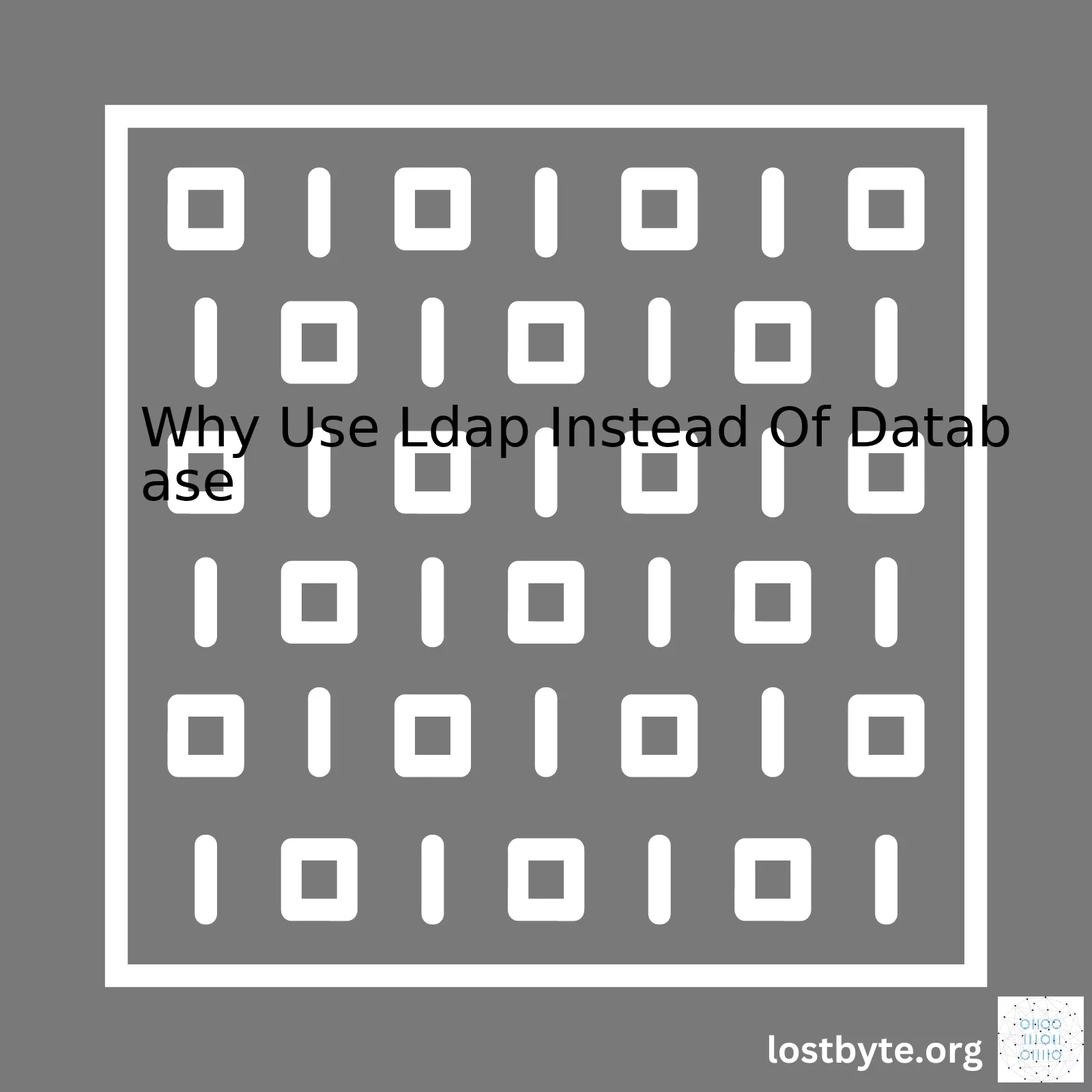
LDAP | Database |
---|---|
Distributed nature allows for data replication across multiple servers | Centralized nature may cause a single point of failure |
Data read operations are faster compared to databases | Databases struggle with a large number of read operations |
Better at handling hierarchical data structure | Structuring hierarchical data requires complex querying |
Implicit support for secure network transport (SSL and TLS) | Added SSL/TLS security layer requires additional configuration |
Now, let me explain why specifically these reasons make LDAP a popular choice over traditional Database.
