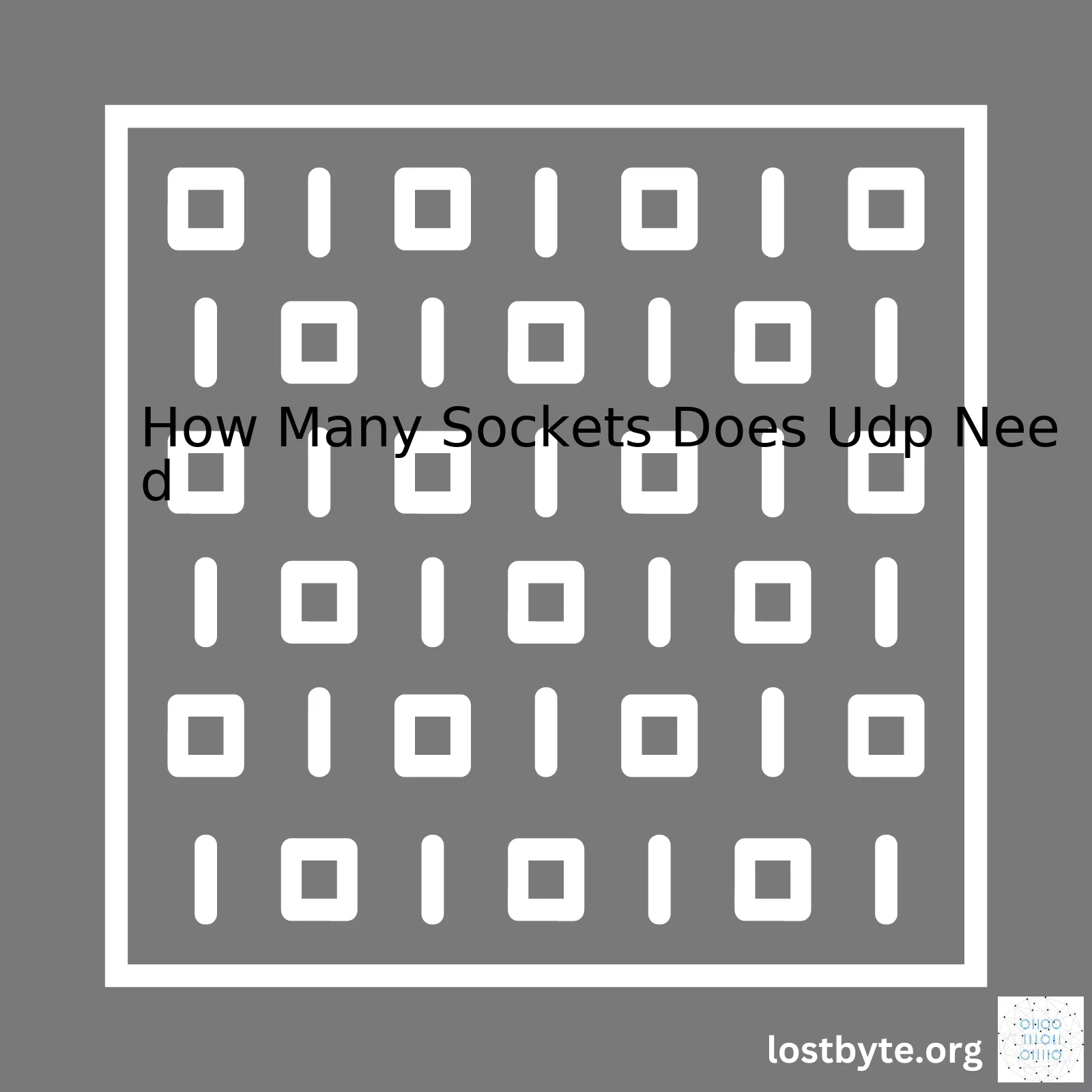
Action | Socket Required |
---|---|
Send Data | 1 |
Receive Data | 1 |
Connection-oriented protocols like Transmission Control Protocol (TCP) require a pair of sockets—one at each end—for communication. In sharp contrast, UDP, being a connectionless protocol, demands just one socket. For sending or receiving data, the same socket can be used; no separate sockets are required at both ends.
