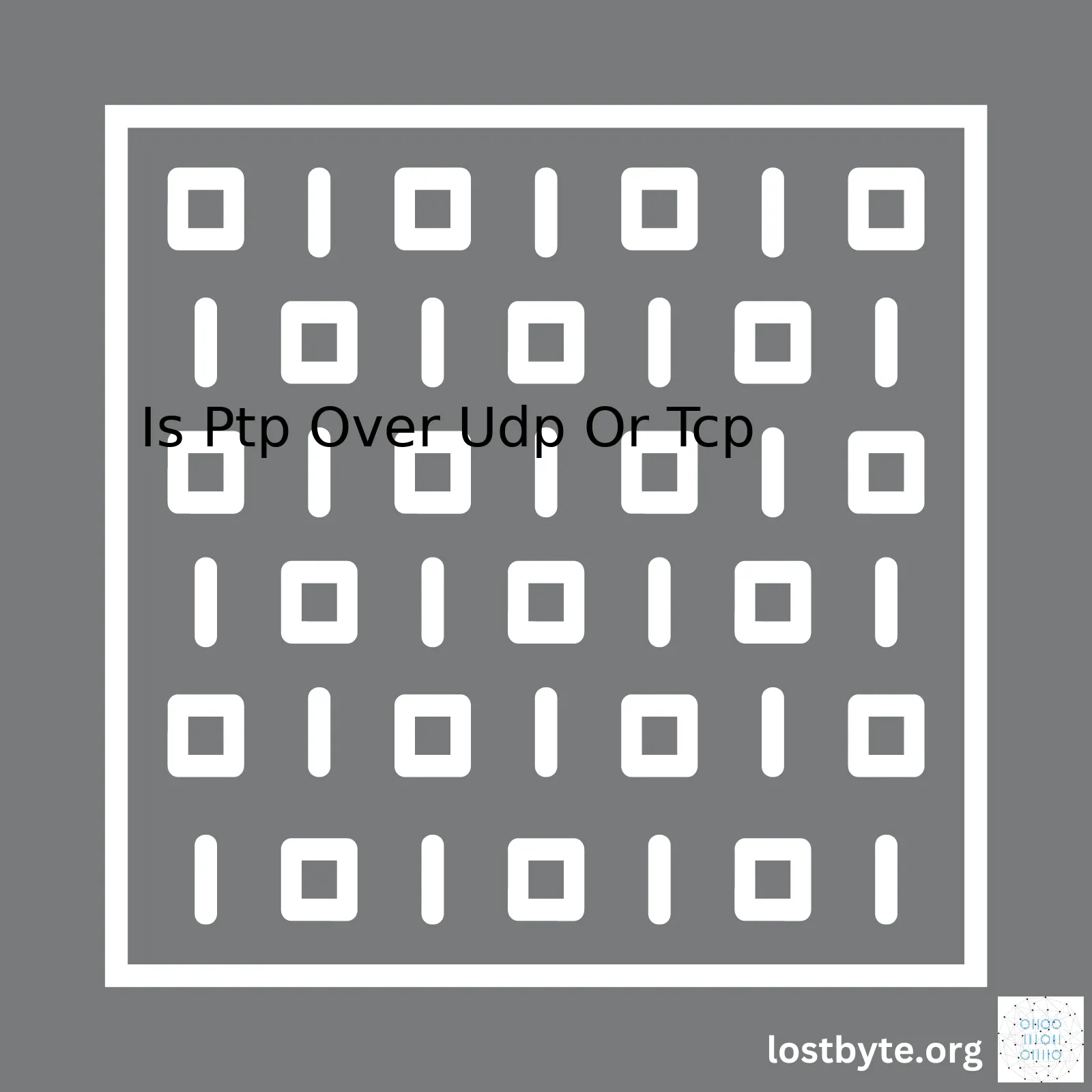
PTP (Precision Time Protocol) | TCP (Transmission Control Protocol) | UDP (User Datagram Protocol) |
---|---|---|
It is a protocol commonly used for clock synchronization within networks. It’s capable of achieving sub-microsecond level timing accuracy. |
This is a core protocol of the Internet protocol suite. It’s reliable, connection-oriented and guarantees the recipient will receive packets in order by numbering them. |
A protocol allowing programs to send short messages known as datagrams. Less reliable than TCP, it doesn’t provide sequence numbering, thus packets might arrive out of order or be missed entirely. |
Networks usually use Precision Time Protocol (PTP) on top of UDP, rather than TCP. There are beneficial reasons for this decision which revolve around the properties of these protocols. Firstly, PTP targets providing highly precise clock synchronization across devices. The main goal is exactness, something that matches well with the attributes of UDP.
