’ role still exists – the difference is just that each participating node takes turns being a ‘client’ and a ‘server’, depending on whether they’re receiving or sending information.
Finally, remember, most times you use your smartphone or computer, you’re in effect a client requesting information from a server through TCP. Servers provide the resources necessary to fill those requests, making the world wide web as we know it possible.
Hence, in an in-depth consideration of TCP, the existence of a serving entity to accept and process these requests while giving appropriate responses is a fundamental requirement by design.
The Transmission Control Protocol (TCP) is a communication protocol that enables data transfer and communication over networks, including the internet. The TCP protocol operates in a client-server model. This means that in TCP, one host or machine is typically a client, which initiates communication, and another is a server that waits to receive communication from clients.
Does TCP Need a Server?
The simple answer is “Yes”. Here’s why:
Data Flow Management
- TCP uses the concept of a server for managing the flow of data. The server listens for incoming connection requests from various clients and manages these connections, ensuring smooth, ordered, and error-check free communication between the devices.
- RFC 793, which outlines the specifications for TCP, defines operations such as establishing, maintaining, and terminating connections. These processes are largely orchestrated by the server.
Reliable Communication
- In the context of TCP, ‘reliability’ refers to the packet delivery guarantee that ensures that all packets arrive at their intended destination, intact and in order. The server helps ensure this reliability by managing retransmission of lost packets, checking sequence numbers to maintain order, and using acknowledgments to confirm receipt of packets.
- For example, if a client sends a message to the server and the server does not acknowledge receipt within a timeout period, the client will resend the message.
Multitasking
- A single server can handle multiple TCP connections simultaneously. The server assigns a unique socket (combination of the IP address and port number) to each connection, allowing concurrent communication with multiple clients. In other words, servers promote multitasking in TCP.
Here is a basic Python example where a server is set up with the socket library to listen to incoming TCP communications:
xxxxxxxxxx
import socket
s = socket.socket()
host = socket.gethostname()
port = 12345
s.bind((host, port))
s.listen(5)
while True:
c, addr = s.accept()
print ('Client connected', addr)
c.send('Server message: Connection acknowledged.')
c.close()
This server code sets up a socket on the host machine on port 12345 and starts listening for incoming connections. When a client connects, it sends an acknowledgment message.
While it’s technically possible to establish TCP-like communications without a dedicated server (as in peer-to-peer connections), the role of a server offers significant advantages in managing traffic, ensuring reliable communication, and supporting multitasking in a professional environment.In the realm of software architecture, we have two broad categories: traditional TCP interaction-based architecture and serverless architecture. As a coder, understanding the difference between these two architectural styles is crucial, especially considering your question – Does TCP need a server?
Traditional TCP Interaction
TCP (Transmission Control Protocol) interaction-based architecture forms part of the foundation of any client-server model network structure. The TCP protocol’s main aim is to provide reliable, ordered, and error-checked delivery of data between applications running on hosts communicating via an IP network.
Here is how TCP operates in a nutshell:
A sender establishes a connection with the recipient using a SYN (synchronization) packet. Afterward, the recipient replies with a SYN-ACK (acknowledgement), then finally, the sender sends back an ACK. This is referred to as the three-way handshake of TCP, which sets up a reliable connection.
The Client-Server Model
The client-server interaction works essentially like this:
- A client sends a request over the internet through TCP/IP to a server.
- The server processes this request and formulates a response.
- Finally, the server sends the response back over the internet through TCP/IP to the client machine.
This type of interchange can’t happen without a server; hence, TCP does require a server for a successful transmission of data packets.
However, it’s important to note that this doesn’t mean that there must be a separate physical machine acting as a server. In the context of software, any machine providing a service to another machine (client), be it a personal laptop or a smartphone, can be referred to as a server.
Typical example:
xxxxxxxxxx
server_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
server_socket.bind(SERVER_ADDRESS)
server_socket.listen(CLIENTS_NUM)
...
