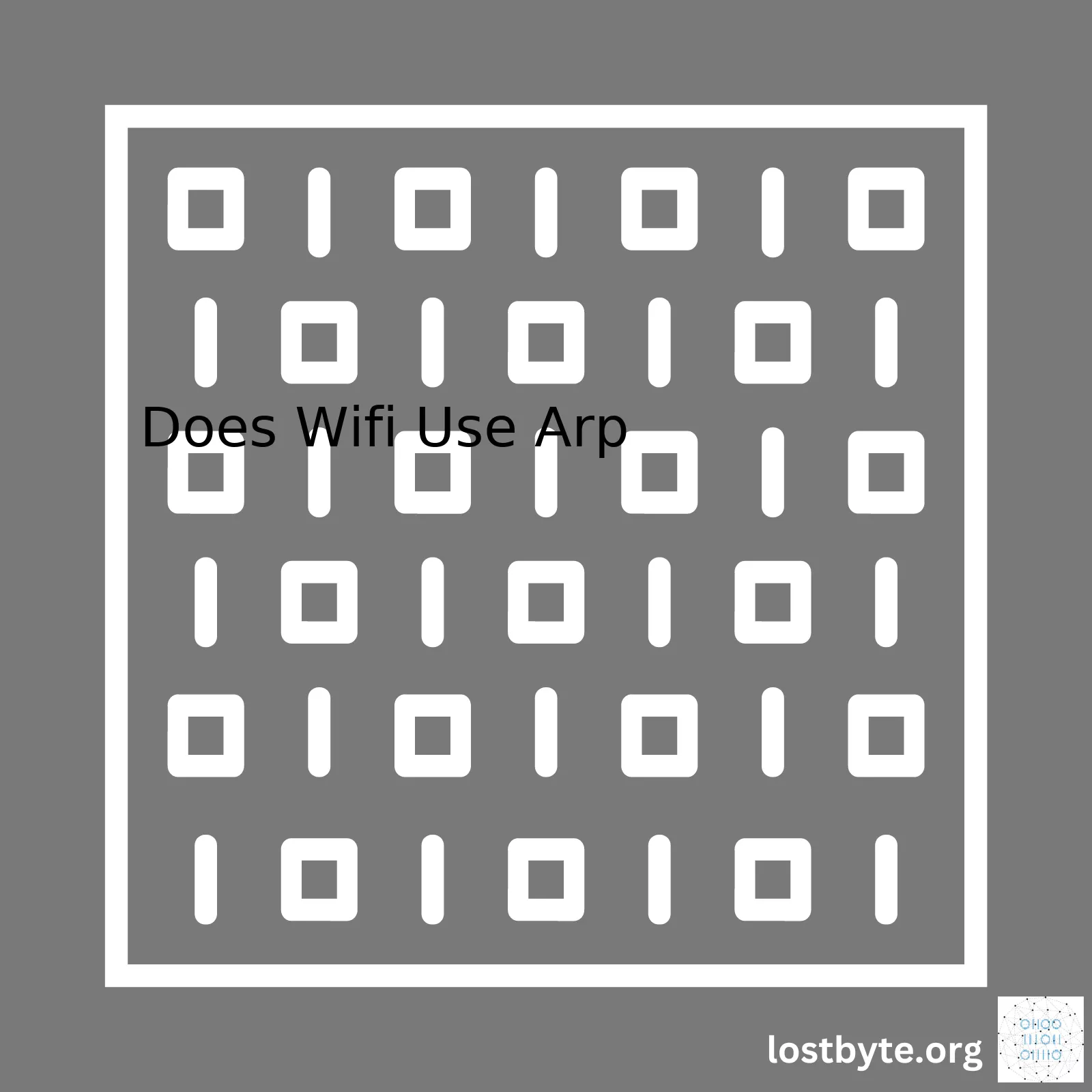
Topic | Details |
---|---|
Does WiFi use ARP? | Yes |
Role of ARP in WiFi | Helps map an IP address to physical MAC addresses of devices on the same network subnet |
Functionality of ARP over WiFi | Allows wireless gadgets to communicate traffic in a mutual language accepted by the network’s internet protocol suite |
Risks involved | Can expose a device to risks such as ARP Spoofing where a hostile element can impersonate a device’s MAC Address in the network |
In discussing how Wifi uses ARP – it’s crucial to realize that these two elements play key roles in managing our everyday digital communications. Wifi operates as an avenue for a wireless connection of devices to the internet while ARP is integral in linking an Internet Protocol address (IP) to the physical Media Access Control address (MAC) of devices existing within the same network subnet.
