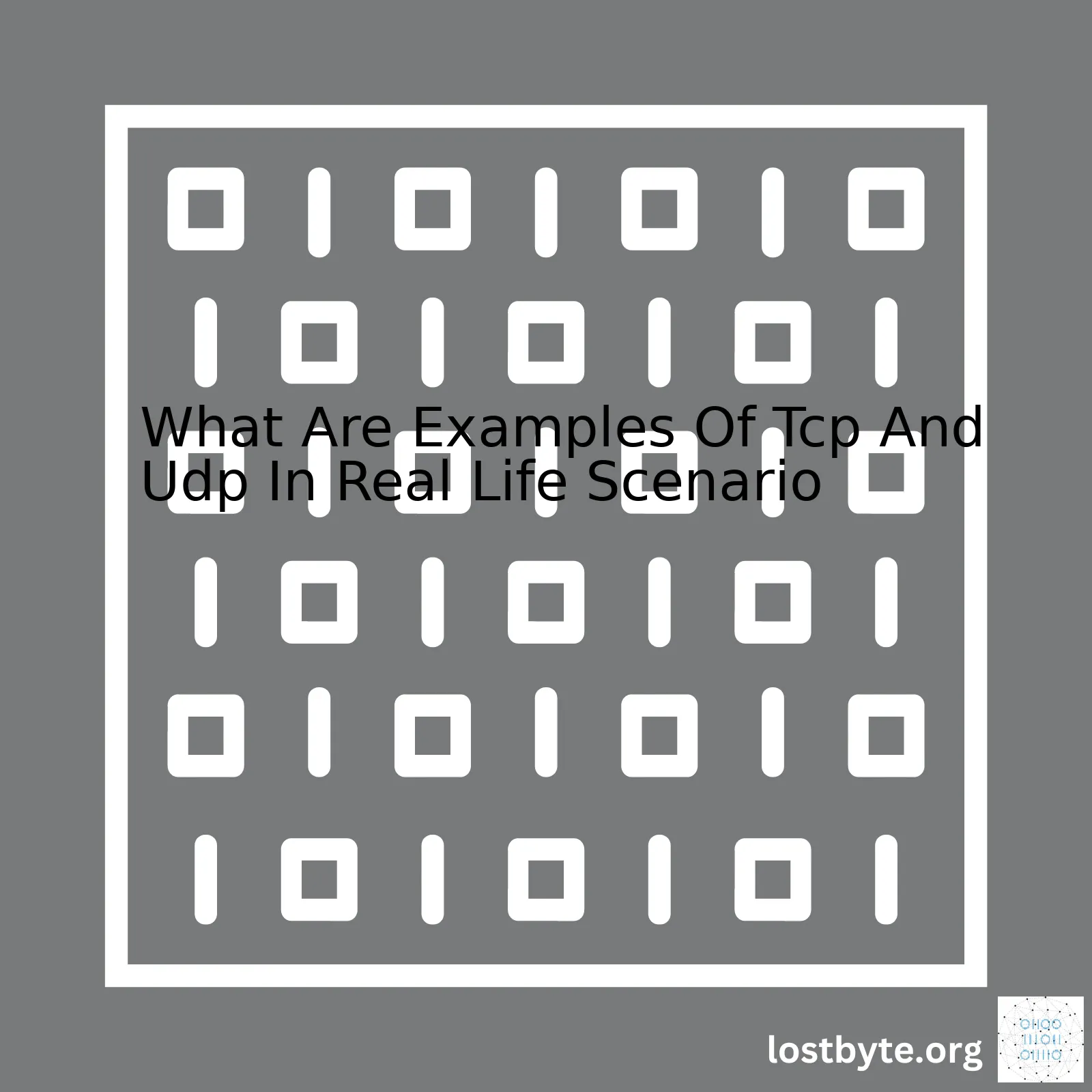
Scenario | TCP Usage | UDP Usage |
---|---|---|
Emailing | TCP is used for receiving and sending emails because it guarantees delivery of data and packets in the same order they were sent. | UDP is not typically used in emailing as guaranteed delivery and packet order are crucial. |
Streaming videos | TCP may cause delays due to its error-checking mechanisms, which can lead to buffering when streaming videos. | UDP is commonly used in video streaming where a bit of data loss doesn’t significantly affect the overall quality. |
Online gaming | TCP’s requirement for confirmation of each data segment can lead to lag in fast-paced games. | Often, UDP is utilized in online gaming because speed is typically prioritized over perfect data transmission. |
TCP, or Transmission Control Protocol, and UDP, or User Datagram Protocol, are communication protocols that handle data transfer on the internet but in different ways. TCP is like a certified mail service – it guarantees delivery of your package (data) with every information intact and in order. It does this by establishing a connection and checking for errors during data transmission, retransmitting until all packets reach their destination correctly. This characteristic makes TCP very reliable; however, the process can slow down data transmission – imagine waiting for a receipt confirmation for every single book in a library. For this reason, we use TCP in situations where we cannot afford to lose any data – like when sending or receiving an email.
