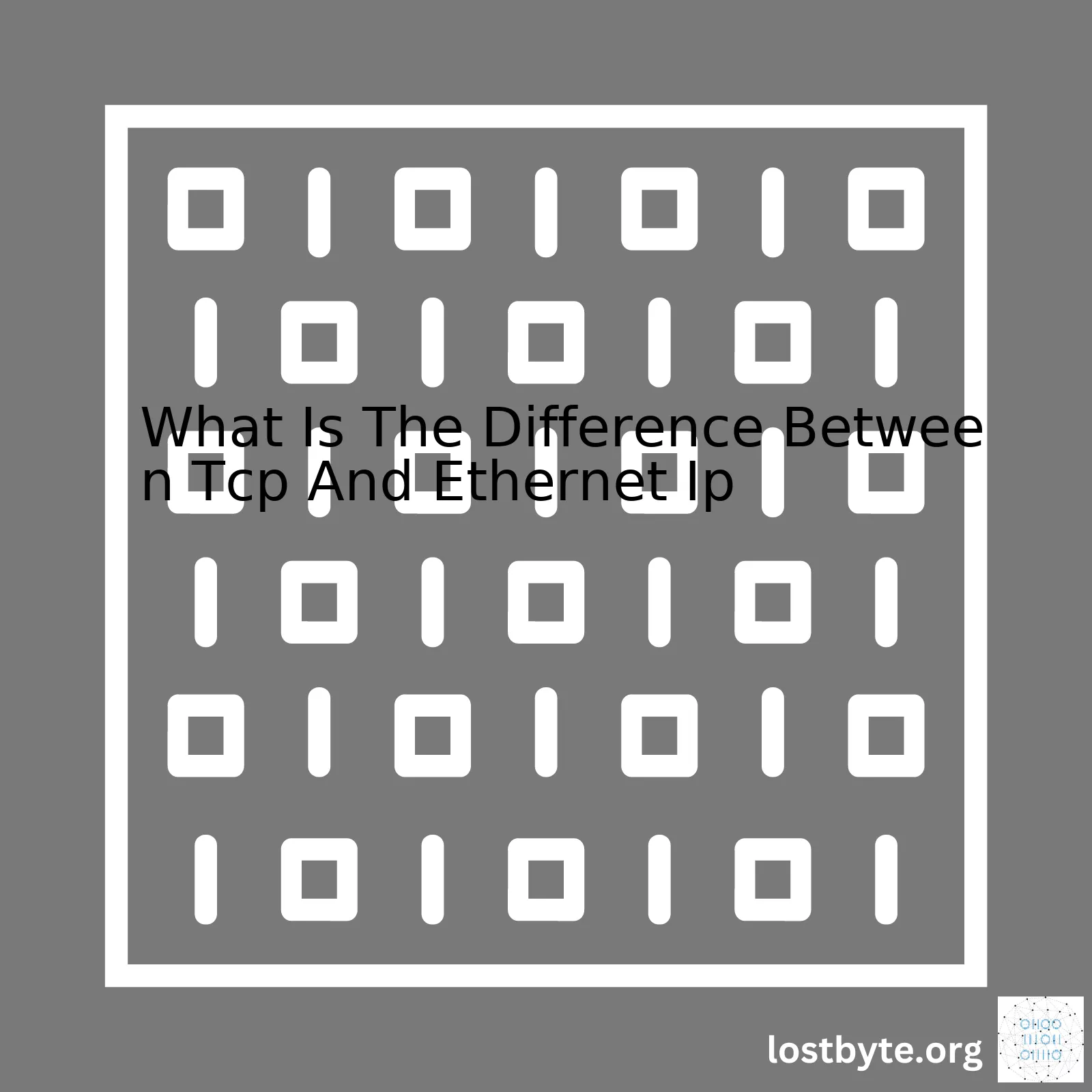
TCP | Ethernet/IP | |
---|---|---|
Full Form | Transmission Control Protocol | Ethernet Industrial Protocol |
Type of Protocol | Transport protocol | Network protocol |
Purpose | To provide reliable, ordered, and error-checked delivery of a stream of packets between applications running on hosts communicating via an IP network. | Used primarily for industrial automation tasks. It moves more complex data between devices and permits much more intelligent control of those devices. |
Data Transmission | Series-oriented connection | Either broadcast, multicast, or unicast pend upon the type of communication |
While both TCP and Ethernet/IP are protocols used in networking, their operations differ profoundly in nature. TCP, short for Transmission Control Protocol, is a transport protocol in charge of ensuring the reliable transmission of packets on the internet by verifying that each packet arrives at its destination. It’s series-oriented and provides a one-to-one form of communication.
On the other hand, Ethernet/IP (where IP stands for Industrial Protocol), is a network protocol used primarily in the realm of industrial automation. This protocol can offer robust control over devices by permitting the transmission of more complex data compared to standard TCP/IP. Ethernet/IP supports different forms of device communication such as broadcast, multicast, or unicast, depending on the type of communication needed.
