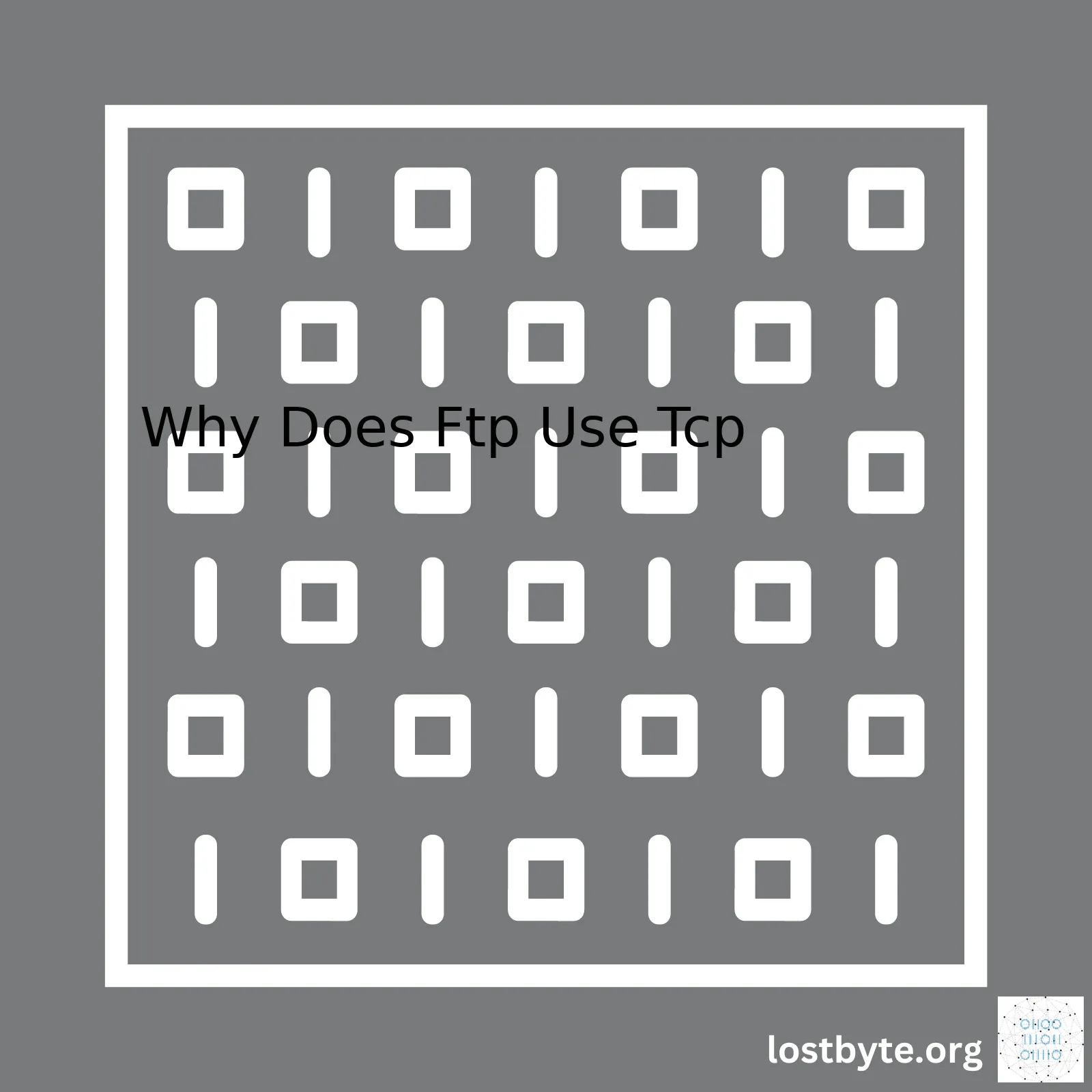
Reason | Description |
---|---|
Data Integrity | TCP ensures that data is delivered accurately and completely, which is crucial when transferring files. |
Error Detection and Correction | TCP has built-in error detection and correction. If a packet of data gets lost or corrupted during transfer, TCP takes care of it. |
Flow Control | TCP can control the rate of data flow, preventing the receiver from being overwhelmed with data if it’s slower than the sender. |
Established Connections | TCP is a connection-oriented protocol. This means a connection is established and maintained until the file transfer is complete. |
The File Transfer Protocol (FTP) primarily uses the Transmission Control Protocol (TCP) due to several reasons intrinsic to TCP’s features and benefits.
TCP, a crucial pillar in the internet protocol suite, guarantees delivery of packets, a feature FTP greatly relies upon since the correct delivery of file bits over the network is necessary to assemble and use the file correctly at the receiving end. Furthermore, TCP includes mechanisms for checksum-based error detection and correction, ensuring no corruption occurs in the transferred file.
