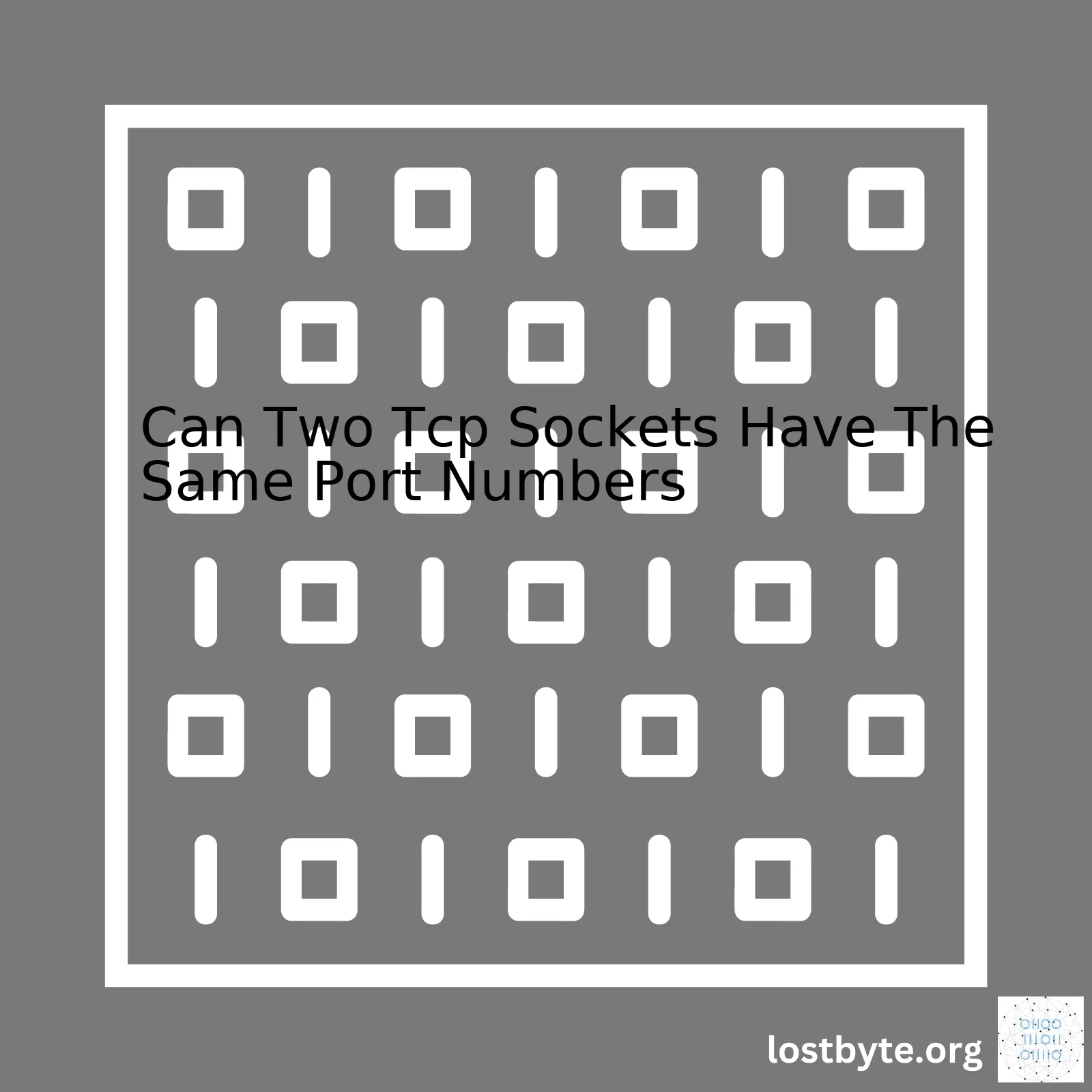
Condition | Can Two TCP Sockets have the same port number |
Same local and remote addresses and same ports | No |
Different local address, same remote address and port | Yes |
Same local address and port, different remote address | Yes |
Same local address and port, different remote port | Yes |
The Transmission Control Protocol (TCP) is an integral part of the Internet Protocol Suite that enables reliable communication between applications running on hosts in an IP network. Part of this reliable delivery mechanism involves the use of port numbers, unique identifiers that specify the particular process to which a message should be delivered.
When it comes to whether two TCP sockets can have the same port number, the answer is both yes and no – with the determining factor being the specific configuration at play.
