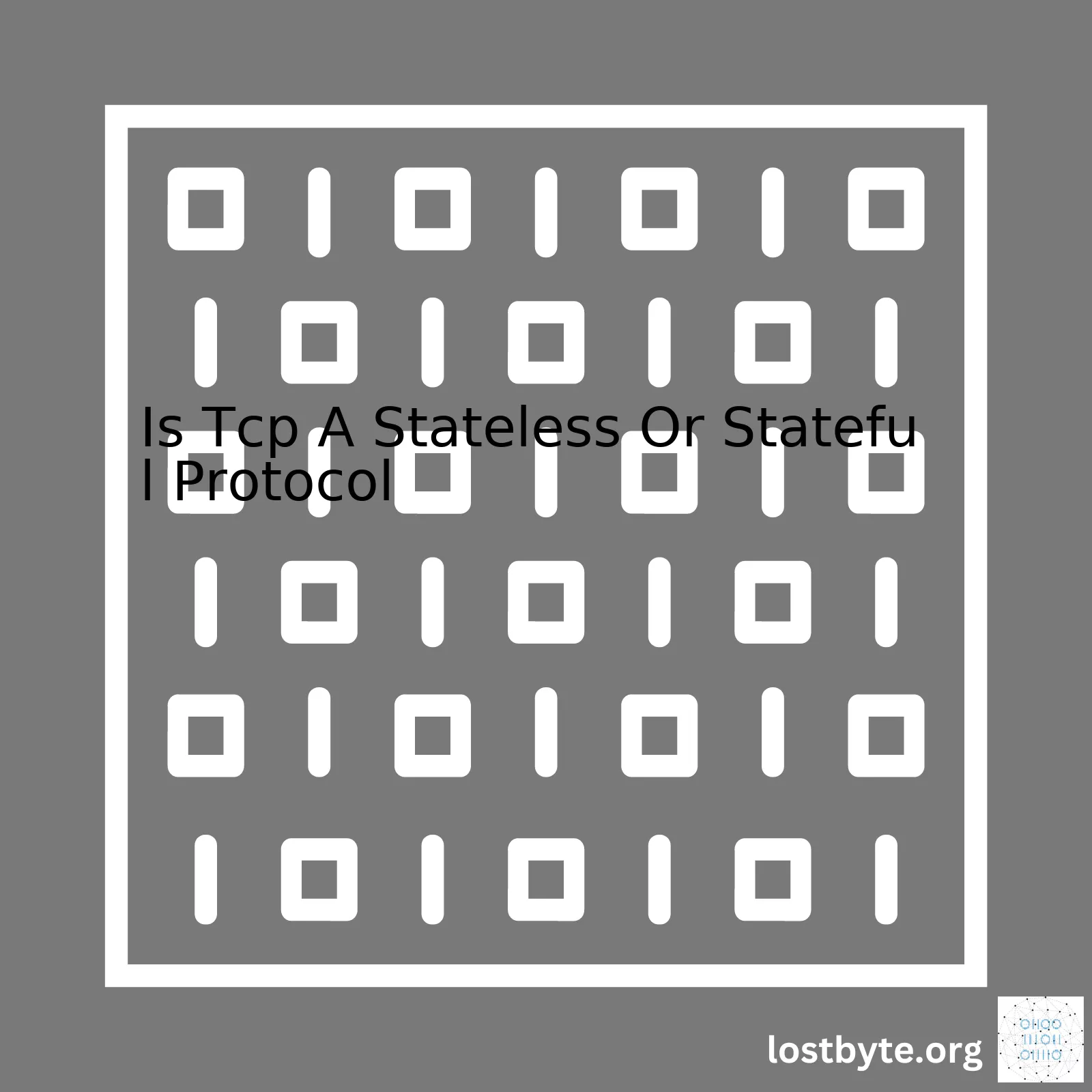
Protocol type | Description |
---|---|
Stateless | These protocols do not keep track of any connection state. They treat each data packet as independent and separate from the others. |
Stateful | These protocols maintain a state or status of each network connection. They are aware of previous and ongoing communication sessions. |
TCP (Transmission Control Protocol) | It is a stateful protocol. TCP establishes, maintains and terminates the network connections while ensuring the reliable transfer of data packets. |
The Transmission Control Protocol (TCP), unlike Stateless protocols (like HTTP), is Stateful. This means that TCP tracks every single transaction made over the network, in a meticulously structured manner. So, when you send a request over the internet, it’s TCP’s job to make sure all packets reach their destination.
To ensure this, TCP uses something called a ‘handshake’. This three-way TCP handshake process is used to establish a connection, exchange data, and finally terminate the connection between the communicating systems.
Here is where the term ‘stateful’ enters; throughout this entire process, TCP keeps track or stays aware (‘maintains a state’) of discernable data variables like sequence and acknowledgment numbers. This meticulous tracking ensures replacement of missing data, correction of out-of-order segments, and optimization of data flow rates.
In technical terms, when a client initiates a TCP connection with a server, the connection on the server goes through several ‘states’ before the connection is established. The states include LISTEN, SYN-RCVD, ESTABLISHED, etc., justifying why TCP is indeed classified as a stateful protocol.
Let me illustrate this using some Python code:
import socket s = socket.socket(socket.AF_INET, socket.SOCK_STREAM) s.bind(("localhost", 9999)) s.listen(1) while True: c, addr = s.accept() print ('Got connection from', addr) c.send('Thank you for connecting') c.close()
This basic script sets up a simple TCP server in Python. It listens for incoming connections, accepts them, sends a message to the client, and then closes the connection. If you scrutinize the code, you’ll notice ‘listen’ and ‘accept’, both methods affirming that TCP follows a stateful protocol by maintaining and transforming the state of connections.
