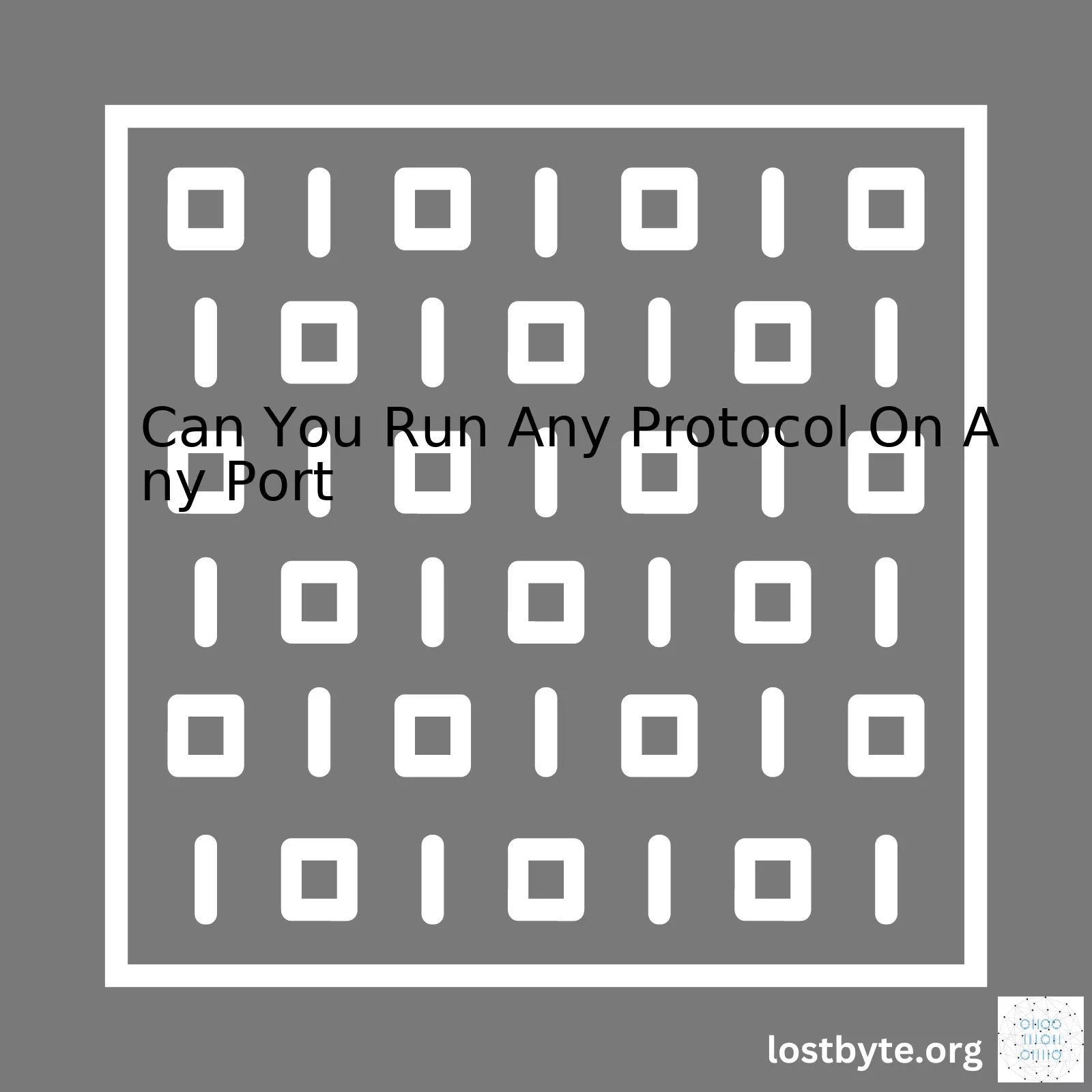
Protocols and ports play a fundamental role in network communication. While it’s technically feasible to run any protocol on any port, that doesn’t mean it’s practical or advisable.
Summary Table: Can You Run Any Protocol On Any Port?
