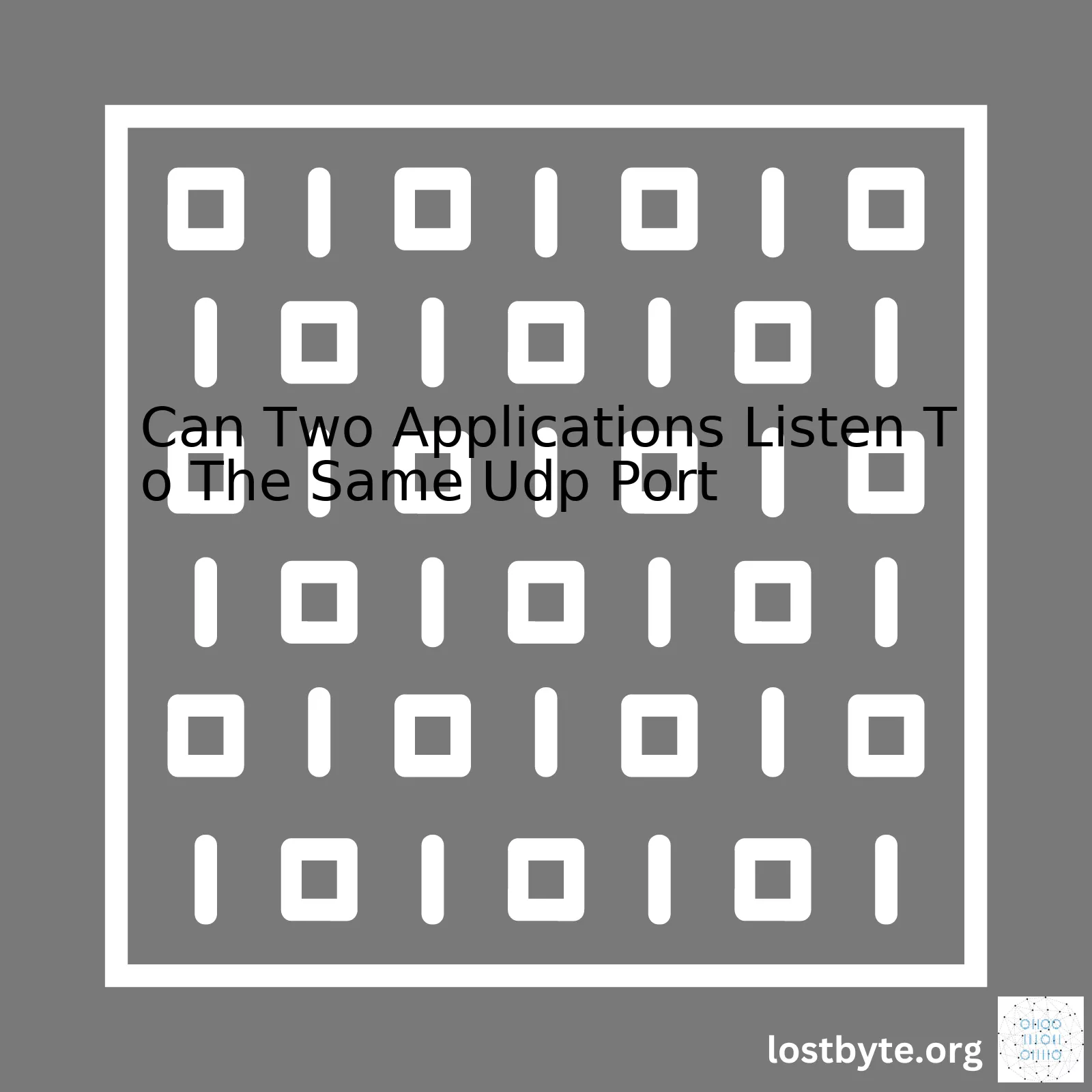
UDP Port | Single-Application Binding | Multi-Application Binding |
---|---|---|
Definition | A UDP port can only be bound to by a single application at a time. | A UIPC (Unix Interprocess Communication) method, such as SO_REUSEPORT, allows multiple applications to listen on the same UDP port. |
Limitation | Once an application has bound to a UDP port, no other application can use it until the binding application is shut down. | Applications that share a UDP port using this method must coordinate with each other to avoid data corruption and loss. |
Use Cases | Games, Video Conferencing, Online Streaming | Scaling socket-level concurrency, microservices running in Docker or Kubernetes environments |
With respect to the question “Can Two Applications Listen To The Same Udp Port?”, typically, two independent applications cannot listen to the same UDP port at the same time due to operating system constraints. A principle in networking called “port binding” restricts one network socket per IP address and port combination for UDP.
However, there is an exception when the applications are designed to use a Unix Interprocess Communication (IPC) technique like
SO_REUSEPORT
. This flag allows multiple applications to bind to the same socket simultaneously, effectively sharing the UDP port and listening for incoming packets. It’s noteworthy that enabling
SO_REUSEPORT
requires special programming considerations to handle concurrent incoming traffic and distribute it among the different applications. If not handled right ,it could lead to data corruption. Application examples include web servers or microservices that may need to scale socket-level concurrency or utilize distributed containerized environments like Docker or Kubernetes.
Recently, Linux and other modern platforms have implemented the
SO_REUSEPORT
socket option to their network stack to better handle the load of modern high-throughput network applications. For reference, refer to the LWN article on this feature.