Python’s socket module, which is used here, builds on top of TCP’s stateful attributes, providing even the most basic scripts the ability to track connection states easily. For people new to networking in Python, understanding that TCP is stateful is vital, as it’s the core reason why the TCP/IP suite is reliable and widely used on the World Wide Web.When it comes to networking protocols, a fundamental principle to grasp is the distinction between “stateless” and “stateful” protocols. TCP, or Transmission Control Protocol, is perhaps one of the most prevalent and significant examples.
TCP is considered a stateful protocol. This connotes that it keeps track of the state of the connection at every point during data transfer. Unlike its stateless counterparts, a stateful protocol does not lose information if the server restarts or if the client reboots. This makes it more robust and reliable for transmitting data, particularly in an unstable network environment.
char data[] = "I Love TCP"; // Creating socket file descriptor int sockfd = socket(AF_INET, SOCK_STREAM, IPPROTO_TCP); struct sockaddr_in servAddr; servAddr.sin_family = AF_INET; servAddr.sin_port = htons(PORT_TCP); servAddr.sin_addr.s_addr = inet_addr(ip_address); // Connect connect(sockfd, (struct sockaddr *) &servAddr, sizeof(servAddr)); send(sockfd, (void*) data, strlen(data), 0);
The example shown illustrates a simplified version of sending data over a TCP communication using socket programming. We can highlight some key elements in relation to TCP being stateful:
To begin the process, TCP starts with a three-way handshake; this is made up of a SYN segment from the transmitter to the receiver, an ACK-SYN from the receiver to the transmitter, and finally an ACK back to the receiver from the transmitter. This exchange ensures both ends are ready for data transmission and establishes the initial sequence numbers for the conversation. The connection remains open until it is closed by either party.
Looking over the TCP/IP stack, you will notice that several layers contribute to the statefulness of TCP. The transport layer (where TCP operates) communicates with both the internet layer below (which uses IP, a stateless protocol) and the application layer above which can use different stateful or stateless protocols. This multilayer communication further validates the stateful nature of TCP, demonstrating its ability to coordinate various application needs and handle interruptions seamlessly, enhancing overall data reliability.
Referencing the OSI model, we can appreciate why the concept of being stateful serves to underpin TCP’s reliability and error-checking mechanisms. Stateful protocols like TCP allow controlled packet retransmission for lost packets and proper sequencing for the order in which packets have been transmitted.
while (1) { int n = recv(tcp_sockfd, recvBuff, sizeof(recvBuff)-1, 0); // If an error occurs if(n < 0) { printf("\n Error : %d \n", errno); return 1; } }
As illustrated in the above code snippet, the receiver can request the sender to resend missing data or data that was packaged incorrectly. It also sends acknowledgments for the successful receipt of correct data packets.
On another note, TCP employs a mechanism called flow control, which adjusts the data transmission rate according to the network's current condition. For instance, if the receiving device's buffer becomes overloaded, TCP allows the receiver to signal the sender to slow down until it's ready again. This dynamic adjustment would be impossible without maintaining a stateful connection.
These potent stateful features make TCP particularly fitting for systems requiring high-level data integrity like HTTP, SMTP, FTP etc.
You might find out more on stateful and stateless protocols here, O’Reilly Media’s guide provides a more thorough overview of TCP. Also, RFC793 on Internet Engineering Task Force(IETF)'s site gives a detailed explanation about Transmission Control Protocol functionality.The Transmission Control Protocol or TCP was first described in a Request for Comments (RFC) document published by Vint Cerf and Bob Kahn back in 1974. It's the result of network research, including packet network interconnection and the host-to-host protocols carried out by them on behalf of the Defense Advanced Research Projects Agency (DARPA). Over the years, TCP has evolved into one of the main protocols in use today, forming the foundation of any network communication that requires reliability on the internet.
Now, answering on whether TCP is stateless or stateful, it needs to be noted that TCP is indeed a stateful protocol. This is because it keeps track of several variables when establishing a connection, with each connection being characterized by the combination of source IP address, source port number, destination IP address, and destination port number. Here are some key aspects explaining why TCP is categorized as a stateful protocol:
- Maintains State Information: When a TCP connection starts, it begins with a handshake process that sets certain variables between the sender and receiver. This establishes a virtual link for communication, and the state of this link is maintained throughout the duration of the connection.
- Reliable Data Transfer: By maintaining state information and unique identifiers for each stream of data or sequence numbers, TCP guarantees successful data delivery. It does so through mechanisms such as acknowledgments, retransmission of lost packets, etc.
- Ensures Order and Error checks: TCP uses sequencing information to ensure data is received in the correct order at the receiving end. It also contains provisions for checksums to detect and rectify errors.
To illustrate the essence of a stateful protocol like TCP, consider this simple example where we're implementing server-client communication:
//Initiating connection from client side Socket s = new Socket("192.168.0.1", 8080); //Maintaining connection state on server-side ServerSocket ss = new ServerSocket(8080); Socket s = ss.accept();
In the above code snippet, a three-way handshake process happens: SYN, SYN-ACK, and ACK, which ensures the connection setup. Afterward, the same socket is used to maintain the stateful connection.
Let's see a simple illustration of how this connection proceeds:
-----------------------------------------------
Client Server Action
--------- --------- ------
SYN -----------> Client sends SYN to the server
<---------- SYN + ACK Server responds with SYN and ACK
ACK -----------> Client acknowledge server's response
DATA ---------> Data transmission begins
-----------------------------------------------
Further resources on understanding how TCP evolves making use of its stateful nature can be discovered more in IETF RFC 793.