Protocol/Port Operation | Feasibility | Practicality |
---|---|---|
TCP Protocol on HTTP Port (Port 80) | Possible | Commonly Used |
UDP Protocol on DNS Port (Port 53) | Possible | Commonly Used |
HTTP Protocol on Random High-Numbered Port | Possible | Not Advised |
SSH Protocol on Email Port (Port 25) | Possible | Not Advised |
Theoretically, you can configure a server to respond to the Hypertext Transfer Protocol (HTTP) on any non-reserved port. For example, running HTTP on port 9999 is technically feasible. The following command demonstrates this with a node.js service setup:
const http = require('http');
http.createServer((req, res) => {
res.writeHead(200, {'Content-Type': 'text/plain'});
res.end('Hello World\n');
}).listen(9999, '127.0.0.1');
console.log('Server running at http://127.0.0.1:9999/');
However, deviating from standard port assignments often leads to confusion, inconvenience, and potentially compromised security. Standard ports are agreed upon by the Internet Assigned Numbers Authority (IANA), ensuring uniform interpretation across different platforms and networks. For instance, web servers generally listen for HTTP traffic on port 80. If configured to listen on a random high-numbered port instead, users would have to include this port number in the URL, which could confuse. Similarly, running a Secure Shell (SSH) server on an email port might lead to functionality issues, security loopholes, and violation of best practices.
In conclusion, while it’s technically plausible to run any protocol on any port given the right configuration, adherence to the standards set forth by IANA provides a smoother internet experience for all users involved.
Certainly! Protocols and ports are fundamental concepts in the realm of computer networks. They are vital components that facilitate communication between computers over a network. A protocol can be likened to a language, which two or more devices can understand to communicate effectively. There are numerous protocols such as HTTP, FTP, SMTP, to mention but a few, each designed for specific types of data communication.
For example, HTTP (Hyper Text Transfer Protocol) is primarily used to transfer data over the web. FTP (File Transfer Protocol) is used for transmitting files between the client-server model. SMTP (Simple Mail Transfer Protocol) is heavily deployed for email services. Conceptually, these protocols dictate how devices should package and interpret data to establish successful communication.
On the other hand, ports can be seen as logical gateways in a host machine for network traffic. Each port is identified by a unique number ranging from 0 to 65535. These numbers help differentiate multiple applications utilizing network services on a single host.
Typically, certain protocols are associated with dedicated ports by convention. For instance:
* Port 80 is often used by HTTP
* Port 21 is reserved for FTP
* Port 22 for SSH (Secure SHell)
However, asking “Can you run any protocol on any port?” delves into an interesting aspect. Strictly speaking, it’s possible to run any protocol on any port. It ultimately depends on your configuration. If you configure an application to use FTP on port 8080 (usually used for HTTP), as long as your client is also configured correctly, it would work.
This is achievable by modifying server configurations to listen on a specified port. For instance, in Node.js, you might write:
xxxxxxxxxx
const http = require('http');
const server = http.createServer((req, res) => {
res.statusCode = 200;
res.setHeader('Content-Type', 'text/plain');
res.end('Hello World\n');
});
server.listen(8080, '127.0.0.1', () => {
console.log('Server running at http://127.0.0.1:8080/');
});
In this code snippet, the server is set to listen on port 8080.
But there’s a caveat; some ports are well-known ports (from 0-1023), and are generally reserved for standard protocols. Using these could conflict with other applications. Further, some systems restrict binding to these ports for security reasons.
To avoid unintended behaviors, it’s recommended to adhere to conventions unless necessary. Assign the conventional ports to their corresponding protocols where possible. Understanding the premise behind protocols and ports sets the foundation for understanding more complex networking topics.
MDN Web Docs (API: Server) includes some learning resources if you want further information regarding server configurations.
A reference table might come in handy for quick referencing:
Protocol | Port |
---|---|
HTTP | 80 |
FTP | 21 |
SSH | 22 |
Understanding the correct usage of protocols and ports is pivotal in optimizing network communications and maintaining secure data transfers.When diving deep into networking protocol classification, it’s important to understand that practically speaking, yes, you can run any protocol on any port. However, from a standardized perspective and considering the designed functions of each protocol, the concept is nuanced by a combination of standard practices, security reasons, and technical constraints.
Network protocols are sets of rules that dictate how data communicates across different types of networks. Examples of common network protocols include TCP, UDP, HTTP, FTP, SMTP, and DNS. These protocols usually have default ports assigned to them ( IANA Service Name and Transport Protocol Port Number Registry). For example, HTTP interactions normally occur over port 80, while HTTPS uses port 443.
Protocol | Default Port |
---|---|
HTTP | 80 |
HTTPS | 443 |
FTP | 21 |
SMTP | 25 |
DNS | 53 |
However, technically, these are not restrictions, but standards meant to streamline network communication and ensure mutual understanding amongst varied systems.
