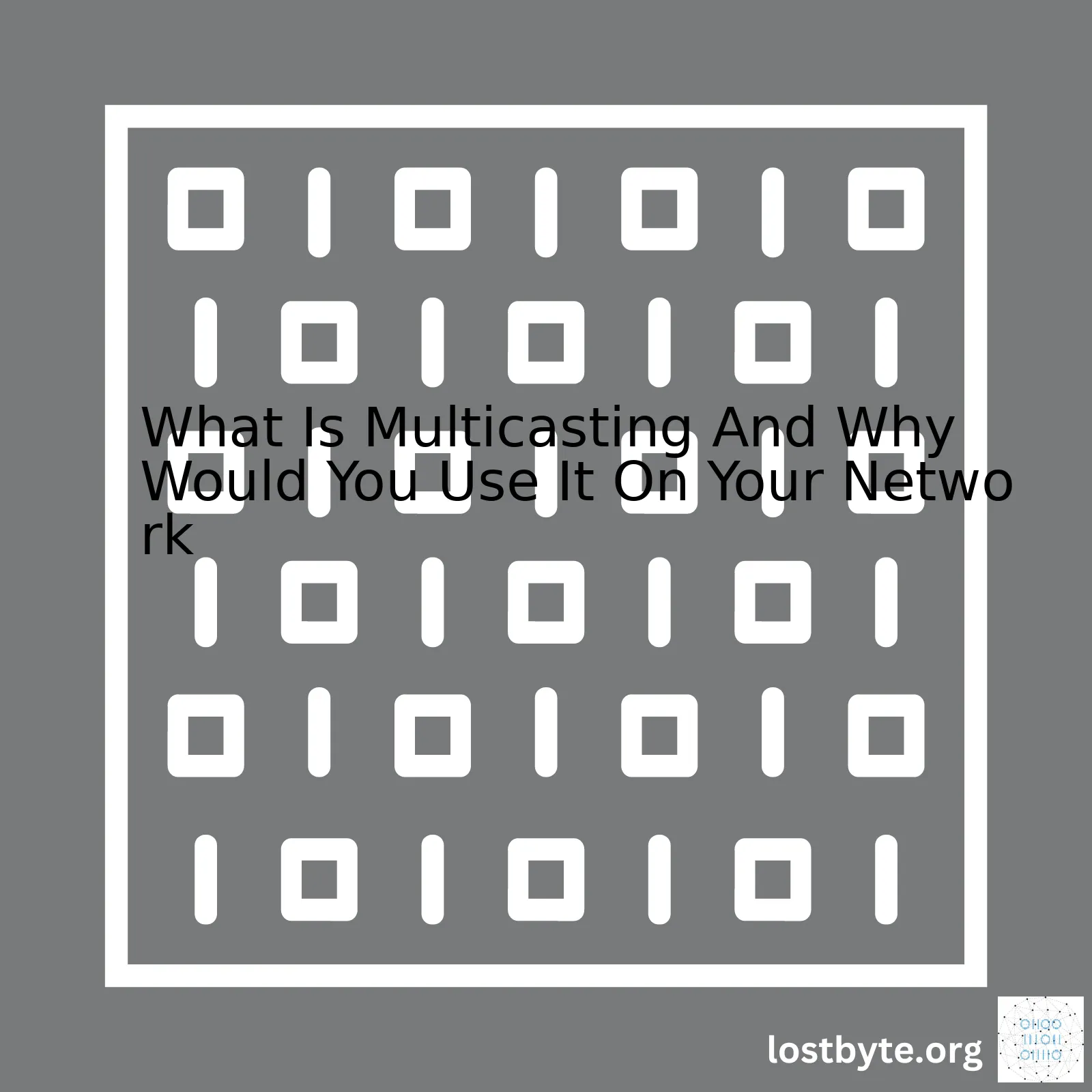
Multicasting is a networking technique where data is transmitted from one point to multiple destinations simultaneously. It is an efficient method for distributing the same information to a substantial number of receivers, thereby conserving bandwidth and system resources. When you have large groups of recipients such as in IP television broadcasting or live video conferencing, multicasting is the optimal choice.
Multicasting | |
---|---|
Definition | A networking protocol where data is sent from one source to multiple destinations concurrently. |
Usage | Ideal for application contexts where there’s one sender and multiple receivers. Predominantly used in IP television broadcasting, online gaming, and corporate communications. |
Advantages | Efficient usage of network bandwidth, it decreases the load on the server, and improves performance by reducing redundancy. |
Challenges | Can potentially increase the complexity of network management due to the necessity of multicast group management. |
To provide an analogy, consider multicast transmission as using a public announcement (PA) system at an airport. Much like the PA system broadcasts announcements to all passengers throughout various terminals, a multicast delivers a single stream of data to many recipients across the network, simultaneously.
