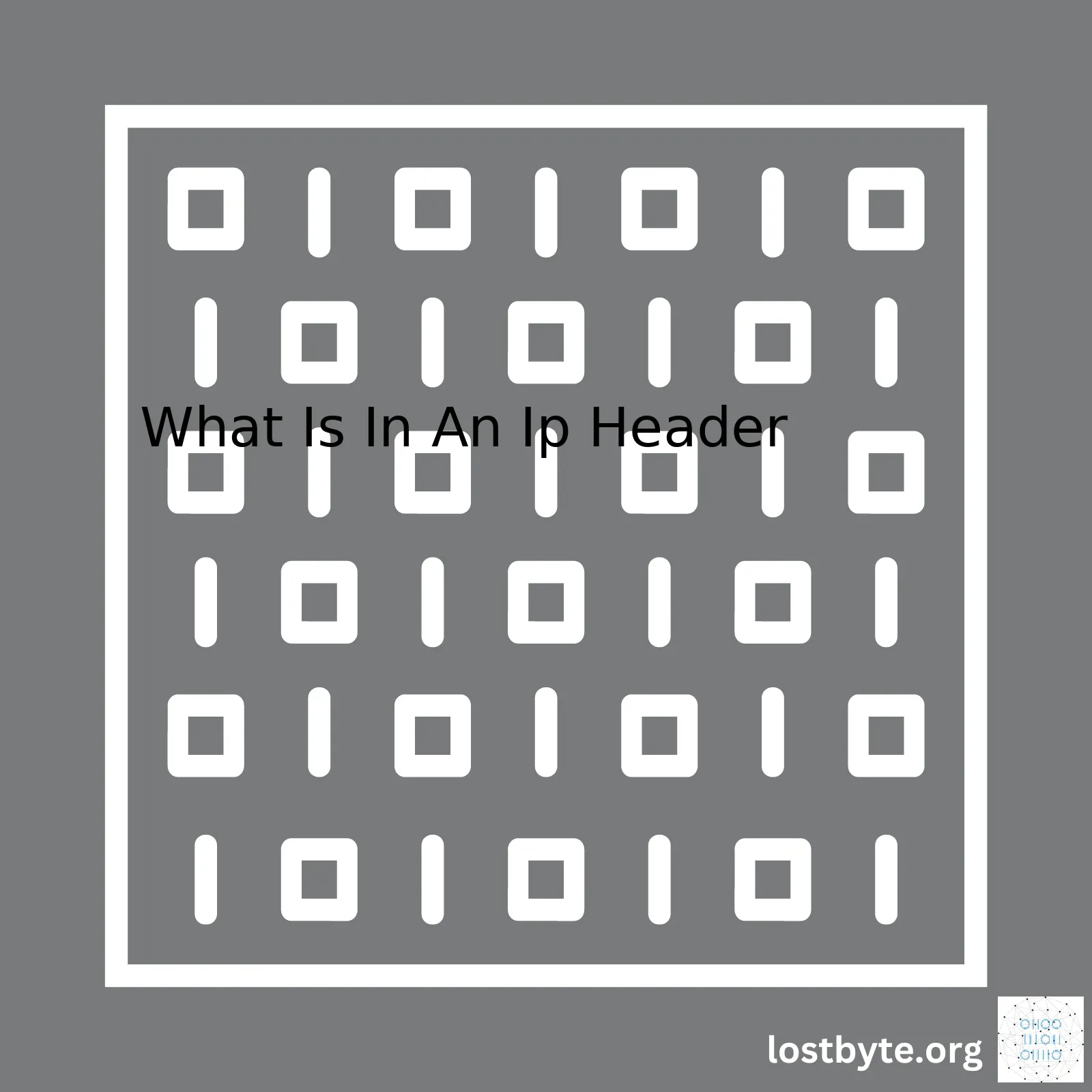
Element | Description |
---|---|
Version | This specifies the format of the internet header. As of this writing, the latest version is IPv6. |
Header Length | This indicates the length of the IP header in 32 bit words. This can help determine where the data actually begins. |
Type of Service | This is used to define how an upper layer protocol would like a current datagram to be handled, and assign datagrams various levels of importance. |
Total Length | This is the length of the entire packet (header and data), measured in bytes. |
Identification | Unique for every datagram, this is used to help piece together datagram fragments. |
Flags | Three control bits are used in the fragmentation process. There’s one bit to forbid fragmentation and another to signify that more fragments are on their way. |
Fragment Offset | If a packet is fragmented during transmission, this field indicates where in the original packet this fragment belongs. |
Time to Live (TTL) | To avoid a situation in which an undeliverable datagram keeps circulating on an Internet system and congesting it, each datagram has its time to live, or number of hops it can travel before it should be discarded. |
Protocol | This specifies which upper-layer protocol sent the data. |
Header Checksum | A checksum on the header only. Since some header fields change (e.g., time to live), this is recomputed and verified at each point that the internet header is processed. |
Source/Destination Address | The IP addresses of the sender/receiver. |
In an Internet Protocol (IP) datagram, an IP header is a prefix that contains descriptive information about the packet. Nonetheless, allow me to break down its core components as shown in the table above:
Firstly we see the version, indicating the IP protocol version being used, like IPv4, and soon IPv6. The header length then follows, marking out the exact range within the dataset pertaining to the header, thereby separating it from the data.