Take a HTTP server for instance:
xxxxxxxxxx
from http.server import HTTPServer, BaseHTTPRequestHandler
class SimpleHTTPRequestHandler(BaseHTTPRequestHandler):
def do_GET(self):
self.send_response(200)
self.end_headers()
self.wfile.write(b'Hello, world!')
httpd = HTTPServer(('localhost', 8000), SimpleHTTPRequestHandler) # running on port 8000
httpd.serve_forever()
The above Python code creates a HTTP server responding to GET requests on port 8000 instead of the standard port 80. This flexibility can accommodate unique system requirements or application-specific settings.
There’s a caveat though: ports below 1024 are privileged ports (RFC6335). Without root access, an application cannot listen on these ports. Also, using non-standard ports might cause your services to be flagged as suspicious or blocked by firewalls due to security policies and thus, isn’t generally recommended unless necessary.
Additionally, running a protocol on a non-standard port won’t enable it to magically acquire features of another protocol. Each protocol has a specific purpose – HTTP was designed for hypertext transfer, SMTP for mail delivery, and so forth. Each one adheres to its own rules of interaction.
So, while it’s feasible to run any protocol on any port, default port numbers exist for better organization, reliability, security, and proper functionality of network protocols. Hence, deviating from the port conventions should be done judiciously.While technically possible, running any protocol on any port isn’t recommended. It’s important to understand that both protocols and ports play a critical role in networking, as they provide the rules and routing for communication across networks. However, to help maintain order and structure, certain standard protocols are conventionally associated with specific port numbers.
Protocols in Networking
In networking, a protocol is an arrangement that orders the way in which data is communicated. TCP (Transmission Control Protocol) and UDP (User Datagram Protocol) are two primary protocols used for data transmission over the internet.
xxxxxxxxxx
TCP/IP_Protocol_Example{
-- E.g. Data transmitted via TCP/IP
}
Ports in Networking
A port is essentially a virtual point where network connections start or end. Ports allow computers to differentiate between different software services or applications. For instance, port 80 is conventionally used for HTTP (Hypertext Transfer Protocol) traffic, while port 443 is utilized for HTTPS (HTTP Secure).
Relating Protocols to Ports
Each protocol usually runs on a specified port number to simplify and manage the vast amount of web traffic. For instance:
Protocol | Standard Port Number |
---|---|
HTTPS | 443 |
HTTP | 80 |
FTP | 21 |
However, the question here is, “Can you run any protocol on any port?” Technically, yes!
Can You Run Any Protocol On Any Port?
There’s no technical restriction preventing you from running any protocol on any port; this is just a matter of convention.
xxxxxxxxxx
//E.g., Running HTTP on a non-standard port
http.createServer((req, res)=>{
res.write("Running HTTP on a non-standard port!");
res.end();
}).listen(3000);
This snippet creates an HTTP server on port 3000 – not the typical port 80 for HTTP. However, diverting from these conventions can create confusion and unexpected behavior. For example, firewalls and routers might be configured to only allow or block traffic on certain ports aligned with their associated standard protocols.
It’s also crucial to avoid running services on well-known ports (0-1023) which have been officially assigned by the Internet Assigned Numbers Authority (IANA) to specific functions.
Therefore, it’s beneficial to stick to the conventional port numbers for common protocols unless there’s a compelling reason to do otherwise. Although it’s technically possible to run any protocol on any port, straying from convention may potentially cause more harm than good.Let’s first dissect the question and understand the backbone of internet communication: protocols. So, what are these Internet Protocols?
TCP: Transmission Control Protocol (TCP) is designed to be a reliable, byte stream-based transmission model [source]. Its main feature is the ability to provide error-checking and recovery methods from data that has been corrupted in transmission.
UDP: User Datagram Protocol (UDP) provides a datagram but unreliable service to the application program [source]. It means if an application needs to send a small chunk of data where reliability isn’t essential, UDP is the way to go.
xxxxxxxxxx
Sample coding using Python's socket library for TCP and UDP:
# For TCP
s = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
# For UDP
s = socket.socket(socket.AF_INET, socket.SOCK_DGRAM)
HTTP: HyperText Transfer Protocol (HTTP) is used for transmitting hypermedia documents, such as HTML, over the web [source].
HTTPS: HTTP Secure (HTTPS) is simply the secure version of HTTP where communication protocol is encrypted by Transport Layer Security or Secure Sockets Layer [source].
Protocol layering helps separate tasks into different elements which can then function independently while communicating with each other as needed. However, deciding whether you can run any protocol on any port depends on some considerations. Let’s explore them.