Yet, while TCP’s statefulness is an advantage in many aspects such as reliability and orderliness, it does introduce additional overheads as compared to stateless protocols such as UDP. That said, the choice between stateful and stateless protocol depends on the specific requirements of your application.TCP, short for Transmission Control Protocol, is an integral part of the internet protocol suite and responsible for managing the delivery of data between systems over the internet. While talking about whether TCP is a stateful or stateless protocol, it's important to first understand these two concepts.
Stateless Protocol:
A protocol is termed "stateless" if it does not require keeping track of interactions and each command is processed individually without regard to the commands that came before. HTTP (Hypertext Transfer Protocol), for example, is a stateless protocol. It treats each request as an independent transaction that is unconnected with any previous request (IETF).
Stateful Protocol:
In contrast, a "stateful" protocol remembers state information from one session to another. It maintains session information, such as settings, events, activity, and other conditions that help in future transactions
.
Regarding TCP, each connection established is unique and packets are delivered in sequence. The protocol "remembers" this connection and the associated parameters throughout its lifecycle. Hence, it falls into the category of a stateful protocol.
// Code displaying TCP establishing and terminating connection tcpClient = new TcpClient(); // Assumed server and port tcpClient.Connect("192.0.2.1", 25); goodConnection = tcpClient.Connected; if(goodConnection == true){ // Connection successfully established, you can start sending data here } // At the termination part: tcpClient.Close(); // or use tcpClient.dispose()
From the code snippet above, we see that TCP establishes a connection (in this sample case, with a server located at "192.0.2.1" on port 25). If the connection is successfully built, `tcpClient.Connected` returns
true
, and hence the state 'connected' is remembered until the line where connection is being closed.
In conclusion, TCP inherently supports connection-oriented sessions and is therefore classified as a stateful protocol. It tracks all connections and related activities for the duration of the communication. This makes TCP reliable as it ensures that all packets arrive at their intended destinations.
Transmission Control Protocol, commonly referred to as TCP, is a method employed by the Internet Protocol (IP) to send data in the form of message units between computers over the internet. While IP takes care of handling the actual delivery of the data, TCP takes charge of keeping track of the 'packets' that a message is divided into for efficient routing through the net.
Is TCP a Stateless or Stateful Protocol?
TCP is known to be a stateful protocol as it maintains and keeps track of a state during its communication process. This characteristic distinguishes TCP from several other protocols that are categorized as stateless protocols.
A stateful protocol like TCP closely monitors the status and reception of all transferred packets of data. It does so by creating a 'connection' between the two points communicating and tracking which packets are being sent at any given time to ensure every piece of data reaches its destination. If packets are lost or failed due to errors, TCP can retransmit those specific packets until they are successfully delivered. You could say that TCP behaves more like a diligent mail carrier who returns to the sender if they cannot successfully deliver a package, ensuring successful delivery always takes place.
Key Aspects of TCP Being a Stateful Protocol
- Connection Establishment: Before any real data transmission happens, TCP requires a connection setup using the three-way handshake method. In this exchange, both sender and receiver agree upon certain parameters to establish a reliable connection.
- Error Checking and Recovery: Each TCP segment contains a checksum which allows the receiver to verify the integrity of the data received. If the checksum does not match, the segment will be discarded and retransmission will be required.
- Ordered Data Transfer: Every byte of data sent has a sequence number which ensures they are put back together in the correct order at the receiver's end.
- Flow Control: TCP incorporates a flow control mechanism which prevents a fast sender from overwhelming a slow receiver.
- Congestion Control: TCP includes algorithms to avoid network congestion, ensuring it doesn't exceed the network's capacity.
Protocol | Stateful/Stateless | Description |
---|---|---|
HTTP | Stateless | Without session tracking, each request from client to server is considered a new one. |
TCP | Stateful | Maintains connection and tracks package delivery till successful receipt. |
UDP | Stateless | No tracking of sent packages, unlike TCP, hence more suited for continuous streaming of data. |
In conclusion, TCP is indeed a stateful protocol - deeply embedded in the maintenance and supervision of its connections and communications. Therefore, it forms the backbone of reliable and orderly data exchange on the internet, succoring stable connectivity on the world wide web. If you would like further reading I do suggest visiting this comprehensive guide about stateful vs stateless protocols.
Stateful vs Stateless Protocols: A Deep Dive into TCP
To truly understand whether TCP is a stateless or stateful protocol, we first need to define and explore the intricacies of Stateless and Stateful protocols.
What Defines a Stateless Protocol?
A stateless protocol does not require the server to maintain a session or keep track of the client's information across multiple requests. Each request is treated as an isolated transaction, independently of any previous request.
To illustrate this, let's consider the example of HTTP. In its basic implementation (without cookies), HTTP is a pure stateless protocol.
Client -> GET /homepage -> Server Server -> 200 OK, [Here's your homepage] -> Client Client -> POST /login, [credentials] -> Server Server -> 200 OK, [You are now logged in] -> Client Client -> GET /user/profile -> Server Server -> 403 Forbidden, [Who are you?] -> Client
As seen here, the server forgot the login action that previously occurred. This is because HTTP inherently does not "remember" previous transactions.
What Defines a Stateful Protocol?
Contrarily, a stateful protocol maintains state between the client and server. The server keeps track of the client's activities from the start of the session until it ends. Essentially, the connection persistently carries the 'state' of the interaction.
One of the best examples is File Transfer Protocol (FTP) where after a successful login, all subsequent FTP commands are associated with that user.
Client -> USER anonymous -> Server Server -> 331 Please specify the password. -> Client Client -> PASS random_password -> Server Server -> 230 Login successful. -> Client Client -> LIST -> Server Server -> 150 Here comes the directory listing. -> Client
In the above scenario, the server remembers that the client has authenticated, hence listing the directories is permitted.