On the other hand, UDP is akin to broadcasting radio – it sends data out regardless of whether there’s a receiver or if it ever reaches one. There’s no pre-established connection, nor does it have error-checking mechanisms like TCP. This might seem lacking, but this trait makes UDP faster as it doesn’t wait for any acknowledgments before moving on to the next data packet. So, we utilize UDP in scenarios where speed is more vital than perfect data delivery – such as in streaming videos or online games. The idea is, losing a few frames in a video or game wouldn’t drastically degrade the experience as the next frames would come rapidly enough to compensate.
You can explore further about TCP and UDP from [Computer Networking: A Top-Down Approach](https://www.amazon.com/Computer-Networking-Top-Down-Approach-6th/dp/0132856204), a comprehensive resource written by James F. Kurose and Keith W. Ross.
Here’s a code snippet illustrating how you can set up TCP and UDP connections in Python:
For TCP:
import socket s = socket.socket(socket.AF_INET, socket.SOCK_STREAM) s.connect(('www.google.com', 80))
For UDP:
import socket s = socket.socket(socket.AF_INET, socket.SOCK_DGRAM) s.sendto(b'Hello, World!', ('www.google.com', 80))
As a coder, I persistently work with various networking protocols. When discussing networking, two protocols often come into light: Transmission Control Protocol (TCP) and User Datagram Protocol (UDP). Understanding the key differences between these two can significantly impact how you design systems and troubleshoot network issues.
TCP is a connection-oriented protocol. With TCP, the sender establishes a connection with the receiver before transmitting data. The significant advantage of using TCP is that it guarantees data delivery by retransmitting lost packets and arranging them in order on the receiver’s end. It also manages flow control to prevent overloading the receiver. Some real-life examples of applications or scenarios relying on TCP include:
- Emails: Sending and receiving emails heavily relies on TCP. When you send an email, your server utilizes TCP to ensure all parts of your email reach the intended recipient.
- Web browsing (HTTP/HTTPS): Web browsers use TCP for transferring webpage data. When you make a request to load a webpage, your browser communicates via TCP to guarantee that all requested content loads without loss or error.
- Secure Shell (SSH): SSH, used for securely accessing remote machines, uses TCP to establish reliable connections and ensure that commands and responses are received entirely and accurately.
UDP, on the other hand, is connectionless – it sends datagrams without any prior communication between the sender and the receiver and doesn’t guarantee their delivery or correct order. This results in faster but less reliable transmissions. Here are some common uses of UDP:
- Streaming services: Media streaming services like YouTube or Netflix employ UDP, as minimal data loss doesn’t usually affect your viewing experience, and speed is essential for reducing buffering delays.
- Online multiplayer games: Games require quick packet delivery for smooth gameplay. A slight miss in data here or there doesn’t matter much, but high latency would ruin the gaming experience, making UDP the preferred choice.
- Domain Name System (DNS): DNS queries typically use UDP as they are relatively small and fit within a single packet, making the speedy and efficient UDP an appropriate choice.