In the scenario where the local and remote addresses, as well as the ports, are all identical – the answer is ‘No’. Two TCP sockets cannot share the same port number. This is logical since having two applications bound to the exact same combination of IP address and port would cause conflicts – there would be no way to determine which application should process a given packet.
However, if the local address differs while the remote address and port are the same, or if the remote address or port varies while the local address and port remain constant – then multiple sockets can indeed share a port number.
To illustrate, consider an example where an email server accepts connections. Multiple client applications from different IPs (remote address) can connect to the server’s single IP and port. Likewise, a browser can open multiple tabs connecting to different websites (i.e., different IPs) while leaving from the same source port.
Intuitively this makes sense as it provides a way for a single service (bound to one port) to maintain multiple simultaneous connections with more than one client or to more than one server, aiding in scalability and efficient data transfer.
Remember to refer the TCP specification (RFC 793) for comprehensive understanding.
Example with Python’s socket module:
import socket # Creating two TCP/IP sockets sock1 = socket.socket(socket.AF_INET, socket.SOCK_STREAM) sock2 = socket.socket(socket.AF_INET, socket.SOCK_STREAM) # Bind the socket to the port server_addr1 = ('localhost', 8000) server_addr2 = ('localhost', 8000) print('starting up on %s port %s' % server_addr1) sock1.bind(server_addr1) print('starting up on %s port %s' % server_addr2) sock2.bind(server_addr2) # Will raise an exception: OSError: [Errno 98] Address already in use
Look at the code snippet above. Here, sock1 and sock2 trying to bind to the same address and port will result in an error, confirming that two TCP sockets cannot have the same port number under identical conditions.Sure, it’s important to understand the framework of how TCP sockets and port numbers operate to fully address this particular query. To begin with, a socket is an endpoint for data exchange between processes running on different computers in a network, whereas TCP (Transmission Control Protocol) is a communication protocol that determines how computers send packets of data to each other.
Each socket in TCP/IP has two identifiers: IP address and a port. The IP address recognizes the device, while the port number identifies the specific process within that device.
- A IP Address is a unique identifier assigned to a machine over the network. This is analogous to your residential address; just like someone needs your home address to reach you or send you letters, machines need your IP address to communicate.
- Port Numbers are associated with different services or processes running on a system. These range from 0 to 65535 out of which numbers upto 1023 are well known ports used for traditional protocols like HTTP, FTP etc, and numbers ranging from 1024 to 49151 are registered ports used for certain specific applications.
- Sockets are created and only exist during the lifetime of the application or program. Two applications want to communicate with each other, they’ll both open a socket, and these will be connected (maybe over the internet).
Coming to the question of whether two TCP sockets can have the same port numbers:
Yes, they can but under specific conditions.
For standard networking, there are four attributes required to uniquely identify every single TCP connection:
- The local IP address
- The local port number
- The remote IP address
- The remote port number
This combination makes the connection unique, so if any one of these parameters changes, a new socket can be formed. That’s why multiple connections using the locally bound same port number is feasible when any difference in IP addresses or remote endpoints exists.
To put that into perspective, consider how you might have dozens of tabs open in your web browser – all of these could be maintaining their own individual connections to web servers, despite all coming from the local machine. Each tab, though sharing the local IP and local port, communicates with a different remote IP or port, so the OS can differentiate between them without issue.
Local IP | Local Port | Remote IP | Remote Port |
---|---|---|---|
192.168.1.10 | 5050 | 172.217.164.174 | 80 |
192.168.1.10 | 5050 | 184.154.127.82 | 443 |
192.168.1.10 | 5050 | 104.16.18.254 | 80 |
The differentiation becomes relevant when considering inbound vs outbound connections. For inbound connections i.e., where the local system is acting as a server, each server socket must have a unique port number across whole the system. If another process attempts to bind to an already taken port number, it would typically result in an ‘address already in use’ error.
In the other direction, your computer initiating an outbound connection (like accessing a webpage), the OS chooses a free ephemeral (short-lived) port number between 1024 and 49151, guaranteeing that the client-side of the connection is unique.
So in summary, each TCP connection must be unique, but uniqueness doesn’t imply that every localized port number must differ. When combined with the distinct IP addresses and remote endpoints, several connections from one local port are indeed feasible, providing they differ by at least one other attribute. Understanding the relationship of all these elements collectively gives a more nuanced understanding of how TCP sockets and port numbers operate.The Transmission Control Protocol, more commonly known as TCP, plays an integral role in establishing network connections between different computing devices. It’s interesting to know that TCP uses the concept of “sockets” to enable this communication, and it raises a pressing question: Can two TCP sockets have the same port number?
