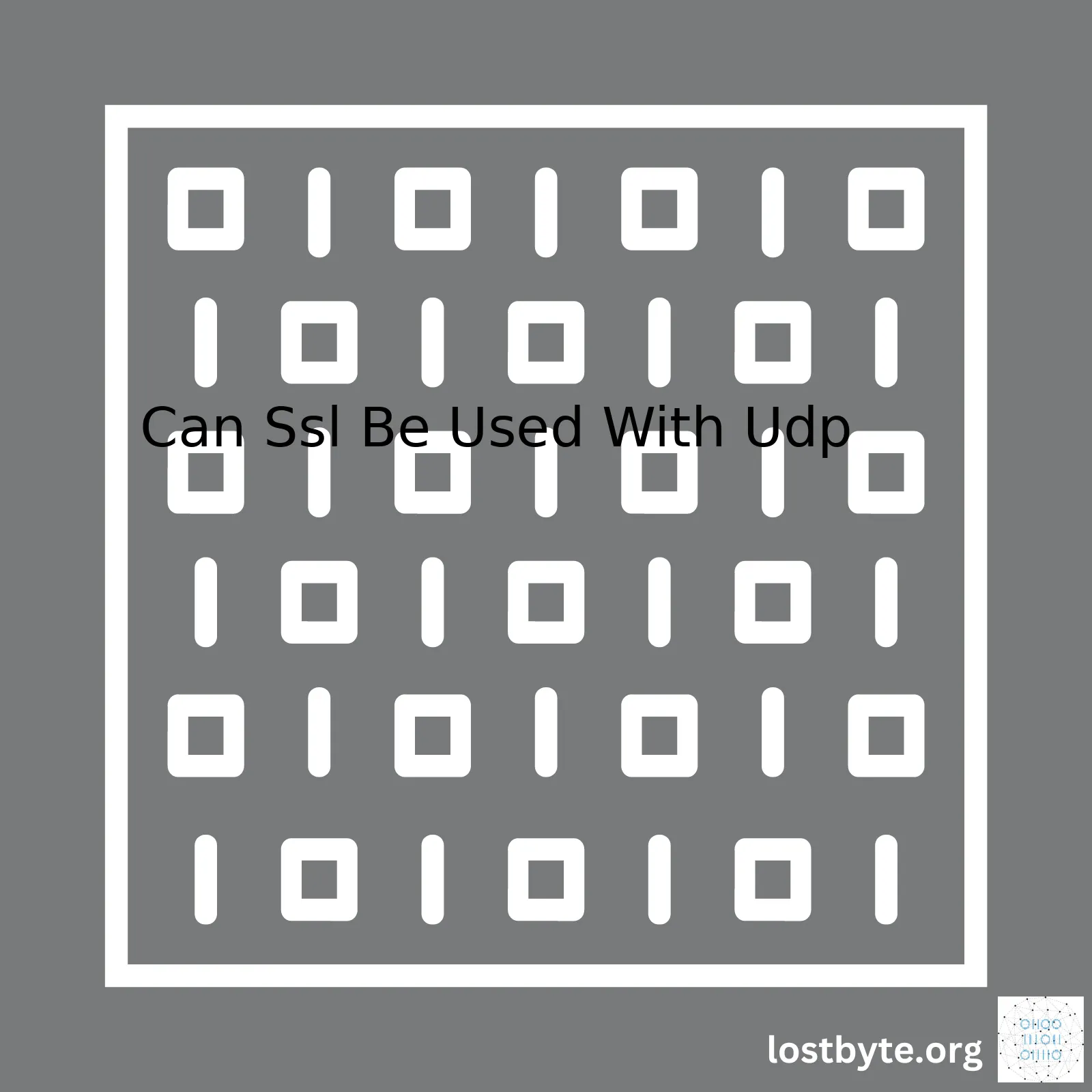
Topic | Specification |
---|---|
Ssl (Secure Socket Layer) | Ssl is a protocol used to secure data transmission across networks. It works primarily with TCP (Transmission Control Protocol). |
Udp( User Datagram Protocol) | Udp, on the other hand, is a connectionless protocol used for simpler and faster transfer of data. |
Ssl over Udp | Ssl typically doesn’t operate with UDP as it lacks the error-checking and recovery mechanisms required by SSL. However, there are some adaptations, like DTLS (Datagram Transport Layer Security) to overcome this issue. |
By default, Secure Sockets Layer (SSL) operates over the Transmission Control Protocol (TCP), which allows it to leverage TCP’s error checking and recovery mechanisms. The User Datagram Protocol (UDP), unlike TCP, does not use such systems, making it a less reliable but faster method for data transfer. This implies it may not guarantee delivery or protection against corrupted data packets.