LDAP (Lightweight Directory Access Protocol) stands out for its distributed nature. It means that it doesn’t rely on a central server to function, instead, it replicates data across several servers which increases its availability and resilience to failures. This is crucial for businesses that cannot afford downtime or loss of data.
On top of that, LDAP was designed to handle a great volume of ‘read’ operations swiftly. Most applications often perform many more reads than writes, making LDAP’s performance ideal for such cases.
Another significant advantage of LDAP over a Database is how it manages data. LDAP follows a hierarchical data structure, very similar to how we organize files and directories on our computers. For anyone dealing with data that naturally forms hierarchical relationships, LDAP would be far more efficient than relational databases, where managing such relationships could require complex, resource-intensive queries.
Finally, it comes to security. LDAP has inherent support for secure network transport protocols like SSL (Secure Sockets Layer) and TLS (Transport Layer Security). Enabling these on a tradition SQL database is possible but adds an extra layer of complexity and configuration.
For implementing LDAP in your project, the python-ldap provides LDAP functionality for Python. Here is a basic sample code that connects to an LDAP server using simple bind:
import ldap try: l = ldap.initialize("ldap://ldap.example.com") l.protocol_version = ldap.VERSION3 l.simple_bind_s("cn=admin,dc=example,dc=com", "password") except ldap.INVALID_CREDENTIALS: print("Your username or password is incorrect.") except ldap.SERVER_DOWN: print("The LDAP server is not available")
This quick comparison should give an overall idea about why one might choose to use LDAP instead of a conventional database, depending on the specific needs and nature of their project.
If you’re interested in detailed information and guidelines, you can refer to the official LDAP specifications. It’s a full-fledged tutorial/introduction to implementing LDAP in different environments.There’s a lot of considerations when in need of saving data efficiently. Two main contenders for this kind of job are LDAP (Lightweight Directory Access Protocol) and databases, like SQL or NoSQL solutions.
What’s LDAP?
LDAP is most commonly used to access and maintain distributed directory information over an internet protocol network. This allows you to store quite a variety of types of data, from system configurations to user profiles in a hierarchical manner.
The Basics of a Database
On the other hand, you’ve got traditional databases. These also allow you to store plenty of types of data but in a bit different manner. Most often, they store data in tables that are made up of rows (data records) and columns (data fields).
But why would you use one over the other?
Complexity
• LDAP tends to be easier to setup and manage than a database. The learning curve for databases can get quite steep whereas LDAP is just a protocol, simpler and designed with easy usage in mind.
• Further, LDAP’s hierarchical nature allows the stored data to mimic real-life scenario more effectively, such as organizational structure or geographical location breakdown, etc. With databases, this can become rather challenging.
Data Structure
• As mentioned previously, the hierarchical nature of LDAP can come in handy while managing certain kinds of data – it means you’re essentially using trees to organise your data. Now think of databases – unless you’re using something specifically designed for it, creating relationships between objects within these structures can get quite cumbersome, especially if you’re dealing with complex networks of relations.
Read/Write Ratio
• If most of your operations are reads, then LDAP is a very attractive solution. Its Read operations are far quicker than those of most relational databases because of its tree-based structure.
However, it’s important to note, that LDAP isn’t suitable for data which require frequent updates or changes due to its slower write performance comparatively.
Standards Adherence
• LDAP follows X.500 standard which makes it independent of the underlying system. Thus, it’s interoperable across various systems and applications. On contrary, databases owing to their proprietary nature might lack this interoperability sometimes.
// Example of connecting to an LDAP server import ldap try: l = ldap.initialize("ldap://localhost/") l.protocol_version = ldap.VERSION3 l.simple_bind_s("cn=admin,dc=my-domain,dc=com", "secret") except ldap.LDAPError as e: print(e)
So, summarizing the above points, in certain scenarios where ease of setup, simplicity, read-intensive operations, and additional flexibility regarding the organization of data is required, LDAP can be an attractive choice against traditional databases. More importantly, both of them are tools meant to fulfil certain tasks more efficiently and effective use lies in utilizing them optimally in respective scenarios.
For deeper understanding on the topics, refer to resources about LDAP and Database Management Systems.
