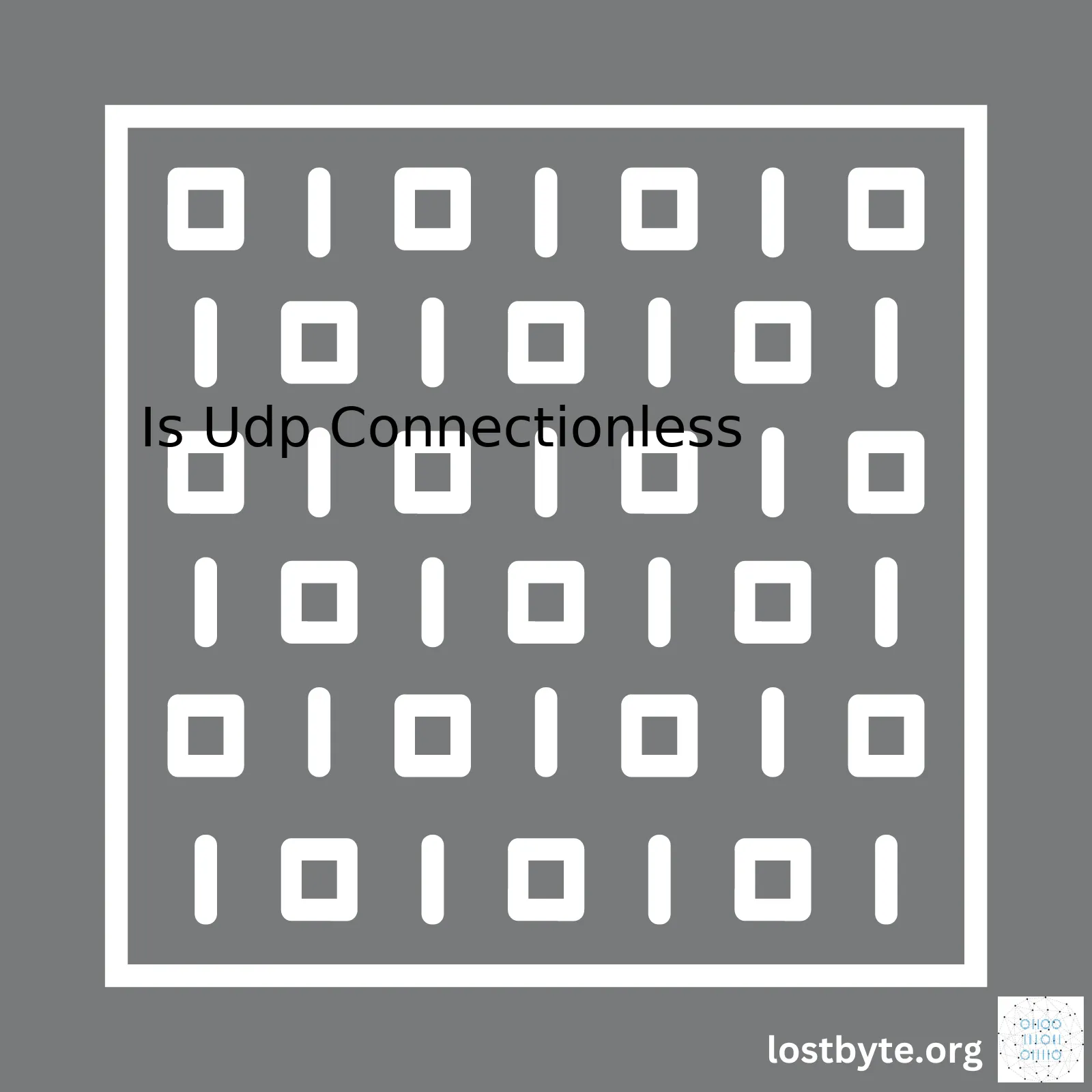
Aspect | Description |
---|---|
UDP | User Datagram Protocol |
Connection Type | Connectionless |
Purpose | Fast and efficient transfer without establishing a connection or maintaining a session. |
Use Case | Streaming services, VOIP, Games, Broadcasting applications to improve their efficiency and speed |
Advantage | No need for acknowledgement prolongs the duration of the live transmission |
Limitation | There is no guarantee of message delivery, order, or duplicate protection |
Breaking it down further, It’s critical to note that UDP (User Datagram Protocol), one of the oldest network protocols in the wild, operates in a connectionless mode, which is unlike TCP (Transmission Control Protocol), its counterpart. UDP stands as a significant fragment of the modern Internet Protocol suite.