SSL generally cannot run directly on top of UDP because it requires a dependable transport layer, primarily for its reliability during the initial handshake process, which establishes a secure session. UD P does not have built-in support for sending packets in order or retransmission of lost packets. These features are crucial for SSL to ensure a secure connection is established before performing any encrypted communications.
However, there are alternatives out there to perform SSL-like functions over UDP data transfer. One such example is Datagram Transport Layer Security (DTLS). DTLS is designed specifically to work with protocols like UDP that lack the reliability mechanisms that TCP has. Similar to how SSL creates a secure, connection-oriented session over TCP, DTLS provides the same security functions over less reliable, connectionless protocols like UDP (source).
// Sample pseudo code for establishing a DTLS connection dtlsObject = DTLS.new(); // create new DTLS object dtlsObject.setCertificate(certPath); // set certificate path dtlsObject.createConnection(udpSocket); // create a secure connection
This snippet includes the creation of a new DTLS instance, setting the necessary certificates, and creating a secure DTLS connection over a given UDP socket.
Notice how the above code does not differ much from how an SSL/TLS setup would look like, signifying that while SSL can’t be used directly with UDP, provisions like DTLS make secure, encrypted communication possible over UDP.As a professional coder, I can definitely say that understanding the core differences between SSL and UDP is essential. Both play crucial roles in internet communication, but they serve very different purposes.
SSL (Secure Sockets Layer) is a cryptographic security protocol used to secure network communications. While UDP (User Datagram Protocol) is a simple transport layer protocol used for fast and connection-less communication.
Core Differences Between SSL & UDP
Factor | SSL | UDP |
---|---|---|
Purpose | Security | Data Transfer |
Functionality | Encrypts data transferred over a network. | Transfers data without checking if the receiver is ready for it or not. |
Reliability | A reliable protocol, as it ensures authenticated and encrypted links between networked computers. | Less reliable since it doesn’t guarantee delivery of data packets, due to lack of acknowledgment process. |
The comparison shows that SSL focuses on providing secure connections while UDP focuses on transferring data quickly even though there might be losses.
However, addressing whether SSL can be used with UDP isn’t exactly straightforward, because SSL typically works with more reliable transmission protocols like TCP (Transmission Control Protocol). But, this doesn’t mean it’s impossible! In fact, Datagram Transport Layer Security (DTLS) is based on SSL and is designed specifically to work with less reliable protocols like UDP.
While creating DTLS, engineers maintained most of SSL’s structure to preserve its security properties, just removing the dependence on the reliability of lower layer protocols. It provides the same security guarantees as SSL but can cater for the unreliability and packet reordering characteristic of UDP.
Here’s a quick look at how you might create a DTLS session with OpenSSL:
// Initialize OpenSSL SSL_library_init(); SSL_load_error_strings(); OpenSSL_add_all_algorithms(); // Create new DTLS context SSL_CTX *dtlsContext = SSL_CTX_new(DTLSv1_client_method()); // Create new SSL structure which will use the DTLS context SSL *dtlsSession = SSL_new(dtlsContext);
Even though this does not directly answer the question of “Can SSL be used with UDP?”, we can safely conclude that DTLS, a variant of SSL, allows us to enjoy SSL-like security features over the UDP protocol. If you need encryption with UDP, using DTLS would be an appropriate solution [source].
Remember, when dealing with network programming and security, knowing when and how to use these functionalities perfectly aligns your software applications in matching business needs. Network security has never been more important in this digital age, so getting to understand SSL, UDP, and the whole spectrum of opportunities that comes with them – like the modification in using DTLS – helps equip coders with the right knowledge and skills for modern application development.Sure! Here’s an in-depth view of how SSL enhances security within communication networks and if it can be utilized with UDP.