To break down the functionality of the ARP over Wifi – consider this instance; let’s say your personal computer wants to correspond with another gadget over Wifi. However, while IPs are unique to each device on the network, physical devices require physical addresses (MAC Addresses) for direct communication. This is where the ARP comes into action. It translates the IP addresses into MAC addresses enabling your PC to send data correctly. The entire process takes place in your router – being the default gateway, it manages the ARP table containing details of all devices and their corresponding MAC addresses (Wikipedia).
The role of ARP in Wifi operations, however, can expose devices to cybersecurity threats such as ARP spoofing. Herein, a malicious element may try to link its MAC address to the IP address of another device on your local area network thereby intercepting communications meant for that device without raising alerts in the network. Protecting against it involves tactics like Private VLANs or usage of security technologies like Dynamic ARP Inspection (Cisco Systems).
# Here is an example using Scapy library in Python showing how one might use ARP from scapy.all import ARP, Ether, srp target_ip = "192.168.1.0/24" #defining the target IP range arp = ARP(pdst=target_ip) #Create an ARP request packet ether = Ether(dst="ff:ff:ff:ff:ff:ff") #Create an ether broadcast packet packet = ether/arp #stacking both packets together result = srp(packet, timeout=3, verbose=0)[0] #Send the packet on the networkand capture the response #A loop to parse the response for sent, received in result: print(received.psrc + "------>" + received.hwsrc)
Certainly, let’s dig into the Address Resolution Protocol (ARP) and its role in WiFi connectivity.
ARP
, a network protocol used to find out the hardware or MAC address associated with a given Internet Protocol (IP) address, plays an integral part in WiFi communications. This vital networking protocol basically ensures that data sent over WiFi reaches the intended recipient accurately and efficiently.
How ARP Operates In A Local Network?
Let’s take an example to explain how ARP works in the scheme of WiFi communications:
Suppose your device wants to communicate with another device on the same local network. Your device knows the IP address but not the associated MAC address of the destination device. Here comes ARP into play.
– Your computer sends out an ARP request broadcast packet across your local network. This packet essentially says, “Who has this particular IP address?”
– Each device on your network receives this ARP request.
– The device with the corresponding IP address then replies back saying, “It’s me!” along with its MAC address.
– Your computer then learns from the ARP reply and stores this mapping of IP to MAC addresses in the ARP table for future communication.
By knowing the target device’s MAC address directly associated with its IP address, your device can now successfully direct WiFi traffic to the correct receiver on the same network.
The Relation of ARP and WiFi
WiFi is essentially a method of transmitting data wirelessly, while ARP is a protocol determining how that data should be routed within a local network based on IP and MAC addresses. Thus, ARP is essential for your WiFi devices – laptops, smartphones, or any WiFi-enabled IoT devices – to “understand” who’s who on the same network and accurately route all wireless data streams.
Remember though, ARP operates primarily in a local area network (LAN) context, which applies to pretty much every WiFi setting: your home network, office network, or even a public hotspot. When communicating beyond that – over the internet – other protocols like the Internet Protocol (IP) come into play.