The core aspect that sets UDP apart is, being an inherently connectionless protocol, UDP doesn’t establish a connection before sending data, nor does it ensure that the recipient is prepared to receive this data. The focus with UDP is more on fast, efficient transfer rather than ensuring delivery with no loss, duplication, or sequence issues. This feature makes UDP the de facto choice for applications like streaming services, Voice over IP (VoIP), games, and broadcasting applications where speed is prioritized over absolute consistency of data.
However, it is crucial to remember that varying speeds or signal quality can result in data loss or out-of-order delivery since there are no algorithms within the UDP protocol itself to counter these problems.
Now, you could be wondering why anyone would use a protocol—at all—with the chance of losing the information. Well, applications tailor their usage of UDP to fit their needs. For instance, Netflix uses QUIC—a protocol based on UDP—whilst adding its reliability layers. On the other hand, high-frequency trading systems use Raw UDP to avoid latency introduced by TCP’s error correction mechanisms.
One illustrative implementation of a simple UDP Client in Python goes like:
import socket
UDP_IP_ADDRESS = "127.0.0.1"
UDP_PORT_NO = 6789
Message = "Hello, Server"
clientSock = socket.socket(socket.AF_INET, socket.SOCK_DGRAM)
clientSock.sendto(Message, (UDP_IP_ADDRESS, UDP_PORT_NO))
As seen here, the socket module from Python’s standard library is employed, and sendto method is used directly without any connect() calls; thus, saving time and resources.
To learn more about UDP, you can read up on “Internetworking with TCP/IP Vol.1: Principles, Protocols, and Architecture” (Comer, 2005).
Please remember that knowing when to use and when not to use UDP comes down to understanding your system requirements and considering aspects such as quality, timeliness, and relevance of the information to be transmitted.
Yes, indeed. UDP, or the User Datagram Protocol, is indeed a connectionless protocol within the Internet Protocol suite, which web developers and programmers use regularly. Diving deeper into this topic will help bring greater clarity.
The Connectionless Nature of UDP
In computer networking, the term “connectionless” describes data communication operations that are not bounded by a dedicated end-to-end path or physical circuit between the source and destination nodes of the network. Each data packet travels independently from its siblings and might take a different route to reach the endpoint, without consideration of whether its fellow packets arrive accurately or at all. This method stands in contrast to a “connection-oriented” communication where a dedicated channel ensures the correct arrival of packets in an organized sequence.
In relation to UDP, the connectionless nature manifests in the following ways:
- No Connection Establishment: In UDP, there’s no handshake procedure (like the three-way handshake in TCP) before sending data packets. A UDP sender can start sending packets to the receiver as soon as it knows the destination address and port.
- No Connection Termination: As there’s no proper connection established, there’s also no need for orderly termination of the session. The sender stops when it has no more data to send.
- No Order Guarantee: In UDP transmission, data packets may arrive out of order, be duplicated, or even go missing without notice. It’s primarily due to the absence of prior connection establishment to guarantee strict chronological packet delivery.
- No Error Recovery: If a packet is lost in transmission over the network, UDP does nothing to recover it. The responsibility for error-checking and recovery usually falls on the application using UDP.
Working Mechanism Of UDP
The functional mechanism of UDP sends ‘User Datagram’ from one node to another, hence the name ‘User Datagram Protocol.’ It’s faster because it doesn’t involve any time-consuming procedures like establishing a connection or ensuring order delivery and recovery. A UDP header comprises four fields:
xxxxxxxxxx
Sender Port Number | Receiver Port Number
Length | Checksum
This light-weight header design further enhances the speed but limits data reliability. However, specific applications can tolerate these limitations.
Ideal Applications For UDP
Applications and services favoring speed over reliability typically adopt UDP. Streaming media, live broadcasts, online games, VoIP (Voice over IP), and DNS (Domain Name System) are some fields where UDP is frequently used. If few data packets drop or arrive late, it wouldn’t significantly impact the service quality in such scenarios.
