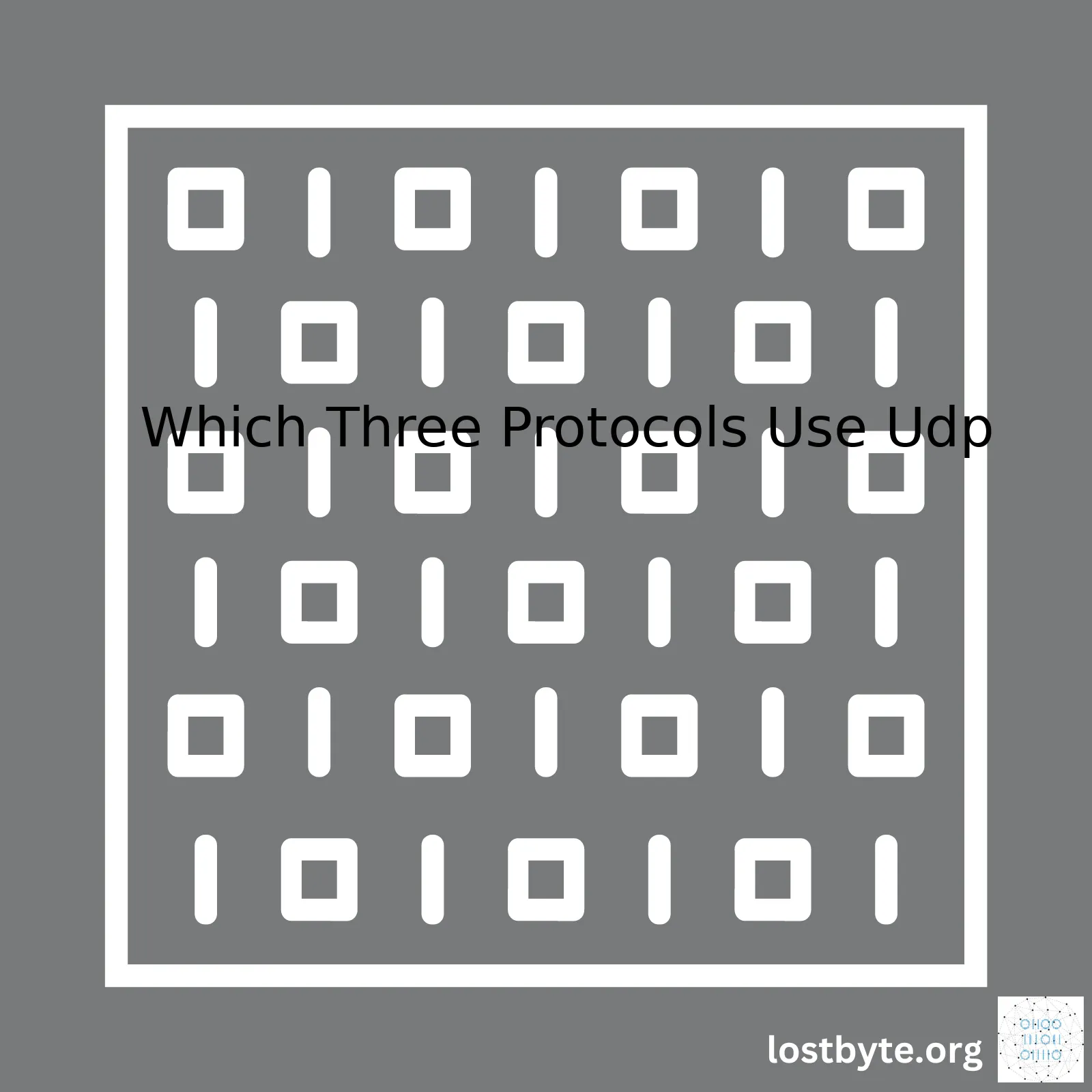
Protocol | Description |
---|---|
DNS (Domain Name System) | This protocol translates domain names to IP addresses. |
DHCP (Dynamic Host Configuration Protocol) | DHCP is used to automatically assign IP addresses and other network configuration to devices. |
VoIP (Voice over Internet Protocol) | VoIP allows voice and multimedia communication via the internet. |
The User Datagram Protocol (UDP) serves as a key component for several protocols visible in modern computing. Among these are DNS, DHCP, and VoIP.