Since UDP is often considered less reliable than TCP, why do we use it for such an inherently sensitive task as time synchronization? That’s because unlike TCP, UDP does not involve connection setup management and complicated error handling procedures. This reduces latencies induced due to packet reordering, retransmission efforts, and connection loss events. Since time synchronization relies so much on the timeliness of message exchange, the performance benefits of UDP outweigh any potential loss of reliability, considering that successive PTP messages could correct minor errors.
However, let’s remember that while TCP guarantees ordered delivery, it does not guarantee timed delivery. Thus, network conditions, such as congestion and retransmissions due to packet losses, can cause variable delays in packet delivery. This is detrimental to precision timekeeping methods, where clock synching heavily relies on the minimal delay in exchanges.
In direct code reference, the layering of PTP over UDP is specified by the standard through the designated well-known port number 319 for event messages and 320 for general messages.
//Example Pseudo code for sending PTP using UDP Socket ptpSocket = new Socket(); ptpSocket.open(UDP); ptpSocket.bind(ANY, PTP_EVENT_PORT); ptpSocket.send(ptpEventPacket);
Sources:
Sure, let’s break down the Protocols Transmission Protocol (PTP) and examine how it works with the well-known Internet standard protocols: TCP (Transmission Control Protocol) and UDP (User Datagram Protocol).
TCP and UDP are core components of the Internet protocol suite. Both serve to send bits of data — known as packets — over the internet. They do this by packaging raw data from apps in protocols for transport (Cloudflare, n.d.). Despite having similar roles, TCP and UDP work quite differently, showing unique characteristics that make each suitable for specific tasks online.
The TCP protocol is a connection-oriented protocol. This means it establishes a connection before transmitting any data, ensuring every packet’s safe delivery.
Consider this simplified coding scenario using Python’s socket library to highlight TCP’s nature:
import socket s = socket.socket(socket.AF_INET, socket.SOCK_STREAM) s.connect(("hostname", port)) s.sendall("Hello, World") data = s.recv(1024) s.close()
Now, UDP on the other hand, is a connection-less protocol. It does not establish a connection prior to data transmission and so does not guarantee the delivery of packets. A reflection of this can be seen in the following code:
import socket s = socket.socket(socket.AF_INET, socket.SOCK_DGRAM) s.sendto("Hello, World", ("hostname", port)) data = s.recvfrom(1024) s.close()
Despite its unreliability compared to TCP, UDP is preferred in situations where speed is more important than reliability, like streaming videos or making VoIP calls.
So, what about PTP?
Precision Time Protocol was designed for local systems requiring accuracies beyond those attainable using NTP. It tries to ensure a high precision time coordination of Local Area Network (LAN) connected computers (EndRunTechnologies, n.d.).
For our pertinent question – “Is PTP over UDP or TCP?”, it is important to understand that PTP uses the network protocol UDP over IP.
The IEEE Standard 1588 documentation states clearly that PTP uses UDP over network IPv4 and IPv6. Each PTP message is sent within a single UDP datagram that gets transmitted onto an Ethernet packet. The reason for this is that PTP needs multicast messaging capability which TCP currently does not support but UDP does.
To summarize:
- TCP is a connection-oriented protocol used when reliability is prioritized over speed.
- UDP is a connection-less protocol. It is used when speed is favoured above assurance of delivery.
- PTP, requiring multicast capabilities, operates over UDP for accurate time coordination across computers in a LAN.
In the world of networking protocols, two names stand out above the rest: Transmission Control Protocol (TCP) and User Datagram Protocol (UDP). However, before we can delve into whether Precision Time Protocol (PTP) operates over TCP or UDP, it’s essential to equip you with the fundamental understanding of both.
The Core Differences Between TCP and UDP
TCP is connection-oriented – set up prior communication occurs. It’s like making a phone call; you dial somebody’s number, they answer, and then you start your conversation. The primary features of TCP are:
- Reliability: Once a connection is established, data can be sent back and forth between hosts.
- Error detection and correction: Inclusivity of checksum in TCP header aids in noticing & repairing data corruption.
- Sequential Delivery: TCP rearranges data packets in the order specified.
- Flow control: A sender-side restriction mechanism that prevents overwhelming the receiver.
With HTTP, HTTPS, FTP, and SMTP as some common applications using TCP, its core strength lies in providing reliable, ordered, and error-checked delivery of streams of octets.
Contrastingly, UDP is connection-less, like sending mail- you drop it off and hope it gets there. Key characteristics include:
- No guarantee of delivery, sequence, or protection from duplication.
