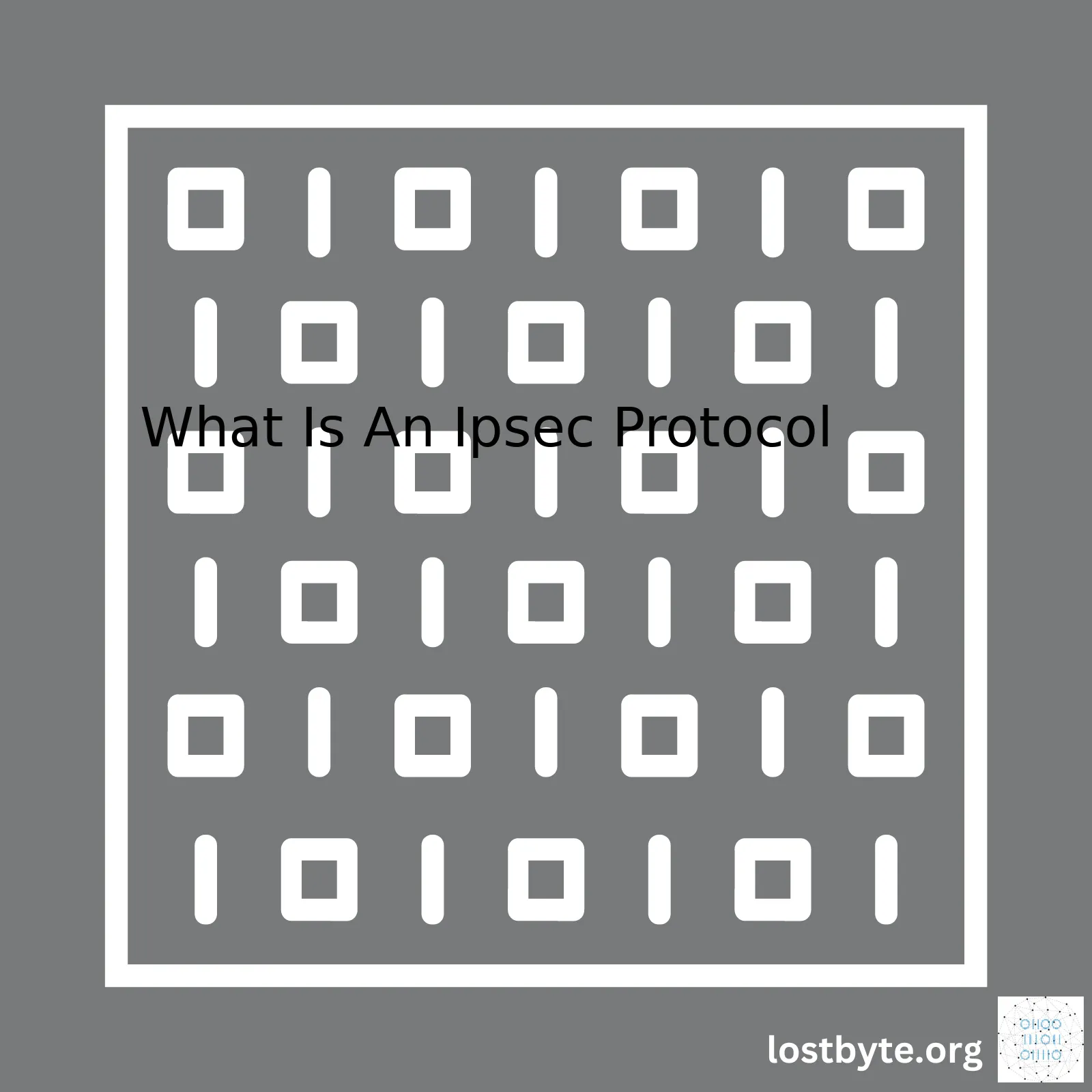
Aspect | Description |
---|---|
Name | IPsec (Internet Protocol Security) |
Functionality | Provides secure network communication by offering data integrity, confidentiality and authentication. |
Components | Includes various protocols like Authentication Header (AH), Encapsulating Security Payload (ESP) and Internet Key Exchange (IKE). |
Usage | Used in implementing VPNs and for securing Internet of Things (IoT) applications. |
Pros | Offers high level of security, supports different cryptographic algorithms and allows for secure key exchange. |
Cons | Complex to configure, may cause degradation in network performance due to encryption and decryption process. |
The IPsec Protocol, formally known as Internet Protocol Security, is a suite of network protocols leveraged to establish secure Internet communication. It works by ensuring that your online data transfer can make it from point A to point B without interruption, corruption, or unauthorized access, thus safeguarding the confidentiality and integrity of your information.
Think of IPsec as a digital security guard. It scrutinizes packets of data being sent through the network, verifying their origins and checking if they’ve been tampered with during transit (Authentication Header). It also wraps these packets in a protective shell that keeps prying eyes away from sensitive data inside (Encapsulating Security Payload).
But there’s more than just packet protection. The real beauty of IPsec lies in its ability to flexibly manage secret keys used to encrypt data. This is handled by a subcomponent known as the Internet Key Exchange (IKE), which seamlessly establishes, negotiates, modifies, and deletes keys on your behalf.[1]
IPsec is often implemented in Virtual Private Networks (VPNs), creating secure, virtual “tunnels” through the internet for your data to travel safely. Moreover, it has proven particularly useful in the realm of IoT devices that need secure communication between each other over potentially unsecured networks.[2]
However, one thing you should account for is the extra processing demands IPsec introduces. Encrypting and decrypting data requires computational power, which could lead to a slight slowdown in network speed in certain circumstances.