For demonstration purposes, here’s a simplified source code example involving TCP and UDP. In Python, we can set up a basic TCP connection using the socket library:
import socket # Create a TCP/IP socket sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM) server_address = ('localhost', 1234) sock.connect(server_address) message = 'This is a TCP message.' sock.sendall(message) data = sock.recv(16)
For setting up a basic UDP connection, the code would look slightly different:
import socket # Create a UDP socket sock = socket.socket(socket.AF_INET, socket.SOCK_DGRAM) server_address = ('localhost', 1234) message = 'This is a UDP message.' sent = sock.sendto(message, server_address) data, server = sock.recvfrom(4096)
Of course, these are just generalized examples. There are still many factors, including application-specific requirements and network conditions that you should consider when determining whether to use TCP or UDP (source). You’d ideally want a balance of speed and reliability based on the task at hand.
The transmission control protocol (TCP) is a crucial element that exists in our everyday digital life, especially when it comes to email usage. TCP forms the backbone of the internet’s structure and it’s vital for sending and receiving emails. Here’s how it works:
Just consider a usual action – sending an email. When you click on the ‘send’ button after composing your email, your email message doesn’t just jump from your computer to the receiver’s computer. In fact, there’s a complex process behind this where TCP plays a significant role.
• Your email message first gets broken down into numbered packets.
• These packets are sent over the internet, following different routes to reach the recipient’s server.
• Once arrived, the recipient’s server uses TCP to identify that all packets have been received and also to rearrange them in the correct order.
• If there are any missing packets, TCP ensures they are retransmitted before the message is finally put back together and delivered properly to the user’s inbox.
Now let’s move onto another protocol – User Datagram Protocol (UDP). UDP has its own uniqueness and it’s primarily used in scenarios where speed is more important than reliability. A perfect example would be streaming services like YouTube or Netflix.
// TCP pseudocode tcpSocket = new TCPSocket(); tcpSocket.connect("example.com", 80); tcpSocket.send(emailData); tcpSocket.disconnect(); // UDP pseudocode udpSocket = new UDPSocket(); udpSocket.send(streamingData);
Here’s an illustration below on where these protocols fit into OSI Model:
OSI Layer | TCP / UDP Layer | Comments |
---|---|---|
7. Application | Application | Where our user applications (ex: Email) operate. HTTP, SMTP are here. |
6. Presentation | This layer formats and encrypts data to be sent across a network. | |
5. Session | Setting up, coordination, and termination sessions between applications. | |
4. Transport | Transport | Responsible for reliable data transmission. TCP/UDP operates here. |
3. Network | Internet | For data packet forwarding. IP operates here. |
2. Data link | Network Interface | Concerned with Physical addressing, error notification, etc. |
1. Physical | Transmitting raw bit streams over physical medium like wiring, cables, etc. |
References:
[OSI Model Explanation](https://www.imperva.com/learn/application-security/osi-model/)
[TCP vs UDP Comparison](https://www.guru99.com/tcp-vs-udp-understanding-the-difference.html)
When trying to understand how these technologies apply in practical environments, taking the time to visualize how common computer activities work behind the scenes can help paint a clearer picture. Whether it’s sending an email or enjoying your favorite show online, there’s a sophisticated structure operating in the background making sure your data gets where it needs to go.Let’s dive into the real-world applications of User Datagram Protocol (UDP) and Transmission Control Protocol (TCP), with a special focus on their use in live streaming, one of the main UDP applications.
Real-Life Application of TCP
The most common protocols for data transmission on the internet today are TCP and UDP. TCP commands a prominent presence due to its reliability and guaranteed delivery of packets, which makes it an excellent choice for various purposes like:
- Web Browsing: When you connect to a website, your computer uses TCP/IP protocol. Here, the Transmission Control Protocol ensures that all data packets arrive and in the correct sequence. It’s the reason why you get a complete webpage every time you browse the internet.
- Email Services: When you send or receive emails, TCP protocol is also at work. For instance, the ‘Post Office Protocol version 3 (POP3)’ and ‘Internet Message Access Protocol (IMAP)’ used for retrieving messages from a mail server, rely on TCP.