The type of service and total length subsequently give insight into the datagram’s handling instructions and end-to-end data blueprint respectively. Crucially, identification alongside flags and fragment offset contribute to the puzzle assembly of potentially fragmented datasets.
Worth mentioning is the Time To Live (TTL) setting, a system guard against infinite data looping by setting a lifetime for each datagram. Lastly, amongst other features, there are slots for source and destination addresses to facilitate direction, the protocol and a header checksum for verifying header’s integrity.
One thing is clear; the intricacies of IP headers go beyond what meets the eye. Next time your data travels over an IP network, remember the amount of metadata coupled with it in the humble IP header, a tiny facilitator for efficient and reliable data communication on the web.[source]Diving into the technicalities of Internet Protocol (IP), it’s essential to understand the structure of an IP header, its elements and how they work in the grand scheme of internet communication. The IP header is a prefix to the data packet that contains information about the packet like its origin, destination, length, and method of transport.
The IP Header comprises 14 distinct fields, each carrying specific information. Let’s breakdown this structure:
Field Name | Description |
---|---|
Vers. (Version) | this tells us about the version of the Internet Protocol being used. |
IHL (Header Length) | this denotes the exact length of the header leaving out the data component. |
Type of Service | details about the Quality of Service requests for the network. |
Total Length | this is the combined length of the IP header and the appended data. |
Identification | a unique identification string assigned to each data fragment. |
Flags | contain values to control or identify fragments. |
Fragment Offset | indicates the exact position of a fragment within the original data set. |
Time To Live | it prevents the packet from circulating indefinitely within the network. |
Protocol | it tells which upper-layer protocol should receive the data after IP processing is complete. |
Header Checksum | used for error checking of the header information. |
Source Address | the IP address of the host where the packet was originated. |
Destination Address | the IP address where the packet is destined to go. |
Option | used for network testing, debugging, security, etc. |
Padding | ensures that the IP header has a multiple of 32 bits length. |
Let’s explore some of these fields through Python code using socket and struct libraries.
import socket import struct def ip_header(data): # Unpack the first 20 bytes of data iph = struct.unpack('!BBHHHBBH4s4s', data[:20]) # Convert bytes to bit string for Version & Header Length field version_ihl = iph[0] version = version_ihl >> 4 ihl = version_ihl & 0xF return {'Version':version,'IHL':ihl} data = b'\x45\x00\x00\x3c\x1c\x46\x40\x00\x40\x06\xa1\x7e\xc0\xa8\x01\x65\xc0\ print(ip_header(data))
Executing this snippet will extract the ‘Version’ and ‘IHL’ from the given data.
For a detailed dive into each field and further practical examples, you can visit the online documentation of Internet Protocol on IETF RFC 791.
So there we go, an exploration of the different components that form an IP header. Knowing the intricate details of the IP Header structure gives us a clearer picture of how data travels over the internet.An Internet Protocol (IP) header is a crucial component of any IP packet that travels over a network, including the internet. Two key parts of this header are the Source Address and Destination Address fields. These addresses play essential roles in packet routing and delivery.
The structure of an IP header can be represented in a tabular form:
Bits | 0-3 | 4-7 | 8-15 | 16-23 | 24-31 |
---|---|---|---|---|---|
Version | IHL | Type of Service | Total Length | ||
Identification | Flags | Fragment Offset | |||
Time to Live | Protocol | Header Checksum | |||
Source IP Address | |||||
Destination IP Address | |||||
Options + Padding (If Any) |
The Source Address and Destination Address are each 32-bits long in an IPV4 header, or 128 bits long in an IPV6 header. These are the launching point and final destination for the packet on its journey through the internet.
Importance of the Source Address:
This is the IP address of the device that originates the IP packet. The source address is pivotal for the following reasons:
- Reply: Servers use the source address to direct their responses back to the originating client. For instance, when you access a website, your computer’s IP address (source address) is used by the website server to send back the requested web page data.