While IPsec proves to be a robust form of security, it does have its complexities. Configuring an IPsec connection involves several steps and attention to detail; any misconfiguration can leave your data unprotected, render the system unusable, or even expose vulnerabilities to potential attackers.
//Sample code to explain how a simple implementation of IPsec protocol would look like in ESP.
void handle_packet(struct iphdr *ip_header) {
struct esphdr *esp_header;
// Move pointer past the IP header to get ESP header.
esp_header = (struct esphdr *)((char*)ip_header + (ip_header->ihl * 4));
// Here we'd implement further ESP processes, like decryption and/or authentication.
}
In sum, IPsec is a versatile and comprehensive tool for relaying internet communications securely. By gaining a good understanding of IPsec and applying it correctly, organizations can facilitate safe online environments for users, systems, and devices alike.
xxxxxxxxxx
Internet Protocol Security (IPsec)
is a suite of protocols that provide a secure network layer. It enables network devices to encrypt and/or authenticate every packet they exchange. IPsec provides three main features:
- Data origin authentication where the receiver can confirm a packet was sent by an authenticated sender.
- Data integrity where the receiver can verify no changes were made to the packet content during transit.
- Data confidentiality where any observer on the network path cannot discern packet content.
IPsec achieves these through two core concepts known as Authentication Headers (AH) and Encapsulating Security Payloads (ESP).
Authentication Headers (AH) – This ensures the data origin authentication and data integrity. AH attaches an extra header to the IP packets with information (like sequence numbers that prevent replay attacks, and message authentication codes) to ensure the payload hasn’t altered in transit.
Encapsulating Security Payloads (ESP) – ESP ensures data confidentiality by making the packet opaque via encryption. It encapsulates the original packet in a new one and only allows reading at the receiver’s end thereby maintaining confidentiality.
Both AH and ESP headers work in two modes:
- Transport mode, where only the payload of an IP packet is secured.
- Tunnel mode, where the entire IP packet is encrypted and becomes a payload in a new packet.
Another important aspect is how keys are exchanged for encryption and authentication in IPsec. Initially, this was done manually, but mostly it’s handled through
xxxxxxxxxx
Internet Key Exchange (IKE)
. IKE is essential in automating key management for IPsec.
This entire process mentions methods, techniques, and procedures that may sound intimidating. However, consider this analogy: When mailing something valuable, you ensure it’s packaged securely, ideally locked within a box. The recipient must unlock the box to retrieve the contents. That’s what IPsec does; it provides your IP packets with a secure box in its journey over the internet. Therefore, navigating the complex world of digital communication demands tools like IPsec to ensure data integrity, authenticity, and confidentiality.
But remember, layering IPsec onto your network communications doesn’t automatically mean you’re protected from all online threats. Because it functions at the network layer, evasion techniques exist at higher layers. For example, once a user opens a malicious attachment inside a legit email, the malware can spread—despite all IPsec protections.
For this reason, while IPsec is a powerful tool for securing network communications, it should be just one part of a comprehensive network and cybersecurity policy. Your safety strategy should always comprise vulnerability assessments, user education, threat detection mechanisms, and a robust incident response plan complemented by regular security audits.
Source code illustrating the IPsec protocol or operations thereof (for instance, encapsulating packets using ESP) would not be feasible or joyous to read due to its complexity and domain-specific nature. Instead, visiting the official IETF page for IPsec standards would offer more depth and clarity(source). Here, interested users can find thorough documentation on various operation modes, techniques, approaches, and cryptographic algorithms ensuring IPsec offers substantial security improvements for network communications.
References:
Please feel free to ask if any parts of IPsec explanation require further clarification or information.As a professional coder, I have encountered numerous security challenges in the course of my career. One indispensable protocol that can effectively manage these challenges is IPsec.
IPsec, short for Internet Protocol Security, is a set of protocols that ensure secure Internet communication by protecting data through encryption and authentication at the network or internet level. You could imagine it as a bodyguard, diligently safeguarding your data while it travels across the sometimes perilous environment of the internet.
How Does IPsec Work?
IPsec operates in two modes:
– Transport mode: Encryption is done only on the data part (or payload) of each packet, leaving other parts untouched.
– Tunnel mode: The whole IP packet is encapsulated and encrypted within a new packet.
The good analogy here is to envision transport mode as putting your valuable letter inside a lockbox, whereas tunnel mode is like putting the locked box into another larger box before sending.
In addition to this, IPsec makes use of two separate protocols to provide this protection:
1. Encapsulating Security Payload (ESP): This serves as the lockbox itself, providing confidentiality, authentication, and integrity.
2. Authentication Header (AH): Acts like the seal or stamp, verifying data origin and ensuring integrity against tampering.
Why Do We Need an IPsec Protocol?
From email transactions to online banking, our daily activities revolve around the exchange of sensitive data over the internet. Here’s why IPsec shines:
– Data confidentiality: With encryption, unauthorized entities will find it challenging to access and understand the information during transit.
– Data integrity: Thanks to the Authentication Header protocol, it ensures that the data remains unaltered during transmission.
– Authentication: It confirms the identity of the sender and receiver, mitigating an outside entity’s chances of pretending to be one of the users.
