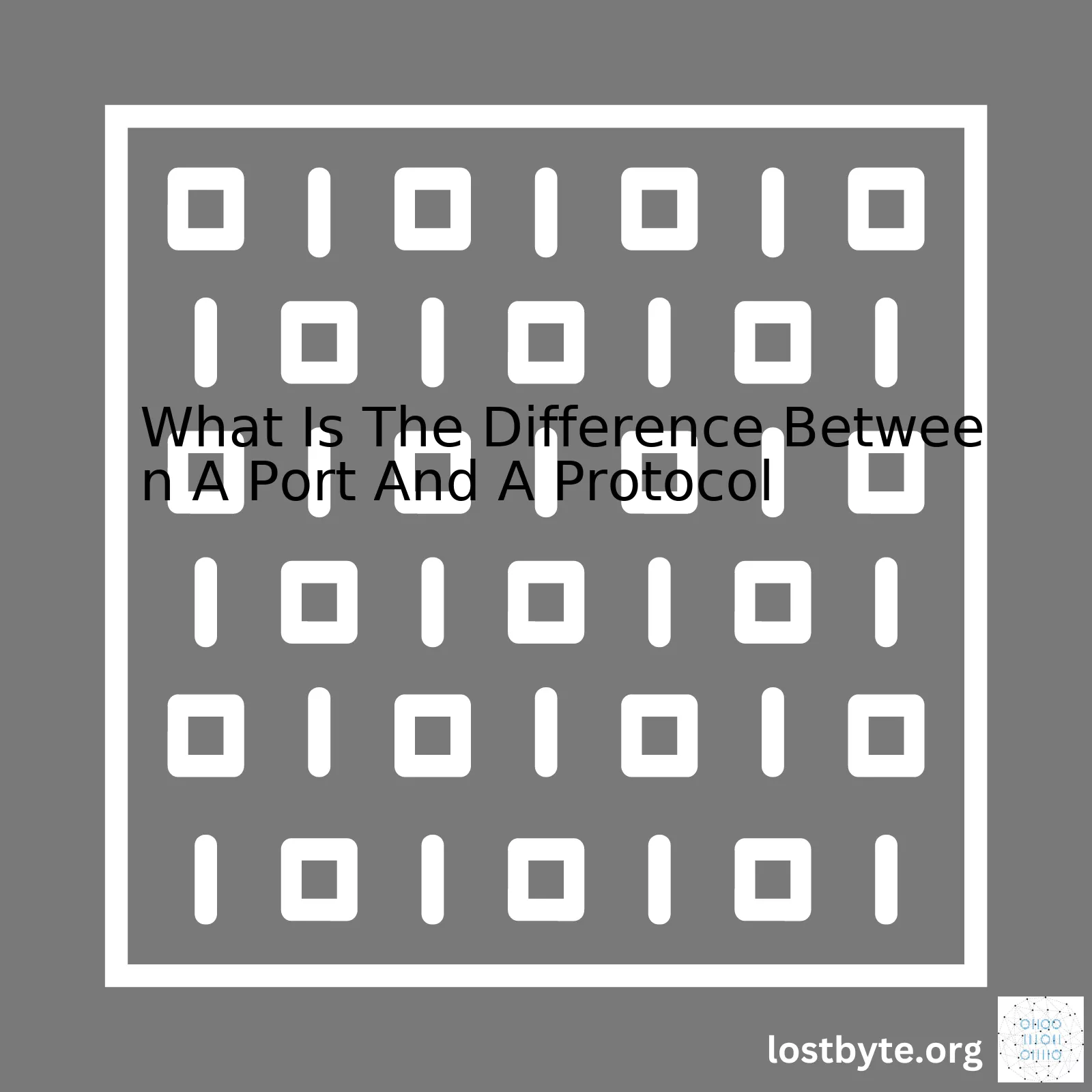
Attribute | Port | Protocol |
---|---|---|
Definition | A port is a numerical label used to identify specific processes or services in a host within a network. | A protocol is a set of rules or common language that computers use to communicate over a network. |
Example | HTTP uses Port 80, HTTPS uses Port 443 | HTTP, HTTPS, FTP, SMTP are all examples of protocols |
Purpose | Ports allow different processes running on the same host to share a single physical network connection. | Protocols ensure uniformity in how data is formatted, transmitted, received and acknowledged on a network. |
With relevance to network communication, both ports and protocols play significant roles but are fundamentally different. Ports can be visualized as doorways into computers for specific types of internet traffic. They serve a purpose in network programming; by specifying the port number, your data packets know where to go among many different services a server may provide.