TCP Protocol: Stateless or Stateful?
Transmission Control Protocol (TCP) exhibits behavior that characteristically aligns itself with stateful protocols. It establishes a 'connection' before data transmission and retains state during the entire connection.
TCP uses a three-way handshake (IBM Documentation) for establishing connections:
Client -> SYN -> Server Server -> SYN, ACK -> Client Client -> ACK -> Server
This sequence solidifies the idea of a 'persistent connection'. The server acknowledges receipt of the synchronization (SYN) packet and reciprocates. The client then acknowledges the server's response. Only post this sequence do they start exchanging data.
Even during data exchange, each piece of information sent from the host to the server or vice versa must be acknowledged. Hence, TCP would quite accurately be termed as a stateful protocol as it maintains a continued interaction, ensuring reliability and ordered data transfer.
The point here is, understanding these inherent differences between a stateful and stateless protocol unveils the true nature of how TCP operates. It's clear that TCP, due to its maintained state throughout an established connection, actually falls more within the realm of a stateful rather than stateless protocol.TCP, which stands for Transmission Control Protocol, is often considered the backbone of internet communications - it's fundamental to how data is successfully delivered from one point to another online. Diving deep into TCP's architecture will shed light on understanding its stateful nature.
Contrary to some other protocols like HTTP, named as a stateless protocol due to its detachment after data delivery, TCP is classified as a stateful protocol. This is because it constantly maintains connection status information during the communication process between two network endpoints.
Now let’s take part in the architectural overview.
In TCP, to send and receive data among devices reliably, an initial connection or "handshake" is established. Below is a simplified representation of this three-way handshake.
Action | Description |
---|---|
SYN (synchronize) | The client sends a SYN packet to the server to initiate a TCP connection. |
SYN/ACK (synchronize acknowledgment) | Upon receiving the SYN packet, the server responds with a SYN/ACK packet. |
ACK (acknowledgment) | Lastly, the client sends back an ACK packet to complete the handshake and commence data transmission. |
The code-level implementation of establishing a TCP connection in Python would look something like this:
import socket # Create a socket object soc = socket.socket(socket.AF_INET, socket.SOCK_STREAM) # Connect to the server at address (localhost, port) soc.connect(('localhost', port))
This whole process is indicative of maintaining a 'state' or 'awareness'. Throughout the life of a TCP session, it records byte order, sender and receiver ip addresses, acknowledges received packets, handles retransmissions for lost data and more. The protocol tags each byte with a unique sequence number so that even if the data is delivered out of order at the receiving end, a correct order can be reconstructed. Hence, it allows reliable and ordered data delivery between application programs over IP networks.
Key characteristics of the TCP being a stateful protocol include:
• Memory of past events (like prior requests).
• Mechanism to understand exactly how far along in a conversation one is (through sequence numbers).
• Capability of actively adjusting to network conditions (such as congestion).
These particular characteristics turn out to be beneficial to many higher-level applications that need reliability and completeness of transmitted data, examples include web browsing, email sharing, file transmissions using FTP, etc., where losing a bit of data could lead to misunderstanding of information.
Therefore, it's clear from the architecture and principles of operation - TCP is rightly classified as a stateful protocol. Given all these features implemented within this protocol, we gain robust error recovery mechanisms able to cope with diverse networking circumstances. This is the reason why the Internet, as we know today, is largely operational owing to the stateful nature of TCP.
References:
• How TCP Works
• Transmission Control Protocol (RFC 793)
• Python Socket ProgrammingThe nature of network communication protocols either being stateless or stateful is paramount in understanding data exchange across the web. The Transmission Control Protocol (TCP), for example, plays a crucial role in this.
Let's dive right into determining whether TCP is indeed a stateless or stateful protocol and its significance in network communication.
The Nature of TCP: Stateful Protocol
At a glance, the TCP is a stateful protocol. What exactly does being stateful mean you might ask? Well, stateful protocols maintain and track information about their state throughout the entire session between sending and receiving devices. When it comes to the TCP protocol, it keeps the status of packets sent - noting whether they arrived at their destination or not.
As part of the design of TCP, it ensures:
• Reliable Data Delivery: Each packet gets acknowledged when received.
• In-Order Delivery: Packets are ordered as per the sequence they were sent.
• Error-Free Data Transfer: TCP performs error checking to ensure data integrity.
An excellent usage example of TCP's stateful nature would be when you're downloading a large file. If there's an interruption during the process, the download can resume from where it left off, instead of starting all over again. That’s the power that the stateful nature of the TCP protocol brings!
The Technical Backbone: TCP Header
To further comprehend the stateful nature of TCP let's look at these elements contained in its header:
Source Port | Destination Port Sequence Number Acknowledgment Number Data Offset | Reserved | Flags Window Size Checksum | Urgent Pointer Options and Padding
Of prime importance here, is the Sequence Number and Acknowledgement Number. The Sequence Number keeps track of a byte in a stream of data from source to destination, while the Acknowledgement Number indicates the next expected byte from the other side.
Protection from duplicate packets is another benefit reaped from the use of sequence numbers in the TCP header.
Handling Network Communication via States
Maintaining states in TCP enhances the handling of communicational aspects such as connection establishment, data transfer, and connection termination. This is facilitated by going through various operational states and transitions, such as LISTEN, SYN-RECEIVED, ESTABLISHED, FIN-WAIT-1, CLOSING among others.
These states guide the interaction flow between the sender and receiver during a TCP session. They interpret the synchronization of sent and received packets, maintaining record of received data packets and even managing connection termination.