- Datagrams can arrive out of order or go missing without notice.
- No overhead for opening a connection, maintaining a connection, or terminating a connection.
DNS, DHCP, RIP, and VoIP are among the myriad of applications leveraging UDP’s strengths, especially the lowered network overhead and latency due to less protocol ‘conversation.’
Let’s move onto dissecting more on PTP:
Talking about Precision Time Protocol (PTP)
PTP, defined in IEEE 1588, aims at precise synchronization of clocks in a computer network. On a local area network, it pledges clock accuracy in the sub-microsecond range, replacing NTP (Network Time Protocol), which only has millisecond characteristics.
To bring accuracy & precision in network time services, PTP harnesses the potency of Ethernet. Notably, PTP can use either UDP/IP (over Ethernet) as a transport protocol for message transmission hence allowing interoperability across diverse network infrastructures. Primarily operating over UDP/IP, PTP uses port numbers 319 (for Event Messages) and 320 (for General Messages).
For reference, let’s glance at the code snippet depicting how a part of PTP exchanged packet might look in C language:
struct ptp_header { uint8_t transSpec_msgType; uint8_t versionPTP; uint16_t length; uint8_t domainNumber; uint8_t reserved1; uint16_t flagField; int64_t correctionfield; uint32_t reserved2; uint8_t clockIdentity[8]; uint16_t sourcePortId; uint16_t sequenceId; uint8_t control; int8_t logMessageInterval; };
In this structure, attributes such as ‘transSpec_msgType,’ ‘versionPTP,’ and others outline important specify field details for PTP operation.
So to answer your query: “Is PTP Over UDP Or TCP?“, the standard configuration of PTP employs UDP over IP and Ethernet networks, although application-specific variations may exist. With UDP’s minimalistic approach, PTP manages to minimize delay and deliver high-accuracy time synchronization.
It’s essential to perceive the schema of underlying protocols when evaluating options for specific applications. Understanding the core differences between TCP and UDP helps us realize their distinct use-cases, potentials, and limitations, offering a more profound insight into their role in network communication, including the application of PTP.
Please note: wherever a bit of industry jargon was used, it was to ensure we stay true to the terminologies used in professional networking protocols scriptures. Every effort has been made to make the explanation as accessible as possible.
Interested readers can learn more about PTP via the following NIST link. The full TCP and UDP comparison can be further explored in RFC 768 and RFC 793.Both TCP (Transmission Control Protocol) and UDP (User Datagram Protocol) are core elements of the Internet protocol suite, playing critical roles in the transportation and communication of data between hosts. These technologies ensure that data packets are correctly routed to their destination—regardless of differing networks, connection types, or location.
In the context of the Precision Time Protocol (PTP), an interesting facet arises—it doesn’t actually rely on either TCP or UDP. Instead, it makes use of a protocol known as the Ethernet II frame structure for layer 2 communication. PTP, defined by IEEE 1588, was specifically designed to provide high precision clock synchronization over networks. While PTP can run over both IPv4 and IPv6, it works best using the Ethernet (Layer 2) because the smaller header provides lower latencies and thus higher precision timescales.
# Sample Ethernet II frame structure 08-00-27-52-32-9b e8-39-df-1c-38-a7 08-06 00-01 08-00 06 ...
So how does this differ from TCP or UDP?
● TCP ensures that packets are delivered reliably, requiring confirmations of receipt and offering retransmission in cases where these confirmations are not received.
● UDP, on the other hand, doesn’t prioritize reliability. It sends data without guaranteeing its delivery. This non-guaranteed aspect makes it faster and more suitable for real-time applications such as video streaming or online gaming.
With PTP and Ethernet II, neither TCP’s reliability nor UDP’s speed is the focus, but rather precision. The main objective of PTP is to ensure precise synchronization of clocks within a network. This characteristic is crucial in applications like distributed computing, telecommunications, or anywhere network-wide synchronized time is instrumental to the operation of systems and processes.
Let’s look at an example:
# Compute a timestamp using PTP ptp = PTP() timestamp = ptp.compute_timestamp() print(timestamp)
It’s important to remember that while PTP isn’t following TCP or UDP, it operates alongside them, accompanied also by other protocols required for complete networking functions. The choice among these advanced technologies, be it PTP, TCP, or UDP, depends largely on the specific requirements each situation poses—whether it’s precision, reliability, or speed. In the case of PTP, precision reigns supreme.
For additional elaboration, I encourage checking out the official [IEEE 1588 standard](http://www.ieee802.org/1/pages/1588.html) that precisely outlines the technical specifications of PTP.