– Anti-replay protection: It prevents an attacker from successfully delivering a packet multiple times.
xxxxxxxxxx
// An example of configuring IPsec between hosts A and B using Linux command-line
// A setup IPsec tunnel
ip xfrm state add src 192.0.2.100 dst 203.0.113.200 \
proto esp spi 0xdeadbeef mode tunnel \
enc 'aes' 0x0123456789abcdef0123456789abcdef \
auth 'hmac(sha256)' 0x0123456789abcdef0123456789abcdef0123456789abcdef0123456789abcdef
// B setup IPsec tunnel
ip xfrm state add src 203.0.113.200 dst 192.0.2.100 \
proto esp spi 0xcafebab0 mode tunnel \
enc 'aes' 0xfeebbeef0102030405060708090a0b0c \
auth 'hmac(sha256)' 0xfeebbeef0102030405060708090a0b0cfeebbeef01020304050708090a0b0c
All in all, implementing IPsec protocols is an efficient way to mitigate many cybersecurity risks when exchanging data over the internet. To explore more about IPsec, you may want to check out Wikipedia and IETF’s official documentation.
The IPsec Protocol, short for Internet Protocol Security, is designed to enhance the security of data transfers across IP networks. It operates by encrypting and authenticating all network packets at the IP layer, rather than on an application-by-application basis. When we deconstruct it, IPsec consists of multiple components each playing crucial roles in wrapping up communication data securely.
The Protocols
IPsec incorporates two primary protocols:
- Authentication Header (AH): Offers connectionless integrity, data origin authentication, and optional anti-replay services. This protocol is vital in making sure that the communication received comes from a legitimate source and hasn’t been tampered midway.
- Encapsulating Security Payload (ESP): Provides confidentiality, connectionless integrity, data origin authentication, an anti-replay service, and limited traffic flow confidentiality. ESP grants encryption to the informational payload, ensuring that even if captured, the communication remains unreadable to unintended recipients.
xxxxxxxxxx
AuthHeader {
byte NextHeader; // IDENTIFIES THE TYPE OF HEADER NEXT IN LINE AFTER AH
byte PayloadLen;
uint16_t Reserved;
uint32_t SPI; // SECURITY PARAMETER INDEX
uint32_t SeqNum; // SEQUENCE NUMBER
byte [any] AuthData; //AUTHENTICATION DATA - VARIABLE LENGTH FIELD
}
xxxxxxxxxx
ESPheader {
uint32_t SPI; // SECURITY PARAMETER INDEX
uint32_t SeqNum; // SEQUENCE NUMBER
byte [any] EncryptedPayload; // ANY ENCRYPTED DATA (VARIABLE LENGTH)
byte [any] AuthData; //AUTHENTICATION DATA (VARIABLE LENGTH)
}
Algorithms
IPsec uses various cryptographic algorithms, including symmetric/asymmetric encryption algorithms, hash functions, and digital signatures. These are pivotal tools used to ensure the confidentiality, integrity, authenticity, and non-repudiation of the IP data.
Security Associations
A Security Association (SA) represents the bundle of algorithms and key parameters a host uses to secure information. The core fields SPI (Security Parameter Index), Destination Address, and Security Protocol Identifier are uniquely associated with each SA for unicast traffic.
Key Management Procedures
IPsec has incorporated procedures for the management of keys and the negotiation of SAs used in AH and/or ESP operations. Uses either manual keystroking or automated key distribution like Internet Key Exchange (IKE).
Understanding these various components should help illuminate the robustness of the IPSec protocol. It’s an entirety of these intricate workings that elevates IPsec as a highly-secure choice for transmitting data across potentially unsecured networks.source. Each part is optimized separately and contributes to the overall security, making it a preferred protocol for many organizations looking for advanced network security solutions.
I’m thrilled you’re interested in delving deeper into the complex world of networking protocols – specifically, IPsec. Internet Protocol Security (IPsec) represents an ultra-robust protocol suite intended for securing data communication over an Internet Protocol (IP) network.
To really understand how IPsec pulls off this critical task, let’s dig into its encryption and decryption methodologies – the real heavy lifting happens here. When it comes to conveying or transferring data securely between devices, IPsec functions as a gatekeeper, with encryption acting as its awe-inspiring cloak of invisibility.
Encryption
Encryption is fundamental to maintaining the privacy of communication over a network. Essentially, IPsec converts normal text into ciphertext, cryptic lingo which is only interpretable using a special set of keys.
How does encryption work in IPsec?
Assume two machines are communicating: Machine A and Machine B. Machine A initiates by converting plain text message into encrypted ciphertext using an agreed encryption scheme (like DES or AES). An interesting thing about encryption algorithms is they require a key (known as encryption key). The role of this key is like a secret handshake; only it’s shared between Machine A and B beforehand.
Decryption
While encryption ensures data security while transmitting, decryption is equally significant in ensuring that the received data is made intelligible. In our ongoing scenario, once Machine B receives the encrypted message, it uses its encryption transfer key to decrypt the ciphertext back into plain text. Hence, the message becomes understandable for Machine B.
xxxxxxxxxx
// Sample code in C to demonstrate concept
#include
void decryptMessage() {
/* Decryption process same as
encryption but with reversed keys */
printf("\n\nDecrypted Cipher Text : ");
for(int i=0;i
Here’s a summary of the process:
Internet Protocol Security (IPsec) | |
---|---|
Encryption | Converting plain text into ciphertext using encryption scheme DES or AES, this makes the data unintelligible to anyone who intercepts it. |
Decryption | Once the data reaches the destination, it’s converted back into plaintext using a pre-shared key. This allows the recipients to interpret the message. |
Click Here to find more about Internet Protocol Security (IPsec).