The Big Picture: Role and Importance in Network Communication
Being stateful has a significant influence on TCP's efficacy in network communication. By retaining the state, TCP provides mechanisms for robust, reliable, and ordered data transfer. Its capacity to maintain sequences, acknowledge receipt, ensure data integrity and handle resends in case of lost packets make TCP an attractive choice in network communications demanding these attributes.
The approach taken by the TCP/IP model, which combines the best of both stateful and stateless paradigms, depending on the layers – TCP layer being stateful and IP layer being stateless, makes it one of the most widely adopted models for network communications.
Therefore, TCP's stateful nature allows us to carry out online activities with much-needed stability and accuracy, making our virtual interactions smooth and facilitating the ever-connected world we operate in today.Sure, let's dive into how TCP's three-way handshake process works and look at how sessions are established and maintained in TCP. During this exploration, I will also address whether TCP is a stateless or a stateful protocol.
Understanding the three-way handshake process is fundamental to understanding the nature of TCP (Transmission Control Protocol). This exchange ensures that both parties initiating a connection are operational and ready for data transmission. It's called a "three-way" handshake because there are three steps:
- SYN: The active open (client) sends a SYN (synchronize) packet to the server.
- SYN-ACK: In response, the server acknowledges the client's SYN packet by sending back its own SYN packet with an ACK (acknowledgement).
- ACK: Lastly, the client acknowledges the server's SYN packet, thus establishing a reliable connection.
The sequence is represented as
SYN, SYN-ACK, ACK
.
Now let's examine where TCP stands - is it stateless or stateful?
Contrary to HTTP, which is a stateless protocol, TCP is indeed a stateful protocol. It maintains the state of a session as long as the connection remains open between two endpoints or hosts. Throughout the communication, TCP keeps track of various parameters like IP addresses, ports, sequence numbers, acknowledgment numbers, window sizes, etc., which define the state of the ongoing conversation.
A table might give us more clarity. Consider the following simplified view of TCP's maintained parameters:
Parameter | Description |
---|---|
IP Addresses | The Internet Protocol addresses of source and destination nodes |
Ports | The logical points for sending and receiving data |
Sequence Numbers | Numbers used to order packets in their correct sequence |
Acknowledgment Numbers | Numbers used to confirm the receipt of packets |
Window Sizes | Defines the amount of data that can be sent without requiring an ACK |
The tracking of these parameters makes TCP highly reliable for data transfer over networks as it guarantees the delivery of packets in sequence and without corruption. It ensures the delivery of packets by using a method called positive acknowledgment with retransmission, where if a sender does not get an acknowledgment, it would retransmit the packet.
This statefulness can mean additional overhead in terms of resource usage, but it provides considerable benefits in delivering reliable and ordered data transmission. So in answering the question, yes, TCP is a stateful protocol.
For example, we can set up a TCP connection in Python with the socket library like so:
import socket # Create a TCP/IP socket sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM) # Connect the socket to the server's port server_address = ('localhost', 10000) sock.connect(server_address) try: # Send data message = 'This is the message.' sock.sendall(bytes(message, encoding='utf8')) finally: sock.close()
To summarize, the stateful nature of TCP, along with its three-way handshake process ensures reliable, ordered, and error-checked delivery of streams of bytes from one host to another. You might want to check out this link to TCP standards documentation for more intricate details about TCP.TCP, an acronym for Transmission Control Protocol, is a foundational pillar of the internet. TCP is a stateful protocol as it constantly keeps track of its state through multiple data exchanges between the sender and receiver.
To understand why TCP is considered a stateful protocol, we first need to delve in-depth into how packet transmission operates within this network model.
Packets in TCP
The communication layer responsible for creating packets from application data is called the Transport Layer. In addition to formatting the data into packets, this layer also ensures that all packets are sent and received in order. While each packet has its own identity with information about sequence number, the destination IP address, and so forth, they operate independently.
Let's take a glance at the series of interactions that uphold the life cycle of TCP:
The Three-way Handshake
This is the initial stage where connection establishment takes place:
- SYN: The sender will initiate the connection by sending a packet tagged with a Synchronization (SYN) flag.
- SYN-ACK: On receiving the SYN flag, the recipient will reply with an Acknowledgment (ACK) flag and another SYN Flag.
- ACK: Finally, the sender confirms the connection by reciprocating back with an ACK flag.
Only after completing this three-way handshake, data transfer begins. A piece of code depicting this process would look like this:
# Assuming sender and receiver are already defined sockets sender.send('SYN') response = receiver.recv(1024) if response == 'SYN-ACK': sender.send('ACK')
Between every transmitted packet there’s an acknowledgment signal returned. If this doesn't happen, the sender knows something went awry and sends the packet again.
Maintaining Connection State
TCP employs sequencing and acknowledgement numbers to retain its connection state. This way, the transferring parties have a comprehensive snapshot of what has been transferred, acknowledged, and what needs retransmission.
Connection Termination
Just as the connection was established through a series of flag exchanges, termination also follows a four-way handshake:
- FIN: Indicating that it has no more data to send, the sender tags its packet with a Finish (FIN) flag.
- ACK: On receiving the FIN signal, the receiver acknowledges with an ACK flag.
- FIN: Once all outstanding data is processed, the receiver sends a FIN flag.
- ACK: Finally, the sender acknowledges the closure with an ACK flag.
This sequence ensures a safe and robust sign-off.
It's evident that TCP continually tracks information throughout this entire life-cycle, rendering it a stateful protocol. Thus, statefulness in TCP ensures reliability, sequenced delivery, and error-checking, making it suitable for applications where data integrity surpasses speed considerations.
