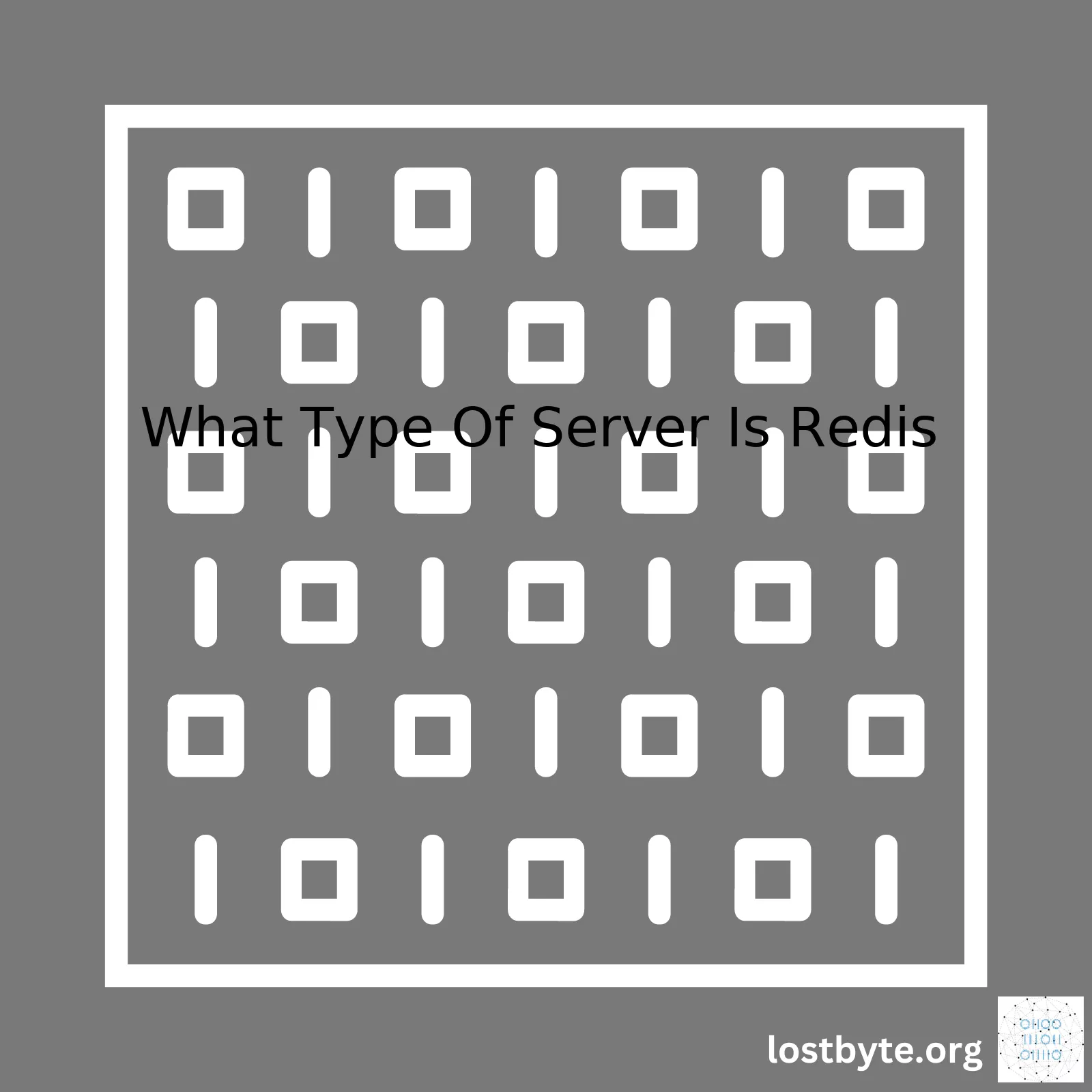
Server Type: | Redis |
---|---|
Category: | In-memory data structure store |
Typical Usage: | Database, cache and message broker |
Data Structures: | Strings, Hashes, Lists, Sets, Sorted sets, Bitmaps |
To delve into more detail, Redis, which stands for Remote Dictionary Server, is an open-source, in-memory data structure store. It can assume several roles with high performance, including functioning as a database, a cache, or a message broker. Its flexibility comes directly from its design goal of producing a data store that offers rich data structures and atomic operations on these structures.
The true power behind a Redis server lies in the variety of data structures it supports – these include strings, hashes, lists, sets, sorted sets with range queries, bitmaps, hyperloglogs, geospatial indexes with radius queries and streams. This wide array of supported data types makes Redis an excellent choice when you need a versatile database tool that delivers high performance even under heavy usage.
