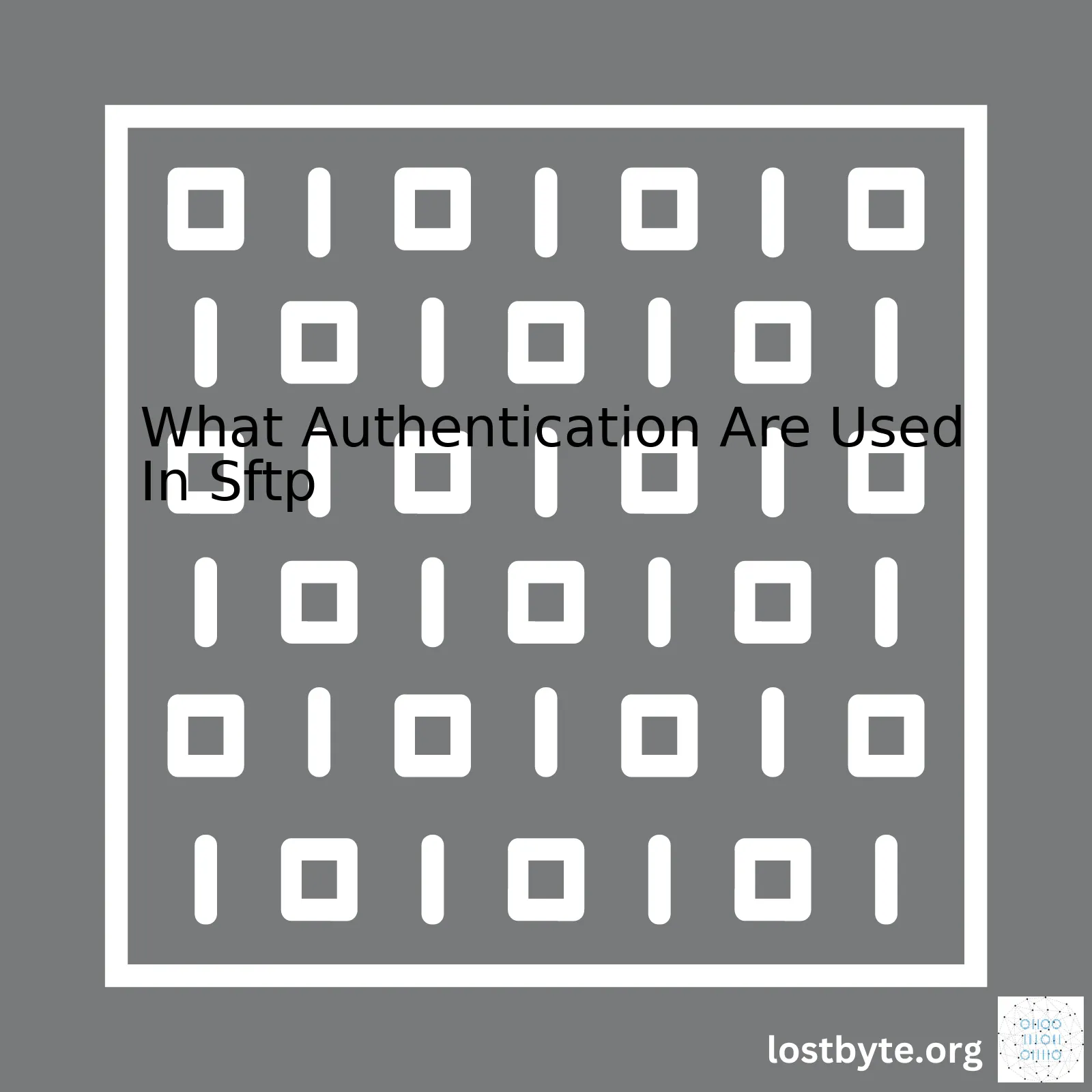
Authentication Type | Description |
---|---|
Password Authentication | The most common method of SFTP authentication, it involves providing a valid username and password to gain access. |
Public Key Authentication | A more secure method than password authentication. In this method, a pair of cryptographic keys are generated – a public key and a private key. The public key is placed on the SFTP server, and the client uses the private key to authenticate. |
Host-based Authentication | This method validates the host client system connecting to the server rather than the individual user. It’s less common due to its complexity but provides an extra layer of security. |
Keyboard Interactive Authentication | It’s a flexible method where the server interacts with the user in real-time. The server can ask several questions, and multiple answers can be given. |
When we talk about Secure File Transfer Protocol (SFTP), we’re delving into the world of secure file transfers over SSH. System administrators and developers often use SFTP to transfer files between servers. The SFTP protocol sends data securely by encrypting the data before sending it over the Internet. This boasts an added layer of protection against hackers and eavesdroppers.