- Accountability: Network security services such as Intrusion Detection Systems (IDS) employ the source address to identify potentially malicious traffic. If an IP address is consistently associated with suspicious activities, network administrators can block traffic from that address.
- Routing decisions: Routers may use source addresses to enforce routing policies in some complex networking scenarios, like source-based routing.
A sample source code which sends packets using socket programming would look something like this:
// Socket creation int sockfd = socket(AF_INET, SOCK_STREAM, 0); // Construct local address structure struct sockaddr_in sourceAddr; bzero(&sourceAddr, sizeof(sourceAddr)); sourceAddr.sin_family = AF_INET; sourceAddr.sin_port = htons(LOCAL_PORT); sourceAddr.sin_addr.s_addr = inet_addr("SOURCE_IP"); // Bind to the local address bind(sockfd, (struct sockaddr*)&sourceAddr, sizeof(sourceAddr));
Importance of the Destination Address:
The destination address guides how the packet traverses the internet. The benefits of having a destination address can be summarized as follows:
- Delivery: The packet is delivered to the device associated with the destination address. Without it, the network wouldn’t know where to send the packet.
- Routing decisions: Routers examine the destination address to determine the best path for the packet. They frequently consult a routing table, calculating the shortest, most efficient route to the destination address.
In conclusion, without the source and destination addresses in an IP header, the packets wouldn’t know where to go or where to return a response. Consequently, the communication over the internet involving millions of devices worldwide wouldn’t be feasible.
If you want to dive deeper into details of Internet Protocol, you can refer to RFC 791.
An IP header is a collection of information that includes various fields. When conducting an IP analysis, it’s crucial to understand these fields and their functions. The fields of an IP header typically contain details such as the version of IP (Internet Protocol), IHL (Internet Header Length), Type of Service, Total Length, Identification, Flags, Fragment Offset, Time to Live, Protocol, Header Checksum, Source IP address, and Destination IP address.
Version Field
This field signifies the version of IP being used. Frequently, the value is 4, indicating IPv4 is in use. Here’s how you can retrieve the version in Python:
import socket import struct def get_version(host): ip_ver = socket.AF_INET if type(socket.gethostbyname(host)) is tuple else socket.AF_INET6 return 4 if ip_ver is socket.AF_INET else 6 print(f'IP Version: {get_version("localhost")}')
IHL (Internet Header Length)
The Internet Header Length refers to the header length in 32-bit words. Thus, the minimum value is five, equating to 20 bytes.
def get_ihl(ip_header): first_byte = ip_header[0] ihl = first_byte & 0xF return ihl * 4 ip_header = b'\x45\x00\x00\x28\xab\x94\x40\x00\x40\x06\xf2\x7f\xc0\xa8\x01\x64\xc0\xa8\x01\x65' print(f'Internet Header Length: {get_ihl(ip_header)} bytes')
Type of Service
This field helps define the quality of service preferences for the datagram.
Total Length
The total length field defines the entire length of the IP packet, including the data and header, in bytes.
Identification, Flags, Fragment Offset
These fields are related to the process of fragmenting the packet across the network.
Time to Live
The TTL field prevents packets from looping infinitely. With every router crossed, the TTL reduces by one. When zero is reached, the packet is discarded.
Protocol
This field determines the protocol of the data encapsulated within the IP packet. Common values include 6 (TCP) or 17 (UDP).
Header Checksum
A checksum value for error-checking, ensuring the header arrived devoid of errors.
Source IP Address and Destination IP Address
These fields represent the sending and receiving IP addresses.
Understanding the different components of an IP header is essential for effective network data traffic analysis, aiding in troubleshooting, securing networks, and optimizing data flow. More details into these fields can be found at this IETF link.Diving straight into the topic, an Internet Protocol (IP) header carries some vital information that is required for the IP protocol to function properly. Specifically, two important fields in an IP header are ‘Version’ and ‘Internet Header Length (IHL)’.
Let’s delve deeper into the functions of each:
Version
The ‘version’ field determines the format of the internet header. This is what enables the IP packets to be interpreted correctly. In the present scenario, we have two versions of IP – IPV4 and IPV6. Here’s how a version element might appear in Python:
ip_version = ip_header[0] >> 4
The ‘version’ value for an IPV4 packet will be 4 and for an IPV6 packet, it would be 6. This differentiation aids in correctly parsing the packet while it traverses through its path to reach its destination.
For understanding purposes, the snippet code above has utilized bit-wise right shift operation (>>). A bit-wise shift operation is performed on an integer number in binary format. In the provided IP handling module, data extracted from headers are in binary format. Thus, to filter the ‘version’ and ‘IHL’ information from the IP header data header[0], bit-wise shift operations are used.