Technically Possible, but Practically?
In theory, yes, you can use any protocol on any port. Protocols are simply rules that govern how devices communicate on a network, and ports are just logical constructs that facilitate multiplexing; they enable multiple connections across a single network interface.
A simple Python UDP server listening on port 12345 would look like:
xxxxxxxxxx
import socket
sock = socket.socket(socket.AF_INET, socket.SOCK_DGRAM)
sock.bind(('localhost', 12345))
This means we could technically run HTTP over UDP, or run SSL over HTTP instead of TCP, binding a different port based on our fictional use-case. But here is where the practicality comes in.
A Matter of Standards and Conventions
Certain protocols are designed with certain transport mechanisms in mind. HTTPS, for instance, is generally expected to run over TCP, as its underlying protocols require a reliable transport medium. TCP’s error-checking and re-transmission features make it suitable for this. Similarly, video streaming applications prefer UDP because missing a few packets (frames) is acceptable and won’t affect the user experience drastically.
So, using an unconventional port might break these expectations. For instance, if I run my HTTP server on port 9876 instead of port 80 (standard), URL requests from a browser wouldn’t reach the server unless I specify the port explicitly (http://example.com:9876).
The Real World Interferes
Firewalls are often configured based on the assumption of these standard ports. If you start running services on non-standard ports, there’s a significant chance security infrastructure will block your traffic.
You also have to consider usability issues. Protocols like HTTP, FTP, or IMAP have standard port numbers because system administrators and users expect them to listen on these ports.
Protocol | Standard Port |
---|---|
HTTP | 80 |
HTTPS | 443 |
FTP | 21 |
IMAP | 143 |
In conclusion, while you can run any protocol on any port in principle, it may not be practically feasible or advisable due to established standards, conventions, and real-world constraints. Hence, thorough thinking should be given before deviating from regular procedures.When contemplating the coexistence of protocols and ports, one might ponder if you can run any protocol on any port. Theoretically, the answer is yes, but in practicality, it’s a bit more nuanced than that.
Let’s delve deeper into this topic by first breaking down the definition of Ports and Protocols. A Port refers to an endpoint of communication in an operating system. It serves as a distinctive location on a particular computer where network services are hosted. On the other hand, a Protocol is simply a set of rules governing how data is transferred over the internet or similar networks.
An important aspect of understanding these ports is recognising their classification into three categories:
– Well-known Ports: These range from 0 through 1023. They’re typically used by system processes that provide widely-used types of network services. For example, HTTP operates on port 80 and HTTPS on port 443.
– Registered Ports: These range from 1024 to 49151. While not strictly reserved like well-known ports, they’re ‘registered’ with IANA to avoid conflicts and duplication of use.
– Dynamic/Private Ports: Ranging from 49152 to 65535, these are available for use without centrally managed oversight.
xxxxxxxxxx
<table>
<tr>
<th>Port Range</th>
<th>Port Type</th>
</tr>
<tr>
<td>0-1023</td>
<td>Well-Known Ports</td>
</tr>
<tr>
<td>1024-49151</td>
<td>Registered Ports</td>
</tr>
<tr>
<td>49152-65535</td>
<td>Dynamic/Private Ports</td>
</tr>
</table>
While virtually any port could technically host any protocol, compliance with these guidelines reduces potential conflict or confusion when multiple services get implemented. Specific ports predominantly host correlating protocols – HTTP traffic on port 80, FTP traffic on port 21, etc.
Ignoring this convention can cause issues. Not only would it require constant manual configuration for hosts and clients, but standard firewall rules often block unconventional port-protocol combinations for security reasons. For instance, if you decided to host your website’s HTTP content on any port other than 80 or 443, users may find themselves unable to access it because their web browsers default to these ports.
So, although it would be possible to run any protocol on any port, it’s usually not a viable or efficient pathway to take. Following the conventions and using the registered port numbers for respective protocols ensures smooth functioning and universally understood systems. This way, network administrators, devices, and software can function predictably and efficiently together.The mapping of applications to specific protocol and port combinations is a standard practice in the world of computer networking. These mappings, known as socket addresses, are a combination of an IP address and a port number. An application typically uses a specific protocol (TCP or UDP) and port combination for communication across networks, based on well-established standards.