Serverless Architecture
On the other hand, we’ve witnessed the rise of serverless computing, which changes the whole dynamics. Don’t let the term ‘serverless’ confuse you – it doesn’t mean that there are no servers involved. Instead, it merely means that developers no longer have to worry about server management.
The key feature of serverless architecture is its event-responsive nature. Functions are launched by specific actions or events, run, and are then de-provisioned automatically.
Typically, third-party services, often known as Backend as a Service (BaaS), handle database operations, cloud storage, and user authentication. Furthermore, Function as a Service (FaaS) platforms run the code snippets, taking care of all operational aspects.
- User interactions trigger Functions as a Service, which process the requests and return responses.
- No dedicated server constantly waits for requests and responses making time and resource usage highly efficient.
Popular examples include Amazon AWS Lambda and Google Cloud Functions.
Example of Google Cloud function:
xxxxxxxxxx
exports.helloWorld = (req, res) => {
let message = req.query.message || req.body.message || 'Hello World!';
res.status(200).send(message);
};
In conclusion, while TCP certainly needs a server to facilitate the interaction in a traditional setup, serverless architecture relieves the coder from explicit server handling and maintenance activities. However, it doesn’t nullify the presence of a server; instead, it abstracts server management away, meaning you don’t deal directly with a physical server or even an always-on server process.
For further reading, check out these resources:
Microsoft Azure’s coverage on Serverless Computing, and a deeper dive into TCP/IP model at Wikipedia’s Internet Protocol Suite page.
Remember, architecture choice depends entirely on the use-case scenario, so evaluate well to find what suits your project best.
Implementing a Transmission Control Protocol (TCP) protocol-based server can be quite challenging due to various factors. These issues relate to the complexities associated with handling TCP state management, dealing with latency in connections caused by network inefficiencies, properly handling errors and packet loss, and managing client load through multi-threading. This ties respectively into whether TCP needs a server.
First and foremost, understanding the state management in TCP is crucial. TCP has a complex life-cycle which includes the establishment of connections (three-way handshake), maintaining a connection for communication and terminating connections (four-segment closing). Each stage comes with its technical quirks that could affect smooth traffic flow. In RFC 793, a standard for TCP issued by The Internet Engineering Task Force (IETF), you can see that navigating different states such as SYN-SENT, SYN-RECEIVED, ESTABLISHED, etc., are described. This complexity needs to be properly handled on both ends: the server side and client side.
xxxxxxxxxx
// A basic server listening for TCP connections will involve following:
import java.net.ServerSocket;
import java.net.Socket;
class Server {
public static void main(String[] args) throws IOException {
ServerSocket serverSocket = new ServerSocket(9000);
System.out.println("Server has started on port 9000.\nWaiting for a client ...");
Socket socket = serverSocket.accept();
System.out.println("Client accepted.");
// your appropriate code to exchange data.
// Closing connection
socket.close();
System.out.println("Connection closed");
}
}
Latency is another issue that often comes up with TCP. With TCP, all packets must be acknowledged by the receiver before the sender continues to send more data. This results in a slower transmission speed, especially when there are network delays. Of course, this sort of reliability is one of the reasons TCP might be chosen over something like UDP, but it means accepting higher latency which could significantly hamper user experience particularly in applications requiring real time communication.
Error Handling | In a TCP server implementation, developers need to handle any occurrence of packet loss or delay appropriately. TCP’s inbuilt error recovery mechanism ensures reliable communication, however, repeated re-transmissions due to high packet loss impact the performance of applications using TCP. |
Load handling | Another challenge is managing multiple simultaneous clients. A server should be equipped to handle multiple clients at the same time. To solve this, usually, each client is assigned a separate thread. The number of threads a server can efficiently manage is limited and it require exquisite tuning for efficient resource usage. |
The interoperability of TCP within distributed systems likely implies a server-client model – whether TCP specifically ‘needs’ a server is open to interpretation. It’s worth noting that both sides of a TCP connection have equivalent capabilities, and therefore there isn’t necessarily a designated ‘server’ or ‘client’. Nonetheless, traditionally and practically, embodiment of services (accessible via TCP requests) reside within a entity typically considered a ‘server’. Hence, for practical and functional means, a TCP-based interaction does conventionally imply server involvement.