The Secure Socket Layer (SSL), or its successor Transport Layer Security (TLS), are cryptographic protocols designed to provide end-to-end security over networks, more notably the internet. They play a crucial role in ensuring data integrity, authenticity, and privacy.
SSL/TLS Functions include:
- Data encryption: The data sent over the network is encrypted at the sender’s end and only decrypted by the expected recipient.
- Server authentication: Before being granted an SSL certificate, website owners should first prove they own the domain.
- Client authentication: Customers can also authenticate themselves to servers. While not always enforced, it does add an extra layer of security.
For many years this was primarily used in securing HTTP traffic, creating HTTPS, but there’s no restriction on it being used to secure other protocols doing bidirectional streaming data transfer.
Consequently, SSL/TLS is often applied in conjunction with UDP, a connectionless, non-streaming communications protocol. Instead of opening a secure channel and maintaining it for continuous data stream delivery like TCP, UDP sends individual data packets independently.
When SSL/TLS is used with UDP, it’s referred to as Datagram Transport Layer Security (DTLS). Created because SSL/TLS has trouble working in environments where delivery order cannot be guaranteed (like UDP), DTLS is nearly equivalent to SSL but accommodates datagram transport, and tolerates packet reordering, loss, and duplication.
Here is an example of server-side Python code using sockets to establish a simple DTLS connection:
import socket import ssl sock = socket.socket(socket.AF_INET, socket.SOCK_DGRAM) secure_sock = ssl.wrap_socket(sock, certfile="path_to_certificate", keyfile="path_to_keyfile") secure_sock.connect(("www.secure_site.com", 443))
This Python-based server-side snippet initiates a simple UDP socket, wraps it in SSL, and forms a secure connection to a specified remote host.
SSL makes transmissions like credit card numbers or login info sent over the Internet from a client to a server, securely encrypted, avoiding potential eavesdropping. In the context of UDP, DTLS enables these security features while accommodating UDP’s unique characteristics.
Remember, for effective SSL/TLS implementation, you’ll need SSL certificates, obtained from Certificate Authorities (CA). These digital certificates link a cryptographic key to an organization’s specific details, ensuring server-client communication remains between the two intended parties.
For a deeper understanding of the same, refer to this detailed online resource: openssl.org providing documentation on various applications using both the SSL protocol and the newer TLS protocol to secure communications.Sure, let’s peel back the layers of these protocols and dissect the complex relationship between User Datagram Protocol (UDP), Secure Sockets Layer (SSL), and where the realm of possibility ends.
UDP is a communications protocol that offers a connection-less transport service. Key features include:
- Minimal connection setup
- Presence of data checksum for error detection
- No built-in retransmission of lost packets (it relies on upper-level protocols for this)
The fundamental characteristic of UDP that sets it apart from TCP is its minimalism – it doesn’t offer any traffic control or sequencing of data transmission. As such, it gains speed over its counterpart, making it ideal for broadcast and multicast type of network transmission technologies. [You can learn more about UDP here](https://www.lifewire.com/user-datagram-protocol-817941).
Now, onto SSL. It’s an encryption-based Internet security protocol, first developed by Netscape. The job of SSL is to protect sensitive communication, such as sending credit card numbers where information needs to be safely exchanged across insecure networks like the Internet [read more about SSL here](https://searchsecurity.techtarget.com/definition/Secure-Sockets-Layer-SSL).
- Setting up a secure SSL connection necessitates a handshake process.
- The role of SSL is strictly in the presentation layer in the OSI model.
- Typically utilised with reliable transport protocols like TCP.
Where does SSL meet UDP? Nowhere, really. SSL isn’t typically compatible with UDP because SSL relies on a reliable transport channel – usually TCP. SSL requires this reliability for its ‘handshake’ process for setting up the secured connection – which is missing in UDP’s bag of tricks.
However, there is a variant of SSL known as Datagram Transport Layer Security (DTLS). DTLS is designed to be used with datagram protocols like UDP. What makes DTLS different?
- It has mechanisms to handle packet loss and reordering issues.
