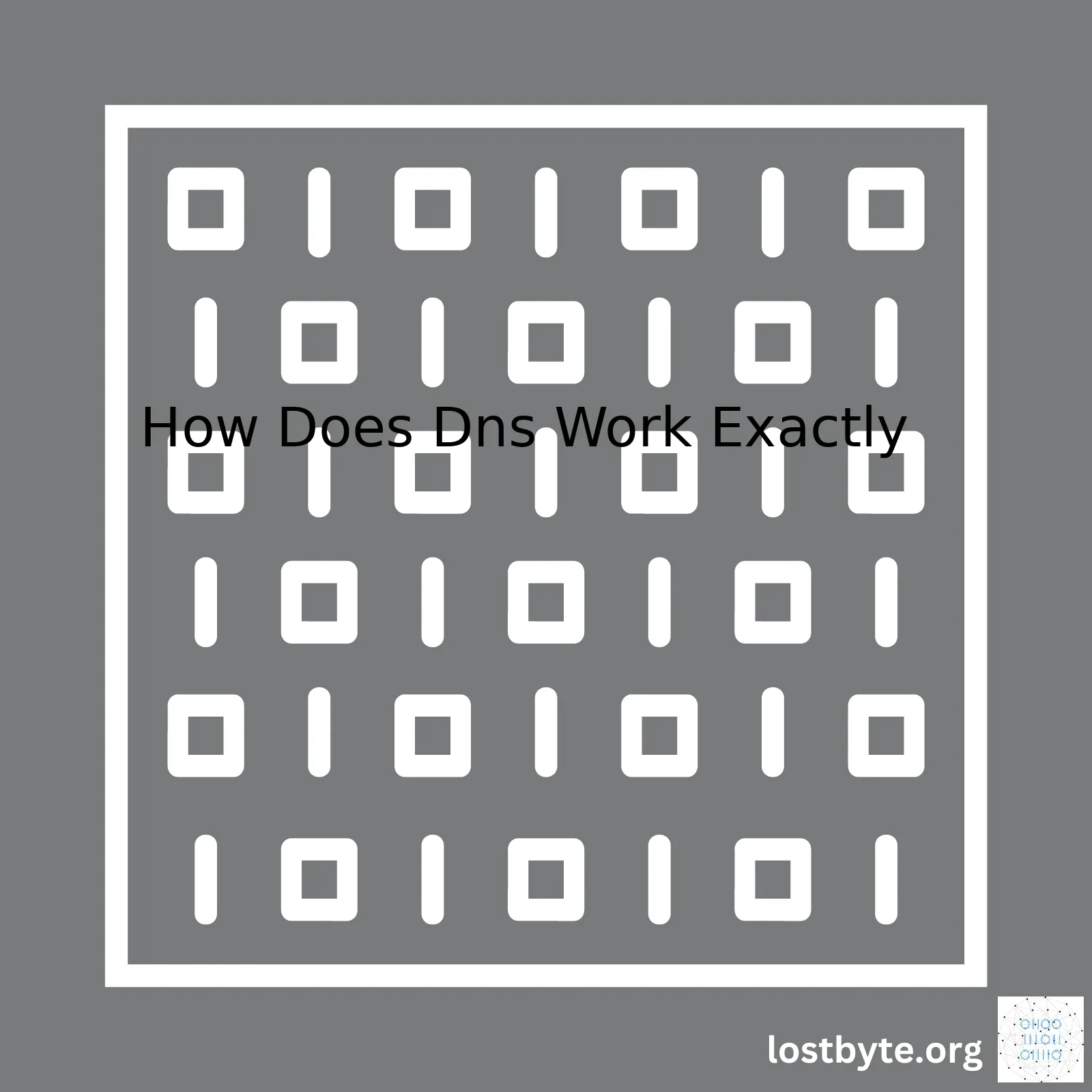
Process Steps | Description |
---|---|
URL Input | You enter a URL in your web browser. |
DNS Resolver Query | Your system sends a query to a DNS resolver, typically managed by your ISP. |
Root Nameserver Query | The DNS resolver sends a query to the root nameservers. |
TLD Nameserver Query | The root nameserver points the resolver to a TLD (Top-Level Domain) nameserver, depending on the domain’s extension (.com, .net, etc.). |
Authoritative Nameserver Query | The TLD nameserver redirects the resolver to the authoritative nameservers for the specific domain. |
IP Address Response | The authoritative nameserver responds with the IP address of the domain you queried. |
Webpage Request and Loading | Your system sends a request to that IP address and loads the content of the webpage. |
When you type a URL into your web browser, it initiates a sequence of events to translate that human-friendly URL to a machine-friendly IP address. It’s like looking up a phone number in a contact list – you know the name (the URL), but you need the numerical address (the IP) to place the call.
First, your system sends a query to a DNS resolver, a server typically managed by your Internet Service Provider. The DNS resolver then sends an inquiry to root nameservers at the top of the hierarchical DNS system.
The root nameservers examine the extension of your queried domain (like .com or .net) and point the DNS resolver to the appropriate TLD (Top-Level Domain) nameserver.
Then, the TLD nameserver guides the resolver to the authoritative nameserver for the exact domain you’re attempting to access. This authoritative nameserver holds the actual IP address for that domain.
Once your system receives this IP address, it uses it to initiate a connection and load the relevant webpage, finally displaying the page you initially typed into your browser.
This complex process happens within milliseconds. DNS caching allows frequently visited sites to skip several steps, as their IP addresses are stored locally for quicker access.