Furthermore, many standard protocols built on TCP such as HTTP, FTP, SMTP, operate in a request-response paradigm, mapping directly onto conventional ‘server-client’ models. For example, in an HTTP Protocol (built over TCP ), a client (browser) sends a request and waits for a response from the server.
xxxxxxxxxx
//Sample HTTP GET Request sent over TCP
GET /index.html HTTP/1.1
Host: www.example.com
Therefore, while TCP itself might not technically ‘need’ a server, functionally and practically, majority of its use-cases anticipate server-side presence for operational manifestation.
Theoretically, yes! The concept of ‘TCP without a Server’ can exist. In certain types of peer-to-peer communication schemes or systems utilizing Datagram Transport Layer Security (DTLS), it could be conceived that there isn’t a traditional server acting as the gatekeeper and organizer of information flow. However, in such architectures, peers still perform duties which can be akin to those performed by servers. TCP, which stands for Transmission Control Protocol, fundamentally is a set of rules defining how devices connect and exchange data on a network.
However, keeping our discussion relevant to the question ‘Does TCP Need A Server?’, it’s crucial to understand that when we typically engage in a dialogue about TCP, we’re expecting an interaction based on the client-server model. TCP, being a connection-oriented protocol, generally operates under a scheme where one party (the server) awaits connections from other parties (clients).
Under this model:
- A server is required to initialize and uphold listen sockets and wait for any incoming connection requests
- Clients are responsible for initiating outbound connections towards these servers
- Servers manage multiple clients simultaneously by establishing dedicated sockets for each one
One excellent real-world application example of this is when you access a website through a browser; your machine (the client) establishes a connection with the website’s server and requests data.
xxxxxxxxxx
// Python code illustrating the creation of a simple TCP server
import socket
sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
server_address = ('localhost', 12345)
print('starting up on %s port %s' % server_address)
sock.bind(server_address)
sock.listen(1)
while True:
print('waiting for a connection')
connection, client_address = sock.accept()
try:
print('connection from', client_address)
while True:
data = connection.recv(16)
print('received "%s"' % data)
finally:
connection.close()
While in many instances, TCP involves servers and clients, it’s essential not to mistake this as “TCP needs a server.” Instead, consider it as TCP often employs a server due to its usage pattern revolving around the client-server model.
For more insightful details about this subject matter, a valuable resource here offers a literature review encompasses precisely this topic including historical developments, advantages and disadvantages along with application scope of TCP protocol.
In sum, no law dictates TCP can’t be used outside of the context of server-based systems. However, given its design and benefits, it is invariably used in scenarios where persistent connections and reliable data delivery are prioritized, features solidified by embracing the client-server architecture.TCP (Transmission Control Protocol) is a connection-oriented protocol typically used over the Internet that guarantees data delivery in the correct order and promotes robust connectivity. In conventional scenarios, the TCP two-way communication implies a client-server model where one entity behaves as a server waiting for incoming connections while another acts as a client initiating these connections.
Does TCP Need A Server?
In response to this query, it’s essential to understand that for TCP-based services, a server in the context of socket programming doesn’t imply a large-scale machine that processes requests from multiple users. Rather, a ‘server’ refers to the role an endpoint plays in a connection [^Reference^]. So yes, TCP requires a passive endpoint or a ‘server’, but it’s not necessarily the conventional high-capacity server we often visualize.
Considering this aspect, let’s delve into three practical usage scenarios where TC/IP applies without a common physical server.