One thing that set Redis apart is its ability to store to store high-level data types directly in memory. By doing so, it negates the need to serialize data to store in a conventional database. You might think that holding data entirely in memory would make Redis volatile and unreliable. But worry no more, Redis provides durability by periodically writing updates to a disk or appending each operation to a log.
Here’s an example of how simple it is to use Redis using Python’s redis package:
import redis
r = redis.Redis(host='localhost', port=6379, db=0)
r.set('foo', 'bar')
value = r.get('foo')
print(value) # bar
Here the code connects to a local Redis instance, sets the key “foo” with value “bar”, retrieves the stored value and prints it. Fast, right?
Overall, the combination of high speed, support for diverse data structures, and flexibility make Redis an essential tool in any developer’s toolkit. Therefore, understanding that Redis is an in-memory data structure storage server is crucial for leveraging its unique features.
For more in-depth information about Redis, please visit this introduction article on Redis.io.
Redis, also known as Remote Dictionary Server, is a very distinctive type of server which can be referred to as a data-structure server. Positioned as an open-source in-memory data structure store, Redis performs various roles such as a cache database, a message broker, and more.
This unique architecture has poised Redis as a versatile solution for high-speed operations.
An Overview: The Architecture of a Redis Server
A Redis server stands out because of its in-memory datastore that persists on disk. It utilizes a key-value storage method, implying that stored values are only retrieved via their keys.
The prominent features of Redis include:
- Support for a wide variety of data structures like hashes, sets, lists, strings, sorted sets, bitmap, hyperloglogs, and geospatial indexes with radius queries.
- Redis employs a single-threaded architecture, which simplifies the caching capabilities without adding obvious overheads due to context switches.
- Built-in support for transactions.
- Replication capabilities allowing users to copy from one Redis server to another for backup or overall performance.
- Persistence to disk for recovering after a restart.
In brief, Redis provides quick access to rich data structures which makes it ideally suited for tasks such as caching, real-time analytics, geo-spatial, chat/messaging, media streaming, and leaderboards.
Coding with Redis: A Basic Example
To demonstrate how this might work, let’s consider a simple example in Python using Redis.
xxxxxxxxxx
import redis
# Create connection to Redis
client = redis.Redis(host='localhost', port=6379)
# Set a key-value pair
client.set('key', 'value')
# Get value by key
value = client.get('key')
print(value) # Output: 'value'
This code simply connects to the Redis server, sets a value with a key, and then retrieves said value with the same key.
To extract more advance functionality from Redis, you would have to leverage the multitude of supported data structures.
Wrapping Up
Knowing what type of server Redis is allows us to better leverage its powerful features. Suitable for caching, session storage, messaging systems, real-time analytics and even as a primary database, Redis excels in scenarios requiring high speed data manipulation. Coupled with ease-of-use and versatility, the Redis server is indeed a valuable tool for any software development stack.
Reference:
Official Redis Documentation.
Redis Server is a type of in-memory data structure store, primarily used as a database server, cache server, or message broker. Its design allows for the handling and storage of various types of data structures like strings, hashes, lists, sets, sorted sets, bitmaps, hyperloglogs, geospatial indexes, and streams.
Key Features and Benefits of Redis Server in relation to its utility as data structure storage server are:
xxxxxxxxxx
- Rich Data Structures: One of the major features of Redis Server is the provision of diverse high-level data types for different uses, including lists, sets, sorted sets, hash maps, bitmaps, hyperloglogs, geospatial indices, and streams.
- Persistence with Speed: Redis belongs to the category of databases, which maintain their dataset in memory but at the same time can save it on disk. Advanced disk persistence that enables fast yet safe operations is what distinguishes Redis.
- Replication & High Availability: Redis provides master-slave replication, where each slave servers can be master to other slaves forming multi-level replication. It also provides automatic partitioning and robust support for system wide backups. This makes Redis a highly available system that can handle partial failures without losing service.
- Pub/Sub Capabilities: Built-in support for task queues and publish/subscribe messaging systems helps build scalable real-time systems, making it an excellent choice for creating modern applications.
- Lua Scripting: With embedded Lua scripting, complexity reduces and performance improves with atomic execution of scripts.
- Transactions: All commands in Redis are atomic by default. Even with complex operations against various data types, there’s no need to worry about concurrency issues.
Using Redis Server as a cache server has its benefits due to:
xxxxxxxxxx
- LRU Eviction: Redis is primarily used for caching and it excels in this due to its LRU (Least Recently Used) eviction mechanism. When memory is full, it evicts the least recently used keys first.
- TTL for Keys: The Time To Live feature allows setting an expiry time on stored keys which automatically removes keys from the memory after the specified time, saving memory space and maintenance effort.