In terms of practical uses, multicasting forms the backbone of many modern applications. Internet Protocol Television (IPTV), video/audio streaming services, collaborative corporate communication tools such as Slack or Microsoft Teams, and real-time online gaming are key examples of multicast implementation.source
Multicasting’s prime advantage is the significant reduction in network traffic compared to unicast transmissions. By sending a single copy of data to all interested receivers, multicasting effectively uses bandwidth and increases the overall capacity of the network. This is pivotal for businesses aiming to maintain a robust and high-performing network under vast data load conditions.
From a coding perspective, data packets in multicast communication have specific addresses that allow devices interested in the packet to ‘listen’ and receive it. This is handled on the transport layer of the IP suite through protocols developed predominantly for multicast purposes; such as Internet Group Management Protocol (IGMP). Here’s an example of how the IGMP code might look:
typedef struct { uint8_t type; uint8_t maxRespTime; uint16_t checksum; uint32_t groupAddress; } IGMPHeader;
This defines the header for an IGMP message.
However, it’s not all rosy – multicasting does have challenges. The most apparent is the added complexity in managing and maintaining the group lists of receivers for each multicast stream. This managerial cost may offset some of its benefits, especially in smaller networks with limited administrative resources.
Multicasting is a powerful networking concept that allows data to be sent from one source to multiple destinations simultaneously. This method of data transmission uses a type of communication where multicast traffic originating from one point in the network is replicated and sent to a set of receivers that belong to the same multicast group. Detailed below is the core functionality of multicasting:
• Multicast sends information over an Internet Protocol (IP) network to many receivers at the same time, using less bandwidth compared to transmitting separate copies of that data to each receiver. In other words, you could think of multicasting as one-to-many or many-to-many distribution.
• It uses efficient ways to share information across the network. Multicasting does it by duplicating packets of information that are ready for transmission and sending them out to the appropriate locations.
• Multicasting’s efficiency could be compared to a radio or tv broadcast: once the content is aired, every device tuned to the right frequency/channel can receive the broadcast. However, the devices not tuned to the particular frequency would not load the broadcast, ensuring there is no unnecessary traffic or processing.
To show how multicasting works consider this analogy:
Consider a tree, where the root symbolises the source of the broadcast, branches are network paths and leaves represent receivers/subscribers. The broadcast signal (or data packet) starts at the root, travels along the branches and ends at the leaves.In standard Unicast mode, separate trees would have to grow for each receiver requiring a broadcast. This would consume time and resources. However, in Multicast mode, a single tree can serve multiple receivers, exemplifying high efficiency.
In terms of practical applications, multicasting comes handy when the same data needs to be delivered to several users simultaneously such as:
• Internet Television broadcasts
• Video conferencing
• Distance learning programs where the same class is attended by multiple students spread geographically
• Distribution of software updates: Rather than having each computer download an update individually, multicasting will allow these computers to simultaneously download updates.
Understanding whether or not to apply multicasting on your network depends on several factors. Some key considerations include:
• Bandwidth usage: If your operation involves sending the same data to multiple points in the network, multicasting can significantly reduce bandwidth usage as it prevents duplication of data.
• Network performance: Since multicasting offers efficient use of network resources, utilizing it might result in an overall improved network performance.
• Application requirements: Particular applications like live video streaming, IPTV and online gaming which demand simultaneous data transmission to multiple users can benefit vastly from multicasting.
• Infrastructure readiness: Last but not least, although modern routers support multicast, outdated hardware or network infrastructure may limit your ability to utilize multicast.
Keep in mind that while multicasting offers significant benefits, it also presents some challenges. A key challenge being setting up a system for clients to subscribe/unsubscribe from the multicast groups. Also, without proper control, multicast transmission can lead to flooding of the network leading to reduced performance or failures.
For more advanced concepts related to multicasting, I recommend reviewing Internet Group Management Protocol (IGMP), Protocol Independent Multicast (PIM), and Multicast Source Discovery Protocol (MSDP). Given its complexity, multicasting comes with a steep learning curve. Understanding these protocols will surely help fine-tune your networking skills.[1].
Let’s look at a simple example using Python’s socket module to create a simple multicast sender and receiver:
Multicast Sender:
import socket import struct message = "Hello Multicast" multicast_group = ('224.3.29.71', 10000) sock = socket.socket(socket.AF_INET, socket.SOCK_DGRAM) ttl = struct.pack('b', 1) sock.setsockopt(socket.IPPROTO_IP, socket.IP_MULTICAST_TTL, ttl) sock.sendto(message, multicast_group)
Multicast Receiver:
import socket import struct multicast_group = '224.3.29.71' server_address = ('', 10000) sock = socket.socket(socket.AF_INET, socket.SOCK_DGRAM) sock.bind(server_address) group = socket.inet_aton(multicast_group) mreq = struct.pack('4sL', group, socket.INADDR_ANY) sock.setsockopt(socket.IPPROTO_IP, socket.IP_ADD_MEMBERSHIP, mreq) while True: print(sock.recv(1028))
These codes provide a basic implementation of multicast communication using Python. The sender sends the message “Hello Multicast” to the multicast group, and the receiver constantly listens to that multicast group and prints any message received.