- File Transfers: The popular ‘File Transfer Protocol (FTP)’ for uploading and downloading files on the web is another TCP-based service. Even ‘Secure Shell (SSH)’, a secure replacement for Telnet, functions over TCP.
Real-Life Application of UDP
Unlike TCP, UDP doesn’t check packet order or ensures they’re even delivered, making it less reliable but faster; thus ideal for services where speed outweighs delivery guarantees.
- DNS lookups: Domain Name System (DNS) queries, where hostnames are converted into IP addresses, mainly utilize UDP as it’s quicker and tolerates loss of few packets without visible impact.
- Gaming: Online multiplayer games favor UDP for its speed. Packet loss or out-of-sequence delivery isn’t generally noticeable during gameplay, but a lag or delay due to TCP’s wait-for-acknowledgment characteristic would affect players’ performance.
- Live Streaming: This brings us to our key focus point – live streaming. Broadcasting live feeds, be it a sports event, a musical performance, or video conference (VoIP), leverages UDP over TCP because:
- In live broadcasts, there’s no provision for retransmitting lost data, so delivery guarantee is redundant. What matters more is seamless, continuous streaming.
- Speed matters significantly in maintaining sync between the video and audio streams. Here, UDP’s lightweight and fast nature comes handy.
- As against TCP’s sequential delivery, UDP permits out-of-order packet reception which might be acceptable in live transmissions.
TCP and UDP working together – An example
A practical scenario reflecting pairing of both TCP and UDP is live streaming via protocols such as HTTP Live Streaming (HLS) [source]. In HLS, the media file gets segmented into smaller chunks fed consecutively to the client. Primarily, the listing file (.m3u8) gets transferred via TCP first, providing information on available media files along with their URLs. Then, the media content segments fetch commonly over HTTP/TCP. However, for real-time streaming cases, these can be transported via UDP adopting suitable streaming protocols like Real-Time Transport Protocol (RTP).
Code Snippet
To grasp the application programming interface differences between TCP and UDP, take a look at this Python code snippet below demonstrating UDP socket creation and message sending:
import socket UDP_IP_ADDRESS = "your_server_ip" UDP_PORT_NO = 8888 Message = "Hello, Server" clientSock = socket.socket(socket.AF_INET, socket.SOCK_DGRAM) clientSock.sendto(Message.encode('utf-8'), (UDP_IP_ADDRESS, UDP_PORT_NO))
Note the use of the
socket.SOCK_DGRAM
parameter in creating the UDP socket. This same call, when replaced by
socket.SOCK_STREAM
, creates a TCP socket instead.
In conclusion, both protocols hold their unique strengths fitting specific application needs. While live streaming instances thrive better exploiting UDP’s speed and free-flow streaming advantages, various other internet services highly lean on TCP’s reliable and error-free packet handling.
TCP vs UDP: Real-Life Scenario Examination for Online Transactions
When we transact online, whether to buy a product, pay our bills or transfer funds, the interchange of data behind the scenes is crucial in ensuring smooth, accurate and secure transactions. Two popular protocols instrumental in this process are Transmission Control Protocol (TCP) and User Datagram Protocol (UDP).
Let’s delve deeper into TCP and UDP with some real-life examples to fully understand their importance and impact on our online transactions.
TCP: Transmission Control Protocol
One of the core protocols of the Internet Protocol Suite, TCP guarantees reliable, ordered delivery of a stream of bytes from applications or sources to their destinations. In simpler terms, it ensures your information reaches its destination correctly — like a courier who double-checks everything is in order before delivering your package.
Examples of TCP Usage in Daily Life |
---|
|
Lifewire offers a comprehensive reading about the vital role of TCP in our everyday internet usage.
Let’s consider a code snippet where Python’s built-in socket module is used to make a TCP connection:
import socket def tcp_connection(): s = socket.socket(socket.AF_INET, socket.SOCK_STREAM) s.connect(("www.python.org", 80)) request = "GET / HTTP/1.1\r\nHost: www.python.org\r\n\r\n" s.send(request.encode()) print(s.recv(4096).decode()) tcp_connection()
UDP: User Datagram Protocol
UDP, on the other hand, delivers datagrams without guarantees much faster and more efficiently. Consider it as postcards thrown in a mailbox, they’ll arrive at their destination but in no particular order nor guaranteed intact.