// Here is a basic node.js code snippet showing usage of udp through dgram module var dgram = require('dgram'); var server = dgram.createSocket('udp4'); server.on('message', function(msg, rinfo) { console.log('Received %s from %s:%d', msg, rinfo.address, rinfo.port); }); server.bind(61234);
DNS (Domain Name System) relies on UDP for quick single transmission based communication, excellent for translating domain names to IP addresses. Unlike TCP/IP which needs to establish a connection before data transfer, UDP is faster since it just sends the packets immediately.
DHCP (Dynamic Host Configuration Protocol) also utilizes UDP’s speed and connectionless nature to dynamically assign IP addresses to devices in a network. The ‘connectionless’ nature of UDP makes it especially useful in situations where you don’t need a permanent connection. For instance, when a device connects to a network and needs IP configuration information. It sends a request to DHCP server and eventually receives what it needs without further communication.
Here we got an example of DHCP usage:
// Example of DHCP request using node-dhcp var dhcpd = require('node-dhcp'); const { DHCP } = require("node-dhcp"); const s = new DHCP.CreateServer({ range: [ "192.168.3.10", "192.168.3.99" ], forceOptions: ['hostname'], // Options that client is supposed to requested by default netmask: '255.255.255.0', router: [ '192.168.0.1' ], dns: ['8.8.8.8'], });
On the other side, VoIP (Voice over Internet Protocol), known broadly for providing telephone service via internet, appreciates UDP’s real-time data delivery features. While it may miss some packets during transmission, its real-time capabilities and low latency make it the optimal choice for voice and video streaming where a second or two delay might alter the whole user experience.
Thus, DNS, DHCP, and VoIP effectively encapsulates the potential of UDP in different applications – Domain Name resolution, Dynamic IP assignment, and Real-Time communication, respectively. Each one making the most out of UDP’s unique advantages while working around its limitations.
For further reading:
Firstly, let’s understand what UDP protocol is. ‘UDP’, which stands for User Datagram Protocol, is one of the principal protocols in the Internet standard suite. It provides a simple but reliable way to send data packets over an IP-based network. Now, when it comes to the protocols that specifically use UDP, we have three main contenders:
– Domain Name System (DNS)
– Simple Network Management Protocol (SNMP)
– Dynamic Host Configuration Protocol (DHCP)
The Role of UDP in DNS
The Domain Name System (DNS) performs a crucial function on the Internet by converting human-friendly domain names into IP addresses. Typically, a DNS query has a small payload, therefore, a connectionless protocol like UDP suffices.
Example of a typical DNS query using UDP:
dig www.google.com
Notice how this command retrieves the IP address associated with www.google.com without needing to establish a prior connection?
The Role of UDP in SNMP
The Simple Network Management Protocol (SNMP) – used primarily for managing devices on IP networks – also relies on UDP. While it could technically employ TCP for enhanced reliability, it typically doesn’t due to its additional overhead and complexity. SNMP mostly sends short messages, usually from a manager device querying an agent device about its status or configuration.
Here’s an example how SNMP uses UDP:
snmpwalk -v2c -c public 192.0.2.1
This walks through the Management Information Base (MIB) of a device at IP 192.0.2.1 using version 2 of SNMP and a community string (password) of ‘public’, all over UDP.
The Role of UDP in DHCP
Finally, the Dynamic Host Configuration Protocol (DHCP) utilises UDP as its transport protocol. DHCP’s purpose is to assign network parameters like IP addresses to devices on a network. When a device first connects to the network, it broadcasts a DHCP DISCOVER message on UDP port 67. When the server receives this, it responds to the client with a DHCP OFFER message on UDP port 68, offering network settings.
Here is an illustration of how DHCP functions using UDP:
Step | Action | UDP Port |
---|---|---|
1 | Client sends DHCP DISCOVER | 67 |
2 | Server responds with DHCP OFFER | 68 |
In summary, several protocols, especially those dealing with simple or small payloads, rely on UDP for transportation. DNS, SNMP, and DHCP are significant examples showcasing the use and efficiency of UDP in different networking scenarios. They signify the importance of understanding UDP and highlight why it’s still widely used despite the advent of other transport protocols.Delving deep into this subject matter, it’s critical to realize that the User Datagram Protocol (UDP) is a fundamental Internet protocol wherein data is transmitted over an IP network. This transport layer protocol draws substantial preference when it comes to speed of transmission irrespective of reliability in delivery. Consequently, multiple protocols leverage UDP largely because of its low-latency, and connectionless nature.
