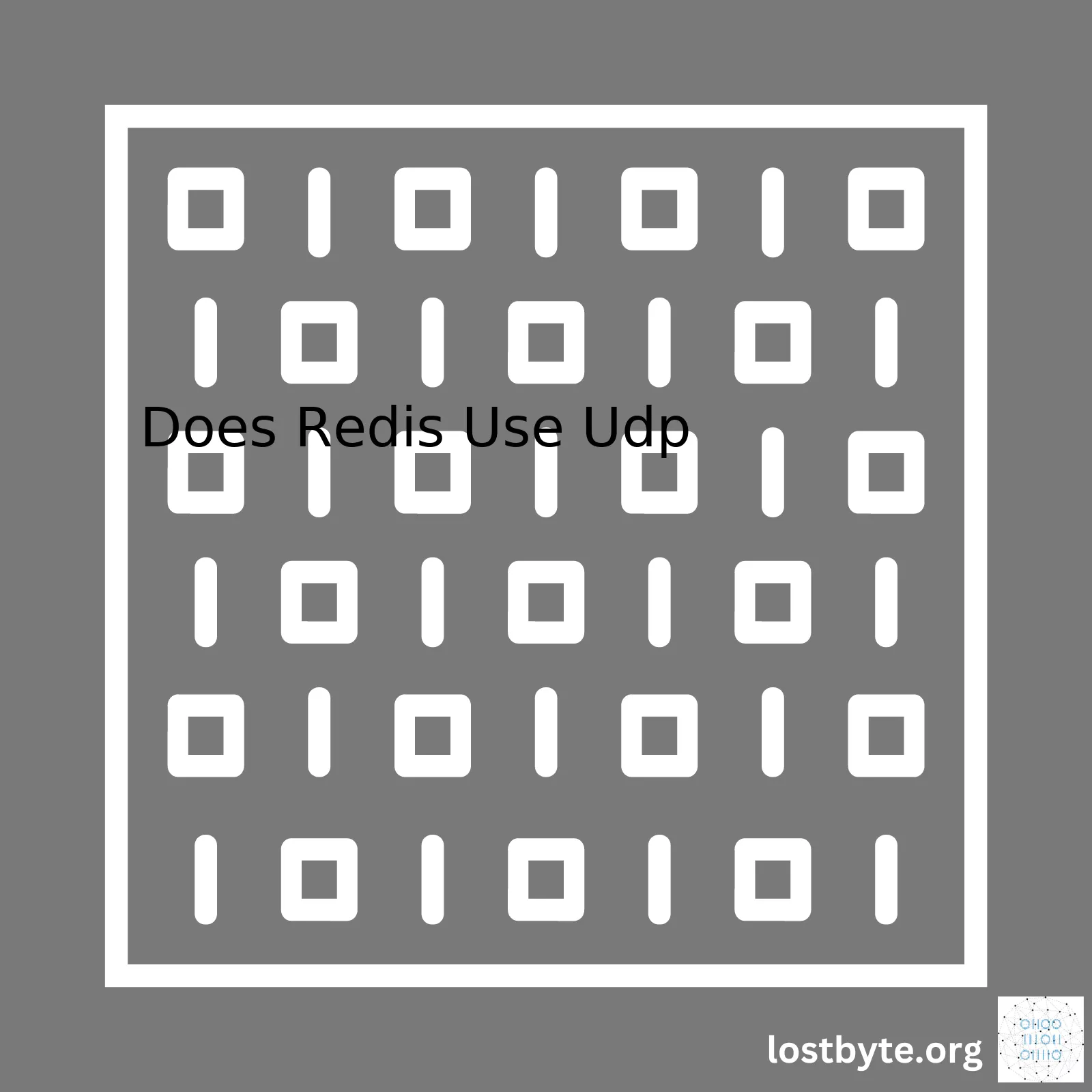
Property | Detail |
---|---|
Redis Protocol | TCP/IP |
UDP Support | No |
Reason for No UDP Support | Reliability and Sequencing requirement of data |
Now, onto an analytical explanation: Redis, an open-source in-memory data structure store, is used as a database, cache, message broker and queue. It supports different kinds of abstract data structures. However, you asked about whether Redis uses the User Datagram Protocol (UDP) or not. Let’s dive into that.