For more detailed explanation, check out this online tutorial. For actual implementation check
dig
command which can trace dns resolution process, refer to this resource.The Domain Name Service (DNS) is a crucial foundation of any internet network. It’s the very essence that connects domain names with their corresponding Internet Protocol (IP) addresses, allowing users to access websites with common language names rather than numeric IPs.
How DNS Works:
Essentially, think of DNS as an internet phone book. Just how phone books connect people’s names with their phone numbers, DNS aligns web domains with IP addresses. The process behind DNS is beautiful in its simplicity. Whenever you type a website’s URL into your browser and hit Enter, the following sequence occurs:
- DNS Query: Your computer generates a query asking for the IP address associated with the domain name you’ve typed in.
- DNS Recursion: A series of servers are visited until the request meets a DNS server that knows the correct IP. Initially, this involves three distinct sets of servers: DNS Resolver (usually provided by your ISP), Root server and Top-Level Domain (TLD) server. The last one leads us to the Authoritative Nameserver which holds the actual DNS records.
- DNS Response: The definitive DNS server returns the accurate IP address to your computer.
- Connection Establishment: Finally, armed with the Website’s IP address, your computer can now establish a connection with the site’s server and load the webpage.
window.location = WEBSITE_IP_ADDRESS
To further illuminate the subject, see the table below for a breakdown of each server’s function:
Server Type | Function |
---|---|
DNS Resolver | Responsible for making the initial request. The ‘ Seeker’. |
Root Server | Tells where to find the TLD Server. |
TLD Server | Directs to the website’s DNS Server. |
Authoritative Nameserver | Holds the DNS records. The ‘Knower’. |
It’s impressive to note that all these steps happen within milliseconds, creating a seamless browsing experience for the user.
In a quest to ensure optimal internet performance, DNS employs caching. Caching stores IP values locally on the DNS Resolver after a successful query completion so that the next time the same domain is requested, the whole recursive search doesn’t have to be repeated.
But no system is perfect. Occasionally, users might encounter errors like “DNS_PROBE_FINISHED_NXDOMAIN”. This typically indicates that the DNS server tried to resolve the domain, but it didn’t exist in the DNS record. In such cases, checking if the entered URL is correct or modifying DNS settings might resolve the issue.
For more detailed insight, refer to this Cloudflare DNS Guide. Understanding the workings of DNS is integral in areas such as setting up your own site or debugging network issues.
So, whether you are an end-user trying to navigate the internet or a seasoned programmer working on an application meant to interact with other servers over the internet, DNS plays a crucial role. By ensuring a swift conversion from human-friendly URLs to machine-friendly IPs, DNS forms an invisible yet vital thread in the vast Web.The Domain Name System, also known as DNS, plays an indispensable role in how the internet operates. It is quite similar to a phonebook of the internet. People access information online through domain names, like google.com or bing.com. But web browsers interact through Internet Protocol (IP) addresses, which are far less human-friendly than domain names. The interaction between these two different ways of understanding the internet’s location marks where DNS comes into play.
Understanding the Basics of DNS
The Exact Working of DNS
DNS follows a sequence of steps each time you enter a website URL, with the goal to translate the easily remembered web address into the numerical IP address that computers can understand:
– Step 1: You Enter a URL
Typically, you start by typing a URL into your browser.
– Step 2: Request Sent to Network’s Recursive DNS Servers
Next, the DNS query travels over the internet and meets the network’s local recursive DNS servers. These are generally handled by your internet service provider, but third-party DNS servers exist too.
– Step 3: Query Travels to Root DNS Servers
If the recursive servers don’t have the required details, they contact the root DNS servers. These do not know the details either but can direct the query towards those that might, such as Top-Level Domain (TLD) DNS Servers.
– Step 4: Inquiry Proceeds to TLD DNS Servers
From here, the query will go to one of many TLD DNS servers responsible for their specific domains (.com, .org, etc.). These servers won’t have the complete records, but they’ll indicate where to find more detailed information, specifically in the authoritative DNS servers.
– Step 5: Authoritative DNS Servers Give the Answer
Finally, the authoritative DNS servers store the DNS records for their respective domains. If the domain is valid, they provide the corresponding IP address. This information returns through the chain to your network’s recursive DNS servers, followed by your system, and then at last to your browser.
At first glance, this process might seem long-winded and slow. However, everything goes so promptly due to caching at multiple levels that the delay is imperceptible in most cases. DNS makes it possible for us to access websites with speed and accuracy on our normal day without taking the burden of remembering a ton of numerical IP addresses.
Here is a
DNS resolution code example
for Python using the
socket
module:
import socket IP = socket.gethostbyname('google.com') print("The IP Address of google.com is: ", IP)
This script requests the DNS server and gets the IP address associated with ‘google.com’. When you run this script, you’ll get the IP address as output.
To better visualize the information about DNS explained so far, we present the following illustrative table.
Action | Description |
---|---|
You Enter a URL | User types in a domain name (URL) in the web browser. |
Request to Recursive DNS Servers | The DNS query sent to the network’s recursive DNS servers. |
Query Travels to Root DNS Servers | If the request cannot be resolved locally, root servers provided information to further route the query. |
Inquiry Proceeds to TLD DNS Servers | The root servers point the recursive servers to the appropriate TLD servers. |
Authoritative DNS Servers Provide the IP Address | The TLD servers point to the authoritative DNS servers, offering the exact IP address matching the domain name. |
Understanding DNS is crucial because it underpins nearly every action taken on the internet. It enables a fluid communication method between the human-friendly domain names we know and love, and the machine-friendly IP addresses computers use to locate each other on the vast network of networks that make up today’s internet.Sure, let’s dive deep into the nuts and bolts of DNS, short for Domain Name System.