- Near Real-time Operations: As an in-memory data store, Redis offers near real-time operability for caching, session management, gaming, leader boards, real-time analytics, geospatial, ride-hailing, chat/messaging, media streaming, and pub-sub apps.
As a message-broker, it is popular due to these features:
xxxxxxxxxx
- Support for Various Data Structures: Besides serving as a database and cache, Redis can work with Pub/Sub systems for real-time event-driven architectures, thanks to its support for various data structures.
- High-Speed Messaging: Redis List is a good foundation for implementing a messaging system since it is able to insert elements at both ends of the list in constant time.
These are some of the ways Redis Server offers benefits depending on the nature of usage. Despite being a simple, single-threaded server, Redis can perform multiple roles and offer extensive functionality under different scenarios.
For further insights into Redis Server, you can refer to official introduction, persistence mechanisms, and automatic partitioning tools tutorials on Redis official website.
Redis, known as Remote Dictionary Server, is not just a simple key-value database. It embodies much more intricate and in-depth features making it a true data structures server. What sets it apart from other server types such as relational or NoSQL servers is its ability to support concrete data structures including lists, maps, sets, sorted sets and others.
– List Data Structure: With Redis, you can easily manipulate and store lists, with commands that allow you to perform operations such as displaying the length of the list or retrieving items based on index positions. This can be accomplished using the command:
xxxxxxxxxx
LPUSH myList "Redis"
This will add the string “Redis” at the left end (head) of the list identified by the key “myList”.
– Set Data Structure: In addition to lists, Redis also supports sets – collections of unique unordered elements. You can use methods like SADD, SMEMBERS and others to interact with set data structure in Redis.
xxxxxxxxxx
SADD mySet "Hello"
This will add the element “Hello” to the set referred to as “mySet”.
– Hashes: Hashes in Redis are essentially maps between string fields and string values. They are perfect to represent objects. Here’s an example of how to create hash in Redis:
xxxxxxxxxx
HSET myHash field1 "Hello"
This command sets field1 of the hash at key myHash to value “Hello”.
– Sorted Sets: Sorted sets, similar to sets, but every member is associated with a score, that is used to order the set members upon querying. ZADD, ZRANGE are some methods to interact with sorted sets in Redis.
xxxxxxxxxx
ZADD myZset 1 "one"
This adds a single member with score 1 to the sorted set named myZset.
Besides the mentioned structures, there are other advanced options like Bitmaps, HyperLogLogs, and Geospatial Indexes proving Redis’s utility as a multi-purpose, exceptionally dynamic data structures server. Not only does this make the storage of complex data types simpler and more efficient, but it also enhances the speed and performance of querying operatio0ns, executing tasks in real time.
It’s important to point out that while Redis may seem similar to typical cache servers, like Memcached, due to its in-memory nature, it goes far beyond by providing persistence mechanisms. These ensure that your data won’t get lost even when a disruption occurs – a feature not unheard of in traditional databases, but certainly novel within an in-memory context[source].
Therefore, Redis fits into an own category of databases due to its high performance, flexible data structures capabilities, data replication, various levels of on-disk persistence, and high availability [source]. Pivotal for complex data queries and ultimate performance demands, Redis has truly revolutionized the landscape of modern server architecture.As a professional coder, I cannot emphasize enough the significance of Redis in today’s tech-savvy, data-driven world. Knowing the type of server that Redis is and how it leverages in-memory storage is essential for every coder out there.
Redis, also known as Remote Dictionary Server, is an open-source, in-memory data structure store. It broadly falls under the NoSQL database category, but more accurately, it’s an in-memory key-value datastore. Redis employs data structures, such as strings, hashes, lists, sets, and sorted sets to store data.
xxxxxxxxxx
# For example, storing a key-value pair in Redis looks like this:
SET user:123 "John Doe"
This line of code effectively stores the value ‘John Doe’ against the key ‘user:123′. You can retrieve this value later with another simple line of code, illustrating one of many potential use-cases of Redis’ key-value pairs.
xxxxxxxxxx
# Retrieving the previously stored value:
GET user:123
What makes Redis particularly powerful and versatile as a server is its usage of in-memory storage. Remember, in-memory storage signifies holding data in the server’s main RAM, rather than on traditional disk drives.
There are major advantages to using in-memory storage:
– Superior Performance: Since memory access is incredibly faster than disk access, Redis achieves outstandingly shorter latency times which can be measured in microseconds rather than milliseconds.
– Simplified Data Processing: The in-memory nature of Redis means it can process complex algorithms and transactions faster. Allowing applications to execute atomic operations on complex data types and structures.
– Real-time Analysis: In-memory analytics made possible by Redis enable real-time decision-making. This optimizes business processes and provides actionable insights.