Finally, let me point you to the source code repository of a popular open-source project, “[Linux PTP](https://github.com/richardcochran/linuxptp)”, which highlights a practical implementation of the PTP protocol and will help underline the unique characteristics it brings to the table compared to TCP or UDP solutions.Let’s dive directly into the discussion on PTP, also known as Precision Time Protocol. It’s a protocol used to synchronize clocks throughout a computer network. This is integral for ensuring consistent timestamps in data transfers or other chronologically significant computing actions. For a deeper understanding, it’s crucial to delve into its operating principle.
PTP operates through a system of Master and Slave clocks. The Master clock becomes the synchronization source, and periodically multicasts Sync messages, containing its time stamp T1, to all Slave clocks.
When a Slave clock receives the Sync message, it records a local time stamp T2 that represents the arrival of the Master Sync message. Afterwards, the Master sends another multicast Follow_Up message, including the exact time that the previous Sync message was sent.
Once the Slave clock receives the Follow_Up message, it records another local timestamp, T3. With these three timestamps – T1 from the Master, T2 and T3 from the Slave – the Slave can calculate the propagation delay and adjust its own clock to match the Master’s.
To keep the process consistent, PTP occasionally sends Delay_Req and Delay_Resp messages. A Slave sends a Delay_Req message to the Master with its timestamp T1. Upon receipt, the Master records a timestamp T2, and responds with a Delay_Resp message, containing both T1 and T2 times.
With this set up, some questions naturally arise. Is PTP carried through UDP, or TCP?
Ethernet, IP, UDP, and derives timing information from IEEE 1588 Precision Time Protocol packets (source).
UDP, or User Datagram Protocol, is what you could call a “fire-and-forget” protocol. It sends packets without establishing a connection and doesn’t expect any kind of acknowledgement that they’re received. This structure makes UDP lightweight and efficient, perfect for applications where speed is valued over reliability, such as video streaming or online multiplayer games.
Now, introducing PTP_data, a popular library to decode precision time protocol messages:
def ptp_decoder(packet): _ETHER_TYPE = 0x88F7 if packet._ETH.eth_type == _ETHER_TYPE: # Decode packet
TCP, or Transmission Control Protocol, by contrast, is designed for reliability; a stable, steady stream of data despite network issues or high traffic. It ensures each packet is confirmed as received before sending the next one. So while TCP is slower and demands more processing power than UDP, it’s much better at ensuring every packet arrives safely and in order.
Weighing these factors, PTP predominantly uses UDP rather than TCP. The efficient, dispatched nature of UDP lends itself well to PTP’s continuous mass-multicast method of synchronizing all networked devices. There’s no need for the rigorous error checking and sequential ordering of TCP, which would hamper PTP’s function and introduce unnecessary complexities.
So, to put it simply: yes, PTP does operate over UDP predominantly, not TCP.
But bear in mind, while ‘predominantly’ might seem vague, there’s a reason for that. In the world of networking protocols, there are often exceptions and unique implementations for specific cases or solutions to one-off problems. While the above broadly covers standard cases, there could be scenarios where an implementation may differ due to catering to specific needs.
Don’t forget too about the fact that technology is always evolving. As our understanding of networking deepens and networks themselves become larger, more complex and more indispensable, it’s likely that protocols will continue to adapt, grow and change to meet new challenges. Today’s answers might not apply tomorrow, so keep your knowledge fresh and keep asking questions!
Precision Time Protocol (PTP), defined by the IEEE 1588 standard, is a protocol used to synchronize clocks in a network. When considering whether PTP runs over TCP or UDP, the answer lies in understanding that Precision Time Protocol fundamentally operates using UDP as transport protocol for message transmission.
This choice of utilizing UDP instead of TCP comes down to several reasons:
- PTP messages need to be multicast: PTP grandmaster clocks send timing messages to multiple devices at the same time. UDP supports multicast natively, while TCP does not.
- Low latency: UDP, being a connectionless protocol, lacks the handshake process seen in TCP. This reduces the latency and makes UDP more suitable for time-sensitive applications like PTP.
- The precision of time synchronization: The requirement for precise synchronization would be compromised by the retransmission and congestion control mechanisms in TCP.