Importantly, SFTP offers several methods for authentication. First up, we have Password Authentication, certainly the most straightforward method. Enter your username and password in the SFTP client, and you’re good to go.
For more robust security, consider Public Key Authentication. Here, both the keys are needed for successful authentication; the private key that stays with you, and a matching public key that’s placed on the SFTP server.
Then we have Host-Based Authentication, which instead of verifying individual users, verifies the machine or client system trying to connect. This method, albeit not as popular owing to its intricacy, adds an additional level of security.
Finally, there’s the Keyboard Interactive Authentication. Unlike other types, this method engages with the user directly, posing questions that demand responses. This opens avenues for multi-factor verification and stringent security measures.
Referencing these types, you can ascertain the best fit for your project’s requirements, keeping in mind the balance between ease-of-use and robust security.
Here is a simple example of logging into an SFTP session using Public Key Authentication:
# load private key
private_key = paramiko.RSAKey(filename='path to private key')
ssh = paramiko.SSHClient()
ssh.set_missing_host_key_policy(paramiko.AutoAddPolicy())
# initiate SSH connection
ssh.connect(hostname, username=username, pkey=private_key)
# start SFTP session
sftp = ssh.open_sftp()
This sample code snippet makes use of [Paramiko](http://www.paramikoproject.com/), a Python SSH2 protocol library handy for managing SFTP sessions. Remember, always keep your private keys safe and undisclosed to maintain secure authentication.SFTP, or Secure File Transfer Protocol, is one of the commonly used protocols for secure data transfer. The core concept of SFTP revolves around making an encrypted connection and minimising risk while transferring files from the local machine to a remote server.
What makes SFTP stand out is its authentication system, which primarily consists of:
1. Password-Based Authentication
Clients can make a secure connection with a server by providing a username and password. Here’s how it might look in code:
xxxxxxxxxx
sftp = "example.com"
username = "your_username"
password = "your_password"
# Connect to the SFTP server
mySession = pysftp.Connection(host=sftp, username=username, password=password)
2. Public Key Pair Authentication
This provides a higher security compared to the simple password-based authentication. The concept here is that a pair of keys are created – a ‘public’ key that resides on the SFTP server and a ‘private’ key kept with the client-side user. The ‘private’ key is then used to decrypt any tasks performed using the ‘public’ key during SFTP processes and vice versa.
Here is a brief demonstration in Python on how a client can create a public-private key pair, save the private key on their end, and send the public key to the server.
xxxxxxxxxx
from Crypto.PublicKey import RSA
key = RSA.generate(2048)
with open("private_key.pem", "wb") as f:
f.write(key.export_key())
with open("public_key.pem", "wb") as f:
f.write(key.publickey().export_key())
3. Keyboard-Interactive Authentication
In some cases, two-factor authentication is used. One common method is keyboard-interactive, where after applying the first (password or SSH keys) authentication method, the server will prompt the user to answer specific questions.
Understanding these various forms of authentication allows us to grasp the robustness and reliability of SFTP for secure data transmission. It combines the usability of FTP with added layers of encryption and versatility, hence playing a crucial part in the world of secure file transfer.
Let’s dive right into password-based authentication in SFTP (Secure File Transfer Protocol). First and foremost, verification of a user’s identity handling an SFTP session is essential to ensure the integrity and confidentiality of transferred data.