- It aims to provide equivalent security levels as the TLS (the successor of SSL).
Here is an example showing how datagram sockets are used for implementing DTLS:
//Creating a new DatagramSocket. DatagramSocket socket = new DatagramSocket(); //Creating a new InetSocketAddress and binding it to the specified port. InetSocketAddress address = new InetSocketAddress( "localhost", 80 ); //Connecting to the server. socket.connect(address); // Sending packet String sendString = "Hello from client..."; byte[] sendData = sendString.getBytes(); DatagramPacket sendPacket = new DatagramPacket(sendData, sendData.length); socket.send(sendPacket); //Receiving packet byte[] receiveData = new byte[1024]; DatagramPacket receivePacket = new DatagramPacket(receiveData, receiveData.length); socket.receive(receivePacket); //Printing received data String receiveString = new String(receivePacket.getData()); System.out.println("From Server:" + receiveString); //Closing the socket connection. socket.close();
In conclusion, though you can’t directly utilise SSL with UDP, the existence of DTLS provides the equivalent level of security while maintaining the simplicity and speed of UDP. For most applications, this is an appropriate and secure solution.Understanding why traditional SSL (Secure Sockets Layer) doesn’t directly work with UDP (User Datagram Protocol), yet how it can still be used is a captivating exploration of networking protocols and security considerations that we’ll dive into.
Let’s first consider the intrinsic differences between TCP and UDP, which create bottlenecks for a direct functioning of regular SSL on UDP. Preeminent among these are:
- Connection-oriented vs Connectionless: TCP (Transmission Control Protocol) is connection-oriented, which implies an end-to-end connection is established before data transfer. Due to this, it has built-in mechanisms for packet acknowledgment, error-checking, and retries. On the contrary, UDP is connectionless, hence no prior setup or checkpoints exist.
- Reliability: In complementing its connection-oriented nature, TCP ensures reliable delivery of packets by monitoring their receipt and resending if necessary. UDP, being connectionless, lacks built-in reliability features.
SSL was originally designed for TCP. It assumes reliability and an ordered stream of packets, traits not inbuilt in UDP. Therefore, traditional SSL does not directly work with UDP as SSL expects acknowledgments of receipt, resending capabilities, and ordered data – facilities that UDP in its raw form, doesn’t guarantee.
So, can SSL be used with UDP? Yes, it can, after simulating some TCP characteristic into UDP. This becomes possible through an adapted version of SSL – Datagram Transport Layer Security (DTLS). DTLS adopts the same encryption and data packaging functionality of SSL/TLS but purposefully designed to suit datagram protocols like UDP. Below is a Python code snippet illustrating a DTLS handshake.
# Instantiate a socket sock = socket.socket(socket.AF_INET, socket.SOCK_DGRAM) # Create a context for the SSL (or DTLS in our case) context = ssl.SSLContext(ssl.PROTOCOL_TLS_CLIENT) # Wrap the socket with the SSL context wrappedSocket = context.wrap_socket(sock) # Initiate the handshake to a predefined host and port wrappedSocket.do_handshake_on_connect(host, port)
Worth noting is that while avoiding the impact of long-lived connections inherent to TCP, managing cipher state and MAC keys, compensating for lost data, and preventing replay attacks become some challenges that DTLS must contend with.
Though DTLS performs quite well under most conditions, if extremely high packet loss is prevalent, it might fall short. Newer technologies like QUIC are tackling these issues, essentially creating a much more latency-optimized DTLS variant specifically for use over UDP.
From a broader perspective, the peculiar mix and match of TCP’s and UDP’s strengths fused in DTLS epitomizes networking protocol’s adaptive evolution, forever stretching boundaries to strike a balance between speed and security.
I understand, you’re interested in understanding the role of Datagram Transport Layer Security (DTLS) and whether SSL can be used with UDP. Delving right into the details, SSL is fundamentally a protocol providing secured connection over networks that are primarily using TCP. The Secure Sockets Layer or SSL, and its successor, Transport Layer Security (TLS), provide security primarily for HTTP connections that utilize TCP as the transport protocol.