Talking about its fundamental role, it acts as the internet’s directory. Just like you wouldn’t know where to send a letter without knowing someone’s address, a computer wouldn’t know how to reach a specific website or other web services without knowing their respective internet addresses, also known as Internet Protocol (IP) addresses. This is where DNS comes to the rescue.
Understanding DNS: Translating Human-Friendly URLs into IP Addresses
When you type in a human-friendly URL, say www.google.com, the DNS quickly transforms the domain name into the corresponding IP address that computers can read and understand for smooth connectivity. Under the hood, it’s much more complex.
// Example of an IP Address 192.168.0.1
Every device connected to the internet has a unique IP address, which is essential for communication. Deciphering those numerical strings by humans can be hard, but not for computers. DNS bridges this gap between convenience for humans and the effectiveness for machines.
How Does DNS Work? Unveiling the Four Core Steps
The process of a DNS resolution, the conversion of a domain name into an IP address, typically involves four fundamental steps:
– DNS Recursive Resolver: The DNS resolver is your first point of contact whenever you make a request for a domain. The Internet Service Provider (ISP) usually hosts it and is responsible for making indirect requests to DNS nameservers on your behalf.
– Root Nameserver: A root server is the first step in translating friendly domain names into IPs. It doesn’t know the IP for the domain but directs the query to Top-Level Domain (TLD) servers knowing where to fetch that information.
– TLD Nameserver: The TLD server handles the next chunk of the URL. In case of www.google.com, it’s the “.com” part. This nameserver won’t have the exact IP either, yet guides the request further to the appropriate Authoritative Nameserver.
– Authoritative Nameserver: This server has the exact IP address mapping for the requested domain name. Once the final bit of information is received, it’s passed back through the chain until it reaches the DNS Resolver again.
These reference points lay the groundwork for how DNS operates, ensuring seamless internet navigation while masking a complex infrastructure underneath. Its importance cannot be understated; it allows us to use the internet in a human-understandable way while optimizing for machine-level efficiencies.
For a more visual approach, imagine walking to the library, finding the appropriate section for the book you’re looking for, then going to the correct shelf, and finally the precise location where the book resides. Each step you take from entering the library till reaching your desired book is similar to how a DNS request is routed.
Remember, this whole process happens in milliseconds, keeping your browsing experience smooth and fast-paced. Next time you enter a website URL, remember DNS effortlessly working behind the scenes ensuring your optimal online journey. As you now see, understanding DNS is essential for anyone working with internet technologies, programming, or system administration.
Diving straight into my description, the Domain Name System (DNS) is an intriguing aspect that plays a vital role in how we access information online. The architecture of DNS is essentially a hierarchical and distributed database system designed primarily around two types of servers:
Authoritative DNS servers
and
Recursive resolver servers
.
On one side, you have
Authoritative DNS servers
. These are the trusted, reliable sources holding the key to mapping domain names to their corresponding IP addresses. They have the “authority” over certain domains, thus the name, maintaining a directory of domain names and translating them to IP addresses upon requests.
To illustrate this, consider this example. If you’re seeking information on the www.example.com website, the server with authority over the ‘.com’ domain can point the way to the server managing ‘example.com’. Simple, right?
On the other side of the coin, we have the
Recursive resolver servers
. These are more like middlemen in this DNS architecture. Their job—if you want to think of it this way—is to chase down the IP address for a host or domain name on behalf of a client (like your web browser). Recursive resolvers have no authority but are rather reliable couriers taking on the task of communication between your computer and authoritative servers till they find what you are looking for.
Now let me give you a typical scenario:
You decide to visit a particular website, say www.myfavoritewebsite.com. Here’s the process, step-by-step:
- Your browser checks its local cache to see if it already has the IP for this site stored.
- If not, a request is sent to a recursive resolver.
- The recursive resolver reaches out to the root nameserver which directs it to the TLD (Top Level Domain) for ‘.com’.
- The TLD server then leads the recursive resolver to the authoritative DNS server for ‘myfavoritewebsite.com’.
- Finally, the DNS record for ‘www.myfavoritewebsite.com’ is retrieved by the recursive resolver and passed back to your browser, which can now fire up a connection to the website using the provided IP address.
And voila! You’re staring at the homepage of your favorite website. This all happens in microseconds, which makes the internet seem instantaneous from our perspective!
This whole process described above can be represented by the following table:
Step | Action |
---|---|
1 | Browser checks local cache |
2 | Request to recursive resolver |
3 | Recursive Resolver contacts root server |
4 | Root server redirects to TLD server |
5 | TLD server redirects to authoritative server |
6 | Authoritative server provides DNS record |
7 | IP Address returned to browser |
8 | Website loaded using IP address |
The resilience of the Domain Name System comes from its distributed nature. No single failure can bring the system down because the information is held in multiple locations—across various servers globally. To add another layer of reliability, DNS data is cached at multiple levels to speed up resolution and reduce traffic.
When it comes to the nitty-gritty of coding, well, interacting with the DNS is often taken care of by libraries and frameworks. However, if you are interested in managing DNS records or writing applications that interact with the DNS at a lower level, you may want to check out Python’s “dnspython” library (dnspython.org). It allows for very flexible and powerful interactions with DNS servers, including sending arbitrary DNS messages and interpreting responses.
In essence, DNS is pivotal to the infrastructure of the internet as we know it today. A simple yet effective solution to the daunting problem of remembering IP addresses, it opens up the endless knowledge of the internet to the users worldwide.
The domain name system, or DNS, serves as the internet’s contact book. It translates human-readable domain names into an Internet Protocol (IP) address that machines can read. This process is essential for network communication, loading web pages, and running numerous other online operations. A solid understanding of how DNS resolves names into IP addresses helps shed light on this complex process.