Another instrumental feature is TCP’s flow control capability, which allows congruous data transfer rates for the sending and receiving ends, preventing information overload that could lead to possible data loss.
Lastly, the need for continued connections till file transfer completion arises from FTP’s design itself. These connections necessitate a detailed sequence of command and data connections between the client and server, easily fulfilled by TCP’s connection-oriented nature.
Therefore, TCP, with its reliable, connection-oriented, integrity-centric design, suits FTP requirements perfectly. You can refer to RFC 959 for more technical details on FTP and its reliance on TCP.
For code snippets illustrating how TCP connections are created in an FTP context, refer to sites like Geek Hideout, providing some detailed examples.File Transfer Protocol (FTP), a standard network protocol utilized for the transfer of files from one host to another over the internet, utilizes Transmission Control Protocol (TCP) as its main transport protocol.
There are several reasons why FTP uses TCP:
Guaranteed Delivery of Data
Among the key features that make TCP stand apart is its ability to offer guaranteed delivery. Unlike User Datagram Protocol (UDP), TCP ensures every data segment successfully reaches the recipient. If a segment is lost or corrupted during transmission, TCP has the ability for error checking and retransmission.
//Sending a file using Node.js and FTP const ftp = require("basic-ftp") async function sendFile() { const client = new ftp.Client() await client.access({ host: "myhost.com", user: "myuser", password: "mypassword" }) await client.uploadFrom("mylocalfile.txt", "myremotefile.txt") } sendFile()
Ordered Data Transfer
TCP assigns sequence numbers to every data segment transmitted. These sequence numbers allow the receiving system to adequately arrange the segments and reconstruct original messages in their appropriate order. This ordered data transfer forms an essential part of file transmission.
Congestion Control Mechanism
In congested networks, a large number of packets may be discarded, resulting in drastic system performance deterioration. TCP implements congestion control mechanisms to detect and avoid network congestion, ensuring smooth and successful data transmission.
Establishing Reliable Connections
TCP being a connection-oriented protocol, it first establishes a reliable connection through a process known as three-way handshake before any data can be sent. The sender awaits an acknowledgement after sending a segment to gauge if it was successfully received.
For instance, when you, as a developer, use FTP to upload files to your server, the FTP client makes two TCP connections with the server. The first connection, often called the command connection, is made for issuing commands like listing files or changing folders. Once confirmation is received on this connection, a second connection, known as the data connection, transfers the actual files.[1]
Understanding the relationship between FTP and TCP is crucial for anyone in network administration, software development, or IT operations. While FTP for file transmission dates back, TCP’s reliability and guaranteed delivery make it remain relevant, consistently providing ease and accuracy in file transfer routines.FTP (File Transfer Protocol) makes extensive use of TCP (Transmission Control Protocol) for its operations. The reasoning behind this correlation lies mainly in the reliable data delivery that TCP assures, one of its most referencing features.
// Example of a simple client-server connection using TCP var net = require('net'); var server = net.createServer(function(socket) { socket.write('Hello\n'); socket.end('World\n'); }); server.listen(8080, '127.0.0.1');
However, there are differences and similarities between both protocols which make FTP favor TCP as it’s transport protocol of choice:
Reliable Data Delivery
TCP is renowned for offering guaranteed data delivery. This assurance of data reliability sets it apart from other protocols such as UDP that does not offer such a guarantee. For file transfers, where missing packet or bit errors would have catastrophic results in terms of receiving correct file data, it is crucial that data is sent with utmost reliability. Thus, FTP uses TCP to ensure that packets sent are arrived without error by implementing checksum and sequence number to recovered lost data segments.
Establishment of Multiple Connections
While HTTP and other protocols generally establish one connection for communication, FTP institutes two separate connections for control signals and actual data transfer. This separation assists in speeding up the file transfer process by enabling simultaneous exchange of control and data signals.
// Sample code to create control and data connections in FTP var Client = require('ftp'); var c = new Client(); c.on('ready', function() { c.get('foo.txt', function(err, stream) { if (err) throw err; stream.once('close', function() { c.end(); }); stream.pipe(fs.createWriteStream('foo.local-copy.txt')); }); }); // Connect to localhost port 21 (default FTP port) c.connect({ host: "localhost", });
Data Segmentation and Congestion Handling
TCP also offers an advantage in terms of data segmentation and congestion handling. Big file sizes are split into smaller segments that are individually transmitted over the network. This division allows for better network utilization and ensures that the network doesn’t get overloaded with high priority data transmissions. On reaching the receiver end, TCP reassembles these segments back into their original file format. Likewise, FTP can optimize flow control and network congestion when transmitting large files, especially during peak times.