For instance, if an individual sets up a server for a website, that server would be set to listen for incoming connections on TCP port 80 or 443 for HTTPS. This is because these are the standard ports that were designated for HTTP and HTTPS traffic by the Internet Assigned Numbers Authority (IANA). Similarly, mail servers typically use TCP protocol on port 25 (SMTP), 110 (POP3), 143 (IMAP).
xxxxxxxxxx
141app.listen(80, function () {
2console.log("Server running on port 80");
3});
4
These configurations make it easier for client devices to communicate with servers using these standard protocols and ports. For example, web browsers automatically use HTTP over port 80 or HTTPS over port 443 when you send requests to retrieve a webpage—
xxxxxxxxxx
161http.get('http://api.example.com/page', (resp) => {
2//console log response data
3}).on('error', (err) => {
4console.log(err.message);
5});
6
However, just because these are the traditional port allocations does not mean that these are hard-and-fast rules. Theoretically, you can indeed run any protocol on any port depending on your requirements and how you configure your server and client programs.
So yes, you could technically choose to have a web server listen on port 8080 or even a non-standard port like 6000. Similarly, you could have an email server using an unconventional combination, such as SMTP over UDP instead of its standard TCP.
xxxxxxxxxx
141app.listen(6000, function(){
2console.log("Email Server running on port 6000");
3});
4
But deviating from these standards can introduce additional complexity. You’ll need to ensure everyone connecting to your server knows they need to use a custom port or change their software’s settings to support the unusual protocol-port pair. Such situations typically arise in testing environments or for security through obscurity purposes, intending to confuse potential attackers by concealing services on non-standard ports or protocols.
In practical terms though, carrying protocols over non-standard ports may violate some network policies, possibly causing IT infrastructure issues. Most network routers, firewalls, load balancers, and other network devices provide features based on standard protocol-port pairs. Deviating from these can lead to complications in managing network connectivity, performance, and security aspects.
To sum up, the common applications map to specific protocol and port combinations to maintain established conventions. This design simplifies global interoperability amongst different software, hardware, and network setups. Nevertheless, these combinations can be changed based on specific needs but often at the cost of added complexity.Absolutely! Understanding the concept of port mappings, particularly in relation to Internet-based applications, is key to becoming an efficient networking professional or developer. At its core, the idea revolves around using specific protocol’s data streams through designated ports.
Now, speaking of dominant Internet-based application-port mappings, it’s virtually impossible to overlook HTTP (Hyper Text Transfer Protocol) and HTTPS (Hyper Text Transfer Protocol Secure), highly involved with ports 80 and 443 respectively. Other major players include SMTP (Simple Mail Transfer Protocol) typically operating on port 25 and FTP (File Transfer Protocol) which bolsters file exchange via ports 20 and 21.
Protocol | Port Number |
---|---|
HTTP | 80 |
HTTPS | 443 |
SMTP | 25 |
FTP (Data) | 20 |
FTP (Control) | 21 |
These application-port correlations grew from de facto conventions into industry standards over time, ensuring effective interaction and communication between different networks across the globe.
Coming to your question – “Can you run any protocol on any port?”, theoretically speaking, yes. For instance, if a configuration demand arises, you could quite possibly set your HTTP server to operate on port 2022 instead of its default port 80.
Here’s a basic snippet that allows modification of an Apache server’s listening port:
xxxxxxxxxx
Listen 2022
However, it’s worth mentioning that such measures are typically restricted to testing environments or for bypassing specific network policies. Assigning the wrong port may result in traffic being blocked by firewalls unaware of the unconventional arrangement. Additionally, clients expecting services under standard ports might fail to connect correctly.
Overall, keeping application-port mappings intuitive and consistent aids troubleshooting, increases interoperability, and maintains a healthy line of order in managing immense virtual traffic. Safety norms and standards exist for valid reasons and deviating from them should only be done with caution and clear purpose.
For a detailed list of service to port mappings, the IANA Service Name and Transport Protocol Port Number Registry provides a complete overview. This recognizes the significance of universally accepted standards while showcasing the variety of protocols and their typical assigned ports.When it comes to customizing your own server setup, you do have the freedom to run any protocol on virtually any port. However, there are some considerations and restrictions that could affect the effective functioning and security of your protocols.
In an ideal world, ports and protocols would be specifically paired: HTTP runs on port 80, SSH on port 22, and so forth. This pairing allows firewalls to permit or deny traffic based on commonly used standards. That said, this ‘default’ configuration isn’t a hard-and-fast rule.
Flexibility in Protocol-Port Pairing
There’s nothing technically stopping you from running a different protocol on these default ports or running the expected protocol on a non-default port. This capability is due to how Transmission Control Protocol (TCP) and User Datagram Protocol (UDP), the backbones of most internet communication, work. They treat ports just as conversation endpoints without checking whether a specific protocol should be associated with that endpoint.