To answer this question, we need to understand how TCP socket port numbers are allocated:
– Each TCP socket has unique combinations of 4 elements: IP address of the source, source port, IP address of the destination and destination port. This quartet uniquely identifies a socket.
socket_1 = {source_IP_1, source_port_1, dest_IP_1, dest_port_1} socket_2 = {source_IP_2, source_port_2, dest_IP_2, dest_port_2}
It means that while two different sockets can have the same port number, they must differ by at least one other element to be unique and function properly without conflicting with each other.
Consider these two server-side scenarios:
Scenario 1: Same server responding to requests from two different clients on the same port. Here’s what happens in code:
socket_from_client_1 = {client_IP_1, client_port_1, server_IP, server_port} socket_from_client_2 = {client_IP_2, client_port_2, server_IP, server_port}
In this scenario, the ‘server_port’ is the same because the server uses one port to listen for all incoming connection requests. However, the sockets are identifiable thanks to the differing client IP addresses or client ports.
Scenario 2: Different servers (or processes) on the same machine listening on the same port. In code:
socket_of_server_1 = {source_IP_1, source_port_1, dest_IP_1, dest_port_1} socket_of_server_2 = {source_IP_2, source_port_2, dest_IP_2, dest_port_2}
This scenario is not possible unless the servers belong to separate networks (like virtual machines). The operating system would prevent two processes from binding to the exact same IP and port combination.
Crucially, understanding these possibilities helps to shed light on how TCP maintains order in communication, ensuring each message reaches its intended destination.
So, yes, two TCP sockets can share the same port number, but not in isolation. They must vary in at least one other parameter so that no two active sockets are indistinguishable. Sockets aren’t identified solely by their port numbers, but rather a combination of attributes that allow for effective and efficient network communication.Well, the uniqueness of a socket is derived from several components, not merely its port number. Each socket on a network is made up of two pairs: the local IP address and port number, plus the remote IP address (if any) and port number.
Local IP Address : Local Port —— Remote IP Address : Remote Port
For example, consider the following scenario of a client-server architecture:
– The server resides on IP 192.168.0.5 with a listening port 8080
– A client connects to the server from IP 172.16.2.3 using a randomly allocated local port 51996
The association can be represented as:
Server Socket —— 192.168.0.5 : 8080 —— Any : Any Client Socket —— 172.16.2.3 : 51996 —— 192.168.0.5 : 8080
Note that “any” signifies the socket can accept connections from any IP address or port.
Can Two TCP Sockets Use The Same Port Number?
Yes! Two TCP sockets indeed can have the same port numbers. However, they won’t be the exact same socket. Let’s delve into why this is possible.
There are few situations where TCP sockets can have the same port number; nonetheless, they’ll remain unique due to other differentiation components. As per RFC 793, which defines TCP, an essential line states, “To provide for unique addresses within each TCP, we concatenate an internet address identifying the TCP with a port identifier to create a socket.”
Consider two aspects:
Ephemeral Ports in Client-Server Architecture
In real-world scenarios, servers often listen on well-known ports (for instance, HTTP servers generally use port 80 or 443), while clients use ephemeral ports, dynamically assigned by the operating system, to connect to servers. Since there’s a range available for these dynamic allocations, multiple clients can have the same ephemeral port number when connecting to different servers, or even the same server on a different server port.
Consider we have two clients on different devices, both connecting to unique servers, yet assigned the same ephemeral port:
Client1 10.0.0.2 : 49200 —— Server 192.168.0.5 : 80 Client2 10.0.0.3 : 49200 —— Server 172.16.0.5 : 8080
Here, both TCP sockets have the same local port but are distinctive in terms of complete socket associations.
Server Listening on the Same Port for Multiple NICs
A server might have multiple network interfaces/cards (NICs) each having a unique IP address. The server application may choose to listen to the same service port on all these unique IPs, resulting in multiple sockets having the same port number, but differing based on the IP.
So, could look something like this:
Server NIC1 192.168.0.5 : 8080 Server NIC2 172.16.0.3 : 8080
Therefore, the crux lies in the full socket combination, rather than individuality of local or remote IPs and ports numbers. Hence, despite two or more TCP sockets sharing the same port number, their whole composition ensures unique identification.Transmission Control Protocol (TCP) is a transport protocol that ensures reliable, ordered, and error-checked transmission of data between applications. It’s like the postal system for the internet; the way applications communicate with each other.