Examples of UDP Usage in Daily Life |
---|
|
To dig deeper into the world of UDP, check out this Ionos article explaining the User Datagram Protocol.
Consider a Python code snippet establishing a UDP connection:
import socket def udp_connection(): s = socket.socket(socket.AF_INET, socket.SOCK_DGRAM) s.bind(('localhost', 9999)) data, addr = s.recvfrom(1024) print("Received messages:", data, "from", addr) udp_connection()
By having these real-life glimpses into how TCP and UDP function during online transactions and beyond, we can better appreciate the complexity and power of the intricate network protocols governing our daily digital experiences.
A key real-world scenario where the Transmission Control Protocol (TCP) comes into play is during web browsing. Whenever you’re connecting to a website, using an application like Google Chrome or Firefox, the underlying technology that ensures data is transferred reliably across the internet from your device to the server and back is TCP.
To understand how TCP works in the context of web browsing, let’s break it down step by step:
- When you enter a URL, say google.com, into your web browser and press enter, your device initiates a request to establish a TCP connection with the server hosting the website.
- This start of communication is known as a TCP handshake, essential for creating a successful connection between your device (also known as the client) and the server.
- Only after this established connection can both entities begin exchanging information. Your device sends HTTP request verb such as GET, POST, PUT, DELETE over the TCP connection to ask for specific resources on the server. In this case, it might be the homepage data of google.com.
- The server then responds with the requested data, breaking it up into sequenced packets. The order of these packets is essential, and TCP ensures that they are correctly ordered when received.
- Finally, when you’re done browsing the website and close the tab or navigate away, your device ends the TCP connection by sending a message to the server indicating that it wants to terminate the connection, which can also be seen as friendly goodbye between your machine and the server.
Your Device: "Hello, can we connect and communicate?" Server: "Sure, let's establish a TCP connection."
Your Device: "SYN" Server: "SYN ACK" Your Device: "ACK"
Your Device: "GET / HTTP/1.1"
Server: "HTTP/1.1 200 OK"
Your Device: "FIN" Server: "FIN ACK"
So, there you have it – a fundamental rundown of how web browsers utilize TCP every time you’re surfing the net.
While discussing examples of protocols in real-life scenarios, it’s crucial to mention the User Datagram Protocol (UDP). UDP contrasts TCP for being stateless and having no built-in mechanism for guaranteeing reliability, which makes it ideal for speed-intensive tasks where dropping a packet or two doesn’t significantly impact the overall result – such as streaming audio or video content.
For instance, when you’re enjoying your favorite Netflix show, under the hood, information packets are continuously being sent from the server to your device mostly over UDP. Why? Because unlike reading a webpage where dropping or misordering a section of text (packet) could severely hinder the user experience, if a frame of a TV show arrives out of sequence or not at all, you’ll likely never notice as subsequent frames will continue to flow quickly, maintaining the uninterrupted streaming experience.
To conclude, TCP and UDP have unique uses stemming from their design constraints–TCP providing reliable data delivery suited for tasks like web browsing, while UDP, offering speedy data transfer with less concern for error checking and recovery, is best suited for streaming media.
As a professional coder, I can tell you that the User Datagram Protocol (UDP) and Transmission Control Protocol (TCP) are two essential protocols in networking. They play important roles in the gaming industry and can often be seen in real-life scenarios when it comes to online gaming experiences.
User Datagram Protocol or UDP is a communications protocol that offers a fast and lightweight method of message transmission across networks. This speed comes from UDP’s primary advantage: It doesn’t require any form of communication to establish a connection before data transmission occurs.
