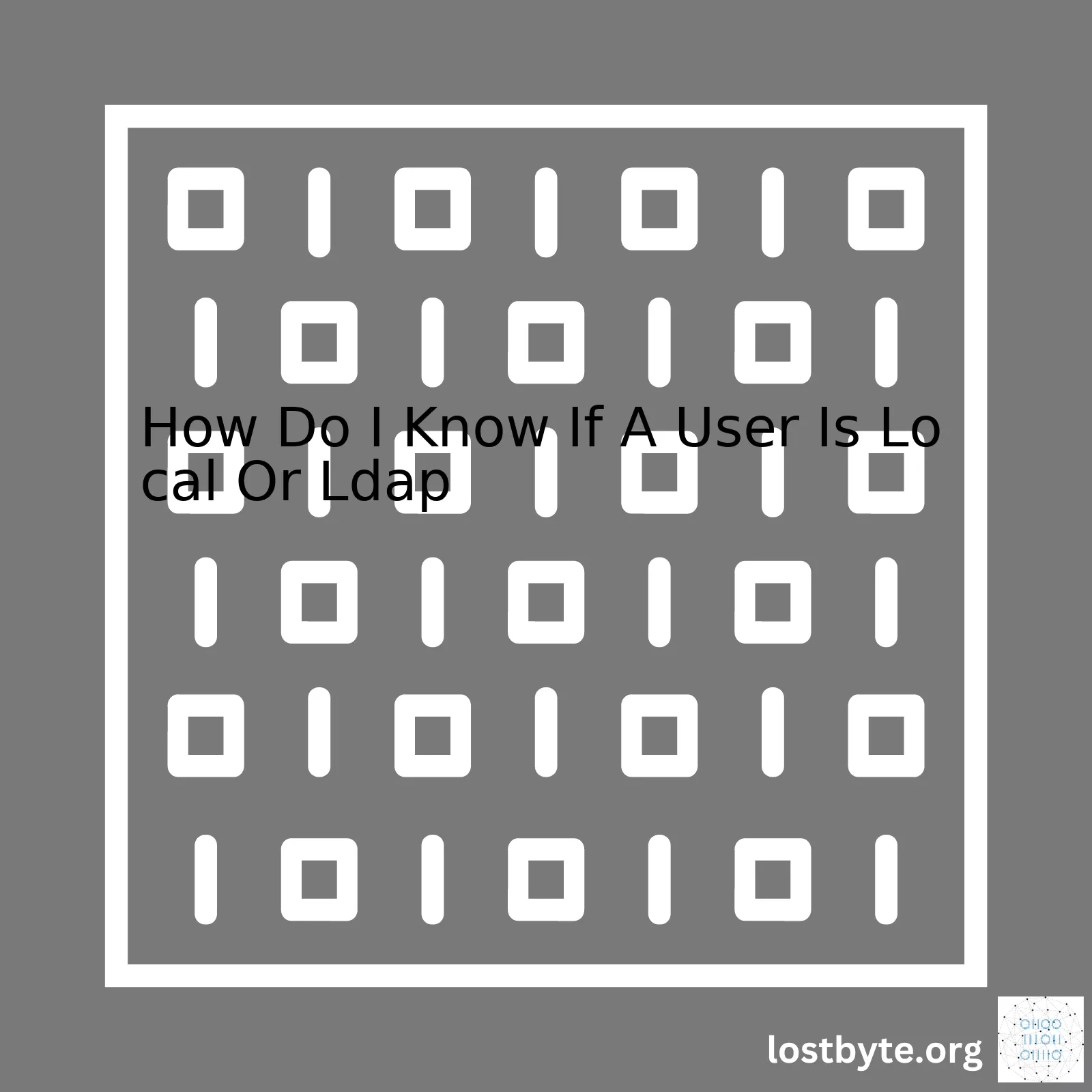
Type of User | Identification Method |
---|---|
Local | Exists directly on the system; can be found in the /etc/passwd or /etc/shadow files |
LDAP | Exists in an LDAP directory; “getent passwd” command returns them |
Determining if a user is local or LDAP fundamentally comes down to where their account information is stored and retrieved from. By default, Unix-like systems (like Linux) store user account information, such as usernames and hashed passwords, locally in the /etc/passwd or /etc/shadow files. This method is simple and doesn’t require any additional infrastructure beyond the machine itself, but lacks certain features and scalability.