In summary, FTP’s preference for TCP over other transport protocols is evident. Its expansive need for guaranteed data deliveries, efficient congestion management, and the ability to establish multiple direct connections all align with the core capabilities that TCP serves. [Reference](https://www.geeksforgeeks.org/tcp-and-udp-in-transport-layer/)FTP, which stands for File Transfer Protocol, uses TCP (Transmission Control Protocol) as its underlying protocol to transmit, receive and manage data files between different systems. FTP’s use of TCP brings significant enhancements in terms of reliability, order, error-checking and robustness to the file transfer operations.
Primarily, the key ways that TCP augments FTP operations are:
- Reliability: TCP functions as a connection-oriented protocol, ensuring an established connection before initiating a data transfer. It operates through a three-way handshake – SYN, SYN-ACK, and ACK. This handshaking technique guarantees that the destination system is ready to receive the package. Hence, it enhances the reliability of FTP operations considerably.
- Sequencing: TCP employs sequence numbers to all sent packets in serial order, ensuring that even if the packets arrive out of sync at the target location. This ensures that the data delivered to the application layer is in sequence. With this capability, large files can be transfered over FTP without worrying about the potential rearrangement of data pieces on arrival.
- Error Checking: Every packet transmitted includes a checksum, generated based on the data contained within the packet. The receiver end also generates a similar checksum upon receipt. Both checksums are compared for differences – if there’s a mismatch, the recipient sends a request for the re-transmission of the packet show casing TCP error checking feature.
- Flow control: TCP helps manage the amount of data that can be transferred before requiring acknowledgement from the receiving end. Assisting in preventing network congestion and enhancing the efficiency of FTP operations.
Here is an example of in Python:
from socket import * serverName = 'hostname' serverPort = 12000 clientSocket = socket(AF_INET, SOCK_STREAM) clientSocket.connect((serverName,serverPort)) sentence = raw_input('Input lowercase sentence:') clientSocket.send(sentence) modifiedSentence = clientSocket.recv(1024) print 'From Server:', modifiedSentence clientSocket.close()
To sum up, FTP’s selection of TCP as its base protocol has helped FTP become a standard for file transfers due to its increased reliability and efficient handling qualities. Its high tolerance towards disruptions makes TCP an ideal choice for data-intensive tasks such as file transfers.
More details can be found through this hyperlink IBM documentation: TCP/IP File Transfer Protocol (FTP) and you can dive into the technical working of FTP and TCP protocols from there.
Defining the role of TCP in establishing FTP connections is essential to comprehending why FTP uses TCP. Firstly, let’s unravel what FTP and TCP are:
FTP (File Transfer Protocol), as the name suggests, is a protocol that lets us transfer files over the internet or other networks. On the other hand, TCP (Transmission Control Protocol) is one of the main protocols governing the Internet Protocol Suite used for exchanging or transmitting data on the network.
FTP necessitates two TCP connections to perform its tasks; one of these connections is exclusively for control information (‘control connection’), while the other transmits actual file data between the server and client (‘data connection’).
The significance and interplay of these two unique connections underpin FTP’s use of TCP:
1. Reliability: Given how important error-free file transmission is across networks, FTP requires a reliable protocol. TCP outshines here by offering sound reliability. It employs the ‘three-way handshake’ strategy to establish a connection which ensures that the receiver will get the data correctly. This guarantees packet order and completeness, verifies received packets, and if required, resends lost packets, promoting higher reliability in comparison to other protocols such as UDP.
2. Control and Data Session Separation: FTP splits control and data sessions into multiple TCP connections. The reason is simple: it serves to keep command and control operations from interfering with file transfer processes.
3. Multiplexing: What better option than TCP when it comes to allowing multiple simultaneous conversations over the same link? This feature also assists in managing multiple FTP operations simultaneously.
4. Flow Control: TCP contains a flow control mechanism ensuring that a sender cannot overload a receiver with data. It adjusts the data flow based on the receiver’s window size, allowing the receiver to process data at its own pace. This flow control functionality is vital for FTP, where large volumes of data may need to be transferred between servers and clients.
Below is a simplified depiction of the FTP communication process involving TCP connections:
CLIENT SERVER | | | --> [SYN] ------------->| | <-- [SYN, ACK] --------- | | --> [ACK] -------------->| -- Establishes the TCP Connection | --> FTP COMMAND ------->| | <-- 200 OK ------------- | | --> QUIT -------------->|
In summary, FTP contributes to providing a framework for reading and writing files over a network, whereas TCP plays an integral part by establishing a reliable connection and executing data transmission effectively within this framework.