Code Example
Since we’re talking about its influence in coding, let’s give you a glimpse of ARP in action. Although manipulating ARP tables is usually not part of an application developer’s job (it’s more of a network engineer’s concern), the following Python code using Scapy library will initiate ARP request concerning an IP address and display the result:
from scapy.all import ARP, Ether, srp target_ip = "192.168.1.1" arp = ARP(pdst=target_ip) ether = Ether(dst="ff:ff:ff:ff:ff:ff") packet = ether/arp result = srp(packet, timeout=3, verbose=0)[0] for sent,received in result: print(received.summary())
Try running the above code in your own environment, replacing “192.168.1.1” with a local IP address in your actual WiFi network. See how that goes to understand more tangibly how exactly WiFi uses ARP.
As we’re discussing the topic of ARP’s relation to WiFi, it’s worth noting that security vulnerabilities exist because of the open nature of ARP requests and responses allowing anyone in your local network to intercept these packets. Be familiar with network security best practices to mitigate risks around ARP poisoning.
In conclusion, ARP is crucial in ensuring successful WiFi communications. Suppose you are keen on learning more about networking protocols and how they shape our digital communications infrastructure. In that case, exploring topics around TCP/IP, DNS, and others will provide broader insights.Sure! Address Resolution Protocol or ARP is integral to any data network, including WiFi. To understand whether WiFi uses ARP, we need to understand how the ARP works.
The Address Resolution Protocol (ARP) is predominantly used to translate IP addresses into MAC (Media Access Control) addresses in a local network. This happens when a device wants to communicate with another device on the same network and it only knows the IP address of that device.
Let’s say for instance, your computer (A) wants to send data to another computer (B), which is connected to the same WiFi. Here is what happens:
- Your computer sends out an ARP request packet onto the network
- This request contains the IP address of Computer B you want to communicate with
- All devices in the network receive this ARP request
- The device that has the corresponding IP address (in this case, Computer B), will respond with its MAC address.
- Your computer then stores this IP-to-MAC mapping in its ARP table for future reference. After this, your computer can directly use the MAC address to send data, eliminating the delay of having to send an ARP request first.
Now let’s bring WiFi into the picture here:
WiFi operates at layer 2 (Data Link layer) of the OSI model where it employs MAC addressing to identify each device in the network. So when two devices want to transmit data over WiFi, they need to know each other’s MAC addresses. Therefore, if the sender only knows the receiver’s IP address (from layer 3/ Network layer), it will use ARP to find out the receiver’s MAC address. Hence, yes, WiFi does make use of ARP.
Here is a Python code snippet to show how ARP requests are generated:
from scapy.all import ARP, Ether, srp target_ip = "192.168.1.0/24" arp = ARP(pdst=target_ip) ether = Ether(dst="ff:ff:ff:ff:ff:ff") packet = ether/arp result = srp(packet, timeout=3, verbose=0)[0] # a list of clients, we will fill this in the upcoming loop clients = [] for sent, received in result: clients.append({'ip': received.psrc, 'mac': received.hwsrc}) print("Available devices in the network:") print("IP" + " "*18+"MAC") for client in clients: print("{:16} {}".format(client['ip'], client['mac']))
This Python script sends out an ARP request over the network and lists all responding devices along with their respective MAC addresses. This personifies the very function of ARP in WiFi networks.
For additional reading, please refer to these references:
Remember, the goal of ARP in WiFi networks is to facilitate communication among devices by efficiently resolving their MAC addresses.Certainly! The Address Resolution Protocol (ARP) is an essential part often used in every Wi-Fi network setup. ARP’s main function is to translate the Internet Protocol (IP) addressing system into the physical MAC address of the device associated with that particular IP.