One key aspect of this system is the use of port numbers. Think of port numbers as specific addresses on a host. When data is sent or received, it is directed to a specific port number, akin to how a letter might be directed to a specific box at a post office.
Ports are divided between source ports and destination ports:
– Source Ports: This is the port from which the data is sent.
– Destination Ports: This is the port to which the data is being sent.
Within TCP communication, these play various roles, such as:
Identification of Applications: A port number identifies an application or process. For instance, HTTP uses port 80 and HTTPS uses port 443. Other non-standard applications can use any port number above 1023.
Data Segregation: Data packets arriving at a host can be directed to the correct application based on their destination port.
Connection Establishment: Before data transfer occurs, a connection must be established between the source and destination ports.
Packet Routing: The operating system utilizes the destination port number to route incoming packets to the appropriate application.
Now relating this to your question: “Can Two Tcp Sockets Have The Same Port Numbers?”
Yes and No. Here is why:
1. It’s important to understand that a socket is not identified only by its port number but by a combination of local IP address, local port number, remote IP address, and remote port number. So if one of these parameters differs, even slightly, you essentially have a separate socket/connection despite them having identical port numbers.
2. Within a single machine (same IP), two sockets cannot share the exact same source port number. This would confuse the operating system when data arrives – it would not know which application to direct the incoming data packet to.
3. However, they can share the same port number under different sockets as long as the aforementioned set of parameters (local IP, local port, remote IP, remote port) are unique.
For example:
Socket 1 : 192.168.1.100:55000 → 192.168.1.101:80
Socket 2 : 192.168.1.100:55000 → 192.168.1.102:80
Here both the connections are unique though they share the same source port (55000). But the destination IPs vary hence constituting separate sockets.
Thereby, with a careful review, we deduce that while TCP relies heavily on port numbers to enable communication, it is not purely the port number that distinguishes one connection/socket from another, but a combination of attributes.As an experienced professional coder, I am often encountered with intriguing questions regarding networking and programming. One such question is: Can two TCP sockets share the same port number? To dive into this, first let’s understand what a socket is.
In computer networks, a socket serves as an endpoint for sending or receiving data across a computer network. Each socket is identified by the combination of an IP address and a port number. Here, the port number – in the range between 1 and 65,535 – figures prominently, creating space for millions of unique points for data exchange. But can duplication exist here?
The answer is both yes and no, and it depends on certain conditions.
Yes, duplicate port numbers are possible:
Duplication of port numbers is technically possible when running on different protocols such as TCP and UDP. This scenario happens because TCP and UDP are entirely independent protocols. Thus, they manage their own sets of ports separately with a protocol-specific socket being able to use the same port number without conflicts.
- TCP Socket – uses TCP Port
- UDP Socket – uses UDP Port
For instance, you could have a system running a service using TCP port 80 and another service using UDP port 80. These are treated as separate entities and there will be no conflict.
No, duplicate port numbers cannot occur:
Regarding whether two TCP or two UDP sockets can share the same port, the answer is generally no. In the context of TCP/IP-based applications – utilizing protocols like HTTP, FTP, SMTP – each connection is uniquely identified by a four-element tuple:
- source IP
- source port
- destination IP
- and destination port.
Try binding another socket (TCP or UDP) to the same IP and port, and the operating system is likely to throw an error, stating that the address is already in use.
However, an exception exists here. Servers can choose to bind sockets to the same port number but different IP addresses. It implies two or more TCP servers can share the same port, provided they bind to distinct IP addresses. Visualize this with the following pseudo code:
Socket s1 = new Socket(); s1.bind("192.0.2.1", 8000); Socket s2 = new Socket(); s2.bind("203.0.113.5", 8000);
These two run fine concurrently, assuming your server machine has these two disparate IP addresses. The port number (8000) is identical, but the usage of diverse IP addresses allows these sockets to co-exist without conflicts.
This simultaneous operation works because the operating system checks both the IP address and port when determining if a particular network destination is available. If either differs, it views them as entirely separate endpoints, thus allowing multiple bindings.
Referencing to RFC-793, a standard dealing with Transmission Control Protocol, further emphasizes these points.