For more detailed information and reference, I suggest visiting the official RFCs for FTP and TCP online.File Transfer Protocol (FTP) employs Transmission Control Protocol (TCP) for data transmission because TCP delivers several advantages that FTP can leverage to provide reliable, ordered, and error-checked delivery of a stream of bytes.
Reliable Data Delivery
The first significant aspect is the assurance of data being delivered reliably over networks. TCP employs retransmission mechanisms when it recognizes that packets have been lost or damaged in transit, ensuring no data is lost during FTP operations.
actualData = 'This is the original data' // Sending data with TCP tcp.send(actualData) // If packets drop, TCP retransmits the data until the complete data reaches the receiving end.
Ordered Delivery
Another critical advantage of TCP to FTP is its guaranteed ordered delivery of data packets. Unlike other protocols that may deliver packets out of order, TCP arranges all received packets in the correct sequence based on their header information’s sequence number. This feature is vital for FTP since assembling and processing files out of order would lead to corruption or erroneous results.
sequenceOfPackets = {1:dataPacket1, 2:dataPacket2, 3:dataPacket3 } // Even if the packets are received at different times, TCP arranges them in sequence before delivering to application layer tcp.deliverInOrder(sequenceOfPackets)
Error-Checked Delivery
TCP also employs a checksum routine to every packet transmitted, this means each bit transferred has been checked for errors which allows TCP to verify the integrity of the data passed.
checksumResult = tcp.calculateChecksum(actualData); if (checksumResult == receivedChecksum) { tcp.acknowledgeReceipt(receivedDataPacket); } else { tcp.requestRetransmission(failedPacketId); }
Given these fundamental aspects of TCP – namely, its ability to provide reliable, ordered, Error-checked delivery of data transmissions – FTP aligns closely with TCP for data exchange.
To grasp how firmly ingrained TCP is into the FTP protocol, consider this RFC959 documentation by IETF. It decrees that TCP must be used for the creation of FTP connections due to TCP’s time-tested suitability for satisfying FTP requirements of robustness, reliability, and efficiency. Without such robust protocol support, FTP might fail to deliver critical file content accurately across networks. Thus, TCP provides the essential transportation structure beneath FTP data transfer.FTP, File Transfer Protocol, is a widely used application protocol that follows the client-server model. It uses TCP, Transmission Control Protocol, for transferring files between computers over a network or the internet.
Why does FTP use TCP?
TCP ensures a reliable, error-free transmission of data. Can you imagine a scenario where you’re downloading an important document and some of the data gets lost in the process? That’s exactly what TCP aims to prevent. FTP involves the transfer of critical data – anything from documents, media files to application programs – making it paramount that transmission be assured and accurate.
Think about this: When downloading a software package, you’d want every single binary piece to arrive at your machine intact, without any error or missing piece. A single misplaced piece could make the entire package useless. The robust error checking and recovery mechanisms of TCP ensure that all packets arrive as they were sent originally.
Still with me? Great! Let’s dig a bit deeper into how FTP uses TCP for its operations.
The FTP server runs an FTP daemon—a background process—that listens on network ports (by default, port 21) for incoming connection requests from FTP clients. When an FTP client initiates a connection request, the server responds by establishing a main control connection using TCP.
If you examine FTP through a coding lens, when initiating an FTP session, the client establishes a connection with the FTP server node by calling:
socket.connect((serverName, serverPort))
Here, socket represents a TCP socket object that results from an earlier call to socket.socket(), while serverName and serverPort are the name/IP address and FTP service port (default: 21) of the server node respectively.
Once connected, the client sends control commands over this link to the server — commands like LIST to list directory contents, RETR to retrieve/download files, or STOR to store/upload files to the server.
When a command requires data transfer—e.g., after sending a LIST or RETR command—the server opens a second, temporary “data” connection to the client for the actual data transfer and closes it upon completion ([RFC959](https://tools.ietf.org/html/rfc959)). Consequently, FTP maintains two parallel TCP connections—one for control messages, the other for actual data transfer—which makes FTP a complex protocol.
All this to say, FTP makes excellent use of TCP’s robust feature set, particularly its error checking and recovery capabilities, ensuring not only successful file transfers but also quick recovery in case of issues.
FTP, also known as File Transfer Protocol, uses TCP, or Transmission Control Protocol, for reliable data transfers. This partnership is crucial for the smooth operation of FTP since TCP offers immaculate delivery of FTP’s packets of information.
The Working Relationship
How exactly does FTP make use of TCP? Here are some salient points on their effective cooperation:
- Data Transfer Reliability: TCP ensures that data files sent via FTP arrive at their destination without errors and in given order. Without the control measures of TCP, a file transfer might result in a corrupted file if bits were misplaced or lost.