Here is a simple Python example illustrating a server setup accepting connections with TCP at a user-specified port:
xxxxxxxxxx
import socket
def start_my_server(port):
server = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
server.bind(('localhost', port))
server.listen(1)
while True:
client, address = server.accept()
data = client.recv(1024)
print('Received:', data)
start_my_server(5678)
In this code, we’ve created a basic TCP server listening on localhost at port 5678. It doesn’t care what kind of protocol messages it receives – it will simply print out whatever data it gets.
Considerations and Restrictions
While this flexibility can be empowering, it also has its downsides:
- Conflicts: If you’re running a different protocol on a standard port, it may conflict with services expecting the standard protocol on that port.
- Compatibility: Some clients expect specific protocols to run on specific ports and won’t function correctly if this isn’t the case.
- Security: Firewalls and other security measures often make assumptions based on port numbers. Running unexpected protocols on certain ports could allow malicious actors to bypass these protections.
In summary, while you can theoretically run any protocol on any port when setting up your server, it’s generally best practice to follow the conventional use unless you have a good reason to do otherwise. Whenever you configure your server contrary to accepted norms, ensure you conduct thorough testing to uncover any compatibility or security issues that may arise.
Customizing Server Setup
As you need to customize your server configuration according to specific requirements, each case can differ from another. Below table shows an example where different protocols are running on various ports:
Protocol | Port |
---|---|
HTTP | 8080 |
SSH | 2222 |
FTP | 2121 |
Please note, this table does not depict a typical configuration; rather, it demonstrates deliberate changes from default settings.
To further expand your understanding and knowledge on the subject, I recommend diving deeper into topics such as socket programming, TCP/IP, and network security. You can find comprehensive guides and tutorials on websites like W3Schools or online learning platforms like Udemy.Let’s jump right into the discussion about protocols and ports. Can you run any protocol over any port? Simply put, yes. In principle, a protocol can be configured to use any available port number. The `Transport Layer` in the `OSI model`source is where specifics for data transmission are defined. This includes options like Transmission Control Protocol (TCP) or User Datagram Protocol (UDP). When these protocols communicate over the internet, they send packets of digital information from one device to another through ports which typically correspond to specific services.
However, there are common configurations that we should note. For instance, some standard protocols have default ports that they connect to by convention. These include:
xxxxxxxxxx
HTTP using port 80
HTTPS using port 443
FTP using port 21
SMTP using port 25
Whether you want to change these defaults hinges on several fronts. Let’s take a closer look at the potential advantages and disadvantages.
Pros:
- Flexibility: You have control over your network system’s configuration.
- Security: Use of non-standard ports can make exploits tied to specific port numbers fail.source
- Traffic Management: Custom configurations can help optimize network traffic flow.
Cons:
- Usability: Can cause confusion when unexpected ports are used. This may lead to more difficulty in troubleshooting and configuration errors.
- Compatibility: Some software may rely on hardcoded port numbers, leading to cross-compatibility issues. For instance, most browsers automatically append port 80 for HTTP and 443 for HTTPS.
- False Perception of Security: Merely changing a protocol’s port does not fundamentally solve security vulnerabilities associated with the protocol itself. Known as ‘Security through obscurity’, this principle is widely disregarded in the cybersecurity community since obscurity should not substitute strong security measures.
As an example, let’s assume that you want to change the Port 80 traditionally used for HTTP traffic to another port, say 8080. In Node.js, a server script could look like this:
xxxxxxxxxx
const http = require('http');
let server = http.createServer((req, res) => {
res.writeHead(200, {'Content-Type': 'text/html'});
res.end('Hello World!');
});
server.listen(8080); // Server running at http://localhost:8080/
This script would start a server, listen on port 8080 and output “Hello World!” if everything works correctly.Sure, let’s delve into the topic regarding the security risks associated with running any protocol on unspecified ports since it is intrinsically connected to whether or not you can run a protocol on any port. It’s imperative to understand that while technically possible, there are certain inherent security risks involved.
First off, protocols and ports essentially play critical roles in the internet communication system. They enable computers to communicate over a network, with the ports specifically designated for each protocol. Running a protocol outside of its default port may result in unforeseen issues and vulnerabilities.
Risk of Misconfiguration
When you run a protocol on an unintended port, the risk of misconfigurations spikes considerably. For instance, suppose a protocol is designed to run on Port A but is running on Port B. The policies and protections established for Port A may not apply to Port B, leaving your setup susceptible to exploits.
