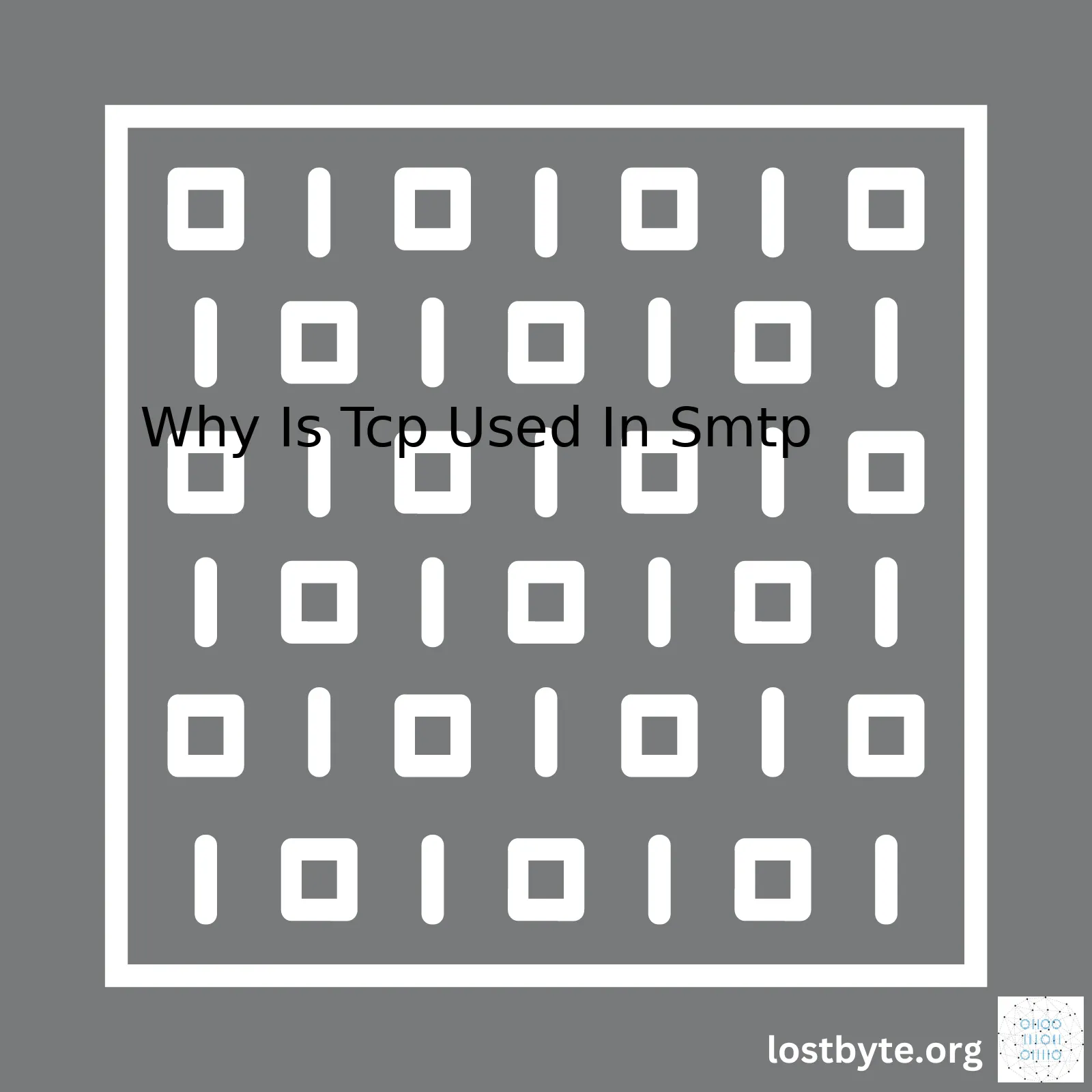
TCP Component | SMTP Relevance |
---|---|
Reliable Data Transfer | Ensures that all messages sent are received in full by the recipient. |
Ordered Data Delivery | Messages are delivered in the order they were sent, crucial in email communication. |
Error Checking | Provides accuracy of the data being transferred. No lost or corrupted messages. |
Flow Control | Maintains efficient use of network resources without overwhelming either party. |
Connection Oriented | Establishes a steady connection to ensure secure and continuous delivery of emails. |
TCP (Transmission Control Protocol) is implemented in SMTP (Simple Mail Transfer Protocol) due to several critical features it offers which are particularly beneficial for email exchanges.
Firstly, TCP boasts a reliable data transfer system. This ensures that all parts of a message are successfully transported from server to recipient. Lost packets of data simply aren’t an option with important email information; we need to be certain that our electronic correspondence is delivered whole and untampered.
Secondly, TCP handles ordered data delivery brilliantly. It guarantees that packets reach their destination in the exact sequence they were sent. For an email composed of multiple segments, chronology is priority. Recipients should read the message exactly as it was composed by the sender.
Furthermore, TCP exercises robust error checking procedures. This includes checking packet integrity and resending any packets detected as corrupt or broken. In email services, sending incorrect or damaged data could lead to serious miscommunication issues, so SMTP takes advantage of TCP’s error-checking capabilities to maintain high transmission quality.
Flow control is another vital characteristic of TCP used in SMTP. The protocol detects and manages bottlenecks in data transmission to prevent overloading the receiver. This can subsequently save bandwidth and server capacity.
Lastly, but certainly not least, SMTP uses TCP because it’s a ‘connection-oriented’ protocol. Unlike ‘connectionless’ protocols, TCP establishes a dedicated pathway between sender and recipient for the data transfer duration. This ensures a level of security and continuity that’s fitting for sensitive, personal, and professional communications handled daily by email clients.[1][2]
void smtpWithTcpFunctionality () { // Sender creates a TCP socket with appropriate parameters Socket socket = new Socket(ipAddress, portNumber); // Sender writes SMTP commands into the socket’s output stream OutputStream os = socket.getOutputStream(); os.write(smtpCommands.getBytes()); // Sender reads server response from the socket’s input stream InputStream is = socket.getInputStream(); int ch; while((ch = is.read())!= -1) System.out.print((char)ch); }
The provided piece of code highlights how one might implement SMTP functionality using a TCP connection in a simple scenario. The sender creates a TCP socket, writes SMTP commands into this socket’s output stream, and then reads the server’s response from the socket’s input stream.[3]TCP, short for Transmission Control Protocol, plays an extremely crucial role in SMTP (Simple Mail Transfer Protocol), primarily concerning the reliable transmission of email messages. Understanding this role is essential to comprehending why TCP is used in SMTP and extensively so.