For a complete understanding of this topic, you might want to explore further resources:
IETF RFC 768,
Wikipedia.TCP (Transmission Control Protocol) and UDP (User Datagram Protocol) are two fundamental protocols used in Internet communications. The primary characteristic that distinguishes the two is that TCP is a connection-oriented protocol, while UDP is a connectionless protocol.
TCP, before exchanging data, establishes a communication link between the sender & recipient through a system known as ‘three-way handshake’. Thus, there is an assurance of delivering packets to their destination. It carries overhead due to its various error-checking mechanisms and services like flow control.
In stark contrast, UDP doesn’t establish a connection before sending data, making it connectionless. Resultantly, UDP has high-speed data delivery but doesn’t ensure safe or intact receipt of messages at the other end.
For instance, think of TCP as making a phone call where you speak, wait for the response, then continue again and UDP as throwing messages in a crowd. You hope they get it, but there’s no guarantee.
Why Connectionless (UDP) Matters:
A few key reasons make UDP, despite its lack of guaranteed delivery, a crucial protocol in certain applications:
• Speed: UDP’s glaring absence of connection-establishment phase cuts the time delay, which makes it ideal for real-time applications.
xxxxxxxxxx
//Pseudo code for creating a UDP socket
Socket mySocket = new Socket(AddressFamily.InterNetwork, SocketType.Dgram, ProtocolType.Udp);
Examples: Video streaming, live broadcasts, online games, VoIP calls.
• Reduced Overhead: UDP discards several TCP features (e.g., error checking, sequence order), thus lightening the protocol overhead. With fewer data to manage, UDP is leaner and quicker than TCP.
xxxxxxxxxx
//Send data via a UDP socket
IPEndPoint remoteEndPoint = new IPEndPoint(IPAddress.Any, 0);
Byte[] receiveBytes = mySocket.Receive(ref remoteEndPoint);
Example: DNS for small query & response pairs, SNMP for network management tasks, BOOTP, DHCP etc.
• Multicast and Broadcast operations: Due to its connectionless nature, UDP allows one host source to send messages to many host destinations simultaneously.
Example: IPTV broadcasting, Live sports casting etc.
However, what shines in one scenario can dull in another. In case your application necessitates reliable data transmission or ordered sequence of data, TCP becomes your go-to choice.
To wrap up, UDP, being connectionless, is instrumental in environments where speed is prioritized over reliability. Its less overhead, multicast capabilities and absence of connection establishment make it handy in specific use-cases. However, the trade-off is risking data loss during transit and without any error-recovery provisions. So it becomes essential to choose the right protocol based on the given requirements and constraints.Sure! Let’s dive into the concept of UDP and understand its role in network communication.
UDP (User Datagram Protocol) is an integral part of IP network communication protocol suite [RFC 768]. It provides a connectionless communication method that offers simplicity, speed, but no guarantee for packet delivery.
Now, you might ask, “What do you mean by connectionless?” Essentially, a connectionless protocol does not require a logical connection to be established between the endpoints before data transfer occurs unlike TCP (Transmission Control Protocol), another protocol used in network communication.
To further illustrate this, let’s think about postcards. When sending a postcard, you don’t need any assurance that your recipient is at their destination nor receive confirmation when they’ve read it. You just send it and hope it gets there. That’s essentially how UDP operates!
But, is it a bad thing? Not necessarily. There are areas where the benefits of such a system can shine significantly:
- Critical real-time applications: In gaming or live streaming situations where speed is paramount and a bit of data loss is acceptable.
- Service discovery & simple query responses: With protocols like DNS and SNMP using UDP due to its lightweight nature, one does not necessitate the overhead of establishing connections.
- Unidirectional data flow systems: Like IoT devices pushing sensor readings to a server where the necessity for acknowledgements is unnecessary.
Looking into its structure, a UDP datagram primarily consists of four fields – source port, destination port, length, and a checksum. To give you a visual representation:
Source Port | Destination Port |
---|---|
Length | Checksum |
Consider the following Python code snippet sending a message via UDP:
xxxxxxxxxx
import socket
msg = "Hello, Server"
sock = socket.socket(socket.AF_INET, socket.SOCK_DGRAM)
sock.sendto(msg.encode(), ('localhost', 12345))
sock.close()
Notice how we didn’t establish any connection with the server before sending the message? That’s the beauty of UDP.