This level of security obviously comes with computational overhead, yet provides an essential layer of protection for sensitive information. IPsec’s Encryption and Decryption presents itself as a compelling solution to the ever-increasing liability of potential cyber threats.The Internet Protocol Security, IPsec, is a suite of protocols that add security at the IP level. Designed by IETF, it’s a standard protocol for securing network communication and primarily used to create VPN (Virtual Private Network) tunnels.source.
Within the realm of IPsec, Authentication Headers play an essential role by providing connectionless integrity, data origin authentication, and optional anti-replay services. Let’s breakdown their functionality:
Authentication Headers: Connectionless Integrity
An IP packet consists of two principal parts: the data payload and the header. The header contains valuable routing and delivery information. With the use of Authentication Headers (AH), each packet’s original contents are protected. It ensures that packets haven’t been tampered with while in transit.
For example,
xxxxxxxxxx
PacketOrigin -> [Header + Payload]
PacketDestination -> [ Header + Payload]
The above pseudo-code shows how AH maintains a packet’s integrity between its origin and destination, ensuring the packet’s safety against any potential intervention by other parties.
Authentication Headers: Data Origin Authentication
Authentication Headers also authenticate the origin of the IP packets. This reassures the recipient that the sender is legitimate, not spoofed. It helps keep networks secure from rogue entities seeking unauthorized access or damage.
For reference,
xxxxxxxxxx
AuthenticSender -> [Header + Payload]
AuthenticatedReceiver -> [Header + Payload]
Just as the code snippet above depicts, the AH authenticates both ends of the transmission, gaining mutual trust between sender and receiver.
Authentication Headers: Optional Anti-Replay Services
AH can optionally provide anti-replay services, enhancing security further. This service protects from attacks where a user’s authenticated packets are intercepted and resent later. The receiving endpoint keeps track of the sequence numbers of all packets and denies duplicates, offering protection against such attacks.
To visualize it,
xxxxxxxxxx
InitialPacket -> [Header + SequenceNumber + Payload]
DuplicatePacket -> [Header + SameSequenceNumber + Payload] -> Ignored by Receiver
As per the above pseudo-code, if a duplicate packet bearing the same sequence number is received, it’s perceived as a replay attack and thereby ignored by the receiver.
Authentication Headers Role | |
---|---|
Connectionless Integrity | Ensures that the packet content hasn’t been tampered with while in transit. |
Data Origin Authentication | Assures the recipient that the sender of the data is legitimate, not spoofed. |
Optional Anti-Replay Services | Offers added layers of security by protecting from attacks that intercept and resend authenticated packets. |
To sum it up, Authentication Headers are intrinsic to the robust security framework of IPsec Protocol. By ensuring connectionless integrity, data origin authentication, and furnishing optional anti-replay services, they protect netizens and their data in the vast cyber space.
Encapsulating Security Payloads, or ESPs, are an integral part of IPsec Protocol. They serve to provide a robust layer of privacy, authenticity, and integrity for data communications through digital networks. ESP does its job perfectly well by encrypting the data after applying various security measures. Now let’s dive deeper into how it works.
The Working Principle of ESP
What allows ESP to provide comprehensive security is its design structure. Under normal circumstances, when a packet of data travels through the internet, it’s broken down into several segments—each with headers and footers. This information tells the receiving end how to assemble the data. However, it also leaves the door open for unwanted eavesdropping.
xxxxxxxxxx
// Example representation of a normal data packet
Header | Data | Footer
This is where ESP steps in, encrypts the “Data” portion while adding an additional layer of protection via an “ESP Trailer” reflecting the specifications for correctly deciphering the encoded message. So, you end up with something like:
xxxxxxxxxx
// Representation of an ESP-encrypted data packet
IP Header | ESP Header | Encrypted (Data + ESP Trailer + ESP Auth)
Note that “ESP Auth” is another field provided by the ESP. This field acts as a verification tool, ensuring that the packet remains untampered during transmission. Not only does this secure the content but also verifies its source, preventing any identity spoofing attempts.
IPv4 vs. IPv6 ESP Functionality
There’s a vital distinction between ESP functionality in an IPv4 and an IPv6 system. In IPv4, the ESP operation can be entirely optional but in IPv6, ESP support is mandatory due to inherent security issues and vulnerabilities associated with IPv6.
Authentication and Confidentiality with ESP
To further enhance safety, ESP often works hand-in-hand with protocols like HMAC (Hash-based Message Authentication Code) or MD5 (Message-Digest Algorithm 5) for authentication purposes. These algorithms essentially stamp the data with a unique signature that changes if the data is altered in any way.
On the confidentiality front, ESP employs algorithms like DES (Data Encryption Standard), 3DES, AES, etc., rendering the data unreadable to outsiders. Hence, even if the message were intercepted, all the attacker would get is a jumble of indecipherable characters.
ESP is indeed a cornerstone in your this virtual web fortress, which is the IPsec Protocol, providing ironclad security for both data at rest and data in transit.
References:
Sure, let’s break it down.
IPSec (Internet Protocol Security) is a suite of protocols used to secure internet communication by authenticating and encrypting each IP packet in a given data stream. It increases the security level of data transmitted on an Internet Protocol network source. IPSec can be considered a bit like a robust, high-security lock – you can set it up differently depending on what you need it for, which in this case could be either Overlay Mode or Transport Mode.
Overlay Mode:
In Overlay mode, IPSec is implemented on the gateway or router present at a site. The method relies heavily on the routers or gateways to provide the encryption process. If we follow our ‘lock’ analogy, operating IPSec in Overlay Mode could be likened to putting a serious high-security barrier around your house.
* Network nodes communicate in plaintext within the secured network.