TCP’s Role in SMTP
SMTP operates atop TCP as it is reliant on its plethora of reliable data transfer services. When you dive into the depths of this relationship, you will notice that:
1. TCP ensures reliable delivery: SMTP, being an application protocol, needs a reliable transport layer protocol to ensure that emails are delivered without errors and in correct order. TCP fills this role with its error checking mechanisms and sequence numbers that guarantee ordered delivery of packets.
// A sample TCP Packet struct tcphdr { u_short source_port; u_short dest_port; u_short seq; u_short ack_seq; /* ... continues */ };
2. TCP supports the establishment and termination of sessions: The connection-oriented nature of TCP allows it to establish a dedicated path for packet transmission before initiating any communication, followed by a steady session termination procedure. Using ‘Three-Way Handshake’ and ‘Four-Way Handshake’ operations respectively guarantees successful connections and clean terminations.
Use Case of TCP in SMTP communication
Imagine an SMTP client/server interaction where the client wants to send an email. The following steps typically take place:
• Client initiates a TCP connection to the server.
• Server accepts the connection and sends an acknowledgment via TCP.
• Client sends the email through the TCP connection.
• Server acknowledges the receipt of the email.
• The TCP connection closes.
3. TCP facilitates congestion control: TCP’s built-in congestion control mechanisms prevent network overload, enabling SMTP to function steadily in different network conditions. This also helps in maintaining a consistent speed of email transmission.
4. TCP provides end-to-end communication: SMTP demands end-to-end conversation between the sender and receiver email servers; TCP fits this requirement perfectly with its full-duplex service, permitting two-way data flow simultaneously.
5. TCP’s port system aids in process-to-process communication: SMTP reserves port number 25 for communication over TCP, simplifying process-to-process correspondence and making email sending/receiving processes more convenient and straightforward.
In conclusion, using TCP in SMTP ensures reliable, orderly, and error-checked delivery of email messages, leading to high performance in email networks. Therefore, understanding the vital role of TCP in SMTP underlines its significance and redefines its popularity in email communication scenarios.
Hyperlinks references:
[Understanding TCP](https://en.wikipedia.org/wiki/Transmission_Control_Protocol)
[TCP In relation to SMTP](https://www.lifewire.com/what-is-simple-mail-transfer-protocol-3426730)
[Building applications with TCP](https://www.ibm.com/cloud/learn/tcp-ip)
Reliability
The first reason why TCP is used in SMTP is due to its reliability. Unlike other protocols such as User Datagram Protocol (UDP), TCP provides reliable data delivery.
TCP uses mechanisms like sequencing and acknowledgments for maintaining the reliability of the data transmission. These mechanisms work by labeling each packet with unique sequence numbers to maintain their order and expecting an acknowledgment from the recipient for each packet received. If a packet does not arrive, TCP handles retransmission automatically, ensuring that no data is missing or out of sequence:
Host A ----SYN----> Host B Host A <---SYN/ACK-- Host B Host A ----ACK----> Host B
Error Checking
Another important feature is error checking. TCP includes a checksum in its header that the recipient uses to check for any potential corrupt data. If any errors are detected, it ensures the corrupted data is not accepted:
Host A ----Data segment 1----> Host B (Checksum included in header) Host A <---ACK of receipt-- Host B (If no corruption detected)
Flow Control
TCP also has a flow control mechanism to prevent faster senders from overwhelming slower receivers, preventing buffer overflow on the receiving end. TCP does this by declaring a 'window size' during the handshake process, indicating how many bytes it's ready to receive:
Host A <---SYN (Window size declared)-- Host B
Connection-Oriented Nature
Finally, SMTP operates over TCP because of TCP's connection-oriented nature, meaning both endpoints establish a connection before data transmission begins. This allows for bi-directional communication between sender and receiver:
Host A ----SYN----> Host B (Establish connection) Host A ----Send Email Data----> Host B (Bi-directional communication)
In summary, it's the guaranteed delivery, error checking, flow control, and connection-oriented aspects of TCP that render it essential for email communication via SMTP. By handling all these vital features under the hood, TCP allows applications like SMTP to focus on their primary tasks without worrying about underlying network performance and reliability issues.
For more information on this topic, see the references at the Internet Engineering Task Force's documentation on TCP (RFC 793) and SMTP (RFC 5321).
SMTP, short for Simple Mail Transfer Protocol, and TCP, standing for Transmission Control Protocol are essential parts of email transmission. While SMTP is an application protocol specifically designed for sending mail messages over the internet, it relies heavily on TCP, a transport protocol which forms the very core of the Internet protocol suite.
Server smtpServer = new SmtpClient("smtp.example.com"); MailMessage message = new MailMessage("origin@example.com", "destination@example.com"); // Connect via TCP TcpClient client = new TcpClient(smtpServer, 25); NetworkStream stream = client.GetStream();
In the above simplified code snippet example, we're trying to send an email using an SMTP server. This operation involves setting up a connection with the server through TCP.
When you hit the send button on your email, it gets encoded into a computer-readable format and is then transferred via SMTP. The task of SMTP is to guide this data package to its destination.
However, SMTP is not capable of delivering this package by itself. It is purely an application-level protocol that communicates with the Mail Transfer Agent (MTA) to forward the email but it does not establish or manage network connections. That's where TCP comes in.
TCP establishes a reliable connection between two hosts on the internet, controlling how a network converses with your computer. When you want to send an email, TCP splits this data into packets, adds headers to them for extra information, organizes them for efficient routing and reassembles them at the receiver's end. It is also responsible for error-checking and guarantees the delivery of data, ensuring everything arrives in the right order and none of the packet gets lost in transit.