Let’s consider a basic Python server that makes use of the
SO_REUSEPORT
option:
import socket # Create a new socket using IPv4 and UDP sock = socket.socket(socket.AF_INET, socket.SOCK_DGRAM) # Enable SO_REUSEPORT sock.setsockopt(socket.SOL_SOCKET, socket.SO_REUSEPORT, 1) # Bind to a port sock.bind(('localhost', 8000))
Every design has its place and purpose where benefits outweigh challenges. In the regular deployment of applications, avoiding conflict over port utilization is organized through port binding. However, when it is necessary, concurrent execution of services on the same UDP port using the
SO_REUSEPORT
becomes applicable, allowing for efficient resource utilization and system performance optimization.
Understanding User Datagram Protocol (UDP) ports involves understanding a bit about how network communication works – specifically, how two applications can listen to the same UDP port.
In terms of network communications, every application that communicates over the network does so using ‘ports’. When data is sent over a network, it is broken down into smaller packets by the Transport Layer of the OSI (Open Systems Interconnection) Model. There are several protocols at this layer, but the most commonly used ones are TCP (Transmission Control Protocol) and UDP (User Datagram Protocol).
data = socket.recvfrom(1024)
UDP is used for tasks that require fast, efficient transmission, such as games or live broadcasts. Unlike TCP which establishes a connection before sending data ensuring data will arrive in the order it was sent, for UDP, there is no formal setup process before transmission. This eliminates delay caused by the establishment of a connection.
UDP packets are sent to an IP address on a specific port, where an application listening on that port would receive them.
Can two applications listen to the same UDP port? The short answer is no, not usually. Ports by design perform a unique role of message routing within an operating system. When a packet arrives, the system needs to know where to direct that packet; this is done using the port number. If two applications were allowed to listen on the same port, there could be a conflict when deciding to which application deliver the received packet.
The kernel differentiates between applications or processes by associating server sockets with 5-tuple consisting protocol, local IP address, local port, remote IP and remote port.
Protocol | Local IP | Local Port | Remote IP | Remote Port |
---|---|---|---|---|
UDP | 192.168.0.1 | 55455 | 192.168.0.2 | 54321 |
However, through the magic of `SO_REUSEPORT`, multiple threads or services can bind to the exact same IP/port combination as long as they all set the option.
socket.setsockopt(SOL_SOCKET, SO_REUSEADDR, 1) socket.setsockopt(SOL_SOCKET, SO_REUSEPORT, 1)
This mechanism serves a practical purpose when considering things like high-performance web servers running on multi-core machines. By binding each worker to the same port, incoming connections get distributed across workers, improving efficiency across the cores on the machine.
To reference this functionality in more detail you can visit Linux Weekly News article by Jonathan Corbet. On systems such as Windows, the usage might differ or not be available altogether. Therefore, keep platform differences in mind while attempting to use this feature effectively and efficiently.
It should be noted though that `SO_REUSEPORT` should be used cautiously due to its drawbacks such as potential “port hijacking”. Multiple applications (not only instances/threads of the same app) are permitted to use the same socket concurrently – and if one of them is compromised, it might intercept all inbound traffic directed to any of its peers.Ah, the magical world of UDP Port Binding! Let’s delve deep into it and also understand how it affects if two applications can listen to the same UDP port or not.
UDP, aka User Datagram Protocol, serves as a basic transport layer system within Internet Protocol suite – think of it as a conveyor belt helping data travel between various systems over a network. It enables processes to send datagrams (chunks of data) to other processes with a minimal amount of protocol mechanism [1] .
So what is this Port Binding?
With port binding the application ‘binds’ to a specific network port and listens for incoming data addressed to that port [2]. Still confused? Let’s unbox it with a real-life analogy. Imagine your application as a gigantic warehouse receiving countless boxes (data packets), but these boxes aren’t just randomly thrown in. They’re delivered to certain labeled docks (ports) depending on their destination details.
Now, can Two Applications Listen To The Same UDP Port?
Here’s when things get exciting. To guess the answer without much ado – No, generally they don’t. When an application binds to a UDP port, it monopolizes that port. A simplified reason being, operating systems prevent multiple applications from broadcasting conflicting data on the same port to avoid any chaotic mess of crossed signals.
Imagine our warehouse again, with only one dock handler assigned to receive boxes at each port. If two handlers were to manage one dock, there would definitely be severe box clashes and catastrophic confusion. That’s precisely the kind of calamity OS aims to prevent. Hence we typically see a ‘address already in use’ error when an attempt to bind a second application to a UDP port is made [3].
But wait, there’s a twist to the tale. Technically speaking, you can actually make multiple applications listen to the same UDP port. This, however, requires utilizing the SO_REUSEADDR socket option which allows more than one socket to be bound to the same socket address [4]. In Linux, this is achieved using IP_ADD_MEMBERSHIP for setting up multicasting [5].
Here’s a handy example code (python-based):
# Making the socket reusable socket.setsockopt(socket.SOL_SOCKET, socket.SO_REUSEADDR, 1) # Enabling multicast mreq = struct.pack("=4sl", socket.inet_aton(MCAST_GRP), socket.INADDR_ANY) socket.setsockopt(socket.IPPROTO_IP, socket.IP_ADD_MEMBERSHIP, mreq)
Beware though! Operating with the same ports can cause potential packet overlapping, so unless it’s absolutely required, it’s mostly avoided.