On the other hand, a protocol can be thought of as the ‘language’ spoken between computers to facilitate communication. It’s like setting rules for how data sent and received should be interpreted and responded to. For instance, HTTP (Hypertext Transfer Protocol) defines how messages are formatted and transmitted over web applications.
In code, you might make a simple HTTP request like this:
fetch('http://example.com', { method: 'GET', })
The URL points to ‘example.com’, with the protocol being ‘HTTP’. The port isn’t defined so it defaults to port 80 – the standard for HTTP traffic. This will send a GET request to example.com following the rules defined by the HTTP protocol through port 80.Sure, let’s get into what the difference between a port and a protocol is.
Understanding Ports
A port can be defined as an endpoint in communication within an operating system. When an application wants to send or receive traffic, it has to use a numbered port between 1 and 65535. This number serves like a courier department inside the operations of a network. Here are some features of ports:
- Type: There are two types of ports: TCP (Transmission Control Protocol) and UDP (User Datagram Protocol). TCP generally supports more robust, connection-oriented protocols, while UDP supports simpler, connectionless communications.
- Well-known ports: These range from 0 to 1023 and are assigned by Internet Assigned Numbers Authority (IANA). Well-known ports are used by system processes that provide widely used types of network services (for example, HTTP uses port 80).
- Registered ports: These range from 1024 to 49151 and are registered with IANA to prevent duplication and misuse.
Here’s a simple bit of code to open a TCP server socket on port 8080:
import java.net.ServerSocket; public class Main { public static void main(String[] args) throws IOException { ServerSocket server = new ServerSocket(8080); System.out.println("Listening on port 8080..."); } }
Understanding Protocols
On the other hand, a protocol sets up the rules for how data is transmitted over a network. It defines things like the structure of the data messages, error handling process, message integrity and security. Some common ones include HTTP (Hypertext Transfer Protocol), FTP (File Transfer Protocol), and TCP/IP (Transmission Control Protocol/Internet Protocol).
Let’s take HTTP for instance:
- HTTP: The HTTP protocol directs how your browser communicates with web servers when you enter a URL. It structures the request and response messages between client and server.
- TCP/IP: TCP/IP is vast and includes many subprotocols, like ICMP and IGMP. Overall, TCP/IP stipulates how data should be packaged, addressed, transmitted, routed and received at the destination.
Here is how we add protocol to our previous Java code:
import java.net.*; public class Main { public static void main(String args[]) throws Exception { TcpServer tcpServer = new TcpServer(); tcpServer.start(); } } class TcpServer extends Thread { String line=null; BufferedReader fromClient = null; Socket clientSocket=null; InetAddress localhost=InetAddress.getLocalHost(); int port=8080; public void run(){ while(true){ try{ System.out.println("TCP Server waiting for client @ IP Address : "+localhost+": & Port :"+port); //After server starts,client can connect to above address to send data }catch(IOException e) { System.out.println("IOException TCP Server"); } } } }
So essentially, the main difference between a port and a protocol is their function within a network operation. A port acts as a gateway through which data enters or leaves, whereas a protocol acts as the rulebook that determines how that data is packaged, sent, received, and interpreted.
It’s important to grasp this difference as they constitute fundamental understanding behind all networking operations. By mastering these concepts, developers can become more competent in creating network applications and troubleshooting network issues.
Firstly, let’s delve into the definition of a port and a protocol in the context of networking. A communication port can be viewed as an endpoint or an interface through which data is sent or received over a network. In terms of software, it’s a specific process identified by its unique port number on an IP address. Data transferred over the internet is managed through protocols, which determine how data is sent and handled, essentially serving as the rules that govern data communication.
In a nutshell:
- A Port is defined as a logical connection place for transmitting or receiving data within a network.
- A Protocol, on the other hand, are sets of rules ensuring the smooth transmission of data between devices.
The relationship between ports and protocols becomes clearer when you consider real-world examples. For instance, when we browse a website, we’re predominantly using HTTP (Hypertext Transfer Protocol) or HTTPS (HTTP Secure). These protocols use respective port numbers 80 and 443 to exchange data over the network.