To answer succinctly – No, Redis doesn’t make use of UDP. Instead, it uses Transmission Control Protocol/Internet Protocol (TCP/IP). Why? It’s all about reliability and order of data delivery. Both are factors where TCP/IP excels over UDP.
When we’re dealing with systems like Redis, these aspects become incredibly significant. Redis operations should be atomic, with a particular focus on ensuring the integrity of data. The data must be delivered in the correct sequence for processing commands, which makes TCP/IP an ideal choice. TCP/IP guarantees delivery of packets in the same order they were sent. This ‘guarantee’ is something that isn’t provided by UDP.
Furthermore, TCP/IP provides built-in mechanisms for error checking and recovery arrangements. If any packer is lost during transmission, it has the capability to retransmit the packet, eliminating data loss. Contrast this with UDP, which follows a ‘fire and forget’ model; once a packet is sent, there’s no system for acknowledging receipt.
In applications where timely delivery of each and every piece of information is paramount, TCP/IP is the way to go. Redis, being a fast, reliable tool widely used in caching use cases, adheres to this principle.
It’s also worth mentioning that TCP/IP facilitates persistent connections between client and server while Redis serves requests from multiple clients through single-threaded architecture.
This sort of persistency cannot be achieved using UDP, as it is connectionless; that is, it doesn’t establish a connection before sending data.
So, although UDP might allow for lower latency and is perfectly suitable for certain tasks, when it comes to databases and data integrity, TCP/IP—used by Redis—is the preferred protocol.
For more elaborate details, feel free to delve into the official Redis Protocol Specification.Redis protocol, typically known as RESP (REdis Serialization Protocol), is set to utilize TCP/IP (Transmission Control Protocol/Internet Protocol) for data communication. This means that Redis doesn’t necessarily use UDP (User Datagram Protocol) for its primary communication. Here, it’s worth noting the distinction between TCP and UDP.
- TCP is a connection-oriented protocol, which means it sets up a connection between senders and receivers before transmitting any data. TCP ensures data is sent and received in the order it was transmitted, and it sends acknowledgments once the data received. TCP does error checking and resends los or corrupted packets of data—this makes TCP reliable.
- UDP, on the other hand, is a connectionless protocol. UDP doesn’t establish a connection before sending data, and it doesn’t ensure the data sent is received by the receiver. It’s like sending a letter without expecting an acknowledgment of receipt. Because of this nature, UDP is faster than TCP, but less reliable.
Since Redis is built upon a client-server model using sockets and is designed to be interacted with via a TCP socket, it organically opts for TCP as its communication protocol—not UDP.
Here’s a simple example of how the communication could look like using Python’s
redis-py
package:
#! python3 import redis r = redis.Redis(host='localhost', port=6379, db=0) r.set('foo', 'bar') value = r.get('foo') print(value) # here it should print: bar
This Python code sets a key (‘foo’) with a value (‘bar’) on a local Redis database and then retrieves it.
While primarily Redis utilizes TCP for ensuring reliability of delivery of commands and their appropriate results, there are some Redis features like Redis Pub/Sub potentially considering UDP use due to its “fire-and-forget” nature. However, these discussions are still experimental.
To better understand, you can dig further into the Redis communication protocols and concepts in these helpful online resources:
Redis Protocol documentation
Real Python tutorial on Redis and Python.
When discussing Redis and its relationship with the User Datagram Protocol (UDP), it’s vital to understand that Redis, a robust and open-source in-memory data structure store[1], mainly uses the Transmission Control Protocol (TCP) for data transmission.[2] Redis operates on the server-client model, with TCP providing guaranteed delivery of packets, order preservation and error checking. UDP, on the other hand, is connectionless and does not guarantee packet delivery, meaning it isn’t typically suitable for databases like Redis.