As complex as it may seem initially, understanding multicasting and its potential advnateages can greatly improve network utilization and application performance. Nevertheless, leveraging multicast requires a thorough understanding of its workings and careful planning to avoid redundancy and achieve a seamless flow of data.
Multicasting refers to the networking process where a single data packet is sent from one point to multiple points simultaneously in a single transmit operation. It is a more efficient way of transmitting data when you need to send the same piece of information to many recipients. This method saves network bandwidth and reduces traffic, as the same data does not have to be transmitted separately.
In creating a multicast system, there are several key components involved:
- Source: The originator of a message that needs to be multicasted. - Multicast group: A set of destination nodes that are interested in receiving a particular message. - Network devices: Routers and switches that support multicast routing protocols to facilitate multicast communication.
From the perspective of a programming interface, the socket API provides the essential functions necessary for multicasting. For instance, programmers can use socket options like
IP_ADD_MEMBERSHIP
or
IP_DROP_MEMBERSHIP
to join or leave multicast groups respectively.
Let’s see how Python leverages the socket module to create multicast applications:
import socket import struct # Create a UDP socket sock = socket.socket(socket.AF_INET, socket.SOCK_DGRAM) # Join the multicast group group = socket.inet_aton('224.3.29.71') mreq = struct.pack('4sL', group, socket.INADDR_ANY) sock.setsockopt(socket.IPPROTO_IP, socket.IP_ADD_MEMBERSHIP, mreq) # Receive/respond loop while True: print('waiting to receive message') data, address = sock.recvfrom(1024) print('received %s bytes from %s' % (len(data), address)) print('sending acknowledgment to', address) sock.sendto('ack', address)
In this Python program, we create a simple multicast receiver that joins a group, listens for messages, and sends an acknowledgement back to the sender.
Multicasting improves efficiency and conserves system and network resources by requiring the source to send out a message only once, even if it has to be delivered to a large number of receivers. Examples of applications that can benefit from multicasting include live video broadcasting, corporate communications, and distributed games.
Apart from local area networks (LANs), multicasting is also crucial in content distribution networks (CDNs). They use IP multicast to stream high-bandwidth transmissions, such as live television or on-demand videos.
However, multicasting can pose its set of challenges. It requires a supportive infrastructure which understands multicast packets. These would include routers configured with multicast protocols and applications designed for multicast communications.
Overall, Multicasting is certainly seen as an efficient and resource-friendly solution for delivering data to multiple recipients. By implementing proper hardware support and designing multicast-aware applications, organizations can fully capitalize on the benefits that multicasting presents.[source]Multicasting is a networking protocol where data transmission is sent from one or multiple sources to many receivers. To put it in other terms, multicasting enables the distribution of information to multiple nodes on a network at once.
What does this mean? Consider this example:
public class BroadcastExample { public static void main(String[] args) { String message = "This is a multicast message"; System.out.println("Broadcasting \"" + message + "\""); // create an instance of multicast socket MulticastSocket socket = new MulticastSocket(); byte[] buffer = message.getBytes(); DatagramPacket packet = new DatagramPacket( buffer, buffer.length, InetAddress.getByName("224.0.0.1"), 4446 ); // Send the packet socket.send(packet); } }
As for the use of multicasting on your network, the main reason lies in its effective resource management. A few typical reasons include:
- Efficient data distribution: If the same piece of data needs to be sent to multiple destinations, multicasting consolidates the transmission into a single stream instead of sending individual packets to each client.
- Bandwidth consumption: Given its nature, multicasting reduces network traffic. This results in less bandwidth consumption, which can be crucial for networks that handle large amounts of data or have limited bandwidth capacity.
- Real-time applications: Real-time applications like video streaming or online gaming benefit from multicasting as it ensures real-time communication between the server and multiple clients.
To manage multicasting efficiently, various protocols are employed, some of which are:
- IGMP: Internet Group Management Protocol (IGMP) is used by hosts and adjacent routers to establish multicast group memberships. IGMP allows the router to learn about the presence of hosts belonging to a specific multicast group.
- MOSPF: Multicast Open Shortest Path First (MOSPF) is an extension of the OSPF protocol to support multicast routing.
- DVMRP: Distance Vector Multicast Routing Protocol (DVMRP) builds a multicast tree for each source and prunes branches with no members.
For more elaborate understanding about multicasting you can find it here.
Overall, while the implementation of a multicasting system might require a deep dive into network infrastructure and significant configuration efforts, the benefits it brings in process optimization and resource management make it a highly valuable asset in any substantial network setup.
Multicasting is an efficient method of network communication that allows data to be routed from a single source and delivered to multiple destinations. It presents a perfect blend between the other two conventional types of network communications: Unicast and Broadcast.
Comparison with Unicast:
Unicast communication, as the name suggests, is a scenario where one device directly communicates with another device on a network. The two devices establish a unique connection, creating a one-to-one relationship, making it suitable for tasks requiring direct interaction. However, this approach can become cumbersome and bandwidth-heavy when trying to send the same data from one point to multiple recipients.