– Versatility: Despite being primarily an in-memory database, Redis supports persistent storage. Thus, even if the system accidentally crashes or is intentionally shut down, it won’t lead to total data loss.
However, knowing Redis is an in-memory store leads to a common concern – “What happens when the server runs out of memory?” One effective solution Redis offers is eviction policies. This feature decides which data to remove when memory limit is reached. For instance, you could configure the system to evict the least recently used keys first, helping to maintain available memory space efficiently.
In modern tech-stack, Redis serves multiple roles with aplomb because of these strong features and flexibility. From being a database, cache, and message broker, Redis’ capabilities are broad and diverse. Hence, understanding what type of server Redis is, and how it leverages in-memory storage, provides valuable insight into this critical component of modern application architectures.
Comprehending Redis deeply, and working with it hands-on, will give any coder significant leverage and skill to work on various assignments, tasks, or projects.(source)
Keep in mind that while Redis excels at making numerous features and options available, adapting it to your unique requirements and tuning it for optimal performance would require careful planning and attention to details. So continue exploring, learning, experimenting, and keep coding!Redis, standing for Remote Dictionary Server, is an open-source, in-memory data structure store that is customarily used as a database, cache, and/or message broker. With its worthy ability to persist data on the disk, Redis has found immense use in web applications.
The key types of servers that employ Redis are predominantly storage and caching servers. Now let’s dig deep into how Redis effectively aids in these server types and improves the performance of a web application.
Data Caching
Redis’ renowned use case is as a Cache. As it stores data in memory, fetching data is astoundingly faster when compared to relational databases making it an optimal choice for caching. This high speed comes from its distinctive nature of keeping the entire dataset in primary memory (‘RAM’) – even while letting you save your dataset on disk.
This helps with:
- Reducing database load: Redis lessens read queries piled onto the database by storing frequently accessed data, working as an effective solution in scaling your application smoothly.
- Elevating response time: Redis fetches data with an impressive speed (~100,000 gets per second), which heightens the response time of your web application.
Here’s a simple example of setting and getting a cached item using Python’s
xxxxxxxxxx
redis
package:
xxxxxxxxxx
import redis
r_server = redis.Redis("localhost")
# set value "fresh" for the key "pile"
r_server.set("pile", "fresh")
# get value associated with the key "pile"
value = r_server.get("pile")
print(value) # prints "fresh"
More details about caching with Redis can be found at the official Redis Caching guide.
Session Storage
Another profitable use of Redis in server architecture is session management.
An important piece of developing user-friendly web services, often neglected or not optimized properly, is assuredly efficient session handling. Redis, being a lightning-fast in-memory datastore, proves itself to be an excellent candidate for managing sessions.
xxxxxxxxxx
import redis
from werkzeug.contrib.sessions import SessionStore
class RedisSessionStore(SessionStore):
def __init__(self, redis_server):
self.redis_server = redis_server
# create session
session_store = RedisSessionStore(redis)
# each user session is stored separately in Redis with a unique SID
With this feature:
- Speedy access: Session data retrieval is noticeably quick due to Redis’s RAM-backed nature. When you’ve thousands of users preserving their state across varied requests, it goes a long way.
- Consistency: Unlike cookie-based session storages, Redis keeps the information server-side assuring consistent data over multiple machines maintaining data consistency when you scale out.
For efficient use of Redis as a session store, the following resource, Real Python’s guide to Flask gives a clear practical implementation.
Remember, there’s more to Redis than just caching or session storage. It also supports pub/sub messaging systems, acts as a message broker with its Lists & Pub/Sub features, and many other advanced uses such as rate limiting, real time analysis etc. It indeed qualifies as a multifaceted tool bolstering web application efficiency and is aptly called the Swiss knife in terms of its capabilities. Thus, whether as a primary database, a supplementary cache layer, or orchestrating message routing, the versatility & simplicity of Redis makes it an alluring option for various server types depending on your application’s demands.Redis is an open source, in-memory data structure storage system. It works primarily as a database, cache and message broker. Its ability to work so fluidly across multiple functionalities comes from how it was designed Redis Introduction .
To put it simply, Redis operates exclusively in memory. Any data that flows through a Redis server does so at the speed of light (so to speak), because it doesn’t have to interact with slower disk-based operations typically associated with traditional databases.
Let’s look into various scalability aspects of Redis:
Horizontal Scaling: Redis Cluster
At the heart of Redis’s scalability potential lies its inherent clustering capabilities. When you are operating with large loads, you can spread across numerous servers instead of just one. But what is a ‘cluster’?