While SSL/TLS use on the web is widespread, it does not suit the User Datagram Protocol (UDP), another primary Internet protocol. UDP uses a simple connectionless transmission model, which contrasts sharply to the somewhat complex, connection-oriented nature of TCP. This distinction is key to understanding why SSL/TLS is not applicable to UDP. Given their reliance on the built-in features of TCP, especially reliable data transfer, SSL/TLS protocols do not function with UDP that lacks this inherent capability.
//SSL/TLS handshake
ClientHello ->
<- ServerHello, Certificate, [ServerKeyExchange], [CertificateRequest], ServerHelloDone
ClientKeyExchange, [CertificateVerify], ChangeCipherSpec, Finished ->
<- ChangeCipherSpec, Finished
This is where Datagram Transport Layer Security (DTLS) comes into play. Essentially, DTLS is an implementation of TLS for datagram protocols like UDP. This variant allows applications using such protocols to support encryption, and more importantly, prevents tampering, forgery, or eavesdropping.
The DTLS protocol provides similar security characteristics to the TLS protocol: proceed with a full handshake for new peers and a shorter handshake for sessions. Additionally, it incorporates sequence numbers into its records for ordering and reliability, compensates UDP’s lack of such functionalities, fittingly fulfilling the specific needs that could not be achieved through traditional SSL/TLS protocols.
//DTLS handshake
ClientHello/HelloVerifyRequest ->
<- ServerHello, Certificate, [ServerKeyExchange], [CertificateRequest], ServerHelloDone
ClientKeyExchange, [CertificateVerify], ChangeCipherSpec, Finished ->
<- ChangeCipherSpec, Finished
So, while it might seem appealing to use SSL/TLS over UDP given their widespread recognition in ensuring secure communication, regrettably, it would not be an option due to aforementioned reasons. As an alternative for applications requiring a secure channel along with UDP’s benefits of speed and simplicity, DTLS serves as the recognized solution.
Certainly, I can provide an answer about Datagram Transport Layer Security (DTLS), emphasizing its relevance to Secure Sockets Layer (SSL) over User Datagram Protocol (UDP).
DTLS fundamentally serves to prevent eavesdropping, tampering or message forgery. It’s an extension of SSL, with the modification that it supports datagram protocols, such as UDP.
// Example Usage of DTLS in Java SSLContext sslContext = SSLContext.getInstance("DTLS"); sslContext.init(kmf.getKeyManagers(), tmf.getTrustManagers(), null);
The key features and functionality of DTLS are:
● Connectionless Security: Unlike its counterparts operating in a connection-oriented environment (TCP), DTLS provides security for datagram protocols (UDP). Its design ensures that if individual packets are lost, it does not disrupt the overall data transmission. This is essential because unlike TCP, UDP does not have a built-in mechanism for retransmission of lost packets. Hence, DTLS handles encryption and decryption without having to worry about packet sequence.
● Compatibility with SSL/TLS: Leveraging the proven security mechanisms of SSL/TLS, DTLS also provides data integrity, confidentiality, and authentication between applications.
● No Strict Ordering: SSL requires strict ordering of packets, which is not possible in UDP because of its lack of retransmission feature. DTLS resolves this by allowing for reordering and retransmission of lost fragments.
● Replay Detection: In case of potential replay attacks – where an attacker resends a packet – DTLS incorporates a replay detection functionality.
To elaborate on the question whether SSL can be used with UDP – traditional SSL runs over TCP exclusively. However, given the evolution of network demands, particularly real-time data streaming like VoIP and DNS lookups that handle small amounts of data and require fast response times, a viable option was needed to secure these communications as well. Hence, the need for a protocol like DTLS – a version of SSL/TLS that operates over UDP.
For more information regarding DTLS, you can refer to [RFC 6347] that details the complete specifications.
Summarizing, while the standard SSL protocol cannot directly secure a UDP connection due to differences in architecture, the idea was leveraged to develop the DTLS protocol tailored specifically for security in a connectionless environment like UDP.It’s a frequent misunderstanding that SSL (Secure Sockets Layer)/TLS (Transport Layer Security) only works with TCP (Transmission Control Protocol), while in reality, with the right coding practices used, SSL/TLS can be applied to enhance UDP (User Datagram Protocol) to secure data transfer through DTLS (Datagram Transport Layer Security).