Unicast | Multicast |
---|---|
One-to-One communication model; | One-to-Many or Few-to-Many communication model; |
Sends data separately to each recipient using separate connections; | Sends data once utilizing network infrastructure to reach multiple recipients; |
Bandwidth consumption increases linearly with number of recipients due to repeated transmission; | Economical use of bandwidth with only one data transmission no matter the number of recipients. |
In contrast, Multicast operates by sending out a single data stream to multiple recipients but without establishing individual connections for each. This efficiently mitigates the massive consumption of bandwidth characteristic of Unicast while effectively distributing data.
Comparison with Broadcast:
On the other hand, broadcasting in networking implies sending data packets to all possible points on a network – irrespective of whether the endpoints require the information or not. While its application can be beneficial in certain scenarios such as emergency broadcasts, it leads to unnecessary network traffic, bandwidth wastage and potential security risks as unrequested data landing at unintended points may reveal sensitive information unintentionally.
Broadcast | Multicast |
---|---|
All devices receive the broadcast message, whether needed or not; | Only specific subscribed devices receive multicast data; |
Wide-ranging impact leading to network congestion; | Controlled impact reducing network congestion; |
Potential for heightened security risks due to unrestricted data broadcast; | Minimal chance of unnecessary data exposure because of targeted data distribution. |
Whereas, Multicast optimizes these shortcomings by only sending the data to those sections of the network that contain interested recipients, thus saving on network resources and bolstering security.
So why would you want to use Multicasting on your Network? The core strength of multicasting comes into play when communicating large amount of data across a network infrastructure more efficiently. It facilitates streaming media services like IPTV, live event broadcasts, video conferencing, and distributed games, amongst other applications.
The effectiveness and utility of Multicasting, as outlined above, ultimately depends on the manner in which you need to transfer and distribute information within your network. Through selecting multicasting over unicast or broadcast, valuable network resources can be conserved, paving the way for faster, secure, and more effective data transactions[source].Sure, I’m going to delve into a meaty discourse about the Internet Group Management Protocol (IGMP), all the while keeping it anchored on our central topic: network multicasting.
Multicasting, in the realm of networking, is a method for transmission of data or information to multiple destinations elegantly and efficiently. Unlike unicast that sends data to a single recipient, or broadcast that indiscriminately sends to all, multicasting is adept at targeting a specific group within a network system.
Let’s dissect why you would want to use multicasting:
– Enhanced Efficiency: It steers away from sending individual copies of data to each host, which can significantly strain network resources. Instead, it dispatches a single flow of data which members of an appointed group clone.
– Better Bandwidth Usage: By eliminating the need for redundant packet streams, multicasting substantially reduces unnecessary congestion of network bandwidth.
– Improved Scalability: As it is agnostic to the number of recipients, multicasting can comfortably accommodate new members without needing proportionate additional resources.
Now, let’s reel in IGMP into this discussion. The Internet Group Management Protocol (IGMP) is an integral inner-workings in the implementation of the IP multicasting. Its primary role is to enable hosts to report their multicast group memberships to any neighboring multicast routers. Essentially, it’s the protocol facilitating the communication between endpoints (hosts) and neighboring routers so that the latter knows to which group they have to deliver certain multicast traffic.
The following
IGMP message types
exist:
IGMP Membership Query, IGMPv1 Membership Report, IGMPv2 Membership Report, IGMPv2 Leave Group, IGMPv3 Membership Report.
Let’s visualize the interaction with a basic table:
Hosts (Endpoints) | IGMP Protocol | Routers |
---|---|---|
Wants to receive Multicast X | Sends IGMP Join Message | Starts Sending Multicast X |
No longer need Multicast X | Sends IGMP Leave Message | Stops Sending Multicast X |
Imagine the multicast packets as specific TV channels, the hosts as viewers, and the IGMP protocol as your cable provider. When you (host) want to watch a show (multicast), you send a request (IGMP join) to your cable provider (IGMP) who then starts broadcasting the channel (router sending multicast). When you are done, you change the channel (send an IGMP leave), and your screen displays the new channel (router stops sending multicast).
Referencing open-source libraries for IGMP protocols can be beneficial to see real-world examples. For Python developers, Scapy library offers functionalities to deal with network protocols like IGMP. Below is an example of how to create an IGMP packet using Scapy [source].
from scapy.all import * p = IP(dst="224.0.0.1")/IGMP()/('x'*60000) send(p)
To conclude, IGMP is the unsung hero crafting behind-the-scenes magic for efficient data dissemination using multicasting. The advantages offered by multicasting position it as an indispensable tool for many bots including video streaming services, multimedia applications, and IoT deployment involving mass device communication. With IGMP, we can ensure that these tools operate in a harmonized environment permeating optimal resource utilization.Navigating through the labyrinth of network management isn’t for the faint-hearted. Among its many features, one that stands out and deserves attention due to its sheer practicality is multicasting. Essentially multicast is a network communication mode where data is sent from a single source to multiple destinations simultaneously. This act of data transmission is done using optimized retransmission of information packets[1](https://techterms.com/definition/multicasting).