The significance of UDP in the context of gaming experiences cannot be overstated. Many online, multiplayer and real-time games use UDP because of its low latency and efficient data transfer capabilities. A perfect example could be “Fortnite”, a popular multiplayer game where timing and responsiveness matter greatly for the user experience.1
import socket def start_udp_server(): udp_server = socket.socket(socket.AF_INET, socket.SOCK_DGRAM) server_address = ('localhost', 12345) udp_server.bind(server_address) start_udp_server()
On the other hand, Transmission Control Protocol (TCP), is another commonly used protocol that ensures the delivery of packets from sender to receiver by establishing a connection. Unlike UDP, it guarantees that data arrives in order and without errors, which makes it highly reliable but slower due to the overhead of connection management.
A practical instance of TCP usage can be found in Multiplayer Online Battle Arena games (MOBA) like “League of Legends”, where players engage in strategic communications and actions that require reliable data delivery rather than ultra-low latency.2.
import socket def start_tcp_server(): tcp_server = socket.socket(socket.AF_INET, socket.SOCK_STREAM) server_address = ('localhost', 12345) tcp_server.bind(server_address) tcp_server.listen(1) start_tcp_server()
Table 1 illustrates the main differences between UDP and TCP in terms of their features as applied in real-world gaming applications.
Feature | TCP | UDP |
---|---|---|
Connection type | Connection-oriented | Connectionless |
Data delivery assurance | Guaranteed | Not guaranteed |
Order of packet delivery | Ordered | Not ordered |
Usage example in gaming | League of Legends | Fortnite |
In conclusion, both UDP and TCP have important roles in creating the best gaming experiences: UDP provides low-latency and efficient data transmission, whereas TCP ensures reliable and ordered data delivery.
The Transmission Control Protocol (TCP) is a crucial component of the Internet protocol suite. It can be found in a wide range of digital environments and everyday real-life scenarios. Just as UDP is essential for tailored, lightweight data transmission, so is TCP vital for ensuring reliable, ordered data delivery.
Role of TCP During File Transfers
For file transfers specifically, TCP shines brightly due to its key feature – reliability. Telnet, FTP (File Transfer Protocol), and SSH (Secure Shell) are three examples of protocols that utilize TCP for transferring files over a network. In essence, the protocol guarantees that each packet of data sent from a source host reaches its target destination, with no lost or duplicated packets, and in the correct order.
TCP achieves this by using various checks and balances:
- Acknowledgments: These confirm successful packet reception.
- Sequence numbers: They keep track of the packet order during transmission – useful when packets get mixed up during travel across different network paths.
- Window Sliding: Minimizes the inconvenience of sending acknowledgments after each packet transmission – essentially sending multiple packets before waiting for an acknowledgment.
// At a basic level, data is transmitted using TCP like so: Send packet 1 -> repeat until acknowledgment received Send packet 2 -> repeat until acknowledgment received
TCP in Real-Life Scenarios
TCP isn’t just utilized for backend operations. It has practical applications in our day-to-day activities on the Web, especially those requiring high reliability. Here are a few situations:
- Email: When you’re sending/receiving emails via SMTP, POP3, or IMAP protocols, TCP ensures your email gets to/from its destination without errors.
- Web browsing: Every time you visit a website, your browser communicates with a web server using HTTP or HTTPS, both of which are layered on top of TCP.
- Online shopping: Surely, you don’t want your orders messed up. TCP guarantees e-commerce transaction data is conveyed accurately from buyers to sellers.
Activity | TCP Protocol at Work |
---|---|
Sending/Receiving Emails | SMTP, POP3, IMAP |
Web Browsing | HTTP, HTTPS |
Online Shopping | HTTPS, FTP |
As evidence to the resilience and importance of TCP, both RFC 793 (The original TCP specification) and RFC 1122 (an enhancement of TCP including error handling) significantly highlight its role in modern networking.
In summary, TCP’s prime function is to provide a reliable mode for data transfer on the Internet, a critical requirement fulfilled with excellent precision and application, from everyday tasks like sending an email or making online purchases to back-end processes such as file transfers.