Internet Header Length (IHL)
The ‘Internet Header Length’ or ‘IHL’, refers to the length of the IP header in 32-bit words. It also marks the point where data actually begins within the IP Packet. The typical length stands at 5 words for base headers. So, an IHL value of 5 would mean that the size of the header is 20 bytes (5 words x 4 bytes/word).
The IHL can be extracted using bitwise operations like this:
ihl = ip_header[0] & 0xF
Contrary to ‘version’, to extract the ‘IHL’, bit-wise AND operation (&) was used with hexadecimal number 0xF.
To summarize, physically these values ‘Version’ and ‘IHL’ occupy only a small part of the total IP-header but figuratively, they hold great importance in setting the stage for the correct interpretation and extraction of information from the enclosed data packet.
References: RFC 791 – Internet Protocol, Bitwise operations on Integer types – Python Documentation.
Tables aren’t necessarily useful in explanation in this case but are always invaluable when one needs to describe structured data or the schema of a database.
Making sense of an IP header necessitates a deep understanding of its components. One such crucial part of the IP header is the Type of Service (ToS). Employed for several years in networking activities, it gives you leverage to prioritize network traffic and manage quality.
Now, let’s dive headfirst into understanding what ToS is and how it applies to an IP Header.
What is Type of Service (ToS)?
ToS is fundamentally an 8-bit field located in an IP header. It contains instructions on how a packet should be prioritized while being forwarded through a network. The protocol configuration allows routers to identify different types of data and treat them appropriately.
Consider this conventional representation:
0 1 2 3 4 5 6 7 +-----+-----+-----+-----+-----+-----+-----+-----+ | | | | | PRECEDENCE | TOS | MBZ | | | | | +-----+-----+-----+-----+-----+-----+-----+-----+
In this diagram:
- Precedence: Uses first 3 bits to determine packet priority where 111 denotes highest priority.
- TOS: Uses next 4 bits to indicate the service type required by the packet.
- MBZ (must be zero): Marks the final bit which should always be zero as per the IPV4 standard.
Even though the ToS field is deprecated by recent updates, it continues to maintain functional relevance in legacy systems.
ToS Significance Within an IP Header
Many mechanisms available today supersede the functionality originally offered by the ToS model. However, one cannot deny its pivotal role within an IP header.
Here’s how it brings the difference-
- Priority Assignment: It provides flexibility to assign varying levels of precedence. This enables the sorting of packets based on their criticality
- Network Traffic Control: Through effective handling of the ToS field, traffic can be optimized which results in improved network response times.
- Reliability: Assigned precedence can be used in tandem with other protocols for error detection and correction. This contributes towards superior reliability.
Generally, getting a firm grasp of the Internet Protocol and its many elements can be quite intricate, but once you understand its core components, like the Type of Service, you’ll find that it all becomes a lot clearer.
For more info on this topic, check out these reference:
RFC 791 – Internet Protocol and
Cisco’s Guide on IP Type of Service.
The Internet Protocol (IP) Header is a crucial aspect of IP packets, responsible for routing the data units across network boundaries. Each IP packet carries metadata associated to it in its header section. One such crucial piece of information is a field referred to as ‘Total Length’.
Total Length Field in an IP Header
By definition, the Total Length field in an IP header is part of the DATAGRAM FORMAT that specifies the entire datagram size, including header and data, measured in bytes. The maximum length is 65,535 bytes when derived from the largest value fitting in the two-byte wide total length field.
/* STRUCTURE OF AN IP HEADER */ struct ipheader { unsigned char iph_ihl:5, iph_ver:4; unsigned char iph_tos; unsigned short int iph_len; // THIS IS THE TOTAL LENGTH FIELD IN AN IP HEADER unsigned short int iph_ident; };
Essentially, it dictates how long the IP datagram needs to be, directing routers or any device processing it, on where the current packet ends and the next begins. It is especially important when packets are fragmented or reassembled in respect to their journey through different networks with varying maximum transfer unit sizes.
If you’re interested in pulling out this value directly from an IP header using coding techniques, this can be accomplished by particularly addressing the second pair of bytes within the IP header structure. Keep in mind that values within network headers are typically transmitted in network byte order (big-endian representation). Hence, your end system architecture influences the process required to correctly interpret this data.
For instance, if you’re dealing with raw socket programming in C, here’s how you would extract the Total Length field:
/* Extracting total length field from an IP Header */ // Assume we have a valid IP packet in variable named packet struct ipheader *ip = (struct ipheader *) packet; // Convert from network byte order to host byte order int ip_packet_length = ntohs(ip->iph_len); printf("Total Length = %d\n", ip_packet_length);
Remember the importance of taking endianess into account. The function ntohs() takes care of converting the value from network byte order (big-endian) to host byte order.