Let’s explore three well-known protocols utilizing UDP:
DNS (Domain Name System),
DHCP (Dynamic Host Configuration Protocol),
and VoIP (Voice over Internet Protocol).
1. DNS (Domain Name System):
DNS is considered the phonebook of the internet, essentially converting human-readable hostnames like ‘www.google.com’ into their equivalent IP addresses. It works on a simple request-response scenario where when requests are sent, you receive an IP address in response. Interestingly, DNS mainly runs on UDP because of its reduced overhead and speedy data transfer characteristics. Here’s a basic example of how a DNS query might be performed programmatically:
import socket socket.gethostbyname('www.google.com') # this returns the IP address for google.com
Explore more about DNS via this online documentation link: https://tools.ietf.org/html/rfc1035
2. DHCP (Dynamic Host Configuration Protocol):
Bootstrapping any TCP/IP network necessitates IP address configuration. That’s where DHCP steps up to bat, providing dynamic IP address assignment ensuring IP addresses are used efficiently. The utility of UDP in DHCP can be realized from two angles; firstly, DHCP requests might be broadcasted to multiple servers. Secondly, since DHCP functions even before a system has an IP address, a handshake (which TCP requires) becomes improbable.
The following tables illustrate a simplified sequence of events happening during an automatic configuration process by using DHCP:
Client | Server |
---|---|
DHCPDISCOVER | |
DHCPOFFER | |
DHCPREQUEST | |
DHCPACK |
Details on DHCP could be found here: https://tools.ietf.org/html/rfc2131
3. VoIP (Voice over Internet Protocol):
VoIP references a plethora of communication protocols facilitating voice communications and multimedia sessions over the internet. As real-time services, these highly latency-sensitive tasks depend largely on UDP as its main transport protocol. Unlike the retransmission characteristic of TCP, which introduces undue latency and jitter, UDP enables quick, continuous voice packet delivery even if some packets fail to land noticeably. A popular example of VoIP is Skype.
An example of a VoIP raw audio data transmission might look something like this:
# consider "s" as the instance of socket creation with UDP raw_audio_data = get_raw_audio_data() # represents function that retrieves raw audio data s.sendto(raw_audio_data, ('', )) # sends the raw audio data to specified IP address
To dive into VoIP further, click on: https://tools.ietf.org/html/rfc3550
Thus, despite certain inherent limitations of UDP like no acknowledgment, no congestion control, and no retransmission, the propensity for swifter transmissions makes it favorable for these three protocols, amongst others.There are numerous benefits to utilizing UDP-based protocols – mainly speed, simplicity, and real-time delivery. While this is generally appealing, it’s important to know about specific protocols that use this transport protocol and when they are most useful.
User Datagram Protocol (UDP)
UDP
is a communication protocol used across the Internet for time-sensitive transmissions such as video playback or DNS lookups. It speeds up communications by not waiting for the recipients of packets to acknowledge receipt before sending the next packet. This is known as an “unreliable” protocol because it doesn’t guarantee delivery or check sequencing.
Benefits of UDP
- Speed: Since UDP doesn’t need to establish a connection before sending data and doesn’t care about the sequence of data packets, it results in quicker data transmission.
- Real-Time Performance: Time-consuming error-checks aren’t significant here. Real-time videos or games require high speed which is achieved by using UDP.
- Simplicity: UDP applications are simple to write due to fewer protocol requirements. Applications or services can manage error checks on their own.
DNS, VoIP, and DHCP – Three UDP Protocols
DNS (Domain Name System): DNS allows your computer to figure out where a server exists in the world, based on its domain name. Speed is crucial for DNS queries, and the overhead of establishing connections or error-checking may delay responses, making UDP preferred over TCP.
nslookup www.example.com
VoIP (Voice over Internet Protocol): VoIP is crucial for real time communication systems like Internet telephony. Just like a normal phone call, people don’t want long waits or repeats just because a few sound bits went missing. UDP handles these inconsistencies efficiently as it allows for packet loss – a few lost packets of audio won’t ruin the entire conversation. An example of a VoIP system is Skype.