For example, you might be familiar with HTML pages sent over TCP from web servers to your web browser. Here’s an illustrative code snippet:
from socket import * serverSocket = socket(AF_INET, SOCK_STREAM) serverSocket.bind(('', 6789)) serverSocket.listen(1) while True: print 'Ready to serve...' connectionSocket, addr = serverSocket.accept() try: message = connectionSocket.recv(1024) filename = message.split()[1] f = open(filename[1:]) outputdata = f.read() #Send header line into socket connectionSocket.send("HTTP/1.1 200 OK\r\n\r\n") #Send the content of the requested file to the client for i in range(0, len(outputdata)): connectionSocket.send(outputdata[i]) connectionSocket.send("\r\n") connectionSocket.close() except IOError: #Send response message for file not found connectionSocket.send("404 Not Found") #Close client socket connectionSocket.close() serverSocket.close()
In this code snippet, a simple web server is implemented that can respond to HTTP requests.
Whereas in case of Ethernet/IP, your interaction would involve programmable logic controllers (PLCs) in an industrial setting. However, unlike TCP where Python libraries are readily accessible for learning and experimental purposes, working with Ethernet/IP needs specific hardware and tools making the hands-on experience relatively limited.
Understanding these differences helps when designing systems that require network communications, as the appropriate selection of protocols according to their characteristics is essential for correct operation and optimal performance.
This
TCP (Transmission Control Protocol)
TCP is a transport protocol, operating in the transport layer of the Internet Protocol Suite, also known as TCP/IP suite. It’s responsible for sending and receiving data packets over the internet. TCP is part of Layer 4 or ‘Transport Layer’ of the OSI (Open Systems Interconnection) Model. Lifewire: Transmission Control Protocol
- Reliability: TCP ensures reliable data delivery with error checking and acknowledgment of packet reception.
- Ordered Data: If the sequence of data packets get mixed up while being transmitted, TCP rearranges them at the receiving end.
TCP connection establishment and termination typically follows a three-way handshake method, sending SYN(Synchronous), ACK(Acknowledgment), and FIN(Finish) signals respectively. This establishes a secure communication channel between the sender and receiver before any actual data transmission occurs.
The source code example below outlines the creation of a simple TCP socket in Python:
import socket # create a socket object s = socket.socket(socket.AF_INET, socket.SOCK_STREAM) # connect the client s.connect(('localhost', 12345)) # send some data s.send(b'Hello, world') # receive data from the server print (s.recv(1024)) # close the connection s.close()
Ethernet/IP (Industrial Protocol)
Ethernet/IP or simply EIP is an industrial protocol derived from the application layer of the Internet Protocol Suite stack. It utilizes Ethernet communication standards to interface real time control applications. EIP makes use of Common Industrial Protocol (CIP) and is part of the physical and data link layers (Layer 1 & Layer 2) of the OSI model. Ethernet/IP Index
- Real-Time Control: Ethernet/IP provides real time control by offering low latency and high-speed data transfer capabilities, perfect for industrial automation.
- Flexibility: Its application on Ethernet allows for wide-ranging scalability and interoperability across multiple devices, making it ideal for large-scale industries.
Ethernet/IP mainly uses UDP services (User Datagram Protocol) instead of TCP for establishing communication.
Differences between TCP and Ethernet/IP
TCP | Ethernet/IP |
---|---|
Operates at Transport Layer of OSI Model. | Operates at Physical and Data Link Layers of OSI Model. |
Used for general purpose internet communications. | Mainly used in industry for automation and control processes. |
Provides reliable data transmission with error checking and sequence maintenance. | Emphasizes on real-time control, low latency and high-speed data transfer even if reliability might be compromised due to no standard error checking mechanism like TCP. |
In summary, when deliberating over the choice between TCP and Ethernet/IP, your selection should encapsulate: the context – industrial or otherwise, the type of data being transmitted, and its significance towards real-time control.
TCP (Transmission Control Protocol) plays a crucial role in network communications. It is one of the main protocols in TCP/IP networks, ensuring reliable and ordered delivery of a stream of bytes from a program on one computer to another on a different computer over Internet Protocols(IP). This protocol uses acknowledgment packets to confirm successful transmissions.
A series of steps are undertaken by TCP to establish a connection:
- Syn – This step involves sending a synchronization message to start communication,
- Syn-Ack – The client machine receives the message, indicating that it’s prepared for a data relay,
- Ack – Finally, the client sends back an acknowledgment signal, completing the three-way handshake.
from socket import * s = socket(AF_INET, SOCK_STREAM) s.connect(('localhost', 80)) s.sendall("GET / HTTP/1.0\r\n\r\n") print s.recv(4096) s.close()
The above python code snippet establishes a TCP connection, sends a GET request, and closes the connection after receiving the response.
On the other hand, Ethernet/IP (Industrial Protocol) stands out as a standard network protocol established on top of Ethernet, allowing effective industrial networking. Unlike TCP, which manages the movement of data packets, Ethernet/IP controls how devices over an Ethernet network interact with each other. Instead of establishing connections like TCP, Ethernet/IP standardizes interactions between devices, such as sensors, robots, or interfaces.