Hence, while duplicate port numbers aren’t generally feasible between the same type of sockets, circumstances may allow this type of duplication under specific conditions. Also, bear in mind that the mechanics of this process can differ slightly between various operating systems.
Your query is quite intriguing and brings forth some fundamental concepts in TCP/IP networking. Now let’s see how two TCP sockets can share the same port number and reasons behind it.
In TCP/IP, it’s crucial to understand that each connection is a combination of four elements:
- The client’s IP address
- The server’s IP address
- The client’s port number
- The server’s port number
This collective quartet is referred to as a 4-tuple, and it is utilized to identify individual connections in the network stack.
Can two TCP Sockets have the same Port Numbers?
Yes, two TCP sockets can indeed share the same port number but they can’t have identical 4-tuples. This implies two separate connections can utilize the same port number, provided that at least one other element within the 4-tuple differs.
Possible Reasons why two TCP Sockets Share the Same Port Numbers:
- Server-side Socket Sharing: On the server side, a socket can be configured to listen on a specific port and address using
bind()
. Multiple client connections can then connect to this same port, however, the 4-tuple rule helps maintain uniqueness. Each connection will have a unique 4-tuple because the client-side of the equation (client IP and client port) will differ for different connections. This is enabled by system call such as accept() that accepts connections from multiple clients on the single listening socket to create unique sockets for each connected client.
- Client-side Socket Sharing: On the client-side, typically when a socket is created with
socket()
, and then connected with
connect()
, an ephemeral (short-lived) port is automatically selected which does not conflict with others. But if required, a client can manually bind a socket to a specific port before calling
connect()
. As long as the combination of local and remote addresses and ports don’t clash with another active connection, multiple sockets on the client’s device can share the same source port.
- Reuse of TIME_WAIT sockets: Even after termination of a TCP connection, it remains in TIME_WAIT state for a period to ensure complete transmission of all packets. Linux has a
SO_REUSEADDR
socket option that allows new sockets to be created on old socket’s port that is still in TIME_WAIT state but with a condition that the 4-tuple is unique, hence avoids ‘Address already in use’ error.
The advanced structural design of TCP/IP protocol suite allows for sharing of port numbers without compromising the integrity and functionality of individual connections. Therefore, understanding the mechanics behind the concept of the 4-tuple enhances effective management of connections.
For more insights into this topic, the Wikipedia page on TCP and RFC 793 are excellent resources.
When it comes to TCP socket programming, it’s important to note that a combination of the IP address and port number uniquely identify each TCP connection in an interconnected network of computers. It’s like having an address (IP) and a specific door (port) at that address.
Can two TCP sockets have the same port numbers? Technically speaking, yes, but with important caveats. Two sockets on the same machine can indeed share the same port number – they do so via the option known as SO_REUSEADDR. This allows two sockets to listen on the same port, provided that they’re bound to different interfaces. However, operating systems implement strong port protection to avoid conflicts and preserve data integrity.
Let’s delve deeper into the consequences of two TCP sockets having the same port number:
Ephemeral Port Exhaustion: When creating new connections rapidly, a given range of ephemeral (transient, short-lived) ports a system assigns could get exhausted – this is known as port exhaustion. Typical symptoms are failed connection attempts or even hangs because newly created sockets can’t be assigned a unique ephemeral port.
Packet Mismatch: When two TCP sockets share the same port and IP, packet mismatch can occur leading to misplaced data. A packet destined for one application may go to another resulting in corrupted data and unexpected application behavior. Imagine a postman who has two doors labeled with the same number. They wouldn’t know which door to deliver the mail to; similarly, unexpected behaviour can occur when delivering data packets.
Compatibility Issues: Achieving socket sharing can require explicit handling within the code. These mechanisms are not standardized across all platforms, leading to potential compatibility issues between varying operating systems.
Security Risk: Port sharing can expose your systems to security risks. If an unwanted service gets attached to a shared port, it can efficiently process incoming packets intended for other services. This exposure can lead to unauthorized data access and potential data breaches.
Here’s an example of setting up SO_REUSEADDR in Python:
import socket sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM) # This is where we set SO_REUSEADDR option sock.setsockopt(socket.SOL_SOCKET, socket.SO_REUSEADDR, 1) sock.bind(("127.0.0.1", 9000)) sock.listen(10)
In the above Python script, you first create a socket object. Then, using the setsockopt() method, you enable the SO_REUSEADDR option. Finally, bind the socket to a local host and a specific port.