Peer-to-Peer (P2P) Communication:
Consider peer-to-peer networks where nodes (‘peers’) are both consumers and suppliers of resources. Here, any node can act as a server at any particular moment. As an example, BitTorrent uses the TCP transmission for reliable file sharing in P2P networks.
xxxxxxxxxx
// Sample Socket Programming
// one peer acting like server accepting connections
int sockfd = socket(AF_INET, SOCK_STREAM , 0);
struct sockaddr_in serv_addr;
serv_addr.sin_family = AF_INET;
server_addr.sin_port = htons(port);
bind(sockfd, (struct sockaddr *)&servaddr , sizeof(serv_addr));
listen(sockfd,3);
// another peer behaving like a client initiating a connection
int sockfd = socket(AF_INET, SOCK_STREAM , 0);
struct sockaddr_in serv_addr;
serv_addr.sin_family = AF_INET;
serv_addr.sin_port = htons(PORT);
connect(sockfd, (struct sockaddr *)&serv_addr , sizeof(serv_addr));
Online Multiplayer Gaming:
Multiplayer gaming applications empower players to host games on their personal computers. Other participants connect to the game using the host’s IP address. This interaction could employ the TCP protocol for reliable data exchange between these gaming clients.
Virtual Private Networks (VPN):
VPNs usually implement the client-server model on a protocol level with the VPN software as the server, regardless of whether the VPN service runs on large-scale servers or consumer-grade hardware. Notably, OpenVPN, a popular VPN solution, uses TCP among its transport layer alternatives[^OpenVPN^].
xxxxxxxxxx
// Basic configuration
dev tun
proto tcp-server //Work as a server when underlying network protocol is TCP
ifconfig 192.168.1.1 192.168.1.2
secret static.key
In each case, it’s clear that although TCP fundamentally requires the client-server model, this doesn’t enforce a specific hardware configuration requiring a powerful server machine. It’s entirely feasible, as demonstrated by the examples, to initiate a ‘server’ process on commonplace machines or even handheld devices. Thus, while the TCP protocol always needs a server listening for incoming connections, this server could be any device programmed to accept such connections.
The TCP (Transmission Control Protocol) is a set of rules that standardizes how systems communicate via the internet by creating data packets. It includes a system for ensuring that these packets reach their intended destination, but this methodology is server-based in structure. Traditionally, TCP has required a server to facilitate communication channels between computers in a network. However, recent advancements in technology might be paving the way for an override on the need for a TCP server, while still maintaining its relevance. This ambivalence arises primarily from two trends:
- Peer-to-Peer Networking (P2P)
- The development of serverless architectures
Peer-to-Peer Networking (P2P)
In a Peer-to-Peer (P2P) network, multiple peer nodes connect directly with one another without the need for a centralized server to mediate the exchange of data. They remain within the TCP/IP model but can interact directly, effectively sidestepping the necessity of a server’s role in traditional TCP communication. Examples of P2P networks include BitTorrent and certain online blockchain platforms like Bitcoin.
xxxxxxxxxx
/**
* A basic P2P connection example
* @param {string} address - IP address to establish connection
*/
public void ConnectToPeer(string address)
{
TcpClient client = new TcpClient(address, port);
NetworkStream stream = client.GetStream();
// send/receive data to/from other peers
}
Serverless Architectures
The advent of serverless architecture is another advancement that challenges the traditional requirement of a server in TCP. Serverless does not mean there’s no server involved; rather, it denotes a cloud-based execution model where the cloud provider dynamically manages resource allocation. Technically serverless application might still use TCP protocol, as they utilize HTTP for requests for which under the hood relies on TCP or any transport layer compatible with HTTP. IBM: What is serverless?
Important to note, these technologies do not make TCP obsolete; they merely modify how we perceive and utilize servers in the realm of TCP.
The fundamentals of TCP – namely its reliability, ordered data delivery, error-checking mechanisms, and congestion control – are integral aspects that are retained regardless of the technological shift towards P2P or serverless paradigms. This means, despite the evolving landscape of network architecture, TCP maintains its relevance by providing crucial functionalities for communication over the internet.
The traditional TCP-server system is one that has endured countless adjustments and upgrades over the years, but it isn’t devoid of limitations. It necessitates the existence of a physical server, which can pose reliability issues and increase operational costs. Hence, it’s worth exploring state-of-the-art alternatives to this arrangement.