SIP INVITE sip:receiver@domain.com SIP/2.0
DHCP (Dynamic Host Configuration Protocol): DHCP assigns IP addresses dynamically within a network so that devices can use reliable IP addresses. With DHCP, rebooted or newly created devices can join the network without manual IP configuration. A dedicated connection for IP assignment isn’t required, making UDP suitable.
ipconfig /release ipconfig /renew
These three protocols reflect well how UDP gives us superior speed, simplicity, and real-time performance in different scenarios. Understanding them helps us choose the right tool for each job and appreciate the versatility built into the networks we use every day.Certainly! Let’s talk about the three important protocols that make use of User Datagram Protocol (UDP):
1. Domain Name System (DNS)
2. Simple Network Management Protocol (SNMP)
3. Voice over IP (VoIP)
Domain Name System (DNS)
The DNS protocol uses UDP when it wants to quickly look up an IP address associated with a domain name (RFC 1035). Upon receiving a query, the DNS server responds with an answer, typically an IP address corresponding to the requested domain name.
For quick interaction like this, TCP would be an overkill because TCP involves more overhead. So UDP is favored for DNS due to its simplicity and speed.
A pseudo-code example for a DNS lookup using UDP may look something like:
import socket s = socket.socket(socket.AF_INET, socket.SOCK_DGRAM) server_address = ('localhost', 53) message = 'example.com' s.sendto(message.encode(), server_address) data, server = s.recvfrom(4096) print ('Received:', data) s.close()
The above code essentially sends a domain name to a DNS server and reads the received response, which should be an IP address.
Simple Network Management Protocol (SNMP)
SNMP is widely used in network management systems to monitor and manage devices across a network. Since network monitoring requires many requests, UDP’s stateless nature makes it ideal for SNMP (source).
An example of SNMP communication coded using UDP would involve sending GetRequest, SetRequest, and GetNextRequest operations to SNMP agents on network devices. This can be pictured as follows:
from pysnmp.hlapi import * for (errorIndication, errorStatus, errorIndex, varBinds) in nextCmd(SnmpEngine(), CommunityData('public'), UdpTransportTarget(('demo.snmplabs.com', 161)), ContextData(), ObjectType(ObjectIdentity('SNMPv2-MIB', 'system'))): if errorIndication: print(errorIndication) else: if errorStatus: print('%s at %s' % (errorStatus.prettyPrint(), errorIndex and varBinds[int(errorIndex)-1][0] or '?')) else: for varBind in varBinds: print(' = '.join([x.prettyPrint() for x in varBind]))
Voice Over IP (VoIP)
VoIP applications, like Skype or Discord, utilize UDP since they require low latency connections. Delay in transmission could result in poor call quality or connection issues on VoIP calls. The acceptability of packet loss makes UDP a good choice for VoIP (source).
Here is a simplified representation of how a VoIP call might work:
import socket # Sender side sock_sender = socket.socket(socket.AF_INET, socket.SOCK_DGRAM) voip_data = "Hello, this is a VoIP call" sock_sender.sendto(voip_data.encode(), ("localhost", 5005)) # Receiver side sock_receiver = socket.socket(socket.AF_INET, socket.SOCK_DGRAM) sock_receiver.bind(("localhost", 5005)) data, addr = sock_receiver.recvfrom(1024) print("Received VoIP Call:", data.decode())
By reviewing these real-world applications of UDP within such significant protocols, it becomes easier to appreciate the versatility and efficiency of UDP in various networking scenarios.
The User Datagram Protocol (UDP) provides an alternative to Transmission Control Protocol (TCP) for the delivery of packets over a network. The protocols that commonly use UDP include Domain Name System (DNS), Simple Network Management Protocol (SNMP), and Real-Time Transport Protocol (RTP).
UDP packet structure: - Source port: 16 bits - Destination port: 16 bits - Length: 16 bits - Checksum: 16 bits
1. Domain Name System (DNS)
In DNS, resolving IP addresses from domain names is an operation where speed is crucial. Therefore, it leverages UDP’s less formally structured nature to deliver quicker results.