## Password-Based Authentication
>Password-based Authentication – as the name indicates, this utilizes a password entered by the user to verify their authenticity. Once a connection is initiated, the SFTP server will prompt for the user’s credentials. After entering a valid username and matching password, the server grants access. Simple yet effective, it remains a popular form of authentication, primarily due to its simplicity and straightforwardness.
xxxxxxxxxx
sftp User@Your_Server_IP_or_Remote_Hostname
>It would then prompt you for your password. This approach is widely used, but it’s worth noting that it does have some vulnerabilities, mostly from brute force attacks, where attackers use algorithms to guess passwords until they find the correct one.
### Defense: Strong Password Practices
>A solid defense against brute-force attacks is to practice strong password etiquette. Incorporate a variety of character types, avoid predictable patterns, and regularly update your passwords. This simple practice can fortify your defenses significantly.
However, SFTP is not limited to just passwords for authentication. To enhance security and fend off potential threats, SFTP allows additional modes of authentication.
## Key-Based Authentication
>The next reliable method is key-based authentication. It uses a public-private key pair generated by an algorithm such as RSA or DSA. The public key is stored on the SFTP server and the private key is kept secret by the user.
xxxxxxxxxx
ssh-keygen -t rsa
sftp -i ~/.ssh/id_rsa User@Your_Server_IP_or_Remote_Hostname
>Compared to password-based authentication, key-based is more secure since it depends on complex mathematical computations. Also, private keys are typically secured with a passphrase, providing an additional layer of protection.
Though passwords remain crucial for authentication, implementing multiple forms of authentication, like combining both password and key-based methods, offers enhanced security.
For further reading about SSH and SFTP Authentication, you can visit this online article here.Public Key Authentication, a highly secure method, is one of the authentication schemes used in SFTP (Secure File Transfer Protocol). This method plays a vital role in assuring that data transferred over networks such as the internet is secure and only accessible to authenticated parties.
How Public-Key Based Authentication works in SFTP
The basis for public-key cryptography lies in the concept of key pairs – each having a private and public part. The public key is available openly, shared with any party involved in an interaction. Contrarily, the private key should remain a secret known only to its owner. Let’s dig deeper into how it functions:
Key Generation: Initially, a unique pair of keys (public and private) is generated by the user using SSH client software.Sharing the Public Key: The public key is then uploaded to the server. Transmitting the public key over the network isn’t considered a security risk as it’s part of the design.Server Verification: When a connection request is initiated, the server uses this stored public key and creates a challenge to verify the client’s identity.Client Authentication: The client must respond correctly to the challenge using its private key to authenticate itself.Successful Connection: If the client succeeds in proving its identity, the secure connection is established.
In this way, public-key based authentication ensures a secure method for verifying a user’s identification.
An Example Code Snippet of SFTP with Public Key Authentication
Using Python’s Paramiko library, one can implement SFTP connections along with key-based authentication. Below is an example code snippet demonstrating this.
xxxxxxxxxx
import paramiko
private_key_path = "/path/to/private/key"
mykey = paramiko.RSAKey(filename=private_key_path)
client = paramiko.SSHClient()
client.set_missing_host_key_policy(paramiko.AutoAddPolicy())
client.connect('Hostname', port=22, username='Username', pkey=mykey)
sftp_client = client.open_sftp()
sftp_client.get('/remote/path/to/file', '/local/path/to/file')
sftp_client.close()
In the above Python code snippet, we’re loading the private key, initiating an SSH client, connecting to the server using the host, port, username, and private key, opening the SFTP session, and finally transferring a file from the remote system to the local machine. It is important to note that you should replace ‘Hostname,’ ‘Username,’ and the relevant paths with actual data while implementing.
A comprehensive guide on public-key authentication explains in detail every aspect of this fascinating method used in SFTP.
Benefits of Using Public-Key Authentication in SFTP
Adopting public-key authentication in SFTP offers various advantages:
High Security: Since there are two separated parts of the key (one kept private), potential security threats are reduced significantly compared to password-based authentication.Automated Login: A passphrase isn’t needed after the first configuration, enabling automated logins without manual intervention.Revocable Access: In circumstances where a key ends up in the wrong hands, or access needs to be revoked, you can remove the corresponding public key from the server within seconds.