You can check the existence of a local user by manipulating these files, for instance using
grep '^username:' /etc/passwd
, where username is replaced by the name of the user you’re querying about, should return a line of text if the user exists locally. It will return nothing if the user is not local.
On the other hand, large organizations or applications might opt to use LDAP (Lightweight Directory Access Protocol), a software protocol for enabling anyone to locate organizations, individuals, and resources such as files and devices in a network. Since LDAP users exist in a separate LDAP directory rather than directly on the system, the “getent passwd” console command (which gets entries from administrative database – specifically, the user account entries) would display both the local and the LDAP users in its output.
By doing something like
getent passwd | grep '^username:'
, where again username is the name of the user you’re investigating, it should return a string if the user exists either locally or in the linked LDAP directory. If you’ve already checked the /etc/passwd file though, and you know the user isn’t local, then a successful result from this command indicates that the user exists in your LDAP directory.
Thus, to tell whether a user is local or LDAP, you can use these different commands and observe the output. This makes it possible to fine tune access control for your application or service and implement the right authentication strategies.
Understanding User Authentication: Local vs LDAP
The process of confirming the identities of users trying to access a system is called “user authentication”. Two common techniques for user authentication are local and LDAP (Lightweight Directory Access Protocol).
Local Authentication
In local authentication, users’ credentials are verified against locally stored data within the application or system. The username and password combination are typically stored in a database that’s directly connected to the app. Once a password is entered by the user, it’s transformed using a secure hash function and then compared with the hashed value stored in the local database.
// Local password verification sample function verifyPassword(inputPassword, storedHash) { const inputHash = secureHashFunction(inputPassword); return inputHash === storedHash; }
The prime advantage of local user authentication is its simplicity and self-contained nature. It doesn’t require any external communication interfaces.
LDAP Authentication
On the contrary, LDAP authentication is used when there’s a requirement to verify user credentials against an external directory over the network, which could be a company’s active directory for enterprise applications. In this approach, the app sends a request to an LDAP server which validates the credentials.
// Sample LDAP authentication import ldap from 'ldapjs'; const client = ldap.createClient({ url: 'ldap://my-ldap-url' }); const opts = { filter: `(uid=${username})`, scope: 'sub', }; client.search('ou=users,o=example', opts, function(err, res) { // Handle result });
LDAP offers a centralized way of managing users across multiple systems.
How Do I Know If A User Is Local Or LDAP?
To identify if a user is authenticated through local system or LDAP, it largely depends on how the authentication process was designed in your application.
- If you’re using both local and LDAP authentication means, then during the sign-up or registration process, implement a mechanism where the user type gets stored in the local database. A ‘userType’ field tied to each account can indicate if a user is ‘local’ or ‘ldap’.
- Apart from the aforementioned method, once a user is logged in, you can check the authentication provider in your backend logic. For instance, if a user’s credentials were confirmed using an LDAP query then you can safely assume that the user is LDAP-authenticated. Conversely, if the password hash stored in the local database was used for verification, then it’s a local user.
- Finding out a user’s authentication source can also be checked by the audit logs, depending upon how detailed your logs are in capturing the authentication process details.
Still, bear in mind that the design should ensure seamless experience for users, regardless their authentication type. In other words, they do not need to explicitly know whether they are local or LDAP users.
For more detailed exploration, here’s a helpful article about Understanding Identity, Authentication, and Access Control.
Distinguishing between Local and LDAP users is essential when dealing with user authentication process. It’s not always straightforward to determine this, especially if you’re new to a system.
- Checking the /etc/passwd file
One basic way to find out whether a user is local or LDAP is by checking the
/etc/passwd
file. Linux systems use this file to store local users’ information. Users under
/etc/passwd
are considered local. Here’s an example of how you can look up a user:
Example:
$ grep 'username' /etc/passwd
If the command returns a line similar to the following:
username:x:503:503::/home/username:/bin/bash
This indicates that ‘username’ is a local user because their account details are defined in the
passwd
file.
- Using Getent Command
Another approach is using the
getent
command. This command checks the central database (defined in /etc/nsswitch.conf) for users, and it will include both local and LDAP users:
Example:
$ getent passwd username
Again, if a line similar to below is returned,
username:*:1234:5678:LDAP User:/home/username:/bin/false
Then ‘username’ could be an LDAP user – signified by the mention of “LDAP User” in the results. But do note that absence of this does not necessarily mean the user is local. Some checks might still be needed as we’ll explain next.