DNS Working Mechanism
While browsing the internet, you generally type in a URL, such as www.google.com. Your computer doesn’t exactly understand what www.google.com means. Instead, it communicates with other machines using IP addresses. To contact the server where Google’s site resides, your computer needs its IP address. That’s where DNS comes to the rescue!
nslookup google.com
The typical steps involved in the transformation of a domain name into an IP address are as follows:
- DNS Query : Your device sends a request known as a ‘query’ asking for the IP address associated with the domain name.
- Recursive DNS Servers: Your Internet Service Provider (ISP) redirects this query to a recursive DNS server. These servers have the sole purpose of receiving DNS queries and responding with the best answer.
- Root Servers: If the recursive DNS server does not already know the IP address, it sends the query to one of the world’s 13 root servers. The root server returns the address of the top-level domain (TLD) DNS server, which stores the information about domains under that TLD (like .com).
- TLD DNS Server : From here, the ISP forwards the query again, this time to the received TLD DNS server address. They respond with the IP address of the respective domain’s DNS server.
- Domain DNS Server : This server finally carries the response containing the IP address of the queried domain name (www.google.com, for instance). As they hold the IP information for specific hostnames, this ends the search.
- Response to Client: The recursive DNS server then returns the IP address to your device. Your device then saves this IP in its DNS cache for future reference, so another round of searching won’t be required if you visit the same website again soon. Browsers also store DNS information in their cache.
Process | Responsibility |
---|---|
DNS Query | Requests for the IP address associated with the domain name |
Recursive DNS Servers | Receives DNS queries and respond with the best answer |
Root Servers | Returns the address of the TLD DNS server |
TLD DNS Server | Responds with the IP address of the domain’s DNS server |
Domain DNS Server | Carries the response containing the IP address of the queried domain name |
Response to Client | Returns the IP address to the querying device |
Additional terms worth mentioning here are “DNS Resolver,” another name for recursive resolvers, and “Authoritative Nameservers,” which usually operate at the TLD and domain level DNS servers. ISPs often manage recursive resolvers while organizations like Internet Corporation for Assigned Names and Numbers (ICANN) handle authoritative nameservers. Feel free to delve deeper if you’re interested. Here’s the link to ICAN’s website
In summary, resolving domain names to IP addresses relies heavily on the efficient functionality of DNS. Even though the process seems complex, it typically happens within milliseconds. DNS intricacies are handled behind the scenes, allowing users to enjoy a seamless internet experience.
As an adept coder, delving into the intricate world of DNS (Domain Name System) is both fascinating and functional. The DNS, essentially, serves as the Internet’s phonebook – transitioning human-friendly website addresses like ‘google.com’ into IP addresses that systems can interpret. This sophisticated mapping relies heavily on recursive resolution. To frame this in a concise way, I’ll break down the process.
Our rendezvous with recursive resolution in DNS begins when we type a URL into our browser bar – say, https://www.google.com. The system is unaware of the corresponding IP address hence, it needs to inquire from the DNS.
Let’s detail this process using code snippets:
Browser: "Hey system, do we know the IP for www.google.com?" System: Checks local cache
If the IP is present, great! If not, the browser asks your configured DNS server, generally your ISP (Internet Service Provider).
Browser: "ISP DNS, do we know the IP for www.google.com?" ISP DNS: Checks its cache
Now, if your ISP has no idea either, we step into the realm of DNS recursion – which involves the following:
* Root DNS Server: This is our starting point. Computers have a hardcoded list of these (IPv4 and IPv6), possessing information about all Top Level Domain (TLD) servers.
ISP DNS: "Root DNS, who holds information about .com domains?"
* TLD Server: The next destination after Root DNS Servers. Each TLD (.com, .org, .net, etc.) has their respective TLD servers.
ISP DNS: "TLD DNS, who holds information about google.com domain?"
* Authoritative Server: Holds the definitive directory records for individual domain names. Once we reach this stage, the resolver retrieves the requested record and sends it back through the chain.
ISP DNS: "So, the IP for www.google.com is..."
This process might sound long-winded, but since most records are cached at multiple steps along the route, it usually occurs much quicker than expected.
Below is a simplified visualization:
Action | Query Performed By |
---|---|
Initial request | Browser |
Request to ISP’s DNS server | ISP DNS |
Query Root DNS server | ISP DNS |
Query TLD DNS server | ISP DNS |
Obtain record from authoritative server | ISP DNS |
To tinker around more with DNS or dig deeper into understanding how DNS resolution exactly works, you might want to check out Cloudflare’s DNS Learning Center. It’s a treasure trove, with expansive knowledge on DNS and how it shapes our Internet experiences day in, day out. For hands-on practice, try executing a manual DNS lookup using the ‘dig’ or ‘nslookup’ commands.The Domain Name System, often abbreviated as DNS, plays a foundational role in how the internet operates. Precisely speaking, it’s the Internet’s equivalent of a phone book, essentially mapping domain names (human-readable website addresses) to IP addresses (computer-readable numerical labels for each device).
At its core, DNS operates through different forms of servers. Among them, the Authoritative DNS Server, holds a position of utmost significance. It is an essential piece in advancing the core function of DNS, which includes:
- Maintaining a directory of domain names and their corresponding IP addresses.
- Responding directly to requests from Recursive DNS servers about a particular domain’s current IP address.
Imagine this scenario: when you type
www.example.com
into your browser, a series of requests and responses take place in the background. This interchange is the DNS process working to direct your browser to the correct corresponding IP address.
To illustrate how an Authoritative DNS Server factors into this process:
- Your computer sends a request to a Recursive DNS server, asking for the IP address associated with
www.example.com
.