Just imagine that you’re in your home and you wish to connect to your Wi-Fi network from your laptop or smartphone. Your computer sends a request to your router for IP information, and it’s ARP who steps in at this point. It helps map the IP addresses to the physical MAC Addresses of devices, something which is needed for communication within the local network. Here’s a simple way to understand this:
- Your laptop has an IP – let’s call it ‘IP_A’
- Your router has an IP – let’s label this as ‘IP_B’
Now, let’s assume your laptop wants to send some data packets over the internet. To do this, it needs to first send them to your router (because that’s your gateway to the internet). But it doesn’t know the MAC Address of the router. This is when ARP comes to the rescue by mapping this relationship as such:
“IP_B belongs to XYZ MAC Address”
This working of ARP can also be understood via the following pseudo code:
If(ARP_Cache contains entry for IP_B) {send packet directly to corresponding MAC} Else { Send ARP_Request for IP_B; Receive ARP_Reply containing MAC for IP_B; Update ARP_Cache; Send packet to received MAC; }
Here ‘ARP_Cache’ represents a temporary storage where ARP maintains a list of IP to MAC mappings for future use.
These actions of ARP ensure seamless communication between different devices on the same network. However, please be aware that this straightforward protocol can also be exploited viciously, mainly in attacks known as ‘ARP spoofing’. In this type of attack, malicious actors send fake ARP messages to link their MAC address with the IP of a legitimate computer or server on the network.
Such an attack causes significant harm, allowing the hacker to intercept, modify, or even stop data transmissions. Therefore, implementing measures like dynamic ARP inspection (DAI) or using VPNs for secured connections become critical in maintaining security within a Wi-Fi network.
For further understanding about ARP and how it functions, you can refer to the official document on RFC 826.Absolutely! Let’s dissect how wireless devices, specifically WiFi, utilize the Address Resolution Protocol (ARP). ARP is an integral aspect of IP networking and is used heavily in the operation of WiFi networks, offering a fundamental bridge between physical and logical addressing.
To understand the utilization of ARP, you first need to grasp what ARP does. ARP serves as a mediator for the Internet Layer and the Network Interface Layer within the TCP/IP model (StudyCCNA). At the Internet layer, IP addresses are used, but at the Network interface layer, every network device has a unique MAC address – also referred to as a physical or hardware address.
Now, if data needs to be transported from one device to another over a network, the IP address isn’t enough. The system needs to know what the corresponding MAC address is. This information is where ARP comes into play: it allows a system to discover the MAC address associated with a particular IP address.
WiFi networks operate with ARP exactly the same way any other Ethernet-based network would. For instance, when a smartphone connects to a WiFi network:
GET / HTTP/1.1 Host: www.example.net
* Its IP stack forms an IP packet to the destination, but it needs to encapsulate this IP packet inside an Ethernet frame.
* The destination’s MAC address is required to build this frame, but the device only knows the IP address.
* So, it queries the ARP table, which holds a list of recent IP-MAC pairs.
* If it can’t find a match, it sends out an ARP request packet asking “Who has this IP?”. This process is called ARPing.
* Any device on the network, having that IP, would respond with its MAC address.
A snapshot of an example ARP packet would look like:
HardwareType | ProtocolType | Operation | SenderMACAddress | SenderIPAddress | TargetMACAddress | TargetIPAddress |
---|---|---|---|---|---|---|
Ethernet | IPv4 | Request | 12:34:56:78:9A:BC | 192.0.2.1 | (empty) | 192.0.2.2 |
Hence, the WiFi communications rely heavily on this simple but essential protocol. It brings together the world of IP (how most applications view network communication) and the world of Ethernet (which is how network infrastructure like Wi-Fi transmits data packets). <+a href="https://techterms.com/definition/arp">TechTerms
// Defining the ARP request packet struct arp_hdr { uint16_t htype; uint16_t ptype; uint8_t hlen; uint8_t plen; uint16_t opcode; char sender_mac[6]; char sender_ip[4]; char target_mac[6]; char target_ip[4]; };
However, it’s worth mentioning that although ARP is a vital component in networking, it also poses some security risks — specifically, ARP Spoofing (also known as ARP Poisoning). In such an attack, a malicious actor sends fake ARP messages over a local area network, misleading other network devices into associating the attacker’s MAC address with an IP address of a legitimate network participant (typically the default gateway). This misuse allows the attacker to intercept, modify or even stop data transmissions.