- Pam configuration checks
Thorough analysis involves checking PAM configurations. PAM, standing for Pluggable Authentication Modules, is essentially the mechanism Linux uses to handle authentication jobs. If you go to
/etc/pam.d/
directory and perform a
grep
for ‘pam_ldap’, you should see which services are configured to rely on LDAP for user authentication.
Example of this check might look like:
$ sudo grep -r 'pam_ldap.so' /etc/pam.d/
- Check LDAP configuration files
Finally, you could also check LDAP configuration files for indications of how the server is set up. The ‘ldap.conf’ located in
/etc/openldap/
or
/etc/ldap/
directories would indicate servers being utilized. If these locations don’t have relevant conf files, chances are high that the system isn’t using LDAP.
Example:
$ cat /etc/openldap/ldap.conf OR $ cat /etc/ldap/ldap.conf
Remember, while these examples provide guidance, they’re not absolute truth. Different entries exist depending on your unique setup, used programs, and utility versions. In most cases, it tends to be a combination of these methods that best helps distinguish between Local and LDAP users, bolstering your ability to troubleshoot and understand your system’s setup.Determining if a user is Local or LDAP can seem like a daunting task when you’re new to the field of coding. However, once you become familiar with the core differences and understand how to use certain tools, it becomes significantly easier.
Local Users:
A local user is typically someone who is registered directly on your machine. To identify local users, you can do so by:
- User Directories: Local users will have their own directory in
/home/[username]
. If there is no home directory present for a user, it suggests that the user may not be set up correctly or possibly doesn’t exist.
- /etc/passwd File: Another effective way to check if a user is local is by cross-verifying the
/etc/passwd
file where user account information is stored. You can use the below command:
grep '[userId]' /etc/passwd
If the above command returns any output, it means that the user is local.
LDAP Users:
LDAP, short for Lightweight Directory Access Protocol, is an open industry standard that uses internet protocols for accessing and maintaining distributed directory info services over an internet protocol network. Here are some ways to identify if a user is LDAP:
- Running LDAP specific commands:
You can use thegetent passwd [userId]
command to check if a user is found in the LDAP directory. This command propagates information from a variety of system databases including LDAP.
- /etc/nsswitch.conf File: This file dictates how the Name Service Switch facility inside the glibc library operates. If LDAP entries are found in this file under
passwd
,
shadow
and
group
sections, it indicates registration of LDAP users.
Feel free to tailor the methods mentioned according to your language preference. A well-maintained codebase with regularly audited access controls allows for swift navigation through lists of both local and LDAP users.
Further readings:
What’s the Name Service Switch?
IBM’s documentation on User Authentication and AuthorizationFor both professional coders and system administrators, distinguishing between a local user and an LDAP (Lightweight Directory Access Protocol) user is a crucial skill. This distinction pertains to the authentication process, wherein a local user’s credentials are stored locally within the system, while those of an LDAP user are not stored locally but rather in an external directory such as AD, OpenLDAP or another LDAP server.
So here are some telltale signs that could help you identify whether the user is local or LDAP:
User id or UID:
In most UNIX-based systems like Linux, local users have user ID or UID values that are less than 1000, generally speaking. On the contrary, LDAP users have UIDs greater than 1000. You can check this using the
id
command.
$ id -u username
/etc/passwd file checking:
This is one of the easiest methods to ascertain whether a user is local or LDAP. Local users always make an entry in the /etc/passwd file, however, LDAP users do not. Thus, just trying to fetch an entry for the said user through grep command would help.
$ grep username /etc/passwd
The output will be accessible if the queried user is local. For LDAP users, the output will be blank.
Reviewing system logs:
If your system uses something like SSSD or nslcd to connect to the LDAP server, then checking the logs of these service might deliver useful information about the user authentication source.
getent command:
The getent command retrieves entries from Name Service Switch libraries, which would include both local /etc/passwd users and LDAP users if your system is configured correctly.
$ getent passwd username
It’s important to note that the methods described above can vary based on the underlying platform and architecture. Hence, it’s recommended to refer to the specific documentation relevant to your environment.
Moreover, always remember, web-pages and portals that provide access to services often have different methods to distinguish between Local and LDAP users. In such cases, read the application/service documentation, or inspect the code if possible. This inspection is particularly applicable if you’re using platforms such as PHP with LDAP library or Spring LDAP.
Computer systems, such as Unix and Linux, use techniques to identify whether a user is a local user or an LDAP (Lightweight Directory Access Protocol) user. Here’s how it works:
To determine the type of a user, computer systems look into their user authentication process. Users are typically authenticated locally using files like
/etc/passwd
and
/etc/shadow
, or in a centralized way by consulting with some kind of directory service, such as LDAP.