To comprehend why you would want to use multicasting on your network, picture this. You’re administering a network where a massive file needs to be shared amidst ocean-sized heaps of nodes. Instead of distributing this file individually to each node (unicasting), or broadcasting it to every component within the network regardless of their need or want of it, you can simply multicast the data to a selective group of receivers. Understandably, the bandwidth saved from avoiding unnecessary data duplication in such procedures helps make more efficient use of network resources.
Now let’s venture into the plethora of supervision tools necessary for effective multicasting. Unlocking exceptional performances in broadcasts requires brainy monitoring software. Here are some tools crafted for exactly that:
Software | Description |
---|---|
1. SolarWinds Network Performance Monitor | Stuffed with an array of customizable network metrics and dashboards, this tool presents network metrics in a visually stimulating manner, making tracking multicast group connections a cakewalk. |
2. PRTG Network Monitor | Equipped with over 200 sensors, including dedicated multicast sensors, this utility keeps a sharp eye on traffic, packet loss, and errors in real-time, offering overall improved network visibility. |
3. Nagios XI | An extensive monitoring tool that provides detailed network, server, and application monitoring. Its quick alert system impressively keeps multicast discrepancies at bay. |
4. Zabbix | A free open-source tool that monitors various network parameters like speed, volume, packet loss, etc. It allows administrators to promptly detect barriers that could hamper multicasting efficiency. |
For a closer look into the execution of multicasting, we can consider Multicast Send-Receive in Python as an example. Let’s examine a sample script that initializes a multicast sender and receiver by defining a multicast group and corresponding port:
import socket import struct MCAST_GRP = '224.1.1.1' MCAST_PORT = 5007 # create a UDP socket sock_sender = socket.socket(socket.AF_INET, socket.SOCK_DGRAM, socket.IPPROTO_UDP) # allow multiple sockets to use the same PORT number sock_sender.setsockopt(socket.SOL_SOCKET, socket.SO_REUSEADDR, 1) sock_receiver = socket.socket(socket.AF_INET, socket.SOCK_DGRAM, socket.IPPROTO_UDP) sock_receiver.setsockopt(socket.SOL_SOCKET, socket.SO_REUSEADDR, 1) # bind to the port that we know will receive multicast data sock_receiver.bind((MCAST_GRP, MCAST_PORT))
The broadcasted data reaches all specified members of the group, ensuring the data has been effectively multicast.[2](https://docs.python.org/3/library/socket.html)
Summarily, in a hard-wearing digital era, using multicasting along with the right supervision tools is akin to saving a great deal of time, energy, and resources. These tools provide deep insights into multicast workflows, helping enhance multicast performance while ensuring smooth, uninterrupted data flow across the network.
The challenge of slicing broadcasting networks can be effectively addressed by implementing multicast technology. Multicasting offers particular benefits for network efficiency and scalability that could be pivotal for broadcasting networks.
Multicast: An Overview
Multicast is a method of routing data in a network that allows a single sender to send information to multiple recipients simultaneously. Rather than sending individual packets to each recipient, as is the case with unicast delivery, multicast allows for the dispatching of a single stream of data that is distributed across an entire group.
Multicasting resembles how broadcast television works; a station sends out one signal, which can be picked up by any television tuned into the station’s frequency. Potentially, thousands or even millions of televisions can pick up the signal without affecting the quality or bandwidth of the broadcast.
Multicasting utilizes
Internet Group Management Protocol (IGMP)
to manage the formation and dissolution of these multicast groups. This is done to ensure efficient utilization of resources and effective management of network traffic.
Why Use Multicasting on Your Network
Broad adoption of multicasting in your network infrastructure to handle slice broadcasting has several advantages:
- Network Efficiency: With multicast transmission, slices will be sent over each network link only once, instead of multiple times. Your network therefore becomes less congested, improving overall efficiency.
- Scalability: Using multicasting, there’s virtually no limit to the number of receivers in a group. As more devices need to receive broadcasts, your network can easily scale to accommodate them without additional overhead cost.
- Reduction of Latency: By sending out a single stream of information, as opposed to multiple separate streams, multicasting reduces the latency often associated with broadcasting.
Implementing Multicast for Slice Broadcasting
To maximize efficiency in a broadcasting network setup, consider this simplified procedure of applying multicast:
├── Define the multicast groups.
├── Set up the multicast routing protocol.
└── Enable IGMP snooping on your switches.
Please note that this is a high-level overview. In practice, proper implementation would require careful planning and configuration based off the specifics of your network topology and requirements.
A multicast-enabled network provides a powerful solution to address broadcast slice sharing. While it involves considerable upfront design work and ongoing maintenance, the improvements in efficiency, scalability, and latency reduction offer clear, ongoing payoffs in terms of system performance and flexible capacity to handle growth and change.