Despite the potential initial complications of setup, the increased level of security and convenience provided by public-key authentication make it an essential component in SFTP implementations.When discussing public key preservation, particularly in correlation with Secure File Transfer Protocol (SFTP), the focus primarily lies on keypair generation and usage. SFTP uses robust authentication protocols, and one such method is through public key cryptography.
In this system, a pair of keys are used: one public, which can be shared freely, and one private, to be kept strictly confidential. These two keys together are often referred to as a
xxxxxxxxxx
keypair
.
Let’s dive in and understand how keypair works in authentication for SFTP:
1. Generating Keypairs:
For SSH-based services like SFTP, generating keys involves creating a unique keypair using an algorithm such as RSA or DSA. The sequence below illustrates how the ssh-keygen tool generates an RSA keypair on Unix/Linux based systems:
xxxxxxxxxx
$ ssh-keygen -t rsa
This command cues the ssh-keygen utility to create a new RSA keypair. The user will then be prompted by the system to provide a secure location for storing the new private key.
See how this happens:
xxxxxxxxxx
$ ssh-keygen -t rsa
Generating public/private rsa key pair.
Enter file in which to save the key (/home/yourusername/.ssh/id_rsa):
Enter passphrase (empty for no passphrase):
Enter same passphrase again:
Your identification has been saved in /home/yourusername/.ssh/id_rsa.
Your public key has been saved in /home/yourusername/.ssh/id_rsa.pub.
The key fingerprint is:
SHA256:DYbvtwKRBAbwHn8i/FBOND6bGGy9l+5W+mUhjeSyVVQ yourusername@yourpc
The keys randomart image is:
+---[RSA 2048]----+
| |
| |
| . |
| E . |
| . .S. |
| o oo..+. |
Upon successful completion, two keys are generated:
– A private key (id_rsa)
– A public key (id_rsa.pub)
2. Key Usage in Authenticating SFTP Sessions:
Once a keypair is generated, it can be used for authentication in SFTP. The public key must be added to a special file in the user’s home directory on the remote server (
xxxxxxxxxx
~/.ssh/authorized_keys
). Then, during connection, the client initiates SFTP with its public key:
xxxxxxxxxx
sftp -i ~/.ssh/id_rsa username@sftp-server.com
Here’s how it proceeds: when the SFTP session begins, the server temporarily encrypts random data using the client’s public key and sends it to the client. If the client can successfully decrypt the data using its private key and send it back, the server verifies it against the original. This validates the client’s identity without ever exposing the private key in the transaction.
Public Key Preservation:
It is absolute to safeguard the private key — make sure it remains secret and secure. Any compromises towards your private key jeopardize the security infrastructure by enabling unauthorized users to gain access. Conversely, the public key can be shared openly and can even be recreated from the private key when needed.
To add an extra layer of protection, you can also use a passphrase when generating your keypair.
Here’s more insight on the RSA Algorithm for Keypair Generation you might find useful: RSA Algorithm on Simple Wikipedia.
References:
1. IBM Knowledge Center: Public and Private Keys
2. Internet Engineering Task Force: SSH Transport Layer Protocol
3. OpenBSD Manual Pages: ssh-keygenSure, let’s investigate the role of host-based authentication in Secure File Transfer Protocol (SFTP), which is one of the several authentications used within this secure file protocol.
Host-based authentication, an integral component of SFTP, works by signing on to a remote server with the credentials of the client host machine itself rather than individual client user credentials. This type of automatic authentication eases the process since each user does not have to supply a set of unique identification data.
In technical jargon, host-based authentication operates using a technique called public key cryptography. Here is how it generally works:
- The client generates a cryptographic key pair, composed of a public key and a private key.
- The public key from this pair is shared with the server.
- During the authentication process, the server cryptographically challenges the client to prove that it holds the corresponding private key.