Voice over IP (VoIP) is a modern method used to provide digital phone services via the internet. Unlike traditional phone systems that rely on circuit transmissions, VoIP leverages the Internet Protocol’s connections for transmitting telephonic signals. Interestingly, it doesn’t limit itself to just one protocol; instead, it utilizes both TCP (Transmission Control Protocol) and UDP (User Datagram Protocol) for different purposes.
Role of TCP in VoIP
TCP is designed to provide reliable, ordered, and error-checked delivery of a stream of octets. It governs the establishment of a connection and ensures that all packets reach their destinations in the right order.
When making calls using VoIP services, establishing the initial communication or “handshake” between two devices is handled through TCP. VoIP utilizes SIP (Session Initiation Protocol) which uses TCP to set up the communication before any conversation takes place.
For instance, in real life, consider you’re making a Skype call. When you initiate the call, the invite message sent by your Skype client to the other party is sent using TCP. The primary goal here is to ensure the initiation information is delivered accurately without missing any data.
//Simplified flow of a typical TCP connection client.connect(); server.accept(); client.send(data); server.receive(data);
Role of UDP in VoIP
On the other hand, UDP enables efficient transmission because it does not perform error-checking procedures and it broadcasts data regardless of whether the receiving device is ready.
Since VoIP involves a continuous flow of data (like voice streams), it employs UDP during the actual call. This is mainly due to the “real-time” nature of audio where even if some packets drop during transmission, the overall quality of the call remains unaffected.
For example, after establishing the connection on Skype, when you are actually talking to the other person, the voice packets are transmitted over UDP. Here, even if some packets are lost, there won’t be a noticeable impact on the conversation.
//Simplified flow of a typical UDP connection client.send(data); server.receive(data);
So, as we see, both TCP and UDP serve their unique roles within the functionality of VoIP. TCP grants reliability during the call setup phase while UDP ensures faster and efficient transmission of voice packets during the call.
Refer to this resource for a more detailed explanation of VoIP’s working.The User Datagram Protocol (UDP) plays quite a crucial role when it comes to the Domain Name System (DNS). While comparing the importance of UDP in DNS and Transmission Control Protocol (TCP) usage in different scenarios, it’s important to first understand how these protocols work.
On the internet, there is massive data being sent between computers and servers, and ensuring that this data travels accurately and quickly is vital. Here, both TCP and UDP come into play. Both are transport layer protocols used for sending bits of data – known as packets – over the web.
UDP, unlike TCP, sends packets without first establishing a connection. This means no effort is made to ensure all packets have been correctly received at the other end. However, due to this lack of extra checkings, UDP-based communications can be faster. That’s where DNS takes advantage of UDP.
In terms of DNS lookup, when you type a URL into your browser like www.google.com, your browser uses UDP to ask the nearest DNS server if they know what IP www.google.com corresponds to. The DNS server responds with the corresponding IP address, which again uses UDP. The choice of UDP here reflects the critical aspect of speed over confirmed delivery in DNS lookups, as these need to be rapid but not necessarily perfectly accurate on every occasion. If a packet gets lost, the client just retries.
//Here's a simplified DNS query using UDP: const dns = require('dns'); const options = { family: 6, hints: dns.ADDRCONFIG | dns.V4MAPPED, }; dns.lookup('example.com', options, (err, address, family) => console.log('address: %j family: IPv%s', address, family) );
For real-world examples of TCP and UDP:
TCP is like making a telephone call. When you call someone, you dial their number, wait for them to pick up, and only then do you begin talking. If they don’t pick up, you hang up and try again later. Reliability is key here; similar to data transmission via TCP.
UDP, on the other hand, is more comparable to dropping letters in the mail. You write the recipient’s address on the envelope and then drop it in the mailbox. There’s no guarantee they’ll receive it, and you get no notification if it goes missing. Any follow-up relies on the recipient reaching out to you by some other means. This is typically suited to services like live streaming or multiplayer gaming where small amounts of data loss are acceptable compared to maintaining speed and continuity.
Thus, though practically different, both TCP and UDP have their unique places in the network communication landscape. Understanding how each is employed in various scenarios aids in understanding why programs behave as they do.
More information on the use of TCP and UDP can be found here.