To summarize, while two applications can listen to the same UDP port in some scenarios, usually it’s neither allowed nor recommended due to the possibility of data contamination and chaos management. Instead, it’s much better to thoughtfully distribute different port numbers among various applications to maintain smoother operations.
I hope this shines a light upon your question about the functionality of UDP port binding and its relationship to two applications listening to the same port! For further reading, checking out standard Application Layer Protocols that utilize UDP, like DNS or DHCP, could prove beneficial in comprehending this critical networking concept even better.Despite the common perception, multiple applications cannot concurrently bind to the same UDP port. Notwithstanding some edge conditions and specialized operating systems, essentially, it is an invalid scenario for two or more distinct processes to listen on the same transport protocol such as User Datagram Protocol (UDP) port.
Let’s understand how network communication occurs. When your computer communicates over a network, it employs what’s known as a socket, which acts as an endpoint of the bidirectional inter-process communication across the Internet. Here’s how you might create a socket using Python:
import socket s = socket.socket(socket.AF_INET, socket.SOCK_DGRAM)
In defining a socket like this, you’re saying you want to use the Internet Protocol (IPv4 in this case), hence the `AF_INET`. `SOCK_DGRAM` indicates that you wish to use UDP.
Following this step, binding a socket to a port is done with `bind()`:
s.bind(('localhost', 12345))
This binds your socket s to port number 12345 on localhost, indicating that the socket will listen on this specified port.
However, if a different application, say Application B, attempts to bind to the same UDP port while Application A is still actively listening on it, the operation won’t be successful. This is due to an error typically denoted as “address already in use.” Here’s a simplified depiction of this scenario:
# App A s.bind(('localhost', 12345)) # Later on, App B tries to bind to the same port>./codes.bind(('localhost', 12345)) # Raises OSError: [Errno 98] Address already in use.That said, there's still a way around this, leveraging IP multicasting methods. Multiple applications can indeed subscribe to the multicast group and listen to the same UDP port concurrently. However, please note that this is a specific use-case scenario wherein broadcasts are sent to an entire subnet set aside for multicast traffic. It isn't any typical scenario where all data packets received on a particular UDP port are processed by multiple unrelated applications.
At last, it is essential to remember that excessively sharing a UDP port is not considered efficient programming practice, as it could lead to data processing anomalies and actually complicates the networking logic than simplifying it, consequently affecting application's performance.Commonly, two applications can't listen to the same UDP port simultaneously due to an issue best described as "port contention" or "bound conflict". This occurs when multiple pieces of software are trying to bind themselves to a specific port number using the Transport Layer's network protocols, in this case, UDP (User Datagram Protocol).
Let's understand how binding works under normal circumstances. When an application wants to receive data via UDP, it binds itself to a specific port. This process is performed by making a system call to the OS's networking interfaces through programming languages like Java, Python, etc.
To implement this in Python, one might write something like:
import socket sock = socket.socket(socket.AF_INET, socket.SOCK_DGRAM) sock.bind(("localhost", 12345))In this program snippet, the socket library is used to create and bind a created socket to localhost on port 12345.
But what happens when another application wants to listen to port 12345? The second application will usually fail when it tries to bind to the port because the operating system prohibits more than one application from binding to the same UDP port at the same time. Therein lies the crux of this common issue - dual listening on the same UDP ports.
This situation often results in exceptions or errors such as `AddressAlreadyInUseException` in Java or `OSError: [Errno 98] Address already in use` in Python. Generally, these represent the reality that UDP, being a connectionless protocol, only allows exclusive binds by default.
However, it's not necessarily a hard-and-fast rule that only one application can listen on a given UDP port. Some operating systems support a feature known as SO_REUSEPORT which allows multiple sockets to bind to the exact same combination of IP address and port number, provided they all set the option before binding.
import socket sock1 = socket.socket(socket.AF_INET, socket.SOCK_DGRAM) sock1.setsockopt(socket.SOL_SOCKET, socket.SO_REUSEPORT, 1) sock1.bind(("localhost", 12345)) sock2 = socket.socket(socket.AF_INET, socket.SOCK_DGRAM) sock2.setsockopt(socket.SOL_SOCKET, socket.SO_REUSEPORT, 1) sock2.bind(("localhost", 12345))Here, we use the setsockopt method to activate the SO_REUSEPORT attribute for both sock1 and sock2 before binding, thereby permitting dual listening on the same UDP port.
Note that using SO_REUSEPORT may lead to some messy situations, particularly with respect to load balancing across different threads. Hence, its use is uncommon and generally not advisable without a compelling reason. Moreover, its effect may also vary depending upon the Kernel version or the Operating System in question.
For further details, consider exploring the following resources related to socket programming:
- Python socket programming guide for information on the Python socket library.
- Man Pages for Socket for detailed insights into the options available when dealing with network socket programming in Linux.