Local User: Username -> /etc/passwd -> Valid? -> Log in LDAP User: Username -> LDAP Server -> Valid? -> Log in.
Now, let’s delve deeper into recognizing whether a user is a local one or an LDAP.
– Local users are directly authenticated by the system itself. Upon entering their username and password, the system checks these credentials against its own records. For systems like Unix or Linux, the system looks at the
/etc/passwd
and
/etc/shadow
files. If the entered credentials match with these records, the system recognises the user as local and authenticates them for login.
– LDAP users, on the other hand, are not authenticated by the system on which they’re logging in. In fact, an LDAP server does this task. It’s actually a centralised server that holds user information, stored securely within a directory, allowing network administrators to manage users’ credentials centrally rather than each computer system having to do so individually. When a user enters their credentials, the system sends those over the network to the LDAP server. The server then checks the credentials and responds back with the result. Depending on whether the server recognized them or not, they are either permitted or denied access.
Now, getting to know if a user is local or LDAP for troubleshooting purposes can be tricky but interestingly, many Unix and Linux based systems are equipped with tools you can utilize. For instance, commands like
getent passwd {username}
and
id {username}
can provide information about a user’s source. Running these commands gives out specific data about the specified user including their home directory, user ID, etc. You may recognize a user as an LDAP user if the latter command shows their group ID (`gid`) as coming from an LDAP domain.
A note to remember is that configurations surrounding user authentication methods, LDAP connections, etc. are typically housed in the
/etc/nsswitch.conf
file on Unix and Linux systems. Checking, understanding, and editing this file requires root or sudo access level, hence, should be approached carefully.
While explaining the mechanisms behind local and LDAP user recognition, it’s worth mentioning that a much larger topic revolves around system security, user management, network administration, and more aspects. I invite you to explore terms like PAM (Pluggable Authentication Modules), NSS (Name Service Switch), and NIS (Network Information Service) to gather a broader view of these topics.
For further reading, you might want to check out [How To Authenticate Client Computers Using LDAP] and [LDAP Authentication](https://wiki.archlinux.org/index.php/LDAP_authentication).Identifying the type of user, whether local or LDAP (Lightweight Directory Access Protocol), through a password storage mechanism was not designed to be straightforward due to security precautions. Password storage mechanisms, as configured within the system, don’t store plaintext versions of password for further evidence of local or LDAP users. Therefore, there isn’t any straight path to determine user type via passwords.
However, this recruitment of identification can be accompanied indirectly, through certain system configurations and design patterns. For instance, in Unix-like operating systems, which include Linux distributions, you will often find that local users have their hashed passwords stored in
/etc/shadow
whereas LDAP users are authenticated against an LDAP server, which stores their encrypted credentials.
For demonstration and better understanding, consider below the table that shows the data you likely encounter:
User Type | Password Storage Location |
---|---|
Local User |
/etc/shadow |
LDAP User | LDAP Server |
Determination of user type based on these details is neither foolproof nor recommended as a standard practice due to security and privacy concerns. However, for debugging or maintaining access controls, your best route might be to establish the source of authentication attempts – often visible in system logs, especially if you’ve set your systems to verbose logging.
Codes like
getpwnam()
could potentially facilitate your code to interact with the
/etc/passwd
and
/etc/shadow
files where local user information is stored. For example, The Linux Man Pages Project provides detailed information about how these function calls work.
#include <pwd.h>
#include <sys/types.h>
struct passwd * getpwnam(const char *name);
This function queries a given username against the local system only, and returns
NULL
if no match is found. Not finding a match here may suggest the user is authenticated through another service, possibly LDAP.
To confirm LDAP-user status, you would typically examine the LDAP server’s logs for matching authentication attempts using LDAP’s built-in tools, such as
ldapsearch
.
Bear firmly in mind that revealing user origin by password storage mechanism requires careful handling—this kind of disclosure can expose sensitive information. Be certain to always consider security best practices when handling user data and access controls.Understanding whether a user is a local system user or has been authenticated through Lightweight Directory Access Protocol (LDAP) is essential in managing user access rights and securing data. Fortunately, using Unix-based diagnostic tools like `getent` can assist in differentiating between the two.
To determine if a user account is local, you’ll want to look for their information in `/etc/passwd` file. An example command might be:
grep "username" /etc/passwd
– If the username exists in the output, then it’s a local user,
– If the username does not show in the output, the next step is to check against LDAP.