Remember that the information assurance practice known as ARP spoofing detection and prevention can help protect against these attacks. Tools like Arpwatch, ArpON, and XArp monitor ARP traffic on a network and alert system administrators about any suspicious activities.
So, while ARP is an essential element in establishing connections and facilitating data transmission in Wifi networks, sound security practices should be reinforced to mitigate potential ARP-related threats.The Address Resolution Protocol (ARP) plays an integral part in facilitating communication over networks, including WiFi. It serves as a critical junction between network and data link layers in the OSI model.
The primary function of ARP revolves around mapping IP addresses to Media Access Control (MAC) addresses. Every internet-capable device has both an IP and MAC address.
Let’s delve into how this works with respect to Wi-Fi connections, wherein the interplay between node IP/MAC address and ARP serves to maintain a stable network connection.
Consider a typical home wifi setup: Your respective device and router have unique IP addresses within your local network. Communication is established via these IP addresses, but data transfer relies on the MAC addresses known only by the router.
This is where ARP steps in – effectively connecting these two aspects of communication. It’s akin to your laptop knowing your router’s nickname (IP address), but needing its full name (MAC address) to send the letter (data packet).
Here’s how the process unfolds:
1. Device A wants to communicate with Device B. A knows B’s IP address but not the MAC address.
2. A sends an ARP request onto the network stating “Who has this IP? Respond with your MAC”
3. The device owning that IP responds with its MAC address.
4. A then caches this information (ARP cache) for further communication without needing repeated ARP broadcasts.
Take a look at a sample ARP request below:
A: “Who owns 192.168.0.10? Reply to 192.168.0.2 (own IP).”
And here’s a dummy ARP reply:
B: “192.168.0.10 is at MAC: 00-14-22-01-23-45”
Crucially for WiFi users, this mechanism enables mobile devices on wireless LANs (WLANs) to seamlessly move across different access points within the same subnet/WLAN. It performs proxy ARP for associated clients, masking physical location changes from the rest of the network.
It’s also worth mentioning about Gratuitous ARP, a type of ARP that helps nodes to update their cache or resolve IP duplicate issues before they happen. It could be either a request/reply to inform other nodes about the IP/MAC binding change of a certain host.
For instance, when you enable Wifi on your smartphone, it might send a gratuitous ARP to announce its presence and pre-empt any IP conflicts.
Code snippet pertaining to Gratuitous ARP might look something like this:
Source MAC: MAC_smartphone Destination MAC: ff:ff:ff:ff:ff:ff (Broadcast) Source IP: IP_smartphone Destination IP: IP_smartphone Opcode: Request (1, for ARP request) or Reply (2, for ARP reply)
In summary, whether wired or WiFi, understanding how ARP operates can aid in troubleshooting network connectivity issues. Developing a more robust grasp of ARP also equips you with better insights about potential security vulnerabilities such as ARP spoofing, a prevalent form of network attack.[1][2][3].Absolutely, ARP or Address Resolution Protocol is an essential part of any network, including WiFi networks. In basic terms, the ARP process is a procedure used to map an IP address (Network Layer) to a MAC address (Data Link Layer) within a Local Area Network (LAN). Considering the nature of WiFi communication, which takes place purely at the Data Link and Physical Layers, the utilization of ARP becomes even more pronounced.
The process begins when a device connected to the network, say Device A, wants to communicate with another device, Device B, on the same network. However, while Device A knows the IP address of Device B, it doesn’t know its MAC address, which is crucial for data link layer communication (which WiFi relies on). Therefore, it has to find out using ARP.