However, there are couple of things to bear in mind. Since it does not involve setting up a dedicated end-to-end connection, we may lose the item entirely without even noticing. Moreover, it doesn’t re-order packets which are out-of-order unlike TCP.
While UDP isn’t perfect, it plays a crucial role in situations where lower latency is preferred over reliability. By understanding its traits and using it wisely, you’ll be able to optimize the system performance to the fullest extent possible.
The User Datagram Protocol (UDP) is indeed a connectionless protocol. This means that it doesn’t establish a handshake or any other connection before sending the data, and as such, it doesn’t necessarily guarantee delivery of packets which makes UDP often labeled an “unreliable” protocol.
The main characteristics of UDP include:
- It’s Connectionless: Data can be sent at any point without any precursor unlike TCP which requires a handshake before data transfer can commence.
- It Operates at Speed: Since no checks are done before the data is sent, this contributes to its processing speed.
- It allows packets to be delivered in an arbitrary order.
Evaluating the Data Transfer in UDP:
The uncertain nature of UDP’s data transfer mechanism can be both positive and negative. Let me dive deeper into this:
- Efficiency: UDP specializes in broadcasting messages over networks since it does not require creating and maintaining connections. This results in less network traffic and a faster rate of transmission.
- Flexibility: UDP offers flexibility. Since it’s a connectionless protocol, it handles intermittent communications better than other protocols. It’s ideally fit for systems that need to handle fluctuating loads.
- Reliability: While UDP can be faster, it does not offer reliability. If packet loss occurs during the transmission the data will not be retransmitted. So, if you’re working with applications where data precision is important, UDP might not be the most effective choice.
To demonstrate with Python programming language, I am providing here a simple code snippet for creating a UDP client socket and sending a message:
xxxxxxxxxx
import socket
# Create socket object
sock = socket.socket(socket.AF_INET, socket.SOCK_DGRAM)
# Define server address
server_address = ('localhost', 65535)
# Message to send
message = b'This is a test message!'
# Send message to server
sent_data = sock.sendto(message, server_address)
This basic example should provide insight into how the UDP protocol works. Note that there isn’t any explicit method to establish a connection – this demonstrates how UDP operates in a connectionless manner.
Understanding UDP is paramount for programmers tasked to develop real-time applications where data needs to be rapidly transmitted and a few dropped packages are tolerable, something TCP protocol may struggle to achieve due to its nature of double-checking every single segment of data. Good examples where UDP is preferred would be streaming video, online multiplayer games or VoIP calls. Here is an additional reference from Wikipedia on UDP for further reading!
Yes, User Datagram Protocol (UDP) is a perfect example of a connectionless protocol that exists within the internet protocol suite. To understand why UDP is considered to be a connectionless protocol, it’s necessary to delve into its attributes and discuss how each attribute contributes to its status as a connectionless protocol;
1. Lack of Connection Establishment And Termination
The main characteristic of connectionless protocols like UDP is their lack of establishing and terminating connections. UDP doesn’t require any preliminary handshake to set up connection before data transmission or after data transmission which makes it completely independent of session.
xxxxxxxxxx
// UDP example code for sending packets without prior connection
int udpSocket = socket(PF_INET, SOCK_DGRAM, IPPROTO_UDP);
struct sockaddr_in serverAddress;
serverAddress.sin_family = AF_INET;
serverAddress.sin_port = htons(1234); // sample port number
serverAddress.sin_addr.s_addr = inet_addr("127.0.0.1"); // localhost address
char *msg = "Hello, Server";
sendto(udpSocket, msg, strlen(msg), 0, (struct sockaddr *)&serverAddress, sizeof(serverAddress));
This model implies a considerable saving of resources because it skips the overhead of creating and maintaining connections.
2. Packet-Based Communication
In UDP, data is sent in individual packets, each of them separately addressed with the source and destination IP addresses and ports. This means that data packets may arrive at the recipient out of order or might get lost during transit.