One technological advancement addressing these needs includes the adoption of Peer-to-Peer (P2P) networks. P2P ensures data transmission without requiring a central server. If your question pertains to whether TCP needs a server in the traditional sense, the answer lies within P2P technology.
Below are brief descriptions of how P2P networks circumvent the need for traditional servers:
• P2P Networks: Nodes or peers in these networks function as both clients and servers to other nodes in the network. Subsequently, users can download and provide resources concurrently. This drastically makes huge volumes of data transfer more manageable, contrary to the limitations posed by traditional TCP/IP servers.
xxxxxxxxxx
//P2P connection, Node A wants to connect to Node B
Node A.find(B);
Node A.connect(B);
• Distributed Hash Table (DHT): DHTs offer a lookup service similar to a hash table; key-value pairs are stored, and any participating node can retrieve the value associated with a given key. BitTorrent’s protocol features a common example of DHT.
xxxxxxxxxx
//Snippet related to DHT implementation.
dht.put("key", "value");
String value = dht.get("key");
As seen from these examples, contemporarily there exist intriguing techniques whereby a traditional client-server model may not be necessary at all. They also prove that TCP can function efficiently without needing a ‘server’ as traditionally conceived.
Nonetheless, it’s helpful to keep in mind that even these innovative methodologies have their set of drawbacks such as:
• Security Concerns: With no centralized control, security is highly dependent on individual nodes. This poses new vulnerabilities as each peer’s integrity is crucial to the overall health of the network.
• Network Size and Scalability: Larger networks impose challenges regarding search efficiency and overall network throughput.
While these newer concepts represent promising alternatives to the traditional TCP-server system, they still need careful consideration based on use-cases and requirements. Each organization must analyze their specific objectives against these pros and cons before deciding on switching or upgrading their existing systems.
TCP, which is an acronym for Transmission Control Protocol, operates in the transport layer of the TCP/IP model. It’s notable for being one of the main building blocks of the Internet by providing reliable streams of data between servers and clients.
Term | Definition |
---|---|
TCP | Transmission Control Protocol, a communication protocol that establishes a connection before transferring data |
Server | A piece of computer hardware or software that provides functionality for other programs or devices, termed ‘clients’ |
The question at hand, as to whether TCP requires a server, is not entirely straightforward. Both ‘yes’ and ‘no’ can be justified depending on the specific context. Let’s dive deeper into understanding this.
In a traditional TCP architecture, there are two roles: the server and the client(s). The server listens for incoming communications (through what is known as a socket), and the client initiates the communication. Here’s a basic representation of a TCP connection:
xxxxxxxxxx
Client --- SYN ---> Server
Server --- SYN/ACK--- > Client
Client --- ACK ---> Server
In this fundamental architecture, you need both a server and a client. Hence, from this perspective, TCP indeed needs a server.
However, it’s worth mentioning that the particular roles of ‘client’ and ‘server’ are less about the nature of TCP itself, and more about the way we use it. Essentially, these roles refer to ‘initiator’ and ‘listener’. TCP doesn’t inherently require a dedicated server machine or even a permanent server process. Any TCP-compatible device can behave as a client or a server based on what role it’s performing.
To illustrate, consider peer-to-peer (P2P) networks, where typical client-server distinctions break down. Nodes in P2P networks can switch roles dynamically – sometimes behaving as servers, sometimes as clients. For example, during a file transfer between two peers using TCP, the individual sending the file (the ‘server’, for that operation) switches to being a ‘client’ when it’s time to receive a file.
We witness such dynamic roles in modern distributed systems, web technologies like WebRTC, and various applications of Internet of Things (IoT).
All in all, while a server-role is central to how TCP typically functions, the protocol itself doesn’t rigidly enforce any rules over which actor must take up this role. Whether TCP needs a server relies significantly on your interpretation of what a ‘server’ signifies.
For further exploration of TCP and related networking concepts, check out “Computer Networking: A Top-Down Approach” (source) and additional online resources such as those provided by Cisco (source).