For examining LDAP users, we use the `getent` command combined with the term “passwd,” which gives us the list of all users, both local and from LDAP. From there, we search the output for our username:
getent passwd | grep "username"
– If the username is on this list, they’re an LDAP user.
– If not, the user either doesn’t exist or is stored under a different authentication configuration.
However, sometimes it’s beneficial to programmatically determine the origin of users. For these situations, consider writing a script to simply automate spotting the user entries:
#!/bin/bash if id -u "$username" >/dev/null 2>&1; then if getent passwd "$username" | grep -q '/bin/false'; then echo "User '$username' is an LDAP user." else echo "User '$username' is a local user." fi else echo "No such user '$username' exists on system." fi
This works because:
– It first uses `id -u` to determine if a user with the supplied username exists.
– If the user does exist, `getent` checks their shell setting.
– LDAP accounts typically have their shell set to /bin/false, according to convention.
Remember, only authorized individuals should handle User Management Tasks. Check your company’s policy before proceeding. Also, ensure that bash scripts have the appropriate permissions and are stored securely to protect sensitive data.
Lastly, while these commands are standard for Unix-like systems, note that they may vary slightly depending on the server’s specific version or distribution, so minor adjustments may be necessary. Beware of running any powerful command like `getent` without fully understanding its implications – misuse can lead to system instability or compromise.
For further information regarding user management in Unix and usage of the `getent` command, feel free to refer GNU C Library.
Directory services play an instrumental role in distinguishing between different user types such as Local and LDAP (Lightweight Directory Access Protocol). Before we dive into differentiation, let’s briefly understand what these terms mean.
A local user refers to the accounts that are directly created on a system. These users will exist and can log in only to the system where they were created. In contrast, an LDAP user is part of an LDAP service and can authenticate across multiple systems where LDAP authentication is enabled.
Identifying User Type: Local or LDAP
For identifying whether a user is Local or LDAP, we primarily depend on the information fetched from the directory service. Here are some key aspects:
-
User’s Distinguished Name (DN): By observing the DN information, you can ascertain whether a user is local or LDAP. A local user will have a DN within the local domain while an LDAP user will reflect the LDAP server’s distinguished name. It’s something like:
uid=username,ou=users,dc=companydomain,dc=com
Note: The ‘username’ here could be any username that’s been assigned to a user on the LDAP server. Also, ‘companydomain’ is the domain of the company where LDAP is managed.
-
Fallback Process: Another way of determining the user type is by utilizing a fallback process. Most platforms, when failing to do an LDAP bind with provided username/password, will fall back to the local user database, permitting a local user to login if it exists. This reveals whether a directory service identifies a user as local or from LDAP.
-
Third-party tools: Applications or packages, such as nsswitch for Linux or similar alternatives for other operating systems, provide the capability to determine the source of user data. If the switch returns the LDAP directory upon querying, this indicates the existence of an LDAP user.
The following piece of Python code can be utilised to verify if the user account is LDAP:
import ldap try: l = ldap.initialize("ldap://ldap.domain.com") l.protocol_version = ldap.VERSION3 l.simple_bind_s(BINDDN, PASSWORD) valid = l.search_s('dc=domain, dc=com', ldap.SCOPE_SUBTREE, 'uid={}'.format(u)) except ldap.INVALID_CREDENTIALS: valid = False
In this script, BINDDN stands for Bind Distinguished Name and PASSWORD is an LDAP password.
Remember that the precise way to identify a user relies heavily on the specific LDAP setup and configuration of your system.
For further understanding, you might find it beneficial to explore other resources. For example, OpenLDAP project’s website or respective system administration manuals are excellent sources to refer to.
Please note that maintaining both local and LDAP user settings requires careful planning and execution to prevent security loopholes. Make sure to align these procedures with the best practices of your organization when implementing solutions concerning these user accounts.
While working on a coding project, particularly one that incorporates differing user types such as Local or LDAP, it’s critical to be able to discern between them. Various profiling methods can help achieve this task. Here, we’ll delve into two main broad categories – server-based and client-based profiling.
Server-Based Profiling
In systems with a server or cloud implementation, the access method can provide clues about the user type. This approach hinges on using server logs for analysis. In languages like PHP, sessions can be used to differentiate users based on their access method.
For instance, our server may have been coded to assign different session variables for Local (let’s say
$_SESSION['user_type_local'] = true;
) and LDAP users (
$_SESSION['user_type_ldap'] = true;
). By checking these session parameters, we can easily tell which user type is currently logged in.