Hiding Malware
Certain malware types strategize by using non-standard ports to bypass your firewall ruleset. By doing so, they avoid detection and establish connections from your machine to their command-and-control (C&C) servers. By permitting any protocol to run on unspecified ports, you welcome this risk into your system.
Increased Attack Surface
Each port in your network forms part of your ‘attack surface’. You’re effectively broadening the attack surface by allowing any protocol on any port. This factor escalates your vulnerabilities because you’re providing more opportunities for a rogue entity to target your network.
Standard Troubleshooting Difficulty
A convention of standardized ports is their recognizability in troubleshooting, making issues easier to detect and prevent. Contrarily, running protocols on arbitrary, unspecific ports obscures these communication lines, thus complicating the process for identifying and resolving problems.
An ideal practice with a holistic approach towards bolstering system security would include assigning default ports for specific protocols and limiting unauthorized access appropriately. Misusing or wrongly setting up ports could potentially invite an array of security vulnerabilities.
The best strategy is to follow the principle of least privilege (POLP). Rely on specialized tools like Nmap or Wireshark to monitor traffic patterns and watch for suspicious activity. And, always keep your systems patched and up-to-date.
Detecting unusual patterns should be high-priority and any protocol found running on an unusual port should be inspected. This precaution holds true not only for incoming internet traffic but also for internal traffic.
For further insight into handling such scenarios, see this SolarWinds article on Port & Protocol Security.
Here’s a short Python code snippet showcasing how you might use the socket library to open a TCP connection using a particular protocol on an arbitrary port:
xxxxxxxxxx
import socket
sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
server_address = ('localhost', 10000) # Using TCP on port 10000
sock.bind(server_address)
sock.listen(1)
Note: Always ensure this falls in line with your network’s security standards. Never carry out such activities without prior understanding and appropriate permissions.
In summary, although you can run any protocol on any port, doing so presents a Pandora’s box of potential security risks. Selecting proper protocols and corresponding ports, limiting access, monitoring traffic patterns and promptly patching discovered vulnerabilities will ensure that the risks are kept at a minimum.
While you can technically run any protocol on any port, it’s considerably important to understand the relationships between protocols, ports and firewalls.
For clarity, protocols, like HTTP or FTP, are sets of rules that computers use when communicating with each other over a network. Ports, on the other hand, are endpoints in the transmission process and they come with specific numbers.
Firewalls impact protocol-port operations significantly. They form an intrinsic part of network security with capability to control incoming and outgoing network traffic based on previously configured security rules. Firewall security settings can be adjusted to block data from certain locations while allowing the relevant and necessary data through.
The Relationship between Firewalls, Protocols, and Ports
Firewalls function by inspecting packets (data sent over the network) and their destination ports. There’s a general understanding that specific protocols will run on specific ports – for instance, HTTP is expected on port 80 and HTTPS on port 443. This convention helps ease the ‘communication process’ between computers on the internet. Now here’s where the firewall comes into play:
- Open vs Closed Ports: A firewall is configured to have both ‘open’ and ‘closed’ ports. For traffic to pass through, the relevant port needs to be open. Thus, even if a specific protocol could function theoretically on any port, the firewall settings would need to allow traffic through that specific port.
- Use of Non-Standard Ports: While services and applications often use standard ports, nothing technically prevents running a service on a non-standard port (with some exceptions for reserved lower port numbers). For example, you might decide to run a HTTP server on port 8000 instead of port 80. However, such cases might raise flags for many enterprise-level firewalls causing blocked access, due to security rules against non-standard ports.
- Evasion and Security: Some malicious practices attempt to bypass firewalls by using non-standard port-protocol combinations – which makes this a recognized security concern. To counteract this, modern firewalls are sometimes equipped with deeper packet inspection capabilities; enabling them to identify if the protocol fits the port it’s using, and act accordingly.
Running Any Protocol On Any Port: Not As Straightforward As It Seems
Although it is technically possible to run any protocol on any port, depending on your perspective it’s not necessarily a practical, efficient or secure idea.
You’ll have to configure both the client and server applications involved in the network communication to utilise the different port number. This flexibility isn’t always available – especially with proprietary software. Perhaps more crucially, if your chosen port is already being used (or reserved for specific utilities), you’ll encounter technical issues.
Additonally, using non-standard ports will likely complicate things for users on networks with serious security measures in place. Many corporate firewalls and proxy servers will only allow network traffic through recognised port-protocol combinations, so unconventional uses could get blocked.