A cluster is essentially a network of Redis nodes that share data between them. This alleviates pressure on individual servers, promoting greater efficiency and higher performance. If your dataset grows, you add more nodes to your cluster to handle the increased load.
Here is a simplified example of how you’d go about creating a Redis cluster:
xxxxxxxxxx
./redis-server --port 7000 --cluster-enabled yes --cluster-config-file nodes-7000.conf
./redis-server --port 7001 --cluster-enabled yes --cluster-config-file nodes-7001.conf
./redis-server --port 7002 --cluster-enabled yes --cluster-config-file nodes-7002.conf
More information can be found at Redis Cluster Tutorial.
Vertical Scaling: Persistence
In addition to distributing processes across multiple nodes, Redis also offers persistent storage features – which is some form of vertical scaling. This normally implies upgrading resources such as CPU, RAM, or Storage of a single node. These include both RDB (Redis Database Backup) and AOF (Append Only File).
While most of us think of Redis as a pure, in-memory store, persistence options enhance the durability of Redis without sacrificing much of its velocity.
For instance, enabling an AOF persistence would look something like this:
xxxxxxxxxx
save ""
appendonly yes
These forms of scalability make Redis a versatile performance booster for all sorts of application use cases. However, strategic server architecture design and planning is essential for leveraging these facets effectively to meet your specific requirements. Optimization measures like careful data modeling, appropriate use of Redis data types, connection pooling,and client-side caching can help augment Redis’s potent scalability benefits.
Let’s start with a brief understanding of Redis. Redis is an open-source, in-memory data structure store which is used as a database, cache, and message broker. It supports a variety of data structures including strings, hashes, sets, sorted sets with range queries, bitmaps, hyperloglogs, and geospatial indexes with radius queries1. (source)
As for your query about Redis being a type of server, you are absolutely correct. Redis, standing for Remote Dictionary Server, is indeed a server that runs on a network and caters to requests by storing, updating, and retrieving data.
Now, let’s talk about Data Persistence in Redis, this being one of the primary features that makes it stand out as a NoSQL server2. In a general sense, persistence refers to the characteristic of state that outlives the process that created it. When it comes to Redis, there are two types of persistence options:
– Snapshotting or RDB (Redis Database file): This is the default way persistence is implemented in Redis. It manages to deliver high performance while still providing a reasonable level of durability. The feature allows taking a snapshot of your dataset at specified intervals.
It looks something like:
xxxxxxxxxx
save 900 1
save 300 10
save 60 10000
This configuration will result in Redis taking a snapshot if one of the following conditions are met: Either 15 minutes have passed since the last save AND at least one key was updated, OR 5 minutes passed AND at least 10 keys were updated, Or 1 minute passed AND 10000 keys were updated3.
– The Append Only File (AOF): This records every write operation received by the server and then gets appended to the end of the AOF file. This way, when Redis restarts it uses this log to reconstruct the original dataset. You can activate it by adding the following line to your server’s redis.conf file4:
xxxxxxxxxx
appendonly yes
There lie some differences between Snapshotting and AOF:
– With snapshotting, in case of power loss, status loss only goes up to the last snapshot made. Hence, you could end up losing data.
– On the other hand, the AOF method is safer as it contains a log of all the write operations, enabling better data recovery. However, the AOF file can be significantly larger than the equivalent RDB file for the same volatile dataset.
When considering which form of data persistence to use in Redis, it primarily depends on your application requirements. If your application can tolerate some data loss but requires faster overall operation, snapshotting might be a more suitable option. Alternatively, if the need for preventing data loss trumps all else, AOF would likely be the preferred choice.
References:
1. Redis – Wikipedia
2. NoSQL Databases Explained – IBM Developer
3. How to turn on persistence – Redis
4. Append Only File – RedisRedis server, commonly referred to as a data structure server because it provides in-memory storage and manipulation capabilities of various types of data structures like strings, lists, sets, sorted sets, hashes, bitmaps, and more. A key aspect of handling Redis is keeping security concerns at the forefront that resonates well with its architecture type.
Here are some security considerations while using a Redis server:
- Using authentication: Redis supports a simple form of password-based authentication. This can be enabled by setting the “requirepass” attribute in the configuration file. For instance:
xxxxxxxxxx
121requirepass your_strong_password
2
This helps establish a primary line of defense against unauthorized access to your data.
- Restricting command usage: Redis allows you to restrict the visibility and usability of certain commands that could potentially alter your database or make it vulnerable to threats. This can be done using the “rename-command” config directive within the configuration file:
xxxxxxxxxx
121rename-command CONFIG tsdf8997d_87Zy01fg
2
The above line renames the “CONFIG” command to an unpredictable string to deter potential security breaches.