Client-Based Profiling
When working with an application that operates directly on end-user devices, other profiling techniques must be employed. JavaScript (JS) technologies are widely implemented to handle these tasks. With JS frameworks, variable assignment based on user input can assist in distinguishing the user type.
Consider a signup page that requires choosing “Local” or “LDAP” during registration. These selections could trigger an action to set a global variable like
var userType
.
However, it’s worth mentioning that JavaScript manipulations should never be relied upon solely for security or profiling procedures as these variables can be manipulated by anyone who knows how to open the developer tools on their browsers.
User Determination in Context of LDAP and Local Users
If our discussion needs to be confined to telling apart Local from LDAP users, things get more specific. Both Classes of Service (CoS) and Role-Based Access Control (RBAC) use mission-critical Lightweight Directory Access Protocol (LDAP) directory servers[1].
Here’s how you might check if a user belongs to LDAP:
import ldap l = ldap.initialize("ldaps://your-ldap-server") try: l.protocol_version = ldap.VERSION3 l.set_option(ldap.OPT_REFERRALS, 0) bind = l.simple_bind_s("your-app-user","your-app-password") base = "OU=Users,DC=YourDomain,DC=com" criteria = "(&(objectClass=user)(sAMAccountName=your-test-user))" attributes = ['dn', 'cn', 'givenName', 'sn'] result = l.search_s(base, ldap.SCOPE_SUBTREE, criteria, attributes) results = [entry for dn, entry in result if isinstance(entry, dict)] print(results) finally: l.unbind()
The above Python code connects to your LDAP server and searches for a particular user (denoted by ‘your-test-user’). If the user is found, that validates they are an LDAP user.
Another direct way is to probe your system’s internal databases, tables or directories to determine if said user is local. A similar command or script to fetch all local users of a Unix system might look like implementing
cut -d: -f1 /etc/passwd
, and then searching through the returned user list. The mentioned command lists all local users in Unix-like operating systems.
These examples hinge on active probing and require access to the respective server resources, and so would only be feasible on a need-to-know basis due to potential security implications. Be sure to always follow best practice in relation to data privacy laws and the security policies in effect where your code will run.
Do bear in mind that this level of identification would generally be performed at authentication-level instead of doing this manually every time, ideally speaking. Your authentication server should know what type of user just logged in — whether local or LDAP. It’s crucial to carefully structure your initial architecture to allow such categorizations subsequent to user login.
References:
Oracle-LDAP
LDAP vs. Local Authentication: Understanding How They Work
Authentication is the process of proving an individual or entity’s identity. The two widely-known methodologies for this process are LDAP (Lightweight Directory Access Protocol) and Local authentication. Let’s delve deeper into these concepts to understand their workings and distinguish a local user from an LDAP user.
Local Authentication
With Local Authentication, user credentials (username and password) are stored directly on the system where the authentication is being performed. This system can range from your personal computer to a server in an organization.
In Linux, for instance, a user’s credentials are stored locally in two separate files:
1. /etc/passwd – stores information about each user account, such as user ID (UID), user name, home directory, shell assignment etc.
2. /etc/shadow – holds actual encrypted passwords.
Whenever a routine authentication takes place, let’s say during login, the entered credentials are checked against the entries in these files to validate user identity.
You can recognize a local user by utilizing the `grep` command. Using the terminal, you just need to input (replace ‘username’ with the username you want to verify):
grep 'username' /etc/passwd
If the user exists, it would return a line containing user details.
LDAP Authentication
LDAP Authentication works slightly differently. In LDAP, user credentials are saved in a centralized LDAP server. An advantage over local authentication is that users’ credentials do not need to be stored on every machine individually, but only on the centralized server. This can simplify security management in an environment with numerous computer systems.
To authenticate a user using LDAP, the system connects to the LDAP directory through distinct protocol standards. Then it compares the presented credentials with the ones saved in the directory. If they match, the authentication is successful.
Often, you’d come across the term “bind” in LDAP context. It’s the method the directory uses to authenticate clients (applications, users, servers).
Identifying if a user is LDAP based could be achieved by querying the LDAP server itself with utilities like ldapsearch. An ldapsearch command example to find a user (‘username’) might look like this:
ldapsearch -x -H ldap://ldsdc.example.com -b "dc=example,dc=com" "(uid=username)"
If the output returns user details, it indicates the user is authenticated through LDAP.