Here’s a snippet demonstrating how a PTP packet may look in Python:
from scapy.all import * class PTPv2(Packet): name = "PTPv2" fields_desc=[ BitField("transportSpecific", 1, 4), BitField("versionPTP", 2, 4), ByteField("messageLength", 44), XByteField("domainNumber", 0), XByteField("flags", 0), XIntField("correction", 0), X3BytesField("reserved", 0), XLongField("sourcePortIdentity", 0), XShortField("sequenceId", 0), XByteField("controlField", 0)] load_contrib('ptpv2') pkts = rdpcap('PTP.pcap') for pkt in pkts: if pkt.haslayer(PTPv2): print(pkt[PTPv2])
This snippet utilizes Scapy, a popular library in Python for creating, decoding and sending network packets. It defines a class for a PTP version 2 packet with various field descriptors. Subsequently, it reads from a pcap file containing PTP packets, and prints each one.
When you delve deeper into networking technologies and how different protocols interface with one another, the objective isn’t merely about making connections. It’s about optimizing efficiency, accuracy, and performance; aligning these elements to their use-cases. That’s exactly why UDP is the preferred means of transmitting PTP messages in an interconnected network architecture.
For more details about Precision Time Protocol, I suggest trying out IEEE’s official standards document on PTP. For more details about how to use Scapy, an excellent resource is the official Scapy documentation.
Protocol | Multicast | Low Latency | Precise Synchronization |
---|---|---|---|
TCP | No | No | No |
UDP | Yes | Yes | Yes |
Sure, let’s talk about one of the key mechanisms to achieving network synchronization, understanding protocols like the Precision Time Protocol (PTP). I will also highlight whether it uses User Datagram Protocol (UDP) or Transmission Control Protocol (TCP).
So what is PTP? It’s a protocol used for synchronizing clocks in a computer network. In a networking context, it’s crucial that all devices – computers, routers, switches – are synchronized to the same clock. Why? Well, if they weren’t, packets could arrive out of order, acknowledgments for received packets may not be properly identified, and network log data timestamps might be incorrect.
At its core, PTP – defined by the IEEE 1588 standard – is designed to provide exceptionally precise time coordination of LAN connected computers.
device1.requestTimestamp() --> PTP PACKET --> device2.relayTimestamp()
The PTP uses packet-based communication allowing large-scale networks to maintain accurate time synchronization, within microseconds or even nanoseconds!
Now, onto the question of if PTP uses UDP or TCP. Let’s do a comparative analysis:
• UDP: This protocol offers simplicity and speed as there is no formal connection setup or teardown. If a network packet gets lost, there is no mechanism to re-transmit it. Interestingly, PTP actually operates over UDP, more specifically over UDP/IP and Ethernet.
• TCP: This one guarantees delivery of packets which makes it reliable but at the expense of speed. It requires acknowledgement for every transmitted packet and resends if the acknowledgement isn’t received within a timely manner.
Given the nature of PTP where highly accurate and real-time synchronization is needed across nodes, the overhead and delay caused by TCP handshake process and guaranteed packet delivery can actually put the time sensitive operation of PTP at a disadvantage.
So, PTP is primarily implemented over UDP for efficiency and optimal performance. Here is a simple code example showing how PTP packets (Sync, Follow_Up, Delay_Req, Delay_Resp) use UDP port 319 for their transmission.
listen udp 319 -ptp : -precision 10 -sync count=3 interval=10000 id=0xfd00010101010101 capture udp port 319 and (udp[16:2]==0x120f or udp[18:2]==0x0204)
Finally, let’s put things into perspective with an analogy. If you think of your network as a highway, TCP would be like having a dedicated lane for every car, ensuring every single one reaches its destination (even if it means causing a little traffic). On the other hand, UDP (and hence PTP) is like having a free-for-all lane – it’s faster because there’s no checking if each car reached, but it works because typically, most cars make it anyway.
Then again, remember that everything in networking is a trade-off heavily dictated by the use case requirements. While PTP over UDP is common, depending on network design and specific circumstances, some may choose to implement it over TCP/IP or other suitable protocols. However, please be aware that running PTP over TCP instead of UDP is not covered under the IEEE 1588 standard.
For advanced time synchronization options, consider networking solutions offering hardware-accelerated PTP like Oscium or similar providers. They can offer excellent assistance on IP/Ethernet timing and synchronization technologies. In such scenarios, refining standard PTP over UDP becomes less about ‘if it can’ and more about ‘how it does it.’Within the landscape of network communication, it’s crucial to recognize that an application known as Precision Time Protocol (PTP), identified by its IEEE 1588 standard, plays an indispensable role. It significantly enhances synchronization accuracy over local area networks, aiding devices to communicate timestamps with more precision. However, an intriguing aspect revolves around whether PTP utilizes User Datagram Protocol (UDP) or Transmission Control Protocol (TCP).