More detailed information about multicasting can be found in Cisco’s IPv4 Multicast Configuration Guide. Solutions like F5 BIG-IP are available to help simplify your multicast infrastructure, offering robust, enterprise-scale solutions for managing multicast operations.Multicasting is a method of distributing network packets to multiple receivers simultaneously, often used to distribute live video and audio broadcasts or real-time data streams. This contrasts with traditional unicast methods that send individual copies of data streams to each recipient.
So why would you opt for multicasting? Here are some compelling reasons:
Efficiency
Since multicasting uses the same data stream for everyone in the multicast group, it saves a tremendous amount of bandwidth compared to unicasting. In unicast distribution, each receiver must establish a separate connection with the sender, resulting in significant duplication of traffic across your network. But by using multicasting, you’re effectively hosting one ‘meeting’ that many ‘attendees’ can join, thereby avoiding this unnecessary replication.
If you imagine a situation where an organization streams a live corporate event to thousands of employees, without multicasting there would be thousands of identical data streams transmitting the same content—every second. With multicasting, there’d only be one stream, with recipients simply subscribing to it. As such, networkworld reports that it significantly reduces network load and conserves bandwidth.
Scalability
The fact that multicasting delivers a single stream of data to many subscribers simultaneously allows it to reach potentially limitless nodes without increasing network or resource load beyond that single stream. This scalability means it can serve an ever-growing number of recipients without compromising on performance or quality-of-service.
Speed and Performance
Efficiency translates to speed too. Multicasting addresses overcome the bottleneck issue commonly found in unicast streaming (miensfeld).ke having to deal with a multitude of TCP connections would dramatically increase network congestion, slow down delivery and degrade overall performance.
Allow me to demonstrate the simplicity of multicasting with a Python code snippet:
import socket # Declare multicast group address and port multicast_group = ('224.3.29.71', 10000) # Create UDP socket sock = socket.socket(socket.AF_INET, socket.SOCK_DGRAM) # Send data to all who joined the group sock.sendto(b'Hello, multicast group!', multicast_group) sock.close()
In the above example, any device on the network running a corresponding piece of code to join the multicast group would receive the message. The device doesn’t need to be specifically addressed. This elegantly demonstrates how efficient and simple multicasting is.
To summarize, while multicasting may initially appear complex, understanding its underlying principle reveals its true value. It is more efficient, faster, and less resource-intensive than traditional unicasting, especially in scenarios that involve large-scale data transmission like live video or audio broadcasting. Thus, choosing multicasting for your system translates into substantial savings in network resources and better overall performance—a major advantage in today’s data-hungry world.
Network multicasting refers to the transmission of an information packet to multiple destinations in a single send operation. It is typically contrasted with unicasting, which uses separate connections for each recipient, and broadcasting, which sends data to everyone in the network regardless of whether they need it or not. Multicasting uses less bandwidth compared to sending individual copy of data bundle separately (unicasting) by finding users who want to receive the data and creating an efficient path to deliver only one copy of that data to all those users.
# Example code showing joining a multicast group import socket import struct MULTICAST_GROUP = ('224.0.0.1', 10000) sock = socket.socket(socket.AF_INET, socket.SOCK_DGRAM) sock.bind(('', MULTICAST_GROUP[1])) group = socket.inet_aton(MULTICAST_GROUP[0]) membership = struct.pack('4sL', group, socket.INADDR_ANY) sock.setsockopt(socket.IPPROTO_IP, socket.IP_ADD_MEMBERSHIP, membership) while True: print(sock.recv(1024))
The applications of network multicasting are found in where there is the necessity to be efficient in communication and distribution of information such as:
- Internet Protocol Television (IPTV): IPTV providers use multicasting to send the same video content via live-streaming to numerous users simultaneously which drastically cuts down on bandwidth utilization.
- Video Conferencing: In video conferencing, multicasting allows the simultaneous transmission of video feeds to multiple recipients without having to replicate the feed for individual connections. This is used heavily in corporate and educational scenarios.
- Network News Broadcast: An excellent example is RSS feeds that broadcast updates and content to numerous users without the need for multiple transmissions.
- Software updates: Companies like Microsoft employ multicasting to distribute software updates. A definitive example would be the Scale-Out File Server for application data, where Windows server uses multicast to serve multiple clients requesting access to the same file. The clients may then cache their own copies of the file for subsequent reads.
Hence, Network multicasting provides a smart and efficient method for content delivery in real-time scenarios on your network. It leverages the principle of simultaneous data dispatch to multi-users while ensuring optimal bandwidth utilization – making it a preferred choice for operations involving bulk data transmission to multiple receivers.