In the world of network programming, listening to a single UDP port from multiple applications simultaneously presents a challenge. The quintessential question is can two applications listen to the same UDP port? The straight answer is that it's not directly possible due to the way the operating schemes are designed. However, with some specific techniques and tools, this hurdle might be bypassed, allowing for a sort of simultaneous listening. These include:
1. Using a Proxy Server
A proxy server plays the role of an intermediary that receives packets on the UDP port and reroutes these packets to other ports where various applications can then listen. A packer sniffer like Wireshark can be used in such an architecture. Here's how you'd implement it in Python with a simple UDP forwarding example:
import socket localIP = "127.0.0.1" localPort = 20001 bufferSize = 1024 msgFromServer = "Hello UDP Client" bytesToSend = str.encode(msgFromServer) # Create a datagram socket UDPServerSocket = socket.socket(family=socket.AF_INET, type=socket.SOCK_DGRAM) # Bind to address and ip UDPServerSocket.bind((localIP, localPort)) print("UDP server up and listening") # Listen for incoming datagrams while(True): bytesAddressPair = UDPServerSocket.recvfrom(bufferSize) message = bytesAddressPair[0] address = bytesAddressPair[1] clientMsg = "Message from Client:{}".format(message) clientIP = "Client IP Address:{}".format(address) print(clientMsg) print(clientIP) # Sending a reply to client UDPServerSocket.sendto(bytesToSend, address)
A proxy server can, however, potentially become a single point of failure. That’s why it’s critical that its implementation has to account for fault tolerance.
2. Multicast UDP
Multicasting addresses the issue by allowing many listeners on the same multicast group address. When one sender sends a packet to the multicast group address, the networking infrastructure copies that packet to all receivers listed in the group.
import socket import struct MCAST_GRP = '224.1.1.1' MCAST_PORT = 5007 IS_ALL_GROUPS = True sock = socket.socket(socket.AF_INET, socket.SOCK_DGRAM, socket.IPPROTO_UDP) sock.setsockopt(socket.SOL_SOCKET, socket.SO_REUSEADDR, 1) if IS_ALL_GROUPS: # on this port, receives ALL multicast groups sock.bind(('', MCAST_PORT)) else: # on this port, listen ONLY to MCAST_GRP sock.bind((MCAST_GRP, MCAST_PORT)) mreq = struct.pack("4sl", socket.inet_aton(MCAST_GRP), socket.INADDR_ANY) sock.setsockopt(socket.IPPROTO_IP, socket.IP_ADD_MEMBERSHIP, mreq)
This is an efficient way to achieve multi-listener communication; however, it may not always give an ideal solution depending upon whether your use cases entirely align with broadcasting or not.
3. Network Namespaces
Another technique involves spawning different network namespaces and binding them to the same port number. It allows processes running within each namespace to bind to the same port number without conflicting with each other. This can be achieved using ip-netns tool.
Thus, while the mechanism of listening on the same UDP port by multiple applications is not natively supported, viable solutions exist to tailor-fit your use case. Remember, every alternative comes with its set of pros and cons, so dissect what you need keenly before proceeding.
Reference:
Can two applications listen to the same port?
The question taps into the fundamental understanding of how Operating Systems handle UDP network communication. To capture the complete essence, we need to break it down at a protocol level with an emphasis on User Datagram Protocol (UDP).
Understanding UDP and Network Sockets
Let's kick off with a succinct understanding of UDP first. UDP is indeed one of the core protocols of Internet Protocol suites. Applications that do not require confirmations of packet receipt often use UDP, such as Domain Name System (DNS), streaming media applications, Voice over IP (VoIP), and Trivial File Transfer Protocol (TFTP). Refer RFC 768 for detailed specifications on UDP.
In network communications, a port is an endpoint in an operating system. It can be either physical or virtual. Since this conversation leans towards application-level networking, our focus will be on the latter, i.e., virtual ports aka network sockets.
Besides, to understand if two applications can listen to the same UDP port, it's crucial to comprehend what exactly it means to "listen" to a port. An application usually listens to a port by creating a socket, binding it to an IP address and port number, plus starting a loop to check incoming messages. The following Python code provides an idea of this:
import socket sock = socket.socket(socket.AF_INET, socket.SOCK_DGRAM) address = ("127.0.0.1", 12345) sock.bind(address) while True: data, addr = sock.recvfrom(1024) print("Received message:", data, "from:", addr)
The above example binds the software-created socket to port 12345 on localhost (loopback address).
Duplicates Handling: One Socket per Application
Due to the distinctive architecture of how OS handles sockets and their bindings, technically it's unfeasible for two applications to listen to the same UDP port simultaneously. When an application cribs the control over a specific UDP port, the Operating System then prevents other apps from stealing focus on that exact port. An attempt to bind another socket to the already occupied port number would typically be repelled with a socket error.
However, some advanced mechanisms such as SO_REUSEPORT socket option pose successful workarounds for bypassing this hurdle. Introduced first in Linux kernel 3.9, SO_REUSEPORT permits multiple sockets to be bound to an identically same socket address concurrently. It’s indispensable to note that SO_REUSEPORT distributes subsequent connections evenly between the sockets bound to the same port.
Hopelessly, the effectiveness of such techniques isn't uniformly distributed across architectures, thereby nudging developers to generally dodge designs that involve duplicate listening sockets.
Unicast, Multicast, and Broadcast
Exploring from a wider lens, remember that there are different types of network traffic: Unicast, Multicast, and Broadcast. Several applications might have Multicast listeners on the same Multicast IP due to Multicast nature being 'one sender and many receivers'. Barring Unicast communications, Multicast and Broadcast allow multiple service listener logic on the same port because they diverge from normative rules - the former keeps the communication channel open for group membership, while the latter sends packets to every host in the network segment.