Let’s illustrate this concept with some Python code. Here is a simple example that demonstrates how to create UDP sockets. You’ll observe that we only need one socket (
sock = socket.socket(socket.AF_INET, socket.SOCK_DGRAM)
) to both send and receive messages:
import socket def main(): sock = socket.socket(socket.AF_INET, socket.SOCK_DGRAM) server_address = ('localhost', 12345) message = 'Hello, Server! This is Client.' try: # Send data print(f'Sending: {message}') sent = sock.sendto(message.encode(), server_address) # Receive response print('Waiting for response...') data, server = sock.recvfrom(4096) print(f'Received: {data.decode()}') finally: print('Closing socket.') sock.close() if __name__ == '__main__': main()
In conclusion, due to the nature of UDP being a connectionless protocol, it only needs one socket for communication, making it less complex and quicker than TCP. For further reading on the subject, refer to the official [Python Socket documentation](https://docs.python.org/3/library/socket.html). The same can be applied to other programming languages following the same principles for socket programming.
When we talk about UDP (User Datagram Protocol), it’s important to understand the way it functions, especially in connection with the number of sockets required. Unlike TCP (Transmission Control Protocol), which needs a socket for each end of a communication line (two sockets overall), UDP is quite different because of its connection-less nature.
UDP operates as a minimalist protocol, meaning it doesn’t add anything but the bare bones of transmission requirements in order to function. This makes it faster and more suitable for applications requiring real-time delivery.
A UDP client requires one socket to send and receive data. Technically, once a socket is “bound” — that is, it’s paired with an IP address and port combination — it can be used to send and receive data to/from any remote host. So, only one socket is needed, because UDP doesn’t retain connection state information between interacting hosts.
Here’s a basic Python example which shows a client-side UDP connection using one socket:
import socket UDP_IP_ADDRESS = "localhost" UDP_PORT_NO = 6789 serverSock = socket.socket(socket.AF_INET, socket.SOCK_DGRAM) while True: data, addr = serverSock.recvfrom(1024) print ("Received message:", data)
In the above code,
socket.socket()
originates a socket holder that would be used throughout the client usage span. The socket uses IP version 4 addresses
(AF_INET)
and a UDP protocol
(SOCK_DGRAM)
. This singular socket is then used to receive data through
serverSock.recvfrom(1024)
. The received message will eventually be printed out on the console.
For completeness, this is a server-side code:
import socket UDP_PORT_NO = 6789 UDP_IP_ADDRESS = "localhost" clientSock = socket.socket(socket.AF_INET, socket.SOCK_DGRAM) while True: MESSAGE = "Hello, Server!" clientSock.sendto(MESSAGE.encode('utf-8'), (UDP_IP_ADDRESS, UDP_PORT_NO))
In both instances, only one socket per-client or per-server is established for all communications. The client sends data out through the same socket where it also receives responses from several servers.
It is thought-provoking to mention – In case you want to mimic TCP-like behavior concerning managing separate sockets per host, it becomes your responsibility to handle that at the application level, since UDP itself won’t give you that out of the box.
Get to know more about UDP from Wikipedia and about Python Sockets from the Python official documentation.
The User Datagram Protocol (UDP) is a communication protocol used across the Internet for time-sensitive transmissions such as video playbacks or DNS lookups. It speeds up communications by not formally establishing a connection before data transfer and throwing error-checking out the window.
Now, if we’re talking about the number of sockets that UDP needs, it’s essential to know what a socket is first:
A socket is defined as one endpoint in a two-way communication link between two programs running over the network. These programs establish connections via their respective sockets. In simpler terms, consider sockets as talking points for programs to share information using network protocols like UDP.
For UDP, one socket is needed per each transmission stream – this is due to the connectionless nature of the protocol. That said, let’s handle two separate scenarios:
– Sending UDP packets
– Receiving UDP packets
When sending UDP packets, you’d typically need only one socket. The sender program creates a single UDP socket, packages the desired data into a datagram format, then sends it off towards the receiver’s IP address and port number. This can be illustrated with some Python pseudocode:
import socket UDP_IP = "localhost" UDP_PORT = 5005 MESSAGE = "Hello, World!" sock = socket.socket(socket.AF_INET, # Internet socket.SOCK_DGRAM) # UDP sock.sendto(MESSAGE, (UDP_IP, UDP_PORT))
This code represents a client that generates a socket (`socket.SOCK_DGRAM` which refers to a UDP socket). After creating that socket, the client can send data to a server listening on an IPV4 address (`socket.AF_INET`) through the specified port using the `sendto()` method.