Certainly, let’s embark on this journey by exploring the importance of User Datagram Protocol (UDP) in video conferencing services and then further dive into real-life examples of both Transmission Control Protocol (TCP) and UDP.
Video conferencing in today’s age is a fundamental part of our daily activities, whether it’s for professional purposes, personal interaction, or even educational learning. It requires real-time transmission of audio and video data between multiple users across different locations, and that’s where UDP plays an instrumental role.
UDP In Video Conferencing Services
UDP is a straightforward transport layer protocol with no congestion control functionalities source. This minimal approach makes it suitable for video conferencing applications where high speed and low latency are crucial.
Here’s why UDP becomes essential:
- Speed: UDP doesn’t waste time establishing a connection before sending data.
- Real-Time Communication: It’s more important for the conversation to continue smoothly than to have every single packet arrive in order—UDP caters to this need.
- Low Latency: UDP avoids network congestion – critical for video conferencing that cannot tolerate significant delays.
Let me provide an analogy. If TCP is like sending a certified mail through post office ensuring each letter sent is received and in order, UDP, on the other hand, would be akin to throwing packages out of a plane to ground regardless of whether anyone is there to catch them.
Examples of Real-Life Use Of TCP And UDP:
1. Web Browsing (TCP):
Every request to load a webpage is sent from your browser to the server using TCP because it provides reliability—an essential feature given that we don’t want missing pieces of webpages; the browser needs all packets to render the page correctly.
TcpClient client = new TcpClient("YourSite.com", 443);
2. Online Gaming (UDP):
Many online multiplayer games use UDP. The reason revolves around its design philosophy: It’s better to drop packets and maintain game speed than ensure reliable delivery, causing lag during gameplay.
UdpClient udpClient = new UdpClient("GameServer.com", Convert.ToInt32("GamePort"));
3. Email (TCP):
Email clients send data via SMTP using TCP. Here, we need every email to get delivered with no parts missing or in the wrong order.
SmtpClient smtpClient = new SmtpClient("smtp.your-site.com"); // Send using TCP smtpClient.Send(messageDetails);
4. Live Streaming/Video Conferencing (UDP):
As discussed, live video communication prefers occasional loss of information over delay in transmission; hence, services like Skype, Zoom, use UDP.
Socket udpSocket = new Socket(AddressFamily.InterNetwork, SocketType.Dgram, ProtocolType.Udp); udpSocket.Connect("zoom.us", Convert.ToInt32("ZoomPort"));
In summarizing, while TCP and UDP serve vastly different scenarios, they both play a significant role in our digital experiences. Whether it’s sending an important professional email, hosting a smooth video conference meeting, browsing a webpage, or just enjoying an online game, these protocols lie at the heart of internet communication.
TCP (Transmission Control Protocol)
and
UDP (User Datagram Protocol)
are both core components of the Internet Protocol Suite, playing pivotal roles in transmitting data between computers over a network. In real life scenarios, these protocols demonstrate their functionality in ways which have direct impacts on our daily digital interactions.
Firstly, TCP is typically used in applications where delivery of data from source to destination is absolutely essential. One classic example is when we send an email [source]. The TCP protocol is responsible for ensuring that every byte sent is received by the destination in correct order, crucial when sending important documents or information via email.
In contrast, UDP is used where speed is more critical than ensuring perfect delivery of data. A quintessential example is online video streaming services like YouTube or Netflix. These services prefer using UDP as it allows for faster transmission of data packets. Even if some packets are lost or arrive out-of-order (which will usually result in minor momentary video glitches), it won’t significantly affect your overall viewing experience [source].
Therefore, both TCP and UDP have distinct usages based on the requirements of the application. Having a clear understanding of which scenarios call for TCP and which call for UDP can greatly enhance application performance. Whether sending a vital email attachment or seamlessly streaming your favourite movie online, these protocols make our digital communications possible in seemingly effortless ways.
Here’s how you could summarize these instances in table format:
Protocol | Use Case Scenario |
---|---|
TCP | Sending emails |
UDP | Online video streaming services i.e. YouTube, Netflix |