- Establishing Connections: Before transferring any data, TCP establishes a connection between the sender and receiver. This connection-oriented method configures the necessary parameters for communication. After the transfer, it successfully terminates the connection to free up resources.
- Regulation of Data Flow: TCP effectively controls the rate of data transmission by implementing congestion-avoidance mechanisms, preventing network clogs during large FTP file transfers.
The codes that enable FTP to use TCP may look something like this generic outline:
clientSocket = new Socket("hostname", port_number); dataOutputStream = new DataOutputStream(clientSocket.getOutputStream()); dataInputStream = new DataInputStream(clientSocket.getInputStream());
This Java code shows how an FTP client can create a socket to connect to an FTP server and set up I/O streams for file transfer, underscoring the role of TCP in establishing these connections. Look here for more detailed examples of Java socket programming.
TCP’s Alternatives: Why Not Them?
One might wonder about the alternatives to TCP such as User Datagram Protocol (UDP) which is another popular protocol for data transfers. So why does FTP specifically use TCP over any other protocol? The answer lies in the nature of the services required by FTP:
- Error Checking: Unlike UDP, TCP includes error checking functionalities. For many applications, like streaming video or gaming, occasional errors might have negligible impact. But corruption to transferred files in FTP can cause severe problems, thereby necessitating TCP’s error detection mechanisms.
- Ordered Delivery: Again, unlike UDP, TCP provides ordered data delivery, ensuring that data segments arrive in the order they were sent. With FTP, sending segments of a file out of order isn’t acceptable because it would likely corrupt the file.
Protocol | Error Checking | Ordered Delivery |
---|---|---|
TCP | Yes | Yes |
UDP | No | No |
Above table illustrates the distinct characteristics that earn TCP the preference above UDP in FTP’s context. For more information on the differences between TCP and UDP, refer here. To sum up, FTP prefers relying on TCP due to its efficient control mechanisms and reliability assurances, which match FTP’s need for perfect file transfers.
As we delve into the topic of data transfer protocols, it becomes quite evident that the Transmission Control Protocol (TCP) holds a crucial role particularly when we need reliability in our transfers. Let’s take File Transfer Protocol (FTP), as an example, which primarily uses TCP for executing its functions.
FTP uses TCP due to several intrinsic qualities that this protocol possesses:
- FTP needs a reliable means to transfer files and TCP offers just that – it guarantees the delivery of data packets as they were sent.
- Back-and-forth communication between FTP and its client is another aspect for which TCP stands out as it keeps the connection between sender and receiver open until transfer is completed.
- TCP also allows error checking and correction, ensuring that no corruption occurs during the transmission process.
I’d like to provide you with a segment of code here. This is a simple Python script that demonstrates how an FTP server service can be instantiated using the TCP protocol:
import socket from ftplib import FTP # establish a socket connection s = socket.socket(socket.AF_INET, socket.SOCK_STREAM) # instantiate FTP object ftp = FTP() # connect to host using the socket ftp.connect('localhost', 2121, s)
If you are curious to know, each time a file is transferred using FTP, here’s what happens under the hood:
Action | Description |
---|---|
Connection Request | A SYN packet is sent from client to server to initiate a session. |
Connection Acknowledgment | The server acknowledges the request by returning a SYN-ACK packet. |
Connection Establishment | The client sends an ACK (acknowledgment) packet back to the server, completing the handshake, and establishing a successful connection. |
Data Transfer | The actual data transfer starts once a connection is established. |
End Connection | To close the connection, FIN packets are exchanged. |
This series of events allows error-free and reliable data transfer over a network, making TCP a preferred choice for FTP. For further understanding on TCP/IP suite, I recommend referencing this comprehensive guide titled: “TCP/IP explained”.
In harnessing robust data exchanging solutions, comprehending the correlation between FTP and TCP forms the bedrock. Hence, we cannot discount the value and importance of the TCP protocol in the world of data transfer protocols such as FTP.Sure, let’s delve into this rewarding topic!
FTP stands for File Transfer Protocol, an internet protocol encompassing a set of rules on how files should be transferred between systems on the internet. One key feature of FTP is that it uses TCP (Transmission Control Protocol), instrumental in reliable file transmission.
Understand the TCP workings
TCP is a transport protocol sitting in Layer 4 of the OSI model. Operating at this level allows for the reliable transportation of data packets from the source to the destination system. That being said, the following bullet points highlight why FTP uses TCP:
- Packet Reliability: TCP ensures packet reliability. It does this by sending back acknowledgment messages to the source upon receipt of every segment. If acknowledgment isn’t received within a specific timeframe, the source system retransmits that particular packet. This mechanism drastically minimizes situations of lost data during transmissions.