To conclude, I hope this probes enough light into navigable territories of the complexity involved in Operating Systems, managing UDP lifecycle, network programming, and whether two applications can listen to the same UDP port.
Yes, two or more applications can listen to the same UDP port. However, these applications must operate under specific circumstances, it is not as simple as it seems. This practice adheres to a networking concept known as multicasting.
In multicasting, numerous recipients receive data from one or many senders using the same IP address. With multicast group communication, network applications can communicate with multiple hosts (other applications) concurrently. This mode of operation permits two or more applications listening on the same UDP port.
The Socket Option: SO_REUSEADDR
When creating a socket, an application usually specifies the IP address and port number that the operating system uses as its endpoint in the network protocol stack inside the OS. Once an endpoint (IP address + port number) has been used by a process, another process cannot use it until the original process ends.
The SO_REUSEADDR socket option tells the kernel that even if this port is busy (in the TIME_WAIT state), go ahead and use it. It is a means to avoid the TCP "TIME_WAIT" state. The primary scenario occurs when you have servers that are starting up and shutting down frequently and trying to bind to the same port.
int yes = 1; if (setsockopt(sockfd, SOL_SOCKET, SO_REUSEADDR, &yes, sizeof(yes)) == -1) { perror("setsockopt"); exit(1); }
The above code snippet shows how you set the SO_REUSEADDR socket option before binding it.
A note of caution while using the SO_REUSEADDR option - care should be taken that three-way TCP/IP handshake completes before reusing the address and port of the old socket. Otherwise, packets intended for the old connection could sneak into your new connection.
Multicast groups
The multicasting behavior allows several applications to join a multicast group and receive datagrams sent to the group over some UDP ports.
struct ip_mreq mreq; mreq.imr_multiaddr.s_addr = htonl(INATDDR_ANY); mreq.imr_interface.s_addr = htonl(INADDR_ANY); if(setsockopt(socket_desc, IPPROTO_IP, IP_ADD_MEMBERSHIP, (char *)&mreq, sizeof(mreq) < 0){ perror("setsockopt"); exit(1); }
The above code snippet demonstrates joining a multicast group using set sockopt function. Upon calling this function, the kernel listens not only unicast packets destined to this socket but also multicast packets destined to the multicast group specified in mreq.
Remember, incorrect usage of these methods can lead to data leakage or unintended data communication between unrelated applications. Always consider whether or not you need multiple applications on the same UDP port before implementing this option, and consider security implications, traffic management, and scalability.
In spite of such challenges, it's fascinating to see how elements like multicasting and socket options overcome challenges and solve real-world problems in network programming using UDP sockets.In the zone of socket programming on various operating systems, comes the topic of UDP (User Datagram Protocol) and its reuse in ports. When it comes to sharing or reusing the same UDP port between two applications, we need to delve deeper and evaluate different aspects covering the notion of "Can two Applications Listen To The Same Udp Port?" Let's break it down analytically:
Socket Options:
Sockets come along with a slew of options which can be set or adjusted according to your requirements. These options allow you to manipulate the behavior of your sockets; one such socket option is
SO_REUSEADDR
or
SO_REUSEPORT
(depending on the OS).
With
SO_REUSEADDR
, you inform the kernel that even if this port is busy (in the TIME_WAIT state), go ahead and use it anyway. It's useful when your server has been shut down, and then restarted right away while sockets are still active on its port.
In case of
SO_REUSEPORT
(available in Linux since version 3.9), a server socket that wants to accept incoming connections can be run by more than one process/thread. When several threads all ask to reuse the port without requiring a client IP address for their accepts, they will all get approximately an equal proportion of the incoming requests.
The code snippet for Socket binding could as below:
// Create socket int sockfd = socket(domain, SOCK_DGRAM, 0); // Enable reuse int enable = 1; setsockopt(sockfd, SOL_SOCKET, SO_REUSEPORT, &enable, sizeof(enable)); // Bind to a specific network interface, or to any struct sockaddr_in addr; addr.sin_family = AF_INET; addr.sin_port = htons(port); addr.sin_addr.s_addr = htonl(INADDR_ANY); //or htons(ip) for a specific ip bind(sockfd, (struct sockaddr*)&addr, sizeof(addr));
Two Listening Applications Issue:
Under normal circumstances, it isn't possible for two different applications to listen on the exact UDP port at the same point in time - especially if none of them are making use of
SO_REUSEPORT
or
SO_REUSEADDR
.
Imagine having an application listening on UDP port, say 8000. If another independent application tried to listen on the exact same UDP port, a bind error would occur indicating that the port is already in use. This is due to the operating system's process-level isolation mechanism that ensures a used resource at one process cannot be inadvertently accessed by some other process.
A Use-Case Scenario:
An exception to above discussion is a scenario where both the applications are part of a collective cluster or group aiming to service a popular protocol. For instance, consider DNS which uses port 53 over UDP, under a circumstance with multiple servers providing load balancing and redundancy.
Also, such scenarios might be handled efficiently using a round robin DNS strategy or more intricate load balancing technique that directs traffic to different servers. Yet, there are circumstances where it makes sense to have multiple processes listening on the same UDP port for incoming datagrams.
Utilizing
SO_REUSEPORT
:
Going back to
SO_REUSEPORT
, non-simultaneous consent by separate applications to share the identical port can be granted by utilizing this socket option. A fantastic use-case for this option would be when performing a rolling upgrade of a service without losing any connections.