On the receiving end, we still require only one socket. The receiver creates one UDP socket bound to the specific receiving IP address and port. When the packet arrives from the sender, it’s then unpackaged from the datagram format into usable data:
import socket UDP_IP = "localhost" UDP_PORT = 5005 sock = socket.socket(socket.AF_INET, # Internet socket.SOCK_DGRAM) # UDP sock.bind((UDP_IP, UDP_PORT)) while True: data, addr = sock.recvfrom(1024) # buffer size is 1024 bytes print("received message:", data)
The corresponding listening server works by initializing a socket and binding it to the expected port. With an endless loop, it waits for incoming data using the `recvfrom()` method, which also gives the client’s address.
So, taking everything into account:
– UDP utilizes a connectionless model hence requires a socket just at every data transfer point (sender or receiver).
– Each sender requires its own single socket to transmit UDP packets.
– Equally, each receiver requires its single socket to receive UDP packets.
Keep in mind that the count of sockets needed doesn’t align directly to UDP but rather to the underlying requirement for data input/output endpoints, i.e., sockets, during networked communication. You might sometimes see more than one socket serving a single application in situations where there’s data transfer moving in differing directions or towards different targets.
Therefore, the exact number of sockets in use becomes less tied directly to UDP as a protocol and more closely related to how individual applications are designed to use networking services.
Regardless, in the simplest design, for every single UDP data stream in operation, expect one UDP socket to be involved.
This exploration goes to show that understanding the foundations of Internet protocols like UDP equips you with vital insight into how our online world communicates. This knowledge subsequently empowers you to build technologies leveraging these protocols in more informed and insightful ways.
For authoritative insights on UDP and sockets, you may refer to [RFC 768](https://tools.ietf.org/html/rfc768), the original source material documenting the User Datagram Protocol. For practical experiences, Python’s official [Socket Programming HOWTO](https://docs.python.org/3/howto/sockets.html) provides hands-on insights into using sockets effectively in your programming journey.Under User Datagram Protocol (UDP), it’s important to understand that each individual connection requires a singular socket. It’s not like other protocols such as TCP where you might need a pair of sockets. So, if you need to establish three connections, you’ll require three sockets – not more, not less. What makes UDP stand out is its simplicity and speed in data transmission – an aspect hinged on the minimal socket requirement per connection.
Let’s break down why only one socket is needed for UDP:
– Connectionless Protocol:
This entails that there isn’t a dedicated end-to-end connection needed to transmit data; which explains why one socket is sufficient. As long as the data reaches the destination, even if it’s jumbled or packets are lost, UDP won’t dwell on ensuring order or reliability.source
– Single Port Communication:
Only a single port is required for both sending and receiving data when utilizing UDP. The system assigns this port to a socket, thereby making one socket quite enough.
Here’s a quick illustration using Python code on how to create a socket and transmit data over UDP:
import socket # Create a UDP socket sock = socket.socket(socket.AF_INET, socket.SOCK_DGRAM) server_address = ('localhost', 10000) message = 'This is our message.' try: # Send data print(message) sent = sock.sendto(message.encode('utf-8'), server_address) finally: # Close socket sock.close()
In this script, just one socket (sock) is necessary to send a message via UDP. This includes binding to a specific local IP address and port number, followed by creating a datagram packet with the desired output message not forgetting to specify server address for sending purposes. The end result, you ask? Successful data transmission using just one socket.
In simple terms, the UDP’s efficient mechanism helps improve speed, reduce latency, and ensure optimal application performance thanks to its single-socket usage policy. It’s a win-win situation overall- you get the job done with less resource consumption, and high-speed transmission is guaranteed.When discussing the transmission of data in network programming, it’s integral to consider how many sockets UDP needs. For User Datagram Protocol (UDP), there are specific factors that influence the number of sockets. These factors include purpose, design and network constraints.
Factor 1: Purpose of Communication
An essential factor for determining the number of UDP sockets needed is the purpose of communication. In a simple client-server model — where one server communicates with multiple clients — only two sockets might be necessary, i.e., one for the server and one for each client. Every new client connection opens another socket connection to facilitate communication.
Factor 2: Network Design
In more complex systems or peer-to-peer communication models, each system node has a different IP address or port. As a result, each node will require its own socket for direct communication. Therefore, the design and architecture of the network considerably affect the number of required UDP sockets.