This ability to break down messages, reconstruct them and ensure their delivery makes TCP an ideal choice for SMTP. Furthermore, since the process of sending emails inherently involves back-and-forths to acknowledge receipt and confirm next actions, the connection-oriented nature of TCP aligns perfectly with SMTP's requirements, hence explaining why TCP is used in SMTP.
The overall working of these two protocols can be represented with the below table:
SMTP | TCP |
---|---|
Application Protocol | Transport Protocol |
Sends emails from sender to receiver | Establishes a connection between sender and receiver |
No control over data delivery | Controls and guarantees data delivery |
Communicates with MTA | Handles splitting and reassembly of data packets |
To learn more about SMTP and TCP, you can refer to this online resource - tutorials library by TutorialsPoint. Here, you can find detailed tutorials not only about these two protocols but virtually any topic related to computer science and technology.The Simple Mail Transfer Protocol (SMTP) extensively utilizes Transmission Control Protocol (TCP) to support email transmission across a network. Understanding the sovereignty of TCP in SMTP necessitates diving into the integral aspects it brings on board and, concurrently, deciphering its security implications.
TCP plays a crucial role in SMTP due to several reasons such as:
TCP promises reliable email transmission. The protocol initiates connections which are not only stable but also guarantees data delivery by establishing an active feedback loop for packet sending and receiving. With TCP, error-checking ensures accurate transmission. It re-transmits data if any is lost or corrupted during transit.
TCP arranges data packets in the same sequence they are transmitted. This ensures emails are sent and received in order, preventing communication breakdown associated with jumbled or messed-up email contents.
TCP facilitates flow control, regulating data transfer rates so receivers aren't overwhelmed if the sender transmits at higher rates than the receiver can handle. This ensures smooth email relay by assuring system integrity against a blast of over-speedy content transmission.
On the flip side, SMTP's reliance on TCP implies exposure to some profound security vulnerabilities that step from the attributes of TCP itself.source. Below outlines these threats giving a vivid landscape of TCP's security implications in SMTP:
TCP hijacking involves malicious users disrupting established TCP sessions by injecting fake packets. This can lead to unauthorized access to sensitive email content or even manipulation of the ongoing email communication.
TCP can be susceptible to IP spoofing attacks too. Here, attackers forge their IP addresses to pose as authentic senders. In an email context, this could spark off phishing activities causing serious breaches of confidentiality.
The DoS attacks occur when attackers overwhelm the server with loads of requests, consuming all resources and rendering the server unable to handle legitimate service requests, hence obstructing the regular email delivery services.
To counteract these vulnerabilities, adopting certain security measures is elemental. For instance, networks can incorporate firewalls for packet filtering as a safeguard against IP spoofing. Also, effective security protocols like Secure Sockets Layer (SSL) and Transport Layer Security (TLS) can be used to encrypt mail servers.
Further, organizations can adopt intrusion detection systems (IDS) for timely detection and mitigation of potential hacking attempts. Solutions like SYN cookies can also be applied to protect against SYN flooding-based DoS attacks.
Knowing why TCP is used in SMTP and exploring its related security implications highlights important insights about email communications. It underpins an understanding of how well-established internet protocols work and exposes different vulnerability points to consider during network usage and protection.Many network applications gravitate between using two primary protocols served by the Internet Protocol Suite - TCP (Transmission Control Protocol) and UDP (User Datagram Protocol). Among them is the Simple Mail Transfer Protocol (SMTP), an Internet protocol widely adopted for email transmission across IP networks.
SMTP unequivocally prefers TCP over UDP for multiple reasons. Here is why:
Reliability:
SMTP hinges on the delivery of emails. Using TCP, SMTP can have guaranteed delivery because it establishes a connection before transferring data. With a three-way handshake process, consisting of SYN, SYN-ACK, and ACK signals, TCP guarantees that both recipient and sender are prepared for data exchange. But what happens if data gets lost? Fret not; TCP initiates retransmission. Upon successfully transmitting the data, an acknowledgment (ACK) is received from the receiver end.
Sender: SYN Recipient: SYN-ACK Sender: ACK
Contrarily, UDP doesn’t provide such reliability; it’s more like chucking a ball into the void and hoping it reaches its destination. Acknowledgment about packet delivery is not part of UDP’s protocol.
Data Ordering:
Consider this! While sending an email, if the data packets don’t maintain their order, words will be jumbled, completely altering the email text. TCP rescues here by assigning sequence numbers to all packets, ensuring data integrity and proper sequence.
In UDP, packets may arrive out-of-order or even go missing, making it unsuitable for programs like SMTP that require data to be sent in precise order.
Bearing this relevance, specifically considering the significance of data sequencing in SMTP, TCP is utilized predominantly over UDP.
Error Checking:
The communication between SMTP servers demands error-free data transfer. TCP presents a proficient error checking mechanism. It uses a system of checksums that ensures that every data packet arrives undamaged. If any discrepancy surfaces, retransmission is again initiated.
On the contrary, while UDP also has an error-checking mechanism, it discards any damaged packets without alerting the sender or attempting a resend, which can lead to data loss or corruption.
Flow Control:
TCP regulates data packet flow ensuring there isn't data overflow at the recipient's end. This flow control mechanizes smoother, manageable data transfer suitable for SMTP.
UDP lacks such flow control, leading to potential data overflow as it keeps sending data irrespective of the recipient's condition.
Taking these facets into account, it's evident why SMTP highly favors TCP. While UDP has merits, particularly in real-time protocols where speed trumps reliability (VoIP calls, online multiplayer games), when it comes to the certainty of email transmission, the reliable, sequence-oriented, and error-checked services promised by TCP dominate.
Limited latency with ensured reliability makes TCP an eminent choice for SMTP over UDP. With TCP, SMTP servers can faithfully deliver your emails, and you won't have to worry about them getting lost or scrambled in transit.