<table> <tr> <th>Protocol</th> <th>Port Number</th> </tr> <tr> <td>HTTP</td> <td>80</td> </tr> <tr> <td>HTTPS</td> <td>443</td> </tr> </table>
The above table indicates the respective port numbers allocated per protocol.
An important aspect to highlight here is that every application or service running on a network system has a specific port connected to it. This includes applications like FTP (File Transfer Protocol), Telnet, SSH (Secure Shell), SMTP (Simple Mail Transfer Protocol), and many more. Each operates on a specific port and employs a particular network protocol for data transmission.
For example, Port 21 is dedicated for FTP to handle file transfer operations, while SMTP uses Port 25 for the transfer of email messages. When we talk about these interactions, there are two main types: TCP (Transmission Control Protocol) and UDP (User Datagram Protocol). The distinction lies in their functionality:
- TCP is connection-oriented, meaning it verifies the receipt of any packets sent. It’s usually adopted for applications where delivery is critical such as sending emails, loading webpages, etc.
- UDP is connectionless where delivering speed is preferred over the assurance of packet arrival. It’s often used in live streams or video calls where latency reduction takes precedence over packet verification.
An essential thing to remember is that a protocol guides the way or manner in which data should be transmitted over the network, whereas a port acts as the door or access point which allows this data to reach a specific process/application within your device. Both act in tandem to ensure streamlined and orderly network communications.
To make these notions even easier to digest, think of the port as the designated address where mail should be delivered, while the protocol serves as the mailing procedures employed by the postal services. Without both crucial components, successful data transmission would be nearly impossible to achieve.
Find out more about ports and protocols from MDN Web Docs.
The functioning of the Internet relies heavily on two essential elements: Ports and Protocols.
A Protocol in the context of data transmission, is a set of defined rules or processes which dictate how data is transmitted over a network. Each protocol has its specific roles in facilitating communication between devices over a network, thereby ensuring that data packets are sent and received accurately and effectively. Protocols determine aspects such as the format of data, error handling techniques, and the sequence in which these data packets are sent or received. Examples of protocols include TCP/IP (Transmission Control Protocol/Internet Protocol), HTTP (Hyper Text Transfer Protocol), and FTP (File Transfer Protocol), among others.
On the other hand, a Port is essentially an endpoint or a logical construct that identifies a specific process or a type of network service running on a system. It operates at the Transport layer of the OSI model. Ports act like ‘postboxes’ or ‘doors’ through which information enters and leaves the system. They help the system to sort out multiple incoming connections and distribute them to correct applications.
To clarify with an example, consider sending an email. The SMTP (Simple Mail Transfer Protocol) handles the transmission of the mail. However, it doesn’t decide where the mail goes on the recipient’s computer. This decision is made by the port. Port 25, for instance, is commonly used for SMTP transmission.
Let me illustrate this further. For web services, we usually use the HTTP or HTTPS protocols, operating typically on ports 80 and 443 respectively. If we put it in the form of a URL, it would look something like this:
http://domain-name.com:80
https://domain-name.com:443
In line one above, “HTTP” denotes the protocol, “domain-name.com” is the server location, and “80” after the colon signifies the port number. Usually browsers hide the port number if it’s a standard port (80 for HTTP and 443 for HTTPS).
Now, take note of this table below:
Protocol | Standard Port |
---|---|
FTP | 21 |
SMTP | 25 |
HTTP | 80 |
HTTPS | 443 |
This table illustrates some standard protocols and their corresponding common port numbers.
From here, it is clear how the role of protocols and ports in data transmission is distinct yet interconnected. While protocols define the ‘how’ of data transmission, ports determine the ‘where’ to on the recipient’s system.
For more understanding about protocols and ports, you can follow this IBM article.
Reference codes:
// An example of defining a HTTP server in Node.js listening on Port 3000 const http = require('http'); const server = http.createServer((req, res) => { // ... }); server.listen(3000);
In this Node.js documentation, the ‘http’ module is loaded first, a server is created using the ‘createServer()’ method, and then the server is set to listen to Port 3000. Note that HTTP is the protocol being used here.
Self-explanatory as it might seem, Network Ports and Protocols form the backbone of any computer network. Both these elements, although differentiated by their functions, work in mission-critical roles to ensure seamless communication between devices across a network.
To explore the notion in terms of programming and networking, let’s dive deeper into understanding each term and clearly illustrate the difference between a port and a protocol.
Network Port
A Network Port can be best defined as an endpoint in the process of data transmission across a network. Think of it like a door through which information enters or exits your device. For each service running on your device, they are identified by unique numbers known as Port Numbers.