Despite these possibilities, do note that this approach may not serve well in every situation, and one must carefully consider potential security implications and practical implications of distributing incoming packets uniformly among all the listeners.
References:
For much more information about
SO_REUSEPORT
, this writeup nicely sums up its benefits and drawbacks. It truly is hard to grasp the functionality behind SO_REUSEPORT and how exactly it should be used, so even if you've read through this post, I advise going through the link to fully comprehend just what the feature does before starting to use it.
Concurrency poses a significant challenge when it comes to two applications listening on the same UDP port due to several factors:
Two Applications Unable to Bind to the Same Port
Networking protocols, like UDP, characteristically only allow an exclusive bind from one application to a definite port at a given time. This is akin to having a unique home postal address where only you are capable of receiving mail.
// Trying to run this code twice will result in an error "Address already in use" var dgram = require('dgram'); var server = dgram.createSocket('udp4'); server.bind(12345); // Attempts to bind to UDP port 12345
The error message, usually "Address already in use", signifies the incapability of two separate applications to concurrently listen for UDP packets on the identical port.
Data Integrity and Security Concerns
Allowing numerous applications to simultaneously listen to the same UDP port brings about potential data integrity and security issues. For instance, if two apps are striving to read the same data packet concurrently, it might lead to a racing condition where the order of processing cannot be ensured. Moreover, both apps receiving all incoming data would create an exploit or implicitly bypass access controls.
Interference with Communication
Pairing an IP address with its port number, creates a socket which facilitates exclusive conversation between two network devices. If more than one app were able to bind to the same port, distinguishing the rightful recipient of a returning data packet could prove complex creating interference in the communication process.
It's important to note that utilizing SO_REUSEPORT socket option available in Linux Kernel 3.9+ allows multiple sockets on the same host to bind to the same port. This capability, however, has limited practical application as it typically fragments the incoming traffic randomly between the servers. Employing a balancing mechanism that can distribute the traffic based on some rule might offer superior control. For example, via systemd's socket activation, or specific implementations within particular languages or frameworks.
However, generally, it remains advisable for each application to maintain its own exclusive UDP port for binding to affirm orderly, secure communication.Absolutely, it is common to wind up in scenarios where there might seem to be a necessity for multiple applications to listen to the same User Datagram Protocol (UDP) port. However, directly achieving this with the standard socket programming approach is not practically feasible due to the way the underlying network stack works. When a packet arrives, the operating system needs to know which application's socket to route that packet to. If multiple apps were allowed to listen on the same UDP port without any restrictions, the OS would not know which socket to forward the packet to.
Although as per the traditional practices, two different applications cannot share one UDP port simultaneously, there are a few workarounds that can help address such requirements. Here they are:
1. Employing a SuperServer
One workaround could be implementing a listening ‘SuperServer’ on the port that requires sharing. The role of this superserver would be to accept the packets via the dedicated UDP port and then re-route these packets directly to the appropriate application based on certain characteristics.
Here's a small Python code snippet to illustrate how you could implement a basic version of your 'SuperServer':
import socket # Creating a UDP server server_socket = socket.socket(socket.AF_INET, socket.SOCK_DGRAM) server_socket.bind(('localhost', 12345)) while True: data, addr = server_socket.recvfrom(1024) # Buffer size is 1024 bytes. # Code here to forward data to the appropriate application. >2. Multicasting Concept
Multicasting is another possible alternate method where each app subscribes to a multicast address rather than a specific port. This allows a form of concurrent receipt of packets from all subscribing applications. Take a look at this Python code demonstrating usage of multicast:import socket import struct multicast_group = '224.3.29.71' server_address = ('', 10000) # Creating the socket sock = socket.socket(socket.AF_INET, socket.SOCK_DGRAM) # Binding to the server address sock.bind(server_address) # Registering to the multicast group group = socket.inet_aton(multicast_group) mreq = struct.pack('4sL', group, socket.INADDR_ANY) sock.setsockopt(socket.IPPROTO_IP, socket.IP_ADD_MEMBERSHIP, mreq) while True: print('\nwaiting to receive message') data, address = sock.recvfrom(1024) print(f'received: {data} from: {address}') >Both of these methods may require changes within each application to support either rerouting or multicasting effectively. They demand alterations in the data flow amongst your applications within your architecture.
With these strategies in action, it should make the idea of integrating multiple applications to listen over a single UDP Port quite realistic.Great question! It's important to understand that in general, two applications cannot listen to the same UDP port. The reason for this is fundamentally related to how network communication operates. Each service (application) on a system has an associated port number. When data arrives at a computer through the network, the system looks at the destination port and directs that data to the appropriate service.
To share some real-world insights, think about DNS traffic, which uses UDP port 53. What would happen if two applications are both trying to interpret the information coming from one packet? Which interpretation is correct? There would be no reliable way for the operating system to know how to handle this.
Now, there are exceptions to this rule. Multicasting allows multiple programs to listen on the same UDP port number for multicast packets on the same IP address. These applications could be seen as 'sharing' the port, but they're actually each opening their own copies of the port. They don't interfere with each other due to the built-in architecture of multicast.