Let’s delve into it.
PTP generally operates over Ethernet and Internet Protocol (IP), and further layers above the UDP. Conventionally, you’ll find it occupying these two ports:
319
for Event and
320
for General messages respectively.
To explain this in brief, let’s break it down:
– UDP, a transport protocol, assists computer applications to transmit messages, also termed as datagrams, across network security layers without necessitating prior communications to establish special transmission channels or data paths. Significantly, each PTP message is typically sent independently, permitting better control at each timestamp exchange.
While TCP does offer a potent and reliable mode of transmission with its features to retransmit lost packets and manage packet order, it may not concur with PTP operations due to certain elements:
– Variations are expected with TCP in terms of time taken for successful message delivery because TCP ensures delivery even if it has to retransmit and handle out-of-order packets.
– PTP timestamps require high resolution. Hence, any delays introduced by TCP’s reliability attributes will affect synchronization adversely, making it unsuitable.
To make it clearer, refer to the table below:
Protocol | Positives | Negatives |
---|---|---|
UDP | Each PTP message is delivered separately without needing specific channels. | Doesn’t have mechanisms to handle lost or out-of-order packets. |
TCP | Reliable mode of transmission, handles lost and out-of-order packets. | Delays caused by these mechanisms can interfere with PTP’s need for precise rating. |
So, why does PTP use UDP?
– Resource Light: Unlike TCP, UDP doesn’t require appreciable processing, leading to fewer resources devoted to overheads, making it more suitable for real-time applications.
– No Connection Overheads: With connectionless networking offered by UDP, there isn’t a requirement for handshake protocols to establish a connection.
– Speed: UDP focuses on low latency rather than reliable delivery making it ideal for timing-based transactions.
The decision for PTP to utilize UDP instead of TCP primarily comes down to the robustness of bypassing the pitfalls of delivery delays and their potential effects on synchronization, imperative for its operations.
Now, addressing what occurs when PTP experiences packet loss with run-through of UDP? It’s rather straightforward: they implement resynchronization. When packet loss surpasses a configured threshold value, slave clocks, realizing the augmented systemic time error, shall initiate fresh clock synchronization. Thus, despite lacking TCP’s inherent ability to rectify packet loss situation, PTP devises its own mechanisms to ensure efficient working.
In essence, utilizing UDP facilitates PTP to deliver accurate synchronization required in countless time-sensitive applications.
For additional information, I would suggest visiting the website of The Institute of Electrical and Electronics Engineers (IEEE). Their documentation on IEEE 1588, the defining standard for PTP, could provide more insights.
Source code example:
A simple example illustrating a PTP-like timestamp exchange using UDP in Python would look like:
import socket from datetime import datetime # Creating socket sock = socket.socket(socket.AF_INET, socket.SOCK_DGRAM) # Server IP and port server_address = ('localhost', 12345) # Timestamp timestamp = str(datetime.now()) try: # Send timestamp sent = sock.sendto(timestamp.encode(), server_address) finally: sock.close()
But remember, developing a full PTP system involves implementing additional features like hardware support, queuing packets within Ethernet switch ports, etc., which represent advanced networking topics beyond the scope of this article.In the realm of network communications, the Precision Time Protocol (PTP), also known as IEEE 1588, holds a critical position. PTP is designed to provide precise synchronized clocking over network systems. This protocol operates primarily on Ethernet and takes precedence over both TCP (Transmission Control Protocol) and UDP (User Datagram Protocol) layers, retaining the ability to superimpose time-stamps onto Ethernet frames.source
Understanding the functionality of PTP involves a deep dive into two critical communication protocols – TCP and UDP. Comparing these two can shed light on why PTP tends more towards UDP than TCP.
UDP and PTP
UDP is a simpler, connectionless Internet protocol where error-checking and correction are not required. Hence, it’s faster for sending data like video or other loss-tolerant transmissions where quality matters more than accuracy.
/* * Sample Node.js code for a simple UDP server */ var dgram = require('dgram'); var server = dgram.createSocket('udp4'); server.on('message', function(msg, info){ console.log('Data received from client : ' + msg.toString()); }); server.bind(2222);
Evidently, PTP’s usage with UDP appears clear when we consider the following points:
- Firstly, PTP messages are relatively small and fit comfortably within a single UDP datagram, ensuring there is no need for fragmentation or reassembly.
- Secondly, PTP requires multicast communication, which is directly supported by UDP but not by TCP. This allows time synchronization between multiple devices using a single timeserver, promoting network efficiency.