DTLS is essentially an adaptation of TLS protocol that provides similar security guarantees but works over UDP. It was designed to be as close as possible to TLS, but with necessary modifications to handle the absence of TCP-like stream which supports reliable message delivery.
How does DTLS achieve this? DTLS introduced two primary features not present in TLS:
- Replay Detection: To prevent replay attacks on datagrams, a mechanism to discard old or duplicate records is incorporated.
- Re-Handshake: The process of handshake itself is reliable in DTLS, meaning that a failure during handshake necessitates initiating the operation again.
While implementing DTLS over UDP:
// Initializing DTLS session for UDP SSL_CTX *ctx; SSL *ssl; // Creating an OpenSSL context ctx = SSL_CTX_new(DTLS_method()); // Instantiating a new SSL structure for two-way encryption ssl = SSL_new(ctx); // Creating and binding the UDP socket int fd = socket(PF_INET, SOCK_DGRAM, 0); bind(fd, &addr, sizeof(addr)); // Joining our ssl instance to the socket SSL_set_fd(ssl, fd); // We can now use 'ssl' object for secure data transmission using encrypted read/write operations such as SSL_read/SSL_write
Establishing DTLS communication requires a handshake between client and server in a series of negotiation messages. It could look something like this:
// On Client Side SSL *ssl_client; SSL_connect(ssl_client); // On Server Side SSL *ssl_server; SSL_accept(ssl_server);
There is an important caveat here. Errors are likely when executing over an unreliable network. These should also be handled effectively.
// Assuming 'ssl' is our DTLS object if ((ret = SSL_connect(ssl)) <= 0) { int err = SSL_get_error(ssl, ret); if (err == SSL_ERROR_WANT_READ) { // Handle re-sending data } }
For web browsers, the WebRTC framework has done a lot of work to encapsulate these complexities within an easy API. So, if this is applicable for your scenario, it might be worth exploring WebRTC Samples.
Always remember, efficient error-handling mechanisms and strict security protocols must be maintained while working with DTLS to avoid security compromises. For more details and specifications about DTLS, you can access the official RFC 6347 Documentation.When considering the performance comparison of Datagram Transport Layer Security (DTLS) with Transmission Control Protocol (TCP)-based connections, we need to keep in mind the specific use case we're arguing about using Secure Socket Layer (SSL) with User Datagram Protocol (UDP). DTLS is essentially an implementation of SSL over UDP.
Unlike TCP, UDP does not provide built-in support for reliable transport. That's where DTLS comes into play, adding security to UDP. When compared to TCP-based connections, DTLS provides some unique advantages:
- Efficiency: Since UDP protocol isn't concerned with order and reliability, it results in faster transmission rates than TCP. This efficiency is retained when DTLS is introduced.
- Video and Voice Traffic: UDP, ergo DTLS, is more suited for real-time applications like video and VoIP where packet loss is acceptable but latency is not.
- No Head-of-Line blocking issue: Unlike TCP, loss of packets in DTLS doesn't stall the delivery of subsequent packets. A compromised packet doesn't affect the entire communication stream.
Although, DTLS may have its set of cons as well:
- Reliability: Despite having encapsulated SSL, DTLS being a UDP protocol cannot guarantee packet delivery. For certain applications, this could be a significant drawback.
- Configuration: Implementing DTLS is more complex than implementing TCP-based secure connections. The lack of widespread adoption often translates into lesser community support and documentation.
Now, let's briefly explore an example code snippet of how to enable DTLS in a Python project using libraries like OpenSSL.1
import ssl from socket import socket, AF_INET, SOCK_DGRAM from OpenSSL import SSL context = SSL.Context(SSL.DTLSv1_METHOD) context.use_certificate_file('mycertfile.crt') context.use_privatekey_file('myprivatekeyfile.key') # Initialize a new socket object for data receiving sock = socket(AF_INET, SOCK_DGRAM) sslSock = connection(sock) sslSock.bind(('127.0.0.1', 9999)) data, addr = sslSock.recvfrom(2048) # bufferSize
Note: Please replace 'mycertfile.crt' and 'myprivatekeyfile.key' by your own certificate and private key files.