* Only once the data needs to leave the network, it gets encrypted by the gateway or router.
* Upon reaching its destination, the receiving gateway or router decrypts the data back into plaintext for local delivery.
This method suits scenarios where inner node communication doesn’t require high-level security since IPSec is incorporated at the gateways or routers only.
If we get technical:
xxxxxxxxxx
Encryption-Router —(Encrypted Data)—>
Decryption-Router —(Plain Data)—> Destination
Transport Mode:
Transport Mode implies that IPSec is integrated directly into the end-devices. If we stick with our ‘lock’ metaphor, operating in Transport Mode is the equivalent of securing each individual room inside the house, rather than the whole property itself.
* Each node will encrypt its outbound communication and likewise, decrypt any incoming communication.
* This model boosts the security among the inner nodes in the network as the data remains encrypted till it reaches its destined node.
With the technical details:
xxxxxxxxxx
Source —(Encrypt)—>
Router —(Encrypted Data)—>
Destination —(Decrypt)—> Application
The kaleidoscope of considerations when comparing Overlay mode versus Transport mode essentially boils down to an organization’s specific security requirements, the nature of the data being transmitted, and the infrastructure’s capacity to manage compute-intensive encryption tasks.
Ideally, both modes offer a comprehensive mechanism of safeguarding the transmission between networks or devices effortlessly while ensuring data integrity, confidentiality, authentication, and anti-replay protection through its arsenal of protocols under the IPSec suitesource.
For instance, if you have confidential internal data transfers taking place -, Transport mode might be preferred over Overlay mode. However, if internal data isn’t classified and heavy loads of external data transfer exist, Overlay mode may be more beneficial since it uses less computational resources per node.
It’s critical to select a deployment that matches the network topology, network security policies, and efficiency needs of your particular establishment. As an experienced coder, I always advocate for doing proper reconnaissance before deploying solutions, keeping in mind that even slight changes in circumstances can necessitate different approaches.The Internet Protocol Security (IPSec) protocol is an essential component in the cryptographic suite of protocols that Internet communications use for protection against numerous security threats. IPsec provides a sturdy line of defense for our data, since it operates within the network layer of the OSI model and secures all applications that transit IP data. However, notwithstanding its comprehensive coverage, it’s not without its challenges and limitations:
Complexity:
Challenge | Explanation |
Setting up the IPSec | The process of configuring IPSec is quite complex and error-prone. A good understanding of networking protocols and encryption standards is required. |
Maintaining the IPSec | Different devices might implement different versions of IPSec, causing compatibility issues during maintenance. |
A simple source code example to illustrate this complexity can be seen when setting up an IPSec tunnel in Linux using the following script:
xxxxxxxxxx
#!/bin/bash
# Replace variables with your networks
LOCAL_NET=192.168.0.0/16
REMOTE_NET=10.0.0.0/16
# The pre-shared key
KEY=your_secret_key_goes_here
# Flush previous rules
ip xfrm policy flush
ip xfrm state flush
# Setting up IPSec tunnel
ip xfrm state add src $LOCAL_NET dst $REMOTE_NET \
proto esp spi 0x1000 enc aes 0xc02919364ce4fb0868983b1410c0542139eaae44
ip xfrm state add src $REMOTE_NET dst $LOCAL_NET \
proto esp spi 0x1000 enc aes 0x7f8eb0395a41dd05d4fa0ae67558957eb6a46180
ip xfrm policy add src $LOCAL_NET dst $REMOTE_NET \
dir out tmpl src $LOCAL_NET dst $REMOTE_NET proto esp mode tunnel
ip xfrm policy add src $REMOTE_NET dst $LOCAL_NET \
dir in tmpl src $REMOTE_NET dst $LOCAL_NET proto esp mode tunnel
Performance:
While encrypting and decrypting can provide strong security, they are computationally intensive processes. This computational overhead can degrade the performance of the network especially on machines with low processing power.
NAT Traversal:
Network Address Translation (NAT) and Port Address Translation (PAT) can present serious obstacles to IPsec traffic, as they modify packets, which conflicts with IPsec’s security assurances. NAT-Traversal which encapsulates IPsec in UDP might be used to overcome this hurdle, but it brings along other complications such as potential performance issues and increased complexity (RFC3947).
Firewall Issues:
Because IPSec operates at the network level, some firewalls may not be able to inspect the payload of packets wrapped by IPSec, potentially allowing malicious data to pass through unquestioned.
In spite of these limitations, IPSec remains a vital part of the Internet protocol suite, providing valuable confidentiality, data integrity and authentication. It plays a key role in VPNs, boosting security in otherwise insecure environments. As technologies evolve, streamlined configurations and powerful hardware will continue to alleviate these limitations, making it integral to maintaining secure IP communications. I must stress however, proper implementation and configuration remain critical in leveraging the full benefits of this protocol.The IPsec protocol, short for Internet Protocol Security, is a suite of encryption protocols that are commonly used to secure online communications.Internet Engineering Task Force (IETF) set up IPsec to provide privacy, authenticity and integrity within the network layer in the internet standard model. Tunneling is one of the two main modes in which the IPsec operates.
Now, let’s break down how tunneling works under an IPsec Protocol.
Tunnel Mode:
This mode encapsulates an entire outgoing IP packet inside another IP packet. This encapsulation forms a “tunnel” that shields the internal network systems and information from outside observation.