Some of the differences between TCP and Ethernet/IP are:
TCP | Ethernet/IP |
---|---|
This protocol manages how packets get assembled and reassigned at the destination point. | This protocol manages device interaction standards over an Ethernet network. |
TCP provides reliable, ordered, and error-checked delivery of a stream of bytes. | Ethernet IP is used primarily for industrial networking systems (Factory Automation). |
Namely used in Web Browsing, Email, File Transfer etc. | Mainly used in devices such as PLCs, Sensors, Robots, Motor Drives, Bar Code Readers, etc. |
In essence, while both TCP and Ethernet/IP play crucial roles in network communication, they have decidedly different responsibilities. Where TCP serves to ensure streamlined communication via data packet management, Ethernet/IP is more focused on maintaining standards for inter-device interactions over Ethernet networks.
For in depth knowledge about network communications, check the online course on
Coursera.Understanding the difference between TCP and Ethernet/IP calls for a deep dive into what they are, how they work, and where they differ. So, let’s slice this topic up and serve it in an easy-to-grasp manner.
Ethernet/IP:
When you hear Ethernet/IP, it refers to the industrial protocol standard that is built upon Ethernet. Originally developed by Rockwell Automation and now managed by the Open DeviceNet Vendor Association (ODVA),1 Ethernet/IP uses the tools and technologies of traditional Ethernet but layers on top a series of industrial devices specific protocols for automation.
As such, it is highly applicable to environments where real-time control is essential like factory floors and various large-scale industrial scenarios.
TCP:
On the other hand, TCP (Transmission Control Protocol) exists as one of the main protocols within the Internet protocol suite. It deals directly with the assembly and disassembly of data packets sent over a network. Essentially, TCP ensures data integrity over the complex path of switches, routers, and diverse networks that comprise the internet.
Ethernet/IP Attributes (relative to its difference from TCP)
Real-time Control | Ethernet/IP was designed explicitly for real-time control in industrial settings. |
Common Industrial Protocol (CIP) | Ethernet/IP employs CIP, allowing various networks to use common devices and applications. |
Deterministic communication | Ethernet/IP has deterministic communication capabilities, reducing latency in command transmission and responses. |
TCP Attributes (relative to its difference from Ethernet/IP)
Data Integrity | TCP ensures the appropriate assembly and disassembly of data packets, maintaining data integrity across multiple networks. |
Wide Use Across the Internet | TCP forms the bedrock layer of internet communication, enabling the breakdown of complex data into manageable packets for delivery. |
Error checking | TCP performs error checking to avoid duplications or lost information during transit. |
The main differences between Ethernet/IP and TCP sit primarily in their design rationale. The former exhibits characteristics optimized for robustness, real-time control requirements peculiar to industrial automation. In contrast, TCP shines in ensuring data integrity, reliability, and order across complex network paths typical of the Internet.
An Ethernet/IP Packet includes details pertinent to industrial automation protocols, such as Object Class, Instance ID, Attribute ID etc. For instance,
Ethernet/IP packet: {CIP encapsulation header: [session handle, status, sender context, options }, {CIP command: [path size, request path], {CIP service: [object class, instance ID, attribute ID]} ]}}
In comparison, a TCP Segment carries more generic information – Source & Destination ports, Sequence Number, Acknowledgement number, Header Length, Flags, Window Size, Urgent Pointer, Option etc. Each detail here forms part of the breakdown of data into packets for reliable delivery.
TCP segment: {Source port, Destination Port, Sequence Number, Acknowledgment Number, Header Length, Reserved, Flags, Window Size, Checksum, Urgent pointer, Options, Padding, data...}
In summary, the principal divergence between TCP and Ethernet/IP roots in their tailored functionality – the former for data integrity across diverse, expansive networks, and the latter for advanced, real-time control in industrial settings.Following is an analysis on the fundamental differences between Transmission Control Protocol (TCP) and Ethernet Industrial Protocol (Ethernet/IP).
Ethernet/IP
The Ethernet Industrial Protocol, or Ethernet/IP, as explained by ODVA, was specifically designed for factory floor networks. Ethernet/IP uses the Ethernet infrastructure to carry out its tasks efficiently, such as access control, routing, and device naming – in both manufacturing and automation industries.
You can think of Ethernet/IP like a common language that your industrial devices use to communicate, including input/output messages and services over an Ethernet network. Industrial protocol is essentially encapsulated into an Ethernet frame which enables interoperability of various participating nodes such as robots, drives etc.
Some key features of Ethernet/IP:
- It uses standard IP-based communication across devices.
- Complies with IEEE 802.3 Ethernet Standard since it is based on Ethernet technology.
- Supports real-time I/O messaging and high-speed connections for motion control.
TCP (Transmission Control Protocol)
Transmission Control Protocol, or TCP, is a transport protocol in the Internet protocol suite, responsible for providing reliable, ordered and error-checked delivery of data packets between systems over the internet.
According to Wikipedia, TCP is a connection-oriented protocol, meaning it ensures a proper data transfer from source to destination. TCP sequentially orders data so that they can be tracked and ensures that data are shielded from damage, loss, or any alterations during transit.