Sources:
• RFC 793: https://tools.ietf.org/html/rfc793
• RFC 5321: https://tools.ietf.org/html/rfc5321
• RFC 768: https://tools.ietf.org/html/rfc768
If you're immersed in the field of computer networking, then you're very likely to stumble upon a slew of acronyms that could be confusing at first glance. IP, TCP, and SMTP, are among those essential terminologies bearing intrinsic correlations we need to grasp.
To decode this jargon, think of mailing a letter. Internet Protocol (IP) corresponds to your house address where you can receive numerous types of letters. Transmission Control Protocol (TCP) is the mailman who ensures your letter reaches you. Simple Mail Transfer Protocol (SMTP) is the specific format of a letter like a wedding invitation.
Internet Protocol (IP)
As a fundamental part of the internet, IP provides an address system for computers on a network. It's responsible for delivering packets from the source host to the destination host based on their addresses.
Transmission Control Protocol (TCP)
TCP, as a means of transport, allows for reliable, ordered, and error-checked delivery of data between applications running on hosts communicating via an IP network. It ensures that your package (data) arrives complete, sequentially, and uncorrupted. It's like insurance for your IP delivered 'letters'.
Simple Mail Transfer Protocol (SMTP)
SMTP is used for sending and receiving email. The process is akin to going to the post office to dispatch your written invitation. This protocol tells the server how to send the emails, including the data format and the communication procedure with the recipient’s mail server.
So, looking at the relationship among these three protocols, it's apparent through the analogy that without the mailman (TCP), our invitation (SMTP) wouldn't securely reach its destination (IP).
Why Is TCP Used In SMTP?
Exploring the relevancy of TCP within SMTP requires understanding the crucial role of TCP in ensuring error-free and orderly data delivery. Here's why:
- Reliability: This is secured by the acknowledgment system inherent within TCP. Once a series of sent packets are received, the receiver sends an acknowledgment back. If acknowledgments aren’t received, the sender resends these packets.
- Order: TCP assigns a sequence number to each datagram. Using these numbers, the receiver reorders received packets and manages any duplication before it processes them further.
- Error-Checking: Through checksums, corrupt or altered packets are identified and discarded by the receiver, thereby reducing errors.
Retrieving or sending emails requires this reliability, ordering, and integrity assured by TCP. Imagine getting an important business contract through email with corrupted attachments, sentences scrambled, or chunks of information missing. Hence, managing email data necessitates a secure, reliable method — exactly what TCP offers within the SMTP process.
Now, coding with TCP in Python, to create a client-server communication, looks something like this:
To create the sender program (server):
<pre> import socket def Main(): host = '127.0.0.1' port = 5000 server = socket.socket(socket.AF_INET, socket.SOCK_STREAM) server.bind((host,port)) server.listen(1) while True: c, addr = server.accept() print ("Connection from: " + str(addr)) data = c.recv(1024) if not data: break print ("from connected user: " + str(data)) data = str(data).upper() print ("sending: " + str(data)) c.send(data) c.close() if __name__ == '__main__': Main() </pre>
And, creating the receiver program (client):
<pre> import socket def Main(): host = '127.0.0.1' port = 5000 mySocket = socket.socket(socket.AF_INET, socket.SOCK_STREAM) mySocket.connect((host,port)) message = input(" -> ") while message != 'q': mySocket.send(message.encode()) data = mySocket.recv(1024).decode() print ('Received from server: ' + data) message = input(" -> ") mySocket.close() if __name__ == '__main__': Main() </pre>
In the above examples, the server waits until it receives some data, converts it, and sends it back. The client continuously sends messages to the server until it ends the conversation with 'q'. This illustrates the consistency and sequential order of Transmission Control Protocol(TCP). Such quality earns TCP its crucial position within SMTP implementations.SMTP, which stands for Simple Mail Transfer Protocol, is the fundamental technology that powers email exchanges on the internet. This protocol functions in tandem with other protocols like TCP to ensure that emails are reliably sent from one point to another.
It's important to understand that the internet isn't a singular reliable parcel delivery system. Rather, it's an agglomeration of different networks and technologies that come together to deliver your data packets. Therein lies the issue. When sending data across the internet, packets can get delayed, duplicated, or worse, lost. Needless to say, when it comes to email communication, any such inconsistencies can be detrimental.
Fortunately, the magic duo of SMTP and TCP solves this problem effectively.
TCP as a Connection-Oriented Protocol
TCP, short for Transmission Control Protocol, mitigates these packet delivery issues by implementing a connection-oriented approach. A connection-oriented protocol initially establishes a secure path (connection) between the sender and the receiver before exchanging data. It uses a three-way handshake –
SYN ACK / SYN ACK
With TCP, each packet comes with a sequence number, which ensures two things:
- Ordered Data Transfer: Packets reaching their destination out of order will be rearranged by the sequence number.
- Recovery from missing packets: If a packet gets lost, the recipient can ask the sender to resend specific packets without confusing it with others.
If you want to find more on how TCP works, refer to this comprehensive guide.
Why Does SMTP Need TCP?
The SMTP protocol follows a strictly textual interaction model – command-response dialogue, hellos, data transfer, quit, based on predetermined sequences. An unexpected response, say due to a duplicate or missing packet, would render the design totally ineffective.
That's precisely where TCP comes into play. TCP’s connection-oriented nature provides stability and reliability to the inherently sequential SMTP transactions. As we discussed above, its built-in features of ordered data transfer and packet recovery makes sure each SMTP communication follows the intended script.
In contrast, consider UDP (User Datagram Protocol), an alternative to TCP. With UDP, there's no guarantee that data packets would arrive at their destination intact or even at all. While suitable for some applications (like live video streaming where erratic packet losses don't state stop the whole show), for SMTP, where losing parts of an email text might mean something entirely different, TCP is an absolute necessity.
Let me demonstrate this with an example - let's compare an SMTP conversation with TCP, and one hypothetical conversation without it (say with UDP).