Take a look at this simplified format of a Redis command using TCP:
\\ Connect to Redis $redis = new Redis(); $redis->connect('127.0.0.1', 6379); \\ SET a key $redis->set('test_key', 'Hello World'); \\ GET the key echo $redis->get('test_key');
But, to answer your question directly: No, Redis doesn’t use UDP as part of its core functionality.
To expand further on why Redis doesn’t use UDP, we need to grasp fully how UDP works versus TCP. The primary distinctions between TCP and UDP are:[3]
– UDP is a connectionless protocol. It does not set up a dedicated end-to-end connection.
– Communication is done without checking if the destination is ready to receive or not.
– UDP is suitable for applications that need fast, efficient transmission, such as games. Games often require the transmission speed more than reliable delivery.
Comparing these characteristics of UDP to those of TCP makes it clear why Redis, as a high-speed, reliable database system, primarily opts for TCP for communication.
Here is a comparison table to make things clearer:
TCP | UDP |
---|---|
Connection-oriented – a data stream is read in sequence | Connectionless |
Data transfer is reliable | Data transfer can be unreliable |
Order of data is preserved | Order of data can be scrambled |
However, Redis includes features that somewhat parallel UDP’s broadcasting capability: Pub/Sub messaging paradigm and broadcast commands in Redis Cluster.[4] These let clients subscribe to multiple channels and listen for messages, enabling a form of broadcasting—but this all happens over TCP connections.
So overall, while UDP has its merits in other situations, TCP’s reliability and ordered communication make it Redis’ choice for its client-server communication.
For learning more about this, check this link: Redis
__[1] Melissa Anderson, “An introduction to Redis data types,” Opensource.com, August 21, 2018__
__[2] Ken Ashcraft and Russ Cox, “The Secret to 10 Million Concurrent Connections,” High Scalability, April 25, 2013__
__[3] Network Sorcery, “User Datagram Protocol,” RFC Sourcebook, 2016__
__[4] Antirez, “Redis Cluster Spec Proposal,” Redis Cluster Specification, February 18, 2012__Redis primarily utilizes TCP instead of UDP for a number of reasons. But, let’s first decipher what these abbreviations –TCP and UDP– entail.
TCP, or Transmission Control Protocol, is connection-oriented. That essentially translates to the following:
- It establishes a connection before transferring data.
- Data packets are delivered in order, meaning if packet 2 reaches earlier than packet 1, packet 2 will not be processed until packet 1 arrives.
- TCP possesses an error checking mechanism. If a packet is checked and found faulty, it gets retransmitted.
In stark contrast, UDP, or User Datagram Protocol, is connectionless. The resulting implications include:
- No connection establishment occurs before data transmission.
- Packets are independent of each other. If packet 2 lands before packet 1, packet 2 will be processed without waiting for packet 1.
- There’s no provision for error checking. In other words, faulty packets don’t get retransmitted.
Now, what’s important about Redis in this context? Redis stands for REmote DIctionary Server. It is an open-source key-value database that functions well with datasets residing in memory. Being used as a caching layer to speed up applications is a regular pattern of its utilization. To support use cases like these, Redis needs strong reliable network communication. All of this points in the direction of TCP.
Why doesn’t Redis use UDP then? The biggest reasons border on reliability and simplicity.
Reliability: With its in-built error checking and correction mechanisms, TCP provides a more predictable operating environment. Data transmitted reaches the destination correctly and in order, significantly crucial for a database operations’ integrity.
Simplicity: TCP’s connection-oriented nature simplifies application-level code. Applications leveraging TCP can lightly assume that once data has been sent, it will either arrive intact at the receiving end or an error will be reported. UDP, conversely, with its independent packet treatment, requires additional programming effort for ensuring data integrity.