There are three main types of network ports:
1. TCP (Transmission Control Protocol) ports: Reserved for reliable communications where packets sent must arrive at the destination.
2. UDP (User Datagram Protocol) ports: More suited for services with low time-criticality requirements, allowing for packet loss.
3. SCTP (Stream Control Transmission Protocol) ports: Ideal for transport of PSTN signaling messages over IP Networks.
You’ll often encounter common port numbers like the 80 for HTTP, 443 for HTTPS, and 21 for FTP, among others.
Network Protocol
On the other side of the networking coin comes the Protocols. They establish rules for communications between two devices. Protocols define how data is formatted, compressed, checked for error, or transmitted within the network.
Notable protocols include HTTP (HyperText Transfer Protocol), FTP (File Transfer Protocol), and SMTP (Simple Mail Transfer Protocol), providing different ways to share information across networks depending on the use-case scenario.
Now, how do these tie together?
Imagine sending a letter through traditional mail. The protocol will represent the entire mailing process—the proper handling, stamp affixing, addressing—and the port would be equivalent to the mailbox slot where letters are dropped.
The unique relationship between a protocol and a port allows a specific process running on a server or device to be addressed directly, and for a request or message to be properly handled according to the rules defined by the protocol.
Here’s a simple code snippet illustrating a client-server communication via TCP protocol using sockets in Python:
# Server import socket # Create a TCP/IP socket sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM) # Bind the socket to a specific address and port server_address = ('localhost', 12345) sock.bind(server_address) print('Server is starting up on port {}'.format(server_address)) # Listen for incoming connections sock.listen(1) while True: # Wait for a connection print('Waiting for a connection') connection, client_address = sock.accept() # Client import socket # Create a TCP/IP socket sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM) # Connect the socket to the port where the server is listening server_address = ('localhost', 12345) sock.connect(server_address)
This demonstrates how ‘localhost’ at port ‘12345’, as declared with server_address, forms the endpoint for this communication session over a network. This further emphasizes that within the ambit of internet and network-programming, the harmony of protocols and ports is crucial in ensuring seamless communication and robust system performance.When it comes to network communications, protocols and ports play a crucial role. The differences can be best understood by analogy: picture a mansion with many rooms (ports) for different activities. Now, the rules or manners to be followed in each room represent the protocols.
### Protocols Explained
In computer networks, a protocol defines how data is transmitted and received. It sets the rules for communication so that devices can understand each other. Some common protocols include:
#### HTTP (Hypertext Transfer Protocol)
HTTP
is designed for use in web browsers for communication with web servers. When you type a URL into a browser’s address box and hit Enter, the browser sends an HTTP request to the server hosting the website.
#### HTTPS (Hypertext Transfer Protocol Secure)
Similar to HTTP, but
HTTPS
makes use of encryption via SSL/TLS to secure the data transmission. This prevents unauthorized entities from eavesdropping or tampering with the data.
#### FTP (File Transfer Protocol)
FTP
is used for transferring files between computers on a network.
#### SMTP (Simple Mail Transfer Protocol)
SMTP
is utilized for sending outgoing emails across networks, typically from an email client to an email server.
Here’s a sample implementation of an SMTP protocol used in Python:
import smtplib server = smtplib.SMTP('smtp.gmail.com', 587) server.starttls() server.login("your-email", "password") msg = "Hello!" server.sendmail("your-email", "to-email", msg) server.quit()
### Ports Explained
A port, in contrast, is essentially a virtual docking point where network connections start and end. Each port has a unique number; for instance, HTTP uses port 80 and HTTPS uses port 443. In perspective, TCP/IP protocols associate port numbers 0 through 1023 as well-defined port numbers, dedicated for specific services like FTP (20-21), SSH (22), Telnet (23), SMTP (25), DNS (53), HTTP (80), HTTPS (443) to name a few.
When a service listens on a port, it can receive data from a client application, process it, and communicate back to the initiator.
Consider this scenario – when you want to access a website, your browser (client) creates a connection to the server that hosts the website. During this process, the browser attaches to a particular Port(the ‘room’ we mentioned earlier) following relevant protocol(rules) to successfully fetch and display the website on your browser.
So overall, although ports and protocols differ on their functionalities, they work together to enable data transfer over networks.