- The Recursive DNS server may not have this information readily available. Therefore, it would forwards the request to the Authoritative DNS Server that is responsible for managing information for the ‘example.com’ domain.
- The Authoritative DNS Server responds with the IP address for
www.example.com
- The Recursive DNS server, now equipped with the correct IP address, forwards it back to your computer.
- Your computer directs your web browser to that IP address, loading the website content.
Here is a simplified representation that further illustrates the interaction between various entities involved in a typical DNS resolution process:
Domain Name Entry | IP Address | Server Type |
---|---|---|
www.example.com | (Forwarded by recursive DNS server) | Browser |
www.example.com | (Request received for IP) | Recursive DNS Server |
www.example.com | 192.0.2.1 | Authoritative DNS Server |
www.example.com | 192.0.2.1 | Recursive DNS Server |
www.example.com | 192.0.2.1 | Browser |
Through this explanation, I hope it is clear why the Authoritative DNS Servers are significant in this process. Artfully balancing load distribution and maintaining website accessibility, these servers operationalize the fundamental reason behind DNS’s existence – transforming user-friendly domain names into machine-friendly IP addresses.
For those who wish to dig deeper or intend to manipulate the DNS settings for purposes such as speeding up the internet connection or bypassing restrictions, you can check online resources like [How-To Geek’s article](https://www.howtogeek.com/122845/htg-explains-what-is-dns/) for a much more detailed run-through of DNS and its inner mechanisms.
This was a simple, yet profound exploration of what goes around each time a URL is typed on a browser. Hopefully, this serves as proof of the magic that resides within coding, the ability to transform complex machine interactions into seemingly simplistic human activities.Forward and reverse lookup zones are integral parts of the Domain Name System (DNS). DNS turns human-friendly domain names, like www.google.com, into binary IP addresses that machines can understand. It’s a bit like the internet’s phonebook.
Understanding Forward Lookup Zones
The primary mechanism of DNS is via forward lookup. This is essentially translating hostnames to IP addresses. Imagine you want to visit a website. You know its name – let’s take www.example.com. But your computer doesn’t recognize this; it works with numbers called IP addresses. So, how does your computer find out the IP address associated with the domain name? That’s where forward lookup zones come in!
A forward lookup zone contains information required for this transformation. It holds records with mappings of domain names to their respective IP addresses. Essentially,
DNS server
queries a forward lookup zone, retrieves the appropriate IP address for a given hostname, and returns this to the requesting client.
Deep Dive into Reverse Lookup Zones
Reverse lookup zones work in the opposite manner to forward lookup zones. As the name suggests, they provide a way for the DNS server to do a reverse query: given an IP address it will return the hostname.
In a reverse lookup zone, resource records maps IP addresses to their corresponding domain names, contrary to a forward lookup zone. Why would we need this? There could be various applications in networking for verifying identities or diagnostic purposes.
When performing a reverse lookup, the DNS server queries the reverse lookup zone, discovers the domain name associated with the requested IP address and feeds back this information to the original inquirer.
To illustrate, check the table below:
Type | Input | Output |
---|---|---|
Forward Lookup | www.example.com | 192.0.2.1 |
Reverse Lookup | 192.0.2.1 | www.example.com |
Ultimately, both forward and reverse lookup zones play significant roles in the operation of DNS, collectively delivering seamless hostname-IP conversion and maintaining the Internet navigation experience as smooth and user-friendly.
For more in-depth coverage on DNS and its operations, I suggest checking out Cloudflare’s detailed guide on What is DNS?.
DNS, short for Domain Name System, acts as the internet’s phonebook. Instead of memorizing IP addresses which are made up of numbers and dots, DNS allows us humans to use more memorable names like www.google.com. When a browser sends a request to a server using a domain name, the DNS server translates that domain name into an IP address which points to the correct machine on the wide web.
Browser -> www.google.com -> DNS lookup -> 74.125.224.72 -> Server
One aspect of DNS that significantly improves its efficiency is caching. Here’s how DNS caching works:
A local cache is created whenever a browser or operating system makes a DNS request. This cache contains temporary storage of recently accessed DNS records, which drastically reduces the DNS lookup time for subsequent requests to the same domain.
When a request for a domain name comes in, it checks the local cache first:
* If the local cache has the requested record – known as a cache hit – it returns the corresponding IP Address immediately.
* If it doesn’t have the record – known as a cache miss – it contacts remote DNS servers to find the IP Address, then saves it in the cache.
if(Cache hit){ return IP_Address; } else{ //Cache miss IP_Address = Remote_DNS_servers.lookup(domainName); Cache.save(domainName, IP_Address) return IP_Address }
The second aspect that’s critical is DNS TTL (Time to Live). DNS TTL values tell local resolver when to purge the cached data for a particular DNS record. The TTL value assigned to a DNS record determines how long this specific record can stay in a cache before it needs to be discarded and looked up again from the remote DNS server. TTL values are typically set in seconds; a typical setting might be 86400 seconds, which equals to 24 hours.