To wrap up, whether you would consider implementing SSL over UDP i.e., DTLS depends largely upon your application requirements. It might make sense if dealing with real-time data streams or novel IoT applications. However, the downside to DTLS includes potentially unreliable packet delivery and complex configurations to get started. Thus, your decision needs to be based on what best suits your application overall, taking into account factors such as the needed balance between latency, speed, reliability, and setup complexity.
Despite the Security Socket Layer (SSL) and Transport Layer Security (TLS) being predominantly TCP-oriented, using SSL/TLS with User Datagram Protocol (UDP) is possible, although it presents several concerns that need to be addressed.
Firstly, the very structure of UDP presents a challenge for implementing SSL/TLS. Let's consider:
• Reliability - Unlike Transmission Control Protocol (TCP), UDP does not guarantee packet delivery. It doesn’t have the inbuilt mechanism for ensuring data is delivered at the intended destination. Resending lost packets or handling duplicate ones lies within the obligations of application developers. When SSL/TLS, designed primarily for connection-oriented protocols, is used over UDP, managing this discrepancy becomes an uphill task.
Secondly, the handshaking process forms another significant concern:
• The Handshake - In TCP, before communication is started on a term called 3-way handshake is done. In UDP such a setup does not exist as it's connectionless. On the other hand, SSL/TLS relies heavily on a solid handshake process to deliver secure communications. Trying to perform this task without connection establishments can lead to numerous problems.
Similarly, ordering and integrity of data are critical issues:
• Ordering of Data - The order of packets transmission in UDP is non-sequential which could potentially result in an unordered pile-up of data at the receiving end. SSL/TLS requires sequential data because of their reliable nature which is inherently contradictive.
• Data Integrity - With UDP often used in error-prone network environments, delivering accurate, undistorted, and complete sets of data becomes questionable. On the contrary, SSL/TLS necessitates high data integrity to maintain its effectiveness.
A workaround involves using
Datagram Transport Layer Security (DTLS)
, a protocol based on TLS, specifically created to work with datagram protocols like UDP. DTLS essentially provides the same security guarantees as TLS but considers the stateless, unreliable nature of protocols like UDP.
Example of adding DTLS: openssl s_client -connect host:port -dtls1
It keeps its functionality equivalent to the latest state of TLS but adapts them accordingly considering the unique features of UDP. DTLS suffers from PKI overhead and lack of widespread availability and is hence used only where absolutely necessary. If you're interested in Qt toolkits supporting DTLS destined for UDP-based applications you could check out the guide on the official website here.
In conclusion, though SSL and TLS are preferably used with TCP due to compatibility and reliability reasons, they can also be operational with UDP under special circumstances and may involve considerable efforts, potential problems, shortcomings, and resorting to substitute measures like employing DTLS as an alternative.Sure, I am going to dive into the intricate details of how SSL (Secure Sockets Layer) can be integrated with UDP (User Datagram Protocol) by using Datagram Transport Layer Security (DTLS). Particularly, I'll shed some light on successful instances where the implementation has been fruitful and analyze why they worked.
Understanding SSL, DTLS, and UDP
Before we begin, let's understand the core concepts of SSL, DTLS, and UDP:
- SSL is a standard security technology that ensures encrypted links between a web server and a browser, safeguarding all data passed between them. An application protocol independent, it provides secure and private communication over the network.
- UDP, on the other hand, differs from Transmission Control Protocol (TCP) as it doesn't require establishing a connection before data transmission. It allows packet data to be sent without initiating a proper session or providing delivery assurance.
- The role of DTLS, then, becomes crucial — as a protocol, it adds security features of SSL/TLS to datagram transport layer protocols like UDP.
This arrangement enhances secure and reliable communication even on an unreliable and connection-less protocol like UDP. The crux, however, is to implement this complex setup successfully.
Implementing SSL Over UDP Successfully: Notable Case Studies
Considering the complexities involved, industries across the globe have made considerable efforts to successfully blend together all these diverse technologies, in order to attain swift, reliable yet safe information transfer systems. Below, I'll discuss some significant case studies:
1. Google's QUIC protocol:
Google introduced its own transport layer network protocol known as QUIC which uses UDP instead of TCP. Besides improving performance on congested networks, QUIC leverages DTLS for encryption and includes features of HTTP/2, TCP, TLS, and more, making the whole process faster and safer. The success behind QUIC lies in its agility to use multiplexed streams without head-of-line blocking.