For better understanding on general computing terminologies like these, I would highly recommend checking out websites like [TechTarget](https://www.techtarget.com/). Their glossary section is well defined, easy to follow and could prove quite useful in gaining more knowledge.
A Port and a Protocol are both fundamental components of data communication over the internet, but they serve distinct functions. In simple terms, a Protocol governs how data is communicated over networks while a Port is an endpoint through which this data is sent or received.
What is a Port?
A Port is defined as a logical endpoint for processes in a network. Firewalls utilize port numbers to control incoming and outgoing traffic. Each port has a unique identifier, which is a number between 0 and 65535. This number specifies certain services or applications. For instance, HTTP uses port 80, while HTTPS employs port 443.
In Python, you can bind a socket to a specific port using the .bind method:
import socket sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM) # Bind the socket to a specific address and port sock.bind(("localhost", 8000))
For a server to properly setup a connection with a client, it must listen for incoming connections to the specified port. This is achieved by calling .listen() method on the socket.
# Listen for incoming connections sock.listen(5)
What Is a Protocol?
A Protocol in networking refers to the set of rules dictating the-format-and-order-data-is-sent-and-received. Protocols ensure seamless communication between devices over the network. Examples of protocols include HTTP (Hypertext Transfer Protocol), FTP (File Transfer Protocol), and TCP (Transmission Control Protocol).
For instance, if a program uses the HTTP protocol to send a request to “http://example.com” it might look like something like this:
GET / HTTP/1.1 Host: example.com
This code instructs the server to retrieve the main page (“/”) from example.com using HTTP 1.1.
In conclusion, while Ports and Protocols are interdependent — working together to enable communication and data transfer over the internet — they have distinct roles. The Protocol sets the rules for transmission while the Port provides the physical gateway for this information to travel. Understanding the differences between these two concepts can be crucial for network setup and troubleshooting, enhancing the operation and security of your systems.When we discuss network communications within the digital landscape, two concepts inevitably come to the forefront: ports and protocols. These fundamental components of computer networking play vital roles in how data is transferred across networks.
Firstly, let’s delve into what a port is. Essentially, a network port is an endpoint or junction through which data flows back and forth between two computers over a network. To visualize it better, you can think of a port as a dock where boats (data packets) enter and leave.
Ports are assigned unique numbers that help identify them for different services or applications. For example, HTTP usually uses port number 80. Here’s how you might specify a port in Python:
import socket s = socket.socket() s.bind(('localhost', 80))
The
s.bind(('localhost', 80))
implies that your service is listening on port 80, therefore if there’s any communication coming towards this port, it will be processed.
In contrast, network protocols, such as TCP/IP, HTTP, FTP, etc., lay out the rules and standards for how data should be transmitted and received over the internet or a network. They’re akin to languages, defining how information should be packaged (format), sent, received, and deciphered. A protocol determines how to chop up a message into small packets, send them, and reassemble them at their destination.
One example of this is the HTTP or HyperText Transfer Protocol. This protocol is mainly used to exchange data over the internet. In python, if you were to make an HTTP request to a server, you might use the requests library like the following:
import requests response = requests.get("http://www.python.org") print(response.status_code)
Within this scenario, HTTP acts as the protocol, enforcing a standardized rule for data transfer.
To give a more comprehensive depiction of how ports and protocols interact, let’s conceptualize them in the context of sending a letter. After composing a letter (packet), you’ll need to decide on the language (protocol) you’ll be using then you’ll place it in an envelope with the address details – let’s call the “stamped envelope” the segment or datagram. Now, the port number would be the specific mailbox (port) at that given address where you will drop off your envelope.
Even though both ports and protocols function differently, they nonetheless work hand-in-hand to facilitate seamless, orderly network communications. By implementing correct protocols, computers ensure that all the devices in a network “speak the same language”. Likewise, thanks to discreet port assignments, distinct processes and services avoid data collision by not trying to access the same data simultaneously. Specifically, they guide arriving packets of data to the right process or service, ensuring the efficient delivery of numerous streams of data from multiple sources.
For further reading, refer to online resources such as [Lifewire’s guide to Ports] and [Cloudflare’s descriptions of Network Protocols].While it is paramount to build on the concepts of ports and protocols, we should not ignore discussing the pivotal role security plays in the overall narrative. Web-based communication happens via these ports and protocols, with a myriad of cyber threats lurking for vulnerabilities. Hence, implementing robust safety measures to secure both ports and protocol transmissions becomes an absolute necessity.