// Pseudocode if(time_now - last_updated_time > TTL){ // Expired parameter, need to re-lookup IP_Address = Remote_DNS_servers.lookup(domainName); Cache.update(domainName, IP_Address) }
Without a cap on how long responses could be cached, we could end up with stale records that lead to non-functional routing. With TTL, we’re able to strike a balance between reducing latency via caching and maintaining up-to-date records.(source)
Remember that not all DNS records are equal. Some rarely change — like a company’s primary domain — while others frequently update — like dynamic IP addresses allocated by ISPs. Servers can fine-tune their DNS TTL values based on how often those records are expected to change thus ensuring optimal network performance.(source)
DNS Record Type | Typical TTL Value |
---|---|
Primary Domain (e.g., www.example.com) | Between 24 – 72 hours |
Subdomains (e.g., blog.example.com) | Between 1 – 24 hours |
Dynamic DNS Records | 5 minutes or less |
To sum it up, DNS uses caching and TTL to efficiently route web users to the right server. Caching can greatly reduce bandwidth and improve response time, while TTLs help ensure that this system remains refreshingly accurate and updated. By understanding these concepts, you can better appreciate and troubleshoot your everyday browsing experiences.(source)Perhaps your question is about two things:
1. Understanding how DNS works.
2. Knowing what error handling mechanisms there are in the Domain Name System (DNS).
First off, to grasp how the DNS system works, let’s simplify it. Think of DNS as a phone book for the internet. You know humans remember words much more easily than numbers. So, instead of typing an IP address number sequence like `192.0.2.0`, you could type into your browser something like `
www.example.com
`. The computer doesn’t understand this human-friendly language, so DNS translates these domain names into IP addresses that computers can understand.
Here’s a simplified version of how it happens:
– Your device makes a request, querying the DNS for the specific IP address associated with the given domain name.
– This request goes to the DNS server, called the Recursive resolver.
– The resolver sends the query to a Root server.
– The Root server directs the resolver to TLD (Top-Level Domain servers—like `.com` or `.org`)
– Eventually, the request reaches the authoritative DNS servers that hold the IP address records for the respective domains. These servers send back the required IP address to the resolver.
– The resolver then replies to your device with the IP address
– With this IP address, your web browser can now fetch the website from the server hosting the content.
Quite a journey, isn’t it? Now onto the second part, Error Handling Mechanisms in DNS:
Much like every system, DNS isn’t free from faults and errors. However, there are mechanisms available in DNS to handle specific error scenarios – categorized by Response Code fields in DNS messages.
Here are examples of common DNS error response codes and what they mean:
– **NoError (0)**: No error condition, query completed successfully.
– **FormErr (1)**: The server faced trouble parsing the request message.
– **ServFail (2)**: Indicates server failure.
– **NXDomain (3)**: Signifies that the domain in the query does not exist.
– **NotImp (4)**: Means the DNS server does not support the requested kind of query.
– **Refused (5)**: The DNS server refused to perform the specified operation for policy reasons.
These error codes form part of DNS’s reliability measures, ensuring messages on problem encounters do not go unnoticed. They allow for eliminations, modifications, or implementations of new strategies in the network infrastructure as necessary.
Let’s take the NXDOMAIN response as an example:
requester -> dns.recursive.request("nonexistentwebsite.com") if dns.recursive.response == "NXDOMAIN" { return "The domain does not exist." }
This informs the user or requester system that the queried domain does not exist. As such, no further attempts to resolve this same domain will occur until the cache record expires.
You may want to delve further into this topic by reading relevant materials onlineWikipedia’s page on DNS is quite informative as well asRFC 1035 which gives the full details of the DNS protocol.
The Domain Name System, otherwise known as DNS, is critical to the functioning of the internet. At the heart of it, DNS operates like a phonebook for the Internet. When you type in a website URL such as ‘www.google.com’, your computer doesn’t inherently understand this. Instead, that domain name needs to be translated into an IP address that your device can understand and connect to.
How DNS works is through a process of translation and communication between multiple servers. This includes:
- The client making a request
- Your ISP’s recursive DNS servers
- TLD (Top-Level Domain) name servers
- Authoritative DNS servers
The client, typically your browser, reaches out to your ISP’s DNS server with a DNS query. The server then consults TLD servers, then subsequent authoritative DNS servers until it receives an IP address corresponding to the domain name. It then sends this response back to the client to complete the connection and load the requested webpage.
Within these operations, there lies inherent security risks which could compromise the confidentiality, integrity, or availability of the information passed across servers. Therefore, certain security measures have been developed and embedded within DNS operations.
One protocol embedded within DNS operations is the Transport Layer Security (TLS) for DNS. RFC7858 outlines DNS over TLS. This provides privacy for DNS operations by adding a layer of encryption to the data in transit. This makes it harder for third-parties to intercept the transmissions and see what websites users are trying to access.
Another protocol structured within DNS operations is the DNSSEC. DNSSEC, also known as DNS Security Extensions, counters cache poisoning attacks, one of the most common types of attacks targeting DNS. In a cache poisoning attack, a malicious party can redirect users from legitimate websites to fake ones, designed to steal personal information. DNSSEC uses digital signatures to verify the authenticity of data, providing another line of defence against these cyber attacks.
# Example Python code for a DNS query using dnspython, considering DNSSEC import dns.message import dns.query request = dns.message.make_query('www.example.com', dns.rdatatype.ANY) request.flags |= dns.flags.AD request.use_edns(ednsflags=dns.flags.DO) response = dns.query.udp(request, '8.8.8.8')
To sum it up, DNS operations rely on several protocols, including DNS and DNSSEC among others to maintain its security levels. These tools provide encryption, prevent spoofing, ensure data integrity, among other methods to combat a variety of threats today’s cyberspace possesses. Thus providing confidence in the internet’s fundamental ability to direct users to their intended destinations.