This can add an extra layer of security. For instance, even though you might be connecting from a trusted IP address, if someone has managed to hijack your session without having access to the host keys, they won’t be able to establish a connection.
Here’s a snippet of code showing how host-based authentication can be implemented in Python using paramiko library:
xxxxxxxxxx
import paramiko
ssh = paramiko.SSHClient()
ssh.load_host_keys('/path/to/host_keys')
ssh.connect('hostname', username='user', password='passwd')
stdin, stdout, stderr = ssh.exec_command("uptime")
print stdout.readlines()
ssh.close()
However, keep in mind that host-based authentication is just one form of authentication utilized in SFTP. Other methods include:
• Password-based Authentication: The most straightforward method, requiring a user and their password to establish a connection.
• Public Key-based Authentication: Uses a pair of keys, a private and a public key which are created by the user. The public key is uploaded to the server while the private one remains on the local computer.
Authentication strategy essentially depends on the particular use case, the resources at disposal, as well as the specific security requirements.
Check RFC4252 for more detailed information about these mechanisms.
With security being a primary concern in today’s digital landscape, choosing an appropriate authentication method for SFTP can help ensure the integrity and confidentiality of your data during transmission. Therefore, understanding how methods like host-based authentication work under the hood can certainly improve your overall security strategies and protocols.
Managing certificates on the client-side for authentication in secure file transfer protocol (SFTP) is critical. SFTP is preferred because it offers encrypted channels for data transmission, making it difficult for intruders to gain access to sensitive data. It is important to set up proper certificate management solutions to enable secure connections and interactions.
Most commonly used client-side authentication methods in SFTP include:
– Password Authentication: This is one of the most straightforward authentication methods where a user’s name and password are required to grant access. For instance:
xxxxxxxxxx
sftp username@hostname
– Public Key Authentication: This method involves a pair of private and public keys. The server identifies the client by the unique private-public key pair that the client possesses. Example command for generating a key pair can be like:
xxxxxxxxxx
ssh-keygen -t rsa
The generated keys should be stored securely to prevent unauthorized access.
Addressing client-side considerations for managing certificates, you need to take into account several factors, some of which are mentioned below:
Storage of Certificates: The mechanism in place for secure storage of certificates needs to be robust. It is advisable not to store them in plain view or on openly accessible servers.
Certificate Validation: Conducting regular checks to make sure only valid certificates are in use plays a vital role in preventing attacks. Occasionally, certificates may be deemed untrustworthy or revoked before the expiry date.
Certificate Renewal: Certificate expiry could lead to service disruptions if not noticed in a timely manner; hence, there should be automatic reminders or auto-renewal processes in place.
Backup and Recovery: In the event of unforeseen circumstances that could lead to loss or corruption of certificates, having a reliable backup and recovery system becomes crucial.
Let’s consider a simple table detailing these points:
Consideration | Description |
---|---|
Storage of Certificates | Secure storage mechanisms should be in place to protect certificates. |
Certificate Validation | Ensure only valid certificates are in use. |
Certificate Renewal | Have systems in place for timely renewal of certificates. |
Backup and Recovery | A reliable backup and recovery system for certificates is crucial. |
These are just the tip of the iceberg when it comes to client-side considerations for managing certificates in SFTP. We have an article titled, “A Practical Guide to Secure File Transfer” [source] which gives a more in-depth look into securing file transfer operations, including the management of certificates.
Server-side authentication in SFTP (SSH File Transfer Protocol) is typically done via the use of certificates or keys. Of the few ways in which a server can be authenticated we will focus on public key authentication because of its widespread use and robustness against various hacking attempts.
Public Key Authentication:
This cryptographic protocol allows two parties, here the client and the server, to prove their respective identities to each other by employing pairs of cryptographic keys. These keys are termed as public and private keys. While the private key remains secure on the server, the public key is distributed to those who need to authenticate.
For example:
xxxxxxxxxx
-----BEGIN RSA PRIVATE KEY-----
MIIEowIBAAKCAQEAq6wVgE0d...