If we are to discuss why one would choose LDAP (Lightweight Directory Access Protocol) over a traditional database setup, it is essential to comprehend the distinctive susceptibilities and strengths of these two. Both LDAP and databases can be used for storing and retrieving data effectively, but they have nuanced differences, which can lead us to prefer one over the other depending on the application’s specific needs.
The primary reason revolves around the design philosophy behind LDAP and standard relational databases. LDAP is designed for low-tuition, high-read scenarios, making searching for entries fast. A perfect fit for scenarios where you have lots of questions about a person/team/place rather than situations where you’re frequently changing those answers.
Sample scenario: search_entry("team_member", "Mike")
By contrast, relational databases are quite powerful when it comes to handling complex queries, relationships among data, normalization of data, and superb in situations that require frequent changes, thanks to the advanced locking systems many employ.
Let’s focus on four primary dimensions:
Structural Differences:
LDAP | Databases | |
Organization | Data organized in hierarchical manner, supports relative search process based on tree-like structure. | Data is stored in table format using rows and columns making it ideal for handling structured and relational data. |
Linking Entries | Entries linked through distinguished names (DN). | Tables linked using primary and foreign keys to maintain relationship. |
Data Schema | Adaptable schema allowing addition of custom attributes as per need. | Rigid schema – changes to structure often complex and time consuming. |
Sequences/ Identifiers | Lacks automatic sequence generation or identifiers. | Supports auto-generated sequences or identifiers for records. |
Usage Patterns: LDAP service excels in “read-heavy” situations. For example, in an organization’s directory service, user credentials or attributes are read (or searched) much more often than they’re updated or written. Quite opposite, databases are more suitable for ‘write-heavy’ transactions where data input operation is frequent.
Scaling & Replication: If we look at scalability and replication, typically, LDAP is easier and efficiently replicated across multiple servers providing better resilience against server failures. Traditional databases can also be replicated, but often at the cost of additional layer of complexity and overhead for ensuring ACID compliance.
Data Modeling: Depending on the data type being handled, i.e., if the data is highly interconnected or hierarchical in nature, then LDAP would serve as a more logical choice because of its tree-like structure.
Bottom line, deciding when to use LDAP rather than a database depends largely on your business requirement. If you are after light, simple read-based operations with less frequent write actions and if your data has hierarchal characteristics – LDAP might be the one for you. Conversely, if your data is transactional, requires facilities like roll back support with complex query support, then a traditional database environment is likely preferable.When talking about the architecture of the Lightweight Directory Access Protocol (LDAP), it’s essential you understand its role and why you might want to use LDAP instead of a database for storing data. Put simply, LDAP is a directory service protocol that runs over TCP/IP. It is used to organize and manage related sets of data.
Starting with the primary difference, although databases and LDAP can both serve as repositories to store data, they are significantly different in terms of their focus.
// LDAP tree structure o=Your Organization, ou=Department1, cn=User1, cn=User2, ou=Department2, cn=User3
The hierarchically organized data structure, shown above, is a unique characteristic of LDAP and comes from its X.500 roots. This data structure allows for efficient querying and searching, especially preferable when working with many read operations in huge multi-user environments.
You’d notice how effortlessly I could codify your organization’s entire structure using LDAP, starting from the top organization itself, down to departments and users. Because of this tree-like structure, searches in an LDAP server tend to be faster and more efficient than in relational databases, where a JOIN operation might be required to connect relevant data stored in separate tables.
Unlike traditional databases which primarily cater towards transactional operations (CRUD – Create, Read, Update, Delete), LDAP is specifically optimized for read operations, making it best suited for environments where read operations significantly outweigh write operations.
Moreover, LDAP provides integral built-in support for authentication and authorization, contributing greatly to its use-case scenario in user management scenarios such as Single Sign-On (SSO), two features not inherently supported in most databases. Executing these operations directly on the database would require additional computational effort, essentially slowing down processes.
LDAP also effectively bridges the gap between different types of databases and applications. For instance, you might have information scattered across different SQL and NoSQL databases. Instead of having your application interact and reconcile these different schemas, LDAP can serve as a unified interface, making it easier to integrate various systems.