Here’s how the whole process takes place:
1. –– Device A sends an ARP request: The ARP request essentially asks "Who has this IP address? Please send your MAC address." This request gets broadcast to all devices on the LAN. This would be something akin to Device A shouting in a room full of people (the LAN), "Who here is Device B?". 2. –– All nodes in the LAN receive the ARP request: Since the request is broadcast, all devices interconnected by the LAN will receive it. Except for two devices - the sender itself and the target device - the other devices will simply ignore this request. 3. –– Only the intended recipient responds: If Device B is in the LAN and hears the request (i.e., it matches the IP address specified in the request with its own), it responds with its MAC address in an ARP reply. This can be likened to Device B saying, "I am Device B, and this is my MAC address." 4. –– Finally, Device A receives the ARP response and updates its ARP table: An ARP table is essentially a "guest list", where each "guest" (device) is associated with "an identification tag" (their respective MAC addresses). Once Device A gets the response from Device B, it can update its ARP table accordingly, associating the MAC address received with the known IP address of Device B. This effectively completes the ARP process.
In essence, the entire WiFi communication hinges quite significantly on the successful operation of ARP requests and replies. Every device accessing a WiFi network needs to resolve the layer-3 IP address to a layer-2 MAC address for successful packet transmission, making ARP instrumental for any data exchange over WiFi.
To answer the initial inquiry again, does WiFi use ARP? Yes, undoubtedly so. It’s precisely because of protocols like ARP that WiFi devices can freely communicate with one another, without needing the end-user to manually mediate these data link-layer communications.
For further reading: For a more comprehensive understanding of ARP in networking, you might want to head over to GeeksforGeeks article on ARP. Also, the role of ARP within Wi-Fi networks is well documented in many books related to network engineering, such as “Computer Networks” by Andrew S. Tanenbaum and David J. Wetherall.Wi-Fi indeed utilizes ARP (Address Resolution Protocol), which has both static and dynamic mechanisms. Briefly, as a protocol used in IPv4 networks, ARP links the IP address (network layer) with the MAC address (data-link layer). It enables devices to find the MAC address of a peer given its IP address when nodes are communicating in the same network.
Static ARP:
In static ARP, entries are manually inputted into the ARP cache reserved memory. If a corresponding entry is found inside this statically configured ARP cache when a request comes in, responding occurs through this table rather than generating a broadcast.
Example of static ARP:
arp -s 192.168.0.1 00-aa-00-62-c6-09
Where, ‘-s’ indicates setting a new entry, ‘192.168.0.1’ is the IP of the mapping system, and ’00-aa-00-62-c6-09′ stands for the hardware (MAC) address equivalent to the IP.
Static ARP requests don’t need any physical interface because they are made directly to the host software. Moreover, such entries don’t terminate except if manually removed.
Dynamic ARP:
In contrast, dynamic ARP is automatic. Here, when two devices want to communicate on a Local Area Network and the destination’s MAC address is unknown, ARP broadcasts an ARP request packet over the network.
Upon receiving the request, every host compares this with their own IP address. When the IP matches, the recipient sends an ARP response containing its MAC address. Thus, the sender can pair the IP and MAC addresses in its ARP table for future use.
Here’s a brief overview of how a computer running Linux may show Dynamic ARP Entries:
ip neighbour show
The benefits of having dynamic ARP lie in its automation, freeing users from inputting mappings manually. However, these expire after a predetermined interval if not refreshed, often leading to a higher network load due to regular ARP queries.
So, essentially, for Wi-Fi or any other Local Area Network (LAN) transmissions, ARP plays a crucial part. Irrespective of being static or dynamic, both methods ultimately facilitate data transfer by interconnecting IP and MAC addresses. By understanding ARP’s importance within their network, administrators can monitor IP-MAC mappings to ensure optimum functioning and prevent potential security vulnerabilities like ARP Spoofing.