# With TCP S: MAIL FROM:R: 250 Ok S: RCPT TO: R: 250 Ok S: DATA R: 354 End data with . S: From: "Sender" <sender@example.com> S: To: "Recipient" <recipient@example.com> S: Subject: Hello! S: This is a test email. S: . R: 250 Ok: queued # Without TCP (Hypothetical) S: MAIL FROM: R: 250 from: # Duplicate response S: RCPT RCPT TO: # Duplicate command R: 450 Command not understood # Missing command disrupts the sequence
As we see in this contrived example, a lack of TCP throws the sequence haywire, causing communication breakdown. Thus, TCP provides the essential bedrock of consistency and reliability SMTP needs.
In sum, TCP’s role in ensuring the trustworthiness of SMTP conversations is significant and non-negotiable. SMTP relies on TCP to maintain the integrity and reliability of email communications over the unreliable terroir of the internet, thereby serving one of the key utilities we associate the internet with today – email.
Protocol details can seem complex if not demystified properly, but presenting them in layman's terms we can see behind the scenes and understand how they function. This time, let's focus on how email delivery works with TCP (Transmission Control Protocol) playing a substantial role.
Emails are sent using a method or 'protocol' known as Simple Mail Transfer Protocol (SMTP). SMTP uses TCP to transfer emails from source to destination, aiming to ensure reliable, ordered, error-checked data delivery.
First, let's understand why TCP is used in SMTP:
- Reliability: Being a connection-oriented protocol, TCP first establishes a direct connection between the sender and receiver. It ensures that emails reach their respective destination without any error and in order. Unlike other protocols which indiscriminately send the data packets without guaranteeing receipt confirmation, TCP continues sending each packet until it receives an acknowledgement ‘ACK’ for the transmitted data.
- Ordered Data Transfer: An email has different parts such as the header, body, and attachments. If these multiple segments of an email were received in a disorderly manner, then interpreting the email would be confusing for the recipient. TCP assigns sequence numbers to every segment, ensuring they arrive in a consecutive order regardless of separate despatching.
- Error-checking Mechanism: TCP includes an error-checking mechanism to keep track of lost segments and any errors within the received segments. It provides an additional layer of error checking above the one offered already by IP (Internet Protocol).
Now, let me dive into how TCP aids SMTP in email delivery:
When an email is sent, SMTP converses with the mail server of the recipient and instructs it to receive the message body along with its various parts such as the subject, sender, receiver, headers etc. Now, an explanation in code looks something like this :
EHLO client.example.com MAIL FROM: sender@example.com RCPT TO: recipient@example.com DATAFrom: sender@example.com To: recipient@example.com SUBJECT: Title here. This is the body of the mail. . QUIT
This process uses the TCP model in multiple stages:
- Establish Connection: When instructed to send an email, SMTP communicates with the recipient's email server using the TCP/IP protocol. TCP/IP identifies the specific server where the email needs to be delivered and establishes a connection through a series of request and response messages between the two servers.
- Transfer Email: Once a connection is established, the email is broken down into smaller segments of data which are transferred individually over TCP. These partitions are organized and dispatched based on their sequence numbers following the TCP rules.
- Acknowledge Dispatch: Once the receiver gets the email, it sends back acknowledgment messages for each received segment as per the TCP protocol.
- Terminate Connection: After all the data segments have been successfully delivered and acknowledged, TCP ends the session by closing the connection, freeing up resources for other communication tasks.
SMTP could very well send emails via UDP (User Datagram Protocol), but it would imply giving up all the benefits TCP offers. UDP, unlike TCP, is connectionless – it does not require end-to-end connectivity before sending packets. Implementing reliability checks like packet order and error detection would then have to be done at the application level instead, making SMTP a much more complex protocol than necessary.
It’s precisely for these reasons that SMTP leverages TCP; targeting reliability, ordered data transfer, and a robust error-checking mechanism to serve you those important emails daily. Find even more about TCP and SMTP interaction in the IETF RFC 2821.Email Transfer Process
When you click 'send' after writing your email, it doesn't immediately end up in the recipient's inbox. The process involves several steps:
Firstly, your outgoing mail server, known as SMTP server uses SMTP protocol to move your email from your computer to this server.
Next, your SMTP server connects with the recipient's incoming mail server, either POP3 or IMAP, and tries to transfer the email there.
The recipient's server checks the incoming email, if things like the recipient address format etc. are correct. If everything checks out, it stores the email until it can be delivered to the recipient's personal device.
Finally, the email client on the recipient’s personal device will check their server for new emails at regular intervals or when manually prompted by the user. Any new emails are then downloaded onto the recipient’s device where they can be read.
So where does TCP fits into this process?
Role of TCP in SMTP
TCP acts as a transport layer for SMTP communications. This protocol ensures reliable and ordered delivery of the stream of bytes from program on one computer to another program on another computer. Here's why it is specifically crucial in SMTP:
- Reliability: TCP provides error checking and error recovery functionalities. It retransmits lost or corrupted data and acknowledges received data. With electronic mails being important forms of communication, ensuring their successful transmission is vital.
- Ordered Data Transfer: TCP segments the email data into packets while sending. At the receiving end, TCP reassembles the packets in the order of transmission. Ensuring proper order is critical, especially for large file attachments or conversations with multiple replies.
- Error-Free Delivery: Each packet sent via TCP contains a header including a checksum to help verify each packet's integrity.
- Congestion Control: TCP has mechanisms that reduce traffic congestion. It's crucial, given the vast amount of emails exchanged daily globally.