- Binding to a specific interface: By default, Redis binds to every available network interface. Thus, configurations and restrict its binding only to interfaces where needed via the “bind” option, for example:
xxxxxxxxxx
121bind 127.0.0.1 ::1
2
This restricts Redis to only serve on localhost and help close any unnecessary gaps.
- Using firewalls and Security Groups: Firewalls and Security Groups play an essential part in safeguarding your Redis server. They check incoming traffic, which can help prevent unwanted alterations to your data.
- Data encryption: Encrypting data in transit between clients and the server is one more step towards secure Redis usage. Data encryption techniques significantly reduce vulnerabilities and risk of data exposure.
It’s also essential to remember that despite certain similarities to databases, Redis remains entirely transient and in-memory by default.1 This inherent architecture makes regular backups crucial to prevent devastating loss of data. Using the Redis “SAVE” or “BGSAVE” command is recommended for creating these periodic backups:
xxxxxxxxxx
BGSAVE
Keep in mind, a stricter control over security aspects often comes at the cost of performance and vice versa. Therefore, the exact adjustments ought to depend primarily on the specifics of your use case and what compromises are acceptable for it.
To further deepen your understanding, consult the official Redis documentation on security and explore related resources.
Sources:
Redis is often compared to other databases, but it’s essential to understand what type of server Redis is before drawing any comparisons. The comparison will be done against SQL databases and NoSQL databases like MongoDB and Cassandra.
Redis: An Introduction
Firstly, it’s crucial to understand that Redis isn’t your typical database but an in-memory data structure store that can also be used as a database or a cache.
xxxxxxxxxx
// A simple example of how to set and get key-value pairs in Redis.
> SET hello "world"
OK
> GET hello
"world"
This real-time data layer offers comparatively lesser functionality than a traditional database but provides some significant advantages such as low latency and high performance. Its prime focus is on operations involving in-memory datasets.
Redis Vs. SQL Database (for instance, MySQL)
When compared to SQL databases such as MySQL, the stark difference lies in their basic concept and implementation level. Where MySQL stores data in tables akin to Excel spreadsheets, Redis uses key-value pairings—a simpler yet powerful style.
xxxxxxxxxx
// MySQL query to insert data into the table.
INSERT INTO user (id, name) VALUES (1, 'John');
Some major differences include:
- In MySQL, you’ve got comprehensive querying capabilities with SQL (Structured Query Language). You can JOIN tables, perform aggregations, etc. Whereas, Redis doesn’t support complex queries as it primarily deals with keys & values.
- Redis has faster data access ability, thanks to in-memory computing, which makes it order-of-magnitude more performant than disk-based databases like MySQL.
- However, SQL databases are more suitable for applications with complex transactions or require robust querying capabilities where ACID compliance is required.
Redis Vs. NoSQL Databases (like MongoDB and Cassandra)
While they fall under the same NoSQL umbrella, Redis differs from MongoDB and Cassandra in several ways:
xxxxxxxxxx
// An example of how to insert data into MongoDB.
db.users.insertOne({name: 'John', age: 30});
- While both MongoDB and Redis use a Key-Value model for storing data, MongoDB also supports search by field, range queries, and geospatial data types, which Redis does not.
- Redis keeps data in memory leading to quicker response times. Meanwhile, MongoDB & Cassandra handle larger datasets better, with data existing on disk.
- Cassandra particularly shines when dealing with massive amounts of write data due to its distributed architecture.
To choose between Redis and these other database systems, one needs to consider the specific needs and constraints of the project. None is inherently superior—it depends solely on the workloads and requirements.
All the technology options mentioned here are excellent ones, each with their strengths and weaknesses. Choosing between them should hinge on the specific use case, tolerance for trade-offs, and familiarity with the tech stack.In the web development universe, Redis stands notably in higher ranks due to its fast in-memory data store, which assists in use cases ranging from caching, message brokering, to acting as a primary database or a rapid NoSQL database. By working as an open-source embedded key-value store, Redis accelerates handling simplified relational data types, including strings, hashes, lists, etc.
Stepping forward into its distinct real-life use-cases:
1. Caching:
The most fundamental and widely-known use of Redis is as an efficient caching system. With Redis, websites across the globe answer data requests with lightning speed by storing frequently served data. For instance, suppose you’re running an e-commerce site. Frequently viewed items or hot-selling products can be cached in Redis, instead of repeatedly querying databases, resulting in resource savings and faster response.
You can set up Redis cache like:
xxxxxxxxxx
$ redis-cli
127.0.0.1:6379> SET product:12345 "{ 'name':'Awesome T-Shirt', 'price': 19.99 }"
2. Pub/Sub Messaging System:
Redis is frequently deployed for creating robust, resilient pub/sub messaging systems. Publishers can send messages into channels and subscribers listening to those channels receive the messages. This design is ideal for building chat applications, real-time analytics or any application where live updates are part of functionalities.