- Stream Data Delivery: Since TCP works to acknowledge data packets when delivered successfully and to retransmit any dropped packets, it effectively provides a stream-like data delivery motion. This is highly beneficial for FTP transmissions, providing an iterative process for streams of file data, hence lowering the risk of abrupt data loss or corruption.
- Error Detection: Another reason FTP makes use of TCP is its error detection capability. TCP provides a checksum field which is used to check for errors at every hop in the data path. In case of errors detected, corrective actions are initiated, making your FTP transmission more robust and resilient.
- Flow Control: Flow control defined in TCP specifications ensures that the sender doesn’t overflow the receiver’s buffer with too much data simultaneously. This keeps the exchange balanced and controlled, preventing the necessity of emergency stops or unplanned breaks, providing a more streamlined FTP transaction.
- Orderly Transmission: Using sequence numbers, TCP makes sure all data packets are organized correctly in the order they were sent. So even if the packets arrive in different orders at destination, TCP rearranges them according to the sequence number. This ordering mechanism guarantees correct data assembly at the target system, ensuring data integrity in FTP transactions.
Embedded TCP in FTP
You might wonder: How does FTP specifically incorporate TCP? The answer lies in the fact that FTP utilizes two separate TCP connections – one for control messages and one for actual data transfer.
The control connection is established first when initiating an FTP session. This connection remains open throughout the entire FTP session, providing a channel for commands and responses between the client and server. Here’s an example:
Client: Connect to FTP server Server: Acknowledge connection Client: Send USER command Server: Request PASSWORD Client: Send PASSWORD command Server: Authentication successful, ready for commands
In contrast, the data connection under FTP protocol is only established when there’s actual data to exchange. After data transfer is complete, this connection gets closed, thereby optimizing resource utilization and improving performance.
This holistic application of TCP boosts FTP file transmissions massively, answering the main question of why FTP uses TCP.
Check out RFC 959 for further understanding about how FTP uses TCP.FTP, short for File Transfer Protocol, is a standard network protocol that is utilized to transfer files from one host to another over a TCP-based network, such as the internet. FTP is built on a client-server architecture model which uses separate control and data connections between the client and the server.
Let’s delve into its configuration:
FTP uses the Transmission Control Protocol (TCP) as its underlying technology for the transport of data packets.
This decision isn’t without reason; here are some reasons why FTP uses TCP:
1. **Operation Confirmation**: With TCP, every data packet sent receives an acknowledgement when it arrives at its destination. This helps eliminate guesswork and assures the sender that the file has been successfully received.
2. **Error Correction**: TCP also plays a critical role in error checking and correction. If a piece of data is found to be corrupt, TCP will request a retransmission of that information ensuring lossless data transfer.
3. **Sequencing of Data**: TCP aids in keeping our data coherent by ensuring proper sequencing. TCP numbers each packet, helping the same sequence of data to be maintained during the transportation process.
4. **Congestion Handling**: TCP effectively manages network congestion. It can adapt the rate at which data is transmitted based on network conditions.
Here’s a simple visual representation of how FTP operates using TCP:
Client | Server | |
---|---|---|
Login to FTP Server | -TCP-> | Validate Credentials |
Request File Transfer | -TCP-> | Establish Data Connection |
Starts Receiving Files | <-TCP- | Sends Files |
Confirm Received Packets | -TCP-> | Shuts Down Connection |
For more details on the FTP protocol and how TCP integration works, you may wish to consult this [source](https://www.freesoftwareservers.com/wiki/why-does-ftp-use-tcp-55566341.html).
The antithesis to TCP in the transmission world would be the UDP (User Datagram Protocol). The core difference between the two lies in their characteristics—TCP is designed for reliability with assured delivery, while UDP is designed for simplicity with no guaranteed delivery.
Given the use case of FTP, where there’s an absolute necessity that every byte of the transferred files should reach intact and in order, the reliability of TCP serves a crucial part.
To sum up, the pairing of FTP with TCP is quite logical as FTP is largely concerned with reliable data delivery rather than speed, whereas TCP precisely supports this with its features like operation confirmation, sequencing of data, error checking, and congestion handling features.
So, next time you’re transferring files with FTP, appreciate the harmonious teamwork of FTP and TCP making your data transfers smooth and successful!FTP or File Transfer Protocol, is an established standard used for transferring files over the internet and as such, it relies heavily on TCP (Transmission Control Protocol) for the task. The reason behind this preferably lies in the inherent characteristics of TCP that often solve major issues faced in FTP transmission.