For instance, when you type in a URL into your browser, the associated DNS queries must be answered promptly so as not to delay the rendering of the webpage. If the TCP protocol was used in place instead, the process would involve setting up a connection, transmitting data, and then closing the connection—significantly lengthening the wait time.
However, using UDP brings about some challenges:
– Possibility of Lost Packets: In UDP, there is no confirmation about whether the recipient received the data, leading to potential lost packets without any notification or retransmission attempts.
– Limited Data Size: UDP-based DNS queries are susceptible to size limitations. For larger payloads, more complicated mechanisms like TCP usage and EDNS extensions are required. These can increase complexity and lead to possible interoperability issues.
2. Simple Network Management Protocol (SNMP)
SNMP uses UDP because of its simplicity and lower resource requirements compared to TCP. SNMP mainly focuses on monitoring and management tasks like gathering statistics from various network devices, which demands a reliable but straightforward communication channel.
Challenges with implementing SNMP through UDP are:
– Security Concerns: SNMP offers limited security features. The third version introduces some fundamental security measures, but full-scale cybersecurity still requires supplemental measures.
– Unreliable Delivery: Similarly to DNS, SNMP knows nothing about the fate of the packets sent out due to UDP’s unreliability. It influences error handling and might lead to missing critical management information.
3. Real-Time Transport Protocol (RTP)
RTP is designed for end-to-end real-time transport of audio and video files over multicast or unicast network services. RTP chose UDP as it provides speedy data delivery without much concern about data loss—which is acceptable in real-time media streaming.
But the challenges around RTP’s implementation are:
– Data Loss: In the context of real-time media, data loss due to UDP’s unreliability can affect playback quality resulting in stutters and lags in transmission.
– Timing Issues: UDP lacks a built-in mechanism for packet sequencing, causing difficulties in managing packet order at the receiver end, potentially disrupting the timely delivery of media content.
Implementing these three protocols – DNS, SNMP, and RTP – leveraging UDP, can present certain challenges, yet offer unique advantages suitable to each protocol. Detailed knowledge about the UDP protocol and well-planned strategies can mitigate these challenges significantly.The Transmission Control Protocol (TCP) and the User Datagram Protocol (UDP) are two of the most commonly used protocols on the internet. Each one has its own advantages and disadvantages, and they are utilised in different situations for optimal performance.
- Transmission Control Protocol (TCP): It is a type of Internet Protocol (IP) communication method that provides a reliable, ordered, and error-checked delivery of a stream of packets between applications running on hosts communicating over an IP network. This mainstay of the web is stateful, i.e., it keeps track of all individual sessions.
- User Datagram Protocol (UDP): As opposed to TCP, UDP operates without session establishment – depicting a “fire and forget” mechanism. This stateless protocol is suitable for purposes where error checking and correction are either not necessary or performed in the application; thus eliminating the need for such at the network interface level.
Three examples of UDP-using Protocols include Domain Name System (DNS), Voice over IP (VoIP), and Trivial File Transfer Protocol (TFTP).
Domain Name System (DNS)
DNS translates domain names to IP addresses so browsers can load Internet resources. Its key characteristics pertinent to protocol selection are:
- The size of data transferred is usually very small – contained in one data packet.
- A settled connection isn’t fundamentally essential, as the requests are discrete and not relationally dependent.
Notably, DNS mainly uses UDP because typically the transaction fit into one packet and as such, TCP’s overheads aren’t justifiable.
Voice over IP (VoIP)
VoIP is a methodology and group of technologies for the delivery of voice communications and multimedia sessions over IP networks, such as the Internet.
- VoIP is extensively real-time, requiring an uninterrupted stream of data.
- It is preferable to miss a packet rather than delay all subsequent packets waiting for retransmissions, which is what happens with TCP.
VoIP actively employs UDP due to its low latency and protocol overhead.
Trivial File Transfer Protocol (TFTP)
TFTP is a simple lockstep File Transfer Protocol which allows a client to get a file from or put a file onto a remote host.
- TFTP uses UDP as it is simpler: there is no “connection” as such, and each data packet is dispatched individually.
- Also, upon failure, recovery can be initiated by the sender, avoiding the need for TFTP to engage in a possibly lengthy back-and-forth with the sender.