Fundamental characteristics of TCP:
- It is generally used along with IP within the Internet Protocol Suite; therefore, you might often hear the term TCP/IP.
- Operates at the Transport Layer of the OSI model offering end-to-end communication.
- Ensures reliability using acknowledgement packet receipt system.
Explicit Comparison: Ethernet/IP vs TCP
To give you an insight on how these two differ from each other, here’s a quick comparison:
Ethernet/IP | TCP | |
---|---|---|
Network Type | Primarily designed for Industrial usage | Used in general IP-based network communication |
Data Handling & Integrity | Real-time management of industrial data | Reliable transmission of data packets ensuring error-checking |
Connection Type | Uses standard IP-based connections | Connection-oriented or ‘Stateful’ method of data transmission |
In conclusion, Ethernet/IP and TCP serve different purposes and are utilized in diverse domains. They operate on different layers of the OSI model and have unique characteristics catering to their respective fields. Ethernet/IP concerns itself with managing real-time industrial data using IP-based connections, while TCP focuses on maintaining the integrity and reliability of data transmitted over a broader internet framework.
The difference between TCP (Transmission Control Protocol) and Ethernet IP (Internet Protocol) can be quite substantial, even though they both play crucial roles in the realm of data transmission on networks.
TCP: Transmission Control Protocol
TCP is a transport protocol that manages data transmission in network systems.
Firstly, to underpin its importance, let me give you a high-level overview of its attributes:
1. Connection-oriented – A connection is established and maintained until such time as the user (or programs at each end) closes the connection.
2. Order-preserving – Data sent first comes first, avoiding disorder.
3. Error-checked – The receiver can detect if any form of damage has occurred during transmission or not.
4. Delivery guaranteed – Missing data are retransmitted.
To further elaborate, consider this piece of code which outlines a simple TCP client-server communication:
//Server side #includeint socket_desc , new_socket , c; socket_desc = socket(AF_INET , SOCK_STREAM , 0); //Client side #include int socket_desc; socket_desc = socket(AF_INET , SOCK_STREAM , 0);
The above code snippet utilizes the architecture provided by our beloved TCP. Every data packet transferred via TCP has its header to identify the source and destination ports, sequence and acknowledgment numbers, among other things.
Ethernet/IP: Internet protocol over Ethernet
Ethernet IP, on the other hand, is a member of the internet protocol suite and sits one layer below TCP on the network protocol stack, namely the network interface layer. It’s responsible for receiving TCP packets from the upper layer, packaging them into IP frames, then delivering these frames to the right device over the ethernet cable.
Some of Ethernet IP’s characteristics include:
1. It works with physical addresses (MAC).
2. It is connectionless – meaning data is transmitted without setting up a direct connection.
3. It does not offer guaranteed delivery – lost packets need to be detected and resent by higher layers.
In simpler terms, Ethernet IP does what a post office would do when dealing with letters. In this analogy, think of TCP as only responsible for writing the letter. It makes sure the message is correctly written before it’s given to the post office (Ethernet IP). Upon receiving the letter, the post office puts it into an envelope (an IP packet), writes the destination address on it, and sends it out.
To indicate how this works, here’s a simplified pseudo-code example showing encapsulation of TCP packet in an Ethernet frame:
// Creating and sending a TCP packet, encapsulated in an IP datagram (and further in an Ethernet frame) #includeint socket_desc; struct sockaddr_in server; char *message; //Initiate the socket socket_desc = socket(AF_INET , SOCK_RAW , IPPROTO_TCP); //Details about the server server.sin_addr.s_addr = inet_addr("10.0.0.10"); server.sin_family = AF_INET; server.sin_port = htons(80); //Message body message = "This is the message body"; //Send the message sendto(socket_desc , message , strlen(message) , 0 , (struct sockaddr *)&server , sizeof(server));
To weave these concepts together, TCP and Ethernet IP are complementary sides of network data transmission, operating at different levels. While TCP cares about the content and order of the data being shipped, Ethernet IP is more concerned about the packaging and transportation to the right address.
Source SourceSure, we can delve into the fascinating world of internet protocols and specifically look at Transmission Control Protocol (TCP) and Ethernet IP, which are two integral networking protocols that enable us to connect and communicate over the internet. These two protocols serve different purposes within the networking stack, with TCP handling transport and session management and Ethernet IP being responsible for data link & delivery tasks.
The standards used by each protocol are vital to ensure robust error detection processes, yet they differ in their methods. Let’s take a closer look at how TCP and Ethernet IP handle error detection:
Transmission Control Protocol (TCP)
TCP is a connection-oriented protocol providing end-to-end delivery of data over an IP network with assurance that packets will arrive in sequential order.
For error detection, TCP uses a mechanism known as a checksum. In simple terms, the checksum is a sum of all the bytes in the packet’s data. To make it even more reliable, before sending, TCP ‘adds’ up all the data in a packet. The receiving end then ‘adds’ the data in the received packet. If these sums are different, then some data were lost or corrupted during transmission, highlighting an error.