Implications of the length of an IP Packet
It’s worth mentioning why it’s so vital to understand the ‘Total Length’ field in an IP header along with other equally significant entities residing there. Packets could be lost, corrupted, or delayed due to a myriad of reasons while being transmitted through the internet. This could leave a receiving device with potentially fragmented or disorderly received chunks of data, puzzling over their proper arrangement and producing inaccurate results.
By employing the Total Length field wisely, you can ensure efficient packet management at every hop in the network journey.
Note: There is much more to IP Headers than just the Total Length field. If you wish to delve deeper into understanding each component’s roles and significance, RFC 791 is always a great starting point.
The Identification field is one central component contributing to the effectiveness of an Internet Protocol (IP) header. Understanding its functionality can make grasping the workings of IP headers far more accessible.
The Identification Field
The identification field in an IP header is a crucial element, which plays a pivotal role when involved with communication over the Internet. If you take a look at this schema, it depicts an IP header:
Version | IHL | Type of Service | Total Length |
---|---|---|---|
Identification | Flags | Fragment Offset | Time To Live |
Protocol | Header Checksum | Source IP Address | Destination IP Address |
Options + Padding |
When having numerous data chunks navigating the network at similar moments, how does every single fragment get treated as a unique entity? The answer lies in the IP header’s identification field.
Functionality of The Identification Field
The identification field assigns each different packet an identifier. Its primary function relates to aiding in the reassembly of fragmented data packets. For instance, if a data packet is chunkier than the Maximum Transmission Unit (MTU), the system will dissect it into more manageable pieces. Every resultant fragment carries the same ID as its parent, thus providing some semblance of order amidst potential chaos.
An imperative way of code that sets or reads the identification field looks roughly like this:
struct iphdr *ip_header; /* Setting an ID */ u_int16_t id = 5; ip_header->id = htons(id); /* Reading an ID */ id = ntohs(ip_header->id);
Results from “htons” and “ntohs” functions are tremendous because they ensure that endianness issues don’t muddle up your fields. This principle observes the byte order used by network protocols, denoted as the Network Byte Order, diverges from your local machine’s Byte Order. Henceforth, these functions transpose bytes into the right place.
Every time a packet defies the odds by passing through a network successfully, credit should go to several players, including the seemingly insignificant identification field. Although tiny, this unheralded part of the IP header could hold the key to understanding why the internet operates with clockwork precision.
For a deeper dive into the individual elements in an IP header, such as the identification field, consult resources such as RFC 791, the official specification for the Internet Protocol. Online education platforms also provide valuable insight into IP headers’ intricate working mechanisms.
When discussing internet communication and data transmission over networks, it’s critical not to underestimate any component’s value – especially not the often-overlooked identification field.
The Internet Protocol, or IP, is a crucial protocol that is employed in the internet layer of the Internet protocol suite. It delivers datagrams between hosts identified by their IP address. An essential feature of the IP protocol is its header: which includes metadata about the packet being transmitted. One of the chief fields in an IP header is the Time to Live (TTL) field.
Understanding the Time to Live (TTL) Field
The Time to Live (TTL) field is an 8-bit binary digit field in the IP header. The primary role of this field is to prevent data packets from looping indefinitely within the network. Each time a packet traverses a router, the TTL value is decreased by one. If the TTL hits zero before the packet reaches its destination, the packet is discarded.
// sample representation of a simplified imaginary IP // packet containing just few fields for illustrative purpose { "version": 4, "headerLength": 20, "totalLength": 200, "identification": 12345, "flags": AllZeros, "fragmentOffset": 0, "TTL": 64 // Here is the TTL field }
This concept is important, as it stops a packet from endlessly bouncing around the network due to routing errors or cyclic routing paths. Also, given that each router must process every received packet, preventing these “endless” packets helps avoid unnecessary strain on network infrastructure.
Other Vital Fields in an IP Header
Although the focus here is on TTL, an IP header contains other vital fields besides TTL each with its own significance. Here’s a quick glimpse into some of the other main ones:
Field | Description |
---|---|
Version | This field specifies the version of the Internet Protocol. |
Header Length | This represents the length of the header, which allows for variable length headers. |
Total Length | This is the total length of both the header and payload (data). |
Identification | A unique identification number assigned to each datagram. |
Flags | Used for fragmentation control. |
Fragment Offset | If a datagram is fragmented, this field tells where in the datagram this fragment belongs. |
Checksum | Used for error checking of the header information. |
To further explore the intricacies of an IP header and understand how each field plays an integral part in transferring data across networks, you can refer to official RFCs like RFC 791 that details the Internet Protocol.