3. Lack of Congestion Control and Flow Management
Unlike TCP which engages in flow control and congestion avoidance, UDP lacks these mechanisms. Therefore, UDP does not adjust its segment size or retransmit behavior based on network conditions. This can lead to packet loss if the network becomes congested.
4. No Guarantee of Delivery
As there’s no acknowledgement mechanism embedded in UDP for acknowledging the successful delivery of messages, there isn’t any guarantee that the packets sent by the sender will reach their intended destination. If reliability is imperative, an application-level protocol needs to be installed to check whether all the packets have been delivered successfully and arrange for retransmission of lost packets if necessary.
xxxxxxxxxx
// Example UDP sender that does not wait for acknowledgments
int udpSocket = socket(PF_INET, SOCK_DGRAM, IPPROTO_UDP);
struct sockaddr_in serverAddress;
serverAddress.sin_family = AF_INET;
serverAddress.sin_port = htons(1234); // sample port number
serverAddress.sin_addr.s_addr = inet_addr("127.0.0.1"); // localhost address
char *msg = "Hello, Server";
sendto(udpSocket, msg, strlen(msg), 0, (struct sockaddr *)&serverAddress, sizeof(serverAddress));
// Close the socket immediately, without waiting for acknowledgment
close(udpSocket);
All these characteristics are synonymous with connectionless protocols. However, despite these limitations, UDP has certain advantages that make it an important component in the internet protocol suite. Particularly where speed is more fundamental than accuracy, such as video conferencing or live broadcasts.
For further insights into UDP and connectionless protocols, this Cisco document provides comprehensive knowledge.
Certainly, I’ll explain the implications of UDP’s (User Datagram Protocol) connectionless model on security and how it relates to UDP being connectionless.
Just as a refresher, the main aspect that differentiates UDP is that it is connectionless. This means that unlike TCP/IP, it doesn’t require handshakes or acknowledgments to ensure that data packets have been received at the other end. Here’s a simple example demonstrating the lack of handshaking in a UDP communication:
xxxxxxxxxx
// Example of Java code for UDP
public class UDPExample {
public static void main(String[] args) throws IOException {
// Creating a socket
DatagramSocket ds = new DatagramSocket();
String str = "Hello World!";
// Converting string into byte array
byte buf[] = null;
buf = str.getBytes();
// Getting IP address by name
InetAddress ip = InetAddress.getByName("localhost");
// Creating datagram
DatagramPacket dp = new DatagramPacket(buf, buf.length, ip, 6666);
// And simply sending
ds.send(dp);
}
}
While this makes UDP more efficient for specific applications like streaming services or DNS lookups, it also has serious implications for security.
Firstly, because there is no connection established before transmitting data, this makes the service vulnerable to IP spoofing. A malicious user can masquerade their IP address as another, tricking the system into thinking the packets are coming from a trusted source.IBM Security.
Secondly, UDP’s lack of acknowledgment mechanism allows Denial of Service (DoS) attacks to be carried out easily. By constantly sending packets to a particular port number, an attacker can overload the target machine.
xxxxxxxxxx
// Example of Java code for UDP DoS attack
public class UDPDosAttack {
public static void main(String[] args) throws UnknownHostException, SocketException, IOException {
// Target machine's IP and Port
InetAddress addr = InetAddress.getByName("targetIP");
int port = targetPort;
byte[] buffer = new byte[1024];
DatagramSocket socket = new DatagramSocket();
DatagramPacket packet;
while(true)
{
packet = new DatagramPacket(buffer, buffer.length, addr, port);
socket.send(packet);
}
}
}
That being said, this doesn’t render UDP useless from a secure communications standpoint. By implementing your reliability protocols and applying strict packet filtering rules, you can still achieve secure communications with UDP. For example, IPsec protocol suite can provide authentication and encryption for UDP trafficSpringer link. This suite secures IP communications by authenticating and encrypting each IP packet in the communication stream.
UDP though less reliable due to its connectionless model, when used accurately considering its features, can be beneficial in specific use-case scenarios.UDP, short for User Datagram Protocol, is indeed a connectionless protocol. This characteristic forms a sharp contrast with connection-oriented protocols such as TCP (Transmission Control Protocol). To enhance the understanding on UDP and how it compares to these other kinds of protocols, let’s delve into a comprehensive comparative analysis.