Factor 3: Network Constraints
Lastly, network constraints such as IP address availability, bandwidth, and network policies can also influence the number of usable UDP sockets. Limited IP addresses may necessitate sharing of UDP sockets among multiple nodes.
A Java program specifying the use of UDP for socket communication looks like this:
import java.net.DatagramSocket; try { DatagramSocket socket = new DatagramSocket(); } catch(Exception e) { System.out.println("Error: " + e.getMessage()); }
Ultimately, there aren’t any upper limits to the number of sockets UDP can utilize apart from those imposed by your specific OS and any restrictions you might face due to IP address availability. This flexibility of being able to establish as many connections as we need is one of the many reasons why UDP is favorable in real-time applications or scenarios involving multithreaded communications.
For additional information on UDP protocol and socket programming, check out this resource. Learning about these factors and other influencing aspects will help draw a comprehensive understanding of how UDP and Sockets interact with each other in various networking environments. Thus, becoming more effective in optimising their usage.Sockets play an absolutely vital role when it comes to UDP communication. To understand the importance of sockets in UDP communication, we should first touch upon what UDP and sockets are.
UDP (User Datagram Protocol) is a simple transport-layer protocol that allows applications to send packets to their peers without requiring any prior communication. Communication done using UDP is often referred to as “fire-and-forget” because there’s no built-in mechanism to detect if the message made it to the recipient, or even if the recipient is ready to receive the message.
Turning now to sockets, these are programming constructs that enable interprocess communication. In the context of network programming, sockets are the endpoints of a bidirectional communication channel—sockets might be thought of as the mailboxes that allow your computer to receive messages from other computers.
Let’s hone in and consider sockets’ significance in UDP communication:
• Transmission of Data: Sockets serve as the endpoints for UDP communication. In order to send data across a network, the sender employs a socket to dispatch it, while the receiver uses another socket to accept it.
• Identification: In network programming, each socket is identified by a unique combination of an IP address and a port number. Given this, a computer differentiates among multiple incoming UDP communications using distinct sockets.
# Python code for creating UDP socket import socket sock = socket.socket(socket.AF_INET, socket.SOCK_DGRAM)
So how many sockets does UDP need?
If you think about a simple client-server communication model, UDP would require two sockets—one on the client side to send the communication, and one on the server side to receive it. Interestingly, this setup does not change even in concurrent communication involving multiple clients, as the server can manage multiple requests using a single socket. By identifying the source of each incoming request (information provided in the datagram), the server can facilitate concurrent communication without requiring a separate socket for each client.
This advantage underscores a key strength of UDP—it significantly reduces overhead compared to TCP, which necessitates separate connections (and thus separate sockets) for each communicating client. Employing less resources to handle multiple client requests by providing connectionless networking makes UDP suitable for broadcasting and multicasting where high performance is desired over reliability.
However, please bear in mind that more sophisticated applications might require additional sockets for varied purposes, such as if a client machine needs to concurrently communicate with several different servers.
Therefore, the number of sockets needed really depends on the exact nature of the communication scenario: primarily, whether it’s unidirectional or bidirectional, and also noting the number of involved parties and the complexity of the application at hand.
Reference: [IPv6 Socket Programming](https://books.google.co.in/books?id=yLsEzl5JNZwC&pg=PA9&lpg=PA9)
Tables:
Table 1: The use of Sockets in UDP communication
| Service | Use |
| — | — |
| Transmission of Data | As send and receive points|
| Identification | Using distinct IP addresses and port numbers |
Table 2: Requirement of Sockets depending on nature of UDP communication
| Factor | No. of Sockets |
| — | — |
| Simple unidirectional Client-Server Communication | 2 (One for sending and one for receiving) |
| Concurrent Communication/Multicasting/Broadcasting | 2 (Single socket managing multiple requests at both ends) |
| Bidirectional Simultaneous Communication | More than 2 |
| Complex Application | Depends on the specific requirements |Establishing a connection between Transmission Control Protocol (TCP) and User Datagram Protocol (UDP) involves a different approach from each protocol due to their inherent design properties.
TCP is termed a “connection-oriented” protocol, which means it ensures that data transmitted over the network reaches its destination intact and in order. On the other hand, UDP is a “connectionless” protocol. It doesn’t establish a formal connection with the receiving computer before sending data packets. In essence, it shoots them off to the destination without knowing whether they will make it successfully or not.