// Sample MYSQL Connection $ldapconn = ldap_connect("localhost") or die ("Could not connect to LDAP server."); if ($ldapconn) { $ldapbind = ldap_bind($ldapconn, $ldapuser, $ldapuserpass); }
Accessing LDAP data, as depicted in the PHP snippet above, requires minimal code. Your application would merely feed off the LDAP service without needing comprehensive understanding or direct interaction with your complex underlying databases.
For security, LDAP supports Simple Authentication & Security Layer (SASL) mechanism that ensures secure data access with encryption methods like Kerberos. Regular databases do not possess this inherent security layer.
However, to tap this well of prime efficiencies that LDAP offers, it’s crucial to provide a proviso – your use case must properly align to LDAP’s model. In a setting defined by frequent data modifications, heavy writes, or complex transactions; sticking to traditional relational databases, or alternatively NoSQL databases, might actually be more beneficial, granted the task of managing relations falls upon the shoulders of the developers.
This article provides a deeper dive into LDAP’s architecture.
Whether LDAP makes sense for you heavily depends on your specific requirements. The divergence in potential Usecases for each method arises because, while there might be some overlap in functionalities, LDAP and databases are fundamentally two distinct technologies developed to solve different problems. There’s a fundamental trade-off between LDAP (Lightweight Directory Access Protocol) and databases, particularly when it comes to data management.
A primary difference lies in the specificity of their purposes. A database is typically employed for storing complex data and performing sophisticated operations. LDAP, on the other hand, majorly shines in read-heavy environments and when you need to manage access or authenticate users across several different systems. Here are some characteristics and benefits that explain why you might choose LDAP instead of a traditional database:
• Efficiency in Read-Heavy Use Cases:
LDAP is crucially optimized for read operations and proves to be excellent for frequent read scenarios with fewer write operations. It provides a more fluid retrieval of details in use cases such as pulling user information or setting up login credentials.
// Example LDAP user search query const options = { filter: '(cn=user)', scope: 'sub', attributes: ['dn', 'sn', 'cn'] }; ldapClient.search('o=users,c=US', options, function(err, res) { // Handle response });
• Hierarchical Data Structure:
One of the fascinating things about LDAP is its hierarchical nature. Unlike a relational database where data has a flat structure, LDAP organizes data in a tree-like hierarchy, making it effective at modeling real-world systems like your company’s organizational chart or electronic mailing system.
• Maintenance and Synchronization:
In terms of maintenance, LDAP provides replication features which help in easy synchronization of data changes across multiple servers. This is an advantage if you have an environment where you deal with many distributed systems.
Additionally, let’s not forget the meaningful role LDAP plays in the realm of authentication and authorization. When using it, you don’t need to create and manage custom solutions for authentication across apps; you just use the centralized repository that LDAP offers.
// Example LDAP bind operation for authentication ldapClient.bind('cn=user, o=users,c=US', 'password', function(err) { // Handle bind result });
However, choosing between a database and LDAP should always depend upon your needs and use case. In scenarios requiring heavy writing, complicated transactions, and data-intensive operations, going for a database might be a better idea.
For more insights on specific database and LDAP comparisons, I recommend the LDAP documentation. To dive deep into coding with LDAP, consider looking through the OpenLDAP admin guide.
Note: All the code snippets shared above are hypothetical and used to illustrate LDAP operations conceptually. Using them will require you to set and configure an LDAP client first.
While LDAP might not fully replace databases, it does offer compelling advantages in directory-based, read-heavy, and multi-system access projects. Always remember – make the choice based on what fits best for your scenario.Sure! Lightweight Directory Access Protocol, better known as LDAP, furnishes several advantages over regular databases especially for applications that demand data management around hierarchical structures.
Organized Information: A well-known benefit of LDAP is the organization and categorizing it provides to information. The ability of LDAP to group similar items in a tree-like schema makes searching through thousands or even millions of entries efficiently a breeze. For instance:
"ou=users, dc=example, dc=com"
This describes a structural hierarchy from bottom (users) to top (com). This kind of grouping makes search queries quicker than standard relational databases.