Reliability
A high degree of reliability is integral to connection-oriented protocols like TCP. When transmitting data, they establish a connection initially and maintain it till the end of communication. They make use of mechanisms such as error checking, error recovery, and acknowledgments to ensure accurate data delivery. Here’s a snippet of TCP header includes several fields dedicated to reliable delivery:
xxxxxxxxxx
{
"Source Port": "16bit",
"Destination Port": "16bit",
"Sequence Number": "32bit",
"Acknowledgement Number": "32bit"
...
}
On the other hand, UDP – a protocol based on transmission without connection – doesn’t provide these features. It sends datagrams to the recipient without setting up a connection or validation about their correct arrival at the destination. This is why you frequently hear that UDP offers no guarantee for packet delivery.
Data Ordering
TCP further ensures that data packets reach the receiver in the same sequence as they were sent owing to its ordered delivery feature. Again, UDP lacks this ability. The UDP packets could arrive out of order or not show up at all.
Delivery Timing
When it comes to timing, being connectionless gives UDP an edge. Without the need for establishing and maintaining a connection, overhead is notably lower, so data transmission happens faster. Such an attribute makes UDP ideal for real-time applications such as streaming media where timely delivery matters more than perfect receipt. For instance, in a live video stream, a few lost frames due to dropped packets aren’t as crucial as ensuring minimal latency.
Overhead and Resource Usage
With all the additional control messages, connections, sequencing, and error handling, protocols like TCP have a higher overhead and consume more resources. Considerably lightweight, UDP, on account of lacking these aspects, consumes lesser network and system resources.
Comparative characteristics can be summed up in the below table:
Aspect | UDP | TCP |
---|---|---|
Connection | Connectionless | Connection-oriented |
Reliability | No Guarantee | Guaranteed |
Data Order | Not Guaranteed | Ordered |
Timing | Faster Delivery | Slower due to control mechanisms |
Overhead / Resource usage | Low | High |
So, is UDP connectionless? Absolutely! But just because it is less reliable and lacks inherent order doesn’t mean it’s inferior. Its design serves specific needs within the broad landscape of network communication, making it suitable for scenarios where speed and efficiency trump absolute reliability and order. Let’s remember that UDP, despite its limitations, continues to play an essential role in the internet architecture today.
Absolutely, when discussing the error-checking capabilities and limitations of a User Datagram Protocol (UDP), it’s essential to incorporate its inherent connectionless attribute into the narrative.
Fundamentally, being connectionless, UDP doesn’t establish a secure link between communicating parties before transmitting data. Consequently, it may lack some stringent error-checking features available in other protocols like Transmission Control Protocol (TCP).
To set the record straight, UDP does offer error checking in the form of header checksums. This capability is limited compared to other protocols, but still significant within the scope of its functions. In the simplest terms possible, think of this as UDP doing a quick assessment of the received data condition.
Illustratively, here’s how UDP packaging with checksums works:
xxxxxxxxxx
0 7 8 15
+--------+--------+
| Source Address |
+--------+--------+
| Destination Address |
+--------+--------+
| Zero |Protocol| UDP Length |
+--------+--------+
| UDP Header | Data |
+--------+--------+
In ‘UDP Header’, you’ll find a two-byte segment known as ‘UDP Checksum’. Its value is computed based on the source and target addresses, protocol, UDP length, and data. You can perceive this as some form of bookkeeping information which aids in assessing if there was any tampering or corruption during transmission.
The receiver then computes its version of these values and compares them. Do both sets tally? Then we have a successful delivery. Are they at odds? It indicates data corruption happened along the line, and the packet gets discarded.
However, this mechanism’s limitations emerge because it also discards erroneous packets without notifying the sender. This is due to the absence of an acknowledgement system, typically found in connection-oriented protocols. Another downside to this strategy is that UDP doesn’t support retransmission of dropped packets.
So, while UDP performs some rudimentary error-checking, the depth isn’t profound given its primary use cases underscored by speed and efficiency, such as Domain Name System (DNS) queries, Voice over Internet Protocol (VoIP), video streaming, and online gaming. In these scenarios, the occasional loss of a few packets rarely affects the user experience or can get circumvented through preparing for such losses in the higher-level application designs.