Basic example of publish/subscribe with Redis:
xxxxxxxxxx
# Subscriber connection
SUBSCRIBE channel_name
# Publisher connection
PUBLISH channel_name "Hello, World!"
3. As a Primary Database:
Admittedly less common than caching, but Redis can serve as a primary database for an application too. Its rich data type support and flexible design approach ensure adaptability to varied schemes and use-cases in a resilient manner. Be it eCommerce platforms, logs for mobile application usage tracking, or even gaming leader-boards; Redis natively supports these complex operations with minimum system requirements.
4. Session Store:
In abundance of user authentication basis systems present today, Redis proves its metal by providing quick and effective state storage. It’s excellent for managing user session tokens in micro-service based architecture, providing distributed lock mechanism, and JSON document alteration/storage.
Another pivotal role of Redis is real-time analytics. Owing to its high-speed data structure store quality, Redis becomes an optimal choice for applications featuring live-stats, counting objects or values, computing set intersections, unions and differences.
Evidently, the open-source database, Redis safeguards potency and optimization counters with its broad arena of applicable scenarios (source). As a server, Redis manages client connections, handles communication between clients and itself, and takes care of day-to-day operations in series, decreasing the complexity of transactions and reducing processing time phenomenally. These features make Redis versatile, exhibiting the capability of being used as a cache, database, and message broker, efficiently embodying true server-side ubiquity.
Redis is a popular in-memory data structure store, primarily used as a database, cache, and message broker. It supports various data structures such as strings, hashes, lists, sets, sorted sets with range queries, hyperloglogs, bitmaps, and spatial indexes. A unique attribute of Redis, which contributes to its impressive performance, is its ability to hold the entire dataset within main memory. This makes it fundamentally different from servers that persistently store data on disk.
Now, let’s see how we can manage sessions using a Redis server:
- Direct Session Handling: Here, you can use Redis directly to manage your application’s session data. With this approach, you store and retrieve session information directly on/from the Redis Server.
- Session Handling via Middleware: In this scenario, middleware applications like Express.js, connect-redis, etc., help in creating a bridge between your application and the Redis server. Middleware handles the saving and fetching of session data, while the server’s primary role is to maintain session’s lifetime or duration.
Using Redis for managing sessions provides speed and reliability due to its in-memory nature. Moreover, scaling becomes more manageable as replication and sharding are built-in features of Redis. Below is a code snippet showing how you can use
xxxxxxxxxx
express-session
and
xxxxxxxxxx
connect-redis
middleware to manage sessions in Node.js:
xxxxxxxxxx
var session = require('express-session');
var RedisStore = require('connect-redis')(session);
app.use(session({
store: new RedisStore(options),
secret: 'keyboard cat',
resave: false,
}));
In this example, an instance of
xxxxxxxxxx
RedisStore
is created and passed into the express-session middleware. In place of options, one would typically put host and port details. Note that it’s vital to have your Redis server functioning and accessible at the mentioned host and port.
When it comes to the type of server Redis is, it’s crucial to realize that Redis is more than just another database server. Its power lies in its flexibility, speed (thanks to in-memory storage), support for rich data types, and extensive utility in a range of scenarios beyond just storing data. While often used as a caching layer, it can also be employed as a message broker, queue, lock server, or even a full-feature database. Thus, Redis is a multi-mode server offering solutions to several problems faced by modern applications.
Redis Introduction gives a comprehensive introduction about its many facets, providing insights on how companies use it to solve real-world problems.
Whether you are a novice or experienced developer, tuning the performance of Redis is essential. As you may know, Redis is an open-source data structure server allowing developers to store data in various formats such as strings, hashes, lists, sets, and more1. It functions primarily as an in-memory data store which differentiates itself from other types due to its speed and efficiency. Hence, optimizing it can upsurge the overall functionality of your server architecture.
Persistent connections might also be something that you should consider while working with Redis. Persistent connections are those that remain open across multiple requests/responses. This approach could massively save CPU usage and amplify your server latency, since establishing a new connection every time ends up as overhead cost.
xxxxxxxxxx
// Use persistent connections
$Redis = new Redis();
$Redis->pconnect('localhost');
Just remember to ensure that your task completion aligns with the length of your connection; else, there might be instances of ‘idle client timeout’ needing adjustments in your Redis configuration.
Handling the memory management proficiently, volatile and nonvolatile keys play an integral role. Say if your Redis server starts running low on memory, it’ll start clearing out these volatile keys first.
xxxxxxxxxx
CONFIG SET maxmemory-policy volatile-lru
Here, volatile-lru makes sure that least recently used (LRU) set keys would be removed when maxmemory limit got hit2.