Here's a source code example of binding a socket in using Python's `socket` module:
import socket UDP_IP_ADDRESS = "127.0.0.1" UDP_PORT_NO = 12345 serverSock = socket.socket(socket.AF_INET, socket.SOCK_DGRAM) serverSock.bind((UDP_IP_ADDRESS, UDP_PORT_NO)) while True: data, addr = serverSock.recvfrom(1024) print ("Message received: ", data)Multiple instances of this code would not be able to run concurrently on the same machine. If you tried, you'd get a `socket.error` exception, indicating that the address was already in use.
To summarize, while it may seem theoretically possible, attempting to have two applications listen to the same UDP port would in reality cause confusion and result in unreliable behaviour. This is why the networking standards were designed to prevent such conflicts from occurring. It's always ideal to assign unique UDP ports for different applications to ensure smoother execution and efficiency.
There are workarounds, like multicasting or running your services in separate virtual machines, but these solutions come with trade-offs and complexity. For most cases, sticking to the standard one application per port paradigm will serve you better.When two applications listen to the same UDP port, several security concerns and potential risks arise. This practice can make distributed systems less secure by dealing ways to attackers to exploit UDP services. Here are some important points to consider:
Risk of Denial-of-Service attacks:
One of the main risk factors is susceptibility to DoS (Denial of Service) attacks. When multiple applications listen to the same UDP ports, they become a target for anyone looking to strain or exceed the server’s capabilities. An attacker can send a flood of packets to the shared UDP port, causing all connected applications to slow down or even crash. A typical UDP Flood attack consists of sending numerous IP packets with a forged source IP address. Because UDP is stateless, it's very difficult to prevent such attacks.
while true do echo 'ATTACK' | nc -udone Data Integrity and Confidentiality:
The more applications that listen to the same UDP port, the higher the risk of data being intercepted or manipulated. UDP being a connectionless protocol, doesn't require any handshake between the sender and receiver, which makes it more vulnerable to information sniffing or spoofing. If an application transmitting sensitive data shares the same port with another public application, attackers can potentially eavesdrop on the communication exploiting vulnerabilities in the latter.
Multiple Socket Creation Errors:
Often in situations where multiple applications listen on the same UDP port, errors can occur during socket creation and binding process. It becomes especially problematic when each application on a UDP port uses its proprietary protocols/functions for socket creation, data transmission, and reception.
In programming terms, if two applications try to bind to the same port without the option
(SO_REUSEPORT), one application will get a
bind: address already in useerror.
// Programming socket in C int sockfd; struct sockaddr_in servaddr; sockfd = socket(AF_INET, SOCK_DGRAM, 0); if (sockfd < 0) { perror("Socket creation failed"); return EXIT_FAILURE; }servaddr.sin_family = AF_INET; servaddr.sin_addr.s_addr = INADDR_ANY; servaddr.sin_port = htons(port); if (bind(sockfd, (const struct sockaddr *)&servaddr, sizeof(servaddr)) < 0) { perror("Bind failed"); close(sockfd); return EXIT_FAILURE; }To ensure that your application stays securely isolated and keep the inherent risks at bay, you should:
• Limit the sharing of UDP ports among applications as much as possible.
• Regularly audit and review the applications that are listening on UDP ports, paying special attention to those sharing the same port(s).
• Implement strict firewall rules to block unsolicited incoming traffic.
• Use UDP services that have built-in protection against various types of attacks.
• Consider utilizing encryption protocols (like DTLS) that provide confidentiality and authentication over UDP.Choosing the right strategy depends largely on your specific networking environment and security requirements. For a comprehensive understanding on network socket programming and security measures, [Computer Networks: A Systems Approach](https://www.amazon.com/Computer-Networks-Systems-Approach-Kaufmann/dp/0123850592) offers a comprehensive guide.There is a common misconception that, in TCP/UDP socket programming, one UDP port can be listened to by only one application. However, let me make it clear here: multiple applications can listen to the same UDP port if designed and administered effectively. This process involves the concept of Multicast UDP.
What is Multicast UDP?
Unicast is the term used when a single sender sends data to a single receiver. Broadcast refers to transmitting data from one terminal to all others. But when data is sent from one point to several terminals, this process called multicasting. By drawing from this, Multicast UDP means transmission of a packet, including messages or block of text, to multiple users/hosts at the very same time.How does it Work?
Multicasting UDP allows different applications to share the same UDP port, but not in the traditional sense. Rather than directly sharing, these various apps join a multicast group identified by the same multicast IP. When a member of this group sends data to the multicast IP, every other application part of the same group receives it.Let's provide an example using Python's socket library for better comprehension:
import socket import struct MCAST_GRP = '224.1.1.1' MCAST_PORT = 5007 sock = socket.socket(socket.AF_INET, socket.SOCK_DGRAM, socket.IPPROTO_UDP) sock.setsockopt(socket.SOL_SOCKET, socket.SO_REUSEADDR, 1) sock.bind((MCAST_GRP, MCAST_PORT)) mreq = struct.pack("4sl", socket.inet_aton(MCAST_GRP), socket.INADDR_ANY) sock.setsockopt(socket.IPPROTO_IP, socket.IP_ADD_MEMBERSHIP, mreq)In our above code, we have set the MCAST_GRP (multicast group) parameter with '224.1.1.1'. The 'socket.SO_REUSEADDR' option allows reuse of local addresses. This is crucial as it permits multiple applications to bind the same address and port number.
Note that multicast IPs range from 224.0.0.0 to 239.255.255.255 (source).