So effectively,
- TCP captures/creates a segment containing a header (which includes control information like sequence number and checksum) and the actual data.
- This data gets delivered to the recipient through IP.
- The recipient’s TCP looks at the segment, recalculates the checksum and compares it with the received one. If they match, we accept the data; if not, we reject the data.
Here’s an example of how a TCP packet’s checksum is calculated, using hypothetical values:
TCP_Segment = [Header + Data] Original Checksum (in Header) = 27684 Data = [byte1, byte2,...] Calculate Checksum: Sum(data) = New Checksum If (Original Checksum == New Checksum) → Accept TCP Segment Else → Reject TCP Segment
Ethernet IP
On the other hand, Ethernet IP is primarily a data-link protocol and essentially defines how data is formatted for transmission and how access to the physical medium is controlled. It specifies how devices on the network share resources and how large data blocks (frames) are broken down into packets and reassembled.
Ethernet’s error checking is using Cyclic Redundancy Check (CRC). CRC is considered superior to checksums because it’s based on polynomial equations which offer better error detection capabilities especially in detecting burst errors. If the CRC values do not match when comparing the sent and receive frames, the frame is discarded.
Let’s simplify this with another example:
Ethernet_Frame = [Header + Data + CRC] Original CRC (in Frame) = 34567 Data = [byte1, byte2,...] Calculate CRC: Apply_CRC(data) = New CRC If (Original CRC == New CRC) → Accept Ethernet Frame Else → Discard Ethernet Frame
In a nutshell:
- Both TCP and Ethernet IP have error detection mechanisms.
- TCP uses checksum while Ethernet uses CRC.
- CRC is generally regarded as more robust against burst errors compared to checksum.
This discussion just scratches the surface of TCP and Ethernet IP protocols, but it should provide you with an idea of how both work, what roles they play, and most importantly, how they handle error detection capability.
For further deep dive on these topics, feel free to refer on Wikipedia TCP | Wikipedia Ethernet Frame.Sure, let’s unpack the significant differences between Transmission Control Protocol (TCP) and Ethernet/IP, highlighting their efficiency factors.
Transmission Control Protocol (TCP)
TCP is a standard protocol that outlines how to establish and maintain a network conversation through which applications can exchange data. It supports reliability, orderliness, and error-checking in data transfer operations.
Key Efficiency Factors of TCP:
- Reliable Delivery: TCP ensures data integrity by using acknowledgements, retransmission of lost data, sequencing, and error-detection mechanism. This means if any packets are lost during transmission, TCP will recover those missing packets.
- Ordered Data Transfer: The feature of sequencing present in TCP makes certain the correct order delivery of packets. Even if the packets arrive out of sequence, TCP rearranges these to restore the original order.
// Example: in file transfer over TCP, received file maintains same arrangment as sent file
- Error Checking: TCP includes a checksum in its header that allows identification and correction of errors that might have occurred during transmission.
// Example: Correcting bit errors on receiving side
Ethernet/IP (Industrial Protocol)
Ethernet/IP is an industrial automation networking protocol that adapts the Common Industrial Protocol over standard Ethernet.
Key Efficiency Factors of Ethernet/IP:
- Information Sharing : Ethernet/IP uses both unicast and multicast packet distribution allowing more than one device to receive information at the same time; thereby increasing network efficiency.
- Incorporation of CIP : The encapsulation of Common Industrial Protocol (CIP) allows it to share application with other CIP networks. This approaches results in increased compatibility and interoperability.
- Real-Time Control : Ethernet/IP includes features for real-time control over output and input; thus ensuring quick actions based on processed information.
Here’s what you need to understand – while being different protocols operating at different layers of the OSI modelsource, both TCP and Ethernet/IP play their own crucial roles in communication systems. TCP operates at the transport layer, providing reliable and ordered data transmission. On the other hand, Ethernet/IP operates at the bottom layers (Data Link & Network), providing real-time control over the network’s physical components and orderly information sharing among devices.
However, these unique efficiency factors don’t make one protocol superior to the other. Their effectiveness depends on the nature of your specific use case, since various network scenarios require diverse functionality.
A real-life scenario could involve a factory monitoring system where Ethernet/IP would be preferred because of its real-time control. Simultaneously, transferring files over the internet wherein data integrity is paramount, TCP would be more efficient.
// Possible code snippet demonstrating TCP and Ethernet/IP \\ Establish TCP connection ip = gethostbyname('www.example.net'); sock = socket(AF_INET, SOCK_STREAM, 0); connect(sock, ip->h_addr_list[0], ip->h_length, htons(80)); \\ Send and Receive Data Over Ethernet/IP new RackDevice("Device1", "192.168.100.1"); \\create a new instance of a device Device1.getInputASCIIData(Module, StartChar, Length); \\<- read input data Device1.setOutputASCIIData(Module, StartChar, DataOut); \\<- send output data
I hope this analysis clearly paints a picture of the inherent efficiency factors of TCP and Ethernet/IP, illustrating the essential difference between these protocols.