Additionally, the intricacies of the data transmission explained above underpin that TCP is full-duplex, bidirectional, meaning both communicating devices can send and receive data simultaneously.
Remember, being stateful comes at the cost of additional consumption of resources such as memory and processing power, as scads of metadata need maintained throughout the lifecycle of the connection. So, TCP works astoundingly when resource constraints are not significant or if your task emphasizes on precision over performance.Session maintenance in a TCP/IP environment is directly tied to its properties as a stateful protocol. Unlike stateless protocols, Transmission Control Protocol (TCP) establishes a connection between two devices before communication begins. Once this connection setup is complete, the conversation can begin and persist until one or both ends signal that they want to end the session. This is conceptualized using a sequence of events known as the 'TCP three-way handshake.'
Here are the steps for initiating a session:
- SYN: The initiating client sends a synchronization packet to the server.
- SYN-ACK: In response, the server replies with a packet acknowledging the synchronization request.
- ACK: Finally, the client acknowledges receiving the server's response, thereby completing the three-way handshake.
This three-step process forms a significant part of session maintenance in a TCP/IP context.
For coding applications, this translates to a series of calls to create TCP sockets and connect them. For instance, writing code in Python would involve importing the socket module and then calling methods like
socket()
,
connect()
,
send()
, and
close()
.
import socket def tcp_client(): host = "localhost" port = 8000 # Create a socket s = socket.socket(socket.AF_INET, socket.SOCK_STREAM) # Establish a connection s.connect((host, port)) # Send some data s.sendall(b'Hello, Server') # Close the connection s.close()
Although there’s no explicit session concept found in the above code, it implicitly happens as part of the process. The
connect()
function initiates the 'TCP three-way handshake' for establishing a session, and it remains active until
close()
is called.
To monitor and handle this connection status, TCP uses a combination of flags, sequence numbers, and acknowledgement numbers embedded in TCP header fields.
Several mechanisms ensure reliable data transport within the session, including:
- Error detection through checksums.
- Sequence numbers to coordinate the order of bytes.
- Acknowledgement numbers to track what has been successfully received.
- Window sizes to manage how much data can be sent at once to prevent overloading the receiver.
- Various control flags (e.g., SYN, ACK, FIN) for establishing, maintaining, and ending sessions.
Coupled with retransmissions if packets are lost or corrupted, these properties make TCP a reliable, stateful protocol. The nature of being stateful allows TCP to maintain a session until explicitly closed by the client or the server.
Further on, remember that being stateful means storing information about every session for its duration. Each established connection requires memory and compute cycles, potentially making the system slower under heavy loads. However, the benefit of ensuring delivery and ordering of packets outweighs this cost in most application contexts.
You may delve deeper into the topic on resources like RFC 793, which provides comprehensive details about TCP.
Today, the discussion revolves around the nature of TCP (Transmission Control Protocol) in context with whether it is a stateful or stateless protocol. Now what does being stateful or stateless mean? Protocols that maintain a record or 'state' of their interactions are known as 'stateful', whereas those who do not save any conversation data are called 'stateless'. This ability to remember or forget has both pros and cons.
TCP is technically a stateful protocol. It maintains an ongoing relationship between the sender and the receiver. With connection-oriented 3-way handshake formation, it embraces the condition of each interaction within a session.
Let's dissect the core reasons behind considering TCP a stateful protocol:
Firstly,
TCP
employs a mechanism known as a 'three-way handshake' to establish connections. Here’s how it goes:
- The initiating device sends a SYN (synchronise) packet.
- In response, the receiving device replies with a SYN-ACK (synchronise-acknowledgement) packet.
- Finally, the initiator sends back an ACK (acknowledgement) packet, marking a successful session establishment.
This process conceives a 'state' for the interaction between the entities involved. Therefore, every packet exchange in that particular session will refer to this state.
Secondly,
TCP
utilizes sequencing and acknowledgments to ensure reliable delivery. Upon transmitting data packets, it anticipates corresponding acknowledgment (ACK) packets from the receiving end. If not received within a specific timeout, it re-transmits the packets, eventually keeping track of the packets which have been acknowledged.
Furthermore, error-checking mechanisms and flow control make
TCP
even more stateful. Error-checking involves including a checksum for each packet, which facilitates detecting changes to the data while in transit. On the other hand, flow control prevents the sender from overwhelming the receiver with packets faster than it can process them.
Here is a simplified representation of how these TCP mechanisms work together:
Entity A Or Initiator (Sender) | Entity B Or Receiver (User) |
---|---|
Sends SYN packet & Wait for SYN-ACK | Receives SYN packet & Responds with SYN-ACK |
Sends an ACK to confirm connection & Starts sending data packets | Receives ACK and then waits for data packets to arrive |
Waits for corresponding ACKs to data packets | Upon receipt of data packets, sends ACKs in return |
If no ACK received within timeout, resends packet |
To get further into details about the Internet Protocol Suite with specific emphasis on Connection Management, check out Wikipedia's IP Protocol Suit.
TCP does an excellent job remembering each action occurred within the transaction creating a stable information pipeline. However, there needs to be careful capacity planning due to its high resource consumption. Given its stateful nature, TCP ensures there is a properly established routing path before any data transmission ensues, ensuring that the delivered packages are correctly sequenced and free from errors for a fruitful and comprehensive end-user experience.
Therefore, without any shadow of a doubt, we can agree upon considering TCP a stateful protocol!
We've touched upon numerous significant features like three-way handshake, sequencing, acknowledgment, error-checking, and flow control—all constituting an information-rich 'state' during a TCP session. No wonder it is the go-to protocol where data reliability triumphs over time-sensitivity!