TCP Connection Establishment
For TCP communications, a connection needs to be established before data transmission can occur. This typically takes place with a procedure known as a three-way handshake:
Server: LISTEN Client: SYN_SENT ----> Server Server: SYN_RECEIVED <--- Client Client: ESTABLISHED <---- Server Server: ESTABLISHED
The client begins by sending a SYN message to the server. The server then acknowledges this by sending a SYN-ACK message back to the client. Finally, the client responds again with an ACK message, and from there, the connection is established and data transmission can proceed.
This model implies that for a single connection, TCP requires one socket on each end of the connection. So effectively, a total of two sockets are required for a standard, one-to-one TCP communication link.
UDP Connection Establishment
In contrast with TCP, UDP is connectionless, meaning it doesn't require a formally established connection to exchange data. Instead, it sends individual data packets, termed "datagrams", independently of each other:
* Client: send datagram ----> Server * Server: process datagram/request <--- Client * Client OR/AND Server: continue to send/receive more datagrams
Each UDP datagram has no idea whether subsequent or previous datagrams were received. UDP simply transmits data packets while hoping they all reach their destination correctly.
Now, about sockets: With UDP, you have two main options depending on your use case scenario:
- One socket per message: Since UDP does not establish a long-lasting session like TCP, you could technically open a new socket for every message, send the message, and then immediately close the socket again.
- One socket for multiple messages: Alternatively, for frequent transmissions to the same endpoint, you might choose to keep the socket open and reuse it for multiple messages. This way, your program needs to maintain only a single socket, assuming only one remote endpoint.
However, in practice, most UDP scenarios would likely utilize one socket per application or per thread of execution. Nevertheless, the number of UDP sockets required really depends on your specific requirements and system constraints.
For source code examples, I recommend referring to sites such as Real Python, where you'll get step-by-step tutorials on setting up TCP/UDP connections.
It's worth noting that while we describe these procedures using abstract terms, actual implementations can vary widely depending upon the specific programming language, operating system and networking environment.
Lastly, remember that whether you choose TCP or UDP largely depends on what you need for your application. If you need reliability over speed, go with TCP. If you need speed over reliability, stick with UDP. And always bear in mind that properly configuring and managing your sockets plays a crucial role in the overall performance and efficiency of your TCP or UDP connections.Online gaming is a popular application that uses multiple User Datagram Protocol (UDP) sockets. Unlike the Transmission Control Protocol (TCP), which guarantees delivery of packets in order, UDP just sends data without ensuring that it reaches its destination. This makes UDP perfect for real-time applications characteristic of online games as they require speed and continuous communication more than absolute reliability.
Let's dive deeper into how many sockets UDP actually requires. Essentially, each client-server connection needs at least one socket. So, if you have a multiplayer game with 1 server and 5 clients playing, you will require a minimum of 6 sockets.
Table-1: Overview
Entities | No. of Sockets |
---|---|
Server | 1 |
Clients | 5 |
Minimum Total | 6 |
//Creating a UDP socket int sockfd; char buffer[MAXLINE]; char *hello = "Hello from server"; struct sockaddr_in servaddr, cliaddr; // Creating socket file descriptor if ( (sockfd = socket(AF_INET, SOCK_DGRAM, 0)) < 0 ) { perror("socket creation failed"); exit(EXIT_FAILURE); }
The code snippet above demonstrates a sample creation of a UDP socket in a C program.
However, this is a simplistic model of sockets requirement. In a real-time practical scenario involving online gaming, each player (client) does not connect to the server through only one socket. They might need additional sockets for different features like audio chat, text chat, gameplay, pushing updates, telemetry etc. In addition, there are other considerations such as whether your design is using peer-to-peer (P2P) connectivity or client-server architecture. These factors can significantly impact the number of sockets required.
Briefly discussing some advanced scenarios, In the case of client-server architecture, the server has to maintain individual connections with each player, resulting in a higher demand for sockets. On the contrary, in P2P connections where each player is directly connected to every other player, the number of sockets escalates exponentially with each new player.
Taking all these aspects into account gives us an idea of how sockets act as the driving force behind UDP-based online gaming. It's important to note that efficient management of these sockets is critical for enhancing user experience by minimizing lag and maximizing real-time response, as desired in online gaming. You may learn more about UDP and how it works with sockets on Lifeware's detailed guide.Understanding the performance of your UDP network under different numbers of sockets is a critical factor in tuning and optimizing networking applications. It's crucial to consider:
1. The number of sockets required by UDP: Unlike TCP, which needs two sockets for communication (one at each end), UDP is connectionless and it only requires one socket for each client sending or receiving data.