Code Snippet:
void Server::Initialize() { QuicConfig config; CryptoHandshakeMessage chlo = GenerateCHLO(); scoped_ptrshlo(config.GenerateSHLO(chlo)); }
2. Cisco's DTLS implementation for VPNs:
Cisco’s AnyConnect VPN solution utilizes SSL and IPsec VPNs with support for Datagram Transport Layer Security - an enhanced version for SSL VPN over UDP. Essentially, DTLS helps to prevent latency issues caused by SSL connections delivered over TCP. Cisco hence successfully lends reliability for real-time applications working over unstable environments without compromising safety.
3. WebRTC framework:
WebRTC incorporates DTLS at its core, utilized for peer-to-peer communication mediums including video/audio communications, file transfers, etc. Adopting DTLS over UDP assures continued real-time data stream despite losing some packets along the way — this prevents delay while maintaining encryption standards high.
Final Thoughts
Implementing Secure Socket Layers over User Datagram Protocol via DTLS can really upraise the game of security along with efficiency. Yes, when used cleverly, SSL can indeed be used with UDP producing reliable, performance-optimized and secure systems. These pioneering case studies, including Google’s QUIC, Cisco’s VPN offerings, and the WebRTC platform, are testimony to the power of combining secure and swift protocols.
All of these victorious applications teach us one common thing - the formative utilization and tweaking of technology to make secure protocols compatible with speed-oriented ones can unlock manifold potentials. Leveraging game-changing inventions and learning from past cases, we can indeed take many strides forward in network technology.SSL (Secure Sockets Layer) is typically used with TCP (Transmission Control Protocol) connections, which are reliable, connection-oriented services. TCP establishes a full connection between the sender and receiver before the actual data communication begins. It ensures that packets arrive in the correct order and detects if any are missing. This reliability feature makes it a perfect fit for transferring sensitive data, like in banking transactions or private conversations. SSL protocol takes advantage of these features to provide end-to-end encryption and secure transmission.
However, UDP (User Datagram Protocol) operates differently from TCP. It's a simpler, connectionless Internet protocol, where there isn't any guarantee that the packets would reach their destination or maintain the order during transit. In an environment like this, implementing SSL can seem impractical, considering its design around TCP’s reliable, connection-oriented service.
But, despite these apparent differences, SSL can be utilized alongside UDP under certain circumstances. The practical implementation of this concept has already been put into action - specifically, in DTLS (Datagram Transport Layer Security). Based on TLS, which succeeded SSL, DTLS allows applications to use secure communication while still using the connectionless nature of UDP. DTLS endeavors to achieve it without introducing significant latencies incurred by streaming protocols like TCP. A major field where the combination of SSL/TLS security and UDP’s fast transfer rate becomes beneficial is real-time communications, including VOIP calls and video conferencing.
So yes, while not directly, but through the use of DTLS, SSL style security can indeed piggyback onto UDP. It’s a wonderful hybrid solution that combines the perks of both worlds. From TCP, we get reliability and from UDP we get speed.
Here is a sample Python code that showcases the usage of SSL with UDP via DTLS:
import ssl import socket # Create a new context with SSL version TLSv1 and use Datagram protocol context = ssl.SSLContext(ssl.PROTOCOL_TLSv1) # Input certificate and key context.load_cert_chain(certfile="mycert.pem", keyfile="mykey.pem") sock = socket.socket(socket.AF_INET, socket.SOCK_DGRAM, 0) sock.connect(('localhost', 6000)) # Instantiate a DataGram Transport Layer Secure Socket secure_sock = context.wrap_socket(sock, do_handshake_on_connect=True) # Now you can send and receive data just as a normal socket secure_sock.send(("Hello SSL").encode())
Further Reading:
- DTLS RFC 4347 provides extensive details about the protocol binding of DTLS.
- OpenSSL: It offers supportive tools and APIs for working with OpenSSL and DTLS.