Remember- both methods have their unique benefits and drawbacks, hence should be employed per the requirement and setup convenience. Furthermore, identifying whether a user is local or LDAP-enabled can greatly aid in managing and troubleshooting user accounts efficiently.Indeed, the configuration of your environment has a big impact on how you can recognize users. Depending upon how the environment is set up, it may be quite straightforward to determine if a user is local or LDAP (Lightweight Directory Access Protocol).
## Local vs LDAP User Recognition
In a web development context, ‘local’ users typically refer to those who are authenticated directly against the system’s user database. Conversely, LDAP users are authenticated through an external directory or server.
Just before diving into code snippets to establish ways to tell if a user is local or LDAP, let’s briefly run through some rudimentary pointers which can guide us:
- Storage Differences: The primary storage for local users is usually the server’s file system, and they are often managed using server terminal tools. For LDAP users, they are contained in the directory server and usually managed via tools that can interact with LDAP.
- Password Authentication: While authenticating, local users’ passwords are usually checked directly against the hashed password stored on the server. LDAP users’ authentication is handled by the LDAP server.
To differentiate between a local and an LDAP user within a coding environment, we’d do something similar in principle, but programmatically.
## Using Code To Determine User Type
Mostly, this distinction is accomplished by making calls to the back-end servers or databases where these users’ data are kept.
Consider that your application runs in Python. Here’s an illustration:
import pwd try: pwd.getpwnam('username') print("User is local") except KeyError: print("User is not local")
In this case, the application attempts to access the username from the local machine’s password database. If it’s successful, then the user is local. If an ‘exception’ is thrown (i.e., there’s a
KeyError
when trying to get that user), it signifies that user does not exist locally.
For an LDAP user, you would connect to the LDAP server and then attempt to search for the user:
import ldap l = ldap.initialize("ldap://your-ldap-server") try: l.simple_bind_s("cn=admin,dc=example,dc=com", "password") r = l.search_s('ou=users,dc=example,dc=com', ldap.SCOPE_SUBTREE, "(uid=username)") if r: print("User is LDAP") except ldap.LDAPError as e: print(e)
In this example, the script again tries to fetch the user but this time from the LDAP server. If there is a result returned from the search, the user is determined to be an LDAP user. If there is an LDAP error instead, it indicates the user could not be found in the LDAP server – at least not given the attempted search parameters.
Remember, these examples are simplified and you will need to adapt them to the needs of your specific environment.
The information I’ve presented here should give you a basic understanding of how to distinguish between local and LDAP users using Python. There are other programming languages like PHP, Java etc.(source) where similar approaches can be used although implementation might slightly vary.
So, depending upon your environment setup, check your existing codebase for clues on how you are connecting to your user database(s) and how your app is handling authentication and storing authentication status/session information.Identifying the type of a user, whether they’re using Local or LDAP (Lightweight Directory Access Protocol), can be effectively found by reading debug log files. Log files essentially track the actions and events of your software applications which includes the authentication process.
In the context of debugging, they are incredibly useful in uncovering and identifying where, when, and how an issue may have occurred. These insights can further assist the developers in troubleshooting and fixing the issue.
When the authentication takes place, i.e., when a user logs into a system, an entry is made in the log file tracking this event. The entry will specify whether the user authentication was local or via LDAP, thereby enabling you to identify the user type.
A standard log entry snippet might look like:
2019-01-01 01:01:01 INFO [AuthController] User johndoe AUTHENTICATED via LOCAL 2019-01-01 02:02:02 INFO [AuthController] User janedoe AUTHENTICATED via LDAP
Here `johndoe` and `janedoe` are usernames, and `LOCAL` and `LDAP` represent their authentication type.
Now, how do we read these log files? You can utilize built-in search functions on your operating system such as grep on Linux or finding .log files on Windows using File Explorer. However, for more complex and consistent log analysis I would recommend using centralized log management tools that facilitate easy searching, filtering, alerting upon certain conditions and visualizing data.
Note: Not all systems make it so clear the authenticated type of the user, sometimes you must determine based on flow of actions which leads to successful authentication.
Please be sure to comply with your security policies, GDPR, and other regulatory practices while working with personal identifying information in log files.
For more information on log management, consider looking at these resources:
Log Management – Ultimate GuideBefore we delve deeper into the answer, let’s establish a fundamental understanding regarding local users and LDAP (Lightweight Directory Access Protocol) users.