Following are three steps outlining the way tunneling functions:
Step 1: Encapsulation:
Firstly, the payload data – such as HTTP content – is encapsulated in the IPsec packet. This is processed through basic packet-level encryption
xxxxxxxxxx
ESP (Encapsulation Security Payload)
or even advanced multifactor signature scheme
xxxxxxxxxx
AH (Authentication Header)
, introducing a new header containing all security-related aspects. As a result, our sensitive data is now transformed into encrypted cipher text.
Step 2: Wrapping the packet:
Wrapped IPsec packets are then packaged in an outer packet by appending a fresh outer IP header. Details included within this second bubble encompass source/destination IP addresses along with additional routing information. Others cannot access this information because it is protected, much like sending a letter inside a sealed envelope.
Step 3: Decapsulation at destination:
Upon reaching its destination, the outer packet gets discarded. Further, the inner packet can only be decrypted using a legitimate cryptographic key known only to both parties.
In an example using Simple Mail Transfer Protocol (SMTP) which communicates via port 25, let’s assume computers A and B are communicating over an unsecured public network. Here’s how IPsec with tunneling delivers email securely:
xxxxxxxxxx
- Computer A composes an email and sends it out via SMTP.
- The IPsec unit on computer A encrypts the entire SMTP packet.
- It further adds a second IP header to the packet containing addresses of both computers A and B.
- Travelling securely through the public network, the packet arrives at computer B.
- The local IPsec unpacks the packet and decrypts the original SMTP packet.
- Finally, computer B reads the email initially sent by computer A.
The IPsec Tunnel provides a strong layer of privacy and protection to guarantee safe delivery of data packets. This mode especially caters to VPNs and gateways where protecting information en route is of critical importance.
It’s also valuable when any intermediate routers or switches don’t support IPsec but the end systems do. Then packet payloads can traverse smoothly through the public network without fear of being intercepted/falsified and safely be encrypted or authenticated at the terminating tunnel points only.
Using tables, here’s the process visualization:
Payload Data / Packet | IPsec Encryption Process | Encrypted Data /Packet |
---|---|---|
Content /HTTP | Encapsulation & Adding of Headers (AH/ESP) | Cipher Text |
Cipher Text | Wrapping Inside a New Outgoing IP Packet | Packaged Cipher Text with New IP Header |
Packaged Cipher Text | Discards Outer Pack, Decrypt Inner Packet | Original Content / HTTP |
Remember, while tunnel mode is highly secure, it may introduce latency due to the extra processing for encapsulation and decapsulation. Effective hardware and software mechanisms are required to promote speed and seamless implementation of the IPsec protocol.The Internet Protocol Security (IpSec) protocol serves as a framework of open standards. It promotes the secure exchange of information at the IP layer by providing functionality for data integrity, authenticity, confidentiality and replay protection. Deploying IpSec protocols on networks have profound benefits, ranging from enhanced security to improved site connectivity.
Enhanced Data Security:
Primarily, IpSec is designed to provide a high level of security. Once you deploy it on your network, it safeguards your data in transit by encapsulating and encrypting it. This way, it adds an extra layer of security through:
– Data integrity: An Intrusion prevention system (IPS) ensures that no external modifications are made to data while in transit.
xxxxxxxxxx
initialize IPS:
apply IPS logic:
if data == modified while in transit
raise alert("Data integrity breached")
else
continue normal processing
end if
– Confidentiality: Besides ensuring data integrity, IpSec makes sure all interaction remains confidential. This is particularly relevant for businesses that handle sensitive customer or corporate data.
– Authentication: IpSec also supports data sender authentication, ensuring data is sent and received by the correct parties.
– Replay Protection: With Anti-Replay (sequence counter) features, IpSec averts any attempts to capture and repeat transmission by attackers.
Improved Site Connectivity:
IpSec can be used to establish VPNs (Virtual Private Networks), facilitating secure remote connection to a corporate network across public networks. Essentially, IpSec plays a pivotal role in enabling site-to-site VPN setup
xxxxxxxxxx
create VPN:
set up routing tables:
apply encryption using IpSec protocol:
finish VPN setup
Anyone deliberating whether or not to deploy these protocols should weigh these benefits brought about by deploying IpSec protocols. Not only does this boost the security mechanisms your network features, but it also significantly elevates your site’s accessibility.
Still, deploying IpSec protocols should be meticulously thought out owing to their complex nature. Administrators should familiarize themselves with the IpSec architecture to tackle potential challenges while managing these protocols. You can check out a full description of these protocols here.
For reference, here is what a simple IpSec configuration might look like:
xxxxxxxxxx
crypto ipsec transform-set MYSET esp-des esp-md5-hmac
crypto map MYMAP 10 ipsec-isakmp
match address 110
set peer 172.16.0.2
set transform-set MYSET
In essence, deploying IpSec protocols on networks bolsters security loopholes by keeping data exchanges secure and shielded. Moreover, they streamline website accessibility, driving business longevity and resilience amidst a host of cyber vulnerabilities.
IPSec (Internet Protocol Security) is a powerful suite of protocols used to protect data as it travels across the Internet. It provides two modes of operation, AH (Authentication Header) and ESP (Encapsulating Security Payload), that offer different functionalities in terms of security.
AH – Authentication Header
xxxxxxxxxx
- Function: Provides only authentication.
- Place in Packet: Inserts into IP header's typical payload area.
-
The main function of AH is to authenticate the sender and verify that information has not changed during transmission. AH protocol operates directly on top of IP, by using IP protocol number 51. It prevents tampering with the packet during transit. As part of an IP packet, an AH segment contains both the standard IP headers followed by the AH headers. However, it doesn’t provide encryption, meaning it can’t secure against eavesdropping or modify data confidentiality.