Sample Code for Different Port Use with Python (Flask)
If you’re working with an application where you can easily change the port – for example, with Flask, a web framework for Python – the code is as follows:
First, import Flask:
xxxxxxxxxx
from flask import Flask
app = Flask(__name__)
Then, define a route:
xxxxxxxxxx
@app.route("/")
def hello():
return "Hello World!"
And finally, when running the app, specify the port:
xxxxxxxxxx
if __name__ == "__main__":
app.run(host='0.0.0.0', port=8000)
This Flask application will now run on port 8000.
In summary, to maintain efficient and secure network communications, it’s generally advisable to stick to standardized protocol-port combinations and not arbitrarily decide to run any protocol on any port. However, in some scenarios – testing, for instance – you might need to go beyond these conventions deliberately. Keep in mind, however, that firewalls might necessitate additional configuration adjustments to accommodate this.
A network socket consists of an IP address and a port, which creates particular endpoints where data can be transferred through. When you are addressing network configuration challenges in assigning new socket pairings, it may make you wonder if you could simply run any protocol on any port.
Running Protocol on Any Port
Technically, yes, you can run any protocol on any port. However, there are standard conventions that apply to ensure compatibility across different applications and systems. For example, HTTP commonly uses port 80, and HTTPS uses port 443. These are application-layer protocols which establish the rules for how a software application can send, receive and use data over a network. The specific ports they typically use allows routers, firewalls, and other network hardware to understand what kind of traffic is expected, and how to manage it efficiently.
Network Configuration Challenges
The challenge with running any protocol on any port manifests in network performance, security, and overall system compatibility. It’s like trying to organize a meeting with people speaking different languages without interpreters. If you designate a non-standard port for a well-known protocol, or vice versa, it can result in:
- Potential conflict with other applications that use the same port
- Unexpected behavior, as network hardware and software defaults will not recognize the distorted pattern
- Increased vulnerability from using obscure ports, which can attract attackers who are aware that these often bypass basic network security measures.
In network programming, for instance, consider this Python code snippet that opens up a TCP/IP socket on a specified IP and port:
xxxxxxxxxx
import socket
def create_socket(ip, port):
my_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
my_socket.bind((ip, port))
my_socket.listen(5)
return my_socket
This simple function takes in an IP address and a port as arguments. There’s no inherent check here to ensure that you’re binding the correct protocol to the right port. You could theoretically bind HTTP to port 22 (which is conventionally used for SSH) without running into immediate errors. But doing so could lead to unexpected issues when you start dealing with actual network traffic.
Considerations
Choosing to deviate from the established conventions for running protocols on certain ports should only be done with clear knowledge of the potential repercussions. As always, remember that just because something is possible in coding, does not mean it is optimal or advisable in practice.
Instead, focus on understanding your network architecture, assessing your security needs, and leveraging the benefits of adhering to standardized practices. Prioritize configuration management to mitigate risks and plan cost-effective operations.
If you have advanced requirements beyond the scope of predefined protocols and ports, consider seeking the help of networking professionals or refer to detailed guides such as RFC 6335, which governs the assignment of Internet port numbers.
Start with precautionary measures. In software development, it’s important to anticipate and design solutions for potential issues like divergence in network configurations. This way, we can enhance user experience while maintaining network integrity and optimizing resource allocation for our applications.
Yes, it is technically feasible to run any protocol on any port. However, this does not always align with best practices for network configuration and security. A protocol represents a set of digital rules for data exchange within or between computers. Traditionally, specific protocols are assigned to specific ports to maintain an orderly system of communication and for the benefit of standardized mutual recognition in networks worldwide.
Ports, represented by integer values between 0 to 65535, can be visualized as doorways through which information flows between applications on a computer network or the internet. Since every protocol has a standard port that it operates on defined by the Internet Assigned Numbers Authority (IANA), messing with these assignments can disrupt networks and become a major cybersecurity risk. Examples of such default assignments include:
– HTTP running on port 80
– HTTPS on port 443
– FTP on port 21
Protocol
Default Port
HTTP
80
HTTPS
443
FTP
21
However, there's no technical obstacle preventing you from running these or other protocols on non-standard ports if you deliberately configure your server to do so. This process could involve something as simple as changing a configuration file like nginx.conf for Nginx servers to reflect the desired port for a particular protocol.
server {
listen 8080;
location / {
proxy_pass http://localhost:8000;
}
}