Remember, it all comes back to aligning the right protocol with your networking needs.
Distinguishing Between TCP and Ethernet IP
Although they essentially perform similar tasks, there are fundamental differences between TCP and Ethernet/IP that affect their use in specific scenarios.
TCP is a transportation protocol sitting in the transport layer of the OSI model widely used in internet communications. It's characterized by:
- A connection-oriented protocol- requiring that a connection be established before any data transfer occurs
- Guaranteed delivery- TCP uses acknowledgment messages to ensure the safe delivery of sent data
- Error Detection and Correction – Data transmitted using TCP includes a checksum for error detection. If errors occur, TCP has mechanisms in place to resubmit the data packets.
On contrary, Ethernet IP is an industrial automation networking system based on standard Ethernet TCP/IP and UDP/IP protocols, thus it relies on upper-layer protocols like TCP and UDP. Unique attributes of Ethernet IP include:
- Real-Time Communication: By making use of the UDP protocol, Ethernet IP supports real-time I/O messaging for high-performance automation applications.
- Natively provides CIP services: A primary strength of Ethernet IP rests in its ability to natively provide Common Industrial Protocol (CIP) services such as control, safety, energy, synchronization & motion, and information.
- End-to-End Connection: Ethernet/IP effectively integrates devices across the plant floor, making them part of the enterprise network.
When to Use TCP or Ethernet IP?
Application's Need for Guaranteed Delivery
if (application.requiresAcknowlegement()) { /* Suitable for TCP */ } else { /* Either TCP or Ethernet IP could work here */ }
If your application involves a mission-critical task and cannot afford loss of data packets, opting for TCP would be strategic due to its guaranteed delivery feature.
Requirement of Real-time Communication
if (application.requiresRealTimeCommunication()) { /* Ethernet IP would typically be more suitable with the usage of UDP */ } else { /* TCP could serve you better for non-real time communications */ }
If your application involves high-performance automation or requires real-time communication, then Ethernet IP would generally be more favorable since it can utilize UDP protocol which is fast and more suited for real-time operations.
The Complexity of Application Design
if (application.complexInDesign()) { /* Due to its robustness, TCP is better suited for complex applications */ } else { /* Simpler designs might not require the comprehensive features offered by TCP */ }
Complex application designs often benefit from the robustness and advanced features provided by TCP, enabling the creation of reliable, high-performing applications.
In summary, TCP may be more advantageous in guaranteeing data packet delivery, handling complex application designs, and providing a broad range of services. On the other hand, Ethernet IP shines in areas where speed, real-time communication, and scalability are key considerations.
The variations in security protocols of Transmission Control Protocol (TCP) and Ethernet/Industrial Protocol (Ethernet/IP) are influenced by foundational differences in the networking layers they operate on, their intended applications, and inherent design features that impacts their vulnerability to threats. We'll delve deeply into these variations to get a comprehensive understanding of how TCP and Ethernet/IP vary in terms of security.
TCP Security
TCP is a transport layer protocol which is responsible for establishing a reliable connection between devices over an IP network (source). The security considerations for TCP primarily rest on its susceptibility to common types of attacks:
- SYN flood attack: Caused by an attacker sending a succession of SYN requests to a target's system, it attempts to consume enough server resources to make the system unresponsive to legitimate traffic. - TCP/IP hijacking: This occurs when an unauthorized user takes over a TCP/IP connection between two computers. Since TCP/IP does not provide native security features, it can be vulnerable to this type of attacks. - Sequence prediction: Each byte sent across a TCP connection has a sequence number. If this number can be correctly guessed by an attacker, they could potentially inject malicious packets into the connection.
Ethernet/IP Security
Ethernet/IP, on the other hand, is at the data link layer protocol used typically for industrial automation systems due to its real-time capability. It enables devices to communicate over Ethernet (source). The following security vulnerabilities arise in the context of Ethernet/IP:
- Packet sniffing: Data packets traveling through an Ethernet network can be intercepted and read by any computer on the network. - MAC flooding: An attacker overloads the switch with packets, each of which carries different source MAC addresses. When the switch's capacity is exceeded, it enters into 'fail-open mode', broadcasting incoming packets to all ports and making the network susceptible to packet sniffing. - ARP spoofing: Under this attack, an intruder creates false ARP messages to link their MAC address with the IP address of a legitimate computer or server on the network.
Each protocol employs a different strategy for countering security threats. TCP relies heavily on trustworthy third-party solutions such as Secure Socket Layer/Transport Layer Security (SSL/TLS) or Internet Protocol Security (IPSec). SSL/TLS provides secure communication at the application layer by encrypting the data, while IPSec provides security at the network layer, protecting the IP packets.
For Ethernet/IP, security measures include using Virtual LANs (VLANs), which isolate network traffic to a specific group of devices regardless of their physical location, thereby increasing network efficiency and reducing the potential for packet sniffing. Additionally, implementing IEEE 802.1X port-based Network Access Control can authenticate devices before allowing them access to the network, thus preventing unauthorized access and MAC flooding.