2. Effect on Network Performance: Overloading a server with too many open UDP sockets may not necessarily contribute to better network performance. If not efficiently used, these open sockets could eat up system resources, causing slower processing times or even system crashes.
Now let us explore how to assess network performance including factors such as latency, throughput, packet loss, and bandwidth usage. You can use tools like iPerf, PingPlotter, or you can code your own programs using socket programming libraries in languages like C, Python or Java.
import socket sock = socket.socket(socket.AF_INET, socket.SOCK_DGRAM) server_address = ('localhost', 12345) message = b'This is our test message.' sock.sendto(message, server_address)Here, a single UDP socket is created, a message is created, and thesendto()function sends the message to the server over UDP. You can tweak this code to create multiple sockets if necessary.
To assess network performance:
• Measure **Bandwidth**: Calculate the amount of data that can be sent via the network in a fixed amount of time.
• Monitor **Latency**: Track the delay before a transfer of data begins following an instruction for its transfer.
• Check **Packet Loss**: Count the number of packets that fail to reach their destination.
• Test **Throughput**: Find the rate at which data travels through a network.
You can use tooling Wireshark to collect this data and then analyze it.
How does increasing or decreasing the number of sockets influence these factors? Remember, UDP is "fire-and-forget" type of protocol. So, having a large number of sockets doesn't guarantee that all packets will arrive at their destination or they will arrive in order.
Whether you should increase or decrease the number of sockets depends largely on the specifics of your application and network constraints. If your application needs to stream data to many clients concurrently, you might need more sockets. On the other hand, if you are dealing with less concurrent connections or small amounts of data, reducing the number of sockets could work effectively without impacting performance. It’s always about finding the right balance.
Keep in mind that the operating system has a limit on the number of sockets that a process can open. Reaching this limit could cause new socket creation to fail, so it's important to manage open sockets appropriately.
In essence, determining the number of UDP sockets needed for optimized network performance will require a careful balance between actionable insights from assessing latency, throughput, packet loss and bandwidth usage; adapting as per the nature of your network and application requirements.When it comes to transmission control protocol, there are two typical socket setups in a UDP (User Datagram Protocol) environment: the single-socket model or the multiple-socket model. Essentially, the key difference boils down to whether you wish to utilize one common socket for both sending and receiving data (single socket) or use different sockets (multiple sockets). The choice between using one socket or multiple sockets ultimately depends on your specific needs and the particular use-case scenario.
Let's delve into the implications and trade-offs of each option:
Single Socket
In the single socket setup, only one socket is employed for both sending and receiving data. Here are some considerations for this setup:
• Performance: Whatever data packet is received is managed by one socket only, possibly leading to less processing overhead compared to handling numerous sockets at once.
• Port Utilization: As you're using one port for your communication through the one socket, you can minimize the number of open ports, which could technically increase security.
• Complexity: Since you have only one place where all packets arrive, you need to employ methods to distinguish which client sent which data, making data management more complex.# Python example of single-socket UDP setup import socket sock = socket.socket(socket.AF_INET, socket.SOCK_DGRAM) server_address = ('localhost', 12345) sock.bind(server_address) while True: data, address = sock.recvfrom(4096) print(f"received {len(data)} bytes from {address}")Multiple Sockets
This model utilizes separate sockets for send and receive data operations. Some points to consider with this approach:
• Performance: Depending on the workload and system capacity, handling multiple sockets could lead to better performance as workload spread over multiple processes.
• Port Utilization: More sockets mean more ports in use, which could potentially result in higher vulnerability due to increased exposed surface area.
• Ease of Management: With distinct inbound and outbound data streams, data management becomes simpler. You always know where data is coming from and where it's going to, based on the socket being used.# Python example of multi-socket UDP setup import socket recv_socket = socket.socket(socket.AF_INET, socket.SOCK_DGRAM) recv_socket.bind(('localhost', 12345)) send_socket = socket.socket(socket.AF_INET, socket.SOCK_DGRAM) target_address = ('localhost', 54321) while True: data, address = recv_socket.recvfrom(4096) print(f"received {len(data)} bytes from {address}") if data: sent = send_socket.sendto(data, target_address) print(f"sent {sent} bytes back to {target_address}")In conclusion, the answer to the question "How Many Sockets Does UDP Need?" is simple: at least one. However, the optimal number often depends on case-specific considerations such as the workload nature, desired performance levels, complexity tolerance, and security preferences. In software design, such decisions often come with certain trade-offs. Therefore, understanding these trade-offs will enable you to make an informed decision that best suits your needs.