So next time when you ping your friend (or maybe a server, who knows!), think about all the actions happening under the hood!TCP, or Transmission Control Protocol, is a stateful protocol. What does this mean? Well, it means that TCP retains memory of what has occurred in the past. This retained memory plays a crucial role in enabling two distinctive features of TCP - reliability and ordered data delivery.
1. Reliability
TCP utilises a mechanism called Positive Acknowledgement with Retransmission (PAR) to ensure reliable transfer of data packets between network nodes. The sender retains copies of all transmitted segments until an acknowledgement (ACK) for each is received from the receiver. In case a timeout event occurs before an ACK arrives for a particular segment, the corresponding data is retransmitted1.
This implies storing information about each packet, including whether or not an acknowledgement has been received. Hence, the protocol can't be stateless as it needs to keep track of the packets. Illustrative code snippet would look something like:
if (!packet.isAcknowledged()) { resend(packet); }
2. Ordered Data Delivery
TCP assigns a unique sequence number to every byte of data. These sequence numbers ensure that the receiver processes incoming bytes in the exact order in which they were sent by the transmitter. Even if packets arrive out of order at the destination, TCP re-arranges them to match their original sequence.
Evidently, such operation requires the protocol to 'remember' the initial sequence number and how many bytes have been sent. It has to constantly relate new packets and their sequence numbers back to that initial state. For example, in a code perspective:
sequenceNumber = initialSequenceNumber + bytesSent; bytesSent += packet.size();
To summarize, the stateful nature of TCP enables it to guarantee both reliability and ordered delivery of packets during transmission. Hence, while handling multiple transactions between different network nodes, TCP maintains separate states for each connection in what we refer to as 'the Connection State'. Each connection state includes various details like IP addresses, ports, sequence numbers, etc., making TCP a robust, reliable, and efficient protocol for data communication over networks.
References
Title | Definition of TCP |
---|---|
Source | TechTarget |
Sure, I can most certainly delve deeper into the error detection mechanisms within Transmission Control Protocol (TCP), while also touching on whether TCP is a stateless or stateful protocol.
Error Detection Mechanisms in TCP
TCP utilizes series of error detection mechanisms to ensure transmitted data is reliable. These mechanisms broadly involve the following key components:
Check Summing:
Coined as one of the première defenses against corrupt data during transmission, it involves each TCP packet incorporating a checksum. This value is calculated from the said packet's contents and then cross-checked by the recipient. Any discrepancies here trigger an error notification.
// Pseudocode for TCP Checksum function calculateChecksum(packet) { var sum = 0; // Iterate through all the bytes in the packet for (var i = 0; i < packet.length; i++) { sum += packet[i]; } // Return the negation of the sum, as described in RFC 793 return ~sum; }
Sequence Numbering:
With this mechanism, every byte of data gets assigned a distinct sequence number. The recipient device then reports back the sequence number of the last byte received without errors, facilitating TCP to keep track of transmission progress.
// Pseudocode for TCP Sequence Numbering function sendPacket(seqNum, packet) { // Send packet includes sequence number sendToNetworkLayer(seqNum, packet); } function receiveAck(ackSeqNum) { // Process acknowledgement with corresponding sequence number processAckNumber(ackSeqNum); }
Acknowledgement System:
Upon receiving a data segment, the recipient sends an acknowledgment back. Thus, if an error slips past the initial checksum test, or incase a segment was lost entirely during transmission, the sender will eventually become aware.
// Pseudocode TCP Acknowledgement system function receivePacket(packet) { // After successful receipt- acknowledge sendAck(packet.seqNum+packet.length); }
Retransmission:
In situations where the sending device does not receive an acknowledgment within a stipulated time-frame, the unreceived segment will be retransmitted. It avoids indefinite waiting and ensures delivery of each segment.
// Pseudocode for Retransmission Mechanism function timeout(seqNum) { // Resend packet if time-out happened resendPacket(seqNum); }
Now that we have a firm understanding of these processing components, let's examine if TCP fits the depiction of 'stateless' or 'stateful'.
TCP: A Stateless or Stateful Protocol?
Despite HTTP (Hyper Text Transfer Protocol) famously being known as a stateless protocol, its underlying transport layer protocol TCP is quite conversely- stateful.
Being stateful implies that TCP maintains a record of the associated connections. It tracks the status, activity, and related information regarding each communication happening over it. From sequence numbers assigned to each byte of data to delivering segments only after accomplishment of a three-way handshake protocol, everything shows that TCP retains connection-related states and information. Hence, making it- a Stateful Protocol.
Referencing RFC 793, TCP’s role as a virtual circuit between the communicating programs guarantees to deliver the data in order and uncorrupted.
If you wish to learn more about the intricacies of TCP functionality, please follow this TCP Explained Comprehensive Guide.Acknowledgment packets are a crucial part of the Transmission Control Protocol (TCP), playing a key role in maintaining the stateful nature of TCP.
We hear terms like 'stateless' and 'stateful' frequently used in network communication protocols. What does it mean for a protocol to be stateful? A stateful protocol, unlike a stateless one, keeps track of our connection status with the network. It recalls prior interactions and utilizes this information to determine an appropriate action based on the present situation.
Let's deconstruct how TCP, being a stateful protocol, utilizes acknowledgment packets.
Every time data is sent over a network using TCP, the sender awaits an 'acknowledgment packet', confirming that the recipient received the transmitted data. This constant back-and-forth exchange tracking is foundational to the functioning of TCP and cements its identity as a stateful protocol.
This concept is best understood through an analogy: Let's imagine sending a parcel via postal mail. You are the sender, TCP is the courier service and the receiver is the receiving host. Once you hand the parcel over to the courier office, they don't immediately forget about the parcel; instead, they keep track of it until it's successfully delivered to the receiver. Similarly, TCP keeps track of the data packages until they reach their destination. If the receiving host fails to acknowledge the receipt of data (like the recipient failing to sign upon receiving the courier), TCP resends the data – ensuring accurate data transfer.