ESP – Encapsulating Security Payload
xxxxxxxxxx
- Function: Encryption.
- Place in Packet: At end of IP packet.
-
The ESP encrypts the actual payload of the IP packet to prevent it from being read if intercepted. ESP functions at the level of the IP header, right below it in stack terms, and uses IP protocol number 50 to do this. The ESP header is inserted after the IP header and before a higher layer protocol such as TCP or UDP. The remaining payload plus a new trailer are then encrypted. This process provides both confidentiality and connectionless integrity of the protected connections.
Features | AH | ESP |
Data Integrity | Yes | Yes |
Data Authentication | Yes | Yes |
Confidentiality (encryption) | No | Yes |
As you can see, the two protocols serve different roles and meet a variety of needs depending on what you’re trying to achieve in your network. Handling AH and ESP together in Transport and Tunnel mode allows for versatility, including being able to provide authentication to the entire original IP packet in addition to its copy, while also offering the potential for confidentiality through encryption.
Sample Code
Please note there’s no direct way to interact with IPsec via code due to its low-level implementation, but you can use systems commands and software like strongSwan and OpenSwan to manipulate IPSec policies.
In Unix-based systems, you can execute commands related to these packages inside your scripting or programming language environment. For example, in Python:
xxxxxxxxxx
import os
os.system('sudo ipsec up myVPN')
The above Python script will initiate an IPSec VPN named ‘myVPN’ (if already configured).
The Internet Protocol Security (IPSec) is employed in cybersecurity strategies to add a security layer to the data as it’s transmitted over an unprotected network, such as the internet. This protocol suite uses cryptographic technologies to uphold scenario requisites like data confidentiality, data integrity, authentication, and anti-replay.
IPSec operates using two different types of encryption keys: symmetrical and asymmetrical, each with its unique advantages and hiccups:
Symmetrical keys:
Under symmetrical key encryption, the identical key is used for both encrypting and decrypting the data. This leads to more streamlined processes, quicker encryption, and faster communication. However, it has a primary disadvantage of both parties needing an identical copy of the key. Sharing the key securely is one conundrum here, which curtails scalability when dealing with a significant amount of parties exchanging encrypted data.
xxxxxxxxxx
// simple illustration of symmetrical key exchange
sender = "message"; //original message
symmetric_key = "secret"; //secret key
encrypted_message = encrypt(sender, symmetric_key); //encrypt the message
decrypted_message = decrypt(encrypted_message, symmetric_key); //decrypt received message with same key
Asymmetrical keys:
In comparison, asymmetric key encryption employs a pair of related keys for the process: a private key kept secret by a party and a public key distributed openly. While one key encrypts the message, the other decrypts it. This structure fortifies security, ensuring only the intended receiver can decode the info conveyed. A persistent downside is its increased complexity and slower speed.
xxxxxxxxxx
// simple illustration of asymmetrical key exchange
sender = "message"; //original message
receiver_public_key = "public_key"; //public key
receiver_private_key = "private_key"; //private key
encrypted_message = encrypt(sender, receiver_public_key); //encrypt with receiver's public key
decrypted_message = decrypt(encrypted_message, receiver_private_key); //only receiver with private key can decrypt
The IPSec Protocol Role Between Keys:
The IPSec protocol fundamentally employs these two technologies at distinct stages for maximized efficiency and security. Under RFC 2401, IPSec uses symmetric encryption (with algorithms like AES or Triple DES) on the actual data packets due to its quick encrypt/decrypt capability. Asymmetric encryption, by contrast, is utilized when establishing secure tunnels during “handshake” phases for exchanging symmetric key cryptography.
Continuing this way, IPSec secures seamless intercommunication between the necessary elements of an unprotected network while upholding data integrity and security. It superbly balances the swiftness of symmetric encryption and the superior security shield delivered by asymmetric encryption.
Source code snippets above are illustrations, not functional code.IKEv1 and IKEv2 are both protocols that apply to Internet Security Association and Key Management Protocol (ISAKMP) frameworks. These two versions of Internet Key Exchange play an active role within the broader context of IPSec protocol, essentially governing the establishment and handling of security associations (SAs).
xxxxxxxxxx
InternetProtocolSecurity(Ipsec): //Method of Authenticating And Securing Data Transfers
{
IKEVersions: {
V1,
V2
}
}
IPSec, or Internet Protocol Security, could be seen as a metaphorical ‘vault’ through which data passes during internet connections. It affords authentication and/or encryption for IP packets, providing a measure of system integrity and confidentiality in communications. Effectively, your data gets protected during transmission, safeguarding it from unauthorized access.
Now, delving into both versions of Internet Key Exchange:
IKEv1
The first version of Internet Key Exchange, or IKEv1 featured prominently at the inception of secure network communications. Pertinent aspects include:
– Phased Approach – Involves two separated phases for SA establishment.
– Flexibility – Can use multiple methods for authentication.
– Compatibility – Supports different encryption and hashing algorithms.
xxxxxxxxxx
IKEV1(Phases 1 & 2)
{
Mode: Main, Aggressive
Authentication:
{
Pre-shared Keys
Digital Certificates
Signature-based
}
Services:
{
Confidentiality
Integrity
Re-cheching
}
}
However, some significant drawbacks emerged over time, such as high complexity due to its phased approach and lack of support for EAP authentication techniques (used commonly in wireless networks).
IKEv2
In response to the limitations found in IKEv1, Internet Engineering Task Force (IETF) developed a more robust version— IKEv2. Highlight features are:
– Streamlined Approach – Simplifies the SA process into a single phase.