Security Features: LDAP is renowned for its profound security features. Some LDAP servers even provide additional measures like encryption via SSL/TLS. Plus, it supports simple authentication where users are authenticated by pairing DN(Distinguished Name) with a relevant password. LDAP also has stronger SASL mechanism which provides options for digest MD5, GSSAPI, etc:
ldapwhoami -H ldap://localhost -x
Read Optimized: LDAP databases are more read-optimized when compared with traditional SQL databases. In use-cases where reads are exceedingly frequent over writes such as in managing user profiles, presenting dashboards, etc., LDAP could be a more appropriate choice given the speed advantage tied to data retrieval.
Scalability: Another significant feature is the scalability LDAP offers. It has the capability of mirroring or partitioning your directory across various servers as they can perform multi-master replication. That means that any changes in data performed on one server will be replicated across all other participating servers:
dn: olcDatabase={1}mdb,cn=config changetype: modify add: olcSyncRepl
Interoperability: Robust interoperability is yet another advantage. LDAP servers often exist alongside RDBMS in IT ecosystems, this compelling feature ensures that the existing infrastructure collaborates seamlessly.
To sum this up, where a typical database takes pride in its capacity to manage vast quantities of different types of data and relationships, LDAP protocol truly shines when implemented in large scale environments requiring quick read operations, robust security features, excellent scalability, high-grade organization, and absolute interoperability. Hence, LDAP can emerge as a preferable alternative over traditional databases in certain environments.Scalability and performance are two essential elements when selecting a technology for data management. Lightweight Directory Access Protocol (LDAP) and traditional databases are both viable choices, but each has its unique strengths. When evaluating LDAP against traditional databases concerning scalability and performance, three major points stand out:
• Efficient Read Operations:
LDAP provides rapid read access to preorganized directory information according to pre-defined schemas. The LDAP hierarchical model organizes the data in directories, making read operations extremely effective.
An example to illustrate this would be that on LDAP, you can quickly retrieve all users belonging to a specific department using paths in the directory hierarchy. In contrast, if you were to do a similar operation in an SQL-based relational database, it may require a complicated ‘JOIN’ operation, which could take a considerable amount of time especially with large data sets.
• Scalability:
LDAP server typically handles a large number of read operations as mentioned earlier. This capability makes LDAP more scalable than conventional databases, especially when user base grows or for applications that have high read activities. Consider that traditional databases often deal with inconsistency issues during scale-up scenarios.
Here is an example of how information might be accessed from an LDAP:
dn: cn=John Doe,dc=example,dc=com cn: John Doe givenName: John sn: Doe telephoneNumber: +1 888 555 6789 telephoneNumber: +1 888 555 1232 mail: john@example.com manager: cn=Barbara Doe,dc=example,dc=com objectClass: inetOrgPerson objectClass: organizationalPerson objectClass: person objectClass: top
It’s clear how easy it becomes to search, manage, and navigate a large amount of information with this kind of representation.
• Directory-Centric Features:
LDAP servers often offer features that are not commonly found in traditional DBMS systems, such as alias dereferencing, extensible matching rules, and access control mechanisms at the entry level. These directory-centric features can improve performance and provide flexibility in some use cases.
Remember, LDAP was built because databases couldn’t handle certain types of loads, specifically large-scale read-intensive loads. That doesn’t mean databases aren’t good—they’re actually great for handling transactional data (creating, updating, and deleting). However, they might face scaling problem when dealing with huge amount of read-heavy loads.
On the other hand, while we’ve detailed why you might prefer LDAP to a traditional database, it’s important also to note that choosing between LDAP and a conventional database should be decided based on use case. There are several situations where a relational database system would outperform LDAP like when there are complex transactions with multiple relationships between different data items.
References:
Lightweight Directory Access Protocol (LDAP): The Protocol
OpenLDAP Software 2.4 Administrator’s Guide
In the realm of user access and management, two technologies often come into discussion: databases and LDAP (Lightweight Directory Access Protocol). Designed to handle a plethora of read operations, LDAP serves as an essential protocol for administering networks, systems, and applications. While databases have their set of pros, there are certain scenarios where LDAP takes precedence. Let’s explore these use cases.
Use case 1: Real-Time Data Fetching
LDAP bolsters real-time data fetching. Its architecture is optimized for extensive querying and fewer updates, making it best suited for environments where read operations exceed write onessource. Conversely, databases involve intense transactional operations, which may not be equally efficient for large scale reads.