References:
RFC 826 – An Ethernet Address Resolution Protocol
Understanding ARP Broadcast ActivityIn an intricate digital dance, Wi-Fi communications and ARP (Address Resolution Protocol) tables interact with each other to support connectivity between devices on a network. If you’re wondering “Does Wi-Fi use ARP?” then yes, it does. To fully appreciate this relationship, we must first understand the complexity of each role in isolation, and consequently, their interaction.
ARP
is a protocol used to map an IP address to a hardware address. In a local network, when a device has information to send to another device, it will need both the IP and MAC addresses of the destination device. If only the IP address is available, the sending device broadcasts an ARP request over the network to determine the corresponding MAC address. The recipient of this broadcasted request responds with its MAC address so that communication can occur accurately.
IP Address | MAC Address |
---|---|
192.168.1.10 | 00:0a:95:9d:68:16 |
As for
Wi-Fi
, it is a wireless networking technology that uses radio waves to provide wireless high-speed internet and network connections. Devices within range can discover and connect to Wi-Fi networks to communicate with other devices or access broader networks like the internet.
With regards to how they work together:
• ARP and Wi-Fi interact closely in WLAN (Wireless Local Area Networks). For a new device to join a Wi-Fi network, it’s not just about having the correct security key. The wireless router also needs to recognize the device’s MAC and IP addresses for it to successfully join and engage this network – here is where ARP tables come in handy.
• ARP manages a cache of known IP and MAC address pairs. From this cache (the ARP table), devices on the network can find out other devices’ addresses without needing to send ARP requests each time.
• When you connect your device to Wi-Fi, the router adds your device’s MAC and IP addresses to its ARP table.
• WiFi can experience ARP spoofing, in which a malicious entity sends falsified ARP messages over a local area network resulting in the linking of an attacker’s MAC address with the IP address of a legitimate computer or server on the network. This could interrupt network traffic or gain unauthorized access to data.
So in general, Wi-Fi does use ARP for establishing initial connection between devices, mapping IP addresses to MAC addresses, managing network traffic and enhancing efficiency of network communications.
What happens when you shuttle data across the WiFi? It’s more than just magic. The combined operations and mutual support of network protocols like ARP and technologies like Wi-Fi ensure that data finds its way to the right place, firmly entwining these two elements in a vital digital combination (networkworld).
For example, let’s take python code snippet getting the MAC address using ARP:
from scapy.all import ARP, Ether, srp target_ip = "192.168.1.10" # IP Address for the default gateway (can be found executing ipconfig command) ip_gateway = "192.168.1.1" arp = ARP(pdst=target_ip) ether = Ether(dst="ff:ff:ff:ff:ff:ff") packet = ether/arp result = srp(packet, timeout=3, verbose=0)[0] clients = [] for sent, received in result: clients.append({'ip': received.psrc, 'mac': received.hwsrc}) print("Available devices in the network:") print("IP" + " "*18+"MAC") for client in clients: print("{:16} {}".format(client['ip'], client['mac']))
When executed, this Python script leverages Scapy library to create an ARP request (targeted at the specified IP address). It then wraps this ARP request with an Ethernet frame. The Ethernet frame aims at the destination MAC address with the special ‘ff:ff:ff:ff:ff:ff’ value –this is recognized as a broadcast frame, which should be picked up by all devices in the network segment. Finally, the script prints out the IP and MAC addresses of all devices that responded to the ARP request.Are Wi-Fi networks susceptible to ARP (Address Resolution Protocol) attacks? Indeed, they are and the peril posed by these potential invasions amplifies the quintessential role of ensuring robust wireless network security. ARP is fundamental in the process of resolving IP addresses into MAC (Media Access Control) addresses which facilitates correspondence between devices on a local network. Here’s an interesting fact. This equation accommodates even Wi-Fi networks. Henceforth, we can’t negate ARPs interaction with Wi-Fi.