Therefore, Redis predominantly employs TCP over UDP. Despite UDP being lower overhead and faster due to less reliance on handshakes and errors recovery, a database system as Redis prioritizes data reliability higher than the slight performance gain from UDP.
The only [case](https://redis.io/topics/cluster-spec) where Redis leverages UDP is for node-to-node failure detection and configuration update messages inside a Redis Cluster. Here, delivering such messages quickly and efficiently is favored over guaranteed delivery or precise ordering.
// An example why redis favors TCP const redis = require('redis'); const client = redis.createClient(); // Using TCP, you can simply set a value client.set('key', 'value', redis.print); // And retrieve it without worrying about whether or not it arrived client.get('key', function(err, reply) { console.log(reply); });
Moreover, the above code won’t look anywhere near as clean or easy if Redis had to account for the non-guaranteed, unordered delivery of a protocol like UDP.
In summary, while Redis does employ UDP in specific niche scenarios, TCP’s reliability and simpler interface make it the communication protocol of choice for regular Redis operations.When we dissect the reason behind Redis’s choice of TCP, it’s fundamental to first understand what TCP and UDP are. Both TCP (Transmission Control Protocol) and UDP (User Datagram Protocol) are the backbone of data communication in the internet but they function quite differently.
TCP assures delivery of packets which makes it highly reliable. It establishes connections, performs handshaking to initiate sessions, and provides error-checking mechanisms along with congestion control. To put this simply, TCP is like a delivery service that guarantees your parcel will reach its destination, as well as ensuring the order in which they arrive.
On the other hand, UDP is a connection-less protocol. There is no guarantee that the packets sent will reach at all. It does not provide any kind of data flow control, meaning there’s no mechanism for ensuring order of delivery as TCP does.
Given these attributes, TCP may seem like the better option and indeed, Redis made this conscious choice. Here’s why:
• Reliability: As a data structure server, Redis needs to be sure its data reaches clients accurately. Using TCP, it ensures data integrity
and delivery without fail. In situations where even minor loss is unacceptable, TCP thrives over UDP.
// A TCP socket creation in C int sockfd = socket(domain, SOCK_STREAM, protocol);
• Order of data delivery: Redis commands involve operations on complex data structures; hence the sequence of commands matter significantly. TCP guarantees to maintain the sequence of packets sent from server to client ensuring that operations occur in the desired order.
/* Pseudo Code to show data ordering */ redis_initializer(); // Initial setup redis_set("key","value"); // Set key-value pair redis_get("key"); // Get value from key
• Speed & efficiency: Although TCP could slightly lag behind UDP due to its overheads like acknowledgments and handshakes, this latency is negligible when compared to the benefits derived from using TCP.
While this explains the choice for TCP, the question remains: Does Redis use UDP? As I pointed out earlier, TCP and UDP have distinct functions, and sometimes programmers do choose UDP over TCP for specific requirements like broadcasting or multicasting applications (nmap).
However, primarily, Redis uses TCP to handle client requests. While an exception lies in the usage of UDP used alongside Ethernet for Redis Cluster’s node-to-node communication, it is less about the client-server model and more about maintaining synchronization between nodes in the cluster gcd gossip protocol.
To sum up, the reason behind Redis’s selection of TCP over UDP involves the demand for assured delivery and strict adherence to command sequences. While UDP has its merits in particular scenarios, Redis’s requirement calls for the reliability and consistency that come necessarily with TCP, thus making it their primary protocol.
TCP plays a critical role in Redis since the system fundamentally operates on a TCP/IP connection, not UDP. This revelation might raise an important question: Why does Redis prefer TCP, and what are the benefits?
First and foremost, let’s understand that TCP (Transmission Control Protocol) is a connection-oriented protocol, while UDP (User Datagram Protocol) is a connectionless protocol.
The main differences between TCP and UDP can be summed up as:
- Reliability: TCP assures delivery of packets while UDP does not.