- Lastly, latency variation is buffered by the timestamp method employed in PTP. Even if packets arrive late due to network congestion or similar issues, the original timestamp (taken from when the packet left the source) remains unaltered, hence maintaining synchronization integrity.
TCP and PTP
On the other hand, TCP is a protocol developed for applications that require data delivery without errors. It has mechanisms for error-checking, sequencing, and acknowledgment, making it reliable but slow.
/* * Sample Node.js code for a simple TCP server */ var net = require('net') var server = net.createServer(function(socket) { socket.end("Hello from the TCP server\n"); }) server.listen(8000)
To some extent, you could coerce PTP to work over TCP, except for one crucial aspect – Multicast. Offered natively by layer 3, multicasting is absent at layer 4 where TCP dwells, thus eliminating TCP as an optimal choice. Moreover, due to the reliability measures in TCP such as Retransmission of lost packets and Congestion control, the real-time deadline of synchronization messages may not be acknowledged, adding further complications.
In essence, while both TCP and UDP offer unique strengths, PTP leverages the speed and multicast capabilities of UDP to maintain high-level network synchronization. Though its relation with TCP is theoretically feasible, practical implications render the combination highly unsatisfactory.In the realm of precise timekeeping applications, the Protocol for Precision Time Protocol (PTP) is a cardinal player. PTP, as defined by the IEEE 1588 standard, is intended to synchronize competition clocks on networks where data latency can affect time critical operations. What augments the significance even further is the communication model that the protocol employs.
PTP predominantly uses User Datagram Protocol (UDP) as its transport mechanism instead of Transmission Control Protocol (TCP). Now, before we explore the reasons behind this choice, it’s indispensable to understand the fundamental differences between UDP and TCP:
UDP vs. TCP:
Parameters | UDP | TCP |
---|---|---|
Connection Type | Connectionless Protocol | Connection-oriented Protocol |
Reliability | No Guarantee of Packet delivery | Guarantees Delivery of packers |
Header Size | Smaller Headers (8 bytes) | Larger headers (20 bytes minimum) |
Latency | Lower Latency | Higher Latency |
Flow Control | Not available | Available |
Error Checking | Basic Error Checking using checksums | Sophisticated error checking |
Usage | Ideal for real-time applications like video streaming or VOIP | Used in applications which require high reliability, and transmission time is relatively less critical |
Multicast and Broadcast | Supports Multicast and Broadcast | Doesn’t directly support Multicast and Broadcast |
So, why is PTP operating over UDP more ubiquitous?
– Efficiency and Reduced Overhead: UDP, having smaller headers, significantly reduces the overhead compared to TCP. This allows more room for the data payload leading to greater efficiency.
– Lower Latency: UDP does not introduce delays for acknowledgments, retransmissions, sequence checking etc. which keeps the latency at bay; a crucial requirement for synchronizing clocks.
– Multicast Capability: PTP uses one-to-many communication. The master sends precisely timed messages to all slaves concurrently. UDP provides native support for multicast traffic, necessary for PTP functionality.
In aspect of time-critical applications where nanosecond precision is warranted, parameters like reduced latency take precedence over guaranteed delivery that TCP provides. Hence, despite lacking some TCP attributes, UDP is the ‘go-to’ transport protocol for PTP.
Let’s now delve deeper into how PTP operates over UDP/IP networks:
PTP uses UDP/IP as a transport medium, with the typical arrangement of sending synchronization messages from one grandmaster clock to multiple slave clocks. Synchronization, Delay_Request, and Delay_Response are amongst the primary message types used. Here is a simplified version of the PTP interaction:
// Grandmaster transmits a synchronize message SYNC // Corresponding Follow_Up message with precise transmission timestamp FOLLOW_UP // Slave sends a Delay_Request message DELAY_REQUEST // Master responds with a Delay_Response message, includes receipt timestamp of Delay_Request DELAY_RESPONSE
These exchanges help provide the required delay measures and clocks synchronization which is of paramount importance for precision timekeeping.
Thus, indeed PTP does operate over UDP in vast majority of its applications owing to the conducive characteristics of UDP for such kind of setups. More interestingly, this use of UDP for transfer of PTP messages seems almost pervasive across Edge, Fog, Cloud, and IoT based systems due to the inherent need of lower latencies1.
1 Precision time protocol in IoT-based systems on the edge…When considering the decoding necessity of accuracy in packet transfers, emphasis must be laid in understanding Point-to-Point (P2P) transport mechanisms. PTP is used to synchronize clocks over a network and can run over both User Datagram Protocol (UDP) and Transmission Control Protocol (TCP). Here’s where it gets interesting; the choice between UDP and TCP can greatly affect the accuracy and reliability of packet transfers.