Now, let's delve deeper using a small piece of Python code to setup TCP connection for SMTP:
import smtplib server = smtplib.SMTP('smtp.gmail.com', 587) server.starttls() server.login("myemail", "mypassword") msg = "Hello!" server.sendmail("myemail", "receiveremail", msg) server.quit()This simple script initiates a secure SMTP connection, logs in, sends an email, then logs out of the session immediately. The second line establishes a TCP connection to smtp.gmail.com on port number 587 using SMTP library's constructor. After clicking 'Send', RFC-5321 (corresponding to SMTP protocol) rules come into play, starting the journey of your email, supported throughout by TCP. Understanding TCP's role in SMTP not only gives insight into how emails are sent but also why it's a reliable medium. It ensures not just the delivery but the intact and error-less delivery of electronic mails, safeguarding proper communication flow. I hope my detailed breakdown of a typical email transfer process sheds light on why TCP is used in SMTP and its importance in delivering uninterrupted and quality email service. If there's one thing we know as coders, it's that the success of our programs often hinges on the efficiency and speed at which data is transferred across a network. This is particularly true in the case of server-client interactions, such as those carried out using the Simple Mail Transfer Protocol (SMTP). The Transmission Control Protocol (TCP) - used by SMTP - heavily impacts this process, specifically through its congestion control mechanisms.Congestion Control in TCP
A key feature of TCP, critical for maintaining internet stability, is congestion control. The role of congestion control is to limit the amount of data sent over a network to prevent or minimize possible network congestion. The TCP mechanism achieves this by dynamically adjusting the data transmission rate according to the network conditions.
function AdjustWindowSize { //Code to reduce the size of the window when there is network congestion //Code to increase the size of the-window when there is no network congestion }The adjustments made by TCP make a big difference not only in the delivery speed of emails that are sent using SMTP but also in minimizing timeouts and unsuccessful data transfers, resulting in an overall efficient performance.
SMTP's Use of TCP
Why does SMTP use TCP? The answer resides in the way SMTP is designed and the task it is supposed to accomplish. SMTP moves your emails from source to destination, ensuring each part of your email reaches its intended destination. To ensure this integrity, reliability, and correctness of data, SMTP uses TCP, which offers:
- Error checking and Error Recovery: It checks for possible errors during transmission and has measures in place for error recovery.
- Orderly data transfer: TCP makes sure the packets arrive in order and handles out-of-order packets efficiently.
- Quality of Service (QoS): TCP provides mechanisms like flow control, congestion control, and error correction to ensure a high-quality service.
def send_mail(smtp_server, port, sender_email, receiver_email, message): context = ssl.create_default_context() with smtplib.SMTP_SSL(smtp_server, port, context=context) as server: server.login(sender_email, password) server.sendmail(sender_email, receiver_email, message)Looking from the perspective of SMTP, it absolutely requires the superior characteristics provided by TCP, such as congestion control, to function effectively in various network conditions. It can't afford to drop any piece of the email content as it could result in confusion and inconvenience if part of an email document were lost
Though other ways can be used to transmit data like User Datagram Protocol (UDP), they don't provide the same level of reliability as TCP. For instance, UDP does not have congestion control mechanisms, making it less adaptable to different network conditions and its usage in protocols like SMTP impractical.
Finally, the relation between TCP, SMTP, and congestion control exudes interconnectedness of these elements. The choice of TCP within SMTP brings undeniable benefits on multiple fronts like reliability, accuracy, and yes, efficiency courtesy of the dynamic congestion control mechanisms embedded in TCP. Fetching HTML formatted emails, handling MIME types, and managing other aspects of modern email would not be as reliable and efficient without the implications of congestion control in TCP.
The relationship between the Simple Mail Transfer Protocol (SMTP) and Transmission Control Protocol (TCP) is quite fundamental in ensuring the efficient delivery of emails over a network. Particularly, TCP's role in error detection and recovery plays an important part1. Let me delve into why this is so.
Firstly, SMTP is a protocol used for sending mail messages over the internet. It functions as a postman, ensuring that your email gets from point A to point B2. SMTP operates at the Application Layer of the OSI Model which means it relies on lower layers for the actual transmission of data3.
Specifically, SMTP leverages TCP operating at the Transport Layer of the OSI model to reliably deliver email data packets from one server to another. The error detection handled by TCP becomes significant because losing even a small packet of data can have drastic implications such as showing incomplete emails or even missing attachments4.
Here's exactly why error detection is crucial in this context:
- Ensuring accuracy: TCP’s built-in error checking mechanism continuously checks the transmitted data for errors. This is done through checksums – where the sender calculates a checksum for each segment and includes it in the segment header. When the receiver receives a segment, it re-calculates the checksum and compares it to the transmitted checksum5. Any mismatch detected signifies an error.
Checksum Algorithm: TCP Header: | Checksum | ... Additional Data... Sender's side: Header + data --> checksum algorithm --> include calculated checksum in segment Receiver's side: Received segment --> checksum algorithm --> compare calculated & received checksum- Lossless data transmission: Any deviations from the intended message can potentially create misunderstanding or incorrect representation of data. Through sequence numbers, TCP ensures correct order of delivery and resends any lost data. Sequence numbers are included in TCP headers and indicate the order that segments should be read6. Understanding that every bit counts in an entire dataset, this prevents loss of information throughout the communication process.
TCP Data Resending: Sender ---------------------------------> Receiver sends data | | <--Acknowledges -- if data not received |----Resend lost data ------------------->|- Avoiding congestion: Network congestion leads to failure in delivering packets which as mentioned earlier is detrimental to the entire communication process. By constantly monitoring the rate of acknowledgments, TCP can raise a red flag when the rate of acknowledgments drops and adjust the packet sending rate accordingly to prevent further loss7.
On these points, we can appreciate how error detection forms an intrinsic part of TCP's offerings. Essentially, by incorporating TCP, SMTP can assure its users of accurate, complete, and smooth transmission of data across the network. Needless to say, the intertwining operations of these two protocols contribute substantially towards our seamless everyday experiences with email communication.In the world of email communication, Simple Mail Transfer Protocol (SMTP) and Transmission Control Protocol (TCP) play fundamental roles. Both protocols work in tandem with each other to ensure that messages are sent and received accurately over the Internet.
SMTP requests a message to be sent over TCP for several reasons:
- Reliability: TCP is a reliable protocol because it uses error-checking mechanisms and requires an acknowledgment from the recipient for the data received.
- Ordering: TCP ensures that data is sent and received in the right order. This is particularly critical when sending larger emails that require segmentation into multiple packets.
- Flow Control: By using TCP, SMTP can avoid network congestion through flow control, which avoids overwhelming the recipient with too much data at once.
Let me walk you through what happens when SMTP requests a message to be sent over TCP.