Here are some of the integral parts that make DNS work:
- A DNS resolver that queries the DNS servers
- A root server that guides to the correct TLD (Top Level Domain) server
- A TLD server that directs towards the authoritative nameserver that holds the DNS records
- An authoritative nameserver that gives out the DNS records for an asked domain
Types of DNS Records
- A Record: Also known as Address Record, it links a domain to an IP address. When your browser fires a query for a domain, the remote server will search for its A record in order to obtain the corresponding IP. For instance:
example.com IN A 192.0.2.4
- CNAME Record: Canonical Name Record lets you alias one name to another. Essentially, it’s like forwarding where you can point one domain to another. For example, you might want ‘www.example.com’ and ‘example.com’ to lead to the same place. Here’s how it’s expressed:
www.example.com IN CNAME example.com
- MX Record: Mail Exchange Record guides email delivery to the appropriate mail servers. For example:
example.com IN MX 10 mailx.example.com
example.com IN MX 20 mailz.example.com - TXT Record: Text Record allows you to input arbitrary text into a DNS record. This is often used for Sender Policy Framework (SPF) to help prevent email spam.
- SRV Record: Service Record aids expressing services provided by a domain as well as showing their location (i.e., hostname and port). Used widely with SIP configurations, XMPP Protocol, etc.
- SOA Record: State of Authority Record carries central information about a DNS zone, including the primary name server, the email of the domain administrator, the domain serial number, refresh and retry times, etc.
- AAAA Record: Similar to the ‘A Record’, this one represents IPv6 addresses.
hostname.com IN AAAA 123f:123f:123f:123f:123f:255 B:FFFF F:123 E
Each of these play critical roles in directing traffic across the internet and enabling the various functionalities we’ve come to rely on. Obtaining a robust understanding of DNS records and their functions will facilitate more efficient networking deployments and problem resolutions.
Want to learn more about DNS? Visit the official ICANN website.
DDoS attacks directly impact DNS operations in several distinct ways due to their direct action on the network infrastructure that supports DNS functionality. For a full understanding, let’s first consider how DNS works exactly.
DNS, or Domain Name System, is essentially the phonebook of the internet. It translates human-readable domain names (like www.example.com) into IP addresses computers can understand.
A simplified snapshot of the DNS process looks something like this:
– You enter a URL into your web browser.
– Your computer reaches out to a DNS server, asking for the IP address associated with that URL.
– If the server knows the address, it sends that info back to your computer, allowing it to make a connection and load the webpage.
– If DNS doesn’t know the IP address, it asks other servers until it finds one that does.
The code snippet below illustrates how such a request might be made programmatically.
import socket ip = socket.gethostbyname('www.example.com') print(ip)
So where do DDoS attacks come into play? DDoS, or Distributed Denial of Service, attacks flood a target with an overwhelming amount of traffic, in an attempt to overload its resources and render it unusable or unreachable. Various forms of DDoS attacks exist, but all have one common aim – to degrade or completely halt network services.
Here’s how these attacks influence a blogwebsite’s operation by hindering DNS processes:
– Overloading the DNS servers: Through amplification attacks, bad actors flood DNS servers with malicious requests, thereby preventing valid requests from being resolved. This directly interrupts the normal functioning of DNS operations.
– Cache poisoning: Attackers exploit vulnerabilities to inject false record entries into DNS caches. This essentially directs users to fraudulent websites even though they have typed in the correct URL. A consequence of this attack is subverting trust in DNS operations.
– Subdomain attack: Here, attackers flood DNS servers with non-existing subdomain requests, causing the server to waste resources looking for something that doesn’t exist and potentially slowing down responses to genuine requests.
These are some of the negative impacts a DDoS attack can have on DNS operations. While counter-measures exist, including rate limiting, IP-based blocking, anomaly detection, and more robust security architecture, it remains a formidable challenge to completely prevent DDoS attacks from affecting DNS operations.
Referenced materials for further reading:
[A Detailed Explanation of DNS](https://www.cloudflare.com/learning/dns/what-is-dns/)
[What Are DDoS Attacks and How Can They Be Prevented?](https://us.norton.com/internetsecurity-emerging-threats-what-is-ddos.html)
Remember, keeping systems up-to-date with the latest security patches, utilizing DNSSEC for DNS security, implementing intrusion detection systems, and maintaining constant vigilance are key strategies in combating DDoS-based disruptions to DNS functions.There are two critical parts to Internet technology that we rely on every day: Domain Name System (DNS) and Hypertext Transfer Protocol Secure (HTTPS). To understand how DNS over HTTPS (DoH) enhances privacy and security, it is essential first to understand how these systems function individually before seeing how they operate in synergy.
Domain Name System (DNS)
DNS is essentially the Internet’s phone book. It translates the human-readable web addresses we type into our browsers (like www.google.com) into numerical IP addresses that computers use for communication purposes.
Below is a crude representation of how DNS function works:
- You type in a URL into your browser
- Your computer sends a DNS query to locate the server which hosts the desired website
- The DNS resolver responds with an IP address
- Your computer connects to the server using the IP address
- The server sends back the data required to load the website on your browser
The protocol successfully allows smooth navigation of the web. However, DNS requests are almost always sent in clear text. This means bad actors can potentially intercept the DNS requests and responses, tracking your web activity or worse, redirecting you to malicious websites, a common cyber attack known as DNS spoofing or poisoning. This is where DoH comes in.
DNS over HTTPS (DoH)
To enhance the security and privacy of standard DNS queries, DNS over HTTPS (DoH) was introduced. DoH takes DNS requests, which were typically unencrypted, and encrypts them using HTTPS. HTTPS uses encryption protocols like TLS or SSL to secure the connection between your browser and the webserver.
So, how does DoH add an extra layer of security and privacy to your online activities?
- It prevents eavesdropping: Since all communications between your browser and the DNS server are encrypted, cybercriminals can’t spy on your online activities.
- It curbs manipulation: By using HTTPS, DNS responses become much harder to tamper with. As such, you’re less likely to be a victim of DNS spoofing or other similar attacks.
- Greater user privacy – ISPs can no longer access domain name resolution data, thus improving user privacy.