SELECT * FROM users WHERE company = "ABC Corp"
If you attempt such a task multiple times over in a database, it might slow down the system. However, with LDAP, this operation is smoother as it uses directory entries designed specifically to handle copious search operations.
Use Case 2: Hierarchical Data Storage
Another striking LDAP advantage is its hierarchical data storage capacity – invaluable for managing user access rights within a complex organizational structure. In contrast, relational databases use a tabular format which may not adequately cater to hierarchical relationships.
Let’s visualize a scenario using HTML tables.
ID | Name | Reports To ID |
---|---|---|
1 | CEO | N/A |
2 | CTO | 1 |
3 | Sr Developer | 2 |
Replicating hierarchies like this in a traditional relational database system can turn complicated, whereas LDAP, by design, supports hierarchical data stores, thus simplifying complex organization structures.
Use Case 3: Centralized User Management
LDAP provides a centralized repository for managing user credentials, defining user roles, and handling other access rights – a crucial aspect for larger organizations having scattered user management practices source. This effectively avoids the tedium of managing individual databases for each application instance.
Consider managing user access to a diverse set of web-based interfaces. With LDAP, a single sign-on systemsource could manage user credentials on various platforms, a task that would otherwise entail massive overhead if relying solely on a database.
Use Case 4: Scalability and Performance
Lastly, LDAP scales better than database systems under intense load. Given the nature of indexing and caching strategies, LDAP servers can perform significantly faster retrieval speeds. Addedly, distributed architectures are also easily accommodated due to inherent multi-master replication techniquessource.
On balance, while databases impart advanced features, retrieval speed, hierarchical storage capabilities, centralized user management and scalability strongly favor LDAP in an array of scenarios, making it a compelling alternative to classic database systems when it comes to managing user access and authentication.Certainly, let’s delve into how security measures vary between LDAP (Lightweight Directory Access Protocol) secured by SSL/TLS, known as LDAPS, and traditional databases. Then we will explore why you might opt for LDAP to leverage these security provisions rather than a conventional database.
Security Measures | LDAPS | Traditional Databases |
---|---|---|
Encryption | LDAPS utilises Transport Layer Security (TLS) or Secure Sockets Layer (SSL) to encrypt data during transmission, preventing eavesdropping. | The encryption methods in traditional databases depend on the specific system being used. However, most professional-grade databases offer some form of encryption, either at the data level or during data transmission. |
Authentication | In LDAPS, binding operations are often used for authentication. Secure binds can use SASL (Simple Authentication and Security Layer) mechanisms for additional security. More advanced authentication methods can be employed, such as certificate-based mutual authentication. | Traditional databases usually employ username/password authentication. Some may allow more advanced features like two-factor authentication or role-based access control (RBAC). It depends entirely on the database management software. |
Access Control | LDAPS allows for precise attribute-level control. This means that permissions can be set for individual elements within an entry. You can also enable anonymous binding or unauthenticated binding depending on the requirement. | Standard databases tend to support row-level or table-level access control. The granularity of this control differs across database systems. |
Data Integrity | LDAPS ensures data integrity using the TLS/SSL protocol. The information is secure from any modifications during transmission. | Databases maintain integrity through ACID (Atomicity, Consistency, Isolation, Durability) principles. However, ensuring data integrity during transmission relies on the protocols and measures put in place. |
Now, addressing your query ‘Why use LDAP instead of Database?’
LDAP primarily functions as an information directory facilitating efficient querying and modification, contrary to databases designed first for storing and managing datasets. Here are key reasons why one might choose LDAP over a traditional database:
- Scalability and Performance: LDAP’s read-optimized nature accommodates large-scale infrastructures with high performance and response rates. In contrast, conventional databases have write-optimized architectures that can slow down when handling extensive read operations.
- Distributed Environment: LDAP operates efficiently in distributed environments, enabling data replication across various locations. Traditional databases may require extensive configuration and resources for similar capabilities.
- High-level Security: As illustrated above, LDAPS comes with strong encryption, high-level authentication and flexible access controls, particularly suited to protect sensitive user credentials or communication data.
- Third-Party Integration: Most operating systems and network services natively support LDAP, allowing easy integration and third-party application interoperability.