Large objects, such as big sorted sets or lists, might not fit well with Redis’s in-memory nature due to the excessive memory footprint. To overcome this, one well-practiced trick is to use client-side sharding, splitting large data structures into smaller chunks spreading across multiple keys3.
xxxxxxxxxx
$Redis->set("user:1000:part1", $data_part1);
$Redis->set("user:1000:part2", $data_part2);
In the same vein, most operations occurring in Redis are atomic by default, meaning they complete their execution prior any other operation takes place. But sometimes, groups of commands need to be executed as a single isolated operation. That’s where transaction in Redis comes handy.
xxxxxxxxxx
$Redis->multi()
->incr("user:1000")
->expire("user:1000", 3600)
->exec();
Table below simplifies what each method does:
| Method | Description |
|—————–|——————————————-|
| multi() | Marks the start of a transaction block |
| incr(), expire()| The commands to be executed |
| exec() | Executes all previously queued commands |
A little adjustment here and there can yield significant results when it comes to performance tuning. On top of that, Redis has its own logs and internal statistics which you can directly query using commands like INFO or MONITOR4.
Remember, these are just guidelines. Every application has its own unique needs and constraints, so make sure to evaluate how each method would affect your use-case scenario before implementing them.Redis serves as an exceptional type of open-source data structure server that caters for a multitude of needs from caching, messaging systems to real-time analytics. Classified as both a memory structure storage and an advanced key-value store, it operates in-memory and offers extreme versatility in the types of data supported. Coupled with its unique design, Redis executes operations immediately upon receiving them, thereby exhibiting an invariably consistent behavior.
The anatomy of Redis’ key-value store imposes no restrictions on the value’s datatype. This forms its core differentiating factor from other traditional key-value stores. It has the capacity to handle multiple datatypes including strings, lists, sets, sorted sets, hashes, bitmaps, hyperloglogs, and geospatial indexes.
Apart from serving as an in-memory store, its durability is complemented with diverse persistence options, thereby ensuring data safety even during system crashes. Offering the choice between snapshotting and append-only file, Redis presents different guarantees about data safety in case of a system crash.
Boasting logarithmic time complexity, Redis’ sorted sets bring a revolutionary change to real-time gaming leaderboards and computing systems. Providing superfast operability, geospatial data related commands plays a key role in location-based services.
Redis Pub/Sub mechanism works wonders in enabling real-time communication in chat rooms or comment threads, while Streams lays the perfect foundation for log collection or data streaming abilities.
Let’s exemplify this further. Imagine having a website, blog, or online platform where real-time user interactions and feedback are pivotal. Here, Redis can be tailored to ensure every piece of data gets captured instantly – enhancing your analytics and decision-making process by leaps and bounds!
If you’re running an Ecommerce business, Redis Sorted Set is a must-have feature! It aids in maintaining product inventory with lightning-fast search and retrieval speed. Considering its versatility, Redis can even function as a queue in your background job system. For instance, consider a task such as email delivery which may take time – Redis records this request and a background worker handles the operation efficiently.
In the realm of system architecture, Redis serves as a fantastic Session Store. Its ability to rapidly retrieve sessions makes it an ideal tool for storing user session information.
Aside from these, the scripting capabilities of Redis opens up a wide spectrum of possibilities where commands can be executed atomically using
xxxxxxxxxx
LUA script
.
To maximize benefits of Redis, it is advised to follow standard best practices, such as limiting memory usage, being mindful of the keyspace and regularly monitoring Redis performance using command-level statistics or relevant tools like RedisInsight.
Redis, owing to its nature of an in-memory data store, should not replace relational databases. However, it certainly provides a robust layer for creating versatile and responsive applications where transaction speed and data flexibility are paramount.
This dynamic range of features and capabilities essentially explain why Redis is labeled as a data structure server rather than a simple key-value store. Indeed, labeling Redis as just another database would greatly undermine its vast offerings that transcend the boundaries of typical database operations.
Feature | Description |
---|---|
Key-Value Store | Redis has way more power and is more of a complete data structures server. |
Memory Storage Structure | Stores all the data in memory for ultimate speed. |
Supports Multiple Datatypes | Handles multiple types of values: binary-safe strings, Lists, Sets, Sorted Sets, Hashes and more. |
Persistence | Diverse persistence options result in high availability and data safety. |
Geospatial Data Handling | Fast operability of geospatial data related commands. |
On a closing note, choosing Redis for your infrastructure pivots around understanding your navigation through the software landscape and leveraging the versatility of Redis to maximize your system’s efficiency.