<section id="packet_loss"> <p>As a professional coder, you need to understand both the theoretical and practical aspects of networking concepts. This includes the concept of packet loss and its effects on networks leveraging multicasting. </p> <p>Packet loss essentially happens when one or more packets of data traveling across a computer network fail to reach their destination, potentially causing disruptions in communication. Multicasting is a networking protocol where a single data transmission is copied and sent to a group of destination computers simultaneously. Both packet loss and multicasting are crucial components of network communication. However, they can impact each other considerably.</p> <h2>Implications of Packet Loss on Multicasting</h2> <table> <thead> <tr> <th>Implication</th> <th>Explanation</th> </tr> </thead> <tbody> <tr> <td>Decreased Efficiency</td> <td>Excessive packet loss can degrade the overall efficiency of a multicast network. If a significant portion of data doesn't make it to the intended destinations, the retransmissions would essentially render the benefits of multicasting null.</td> </tr> <tr> <td>Increased Latency</td> <td>With high packet loss rates, re-transmission attempts increase, leading to high latency and causing delays in the network service. This could be problematic, especially for real-time services like video conferencing or online gaming that depend heavily on multicasting.</td> </tr> <tr> <td>Reduced Reliability</td> <td>A multi-cast protocol typically does not provide any form of acknowledgment for received messages. So, the sender cannot definitively know whether all intended recipients have received the message, potentially resulting in an unreliable service.</td> </tr> </tbody> </table> <h2>Why Use Multicasting?</h2> <p>Despite these implications, multicasting has many applications and provides several benefits, including:</p> <ul> <li>Efficient network resource usage: It reduces the amount of bandwidth required by sending data to multiple recipients simultaneously instead of individually.</li> <li>Time saving: Multicasting saves time as it allows for the simultaneous distribution of information across the network without the need for separately addressed packets for each recipient.</li> <li>Scalability: Multicasting supports expanding network capabilities since it can handle a growing number of nodes (devices and endpoints) efficiently.</li> </ul> <p>Although packet loss might pose some challenges to multicasting, the use of robust network monitoring tools, efficient routing strategies, and appropriate equipment can mitigate these issues, thus allowing organizations to reap the benefits of multicasting.</p> <p><a href="https://www.icann.org/resources/pages/multicast-2013-05-03-en" target="_blank">Reference</a></p> </section>
Multicasting is a networking protocol where information is sent to multiple destinations at once without added bandwidth strain. It is useful in instances such as video conference meetings, live footage broadcasting or real-time system wide updates in a network of systems, facilitating simultaneous data delivery to numerous recipients.
Understanding how multicasting works sets an ideal basis for exploring the security aspects related to its implementation. Let’s consider some important points.
Security Breaches:
Network multicasting offers an alluring route for cyber-attack vectors since it broadcasts data across multiple nodes at once. A compromised data transmission can lead to widespread system vulnerability. For instance, multicast traffic does not provide authentication by default and could allow malicious individuals to listen to communications meant for specific subscribers.
// Example demonstrating a potential attack vector // Assume we have a multicast group that shares confidential communication socket.joinGroup("224.0.0.1"); // Joining a group ... socket.receive(packet); // Receiving data packets
Countermeasure – Authentication and Encryption:
Including authentication mechanisms for group communications can mitigate unauthorized access risks. Also, encrypting data before transmitting over the network provides a deterrent against sniffing attacks.
// Example with authentication and encryption // The following are high level notions rather than concrete code Authenticate user; // Ensures user is authorized Encrypt data; // Encrypts data before sending socket.send(packet); // Sending encrypted data
Unauthorized Multicast Registrations:
An entity might register itself to irrelevant multicast groups posing threats. A cyber-criminal could exploit this to disseminate harmful instructions or data across this undeserving audience.
Countermeasure – Access Control Lists (ACLs):
Setting up ACLs on your routers can prevent unauthorized entities from joining multicast groups thereby protecting your systems from inappropriate transmissions.
// Again, the following are high-level notions Set up ACL for router; Grants permission to authorized entities; Restricts permission to unauthorized entities;
Violating Network Policies:
Multicasting may inadvertently violate existing network policies leading to unnecessary traffic or latency issues which may compromise overall network performance.
Countermeasure – Proper Oversight and Monitoring:
Conducting regular audits and implementing strong monitoring mechanisms ensures adherence to network policies.
// High-level description Monitor multicast traffic; Check irregular activities; // Unusual spikes or patterns Audit regularly; // Significant policy deviations
A table highlighting these considerations and countermeasures:
Consideration | Countermeasure |
---|---|
Security Breaches | Authentication and Encryption |
Unauthorized Multicast Registrations | Access Control Lists (ACLs) |
Violating Network Policies | Proper Oversight and Monitoring |
You can use different measures including but not limited to the above recommendations for securing your multicast implementations. The choice majorly depends on your specific environment and use case.
Using multicasting on your network allows you to efficiently share resources, promoting faster application deployment and reduced network load. Nonetheless, it brings attention to certain security risks (source). Therefore, to enjoy the benefits without falling victim to the associated vulnerabilities, have a comprehensive risk mitigation plan in place. Make sure to monitor for any abnormal behavior while keeping the infrastructure updated against the current threat landscape.