First, let’s understand the difference between a port and a protocol before delving into their respective security measures.
A Protocol essentially represents a set of rules that govern the way data is transferred over the internet. Protocols determine the methodology for different kinds of network communication. HTTP, FTP, TCP/IP are common examples of such Internet Protocols.
tcpip_protocol=TCP(IP(src="192.168.0.1", dst="192.168.0.2"), dport=8080)
On the other hand, a Port serves as an endpoint in networking. Operating systems utilise ports (identified using unique numbers) to coordinate network connections for specific services or applications. For example, Port 80 is reserved for HTTP, while Port 21 for FTP.
socket = socket.create_connection(("localhost", 21))
Now, understanding the significance of security holistically for both ports and protocol transmissions:
* Port Security: Ports, if left unattended without proper monitoring, can invite unauthorized access, leading to serious data security breaches, encrypted traffic interception, or denial-of-service attacks. Here are some key measures employed to enhance port security:
* Utilising firewalls: Firewalls allow you to block connections coming towards particular port numbers.
* Secure Sockets Layer/ Transport Layer Security (SSL/TLS): These cryptographic protocols ensure secure transmission of data over network ports.
* Port knocking: A stealth method used to externally open ports, helping to avoid exposure to attackers.
* Network Address Translation (NAT): It maps IPs to Ports thus adding an extra layer of ownership and therefore providing higher security levels.
iptables -A INPUT -p tcp --dport 22 -j ACCEPT
* Protocol Security: Different protocols have inherently varied security levels. For instance, HTTP is less secure than HTTPS as the latter uses SSL/TLS encryption. Protocol security prevents actors from tampering with your data during transit. Here are ways one can bolster protocol-level security:
* Adding encryption: Encrypting data before transmission ensures that even if intercepted, the data remains of no use without decryption keys like in HTTPS or IMAPS protocols.
* Using Virtual Private Networks (VPN): A VPN establishes an encrypted pathway often called tunnel between the source & destination shielding protocol transmissions.
* Employing Secure Shell (SSH) Protocol: Better than Telnet, SSH encrypts all transmitted data for secure remote command-line, login, and command execution.
ssh user@hostname
Lastly, regularly auditing networks, implementing rigorous access control, and deploying reliable security software also contribute significantly towards fortifying the wall around your networks against malicious efforts. The pursuit to understand the difference between a port and a protocol should always run parallel to recognising and acknowledging their inherent security facets. Therefore, ensuring security for both ports and protocol transmissions truly upholds the integrity and efficiency of our interconnected digital environment. For more insights, refer to this link on Computer Protocols and Port Security.As a professional coder, it’s crucial to understand how ports and protocols can impact your application’s performance and speed. Here, I’ll explain what both are, how they differ, and how they influence your app’s efficiency.
What is a Port?
A port serves as a communication endpoint for devices on a network. Each port is associated with a specific process or service running on a device. For instance, port 80 is typically used for HTTP connections, while port 25 is often assigned to SMTP for email transmitting. This way, when data packets reach a device, the operating system knows which application should handle them based on the connected port.
What is a Protocol?
A protocol is essentially a set of rules that dictates how data is formatted and transmitted over a network. It defines error handling procedures, authentication methods, and other operational aspects. Common protocols include HTTP, FTP, and TCP/IP.
How Do Ports and Protocols Differ?
Primarily, the difference lies in their functions:
- Protocol: Governs the exchange of data over a network (the ‘how’).
- Port: A location where information is sent and received (the ‘where’).
Impact on Efficiency
Now, let’s delve into how ports and protocols can influence speed and performance:
Impact of Ports:
Ports have a subtle yet significant effect on the performance. Here is how:
- Port availability: If multiple services try to use an already occupied port, there could be connection issues leading to slowdowns or failures.
- Port assignment: Inappropriate port assignments could lead to mix-ups, where incorrect applications receive the data, potentially causing delays or errors.
To illustrate, consider the following source code example:
Socket socket = new Socket("localhost", 8080); // Using the port 8080 OutputStream os = socket.getOutputStream(); PrintWriter pw = new PrintWriter(os, true); pw.println("Hello, World");
If another service tries to access port 8080 while our application is using it, it would cause disruption and performance issues.
Impact of Protocols:
Different protocols have varying impacts on application performance based on their design:
- TCP vs UDP: TCP ensures data delivery but requires more resources, making it slower. However, UDP is faster as it doesn’t ensure delivery or follow any sequence — ideal for real-time services like video streaming.