To cap it off, remember that, even though it lacks advanced error management functionalities, UDP remains a staple, ruthlessly efficient beast in situations where speed trumps reliability.Computing devices use the Transmission Control Protocol (TCP) and the User Datagram Protocol (UDP) to transmit internet data in different ways. UDP is commonly identified as a ‘connectionless’ protocol, whereas TCP operates through establishing a connection. This primary distinction influences their behavior concerning transmission speed.
The term ‘connectionless’, referring to UDP, implies that this protocol transmits data without requiring a connection or confirmation from the receiving end.
xxxxxxxxxx
UDP.send(data)
will send data regardless of whether the recipient is ready for it. On the contrary, TCP requires an acknowledged connection before transferring any data; you can’t
xxxxxxxxxx
TCP.send(data)
until the recipient has confirmed their readiness to receive. This characteristic saves time in urgent scenarios where rapid communication, albeit potentially lossy, is a priority.
Here’s an analogy: think of TCP as certified mail, where delivery is confirmed and if not reached, retries continue. Meanwhile, consider UDP as regular postcards — messages are sent, but no confirmation on the reception is expected. As a result, UDP is much faster since it doesn’t pose delays with confirmations. Hence, streaming services, live broadcasts, and video games commonly employ it due to its high-speed efficiency.
Nonetheless, consider the broader context:
- Data integrity: Despite being slower, TCP ensures more reliable data transfer because it acknowledges receipt and resend lost packets. UDP skips these steps, hence gaining speed but compromising on complete data accuracy.
- Error Checking: A fundamental differentiation factor is error-checking. TCP inspects data quality thoroughly hence higher overhead. Although UDP checks for errors such as bad headers, it does not guarantee packet order and may skip damaged ones – another aspect indicating velocity over accuracy.
- Connection Overhead: Establishing a TCP connection involves a three-way handshake, which although ensures a clear path, brings extra overhead. On the other hand, UDP, being connectionless, evades this overhead but places the responsibility of interpretation and maintenance on the application level.
A practical choice between TCP and the ‘connectionless’ UDP depends on the situation. In scenarios where the speed of delivering the message matters more than its absolute completeness (such as VoIP calls, live broadcasts etc.), UDP becomes a preferred protocol. Conversely, if data integrity takes precedence, a little delay would be acceptable, and hence TCP works best.
In conclusion, both delivery mechanisms have their pros and cons relating to overall transmission speed. Analyze requirements cautiously while deciding on one, considering not only speed but also reliability and data integrity aspects. For further exploration, I recommend reading online resources like Guru99’s comparison guide on TCP and UDP.When considering the User Datagram Protocol (UDP), getting to grips with its connectionless nature is essential. To understand how real-world applications use UDP despite its connectionless nature, we can look at some examples where this protocol shines.
As a professional coder, I often reach out to UDP when I seek fast data transmission without any integrity checks. A thorough understanding of the UDP protocol begins with verbalizing what “connectionless” means in this context. Essentially, unlike its counterpart TCP (Transmission Control Protocol), UDP does not set up a dedicated end-to-end connection. Rather, it uses the “fire-and-forget” method, throwing data across to the receiver with no prior arrangements or post-transmission checks. This removes overheads such as handshaking and retransmissions, making data transfer faster.
UDP in Real-World Applications
Consider these real-world applications that make judicious use of UDP’s unique features:
• DNS: The Domain Name System extensively uses UDP for querying domain names. The fast-paced, connectionless nature of UDP shines here as quick query-resolution is important. For instance, when you type “www.google.com” into your browser, a DNS lookup is performed using UDP to resolve the name to an IP address.
xxxxxxxxxx
nslookup google.com
• Video Streaming: Real-time services like online gaming and video streaming can tolerate some data loss. These applications prioritize real-time user experience over perfect data integrity. Hence, they often opt for UDP with its low latency over TCP which ensures data accuracy by retransmitting lost packets but increases latency.