If you’re utilizing multicasting on your network, it implies that you’re transmitting data from a single source to multiple destinations. You’d typically use this approach in instances where the same data needs to be sent out to numerous receivers simultaneously. Examples include live streaming events (where the event needs to be transmitted to multiple users at once) and IPTV distribution.
However, while multicast networks offer significant benefits in terms of bandwidth utilization and processing power, they do come with their own sets of challenges:
Challenge 1: Congestion and Latency
One common issue is congestion which can cause latency. This occurs when too many receivers are attempting to access the same content simultaneously. It becomes difficult for routers to keep up with demand and ensure timely delivery.
Challenge 2: Lost Packets
Lost packets are also a key concern. In multicasting, the ‘send once, receive many’ nature means that if a data packet goes missing, all receivers miss out. And, unlike unicast protocols, there are no automatic retransmission mechanisms in place.
Challenge 3: Complex Configuration and Management
Management of multicast groups can be complex, as it involves configuration on both senders and receivers end.
<sender></sender> <receiver></receiver>
This further requires programming skills to handle this complexity.
While these challenges might initially seem like barriers, they can be effectively managed with proper planning and strategies:
For Congestion and Latency, one solution involves properly segmenting your network, placing a limit on membership for each multicast group and ensuring you maintain sufficient bandwidth reserves.
In the case of Lost packets, a protocol called “Reliable Multicast Protocol” or “RMP” can be used. This protocol allows for error recovery by requesting that missing data packets be resent.
When it comes to the Complex Configuration and Management challenge, savvy network administrators will leverage tools like IGMP snooping and make smart use of an Internet Group Management Protocol (IGMP) that simplifies the management of devices joining and leaving a multicast group.
Each step taken to address these challenges ensures stability in the communication process. They allow for more effective network scalability and performance under a variety of conditions.
Multicasting as a mechanism is extremely useful for network communication. With its ability to conserve system resources and provide simultaneous information dissemination, it remains an impactful part of today’s networking environment, provided that its inherent challenges are well-managed.
Here’s a simple table outlining the challenges and their respective solutions:
Challenges | Solutions |
---|---|
Congestion and Latency | Properly segment your network and limit group membership |
Lost Packets | Use Reliable Multicast Protocol (RMP) |
Complex Configuration and Management | Leverage IGMP snooping and Internet Group Management Protocol (IGMP) |
To understand more about multicasting and its practical applications, feel free to refer to this detailed guide here.
Further reading on the challenges of multicasting and how to tackle them can be found here.
Note: HTML tags such as <pre>, <a href>, <table> and others have been showcased to convey formatting but aren’t actual code. For the actual usage of these tags, please follow standard HTML syntax.Multicasting, a critical concept in network communications, leverages the functionality of one-to-many data distribution model. This model holds an edge when there is a need to transmit the same information simultaneously to multiple recipients, eliminating the requirement for duplication and economization of bandwidth. Networks like IPTV systems, video conferencing software, etc, use multicasting.
The core concepts that lay a strong foundation for multicasting include:
- An Addressing scheme: The addressing model implements IP address scopes in multicasting. - Traffic control: Routers and switches manage this aspect by controlling the data packets delivery. - Monitoring: Ensures the network's overall performance and reliability.
Do you perceive this to be complicated? Then let me simplify it. Consider a scenario where a teacher needs to give the same homework assignment to all his students. Instead of telling each student individually, he will provide it at once in class. That’s what multicasting does to your network. It transmits the same data package to several destinations simultaneously. You would use it on your network to deliver IPTV, live video broadcasts, or online multiplayer games.
To further optimize your SEO results related to multicasting, considering using specific keywords such as “network communication”, “multicasting”, “one-to-many data”, “traffic control”, and others that blend seamlessly with your content about multicasting.
As we talk about the importance of multicasting, remember terms such as IPv4, multicast groups, data efficiency, bandwidth optimization, and ethernet switches. These are crucial cogs within the whole mechanism of multicasting, making it invaluable for efficient network communication.
For more expansive articles and a deeper dive into the technical aspects, forums, discussion pages, blogs, and scholarly articles are adequate resources1. Online courses also offer structured lessons covering from basics to complexities2.
Finally, always remember; while coding forms a significant part of network communications and multicasting, understanding these concepts and their implementation are just as important. For instance, here’s a Python code for sending data to a multicast group:
import socket message = "Hello Multicast Group!" multicast_group = ('224.3.29.71', 10000) sock = socket.socket(socket.AF_INET, socket.SOCK_DGRAM) sock.sendto(message.encode('utf-8'), multicast_group)
This piece of Python script connects to a multicast group and conveys a simple message to all devices linked to the said group. By using multicasting on your network, not only do you conserve network resources, but also ensure efficient real-time data transmission, rendering multicasting a truly empowering tool.3. Clearly, to create expansive, interactive domains, multicasting forms one of the fundamental building blocks, one you might want to leverage for better networking solutions.4.