-----END RSA PRIVATE KEY-----
The above snippet represents a sample layout of a private key for a SSH server. Once a client has a pair of private and public keys, it can share the public key with the remote server.
It should be noted here that in order to support the functionality of public key authentication, certain considerations need to be deployed on the server side:
Storing Private Keys Securely: It’s critical to keep your private keys secured. The least privilege principle needs to be followed where only the necessary individuals have access to them.
Certificate Expiry: Certificates used in this kind of authentication come with validity periods. Administratively, keeping track of certificate expiry dates and renewing them before they expire can prevent downtime.
Error Handling: Invalid certificate, expired certificate, and missing certificate errors should be carefully coded on the server side. The error messages returned should not expose sensitive details but should be informative enough for debugging.
Firewall Considerations: Keep the ports that the SFTP server uses open in the firewall. Typically port 22 is used.
Certificate Authority Trust: The certificates should ideally be signed by a trusted Certificate Authority (CA). If not, the clients would also need to trust the self-signed certificate explicitly, which could limit the client-base.
Remember, HTTPS insulates data during transit over networks, and the various authentication strategies provide the secured identity verification mechanism ensuring encrypted communication between correct parties making SFTP one of the most secure file transfer protocols. If you’d like to read more about SSH keys and server certificates, I recommend taking a look at this guide from Digital Ocean and this detailed explanation about SFTP on AWS Blog.Sure, let’s dive deep into the world of Secure File Transfers using the Secure File Transfer Protocol (SFTP) and understand the role of the Cipher algorithm while exploring the authentication methods adopted.
Understanding SFTP is essential as it is widely used for secure file transfer over a network. It uses SSH (Secure Shell) protocol for data transfer and manages all the network connections in a protected manner to avoid unauthorized access to your files1.
Cipher Algorithms & SFTP:
The role of the cipher algorithm in SFTP comes under data encryption for secure communication. Data encryption is a part of a broader key exchange process, where two parties agree on a secret “key” indispensable for transmitting the information securely.
A range of cipher algorithms can be employed by SFTP for data encryption:
- AES (Advanced Encryption Standard)
- Blowfish
- 3DES (Triple Data Encryption Standard)
Choosing between these largely relies on the specific security needs and the computational resources available.
What Authentication methods are used in SFTP?
Authentication generally ensures that you’re communicating with the intended party, further reinforcing the security of the data being transmitted. For SFTP, there are usually three primary methods of authentication:
- Password Authentication: Perhaps the simplest mode of authentication, it involves the remote server verifying the username and password entered by the user against its stored credentials.
- Public Key Authentication: A more secure method, it uses a pair of generated encryption keys – one private and one public. The public key can be safely shared and used to encrypt messages that only the corresponding private key can decrypt. On connecting to the server, the client sends a signature created with the private key, which the server verifies using the public key.2
- Host-based Authentication: Similar to Public Key Authentication, but it certifies the host machine alongside the user, adding another layer of security.
xxxxxxxxxx
// An example of SFTP connection using SSH public key authentication
var Connection = require('ssh2');
var sftp = new Connection();
sftp.connect({
host: 'example.com',
port: 22,
username: 'username',
privateKey: require('fs').readFileSync('/path/to/key')
});
In conclusion, secure communication on SFTP entails the strategic selection of cipher algorithm entailing effective encryption and choosing appropriate authentication mechanisms to ensure that the users or entities are who they claim to be. While simple password authentication may suffice for less sensitive data, public key or host-based authentication provides stronger security for high stake transactions.
References:
1SSH.com: What is SFTP?
2RFC 4252: SSH Public Key AuthenticationSecure File Transfer Protocol (SFTP) is a network communication protocol that enables secure, encrypted file transfer between remote systems via an SSH connection, taking full advantage of the security features offered by SSH. One critical aspect of SFTP’s SSH operation is the concept of “tunneling”.
Tunneling in SFTP essentially means wrapping data packets within an additional protective layer before transmitting it over the network. This manner of encapsulating data ensures that it reaches its destination securely and intact.