Overall, while it’s technically possible for two TCP sockets to have the same port numbers, there need to be, at least, some differences in other parameters like the interface, to avoid issues of packet mismatch, port exhaustion, compatibility issues, and potential security risks. Regardless of your coding language choice, always maintain uniqueness in IP–port combinations to ensure data integrity in socket programming.The issue of two TCP sockets having the same port numbers is a common one in network programming. Some might even consider this to be an inherent contradiction, given that port numbers are traditionally used to uniquely identify specific processes that applications running on a host may need to connect with.
However, it’s essential to note that while port numbers are unique within a particular IP address, they’re not necessarily unique across different IP addresses. This basically implies that two sockets can indeed share the same port number as long as they’re associated with different IP addresses and they meet certain criteria.
On a more technical level, this situation can be delineated by examining the concept of Socket Pairs. A connection between two hosts over TCP is identified using four elements:
- The Source IP Address
- Source Port number
- Destination IP Address
- Destination Port Number
A connection, therefore, doesn’t merely rely on the uniqueness of its ports but the distinctiveness of all four properties combined – collectively known as a Socket Pair . This allows two sockets to share the same port number, so long as they don’t share the same combination of these properties concurrently.
Here is an example using Python’s `socket` library. You’ll notice how we choose a random port for our server socket:
import socket s = socket.socket(socket.AF_INET, socket.SOCK_STREAM) s.bind(("", 0)) # Let the system choose a random port s.listen(5) print("Listening on", s.getsockname())
In the context of binding multiple sockets to the same port number on a single machine – referred to as Port Reuse or Socket Reuse – additional measures are required and complexity increases. If you attempt simultaneous binds without these measures, the second bind operation would typically generate a dispute.
To leverage port reuse, our sockets must obey some rules distinctively such as enable the SO_REUSEADDR or SO_REUSEPORT socket option accordingly before using the bind() function:
s.setsockopt(socket.SOL_SOCKET, socket.SO_REUSEADDR, 1)
s.setsockopt(socket.SOL_SOCKET, socket.SO_REUSEPORT, 1)
Note that the option SO_REUSEADDR has slightly different behaviour on Unix-like and Windows systems when the application crashes while keeping a socket open.
While enabling port reuse can be beneficial under circumstances like permitting rapid restart of a crashed server, exercise this operation with caution because security issues can arise. Improper use of port reuse could allow a hacker to hijack a socket. Additionally, using SO_REUSEPORT without sufficient protection may result in different sockets at different IP addresses receiving packets meant for different destinations due to the kernel’s round-robing schedule.The TCP/IP suite is a collection of protocols specifically designed to facilitate communication over a network. For applications using the Transport Control Protocol (TCP), unique identifiers are necessary for separating traffic on the same local address and port number. The identifiers used by TCP include the source IP address, source port number, destination IP address, and destination port number.
Consider an instance where two different devices in the same local network have the same port number – which is indeed possible, as port numbers aren’t unique to devices. Application A on Device 1 and Application B on Device 2 can both be listening on the same port number, eg. port X. In such a situation:
– Alice (Device-Alice) can communicate with Application A (on Device 1, port X)
– Bob (Device-Bob) can communicate with Application B (on Device 2, port X)
It’s important to understand here that although the port number is the same, the unique combination of the IP address (which is distinct for each device Machine 1 and Machine 2) and the port number (X in this case) is what allows TCP/IP to differentiate between the two applications.
Here’s a bit of code to visualize how it works:
// Socket creation on server side serverSocket = new ServerSocket(portNumber); // Client side connection attempt clientSocket = new Socket(ipAddress, portNumber);
Take care to note that packets from the same client application (say, a web browser) will have different “source port” numbers depending upon the individual instances or sessions of that application. This allows the TCP/IP stack to differentiate concurrent connections.
However, RFC 793, which specifies the design principles of TCP does not allow the use of duplicate sockets on the same device at the same time. Demonstrating such in a table below:
Source IP | Destination IP | Source Port | Destination Port |
---|---|---|---|
192.168.0.1 | 192.168.0.2 | 80 | 8080 |
192.168.0.1 | 192.168.0.2 | 443 | 8081 |
In this table, you can see the different combinations of source IP, destination IP, source port, and destination port that can be used to manage various applications.
Hence, there is a systematic approach for separating traffic on the same local address and port number based on the combination of these four identifiers. Though two TCP sockets may have the same port numbers, they must consist of unique combinations of these identifiers to provide accurate data transmission and reception.