Indeed, one of the most significant fields in an IP (Internet Protocol) Header is the Protocol field. Protocol field plays an essential role in guiding the data transmission process across network interfaces. The Protocol field primarily identifies the upper layer protocol that is used by the payload data carried in the IP packet.
The Internet Assigned Numbers Authority (IANA) officially assigns values for this field. Valuable examples include
1
for ICMP (Internet Control Message Protocol),
6
for TCP (Transmission Control Protocol), and
17
for UDP (User Datagram Protocol).
Since we are looking at how an IP header works, here’s a quick view of a typical IPv4 header structure:
Version | IHL | Type of Service | Total Length |
---|---|---|---|
Identification | Flags | Fragment Offset | |
Time to Live | Protocol | Header Checksum | |
Source IP Address | Destination IP Address | ||
Options + Padding |
This structure comprises different fields dedicated to specific functions. Here, the Protocol field finds its space, vital enough to decipher the kind of protocol being handled. This information is crucial as it facilitates the routing of packets to their appropriate destinations.
Without the Protocol field in an IP header, it would be challenging to correctly route packets over a network. When a router receives an incoming packet, the Protocol field informs it about the type of protocol that it needs to send up to the transport layer.
For instance, imagine if a packet arrives with the Protocol field set to
6
. The router would then understand that the IP payload must be passed on to the TCP processing section. If the value was
17
, conversely, it would be delivered to the UDP processing unit.
Recognizing the integral nature of the Protocol field can truly help one comprehend the architecture of Internet communication protocols – the backbone of today’s global entity propagation.
Links for more understanding:
IP Protocols RFC
IANA Protocol Assignments
Here is an example that parses an IP packet including the Protocol field in Python:
import struct def parse_ip_header(data): ip_header = struct.unpack('!BBHHHBBH4s4s', data[:20]) version_ihl = ip_header[0] version = version_ihl >> 4 ihl = version_ihl & 0xF iph_length = ihl * 4 ttl = ip_header[5] protocol = ip_header[6] s_addr = socket.inet_ntoa(ip_header[8]); d_addr = socket.inet_ntoa(ip_header[9]); print(f"Version : {str(version)}, IP Header Length : {str(ihl)}, TTL : {str(ttl)}, Protocol : {protocol}, Source Address : {str(s_addr)}, Destination Address : {str(d_addr)}") return data[iph_length:]
So, to grasp how the internet operates, it is pivotal to understand the implication and interpretation of each field within an IP Header. Among those, as denoted earlier, the Protocol field profoundly influences the routing and processing of IP packets.When discussing what is in an IP header, a critical component to highlight is the checksum. This is an essential feature of an IP header and operates as its error-detecting system.
The IP checksum is typically a 16-bit field within the header that ensures data integrity during transit RFC 791. It’s calculated through a straightforward summation process: every 16-bit word of the header is added together (ignoring any overflow), and then the result is one’s complemented.
This binary mathematical operation is performed by the device sending the data package and the receiving device. The sending device calculates the checksum based on these bits and includes this sum in the IP packet. Upon receipt at the target, the destination device conducts the same operation on the received data to produce a checksum value, which it compares to the value embedded in the message. If the two don’t match, an error has occurred during transmission, and the packet can be discarded or a request for retransmission can be made.
public short calculateChecksum(byte[] buf) { int length = buf.length; int i = 0; long sum = 0; while (length > 0) { sum += (buf[i] << 8 & 0xFF00) | (buf[i + 1] & 0x00FF); i += 2; if ((sum & 0xFFFF0000) > 0) { sum = sum & 0xFFFF; sum += 1; } length -= 2; } return (short)(~((sum & 0xFFFF) + (sum >> 16))); }
Let’s discuss the structure of how this 16-bit field fits into an overall IPv4 header graphic represented as a table:
Field Description | Size (Bits) |
---|---|
Version | 4 |
IHL (Internet Header Length) | 4 |
Type of Service | 8 |
Total Length | 16 |
Identification | 16 |
Flags | 3 |
Fragment Offset | 13 |
Time to Live | 8 |
Protocol | 8 |
Header Checksum | 16 |
Source IP Address | 32 |
Destination IP Address | 32 |
Options (if IHL > 5) | Variable |
Note that in the IP header, we have the ‘Header Checksum’ positioned as an integral part of the overall architecture delivering complexity-free, yet effective error detection.
In essence, the checksum serves a crucial function within the IP header. By guaranteeing the accuracy of transmitted data, checksums ensure seamless communication in the digital sphere despite inherent network instability. It’s a robust front-line mechanism detecting errors related to bit-level data corruption during the transport process.When talking about the importance and depth of flags and fragment offsets, one cannot ignore its fundamental relationship with Internet Protocol (IP) headers. This is because these key elements play an integral part in shaping IP fragmentation and reassembly procedures.