In terms of coding, if you are a Firefox user, the DoH feature can be enabled through the following steps:
- Type about:config in the address bar. If a warning page appears, click “Accept Risk and Continue”.
- In the search box, type network.trr.mode and hit Enter.
- A list of preference names will appear. Right-click on network.trr.mode and select “Modify”.
- Change its integer value based on what you want:
- 0: let Firefox decide which to use, insecure DNS or DoH. The default in Firefox is 0.
- 1: Use DoH after all methods are tried and failed.
- 2: Use DoH; fall back to insecure DNS under certain conditions
- 5: Strict mode. Only use DoH. Never use the insecure DNS.
In conclusion, while typical DNS requests carried out their tasks efficiently, they fell short on privacy and security fronts. DoH, by encrypting these requests and responses using HTTPS, significantly improves the privacy and security of our online experiences further cementing the importance of having a securely coded and configured connection.
For more information, please refer to the IETF’s documentation on DoH here.
`
network.trr.mode
` is a configuration preference within the Firefox browser that can be used to enable or disable DoH, or modify how the browser should deal with DoH according to Mozilla’s official documentation, which you can view here.The beauty of the internet, as we know it today, is predominantly attributed to the behind-the-scenes working of the Domain Name System (DNS). As complicated as the DNS may sound, its function rolls down to one main task – translating human-friendly URLs like www.google.com into computer-friendly IP addresses such as 172.217.14.206. Without it, accessing websites would be a far more challenging endeavor!
What exactly happens when you type a URL in your web browser?
- Type ‘http://www.website.com’ on the search bar.
- Your computer asks the local DNS server for the IP address of ‘www.website.com.’
- If the local DNS server knows the answer, it replies with the IP address. If it doesn’t, it sends the request to another DNS server.
- This process continues until the addressing DNS server is located and gives the requested IP address.
- The web browser uses this IP address to fetch the website content.
funtion dnsLookup(domain) { // ... var ip = lookUpInCache(domain); if (!ip) { ip = askMyDnsServer(domain); if (!ip) { ip = recursiveLookUp(domain); } } return ip; }
Throughout the decades, DNS operation has evolved and seen significant advancements spurred by technological innovations.
Firstly, DNS resolutions have become faster thanks to cutting-edge technologies like DNS over TLS (DoT) and DNS over HTTPS (DoH). Both protocols encrypt DNS traffic, increasing security and privacy. DoH even allows port 443 for communication, cleverly bypassing possible firewall restrictions because port 443 is typically used for encrypted HTTPS traffic.
Secondly, effectiveness of caching in DNS has vastly improved. Caching refers to temporarily storing recent DNS lookup results for faster resolution of subsequent requests. Modern software techniques, such as TTL (Time-to-Live) caching from Nginx, have made caching mechanisms more proficient.
It’s crucial to mention the advent of decentralized DNS systems, which are becoming increasingly popular. Blockchain-based DNS alternatives like Ethereum Name Service or Handshake are notable examples. These offer potential benefits including enhanced user privacy, censorship resistance, and reduced reliance on centralized authorities.
The emergence of AI and Machine Learning has impacted DNS by creating intelligent predictive analytics capabilities. Predictive caching represents an exciting area. Machine learning algorithms can predict upcoming domain query patterns based on historical data, fetching relevant content ahead of time which significantly speeds up browsing.
Table showing Predictive Caching Example :
Day | Domain Queries | Predicted Queries (Next Day) |
---|---|---|
Monday | Google, Github, Stackoverflow | Google, Github, Facebook |
Tuesday | Google, Github, Facebook | Facebook, Google, Twitter |
Wednesday | Facebook, Google, Twitter | Twitter, Linkedin, Google |
So, the DNS evolution journey has navigated through several milestones, responding to changing needs while embracing technological advancements for more robust, faster, and secure dynamics. A steadfast testament to the persistent creativity and innovation driving modern technology.
So, to wrap up our discussion on ‘How Does DNS Work Exactly’, it is clear that the DNS or Domain Name System acts as the internet’s phone book, transforming human-friendly website addresses into computer-friendly IP addresses. This transition occurs in a matter of milliseconds, whisking us away to our desired webpage with just a simple click. Here are key takeaways:
– The DNS process starts when we enter a URL into our browser.
DNS request -> Recursive DNS servers -> Root name servers
– The root server doesn’t know the IP – but it knows who will. It directs our query to TLD (Top-Level Domain) servers. Based on if .com, .org etc. it goes to the respective TLD.
Root name servers -> TLD servers -> respective domain based on (.com, .org, etc.)
– After accessing the relevant directory, it reaches the authoritative DNS servers that hold the IP ‘phone number’ for the specific site we want.
TLD servers -> Authoritative DNS servers -> Specific website IP
The process might seem complex, but it takes place within mere seconds, offering you a seamless browsing experience. Moreover, knowing how this intricate system works offers a fascinating glimpse into the digital mechanics working constantly behind the scenes of our daily web surfing activities.
To deep-dive into this topic, refer to DNS Guide for an easy-to-understand yet comprehensive understanding of the world of DNS.
For those interested in coding and programming around DNS structure, find complete code chunks at DNSTechCode. Always remember, the more efficiently you can write code to interact with DNS, the more effectively your applications will perform over the network.
Let’s illustrate this with a simple Python code snippet that uses the socket library to perform a DNS look-up.
import socket def get_host_name(IP): host_name = socket.gethostbyaddr(IP) return host_name
From quietly powering our day-to-day internet surfing to helping businesses establish a reliable online presence, the Domain Name System, or DNS, plays an invaluable role in shaping our modern digital world.