– Mobility Support – Includes Mobile IPv6 (MOBIKE) protocol, mainly aimed at maintaining VPN sessions while switching across different networks.
– EAP Authentication – Provides Extensible Authentication Protocol (EAP) method support useful for wireless network security.
– Improved Efficiency – Consumes less bandwidth due to fewer message exchanges during the SA process.
– Enhanced Error Handling – Offers better message integrity checking and robustness under unstable network conditions.
xxxxxxxxxx
IKEV2(Combined Phase)
{
Mode: Initiator, Responder
Authentication:
{
Pre-shared Keys
Digital Certificates
EAP Mechanisms
}
Services:
{
Confidentiality
Integrity
OriginAuthentication
Anti-replayService
}
}
While IKEv2 offers numerous improvements, its newer nature results in reduced compatibility with older networking equipment—an aspect where IKEv1 often fairs better.
In encapsulation, both IKEv1 and IKEv2 can coexist within the IPSec protocol, serving specific functions based on the requirements of individual clients and servers. Having higher efficiency and advanced functionality, IKEv2 is recommended wherever feasible; however, compatibility constraints might sometimes necessitate sticking to the initial version—IKEv1. Source .
IPSec and SSL/TLS are both protocols commonly used for secure internet communications. Each of these has their own set of advantages and use cases. In this narrative, I will be focusing on the IPSec protocol, its functionality, as well as a comparative analysis between IPSec and SSL/TLS with respect to performance and use cases.
IPSec Protocol
IPSec or Internet Protocol Security is a suite of protocols that provide a cryptographic layer to IP (Internet Protocol) traffic, encompassing both IPv4 and IPv6. Its primary purpose is to protect data by encrypting it before transport so that even if the data is intercepted, it cannot be read without the correct encryption key.
Some standout features of utilizing IPSec includes:
- Secure communication over untrusted networks: Encryption allows secure data transfer across potentially insecure networks like the internet.
- Data Integrity: IPSec ensures that the data is unchanged from source to destination by using cryptographic checksums.
- Authentication: The protocol verifies that the data originates from a trusted source.
Here’s an example of how an IPSec connection might be implemented:
xxxxxxxxxx
<connection name="My IPSec Connection">
<vpn>
<ipsec-settings>
<remote-ip>203.0.113.25</remote-ip>
<preshared-key>s3cr3tk3y</preshared-key>
<protocol>ESP</protocol>
</ipsec-settings>
</vpn>
</connection>
This establishes a VPN connection with a specific remote IP address using the pre-shared key for authentication, and the ESP protocol for encryption.
A Comparative Analysis: IPSec versus SSL/TLS
While both IPSec and SSL/TLS provide security for data in transit, they cater to different needs based on their design.
Performance: In general, SSL/TLS tends to have lower overhead and thus better performance because it operates at the application layer and only secures specific applications that are SSL/TLS-aware. On the other hand, IPSec operates at the network layer, securing all traffic that passes through the configured tunnel which could lead to higher overhead and potentially slower performance.
Performance | |
---|---|
IPSec | Can be slower due to higher overhead |
SSL/TLS | Generally faster with lower overhead |
Use Cases: If the goal is to secure connections between entire networks (site-to-site), then IPSec is indeed the optimal choice due to its operation at the network layer. However, if individual connections need to be secured (end-to-end), SSL/TLS is normally preferred due to its compatibility with most web browsers and no requirement of client software installations.
Use Case | |
---|---|
IPSec | Site-to-Site Secured Connections |
SSL/TLS | End-to-End Secured Connections |
Hence, IPSec and SSL/TLS serve different purposes and are used based on the specific requirements of the system’s architecture. To dive deeper into the intricacies of these protocols, I would recommend exploring resources available online, such as Cisco’s extensive documentation, that can provide more comprehensive technical knowledge.The IPSec or Internet Protocol Security is a suite of protocols that are applied to ensure the confidentiality, integrity, and authentication of data communications over an IP network. To provide security, the IPSec uses cryptographic security services and encryption algorithms.
IPSec operates in two modes – Transport mode and Tunnel mode:
– In Transport mode, only the payload (the data you want to communicate) is encrypted.
– The Tunnel mode, on the other hand, encrypts both the header and the payload.
Furthermore, IPSec encompasses two primary component protocols: Encapsulation Security Payload (
xxxxxxxxxx
ESP
) and Authentication Header (
xxxxxxxxxx
AH
).
– With
xxxxxxxxxx
ESP
, the principal purpose is to provide confidentiality, connectionless integrity, data origin authentication, and an anti-replay service.
– The
xxxxxxxxxx
AH
protocol provides connectionless integrity, data origin authentication, and an optional anti-replay service.
Now, let’s delve a bit into real-world applications where IPSec Protocols find their applications.
VPNs
In Virtual Private Networks (VPNs), IPSec protocols are typically used. VPN services provide secure internet connections to corporate networks across the Internet, allowing remote users to work as if they are physically present at the office.
Consider this scenario – an employee traveling out of the country can use VPN with IPsec protocols to access the company’s internal systems securely. No outsider can spy on the communications because IPsec encrypts the data packets transmitted over the connection.
Secure Branch Office Connectivity
Organizations with numerous offices scattered in different locations often use IPSec to create a direct and secure communication line between two offices.
For example, a financial organization may have its main headquarters in New York City and a branch office in San Francisco. The administrators can establish a secure communication line via a WAN link using IPSec protocols. This assures the safe transmission of sensitive information like customer account data.