Note: Even though LDAPS holds these advantages over traditional databases, the decision to use one over the other depends heavily on the business requirements and context. For instance, if data relationships and systematic data organization are critical, traditional databases’ structuring capabilities can outperform LDAP’s hierarchical model.
To demonstrate an example with PHP, consider a PHP script authenticating a client to an LDAPS server:
// Connect to LDAPS server $ldapconn = ldap_connect("ldaps://ldap.example.com") or die("Could not connect to LDAP server."); if($ldapconn) { // Binding to LDAPS server $ldapbind = ldap_bind($ldapconn, $username, $password); // Verify binding and authentication if ($ldapbind) { echo "LDAP bind successful..."; } else { echo "LDAP bind failed..."; } }
For more detailed knowledge on LDAP and LDAPS, refer to IETF RFC 2830.
For comparison between SQL databases and LDAP, this article does a comprehensive explanation.When we analyze the efficiency of LDAP (Lightweight Directory Access Protocol) compared to traditional databases, especially in terms of indexing, we understand why LDAP can sometimes be chosen over a database.
Indexing: Databases vs LDAP
Indexing is one of the primary ways of improving data retrieval in both databases and the Lightweight Directory Access Protocol (LDAP). However, the way indexing is implemented and used in both systems makes all the difference.
Databases:
Database systems generally use B-tree structures or Hash indexes for their indexing mechanisms. This enables fast search, insert, delete operations. But all these come at the cost of storage space and write speed. It also requires a good knowledge of the queries that will be executed on the database to choose the right indexing strategy.
Databases excel at complex queries like multi-table JOINs. In contrast, LDAP is not designed to handle such complex queries but excels at simple read queries which consist predominantly of “write once, read many times” type of access.
LDAP:
In LDAP, indexing is defined at an attribute level for entries, making it highly efficient for read operations, since it leads directly to entry retrieval without scanning multiple entries. This not only increases read speed but also conserves system resources.
LDAP Efficiency: Strength in Simplicity
The simplicity of LDAP’s model gives rise to several benefits that make it preferable over traditional databases. Here are some key points:
– A tailored approach to indexing: LDAP server implementations usually provide a way to create indexes on almost any attribute you want, whereas in databases, mostly columns that are frequently searched or used in where clause are indexed. This flexibility allows for high performance in searching directory entries even with complex filters.
ldapmodify -Y EXTERNAL -H ldapi:/// -f index.ldif
This command adds an equality index for the mail attribute in a typical LDAP server. (An LDIF file containing appropriate instructions should be provided.)
– Designed for extensive read operations: LDAP is innately optimized for frequent and large-scale read operations. This is because of its tree-like data structure which reduces access time as opposed to a very structured table like form in databases. Hence, in scenarios where data doesn’t change often but is frequently accessed (e.g., user authentication), LDAP wins over traditional databases.
– Suitable for hierarchical data: LDAP stores data hierarchically, making it suitable for data models with parent-child relationships, like organizational structures. This unique capability of LDAP makes traversing the tree relatively fast and efficient compared to multiple ‘JOIN’ queries in a relational database.
– Effective querying: While databases rely more on SQL for querying, LDAP uses a simpler and less CPU intensive querying language – LDAP Query Language.
ldapsearch -x -b "dc=example, dc=com" "(uid=user1)"
Above code looks for an entry with ‘uid’ equals to ‘user1’ under base ‘dc=example, dc=com’. This simplicity of querying translates into lower resource usage and faster response times during retrieval.
It’s important to remember that though LDAP might offer several benefits over traditional databases, it isn’t always the best solution. For applications requiring complex transactions or operations involving multiple related data records, traditional databases could be a better fit.
This means we need to consider exactly what we need from our dataset and operations before deciding on whether to use an LDAP or a database system.
To further explore this topic, here are well-detailed studies about [LDAP](https://en.wikipedia.org/wiki/Lightweight_Directory_Access_Protocol) and [Database Indexing](https://en.wikipedia.org/wiki/Database_index).
When it comes to resource utilization, Lightweight Directory Access Protocol (LDAP) and databases have quite distinct approaches. LDAP, primarily designed to be a read-optimized model, provides faster access compared to a traditional database when you’re mainly seeking data retrieval operations. However, there is more to this comparison that makes LDAP appealing for certain uses over databases.