In summary, while TCP is used when reliability of data transmission takes precedence, the speedier, less demanding UDP is employed in instances where quick, real-time transfer is crucial. VoIP, DNS and TFTP are clear examples of places where UDP shines brightest.Sure, let’s dig deeper into the three protocols that predominantly use User Datagram Protocol (UDP). These are Domain Name System (DNS), Simple Network Management Protocol (SNMP), and Real-Time Transport Protocol (RTP).
Domain Name System (DNS)
The DNS protocol essentially helps in translating human-readable hostnames into IP addresses. This action allows computers to communicate with each other over a network or across the internet efficiently.
1. Defining Feature: DNS essentially functions as the phonebook of the internet. It maps user-friendly domain names to their corresponding IP addresses. In doing this, it directly aids people in remembering names instead of number sequences for websites.
Here is a simple snippet of how a DNS request might look like in code:
from scapy.all import DNS, DNSQR, IP, UDP send(IP(dst="8.8.8.8")/UDP(dport=53)/DNS(rd=1,qd=DNSQR(qname="www.google.com")), verbose=0)
2. Relationship with UDP: Typically, DNS uses UDP because it is faster, and speed is crucial when resolving domain names. Sessions or streams are not needed for lookup information, making UDP’s connection-less nature suitable for DNS requests.
Simple Network Management Protocol (SNMP)
SNMP is widely used in network management systems to monitor and manage network devices.
1. Defining Feature: SNMP offers insight into device conditions by collecting data from various network devices like routers, switches, printers, servers, workstations, among others. With SNMP, you can manage network performance, find and solve network issues, and plan for network growth.
Here is how an SNMP request may look like:
from pysnmp.hlapi import * errorIndication, errorStatus, errorIndex, varBinds = next( getCmd(SnmpEngine(), CommunityData('public', mpModel=0), UdpTransportTarget(('demo.snmplabs.com', 161)), ContextData(), ObjectType(ObjectIdentity('SNMPv2-MIB', 'sysDescr', 0)) ) )
2. Relationship with UDP: SNMP utilizes UDP as it simply reports the current state of the device. It doesn’t require the overhead carried by a transport protocol like TCP, which acknowledges every packet sent.
Real-Time Transport Protocol (RTP)
RTP is primarily designed towards providing end-to-end delivery services for data that requires real-time characteristics such as interactive audio and video.
1. Defining Feature: RTP plays a key role in the delivery of audio and video across the network. It handles the data transfer rate adjustment and maintains the quality of service.
2. Relationship with UDP: Since RTP is used for time-sensitive applications, it uses UDP due to its lower latency and no-retransmission features. This approach compares favorably to TCP, where lost packets could cause noticeable pauses or glitches.
I invite you to further delve into online material that describes these protocols in detail if your interest has been peaked. For example, you can visit site learn-networking.com to tutorialize yourself better about these and other protocols.
Network protocols are specific rules or standards that signal how computers on a network should interact with each other. UDP (User Datagram Protocol) is one such communication protocol used online. Yet not all tasks require the use of UDP; it’s crucial to understand where it excels before designing an application around this protocol.
The optimal Use Cases for User Datagram Protocol (UDP)
- Streaming Services: Streaming services often make use of UDP due to its speed and the non-persistent nature of video data. Once a frame of video passes, we don’t want to slow down the stream trying to resend lost frames.
- Online Gaming: Similar to streaming, online games require speediness for seamless interactions. Here, losing a bit of information is preferable over experiencing lags in gameplay.
- Simple Query Services: DNS and SNMP use UDP since these services usually entail straightforward, uncomplicated exchanges of information where not every byte needs acknowledgement.
These applications primarily revolve around the same strengths of UDP – speed and simplicity. It does not support “handshaking,” unlike TCP (Transmission Control Protocol), and as such, packet delivery isn’t guaranteed with UDP. This makes UDP faster and more efficient for tasks that don’t require every bit of data to arrive at their destination.