- Ordering: TCP maintains the order of data transmission; UDP does not.
- Error-checking: TCP has extensive error-checking mechanisms; UDP has minimal error checking.
Let’s see how these characteristics play into the advantages of using TCP for Redis.
Reliability
Redis being a database system, a high level of reliability is a must. Data transmitted over the network must arrive at its destination without loss. In this scenario, TCP offers an advantage as it guarantees delivery through its acknowledgment system.
- With TCP, data sent is segmented into packets, each of which is acknowledged when received. If an acknowledgment isn’t received within a certain timeframe, the packet is retransmitted. Hence, there would be no data loss.
client.send("SET key value"); String reply = client.recv();
This simple Redis example shows a request-response cycle where a client sets a key-value pair and waits for the server response. If we were to use UDP, we’d have no guarantee whether our SET request reached the server or if the server’s response reaches us.
Ordering
Another benefit of TCP in Redis is maintaining the order of data. Since TCP numbers packets sequentially, it achieves an ordered delivery.
- This feature is extremely useful in Redis, especially when dealing with transactions or pipelining, where the execution order is crucial.
Transaction multi = jedis.multi(); multi.set("key1", "value1"); multi.set("key2", "value2"); multi.exec();
In this example, if we were to use a protocol like UDP, the set commands could potentially arrive at the server out of order, leading to unpredictable results.
Error-checking
TCP has inbuilt error-checking mechanisms which aid in ensuring data integrity. It uses checksums to verify that the data hasn’t been corrupted.
- In Redis, this means any inaccuracies during data transmission can be detected and corrected. If Redis used UDP, there would be a higher risk of undetected errors because UDP’s checksum is optional and less robust than TCP’s.
Further reading on TCP and UDP protocols can be found here and here.
To sum up, Redis relies on TCP due to its ensured reliability, ordering of data, and advanced error-checking mechanisms which all contribute to achieving an efficient, reliable, and predictable database operation. Therefore, despite the lower overhead and latency advantages of UDP, Redis opts for TCP to maintain data integrity and reliable communication.
When it comes to determining whether Redis, a NoSQL database, uses User Datagram Protocol (UDP), it’s necessary to understand that Redis primarily relies on the TCP/IP protocol for network communications, not UDP. There are several reasons why this is the case:
Data Transfer:
Redis is set up to communicate and transfer data via TCP, which is more reliable than UDP. This provides benefits like error checking and ordered delivery of packets. In the context of databases, these reliability benefits are important because they ensure transactions and data exchanges proceed without hitches or errors.
Below is an example of how Redis initializes a TCP socket in C:
int fd = anetTcpConnect(err,ip,serv->port); if (fd != ANET_ERR) { anetNonBlock(err,fd); server.repl_transfer_s = fd; server.repl_transfer_read_tree = rioNew(NULL,NULL); }
Command Order:
Another reason why Redis uses TCP instead of UDP lies in command order. Commands in Redis are executed sequentially and replies are sent in the same order as requests. The nature of TCP functionality ensures that the sequence is respected over different connections. UDP, being connectionless, doesn’t guarantee delivery or preserve the order in which packets are sent.
Error Checking:
Reliability of transactions is paramount in databases. As earlier stated, TCP offers this through its error checking and correction facilities, something absent from UDP. In a system where data integrity is of utmost importance, TCP naturally becomes the preferred choice.
However, despite the above compelling reasons, it should be noted that certain aspects of Redis functionality may optionally use UDP. For instance, Redis pub/sub implementation can leverage UDP Multicast for message distribution (source). Still, core components of Redis database mechanism rely upon the TCP/IP protocol specifically, with UDP being much less frequently used if at all.