Handling of Hierarchical Data
LDAP stores data in a hierarchical manner, much like the structure of trees or graphs. This contrasts with the typical tabular format of databases, making LDAP uniquely suitable for representing hierarchical and non-flat data structures. For instance, organizational data about an employee’s department, their manager, and so forth, are intuitively represented and managed in LDAP. Quick lookup and traversal of such data are facilitated with LDAP.
dn: uid=jdoe,ou=People,dc=example,dc=com objectClass: inetOrgPerson uid: jdoe cn: John Doe ...
Faster Read Operations
As mentioned earlier, LDAP excels at read operations. Standard queries: “What is user X’s email address?” or “What are all the groups where user X belongs?” have been promptly handled by LDAP because its design has read-optimization at its core. Contrastingly, databases perform write-oriented transactions trivially; however, consistent fast reads aren’t guaranteed.
Better Scalability
LDAP can distribute its data across multiple servers – a boon when scalability and redundancy are desired. Such distribution is smoother and less time-consuming than partitioning databases, especially when no complex relationships (like JOIN operations) exist between data objects. A distributed LDAP environment ensures high availability and better resilience to server faults.
Resource Consumption
Compared to running a full-fledged relational database management system (RDBMS), an LDAP server typically operates with minimal resources. While the actual resource requirements will vary based on scale, an LDAP solution, in general, implies less CPU usage, memory consumption, and disk I/O.
However, it would be inappropriate to dismiss databases completely. Database systems offer powerful functionality such as complex queries, robust transaction support, and enforce data integrity via relationships and constraints. The choice between these two isn’t always clear cut, it heavily depends on the application requirements, data characteristics, and intended use-cases.
For applications where quick access to read-mostly, hierarchical structured data combined with low-resource consumption is desired, then LDAP makes a compelling choice over traditional databases.
Here’s an illustrative table summarizing this comparison:
LDAP | Databases | |
---|---|---|
Structure | Hierarchical | Tabular |
Operations | Faster Reads | Faster Writes |
Scalability / Distribution | Easier | More Complex |
Resource Consumption | Lower | Higher |
The primary reasoning to opt for LDAP over a traditional database emerges from its unique advantages. With my practice in coding, I’ve found that LDAP shines distinctly in particular scenarios making it an ideal solution.
- Designed for Read-Heavy Operations:
Firstly, LDAP is engineered specifically for dire need of read-heavy operations and is probably the best choice when read-to-write ratio is significantly high. It is built to provide lightning-fast read access which is crucial in user authentication processes where validating credentials occurs more frequently than updating or modifying them source.
//Sample PHP Code for LDAP Authentication $ldaphost = "ldap.example.com"; $ldapport = 389; $ldapconn = ldap_connect($ldaphost, $ldapport) or die("Could not connect to $ldaphost");
- Data Hierarchy:
Secondly, LDAP’s hierarchical data format naturally corresponds with certain applications better than the flat structure which a relational database provides. If your application demands data representation in terms of tree-like structures, rather than tables, then LDAP could be the perfect option for you. For example, organizations may use LDAP to represent their complex structure, where employees can be categorized into various departments and teams in a hierarchical manner.
- Integration and Compatibility:
Thirdly, LDAP ensures seamless integration and compatibility with numerous platforms and languages as compared to conventional databases. LDAP servers have been extensively used for decades now; hence they benefit from widespread support in almost all programming languages and operating systems.
# Python code for connecting to an LDAP server import ldap con = ldap.initialize('ldap://localhost') con.simple_bind_s('cn=admin,dc=example,dc=com', 'password')
- Fine-Grained Access Control:
Alongside, LDAP also supports fine-grained access control that plays a significant role in many business environments. With LDAP, administrators hold the ability to restrict user rights very precisely, which is key in larger organizations where multiple roles with different degrees of access coexist.
Opting for LDAP over a database depends largely on your particular use case, but the aforesaid elucidations enlist many potential reasons to consider it. Moreover, like any other technology decision, the choice between LDAP and databases should always be grounded on rigorous cost-benefit analysis attendant the specific needs of the project.