Flag and Fragment Offset: Basic Concepts
An IP header’s structure consists of several components. However, our main focus today will be on Flags and Fragment offset fields. Regarded as vital aspects of the IP header, these entities are instrumental in controlling the IP packet’s fragmentation process.
Every IP packet includes a
FLAG
field. Consisting of three bits—namely, ‘Reserve Bit’, ‘Don’t Fragment’ (DF), and ‘More Fragment’ (MF)—this sector plays a crucial role. The DF flag prevents the IP packet from getting fragmented, while the MF flag notifies if there is more data to follow or not. Remember, for the last fragment, the MF flag is always set to zero.
In contrast, the
Fragment Offset
helps indicate where a certain fragment fits within the original IP datagram. Fundamentally speaking, this field tells us the starting position of the data contained in a fragmented IP packet, thereby making it easier to rearrange the fragments back into the original order.
With their roles understood, let’s now dive deeper into understanding their significance.
The Importance of Flags and Fragment Offset
IP fragmentation serves an essential purpose in data transmission operations. It breaks down large packets into smaller ones so that they can traverse networks with varying Maximum Transmission Unit (MTU) sizes. Here’s where Flags and Fragment Offset step into the picture.
– Preventing Unnecessary Breakdown: The ‘Don’t Fragment’ flag ensures that packets are not unnecessarily broken down, especially during routing via networks with smaller MTUs. When a packet larger than the MTU size tries to get across, instead of breaking down, it gets discarded due to the DF flag’s presence.
– Identifying Packet Conclusion: ‘More Fragment’ lets us know when we have received all fragments since it is set to 0 for the final fragment. This information prevents premature assembly attempts, thus avoiding data corruption.
– Ensuring Correct Assembly: The Fragment Offset assists in properly assembling the fragmented packets back into their original form. With each unit representing eight bytes of data, it defines the accurate place where a packet piece needs to fit in, ensuring seamless reassembly.
An Example to Illustrate:
For instance, consider an IP datagram of 4000 bytes needing to travel through a network path having an MTU limit of 1500 bytes. In this case, IP fragmentation breaks down this datagram into three parts—one of 1480 bytes (
Fragment Offset = 0
, with MF = 1 indicating more fragments) and two of 1500 bytes (
Fragment Offset = 185 for the second, and Fragment Offset = 370 for the third
. The ‘More Fragment’ bit in the last fragment would be 0, signaling that it’s the last fragment).
To conclude, Flags and Fragment Offset both lie at the core of IP fragmentation mechanics. These constituents mark the distinction between seamless data communication and complete mess, pulling off the complexity of data packet assembly perfectly.
Refer to RFC 791 for more insights on IP Headers, Flags, and Fragment Offset.The IP (Internet Protocol) header is an integral part of the data packet sent and received over a network. By understanding two key areas within this header—the Options and Padding Areas—we can develop a deeper appreciation for how digital communication is organized and delivered, from its precise time of departure to its final destination.
Let’s delve into these topics one-by-one:
Options Area
The Options Area in an IP Header allows altering the default behavior of IP. Unlike other sections of the IP Header, this section is not mandatory; it might be absent sometimes. The Options field has variable length, it can range between 0 to 40 bytes.
The primary purpose of this area relates to network testing, debugging, security, and handling special types of data. In particular, this function supports:
- Record Route: It monitors the route that packets take when traversing a network.
- Timestamp: This option is added to store the timestamp of each point along the route.
- Security: Security level is specified as per the United States Department of Defense requirements.
- Loose Source Routing: It permits the sender to give a loose list of routers that IP should use to reach the destination.
- Strict Source Routing: It permits the sender to specify the exact router sequence for routing the packet till the destination.
Let me show you what a typical Options Field looks like:
Copy Flag : 3 bits Option Class : 2 bits Option Number : 5 bits Option Length : Variable Option Data : Variable
Padding Area
The main intention of this Padding attribute is to ensure that whatever combination of options are included, the header ends on a 32-bit boundary. It basically uses extra zero bits to fill out the header to its required size. A header must be a multiple of four bytes (which equates to 32 bit). If it doesn’t quite fit, then padding bits are used to bump it up to the next multiple. The simplicity of this method also helps to reduce processing overheads when constructing or decoding packet headers.
To showcase this, imagine if an IP Header’s total length came to 457 bits. To make it fall on the 32-bit boundary, we will have to add 7 more bits (padding bits). So, after padding the total length of the IP header becomes 464 bits which falls perfectly on the 32-bit boundary.