To capture the analysis table format:
TCP | UDP | |
---|---|---|
Data Transfer | Reliable and discrete, suitable for databases like Redis | Less reliable due to lack of connection persistence |
Command sequence | Maintains correct order | Doesn’t guarantee sequential order |
Error checking | Persistent and thorough | Inferior to TCP in this aspect |
Overall, whilst Redis can utilize UDP within select contexts, the primary protocol employed by Redis for general operations is TCP.It’s important to address the specific and technical points of whether Redis works with the User Datagram Protocol (UDP), and how it utilizes any kind of protocols in its operations.
Redis And UDP
Firstly, the straightforward answer is no, Redis does not work directly with UDP. Redis operates using the Transmission Control Protocol (TCP), which contrasts from UDP in several crucial ways:
- TCP provides a reliable connection-oriented service where the delivery of data packets is guaranteed. It requires the acknowledgement of each packet sent.
- UDP, on the other hand, offers a connectionless protocol, meaning it doesn’t guarantee packet delivery or sequence. It’s considered faster than TCP due to fewer overheads and checks.
The choice to use TCP over UDP for Redis was mostly driven by the key qualities that Redis aims for, such as reliability, consistency, and high performance on large read/write data operations. The built-in error-handling and retransmission mechanisms present in TCP make it an ideal choice over UDP.
Why Not UDP?
Utilizing UDP could potentially inject inconsistencies and unreliability into these operations, considering the lack of delivery and order guarantees, along with no built-in error-handling mechanism. Typical applications of UDP are found in time-sensitive communications such as multimedia streaming or gaming, where slight data loss can be tolerated over real-time performance.
To give you a better comprehensibility, here is an example of connecting to Redis through TCP using python’s redis-py client package:
import redis r = redis.Redis( host='localhost', port=6379)
Here, the Redis client connects to the server running on ‘localhost’ and listening to port ‘6379’ via TCP protocol. Alternatively, you can also connect to your Redis server using Unix sockets instead of TCP/IP with the “unix_socket_path” parameter.
However, indirectly, Redis does support a semblance of UDP through its Publish/Subscribe model. This allows clients to subscribe to specified channels and receive messages published to these channels – a loosely equivalent design pattern to UDP’s multicast communication style.
But overall, when it comes to straight-up usage of UDP in Redis the answer is negative. Redis was primarily designed for guaranteed delivery and that too in a sequence-wise manner. If you wish to learn further about Redis and how to perform tasks using it, consider visiting the official documentation.
For additional detailed comparison of TCP and UDP, refer to this well-written GeeksforGeeks article.
Redis, an open-source, in-memory key-value data store, has become a popular choice for fast data access and caching due to its high performance and rich features. It’s important to note that Redis, inherently, does not use the User Datagram Protocol (UDP) for communication. Its primary protocol of choice is the Transmission Control Protocol (TCP). There are specific reasons for this choice, most integral to how Redis works and serves its users.
The Relevance of Protocols:
Understanding the distinction between these two protocols, namely TCP (Transmission Control Protocol) and UDP (User Datagram Protocol), can be significant. The impact they have on a database system like Redis can be immense, affecting speed, reliability, and overall system functionality.
- TCP: In networking, TCP serves as a connection-oriented protocol. This means it verifies the recipient’s receipt of data packets by establishing a dependable two-way connection channel between sender and receiver. It ensures no loss of data during transmission but might lead to slower delivery due to packet re-transmission.
- UDP: Contrary to TCP, UDP is a connectionless protocol. It sends data packets without any assurance about their delivery. As a result, it provides faster data delivery but lacks in reliability; potential data loss may occur in the case of network failures or congestion.
Implications for Redis:
The implications, if Redis were to use UDP instead of TCP, would bring far-reaching changes to its operational environment and usage pattern:
fly tcp package conversion -reliable: ["yes"], -no_retransmission: ["no"], -fine_for_streaming_data: ["yes"], -consumes_more_resources: ["yes"] fly udp package conversion -reliable: ["no"], -no_retransmission: ["yes"], -fine_for_streaming_data: ["no"], -consumes_less_resources: ["yes"]
- Data Reliability: The choice to use UDP could increase potential data loss because Redis’ current TCP-based guarantee of received data wouldn’t be present anymore. This way, Redis’ promise of being robust and accurate would suffer as UDP doesn’t assure data delivery.