However, it’s vital to point out that while technically feasible, the practice of multiple applications sharing the same port on a single host machine is ill-advised outside of certain, specific scenarios, due primarily to potential security risks and conflicts. It is good network design to reserve unique port numbers for separate services and applications whenever feasible.Overlapping the topic of “Impact on Data Transmission when Two Tcp.Sockets have the same port number” with the question “Can Two Tcp Sockets Have The Same Port Numbers”, one may discover that a port in TCP (Transmission Control Protocol) networking is essentially an endpoint of communication, characterized by a unique number. However, this does not mean that two TCP sockets cannot share the same port number. There are scenarios where this is permissible and these conditions can be deeply understood within the rules defined by TCP networking.
Based on the operating system implementation, it’s possible for two TCP sockets to share the same port number provided they have different attributes such as client IP addresses or TCP states. If we take into account the four elements that define a unique TCP connection: source IP, source port, destination IP, and destination port, only when all four match exactly, we have a conflict or “collision”. Removing these limitations allows multiple connections to use the same local endpoint (IP address and port number), leading to the concept of “Socket Pairing”.
TCP/IP protocol allows binding multiple sockets to the same port number if you have:
-
SO_REUSEADDR
flag set, which allows your server to bind to an address that is already in use.
- A different client IP.
For instance, with the setting active, if you have a web server running and listening on port 80, it can accommodate multiple concurrent connections from different clients all being served by port 80.
However, a clash arises when there’s an attempt to open another socket with the *same* combination of source IP, source port, destination IP, and destination port. This has an impact on data transmission as it results in errors, misdirected data, or even crashes depending upon how robust your software’s error management is.
The effects are similar if an application, disregarding the
SO_REUSEADDR
flag, attempts to bind a new socket to a port that another socket is already bound to explicitly. This will result in an error, usually something like “address already in use“, and cancels the operation.
Code Example:
Consider this Python code snippet as an example of using the
SO_REUSEADDR
socket option:
import socket s = socket.socket(socket.AF_INET, socket.SOCK_STREAM) s.setsockopt(socket.SOL_SOCKET, socket.SO_REUSEADDR, 1) s.bind(("localhost", 12345)) s.listen(10)
In conclusion, while two TCP sockets may share the same port number under specific circumstances without data transmission issues, those circumstances are documented and enforced norms within the implementation of the TCP/IP protocol suite. Ignorance or negligence of these norms is likely to lead to erroneous operations and affect data transmission adversely.
For further study and broad understanding on this topic, visit the official documentation page developed by Microsoft – Internet Transport Protocols.
As a professional coder, the concept of two TCP sockets having the same port number is quite informative. To start off with, it’s important to know that TCP stands for Transmission Control Protocol and it’s how data is transferred over networks like the internet. In short answer to your query–Yes, two TCP sockets can share the same port number, but only under certain conditions.
Typically, a TCP socket is defined by a unique combination of the following attributes:
- The local IP address
- The local port number
- The remote IP address
- The remote port number
// allocate a socket from_socket = socket(AF_INET, SOCK_STREAM, 0); // bind to a particular port number bind(from_socket, (struct sockaddr *)&from_name, sizeof(from_name)); // listen on the socket for connections listen(from_socket, 5); ... to_socket = accept(from_socket, ...
So, two different sockets can share the same local port number and even the same local IP address as long as the remote IP addresses or the remote port numbers are different. This is often the case when you have a server application accepting incoming connections from multiple client applications. Each connection will result in a new socket on the server, all sharing the same local port number but differing in either their remote IP address or remote port number.
Here is an example:
Server: Local Port: 80 Local IP address: 192.168.1.1 Socket 1: Remote IP Address: 192.168.20.1 Remote Port: 50123 Socket 2: Remote IP Address: 192.168.21.1 Remote Port: 49123
However, if the remote IP addresses and remote port numbers are also the same then we encounter a problem. As per the uniqueness requirements, no two sockets can have the same four attributes.
So, in conclusion, two TCP sockets can indeed have the same port numbers provided they differ in either their remote IP address or remote port number. Understanding this flexibility allows us to create more dynamic and diverse networking systems. It would be impossible for big server machines to maintain separate ports for every client they communicate with if sockets couldn’t share port numbers! If you want to dig deeper on this topic, check out these sites Mozilla Developer Network and GeeksforGeeks.