Downsides to UDP Port Sharing
A caveat to keep in mind, though, is managing possible performance issues due to data contention. Data arriving at a shared UDP port will arrive as a stream. If multiple apps are listening and retrieving data, coordination is necessary to avoid data loss.
Another hurdle might be firewall settings. They could disallow incoming connections on the select UDP port and would need adjustments accordingly.
So, while some care needs taken with structure and network security, advanced TCP/UDP socket programming techniques like Multicast UDP facilitate the process of allowing multiple applications to listen to the same UDP port.Yes, Kernel modules can intercept network traffic. This capability can be particularly helpful when multiple applications are trying to listen to the same UDP port. Let's understand how this process works.
Kernel Modules and Network Traffic
To comprehend the role of a Kernel module in network communication, one must understand networking itself. When a network packet enters a system, the source IP and the destination ports of the packet are checked. After this check, the appropriate application needed to handle that packet is determined.This is where a Kernel module comes into the picture. The Kernel has a mechanism whereby it can load and unload modules on demand, such as device drivers or file systems. A kernel module enables you to extend the functionality of the kernel without rebooting the system stackoverflow.com: Difference between kernel module and kernel driver?.
A Kernel module can run within the network stack which can analyze the network traffic (
nf_register_hookis often used for this). It has the capacity to catch packets with very minimal modification. For example:
struct nf_hook_ops { struct list_head list; /* User fills in from here down. */ nf_hookfn *hook; struct net_device *dev; // Network device packets came from int pf; // Packet family (ETH_P_IP, etc) int hooknum; // When to call the function (NF_IP_PRE_ROUTING, etc) /* Hooks are ordered in ascending priority. */ int priority; };The structure above describes certain network hooks available to the Kernel modules that can act on different stages of packet flow inside the Kernel.
Handling UDP Packets
With regard to handling UDP packets, technically, only one software can actively listen to a specific UDP port at a time. From a raw access perspective, it would not be possible for two different applications to directly open and listen on the same UDP port. If an attempt is made to assign the same UDP port to another program or process while it’s already assigned to a program, you will typically receive an error message such as "address already in use" or "socket binding failed".Data Duplication through Kernel Module
To allow multiple applications to listen to the same UDP stream, we need to create a custom Kernel module. Essentially, what you would do is create a module that makes a copy of the incoming packet and then sends a duplicate packet to another UDP port. So, your first application would listen on the original port, and your second application would listen on the secondary port that you set up to receive the copied data. It should be noted, however, that creating such a module is non-trivial and any error in the code could lead to security vulnerabilities or system crashes.Use of In-Kernel Solutions Like IPTABLES and TEE Target
If possible, it would always be preferable to use in-kernel solutions like IPTABLES and TEE target to avoid the complexity of writing Kernel modules. This way you avoid many potential pitfalls associated with custom Kernel programming, and you also take advantage of the extensive testing and development effort that has been put into these mature and robust frameworks over the years.To summarize, a Kernel module can intercept network traffic and effectively allow multiple applications to listen to the same UDP port via data duplication. But always remember, creating custom Kernel modules involves considerable complexity and risks, so it's often preferable to use in-kernel solutions wherever and whenever possible.
Code Source:
elixir.bootlin.com: netfilter_ipv4.h codeNo, two applications can't listen to the same UDP (User Datagram Protocol) port. This situation is sought to be avoided by leveraging a mechanism called Socket binding, where each application binds itself to a unique port number.Node.js example: const dgram = require('dgram'); const server = dgram.createSocket('udp4'); server.on('error', (err) => { console.log(`server error:\n${err.stack}`); server.close(); }); server.on('message', (msg, rinfo) => { console.log(`server got: ${msg} from ${rinfo.address}:${rinfo.port}`); }); server.on('listening', () => { var address = server.address(); console.log(`server listening ${address.address}:${address.port}`); }); server.bind(41234); // Throws error when trying to bind to the same port const anotherServer = dgram.createSocket('udp4'); anotherServer.bind(41234); // Error: bind EADDRINUSE 0.0.0.0:41234A socket in programming refers to a software endpoint that allows bidirectional communication between a client and a server. When an application wants to listen for network traffic using a specific protocol, whether it's UDP or TCP (Transmission Control Protocol), it creates a socket and binds it to a port number - this is a number in the range of 0-65535 designated by the OS (Operating System).
Below are a few relevant conditions:
- An ephemeral port (range of 49152–65535, this varies with different systems) is assigned when a socket initiates a connection to a server. These are temporary and released when the connection ends.
- A server listening to incoming connections will use a known well-defined port (like HTTP servers typically use port 80).
- UDP doesn't provide the in-built session tracking ability of TCP.
When two applications try to bind to the same port, an 'Address already in use' error is generally thrown by the OS. It's because listening to the same port can create security risks and data confusion issues. Therefore, having one port being utilized by one application at a time prevents such issues from occurring. Advanced techniques like SO_REUSEPORT or fork system call clone (in Linux) allow multiple sockets on the same port under certain strict conditions.
Oracle's documentation on Sockets takes a deeper dive into the mechanisms involved in socket programming, which gives a better grasp of how applications communicate effectively without creating overlap or conflicts.
Remember though, in the broader perspective of networking, data communication involves more than just sockets and ports. IP addresses, Network Interfaces, and Routing Tables also play critical roles.