- HTTP/2: It’s faster than HTTP/1.1 thanks to its multiplexing, binary nature, and server push capabilities.
With protocols, therefore, it’s all about picking the right one for the task at hand for increasing efficiency.
Refer Here for a comprehensive understanding of HTTP protocols.
Thus, correctly harnessing ports and picking apt protocols can significantly optimize your application’s speed and performance.In the modern world of information technology where everything revolves around connections and data exchange, two terms are frequently mentioned: ports and protocols. To understand the evolution of technologies, particularly in network management and internet communications, it’s essential to comprehend what these two profoundly different, yet interconnected concepts entail.
Ports can be imagined as hypothetical docks within your computer or server. When the internet packet ships (data transmitted over a network), arrive they dock at designated ports depending on the type of service required [see e.g. Lifewire]. Ports are unequivocally associated with an IP address of the host and protocol type creating a final destination address for packets.
However, Protocols, on the other hand, are like languages spoken by devices to communicate. Protocols establish rules for data transmission over a network. They stipulate how data is to be packetized, addressed, transmitted, routed, and received. Prominent examples include HTTP, FTP, TCP/IP, among many others.
While both play crucial roles in facilitating seamless communication and interaction over networks, they occupy markedly distinct realms:
Port | Protocol |
---|---|
Identified by port numbers ranging from 0 to 65535 |
Defined by their unique names such as 'HTTP', 'TCP', etc. |
Designated for specific services: e.g., Port 80 for HTTP or Port 21 for FTP traffic |
Defines rules for communication and data transfer across networks |
Allows multiple services to use network resources simultaneously |
Enables error recovery, flow control & encapsulation of data being transferred |
As technologies have evolved, so have the usage protocols and our approach towards ports management. Legacy protocols like FTP are being replaced by SFTP and FTPS due to improved encryption methodologies. IPv4 addressing scheme is being slowly superseded by IPv6 to cater to the ever-expanding roster of internet-enabled devices. An interesting development is the emergence of dynamic and private ports for ephemeral conversations providing additional flexibility in how we manage and allocate resources for communication.
//Old FTPClient ftpClient = new FTPClient("ftp.example.com","username", "password"); ftpClient.connect(); //New FTPSClient ftpsClient = new FTPSClient(); ftpsClient.addProtocolCommandListener(new PrintCommandListener(new PrintWriter(System.out), true)); ftpsClient.connect("ftp.example.com"); ftpsClient.login("username", "password");
Recognize that the way computers and servers interact is constantly changing, and as developers, it’s incumbent upon us to stay informed about these shifts. Staying up-to-date with evolving technologies gives us leverage over potential vulnerabilities, improves the efficiency of our codes, and prepares us better for future innovations. Various online resources and communities, such as Stack Overflow, GitHub, or tech blogs on Medium, can provide ample opportunities to learn from professionals worldwide while staying updated with technological progress.
In the realm of computer networking, two crucial concepts that dictate how data transmission unfolds are ports and protocols. Before we delve into understanding the errors and troubleshooting methods related to these concepts, it is imperative to differentiate between a port and a protocol first.
A protocol in computer networking refers to a standard set of rules that enable computers on the network to communicate. Protocols define procedures and formats that the connected devices follow to perform tasks and deliver data across networks. For instance, protocols like HTTP (Hypertext Transfer Protocol) and FTP (File Transfer Protocol) are designed for specific types of network interaction.
On the other hand, a port is an endpoint in the communication process between applications and operating systems on a network using the TCP/IP protocol suite (Transmission Control Protocol / Internet Protocol). A port acts as a unique identifier for different applications; they help the operating system manage multiple concurrent connections from different applications.
Errors related to ports and protocols can critically disrupt network communication. They can be triggered due to various reasons such as incorrect settings, hardware issues, software bugs, network congestion, or malicious activities like attacks and hacks.
Here is an example situation where you may face a port-related error:
netstat -vanp tcp | findstr 3306
Executing this command on your server aims to check if port 3306 (often used by MySQL databases) is open and listening for connection calls. Suppose no output is returned, this indicates a problem – the MySQL server might not be running or there could be a firewall blocking access to the port.
Troubleshooting steps in this scenario could involve:
• Checking whether the MySQL service is active.
• Inspecting the firewall settings to see if port 3306 is unblocked.