For more technical insights and analyses, you may refer to the official documentation of the JAVA datagram (UDP) and Python's socket library.While discussing UDP sockets, it's imperative to throw light on port association and its role in determining the number of required UDP sockets.
The UDP or User Datagram Protocol is a communication method utilized by networking software such as the DNS servers or Voice over Internet Protocol (VoIP) services. Keep in mind that each service that uses UDP prepares packets to be sent across the internet using the protocol, but they don't create the connection for transferring these packets.
Each UDP packet has its origin and destination addresses - IP and port. This is how port association comes into play when discussing UDP and its need for sockets.
Now, aligning this theme with "How Many Sockets Does UDP Need", we must understand:
* Each socket used by UDP corresponds to a particular service within a computer system, effectively the application that needs to send or receive data.
* As per its nature, UDP itself does not establish a connection like TCP; instead, it sends and receives individual packets independently of one another.If we talk about the number of sockets required, one must not forget that a single UDP server can interact with multiple clients simultaneously through the process of multiplexing. This necessitates a different approach to count the number of sockets.
Recall that, in the context of a single-server-multiple-clients architecture, the total number of sockets implemented is dependent on the number of clients connected at any given time, which could change dynamically.
To illustrate, let us analyze a Python code snippet that represents a simple UDP echo server.
import socket server_socket = socket.socket(socket.AF_INET, socket.SOCK_DGRAM) server_socket.bind(("", 12345)) while True: message, address = server_socket.recvfrom(1024) server_socket.sendto(message, address)In the above code, there is only one socket
server_socket, which listens to port 12345. It receives a client's message and sends it back to the same client's IP address and port. This is an example where one socket serves the purpose for multiple clients at different times, effectively requiring only a single socket for many clients.
To reiterate, the number of sockets required strictly depends on your application design and requirements, and port association also plays a significant role in this scenario.
Let's assume you have five different applications running as a UDP server. All of these will require separate UDP sockets because each would routinely bind with a unique port, distinguishing them from each other.
Here's the information summed up in a table format:
UDP Server Port Association No. of UDP Sockets Application 1 12345 1 Application 2 12346 1 Application 3 12347 1 Application 4 12348 1 Application 5 12349 1 It signifies that if each application binds to a unique port, despite being served by the same UDP protocol, each would need a distinct UDP socket.
Remember that using a unique UDP socket for each client enables the tracking of the source address and port for incoming datagrams and sets the destination for outgoing datagrams, a crucial aspect while dealing with distributed systems over a network.
Hence, while concluding, it's fair to infer that the number of UDP sockets required is majorly influenced by both the structure of the application and its demand for segregating data logically via distinct ports. For further reading, one can refer to Python Socket Programming, which offers excellent insights into UDP socket programming and its usability scope.When we talk about User Datagram Protocol (UDP), it's a connectionless protocol, meaning that you don't need to open or close the connection as in Transmission Control Protocol (TCP). However, setting up concurrent connections is still a relevant topic.
To establish concurrent connections using UDP, each individual data packet gets associated with an address and port number. This means, technically speaking, you could use only one UDP socket to handle multiple "connections" by differentiating packets based on their source IP and port.
Even if you take this approach though, there are certain factors that may steers us away from having just the single UDP socket:
- Code complexity: If everything goes through one socket, your code has to handle multiplexing and demultiplexing of all communication. If you separate these out into different sockets then your operating system will do this for you.
- Buffer overflows: All the incoming data goes into one single buffer. If you're receiving a lot of traffic this buffer might overflow before you have time to read all the packets.
- Parallelization constraints: Receiving from one socket typically needs to be done from one thread. By designating tasks to more sockets and threads, you can achieve better distribution of work and improved performance.