The process of acknowledgement in TCP can be summarized in four key points:
- The sending device transmits the data along with a send sequence number.
- The receiving device gets this data and sends back an acknowledgment packet with the next expected sequence number.
- If the acknowledgments received by the sender match the expected sequence numbers, the data exchange continues normally.
- But if the acknowledgment does not arrive within a stipulated time frame ('Retransmission Time Out' or RTO) or unexpected sequence numbers are received, the missing or improperly sequenced data packets are retransmitted.
In HTML code, the flow can be referenced as follows:
Send(DataPacket, SequenceNumber) Receive(DataPacket) Send(AcknowledgementPacket, NextSequenceNumber) If Timeout OR UnexpectedSequenceNumber: Resend(DataPacket, SequenceNumber) Else: Continue
That’s the nutshell of acknowledgment packets within the scope of TCP. Just as the courier service doesn't forget the parcels handed over to them for delivery, TCP maintains a record of all current connections, enabling reliable and ordered data transmission, solidifying its position as a stateful protocol. For further reading, I recommend RFC 793 which provides in-depth detail about the TCP and the use of Acknowledgment Packets.Certainly, let's take a deeper dive into communication protocols and specifically the transition from a stateless to a stateful model. The context of this discussion is in relation to TCP (Transmission Control Protocol), a fundamental protocol within the suite of Internet Protocols that govern online communications.
In essence, whether a protocol is classified as stateless or stateful has everything to do with how it manages information.
A stateless protocol, like HTTP, does not maintain any knowledge of past requests. Each transaction is processed independently without any memory of previous transactions. This comes with several advantages:
- Scalability: Because each request is treated separately, servers can handle more simultaneously active clients.
- Simplicity: Stateless protocols are generally easier to implement and understand, and there's less complexity in managing connections.
- Fault tolerance: In a stateless model, if something goes wrong, the process can pick up where it left off in the next request.
On the contrary, a stateful protocol like TCP maintains data about each client during the course of the session, keeping track of all pending operations, past resource associations, and other specific details. Such characteristics offer its own set of benefits:
- Reliability: Packets arrive reliably, in-order, and error-checked due to sequencing and acknowledgement features.
- Flow-control: It prevents network congestion by ensuring sender doesn't transmit data faster than receiver can consume.
- Connection-oriented: Before data transmission, it sets up a proper channel between sender and receiver, which further ensures reliability.
The transition from a stateless model to stateful for communication protocols such as TCP brings a significant changes regarding reliability and orderly delivery of packets.
Stateless Model: Request --> Server --> Response Stateful Model (TCP):Connection Setup --> Data Transmission --> Connection Preamble
Stateless | Stateful (TCP) | |
---|---|---|
Reliability | Low as no acknowledgement | High as packets acknowledged |
Orderly Delivery | No guarantee | Guaranteed |
Congestion Control | No flow control mechanisms | Implemented |
So answering your question, TCP is absolutely a stateful protocol. It maintains the state of each connection during a conversation because it must ensure that packets are delivered to the intended recipient in the correct order without errors. As a result, if we compare it to stateless protocols, TCP offers excellent data integrity and reliable delivery of packages.
However, though TCP provides a more robust solution for data packet delivery compared to stateless protocols, we should remember that the stateful nature of TCP might make it less scalable and slower over high latency networks because of the need to establish connections and wait for acknowledgements before sending more data.
For a deeper understanding, you can also refer to insightful resources like this comparison guide on different protocols, diving into further specifics of TCP and UDP (User Datagram Protocol - another primary protocol in the IP suite).
Delving into the crux of the matter, TCP stands as a stateful protocol due to its ability to keep track of the data flow during a communication session between systems. When asked about whether TCP is stateless or stateful, important considerations include:
TCP establishing a connection before data transmission TCP tracking the state of transmitted data TCP's use of sequence numbers to order data packets
Firstly, TCP initiates a three-way handshake which establishes and then maintains a connection until the conversation between sender and receiver is complete. This aspect denotes it as a stateful protocol because a specific state (connection established, data transmitted, or connection closed) is maintained throughout the session.
The next rating element is packet tracking. During transmission, TCP keeps a record of packets sent, received, and those that need retransmission due to occurrence of errors. As a result, any lost or distorted data is replenished effectively, reinstating TCP's designation as a stateful protocol.
Furthermore, TCP employs sequence numbers to maintain the orderliness of data packets. The protocol ensures that data is not only delivered but arranged appropriately at the receiving end, regardless of their dispatch order. So, the reliable and orderly way of handling data backs up the claim of TCP being a statefully structured protocol.
Among other vital features of TCP like error checking, flow control, and congestion avoidance strategies, all these facilitate in maintaining an uninterrupted and well-managed communication channel, testifying TCP's qualifications as a stateful protocol.
To understand more deeply, this RFC document on TCP from IETF can provide comprehensive knowledge about how TCP operates.
Overall, from setting up connections rigorously, recovering lost data, and keeping a strict eye on sequence numbers till the proper termination of a session, TCP manifests all traits of a stateful mechanism and confirms a steady and error-free data transmission. However, the use of TCP or any stateful or stateless protocol entirely depends upon the requirements of your application. Various protocols come with different benefits and your choice should align with your specific communication demands.
Protocol Type | Traits | Example |
---|---|---|
Stateful | Maintain connection state, keep track of packet data | TCP |
Stateless | No track of preceding interactions, independent requests treated separately | HTTP |