To further cement your understanding, allow me to delve deeper into ARP’s mechanics. As a software layer protocol in the TCP/IP stack, ARP can be termed as the “middleman”, operating for the seamless routing of messages within the network. Picture this—an incoming packet assigned to your IP address lands on your router. How does it find its way to your specific machine? ARP takes center-stage here, providing the necessary roadmap.
ARP request: Who has 192.168.1.2? Please reply with your MAC. ARP reply: 192.168.1.2 is at AA:BB:CC:DD:EE:FF
The genuine use of the protocol is unsuspecting; however, the absence of an authentication mechanism flips the coin. Attackers can seize this opportunity to launch ARP attacks which primarily include ARP poisoning or spoofing, leading to adversities such as data interception and network downtime—threats that no organization can afford to dismiss lightly.
Type of ARP Attack | Method | Repercussions |
---|---|---|
ARP Poisoning/Spoofing | Deceitful linking of an attacker’s MAC address with an authentic IP address through fraudulent ARP messages. | Data theft, session hijacking, denial of service. |
Read here for an in-depth view into ARP Spoofing attacks.
Realizing the tremendous consequence of underrated Wi-Fi security practices, I implore you to take stringent measures to save your networks from the fangs of ARP attacks now that you fathom the implied vulnerabilities. Note that several countermeasures exist, including:
* Encryption mechanisms like WPA3 or IEEE 802.1X
* Static ARP entries that nullify false mappings
* Traffic monitoring using Intrusion Detection Systems (IDS)
Astonishingly what seems trivial—an exchange of a few bytes of data—is capable of inflicting massive damage if left unchecked. Wi-Fi, despite its convenience, indeed interacts with ARP, drawing in associated threats. Instituting formidable barriers against these intrusions is unquestionably high priority.
In this era of rampant cybersecurity violations, you shouldn’t wait until tomorrow. Putting off safeguarding your Wi-Fi network opens up unnecessary gateways for ARP attacks. Make the move now and laud your decision in posterity.Yes, Wifi indeed uses ARP (Address Resolution Protocol). When working in a wireless network environment, the computers or devices connected to this network interact with each other using their IP addresses. However, these IP addresses are not sufficient when data packets need to be transmitted at the link layer of the network stack. This is where ARP enters into action.
ARP is an essential networking protocol used by Internet Protocol (IP) for mapping an IP address to a physical or MAC (Media Access Control) address. It allows a system to find the MAC address of a device given its IP address.
ARP Request: Who has 192.168.1.70? Tell 192.168.1.101. ARP Reply: 192.168.1.70 is at 00: 0c:29: cb:b8:00
In such a typical example, 192.168.1.101 is the IP address that needs to know the MAC address of 192.168.1.70. Once it gets the MAC address through ARP reply, it can transmit data packages correctly.
There are two types of ARP messages:
– ARP Request messages act as questions, asking “who has this IP address?”
– ARP Reply messages are answers to these requests, stating “here is the MAC address linked with that IP.”
In the case of WIFI networks, the process is no different than wired ones. When two devices on a Wi-Fi network want to communicate, they also use ARP to find out each other’s MAC addresses so they can start exchanging information. A device sends an ARP request packet to all devices on the same subnet. The device with the matching IP responds to the request with an ARP reply, containing its MAC address.
If we need to diagnose situations where the communication is failing, you may use tools like Wireshark to inspect ARP traffic, or ARP-scan to list all live hosts in your local network.
The extensive use and criticality of ARP in networks might bring some security considerations. ARP spoofing attacks, where an attacker sends fake ARP messages to link its MAC address with the IP of a legitimate device is one of them. In such cases, you can make use of utilities like ARPON, which is capable of mitigating ARP poisoning/spoofing problems.
To recapitulate, WiFi does make use of ARP for associating IP and MAC addresses to ensure connectivity within the network. Whether you’re discerning why device A can’t talk to device B on your home network, or attacking a security issue on a large corporate network, understanding how ARP functions is key.