• VoIP: Voice over IP services like Skype also utilize the UDP protocol. In VoIP calls, fast delivery takes precedence over missing or unordered data chunks. You probably wouldn’t even notice if a small sound byte from your conversation was dropped. That’s why UDP suits VoIP perfectly.
To provide a simple example in Python:
xxxxxxxxxx
import socket
# UDP broadcast
sock = socket.socket(socket.AF_INET, socket.SOCK_DGRAM)
sock.setsockopt(socket.SOL_SOCKET, socket.SO_BROADCAST, 1)
msg = 'Hello World!'
sock.sendto(msg.encode('utf-8'), ('', 37020))
sock.close()
The above code snippet shows how to send a UDP broadcast packet in Python. There’s no respect for any established session as seen in TCP protocols – it’s essentially just sending the message off hoping it reaches the defined port.
Despite its being connectionless, UDP serves as an efficient workhorse in handling speed-sensitive tasks where the complete accuracy of data isn’t critical. Combine this with modern error and congestion control techniques, and it’s clear why many modern applications still prefer UDP under the hoods.
Looking at these examples, we can observe that most applications using UDP are either tolerating some level of data loss or have implemented customized mechanisms to ensure data reliability. The application-bound control over fail-safes and data integrity allows developers to optimally balance data speed vs accuracy in line with their specific needs. Hence, even though UDP is connectionless, it remains the ideal choice for specific use-cases where speed triumphs over data integrity.
Keep in mind that ignoring the need for connection might not be the best strategy for every application. However, understanding the strengths and limitations of a connectionless protocol like UDP paves the way for informed decision-making when designing network-spanning applications.At the core of network programming and internet communication, the User Datagram Protocol (UDP) brings a distinct characteristic to the table: it is connectionless. This term might sound confusing at first, especially for those new to the world of network protocols and, specifically, to the comparison between UDP and the more commonly-discussed Transmission Control Protocol (TCP). However, understanding what being ‘connectionless’ means in this context is quintessential to grasp the strengths and weaknesses of UDP.
Understanding Connectionless Protocols
The principle of UDP being ‘connectionless’ refers to how it sends packets without establishing a direct connection with the receiving end first. In other words, UDP sends data without checking whether the recipient is ready to receive it or not.
In contrast, TCP ensures a reliable connection – if a piece of data gets lost on its way to the receiver, TCP will notice this and resend it. UDP doesn’t give any such guarantee – it’s like throwing a paper airplane into the air, then walking away, regardless of whether it reaches its destination or not. It’s important to note that while this approach by UDP may initially seem reckless, it can save significant time and overhead in specific use-cases.
The Advantages and Disadvantages
From the above, we can infer that:
– UDP might be an excellent choice for applications where speed is crucial, minimizing latency being paramount to the nature of some operations. Instances include real-time systems such as VoIP, video streaming, and online gaming.
– Furthermore, it removes the need for proper handshake procedures, which implies less system resource utilization and lesser network traffic, making it undoubtedly faster than connection-oriented protocols like TCP.
– On the downside, it’s up to the application to monitor loss and delivery of the information and to retransmit as needed. Its lack of reliability mechanisms makes it unsuitable for large file transport or critical bulk data transfer, where missing data can significantly affect the outcome.
Fine-tuning network communication protocols to cater to different situations are one way programmers and system administrators can ensure efficient use of resources and optimize overall performance.
xxxxxxxxxx
# UDP Client code
import socket
UDP_IP = "127.0.0.1"
UDP_PORT = 5005
MESSAGE = b"Hello, World!"
sock = socket.socket(socket.AF_INET, # Internet
socket.SOCK_DGRAM) # UDP
sock.sendto(MESSAGE, (UDP_IP, UDP_PORT))
This Python script is an example of using UDP to send a message. No connection is established before sending the message, which highlights the connectionless nature of UDP.
To learn more about how to use UDP in your applications, consider exploring further on Python’s socket library documentation or even taking a deeper dive into RFC 768, the formal definition of UDP.
We thus arrive at the conclusion that calling UDP ‘connectionless’ paints half the picture. While it indeed does not establish a traditional connection before data transmission, it has unique advantages that make it suitable in scenarios where speed trumps reliability. Therefore, understanding this characteristic of UDP can help us leverage its benefits more effectively in our programming journey.