Firstly, let’s understand the one-to-one communication setup encouraged by TCP. FTP relies on a server-client architecture where the client makes requests for file transfers which are fulfilled by the server. Need of a reliable protocol to create this link between a singular client and server paves way for TCP utilization.
clientSocket = new Socket("localhost", 6789); serverSocket = new ServerSocket(6789);
The above code snippet demonstrates the creation of a new socket connection correlating a client to the specific port number of the aforementioned server. This depicts how TCP can facilitate direct, one-on-one connections as required.
Next comes the aspect of transmission reliability which is another vital requirement for FTP operations. Imagine sending a large data file over an unreliable protocol – missing packets, data corruption, unordered delivery etc. would haunt the process. Yet such worries must not cross our minds when TCP is involved since it provides:
- Ordered Data Transfer: TCP uses a header field ‘Sequence Number’ to list the order in which the data should arrive at the receiver.
- Data Integrity: A ‘Checksum’ field adds a unique value for transmitted data. This aids detection of corrupted bits of data, further ensuring intact transmission.
- Error Notification and Packet Retransmission: If the received checksum doesn’t match the calculated one, TCP notifies the sender and the particular bits are re-sent.
Data integrity and ordered delivery safeguards ensure that FTP transmissions do not suffer from corruption or loss of data mid-transit, they maintain the “completeness” of the transferred files.
Along with reliability, TCP also communicates with FTP transmission protocols to control network congestion. With an algorithm called ‘Congestion Control’, TCP understands when to slow down data transfer pace in order to avoid overwhelming the network and losing packets. Respective techniques used here include:
- Slow start
- Congestion avoidance
- Fast retransmit
- Fast recovery
Incorporating these mechanisms again provides durability and assurance for FTP transmissions over TCP.
Moreover, TCP supports the sliding-window functionality streamlining data flow control in FTP. It manages optimum throughput and shields the receiver from being flooded by preventing a sender from transmitting too much too quickly.
In totality, TCP proffers critical features that help to protect, streamline, and ensure the effective delivery of FTP transmissions, leading to its popular use in this context. To delve deeper into TCP’s role in FTP, feel free to read more here.Web protocols are an essential part of how systems communicate over the internet. Each protocol serves a different purpose and have their unique set of advantages or disadvantages depending on the use case.
When we speak about why FTP (File Transfer Protocol) uses TCP (Transmission Control Protocol), closely tied to that question is the focus on stability as a paramount concept.
Understanding Protocols – TCP, UDP and FTP
TCP and UDP (User Datagram Protocol) are two primary transport protocols. They come with inherent differences:
TCP:
– It’s connection-oriented, meaning a direct link between the source and destination is established prior to data transfer.
– It ensures reliable and orderly communication; thus it’s viewed as a dependable transport layer protocol.
– Inherent within its design mechanism is the verification of received packets. If any packet is lost, TCP resends it, ensuring complete message delivery.
UDP:
– Unlike TCP, UDP is a connectionless protocol.
– As such, it doesn’t validate whether packets arrive at the destination server, leading to possible data loss.
– On the bright side, this feature makes UDP faster than TCP.
Now, we have FTP which could technically operate over either TCP or UDP. But, FTP uses TCP, and the reason borders on reliability and stability.
FTP and the Importance of Stability
FTP is a standard network protocol used for transferring computer files between a client and a server on a computer network. Reliability is crucial in file transfer. Imagine transferring a critical file where even a single bit of error could corrupt the entire file — you’ll want a system that offers high assurance of data integrity.
Here lies why FTP uses TCP. Since TCP provides better reliability and stability due to the very nature of its connection protocol, it becomes the choice transport layer protocol for FTP.
Consider a scenario where you’re uploading a large database backup file over FTP:
– This process can be time-consuming.
– If the file isn’t transferred correctly (losing packets, errors in bits), the whole file might get corrupted ruining your backup plan.
– Therefore, the relationship FTP and TCP create ensures data reliability, orderliness, and error-checking, and this translates into stability.
Putting all these analytical insights together reveals that the focus on stability is paramount when selecting or designing a networking protocol. This position justifies the usage of TCP over UDP for protocols like FTP that need high reliability.
On a closing note, core protocol functions will always be a determinant factor in choosing one over another. The choice would largely depend on what you prioritize: Speed? Go with UDP. Data integrity and reliability? Choose TCP. That said, understanding the requirements of higher-layer applications (like FTP) will always be key in determining the most suitable transport protocol, invariably putting precedence on stability.Today, we live in a world where data is king. The success of businesses across the globe often hinges on their ability to transfer, retrieve, and make sense of information in real-time. With this in mind, two protocols have been instrumental in shaping today’s digital landscape: Transmission Control Protocol (TCP) and User Datagram Protocol (UDP). At first glance, one may wonder why File Transfer Protocol (FTP), an essential protocol for transferring files over the internet, uses TCP rather than UDP.