A local user refers to a user account that is stored directly on the system you’re using, mostly in the form of hashed passwords in the `/etc/password` or `/etc/shadow` directory. On the other hand, an LDAP user refers to a user that is authenticated through an LDAP server. It means that it does not depend on the system you’re using rather it depends on network access to that server. These concepts bring us to the meaty topic of examination of permissions and access control for identification.
You can check whether a user is local or LDAP by incorporating certain command lines such as
getent
, which are utilities in Unix-like operating systems like Linux or macOS.
To see if your user is local:
$ getent passwd | grep username
If you don’t receive any output, the user probably isn’t localized. However, if you get feedback, then the user is local.
Now, for LDAP users, you can employ a similar tactic. Keeping in mind that these individuals are recognized via your system’s nsswitch.conf, configured to look for passwd entries coming from ldap.
$ getent passwd | grep username
Unless your LDAP setting is correctly placed within
nsswitch.conf
, you’ll find no result here. If you do receive output, this indicates that your “username” could be a local user or someone taken from the LDAP.
To confirm, you can cross-verify by trying to grab password entries strictly from LDAP:
$ getent -s ldap passwd | grep username
Remember, results here indicate that your ‘username’ is an LDAP user. No result? Well, they might still be local. To reconfirm, you can also examine the
/etc/passwd
file.
Moreover, we should note the discrepancy between local accounts and LDAP. Local accounts have their account info saved on each machine they’ve created; at the same time, LDAP accounts only require them to store their info on the LDAP server, permitting access to every computer within the LDAP domain.
These commands give an important insight into the nature of the user – whether it’s local or LDAP. This decision further braces up decisions pertaining to permissions and access control strategies. For instance, local users might have more comprehensive permissions on the system than LDAP users who might be provided with more controlled and limited access.
Checking the origin of a user can also improve the system’s overall security. Access to local users can be limited to essential personnel while non-essential staff can be restricted to LDAP accounts which draw customary rules from the central directory rather than higher-access local accounts.
By segregating roles based on whether users are local or LDAP, not only you bolster your system’s security setup but also personalize user experience in a workflow-efficient manner – making this identity check a fruitful endeavor.
In light of all, it becomes evident that identification confirmation of whether a user is local or LDAP molds the trajectory of your permission and access control deliberations, enabling you to formulate meticulous system strategy.As a coder, unraveling the identity of a user – discerning whether they’re local or LDAP (Lightweight Directory Access Protocol) – is often a cornerstone task in managing authorizations and privileges. Your course of action primarily depends on the system you employ to manage your users, but here are the basics for most common systems.
Highlighting the key differences: local users are usually created directly on the system and their credentials specific to that system alone. Conversely, LDAP users are part of an external server’s database – like Microsoft’s Active Directory or OpenLDAP on Linux – and can authenticate across multiple systems.
Checking If A User Is Local
The process of identification varies according to the operating system in use.
For Windows, one can sift through the list of local users in the Local Users and Groups Manager accessible via Computer Management. Also, running the
net user
command in Command Prompt fetches the same results.
On Unix/Linux systems, you can see if the user appears in the file where local accounts are stored such as /etc/passwd using the
grep
utility:
grep username /etc/passwd
If there’s a match, the user is local. Other commands like
getent passwd username
and
id username
can also provide useful information.
Validating An LDAP User
Any users not found to be local can be assumed to be LDAP users. To validate this assumption (or to check directly), you can use LDAP-specific commands:
getent passwd
or
id username
These commands should return details about the user if valid in the LDAP directory. Alternatively, you can leverage LDAP tools such as ldapsearch.
To recap, bear in mind that the steps above are tailored to suit generic use-cases. It essential to tweak them to fit into your context. Also, depending on the system, other user directories like NIS could be involved. Ensuring optimal system security aligns with understanding the provenance of your users.
Do remember, the goal is never to lose track of the possibility of interacting systems authenticating LDAP users locally. Unraveling the true identities of users requires a combination of keen scrutiny, brilliance, and sometimes just knowing where to look.
IBM Documentation has further literature on LDAP user authentication that may prove helpful.
Summarizing, determining whether a user is LDAP or Local is pivotal in regulating access and permissions beyond the purview of manual oversight. The method differs based on the system infrastructure but can be narrowed down by correct identification of whether the user is local or present in an LDAP directory. Remember, a unique user name doesn’t always translate to a unique user identity. Awareness towards this nuanced difference helps curb unauthorized access and fortifies system integrity. Through meticulous monitoring and implementation of these steps, ensuring a secure, optimized workspace isn’t far from reality.