Three Protocols that use UDP:
Protocol | Description |
---|---|
DNS (Domain Name System) | The aim here is speed and simplesity, where the client requests information about a domain, and the server responds with the relevant IP addresses. If data packets got lost, applications generally just retransmit the request. The need to establish a connection like you would with TCP might slow down the process.Example code: socket(AF_INET, SOCK_DGRAM, IPPROTO_UDP); |
SNMP (Simple Network Management Protocol) | SNMP aids administrators in managing devices on a network by exchanging management information between networked devices. Again, given the simple queries here, UDP comes in handy.Example code: socket(AF_INET, SOCK_DGRAM, 0); |
RTP (Real-time Transport Protocol) | RTP provides end-to-end network transport functions suitable for applications transmitting real-time data, such as audio and video. Here, minimal delay matters more than the secure arrival of every packet.Example code: RTP_Header *header = rtpPacket->GetHeader(); |
Given each protocol’s purpose and the types of data they handle, using UDP makes sense. But, if your task requires reliable data delivery, establishing connections, and error-checking functionalities, you may need to consider TCP-driven protocols instead.
Remember, supporting speedy and straightforward data exchange is what makes UDP ideal for certain tasks. Basing your choice of protocol on the specific needs of your application is critical to ensure optimal performance.
– RFC 768 – User Datagram Protocol
– Cisco – Understanding User Datagram Protocol
– IBM Knowledge CenterNetwork communications are hinged on the agility, reliability, and efficiency of protocols that regularly moderate data exchange. To zone in on our exploration, it’s crucial to specify three specific scenarios—DNS (Domain Name System), VoIP (Voice over Internet Protocol), and DHCP (Dynamic Host Control Protocol) —all of which primarily utilize UDP (User Datagram Protocol).
1. User Datagram Protocol (UDP)
As a transport layer protocol in the OSI model, UDP enables the transfer of datagrams across network connections that are potentially unreliable. As opposed to TCP (Transmission Control Protocol), UDP delivers substantial speeds since it doesn’t invest time establishing connections or verifying the receipt of packets. Here’s how to write a simple server with an open UDP connection:
import socket def udp_server(): server_socket = socket.socket(socket.AF_INET, socket.SOCK_DGRAM) server_socket.bind(('', 12345)) while True: message, address = server_socket.recvfrom(1024) print('Received message:', message, 'from:', address) udp_server()
2. Domain Name System (DNS)
DNS, a vital part of any internet-based application, frequently uses UDP for communication. Its role entails translating domain names into IP addresses; thus simplifying how users access resources online. It is designed to favor transaction speed over data integrity, hence its decision to rear upon UDP. For instance, when initiating a DNS query:
import dns.resolver def resolve_domain(domain_name): resolver = dns.resolver.Resolver() ip_address = resolver.resolve(domain_name, 'A')[0].to_text() print('IP Address:', ip_address) resolve_domain('www.example.com')
Links:
Wikipedia – Domain Name System
3. Voice Over Internet Protocol (VoIP)
VoIP essentially converts voice signals into digital packets, then transmits them via the internet. Thus, facilitating voice communication without resorting to traditional telephone networks. VoIP capitalizes on the speed associated with UDP, low latency being integral for uninterrupted calls and high audio quality.
4. Dynamic Host Configuration Protocol (DHCP)
Designed to allocate IP addresses to devices within a network automatically, DHCP also often veers toward using UDP. Reason? Many clients may not have IP addresses defined prior to the DHCP process. UDP, ensuring quicker delivery with no strict verification processes, aligns well with DHCP’s functionality.
In overview, these three scenarios—DNS, VoIP, and DHCP—highlight the importance of UDP in fostering swift and efficient network communication. They each encapsulate varying facets of networking from accessing online resources, enjoying voice communication over the internet, to configuring network devices seamlessly.
Sure, let’s dive into the depths of three very significant protocols that use User Datagram Protocol (UDP) – DNS, VOIP, and DHCP. Careful usage of technical jargon is critical for understanding these concepts; thus, a breakdown analysis will be discussed in more detail for each term. The fundamental purpose behind such study unfolds how information across networks is channeled and controlled.
DNS (Domain Name System)
Codenamed as ‘phonebook of the internet’, DNS transforms human-friendly website names into computer-friendly IP addresses:
www.example.com becomes translated to 93.184.216.34.
-->Example of a typical DNS query: Client-> DNS Server: www.example.com DNS Server -> Client: 93.184.216.34
This protocol primarily operates over UDP for reduced latency and speed. An important characteristic of UDP is that it lacks an assurance of packet delivery. However, with DNS, if a query fails over UDP, it will retry on TCP, usually due to large DNS responses.