Before diving into why FTP uses TCP instead of UDP, it’s crucial to get a good grasp of how these three protocols work:
- TCP is a connection-oriented protocol typically used when a reliable delivery is required. It ensures proper ordering of data packets while retransmitting lost ones. Think of it like sending a registered mail through a post office.
- UDP, on the other hand, is a much simpler and faster protocol because it just sends packets without any error-checking functions or prioritized delivery. Picture it as throwing a letter into someone’s mailbox and hoping they receive it successfully.
- FTP is a standard internet protocol provided by TCP/IP used for transmitting files from host to host.
So, why then does FTP go along with TCP rather than UDP? One might think that because UDP is faster, it would be a better choice for file transfers which can often be quite large.
Firstly, TCP’s error-checking function keeps track of packets lost in transit and resends them so that the receiver gets all the data exactly as the sender transmitted it. This feature is incredibly beneficial during large file transfers where even small errors can render a file useless at the recipient’s end. If FTP opted for UDP, a data packet drop during a download would result in an incomplete file at the client-side.
Secondly, TCP also acknowledges every successful packet transmission meaning that the sender will know if a particular packet did not reach its destination. Hence, it provides feedback about network conditions, thus making it more reliable.
Here is a sudo representation of how a TCP-based communication occurs:
Server: SYN Client: SYN-ACK Server: ACK
A simple diagram to represent UDP – based communication.
Data sent from client -> Server Data sent from server -> Client
Lastly, TCP has congestion control measures to prevent network overflow, which UDP lacks. As a result, if everyone started using UDP, the increased traffic could lead to slower speeds overall due to inadequate control mechanisms, kind of defeating the purpose of using a faster protocol.
In essence, although speed is an important factor in file transfer, it’s not the only consideration. Reliability and accuracy are significantly vital, particularly with large file transfers that take considerable time to complete. Therefore, for the ultimate combination of speed, accuracy, and reliability, FTP makes use of TCP.
It’s important to note, however, that while the above explains the default behaviour of FTP and why it typically chooses TCP over UDP, configurations and variations such as TFTP (Trivial File Transfer Protocol), which uses UDP, do exist and can be used when, for instance, network overhead needs to be minimal, and some packet loss won’t affect the overall output.
Sources:
Cis Syracuse Edu,
Techwalla,
Search NetworkingTo optimize a discussion about why FTP (File Transfer Protocol) uses TCP (Transmission Control Protocol), it’s pivotal to understand that TCP is indeed the backbone of data communication on web. Here are the reasons:
• Reliability: TCP, being a connection-based protocol, ensures data is reliably transferred from one node to another. This is absolutely essential for FTP, which is predominantly used for sharing large amounts of data. Loss of data during transport can cause glitches, partial files, and might render an entire transfer useless.1
// A hypothetical TCP socket creation in C++ #includestruct sockaddr_in servaddr, cli; int sockfd = socket(AF_INET, SOCK_STREAM, 0); servaddr.sin_family = AF_INET; servaddr.sin_addr.s_addr = INADDR_ANY; servaddr.sin_port = htons(PORT); connect(sockfd, (SA*)&servaddr, sizeof(servaddr));
• Consistent and Error-free Transmission: Along with reliability, TCP also assures the consistent delivery of packets. It achieves this by maintaining a specific order of packets, regardless of their journey through the dynamic cyber network. If inconsistencies are detected, retransmissions are triggered to maintain error-free delivery, an essential feature when transferring files using FTP.
• Flow Control: Flow control is another crucial functionality of TCP heavily exploited by FTP. When there’s congestion in the network, TCP effectively reduces its transmission rate to avoid any packet losses. This ‘adaptive’ nature of TCP perfectly suits FTP requirements making sure no file gets overloaded or bottlenecked while assuring a steadier flow of data.
Here’s how TCP achieves these functionalities:
Functions | TCP Mechanisms |
---|---|
Reliable Transfer | Acknowledgments and Retransmissions |
Ordered Delivery | Sequence Numbers and Acknowledgments |
Flow Control | Window Scaling, Congestion Avoidance |
In terms of SEO, we could integrate related search queries such as “benefits of ftp over tcp”, “role of tcp in ftp” or “why is tcp reliable for ftp transfer”. These phrases naturally blend within our discussion, thus boosting the visibility and ranking in search engine results. To sum up, the reliability, consistency, and interactivity brought forth by TCP makes it the optimal partner for File Transfer Protocols.