In conclusion, while TCP and Ethernet/IP differ greatly in their inherent security protocols, both offer their own unique advantages and trade-offs depending on the context in which they are applied – whether in general internet applications or industrial control systems respectively. Understanding these differences will help in designing a more robust and secure network infrastructure.The comparison of TCP (Transmission Control Protocol) and Ethernet IP resides primarily in their functionality. TCP operates at the Transport layer of the OSI model, while Ethernet operates at the Data link layer.
Looking first at TCP, it’s pivotal to understand that it is a protocol designed for transmitting data over network devices like computers. To comprehend it further, let's delve into the specifics:
TCP
TCP utilizes a strategy known as 'connection-oriented' (source). Essentially, before transmission commences, TCP establishes a connection between the sender and receiver. Consider this transaction like a phone call where before exchanging words, both parties must connect.
The code snippet below uses the Python socket library to create a TCP connection.
import socket # Create a socket object s = socket.socket(socket.AF_INET, socket.SOCK_STREAM) # Connect to the server s.connect(("www.google.com", 80))
This process involves three phases: synchronization, data transfer, and termination, often called a three-way handshake.
Features of TCP include:
- Reliability: TCP verifies that all data segments have been received successfully. In any case of loss or corruption, the missing packets are sent again.
- Ordered Data Transfer: Segments are numbered sequentially, ensuring systematic data reassembly at the destination.
- Error Checking: Header checksums are used to identify corrupted data. If an error occurs, the sender is notified, and lost packets are retransmitted.
- Congestion Detection Control: TCP detects network congestion and limits its data transfer rate accordingly.
Conversely, Ethernet IP is a part of LAN technology responsible for permitting communication among devices with MAC addresses. It is utilized for data-link communication within a network segment.
Ethernet IP
Ethernet IP enhances connectivity by utilizing CIP (Common Industrial Protocol), which permits compatibility with several automation applications of industry (source).
Ideal for industrial environments, EtherNet/IP supports transfer modes of various types, including messaging-based I/O, hard real-time connections, and standard UDP/IP and TCP/IP communication. This capability gives flexibility to users considering the device implementation.
Consider a scenario where data needs to be transferred through an Ethernet frame using Java. The data's structure would look something like this:
EthernetFrame ethernetFrame = new EthernetFrame( "FF:FF:FF:FF:FF:FF", // Destination MAC Address "00:0A:95:9D:68:16", // Source MAC Address EthernetFrame.ETHERTYPE_IP, // Ethertype data); // Data carried by this Ethernet frame
So, what are the primary features associated with Ethernet IP?
- Compatibility: Devices from different vendors can seamlessly interact due to standardized communication practices.
- Data Delivery: Capable of prioritizing real-time I/O data, reducing possible system latency.
- Security: Provides mechanisms to ensure limiting access to authorized personnel only, contributing to robust security.
Therefore, when comparing speed aspects, keep in mind that both TCP and Ethernet IP serve different roles within a network infrastructure. TCP ensures reliable connection over a wide range of applications, possibly impacting speed. On the other hand, Ethernet IP focuses on the quick delivery and interoperability within a localized environment. Consequently, choosing between the two depending on your specific requirements could mean trading off between reliability, speed, and compatibility.At the core of numerous network communications, TCP (Transmission Control Protocol) and Ethernet IP (Internet Protocol) play significant roles. However, there lies a principal distinction between the two regarding their operating layers on the OSI model and their flow control mechanisms. It’s essential to comprehend such differences because it determines how data is transmitted over networks efficiently, which impacts every internet-connected device's performance.
TCP operates at the Transport layer of the OSI model - the fourth layer - and it's in charge of providing end-to-end connectivity and confirmation of packet delivery. Such features are facilitated by TCP's flow control mechanism, which unfortunately Ethernet IP lacks since it operates at a lower level in the OSI model.
Let's delve deeper into how the flow control mechanism works in TCP, but first, it's important to look into TCP more closely.
TCP is designed to give reliable, ordered, and error-checked delivery of a stream of bytes between applications running on hosts communicating via an IP network. Its flow control mechanism aids in controlling data packet transfer rate from the sender to the receiver to ensure no data packets get lost in transit due to overflow conditions, which can occur if the sender transmits data packets faster than what the receiver can handle.
The primary means by which TCP implements its flow control is through the use of a sliding window technique. Here's a piece of pseudocode explaining the basic concept:
while (true) { receivePacket = receiveTCP(); sendAckFor(receivePacket); slideWindowTo(receivePacket.end()); }
The `receiveTCP()` function collects a new packet from the stream. The `sendAckFor(receivePacket)` mechanism sends back an acknowledgment for the current packet, while `slideWindowTo(receivePacket.end())` moves the receiving window towards the end of the packet received.