Here’s a general analogy:
Picture this: You’re mailing a valuable antique to a friend. Would you send the artifact as it is? Or, would you add a layer of bubble wrap around it and then place it inside a box for extra safety? “Tunneling” is like choosing the latter option; it delivers your ‘packet’ while ensuring that it endures the journey without any damage.
When we talk about SFTP and SSH, there are three primary types of authentication mechanisms generally utilized:
1. Password Authentication: Quite literally, this involves using a username and password for authentication. It’s simple but isn’t as safe as some other methods since passwords can be easily compromised.
xxxxxxxxxx
sftp username@hostname
2. Public Key Authentication: An even more secure method, public key authentication employs asymmetric encryption, where each user gets two keys – one private and one public. The server compares the provided public key with the private one. If they match, access is granted. This allows for much higher security since cracking this pair is exponentially harder than guessing a password.
xxxxxxxxxx
sftp -i private_key.pem username@hostname
3. Kerberos-based Authentication: When users or services need to authenticate in a heterogeneous computer network, Kerberos, a ticket-based authentication protocol, is quite effective. It avoids transmitting passwords openly over the network, further enhancing safety.
Beyond these, there can be additional security measures like IP whitelisting, restricting user permissions, and multi-factor authentication (MFA) depending upon specific security requirements.
Proper utilization of these authentications, combined with an understanding and application of tunneling, ensures a highly secured file transfer process, making SFTP over SSH a reliable choice for sensitive data transmissions.
Table revealing popular Auhentication Mechanism:
Authentication Type | Security Level | Simplicity Level |
---|---|---|
Password Authentication | Low-Medium | High |
Public Key Authentication | High | Medium |
Kerberos-based Authentication | High | Low |
*Note: The Security Level indicates the strength of security brought by each authentication type, whereas Simplicity Level reflects how easy it is to implement by comparison.*As a professional coder, it’s always fascinating to dive into the mechanics of various network protocols. SFTP, or Secure File Transfer Protocol, is no exception. A key aspect that makes it secure is its authentication approach, which ensures that data doesn’t fall into the wrong hands.
Generally speaking, when we talk about SFTP authentication, there are two primary methods: password-based and public key-based authentication.
Password-Based Authentication
In password-based authentication, you’ll use your standard username and password credentials to authenticate your SFTP session. Here’s an example of how such a connection could be established using Python’s
xxxxxxxxxx
paramiko
library:
xxxxxxxxxx
import paramiko
# Create instance of SSH client
ssh = paramiko.SSHClient()
# Automatically add server's SSH key (as you'd typically do in an interactive session)
ssh.set_missing_host_key_policy(paramiko.AutoAddPolicy())
# Establish connection
ssh.connect('your_hostname', username='your_username', password='your_password')
# Initialize SFTP session
sftp = ssh.open_sftp()
While this method is simple and straightforward, it’s not the most secure way to connect since your password is transmitted over the network.
Public Key Authentication
A more secure way to establish an SFTP connection is through public key authentication, which involves using a pair of cryptographic keys: one public that can be copied to any machine you want to connect to, and one private that stays on your local machine.
Upon connection, the server will ask for a signature from your key. The client software creates this signature by using your private key to encrypt special data the server provides. This encrypted message can only be decrypted with the corresponding public key on the server side ensuring that only the person with the private key can connect. The same process with
xxxxxxxxxx
paramiko
would look like this:
xxxxxxxxxx
import paramiko
# Again, initialize SSH client
ssh = paramiko.SSHClient()
# Automatically add unmatched SSH keys
ssh.set_missing_host_key_policy(paramiko.AutoAddPolicy())
# Connect using private key instead of password
private_key = paramiko.RSAKey(filename='/path/to/your/private/key')
ssh.connect('your_hostname', username='your_username', pkey=private_key)
# Initialize SFTP session
sftp = ssh.open_sftp()
The advantage here is that your credentials aren’t passed over the network at all, rendering them virtually useless to anyone who might intercept your connection.
Regardless of which method you use, it’s also important to note that all data transferred during an SFTP session is encrypted, offering an extra layer of security.