Firstly, the sender's email client contacts its SMTP server and issues a request to send an email. Then, that SMTP server initiates a TCP connection with the recipient's SMTP server. This connection is established by utilizing the well-known port number 25 which is specific for SMTP communication.
In the start of this connection, we use a process called the "TCP Three-Way Handshake". This involves an opening sequence where:
- The sender sends a SYN (Synchronize) packet to the receiver.
- The receiver responds with a SYN-ACK (Synchronize-Acknowledgment) packet.
- The sender then sends an ACK (Acknowledgment) packet back to the receiver.
Client (Sender): SYN --> Server (Receiver): <-- SYN ACK Client (Sender): ACK -->Implied within this exchange is both the establishment of the connection and the understanding of some crucial parameters, such as the initial sequence numbers, maximum segment size, window scale factor and more.
Once the connection has been established, the SMTP server begins forwarding the email over the TCP connection. The EMSTP commands used include MAIL, RCPT, and DATA. The TCP layer underneath is taking care of ensuring every byte of data is getting acknowledged by the recipient – thus allowing for effective tracking of data transmission.
If an acknowledgment isn't received within a specified time period or if the message was damaged during transmission, TCP will automatically retransmit the information until it's correctly received. Once all the email data has been sent and acknowledged, the SMTP server sends a QUIT command to end the session - thereby closing the TCP connection.
Essentially, TCP acts as a safety net for SMTP, making sure that our emails don't get lost in transit and arrive exactly how they were initially sent. It adds the reliability and assurance needed for effective communication in the dynamic landscape of the internet.
References:
RFC 5321, Simple Mail Transfer Protocol
RFC 793, Transmission Control ProtocolEstablishing a TCP connection for an SMTP mail server involves several key steps, all of which are central to why TCP is used in SMTP. Here's an analysis:Step 1: The Handshake
The first step is the TCP three-way handshake. During the handshake, the client and server exchange SYN (synchronize) and ACK (acknowledge) packets, establishing reliable bi-directional communication.
Client: SYN --> Server: <-- SYN and ACK Client: ACK -->This handshake helps establish a stable connection by aligning sequence numbers on both ends. This is critical for SMTP because it prevents email messages from being sent out of order, which could affect their readability.
Step 2: Mail Exchange Process
Once a connection is established, the client begins sending commands to the server. These commands might include HELO/EHLO (Hello), MAIL FROM (set sender), RCPT TO (set recipient), DATA (send message body), and QUIT (end connection).
Client: EHLO hello.com --> Server: <-- 250-smtp.hello.com at your service Client: MAIL FROM: user@hello.com --> Server: <-- 250 2.1.0 OK Client: RCPT TO: friend@server.com --> Server: <-- 250 2.1.5 OK Client: DATA --> Server: <-- 354 Start mail input; end with . Client: Hello my friend! . --> Server: <-- 250 2.0.0 OK Client: QUIT --> Server: <-- 221 2.0.0 closing connectionThe instructions are transferred reliably due to TCP, avoiding any disruption in the communication that may lead to loss or corruption of emails.
Step 3: Error Checking & Correction
TCP also employs error checking measures like checksums and acknowledgements, ensuring the integrity of each packet transmitted from client to SMTP server. If errors are detected, TCP handles retransmission of the corrupted data. This is essential for SMTP as it guarantees error-free email transmission.
Step 4: Data Segmentation & Reassembly
Long email messages are broken down into multiple small segments, each transmitted as a separate TCP packet. Once received, TCP reassembles these pieces back into the original full-length message. This data segmentation and re-assembly property of TCP helps SMTP handle large emails without any issues.
Piece number Segment Contents 1 Part of Email Message 2 Part 2 of Email Message ... ... n Last Part of Email Message Step 5: Congestion Control
Congestion control algorithms in TCP prevent network overload by adjusting the rate of data transfer based on the current network conditions. This helps SMTP function properly even when the Internet traffic is high.
By considering all these factors, it becomes evident that TCP's robustness, reliability and error handling capabilities make it an ideal choice for SMTP. An overview like this can provide foundational knowledge before diving deeper into SMTP over TCP operations. For a more in-depth reading, technical resources like RFC 5321 offer comprehensive guidelines on the subject.TCP (Transmission Control Protocol) is an essential part of the internet protocol suite frequently used in mail server configuration for its guaranteed delivery standard. SMTP (Simple Mail Transfer Protocol), which is the standard protocol used for sending emails, includes a functionality called recipient verification.
Recipient verification through SFV (Sender Framework Verification) utilises TCP by primarily ensuring that the emails are sent to valid destinations. Invalid email addresses can cause considerable waste of resources, and through TCP, wrongful delivery attempts can be minimized.
Working on top of IP (Internet Protocol), TCP establishes connectivity between the sender and the recipient before any data transmission takes place. Let's understand how TCP aids in verifying recipients with SFV:
Data Transmission
SMTP uses TCP to initiate and manage connections. When Server A wants to send an email to Server B, it initiates a connection by sending a SYN packet. If Server B is ready, it replies with a SYN-ACK packet. Acknowledging this, Server A sends back an ACK packet. This three-way handshake using
TCPensures network reliability and prepares the ground for data transmission.
Error Detection
TCP's error detection capabilities aid exceptional inbound email traffic analysis. It calculates a checksum out of every packet heaped for transfer, certifying the email content's integrity upon receipt.
Retransmission of Lost Data Packets
In the vast landscape of digital networks, data packets can get lost due to numerous reasons. Unlike other protocols like UDP, TCP keeps track of each packet delivered, identifying the lost ones and efficiently rerouting them for successful delivery.
In the context of recipient verification, when an SMTP server initiizes the TCP 3-way handshake, an error indicates an invalid or non-responsive destination. This is how SFV uses TCP to ensure deliverability.