Contrastingly, the Ethernet IP protocol operates solely at the Network interface layer (the second layer in the OSI model), and as such, it primarily serves the purpose of connecting devices within a local network. Since it operates at a lower package level, Ethernet IP does not inherently incorporate robust flow control or packet acknowledgement mechanisms similar to TCP. It's principally concerned with transferring packets over network interfaces; see below a rough pseudocode visualization of this:
while (true) { incomingDataPacket = receiveEthernet(); if (dataIsValid(incomingDataPacket)) sendEthernet(incomingDataPacket); }
In the above pseudo-code, `receiveEthernet()` receives an incoming data packet and if the data is valid confirmed by `dataIsValid(incomingDataPacket)` then it passes the packet using `sendEthernet(incomingDataPacket)`.
Finally, the Ethernet IP utilizes MAC addresses for devices identification within the network. It essentially transfers data packets from one hardware (network interface card) to another on the same network segment. These fundamental elements encompass Ethernet IP's mandate.
Having understandable disparities between the operational principles of TCP and Ethernet IP is outstanding. TCP provides a robust flow control mechanism that ensures packet transmission reliability across networks (end-to-end). In comparison, Ethernet IP, being at a lower level in the OSI model, primarily focuses on ensuring devices within the same network segment manage to communicate successfully, but it doesn't intrinsically offer flow control-like capabilities like TCP.
When we delve into the world of data sequencing, it is impossible to overlook two vital protocols: Transmission Control Protocol (TCP) and Ethernet/IP. Both these mechanisms have their own unique characteristics and areas where they exhibit optimum performance. Therefore, understanding their differences becomes pivotal.
Data Sequencing in TCP:
TCP or Transmission Control Protocol is a transport-layer protocol that provides reliable, ordered and error-checked delivery of data packets. It functions on the principle of the Internet Protocol Suite, commonly known as TCP/IP. An essential aspect of TCP is that it ensures data sequencing with its inherent mechanism for maintaining the order of packets sent over a network. In simple terms, each data packet transmitted via TCP is assigned a specific sequence number which helps in sorting at the receiving end. This numbered arrangement is beneficial in situations like video calls or gaming wherein the order of data matters significantly.
[source](https://networkengineering.stackexchange.com/questions/30976/difference-between-tcp-and-ip)
#sample TCP code snippet in Python, using socket module import socket s = socket.socket(socket.AF_INET, socket.SOCK_STREAM) s.connect(('www.google.com', 80)) request = "GET / HTTP/1.1\nHost: www.google.com\n\n" s.send(request.encode())
Data Sequencing in Ethernet/IP:
Ethernet/IP, standing for Ethernet Industrial Protocol, works on the application layer in the Open Systems Interconnection (OSI) model. Utilizing the robustness of Ethernet and structuring atop the Common Industrial Protocol (CIP), Ethernet/IP offers broad data accessibility and compatibility with industrial equipments like PLCs and I/O devices.
In contrast to TCP, Ethernet/IP does not offer an intrinsic mechanism to maintain the sequence of data transmission. Instead, Ethernet/IP has been designed to prioritize real-time communication, providing run-time data access, control, and configuration information. Owing to this focused feature, Ethernet/IP is primarily deployed in industrial automation processes.
[source](https://www.odva.org/What-is-EtherNet-IP/Introduction-to-EtherNet-IP)
Differences between TCP and Ethernet/IP:
Feature | TCP | Ethernet/IP |
---|---|---|
Data Sequencing | TCP maintains an inherent mechanism to keep the sequence of the data packets being transmitted. | Ethernet/IP does not specify any built-in data sequencing system. |
Application Area | TCP is ubiquitous and used for several general-purpose applications such as web browsing, file transfer and e-mailing. | Ethernet/IP is typically used for real-time industrial process monitoring and control. |
Reliability | TCP ensures reliable data transmission by including error-checking facilities. | Ethernet/IP, being based on UDP, often prioritizes speed over reliability of data transmission. |
All in all, when dealing with data sequencing and other related challenges, your selection between TCP and Ethernet/IP would largely depend on the specifics of the tasks at hand.
References:
In dissecting each network protocol, the differential factors between TCP (Transmission Control Protocol) and Ethernet/IP (Industrial Protocol) lay mainly in operational influence, data transmission approach, and specific design principles.
TCP is a transport protocol, that provides the service of delivering packets from the source host to the destination host solely based on the IP addresses in the packet headers. It stands out for its reliability, flow control, and ensures all packets reach their destination without error and in order.
// Pseudo code for TCP transmission if(packet is received) { send acknowledgement; } else { retransmit packet; }
On the other hand, Ethernet/IP is an application layer protocol used virtually everywhere in the industrial environment. It relies on both TCP and UDP for transportation of CIP objects. Its unique selling point is that it allows individual devices to communicate directly with each other in a plant-floor type of environment.
To encapsulate the prime comparative aspects:
TCP | Ethernet/IP |
---|---|
Transport layer-end-to-end connection | Application layer-open network |
Ensures reliable transmission | Relies on TCP/ UDP for transmission |
Data sequenced properly | Object-oriented information encapsulation |
In the realm of network protocols, TCP and Ethernet/IP are not competing but rather, they work together in various areas of network communication. They each serve different purposes, targeting diverse segments within data transmission processes. For a more comprehensive understanding of these two concepts, I would recommend reading Introduction to TCP/IP and Introduction to Ethernet/IP.