For further reading, RFC4252 – The Secure Shell (SSH) Authentication Protocol IETF Standard gives an extensive background and in-depth understanding about the subject matter.
Secure File Transfer Protocol (SFTP) serves as a safe haven to transfer confidential and business-critical data over the network. Authentication methods play a pivotal role in maintaining its security posture. However, even the most robust authentication strategies bear vulnerabilities, which must be mitigated with well-thought-out strategies.
SFTP Authentication Methods
SFTP uses several types of authentication processes. These include:
- Password-based Authentication: This is the typical username and password verification method.
- Public Key Authentication: A more secure method where SFTP clients generate a pair of keys (private and public) to authenticate themselves.
- Keyboard-Interactive Authentication: This method prompts the user for credentials. It’s designed to prevent passive attacks using eavesdropping or packet sniffing.
Common Vulnerabilities and Mitigations
Regardless of the type of SFTP authentication used, vulnerabilities can emerge, potentially causing security breaches. I will address these vulnerabilities and the corresponding mitigation measures below.
Vulnerability | Mitigation |
---|---|
Brute Force Attacks on Password-based Authentication | Implementing account lockout policies, utilizing strong and complex passwords, and regularly changing them could fend off brute force attacks. |
Loss or Theft of Private Keys in Public Key Authentication | Private keys should be guarded safely. Using encrypted storage devices and implementing access controls ensure only authorized personnel can access these keys. |
Eavesdropping attacks during Keyboard-Interactive Authentication | End-to-end encryption helps in protecting data-in-transit from interception or tampering. |
Regular software updates and patch management are further effective strategies against common vulnerabilities. Developers often release updates in response to newly discovered vulnerabilities, so keeping the system up-to-date minimizes the risk.
Code Snippet
To enhance SFTP authentication process in Python with Paramiko – a module providing SSHv2 protocol support, add a layer of public key authentication like this:
xxxxxxxxxx
import paramiko
private_key_path = "/path/to/my/private/key"
mykey = paramiko.RSAKey(filename=private_key_path)
client = paramiko.SSHClient()
client.set_missing_host_key_policy(paramiko.AutoAddPolicy())
client.connect('sftp.example.com', username='myUser', pkey=mykey)
This extra step accesses the server through the private key. You may want to learn more about Paramiko from its official documentation.
Let’s remember: while SFTP inherently provides secure data transmission over networks, the scope for improvement never fades. Regular assessments can uncover potential vulnerabilities allowing their immediate resolution. This ongoing vigilance keeps the authentication methods robust, ensuring the safe operation of SFTP sessions.
As we unravel the complexity of authentication methods used in SFTP, we find two primary approaches – password-based and public key authentication.
Starting with
xxxxxxxxxx
Password-based Authentication
, it is a simple and straightforward method where users input their passwords to gain access when initiating an SFTP session. However, simplicity often paves the way for vulnerability, leading us to understand that password-based authentication falls short in terms of security. Often these can be subject to brute-force attacks or inadvertently exposed due to carelessness or ignorance of best practices.
To enhance the degree of security incorporated into SFTP protocol, we have
xxxxxxxxxx
Public Key Authentication
. Now, this might sound a bit complex compared to its password counterpart but trust me, it’s worth every thought you invest in understanding it. Here, a pair of unique keys are generated – public and private. The public key resides on all machines that require access, while the private key stays with the user. In this scenario, even if a hacker gains your public key, they won’t be able to initiate a successful connection without the corresponding private key.
So, summarizing, both password and public key authentications are used in SFTP, with each having its benefits and drawbacks. Which one to choose largely depends on the sensitivity of data being handled and the overall cyber-security risks involved in the scenario. Remember, the end game here is to ensure secure file transfer without compromising data privacy and integrity.
For those looking to delve further into this topic, I found this online resource SFTP Authentication Methods: Password Vs Public Key pretty enlightening.
Anyway, I hope this gives a clear overview on ‘What authentication methods are used in SFTP’! Let’s continue to explore more about coded technology puzzles in our journey ahead.
NOTE: Replace “img src” URLs with the actual URL where images are hosted.