Note: As a professional coder, I urge you to (Refer here) and read about HTTP Headers for gaining more insight about it.
In wrapping up, it’s clear that the Options and Padding Areas lend the IP header its flexibility and efficiency. They allow for customization and precision in data transmission, fulfilling needs that extend beyond the basics. It demonstrates the intricate balance between functionality, performance, and security—a testament to the core principles of computer networking.
Certainly, let’s delve into a fascinating discussion about Load Balancing, sourcing rules and features offered by modern networking technologies, all in relation to the subject of IP (Internet Protocol) Headers.
First, it is indispensable to understand what an IP Header is. It’s essentially a prefix that includes a combination of various components verifying and navigating an IP packet’s journey. Some parts include:
– Version (IPv4 or IPv6)
– Internet Header Length
– Total length
– Flags
– Time to Live
– Protocol
– Header checksum
– Source IP Address
– Destination IP Address
Now, let’s connect this with Load Balancing and Sourcing Controls Features.
Load Balancing:
Load balancing is a method for evenly distributing network traffic across multiple servers, ensuring no single device gets overwhelmed. How does it relate to the information contained in an IP header?
Well, when a packet is destined for a load balanced service, Load Balancers decide on which server to forward that request. They examine the destination IP address from the IP header of the packet, along with other factors like current server load and health status, to make their decision. The selected server’s IP then replaces the original destination IP in the header before the packet is sent off again.
For instance, suppose you have the following simple configuration of a Load Balancer:
LB1: Server1 – 10.0.0.1 Server2 – 10.0.0.2 Server3 – 10.0.0.3
Assume the incoming packet has a destination of LB1’s IP. The Load Balancer might determine Server2 as the optimal choice given its current situation. Thus, before forwarding the packet, the destination IP will shift from the Load Balancer’s IP to the selected server’s, so in this case, ‘10.0.0.2’.
Sourcing Controls:
Sourcing Control, known in technical jargon as Source Routing, enables the sender to define the path packets should follow through the network. Here too, our trusty IP headers come into play.
In Source Routing, the sender places intermediate and final destination IPs inside the IP header of the packet. This roll call dictates the specific order nodes or routers the package will traverse en route to its endpoint.
The feature can be really handy for certain conditions where specific route control is required, such as:
– Debugging, testing, or troubleshooting paths.
– Preventing “ping pong” effects when two Load Balancers keep referring a client to each other.
All in all, the intelligence and functionality associated with Load Balancing and Sourcing Controls owe a significant amount to the little packet prefixes we call IP Headers. Through their included data, these operations can more decisively mate packets to servers or preferential routes.
Note: Tables were not included due to the nature and context of the query. But depending on the entirety of your article, you could incorporate one tabulating distinct fields within an IP Header.Delving deeply into the understanding of an IP Header, it’s clear how essential it is in providing crucial information for data transmission over a network. An IP header contains specific fields to ensure that packets are routed properly and efficiently. What goes inside an IP Header?
– The Version Field signifies the version of the Internet Protocol.
– The IHL (Internet Header Length) defines the complete length of the IP header.
– The Type of Service (ToS) specifies the purpose of using certain service parameters in the datapacket.
– The Total Length, as the name suggests, is the total length of the packet including both the header and data.
– The Identification Field assigns a unique identifier for each datagram.
– The Flags field informs about fragmentation status of the IP Datagram.
– The Fragment Offset reflects the starting position or offset of the data part relative to its original entity.
– The Time to Live (TTL) field stops a datagram from looping indefinitely across the network.
– The Protocol Field describes which protocol will be consuming the data content.
– The Header checksum helps with error control by checking integrity of received packets.
How these details are integrated and their effect on interactions between networks define the strength and reliability of our internet connections.
An interesting point to note here is how these fields can be manipulated. As pointed out by RFC 791 (Internet Protocol, DARPA Internet Program Protocol Specification), while the IP doesn’t essentially provide a method to handle errors during the transmission process, the use of
Header Checksum
provides a high level of error-checking functionalities.
From the source to its final destination, every step is determined using this information, ensuring seamless communication with agreeable margins of error.
Furthermore, having the knowledge of these IP fields can prove beneficial in enhancing security measures. For instance, being aware of abnormal TTL values can help detect potential intrusions in a network, highlighting the importance of understanding the packaging of data within an IP header.
In conclusion, the IP header serves as a cornerstone in shaping the communication foundations over the internet. By providing necessary details like addressing, fragmenting, routing, and dealing with errors, it ensures optimal communication over a network. As we delve further into the digital age, the importance of such protocols only becomes more relevant, bridging different platforms and technologies with efficient translation of information.