• Looking at the database configurations to confirm if it’s bound to the correct port.
On the other hand, consider a protocol-related issue where a website does not load properly in a browser – a common occurrence when an improper HTTP version is requested by the client or responded with by the server. In such cases, troubleshooting can include measures such as:
• Clearing the browser cache and cookies.
• Trying to load the same website in a different browser to isolate the problem.
• Analyzing server response headers using tools like curl to see if there’s an issue with the HTTP version.
• Reviewing server logs to identify any anomalies.
When facing port and protocol issues, the utilization of specific debugging tools like Wireshark or netstat can be extremely useful. They provide insights into the network traffic, helping pinpoint errors through packet analysis and real-time data monitoring. Further aid can also be sought from online technical communities and forums like Stack Overflow, which harbor a vast plethora of solutions shared by coding professionals across the globe.
Remember – the key to effective troubleshooting is iterative testing. When applying a fix, make sure to test its effect before moving onto the next possible solution. Enjoying this systematic approach can ensure efficient network troubleshooting, and potentially earn you the title of your team’s tech-savvy problem-solver!The distinction between a port and a protocol is crucial when discussing network communications. A port is essentially an endpoint in a network communication, while a protocol is the set of rules that dictate how data is sent and received over a network.
Port | An endpoint in a network communication, which provides a specific process for data transmission with unique identification. |
---|---|
Protocol | A set of rules and standards, providing guidelines for how data packets are moved across a network. |
On one hand, every service or application running on a system will use a specified port number. For instance, common web traffic typically passes through port 80 (HTTP) and 443 (HTTPS). Systems use these ports to organize data and ensure it gets from one point to another successfully. The port numbers are specified within the TCP or UDP packet headers, allowing hosts to correctly route incoming packets to the appropriate application.
On the other hand, protocols are responsible for defining the rules of how the “conversation” should proceed once the connection is established via ports. Examples include HTTP, HTTPS, FTP, SMTP, and others. These protocols determine the structure, compression, error checking methodologies, and many other facets of the data communication.
To give you a code example, consider an HTTP request used in a Python code,
import requests response = requests.get('http://www.example.com')
In this simple Python script, we are using the HTTP protocol to send a GET request to www.example.com. The underlying OS would establish a connection using port 80 (the default port for HTTP) unless otherwise specified.
Understanding the difference and interaction between a port and a protocol is crucial when working on network related tasks in programming, be it web development or designing a network software. It allows you to effectively communicate with different services and have an understanding of the link reliability, data transmission speed and security aspects among others.
Including both port and protocol considerations while designing a networked system can provide a significant advantage for your applications, ensuring efficient, reliable, and secure data transmission.
Making sense of the intricate web of computer networking, deciphering between ports and protocols can sometimes appear to be an overwhelming task. But let’s simplify!
A port in computer networking is a unique identifier for different types of data connections on a computer. An easy way to equate a port would be to think of it as a digital dock where different data packets come ‘docking in’. Here’s an example of what these identifiers look like:
TCP 80 – HTTP TCP 443 – HTTPS
In contrast, a protocol is a set of defined rules that dictate how data is sent and received over the network. Existing in multiple layers of a network, these rules provide directions for both sender and receiver. Common protocols you might come across are HTTP (Hypertext Transfer Protocol) or FTP (File Transfer Protocol).
Therefore, even though ports and protocols belong to the same world of computer networking, they are quite distinct from each other. In essence, a protocol determines the rules of transmission, while a port signifies where specific types of data need to go. It’s a harmonious synergy enabling seamless communication across networks.
To visualize this, consider sending mail through the post.
- The protocol is akin to the set of rules you follow, such as writing the address correctly, stamping, and posting.
- The port is comparable to the recipient’s address you write on the envelope of your mail; it tells the postal service exactly where your mail has to reach.
Implementing structured protocols and clear port identifications is crucial when coding networked applications. Here’s a simple practical example:
import socket # Create the socket s = socket.socket(socket.AF_INET, socket.SOCK_STREAM) # Connect to the server s.connect(("hostname", 80))
This Python script creates a socket and connects to a server using port 80 (HTTP). Understanding ports and protocols greatly simplifies coding practices. Always remember, knowing the essential difference and application of ports and protocols puts you ahead in the game of computer networking.
For further reading, Wikipedia’s article on Computer Ports and Communication Protocols delve into extensive details providing a more thorough understanding.