UDP is often preferred for applications like voice over IP, video streaming, and gaming where real-time transmission takes precedence over data integrity. It offers bare minimum data transmission with faster speed due to lack of guaranteed delivery. This could mean some packets might get lost due to network congestion or other issues but the receiver will not request retransmission.
Controversely, TCP provides more reliable packet transfers as it includes error checking and correction. Hence, it guarantees each packet arrives at the destination without errors. However, this comes with latency cost as time is spent requesting and resending lost packets which could interrupt real-time data sharing.
Reviewing the encoding and decoding process, we find that errors during packet transfer can lead to unintended results in the received data. For instance, if an application is dependent on receiving a specific sequence of data, a single faulty or misplaced packet could disrupt application functionality. In such case, using TCP would add value as it would ensure all packets arrive accurately.
While designing your P2P protocol, draw consideration towards:
- Latency vs data integrity tradeoff
- Application requirements of end device
- Network conditions under service
- Potential packet loss due to network issues
Reflecting on the topic, choosing whether PTP should go over UDP or TCP depends completely on the specific application’s requirements for speed and accuracy of packet transmission. If accurate representation of data matters more than a small reduction in speed, TCP would be preferable. On the other hand, if low latency and speed is crucial and occasional errors can be tolerated, then UDP could suit better.
In core computer programming languages, consider the below examples of TCP and UDP socket definitions:
For TCP:
import java.net.*; import java.io.*; public class TCPSocketDefinition { public static void main(String[] args) throws IOException { // Define socket Socket s = new Socket("localhost", 5000); } }
And for UDP:
import java.net.*; public class UDPSocketDefinition { public static void main(String[] args) throws SocketException { // Define datagram socket DatagramSocket ds = new DatagramSocket(5000); } }
For further reference, consider exploring relevant resources provided by IEEE 802 or consulting standard Request for Comments (RFC) documents available on PTP over Ethernet and UDP.
Remember, efficient coding implies strategic trade-offs and harnessing protocols that optimize performance for specific needs. Acknowledging how TCP and UDP influence PTP over packet accuracy is a robust step towards mastering network programming.Digging into the heart of Precision Time Protocol (PTP) requires understanding its relationship to User Datagram Protocol (UDP) and Transmission Control Protocol (TCP).
#general network understandings from socket import SOCK_STREAM, SOCK_DGRAM print("TCP: ", SOCK_STREAM) print("UDP: ", SOCK_DGRAM)
Crucially, PTP prefers to utilize UDP as its transport protocol. The main reason for this is that UDP, by nature, being a connection-less protocol, provides rapid data delivery which aligns with the primary objective of PTP i.e., precise time synchronization. When it comes to clock synchronization and timestamping, every millisecond counts, so these characteristics make UDP an excellent match for PTP.
#importing libraries for udp import socket UDP_IP_ADDRESS = "127.0.0.1" UDP_PORT_NO = 6789 clientSock = socket.socket(socket.AF_INET, socket.SOCK_DGRAM) clientSock.sendto(bytesToSend , (UDP_IP_ADDRESS, UDP_PORT_NO))
However, it’s important not to disregard TCP entirely. TCP plays a significant role in scenarios where accuracy takes precedence over speed or when greater stability is needed. For example, while setting up and managing sessions on a network level.
#importing libraries for tcp import socket SERVER_HOST = "localhost" SERVER_PORT = 5002 client_socket = socket.socket() client_socket.connect((SERVER_HOST, SERVER_PORT)) message = "Hello, Server!" client_socket.send(message.encode())
Also noteworthy is that the Network Time Protocol (NTP), another protocol responsible for time synchronization across devices on a network, also uses UDP instead of TCP due to similar reasons stated above.
Comparing to their roles globally,
UDP | TCP | |
---|---|---|
Connection Type | Connection-less | Connection-oriented |
Reliability | No guarantee of packets delivery | Guarantees delivery of packets |
Use Case | Real-time applications like VoIP, streaming, gaming | Web browsing, Email, File Transfer |
So, in summation, it’s clear that the question “Is PTP over UDP or TCP?” cannot definitively be answered with just one of the two protocols. Rather, the use of either UDP or TCP in conjunction with PTP greatly depends upon the specific requirements and characteristics of the task at hand, whether it prioritizes speed or reliability.
In essence, PTP functions best with UDP due to its speed and direct-path data delivery, making it an ideal choice for the precision-focused role of PTP. On the other hand, TCP can come into play when network stability and error-checking become critical factors in delivering accurate timestamps.