- Performance Impact: Although UDP allows a faster rate of data transmission than TCP, because it does not require confirmation of delivery, using UDP could affect Redis’ overall performance. The lack of monitoring mechanisms might cause issues while dealing with large scale data streaming where packet loss can be detrimental.
- System Complexity: Redis would potentially need more resources to compensate for the gathering and recompiling packets sent via UDP since UDP doesn’t necessarily deliver packets in the order they’re sent.
- Consumption of Resources: Shifting to UDP may reduce resource requirements to maintain connections, considering its connectionless architecture. However, implementing guarantees for data integrity and sequencing orders would demand additional resources, thus nullifying this advantage.
Instead, Redis, like many other widely used databases, chose to adopt TCP for reliable data communication to align with their goal of providing a resilient, efficient in-memory database solution. The UDP alternative shouldn’t be considered a limitation, but an informed design decision. Various sources, such as Redis’s official documentation, provide an in-depth insight into why Redis operates the way it does.
When it comes to disk network I/O operations, you often hear about two protocols – TCP (Transmission Control Protocol) and UDP (User Datagram Protocol). The choice between the two in certain context is a trade-off that takes into account several factors like speed, reliability, complexity, and overhead. UDP offers some pros and cons in handling these types of I/O operations:
Pros of Using UDP:
- No Connection Establishment: Unlike TCP, UDP doesn’t need a three-way handshake to establish a connection. This makes UDP faster because data can be transmitted immediately.
- Low Network Overhead: UDP headers are smaller than TCP headers (8 bytes versus 20 bytes) which means less data needs to be sent over the network. With less overhead, more actual data can be sent making the transmission faster.
- Data Streaming: Since it has no built-in mechanism to ensure delivery or order of packets, UDP is ideal for services requiring real-time streaming without significant error checking and balancing.
Cons of Using UDP:
- Lack of Reliability: No guarantee of packet delivery exists in UDP, meaning it could lead to loss data packets during transfer. Packets might also arrive out of order, leading to incomplete or incorrect data on the receiving end.
- No Congestion Control: Unlike TCP, UDP does not have a congestion control mechanism. If there’s a bottleneck in the network, UDP will continue to send packets at the same rate which might lead to packet loss and degrade performance.
- No Error Correction: UDP doesn’t correct errors like TCP does. If a packet arrives with errors, UDP discards it. Therefore, the application must handle all error correction.
Now, let’s bring this into context with Redis. Redis is an open-source, in-memory data structure store primarily used as a database, cache, and message broker. By default, Redis uses TCP rather than UDP for its operations. While Redis could theoretically use UDP instead of TCP, due to several reasons it does not. Here are some key reasons why Redis elects to use TCP:
- TCP Guarantees Delivery: Given how critical data accuracy is for Redis operations, the guaranteed delivery that TCP provides outweighs the potential speed advantages of UDP. Redis doesn’t want to risk any data loss while transmitting.
- Error Checking Abilities: For similar reasons, Redis prefers the error checking abilities inherent in TCP. Data integrity being paramount in database operations, Redis would rather deal with the greater network overhead and latency that TCP incurs to ensure that the data received is accurate and uncorrupted.
- Congestion Control: Lastly, the lack of UDP’s congestion control can potentially lead to performance issues in networks experiencing high traffic. As a database that’s often subjected to heavy loads, Redis benefits from TCP’s inherent congestion control mechanisms.
In conclusion, while UDP might offer certain speed advantages for Disk Network I/O Operations, its other limitations make it less preferable for a server-client communication model especially when data integrity is necessary. Therefore, systems such as Redis choose TCP as it aligns more with their goals and requirements.Certainly, Redis is among the top choices when it comes to high-performance in-memory data storage systems. As a coder, you’d be interested to know that Redis does not use UDP (User Datagram Protocol). Primarily, Redis uses TCP (Transmission Control Protocol) for communication between clients and the server.