If you decide to rely on multiple UDP sockets, although technically it doesn't require separate sockets for each connection like TCP, you can create separate sockets for different clients in practice to make easier the separation of concerns. Here's a simple example with Python
socketlibrary:
import socket
sock1 = socket.socket(socket.AF_INET, socket.SOCK_DGRAM)
sock1.bind(("localhost", 8001))sock2 = socket.socket(socket.AF_INET, socket.SOCK_DGRAM)
sock2.bind(("localhost", 8002))
In this snippet, two UDP sockets were created, sock1 bound to port 8001 and sock2 bound to port 8002. They act independently and can communicate with different peers concurrently.
In conclusion, while theoretically you don't need to have multiple sockets for handling multiple UDP conversations, in practice and for simplicity, robustness and efficiency reasons, considering using multiple sockets could be a good idea. The exact number would depend on your specific case, including factors such as available system resources and expected load.
Source: Python for beginners - Port scanner codeWhen it comes to UDP (User Datagram Protocol) in real-time systems, the concept of sockets plays an instrumental role. Sockets provide an interface for programming networks, serving as an endpoint in a two-way communication link where network nodes exchange data.
In essence, at least one socket is required per each UDP connection and this holds true across both client-server and peer-to-peer models. However, when we align this concept with the context of a real-time system, the number of required UDP sockets could change depending on the complexity of the system.
## Multiple UDP Sockets in A Single Real-Time System
A single real-time system can require multiple UDP sockets for several reasons:
- **Differentiating between data types**: Different types of data may be transmitted over separate sockets. For instance, one socket might handle text-based commands while another handles binary audio or video data.
- **Allowance for parallel processes**: By creating separate sockets, different processes or threads can send and receive data concurrently without interfering with each other.
- **Isolating communication channels**: Each socket can serve as isolated communication lines between units within a system or across diverse systems.
Using this approach has potential benefits such as improving reliability and robustness of a system as it can ensure that if one socket fails, others stay operational thereby maintaining the system performance.
## How Many Sockets Does UDP Need?
The exact number of UDP sockets needed highly depends upon the requirements and design of your real-time system. Unambiguously, you need at least one socket for basic point-to-point communication.
However, in scenarios involving multi-threaded servers, multicast transmission, or simultaneous transmission of different data types, you could require multiple UDP sockets. Thus, the number can vary greatly ranging from just one to tens or even hundreds in complex, large-scale systems.
This code snippet illustrates how to create a simple UDP socket in Python:
import socket sock = socket.socket(socket.AF_INET, socket.SOCK_DGRAM) server_address = ('localhost', 12345) sock.bind(server_address)
This will create a UDP socket bound to the local host on port 12345. You can create and manage multiple such sockets based on your system needs.
To reiterate, using multiple UDP sockets in real-time systems can provide benefits such as concurrent data processing and resilience, potentially increasing the efficiency of data handling in crucial real-time applications. However, care should be taken to manage these sockets effectively to prevent unnecessary resource usage and complexity.Understanding the concept of how many sockets UDP (User Datagram Protocol) needs, it'll be essential to delve deeply into what exactly UDP is and how it operates. Essentially, UDP is one of the core protocols within Internet protocol suites. It's widely recognized for its simplicity as it neither requires a sequential stream of data nor operates as a connection-oriented protocol.
When it comes to the number of sockets involved in a UDP, you only need two sockets:
- One socket on the client-side machine
- The other on the server-side machine
The sender pushes the datagram out onto the network through its socket, and eventually, the datagram lands up at the receiver's socket.
// Here's a simple example of how a UDP socket is created. // For instance, in Python: import socket sock = socket.socket(socket.AF_INET, socket.SOCK_DGRAM)
The sheer advantage offered by UDP is its highly efficient and rapid communication capability, which typically needs just one pair of sockets i.e., 2 sockets in total. However, do note that a socket is not tied exclusively to another socket. That means one socket can send out a multitude of UDP packets to different destination sockets (source). Therefore, multiple communications can be established simultaneously using just one single socket.
Sender | Receiver |
---|---|
One socket | One socket |
Unlike TCP that involves creating a connection before sending the data, thus requiring more resources including multiple sockets if you’re connecting with multiple destinations, UDP has no such constraint. This is why gaming and streaming services often use UDP due to its ability to handle packets independently.
To sum up, despite being connectionless, UDP typically only needs two sockets for interaction - one individually from the sender's and receiver's side each. Even when operationally interacting with multiple destinations, one socket remains feasible because UDP handles packets individually without establishing connections.