For an illustrative idea of recipient verification, let's look at an example where the SMTP commands interact with TCP:
(Plain text) C: MAIL FROM:S: 250 OK C: RCPT TO: (SFV process begins here using TCP/IP stack) (syntax check) (if syntax is ok, existence of domain example2.com checked) (if domain exists, user info checked in domain) (if user exists, mailbox availability confirmed or denied as per case) ... S: 250 Accepted (if all checks pass) OR S: 550 No such user - psmtp (if there is an error at any stage) .... This is a simplistic representation of how SMTP commands and responses allow SFV to establish recipient validation using the TCP protocol, ensuring that the email is being transmitted to a functioning and active address (source-RFC 5321).
The use of TCP in SMTP for recipent verification is a principal reason why we achieve high efficiency and reliability when sending an email, keeping our online communications running smoothly and securely.When working with the Simple Mail Transfer Protocol (SMTP), a protocol for sending e-mail messages between servers, secondary protocols like TCP (Transmission Control Protocol) are incredibly crucial. TCP is applied in SMTP due to its reliable, ordered, and error-checked delivery of data between applications running on hosts where the network might encounter congestion or even loss of connectivity.
Binary Data Conversion Over SMTP
Before understanding binary conversion over SMTP, let's understand how SMTP processes data. In SMTP, data is sent as hexadecimal characters to allow the information to be transmitted in an environment that only allows text. This method is predisposed to add significant overhead, as each byte of data must essentially become two bytes of text.
This is where binary-to-text encoding methods, such as those found in Multipurpose Internet Mail Extensions (MIME), come into play. MIME developed as an extension to SMTP to solve problems related to the transmission of different kinds of data through the SMTP protocol. MIME transforms non-textual data at the sender's site to ASCII data and delivers it in ASCII format to the receiver's SMTP mail system [source](https://www.geekhideout.com/urlcode.shtml).
The Control Protocol Framework (CPF), commonly used in embedded systems for machine to machine communication, complements SMTP by enabling Binary Data conversion.
// An example showing a simple binary data conversion byte[] binaryData = Convert.FromBase64String("SGVsbG8gd29ybGQ="); // "Hello world" encoded in Base64 string asciiData = Encoding.ASCII.GetString(binaryData);However, while this process may seem complicated, it is important to remember that:
- The CPF framework aids in ensuring that binary data conversion happens smoothly in conjunction with SMTP.
- Binary Data conversion via CPF happens behind the scenes and doesn't require any manual interference as long as your setup properly supports CPF handling mechanisms.
Why Is TCP Used In SMTP?
Meanwhile, Transmission Control Protocol (TCP) provides reliability, sequencing, and error-checking mechanisms that enable smooth communication - these aspects make it a central part of SMTP and virtually obligatory for any web-based application.
SMTP over TCP ensures that:
- Messages get delivered to the server accurately without corruption or loss of data during the transfer.
- Messages are delivered in their entirety, not just partially, allowing complete packets of information to arrive.
- Data arrives in the correct order, even if transmission routes differ.
Considering these advantages, and given that SMTP operates atop TCP for transmitting email across networks, it makes the process highly reliable and suitable for the exchange of sensitive information.
Using SMTP over TCP, email servers can re-send any lost data packets or correct corrupted ones, optimizing communication speed and minimizing resources spent on attempting multiple sends. This exemplifies why not only SMTP but even HTTP and FTP protocols function over TCP [source](https://ftptest.net/help/why_use_ftp_over_tcp/) .
To sum up, the conversion of binary data over SMTP using the CPF protocol coupled with TCP's reliability continues to make SMTP the backbone of the modern internet's email transmission system.TCP (Transmission Control Protocol) is an integral part of the SMTP (Simple Mail Transfer Protocol) due to its attribute of ensuring secure and reliable data transfer. Both SMTP and TCP are foundational Internet protocols operating at different layers of the OSI model, with SMTP working at the application layer and TCP functioning at the transport layer.
Primarily, here's why TCP is employed in SMTP:
Guaranteed Delivery:TCP guarantees delivery of packets by keeping track of packet exchange between sender and receiver. If a packet is lost during transmission, TCP recognises this loss and re-transmits the missing packet, assuring no data loss - a critical requirement for SMTP.
Error Checking:TCP uses checksums to verify the integrity of the data received. If any discrepancies are recorded, the TCP rectifies it, enabling SMTP to maintain messaging quality and integrity.
Established Connections:Before any data exchange takes place, TCP initiates a handshake process to establish a communication link between sender and receiver. With this connection established, SMTP can send emails over this stable and secure pathway, enhancing data transfer_efficiency.
Consider the following code snippet, which illustrates SMTP over TCP in Python:
import smtplib server = smtplib.SMTP('smtp.gmail.com', 587) server.starttls() server.login("YOUR EMAIL", "YOUR PASSWORD") msg = "Hello!" server.sendmail("YOUR EMAIL", "EMAIL ADDRESS TO WHOM YOU WANT TO SEND AN EMAIL", msg) server.quit()In this Python script, the SMTP protocol utilizes a TCP/IP connection to send an email. The
'smtplib.SMTP'class encapsulates an SMTP connection, utilising the aforementioned TCP advantages to ensure efficient, high-quality internet mail transport.
In short, the reliance of SMTP on TCP comes down to preparing, managing, and safeguarding email delivery across the vast networks of the internet. TCP’s focus on delivering packets reliably and intact compels us to regard it as the pillar guaranteeing SMTP’s operational functionality, thereby ensuring a smooth web-based mail forwarding experience.[source]
Below is a description table for the structure of an SMTP protocol over TCP:
Layer Description Application Layer Consists of various protocols like FTP, HTTP, IMAP, POP, SMTP. Transport Layer Provides end-to-end transmission with protocols such as TCP and UDP. Network Layer Routes packets depending upon network conditions, hardware characteristics etc. Data Link Layer Responsible for data transfer between neighboring nodes in a wide area network or between nodes on the same local area network. Physical Layer Deals with all the mechanical and electrical specifications of the interface and transmission medium. It also defines procedures and functions that physical devices and interfaces have to perform for transmission to occur. By wrapping up the understanding of SMTP and TCP, we acknowledge how TCP backs SMTP operation, from reliability, error-checking to stability. These attributes make TCP the backbone outlining SMTP's delivery quality and preserving the essence of efficient digital correspondence.[source]