Redis is specifically designed to provide scalability within the realm of caching, serving as an effective way to alleviate database load (source). However, its way of achieving scalability lies with TCP rather than UDP. Applying other protocols such as UDP would not align with important goals and functionalities built into Redis, potentially impacting its performance and reliability.
Diving further into how this non-choice of UDP affects scalability aspects for Redis:
- Order and Reliability:
Remember that UDP is a connectionless protocol without built-in error-checking mechanisms. It doesn’t guarantee order or reliability, not even confirmation of receipt. On the contrary, however currently envisaged, Redis operations often need to be applied in a certain order to maintain consistency. For instance, pushing items onto a list using LPUSH needs acknowledgment for completion. This isn’t possible if Redis was to utilize UDP with no guaranteed deliverability. - Transaction Support:
Redis also supports transactions through MULTI/EXEC commands which provide an all-or-nothing form of operation execution to preserve data integrity. The lack of delivery assurance in UDP could mean that some commands within a transaction might be lost or arrive out of order, undermining the dependability of Redis transactions. - Data Integrity:
Accurate data transmission is crucial for Redis’ function as an in-memory storage system, where data loss may lead to serious consequences. TCP, on the other hand, has built-in error checking, makes retransmissions if necessary, thereby providing better reliability and data integrity compared to UDP.
A balance of speed and reliability tailored for in-memory operations is crucial for Redis to serve its purpose effectively. In line with this focus on balanced performance, the use of TCP over UDP becomes apparent, as TCP offers that crucial mix of speed and reliability essential for Redis’ processes.
Yet, despite these potential pitfalls, does this mean UDP is completely unusable for scaling? Not at all. We can look at QUIC Protocol, a transport layer network protocol developed by Google that operates atop UDP (source). QUIC delivers superior performance for connections that experience packet loss, making it advantageous for poor-quality network links.
However, utilising this protocol would require significant changes to Redis’ communication model which could affect platform stability and backward compatibility.
Overall, whilst UDP does have benefits, particularly in terms of resource efficiency such as lower latency and less bandwidth use, in the specific case of Redis these aren’t sufficiently robust or necessary to make UDP a viable protocol choice. Instead, the ability to offer ordered, reliable communication with integrated error checking provided via TCP, is far more compatible with the requirements of Redis supporting high-transactions and data-integrity reliant applications, ensuring Redis delivers as an efficient, robust in-memory database.
Remember that each technology has its use cases, and understanding the implications and trade-offs helps inform which protocols are most appropriate for given services and systems like Redis.
Here is a code snippet showing a basic interaction with Redis using TCP in Python using the ‘redis’ library.
import redis # create a connection to the localhost Redis server instance, by # default it runs on port 6379 redis_db = redis.StrictRedis(host="localhost", port=6379, db=0) # set a key/value pair redis_db.set('key', 'value') # retrieve and print the value for 'key' print(redis_db.get('key'))
As seen in the code above, there’s no explicit mention of TCP protocol since it is the default mode of communication used by Redis. TCP aims to ensure data correctness and order, making it fit for purpose for applications that Redis commonly powers.
For further delve into Redis and TCP/IP protocols, refer to the
Redis protocol documentation.Pulling all the vital pieces of information together, it’s clear that Redis doesn’t use UDP because of various reasons. Primarily, Redis makes use of TCP/IP as its network protocol.
Here’s the proof in simplifying terms:
Redis’ reliance on TCP:
TCP is inherently a connection-oriented protocol used by Redis, which facilitates an ordered data stream and retransmission of lost packets. This ensures that all commands are executed in the sequence they were given, and none gets overlooked or mishandled.
You can notice this by taking a look at how Redis manages client communications:
code
struct redisClient {
… // client state
int fd; /* Client socket. */
sds querybuf; /* Buffer accumulating client queries. */
}