More details can be found here.
VOIP (Voice Over Internet Protocol)
VoIP enables voice traffic transportation over IP-based networks. Facilitating phone calls over internet connections, cost savings are tremendous. It runs over UDP because of its superiority in providing time-sensitive services:
In VoIP communication: | When a word is spoken, it is converted into digital format, |
Encoded via codecs | Then sent over the network in data packets utilizing UDP. |
Explicitly, efficiency matters where every millisecond wasted causes delay or sound distortion on the receiving end. Where error correction isn’t fundamentally necessary, UDP emerges as the winner – trading off reliability for real-time communications.
Here’s a code snippet hinting towards using UDP packets in implementing a basic VoIP system.
#Instantiate the socket object using UDP's SOCK_DGRAM call = socket.socket(socket.AF_INET, socket.SOCK_DGRAM)
In-depth reading on this topic is available on the Cisco Developer page.
DHCP (Dynamic Host Configuration Protocol)
A server-client protocol, DHCP authorizes automatic allocation of network parameters (like IP addresses) to devices. Fundamentally, providing expediency in configuration management. Here’s an example of a DHCP exchange:
Client-> DHCP Server: "I need an IP address!" DHCP Server -> Client: "Sure, you can have 192.168.1.2 for the next 24 hours."
Over UDP, DHCP excels as it doesn’t need the overhead of establishing a connection before transmitting data, enabling faster boot times. Usage of both LAN broadcast capabilities (send to all devices on a network) and point-to-point transmission makes UDP the perfect fit.
Through this exploration, one concludes UDP’s integral part of these protocols’ architecture. Building upon it’s quickness, even sacrificing guaranteed delivery or ordered receipt of data packets which TCP provides. Governed by requirements, this demonstrates technology’s adaptability to fulfill certain demands. Undeniably, these protocols’ respective design and functionality revolve around the nuances that UDP affords them. Employing best performances given circumstantial domain criteria, they bring forward the beauty that exists within the complexity of computational systems.Uncovering the Trio of UDP Protocol Usages
One cannot ignore the undeniable contribution of the User Datagram Protocol (UDP) in the realm of internet protocols. It pulls off the seemingly herculean task of facilitating communication between systems without necessitating a formal connection – truly a masterful feat. Delving deeper into this domain, three specific protocols leverage the power of UDP – they are Domain Name System (DNS), Simple Network Management Protocol (SNMP), and Trivial File Transfer Protocol (TFTP).
[DnsProtocol] public abstract void RequestDnsRecord(string fqdn); [SnmpProtocol] public abstract void RequestMibValue(string oid); [TftpProtocol] public abstract void RequestFileTransfer(string fileName);
The Domain Name System (DNS) counts on UDP to effortlessly perform its magic of translating human-readable website names into their corresponding IP addresses. It finds its strength in the lesser overhead of UDP as compared to TCP, leading to accelerated response times.
Up next is the Simple Network Management Protocol (SNMP). A vital tool for network administrators globally, SNMP allows for real-time monitoring and control of network devices. Leveraging UDP, SNMP efficiently communicates with multiple devices simultaneously, ensuring their continuous operation.
Last but not least, the Trivial File Transfer Protocol (TFTP) sees purpose where simplicity and efficiency reign over data integrity–like network boot services or simple file transfers. Operating on UDP, it provides an excellent solution for resource-constrained devices that struggle with the computing demands necessary for employing more robust protocols.
Protocol | Use Case |
---|---|
DNS | Website name to IP translation |
SNMP | Network device monitoring and management |
TFTP | Lightweight file transfer |
By virtue of succinctness and minimalistic design, these three protocols—DNS, SNMP, and TFTP—make impressive strides in their respective spheres, unquestionably supported by UDP’s inherent properties of speed and simplicity. As such, the crucial role UDP plays in underpinning them becomes transparently clear. With burgeoning internet traffic volumes, its necessity is only poised to grow, as more applications require high-speed, efficient transmission—genuinely exemplified by this triumvirate of protocols utilizing UDP. Hard to envisage a well-functioning Internet without them, indeed!